Welcome to Kotlin
Introduction for Programmers
Rainer Kern 2019

Hello World
-
Statically typed C-like programming language
-
object-oriented and functional hybrid
-
Runs on the JVM with full bidirectional interoperability
-
Runs natively on Android (generates 1.6 Bytecode)
-
Possibility to compile to Javascript using LLVM
-
Kotlin Native (beta) for iOS, MacOs and Windows
-
Open source compiler and tools
YAJL - Yet another JVM Language!
- Jetbrains is well-known and offers good tooling support
- Nothing really new - just the best of all of them
- It’s about the Java ecosystem, not the language
- Feels like Java 2.0 (Java on steroids)
// Kotlin is
(1..6).forEach{ println("very") }
// easy to learn
log -pretty --date=short
2010 Start of Development by JetBrains
2011 Unveiling of Project Kotlin
2012 Open Source under Apache 2
2016 Release of v 1.0 in Feb
2017 Android Native
2019 v. 1.3.52

Kotlin is also an Island

Why Kotlin
-
Syntax similar to JAVA / C#
-
Expressiveness makes more readable.
-
Conciseness makes more understandable.
-
Flexibility - It allows developers to declare the
-
functions at the top level
-
No static declaration (can cause problems to Java devs)
-
Has Coroutines
Basic Syntax
kotlinlang.org/docs/reference
// Java
System.out.println("bar");
// Kotlin
println("bar")
fun main() {
println("Hello World")
}
package com.systemkern.demo
fun main(args: Array<String>) {
val name =
if (args.length > 0) args[0]
else ”Kotlin”
println("Hello $name")
}
// immediate assignment
val a: Int = 1
// 'Int' type is inferred
val b = 2
// ininitialized 'Int'
val c: Int
c = 3
// variable of type int
val one = 1
val million = 1_000_000
// variable of type string
val str = "foo"
//explicit declaration
val d: Double = 13.37
val strList = listOf("foo", "bar")
// 'Int' type is inferred
var x = 5
x += 1
// top level variables
val PI = 3.14
var x = 0
fun main() {
x += 1
}
// traditional usage
var max = a
if (a > b) max = b
// with else
var max: Int =
if (a > b) max = a
else (a > b) max = b
// when replaces the switch operator
when (x) {
1 -> print ("x == 1")
2 -> print ("x == 2")
else -> {
print ("x is neither 1 nor 2")
}
}
// for loops
for (item in collection) {
println(item)
}
for (i in 1..10)
println (i)
for (i in array.indices)
println (array[i])
// while loops (and do..while)
while (x > 0) {
x--
}
do {
val y = retreiveData()
} while (y != null)
Language Features
kotlinlang.org/docs/reference
Nullability

//nullability types checked by the compiler
val sure: String = null // error
val maybe: String? = null
if (maybe == null)
println("nullable is null")
if (maybe != null)
println("nullable is not null")

val nullable: String? = null
nullable.length // compiler error
val l1: Int = nullable!!.length
val l2: Int? = nullable?.length
val l3: Int = nullable?.length ?: -1
// smart casting
var local: String? = null
// maybe = "can be reassigned"
if (local != null)
println("length: " + local.length)
print (local ?: "Elvis operation")
if (ext != null)
println("length: " + ext?.length)
Class Declaration

// single ctor initializing prop
open class MyClazz(
private val member: String
) {
// optional init =^= constructor
// init {
// }
fun alert() {
println("Member is: $member")
}
}
Multiple Classes per File

Extensions

// Javascript like top level functions
fun add(x: Int, y: Int): Int {
return x + y
}
// expression functions
fun add(x: Int, y: Int): Int = x + y
// extension functions
fun Int.third():Int = (this / 3)
val res = 18.third() // 6
// extension properties
val big1 = BigDecimal(100) // this is tedous
val Int.bd.BigDecimal
get() = BigDecimal(this)
val Double.i
get() = this.toInt()
big2 = 100_000.bd
int1 = 13.37.i
// extension properties
// cannot modify the class
// so no backing field
val globStore =
mutableMapOf<Int, String>()
var Int.description: String
get() = globStore[this] ?: ""
set(value) {
globStore[this] = value
}
fun main() {
42.description = """
| The answer to the ultimate
| question of life,
| the universe and everything
""".trimMargin()
println(42.description)
}
// insanely powerful

The Collection API
kotlinlang.org/docs/reference
listOf().firstOrNull() // null
val list = mutableListOf(2,1,null,4,3)
.filterNotNull() // 2,1,4,3
list.first() // 2
list.first { it > 2 } // 4
list.filter { it > 1} // 2,4,3
list.firstOrNull { it > 10 } // null
?: -1 // -1
list.forEach { println(it) }
list.map { "_$it_" } // list of strings
Higher Order Functions
kotlinlang.org/docs/reference
fun higher(
str: String = "",
transform: (String) -> (String)
): String = transform(str)
higher("baz") { "$it postfix"}
// compare to java
higher(baz,(String str) -> {
str + "postfix"
});
More Language Features
kotlinlang.org/docs/reference
//Pojos
public class Pojo {
private String id;
private int amount;
public Pojo (String id, int amount) {
this.id = id;
this.amount = amount;
}
public String getId() { retrun id; }
public void setId(String id) { this.id = id }
public int getAmount() { return amount; }
public void setAmount(int amount) { this.amount = amount; }
}
//Pojos
public class Pojo (
var id: String,
var amount: Int
)
//Pojos "Java Style"
public class Pojo (
var id: String,
var amount: Int
)
val myPojo = Pojo("foo", 100)
myPojo.amount = 50
//Pojos Kotlin Idiomatic
public class Pojo (
val id: String,
val amount: Int
)
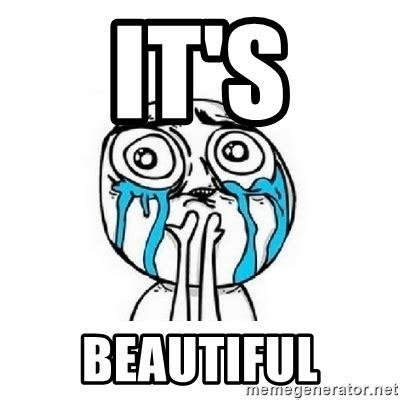
Named & Default Args
kotlinlang.org/docs/reference
fun printGreeting(
name: String = "World",
str: String = "Hello",
t: Int = 1
) {
for (i in 1..t) println("$str $name")
}
printGreeting("Vanessa")
printGreeting("Angie", str = "Hi")
printGreeting(t = 7, name ="Gloria")


Kotlin @ Google IO 2017
http://youtube.com/watch?
Try Kotlin Online
try.kotlinlang.org
Learn Kotlin
kotlinlang.org
Kotlin Vienna User Group
kotlin.wien
Talking Kotlin podcast
talkingkotlin.com
kotlinlang.slack.com
https://surveys.jetbrains.com/s3/

Welcome to Kotlin
By Rainer Kern
Welcome to Kotlin
Introduction to the Kotlin programming languages. Its design concepts and features. Target Audience: Programmers with no Kotlin experience
- 1,425