Introduction to React.js

Rafał Wiliński

Frontend Dev @ Netguru.co
@RafalWilinski


rwilinski.me
What is React?
A JAVASCRIPT LIBRARY FOR BUILDING USER INTERFACES






SOME HISTORY
Created by Jordan Walke @ Facebook at 2011
Inspired by PHP Components
Deployed to production on Facebook.com in 2011
YEAR LATER ON INSTAGRAM.COM
Other websites running on React:
Netflix
Dropbox
Imgur
New york times
YAHOO
AIRBNB
WHY REACT IS SO COOL?
Core Principles
1. Declarative
2. COMPONENT-based
3. learn once, write anywhere
DECLARATIVE
Imperative Pseudocode
Declarative Pseudocode
function addText(text) {
var textNode = document.createElement(text);
document.body.appendChild(textNode);
}
<button onClick="function() {
addText("Im activated");
}>
Click Me!
</button>
state = 'deactivated';
<button onClick="function() {
state = 'activated';
}>
Click Me!
</button>
while(true) {
if (state === "activated") {
<p>I'm Activated</p>
}
}
Component-based
IN HTML
IN REACT
<div>
<h1,2,3,4,5,6>
<form>
<button>
<p>
<a>
etc...
// HTML + Your Own Components!
<InputWithConfirmButton />
<TitleWithSubtitle />
<VideoPlayer />
<ValidatablePasswordInput />
<InputWithConfirmButton />
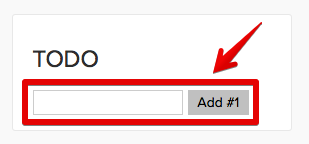
<ValidatablePasswordInput />

Lets CODE
class HelloMessage extends React.Component {
render() {
return <div>Hello {this.props.name}</div>;
}
}
// Usage - mount component in webpage
ReactDOM.render(
<HelloMessage name="foo"/>,
document.getElementById('root')
);
Simplest possible component
What language is that?
IS it javascript?
IS it HTML?
WHAT is "this.props"???
inheritance in JS???
How to display it?
React Uses JSX
1. Syntax extension to JavaScript
2. Created just for React
3. Compiles to JS which is understood by browsers
4. Combines HTML with JS
class HelloMessage extends React.Component {
render() {
return <div>Hello {this.props.name}</div>;
}
}
INPUT
class HelloMessage extends React.Component {
render() {
return React.createElement(
"div",
null,
"Hello ",
this.props.name
);
}
}
COMPILED JS

What language is that?
INHERITANCE IN Javascript?
WHAT IS "THIS.PROPS"?
HOW DO I RENDER IT?
WELCOME TO ES2015/ES6
Classes, inheritance, arrow functions, destructing assignment, enhanced object literals, default + rest + spread, generators, module loaders
and much more...
class HelloMessage extends React.Component {
render() {
return <div>Hello {this.props.name}</div>;
}
}

"use strict";
var _createClass = function () { function defineProperties(target, props) { for (var i = 0; i < props.length; i++) { var descriptor = props[i]; descriptor.enumerable = descriptor.enumerable || false; descriptor.configurable = true; if ("value" in descriptor) descriptor.writable = true; Object.defineProperty(target, descriptor.key, descriptor); } } return function (Constructor, protoProps, staticProps) { if (protoProps) defineProperties(Constructor.prototype, protoProps); if (staticProps) defineProperties(Constructor, staticProps); return Constructor; }; }();
function _classCallCheck(instance, Constructor) { if (!(instance instanceof Constructor)) { throw new TypeError("Cannot call a class as a function"); } }
function _possibleConstructorReturn(self, call) { if (!self) { throw new ReferenceError("this hasn't been initialised - super() hasn't been called"); } return call && (typeof call === "object" || typeof call === "function") ? call : self; }
function _inherits(subClass, superClass) { if (typeof superClass !== "function" && superClass !== null) { throw new TypeError("Super expression must either be null or a function, not " + typeof superClass); } subClass.prototype = Object.create(superClass && superClass.prototype, { constructor: { value: subClass, enumerable: false, writable: true, configurable: true } }); if (superClass) Object.setPrototypeOf ? Object.setPrototypeOf(subClass, superClass) : subClass.__proto__ = superClass; }
var HelloMessage = function (_React$Component) {
_inherits(HelloMessage, _React$Component);
function HelloMessage() {
_classCallCheck(this, HelloMessage);
return _possibleConstructorReturn(this, (HelloMessage.__proto__ || Object.getPrototypeOf(HelloMessage)).apply(this, arguments));
}
_createClass(HelloMessage, [{
key: "render",
value: function render() {
return React.createElement(
"div",
null,
"Hello ",
this.props.name
);
}
}]);
return HelloMessage;
}(React.Component);
What language is that?
INHERITANCE IN Javascript?
WHAT IS "THIS.PROPS"?
HOW DO I RENDER IT?
class HelloMessage extends React.Component {
render() {
return <div>Hello {this.props.name}</div>;
}
}
// Usage - only in JSX! doesn't work in HTML
<HelloMessage name="put something here" />
Props is properties
<div>Hello put something here</div>

What language is that?
INHERITANCE IN Javascript?
WHAT IS "THIS.PROPS"?
HOW DO I RENDER IT?

What language is that?
INHERITANCE IN Javascript?
WHAT IS "THIS.PROPS"?
HOW DO I RENDER IT?
EXERCISE
EXERCISE
1. Changing State of component
import React, { Component } from 'react';
class DeactivatableComponent extends Component {
constructor(props) {
super(props);
// Set initial state of component
this.state = {
isEnabled: true,
};
}
render() {
return (
<div>
<button onClick={() => this.setState({ isEnabled: false })}>
Click me!
</button>
{this.state.isEnabled ? 'Enabled' : 'Disabled'}
</div>
);
}
}
2. Rendering a filtered collection
import React, { Component } from 'react';
import TaskItem from './TaskItem';
class DeactivatableComponent extends Component {
constructor(props) {
super(props);
this.state = {
showOnlyDone: true,
tasks: [{
isDone: true,
text: 'Make homework',
}]
};
}
render() {
return (
<div>
{
this.state.tasks
.filter(task => this.state.showOnlyDone ? tasks.isDone : true)
.map((task) => (
<TaskItem text={task.text} />
))
}
</div>
);
}
}

We're hiring!
Ruby on rails
frontend
iOS & Android
https://github.com/RafalWilinski/todo_list
Boilerplate with tips:
https://facebook.github.io/react/
React docs:
React
By Rafal Wiliński
React
- 563