Jumpstart
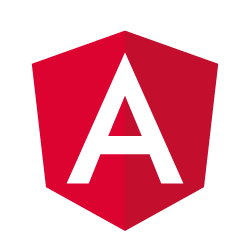
+
Elements
+
Agenda
- JumpStart Overview
- WK-Elements Overview
- Integration with Angular
- AngularJS to Angular migration strategies
- Current status
The basics
We need to start with the required polyfills wk-elements depends upon.
/************************************************************
* BROWSER POLYFILLS
*/
import 'wk-elements/bundles/polyfills';
Module configuration
CUSTOM_ELEMENTS_SCHEMA needs to be included inside the @NgModule to tell Angular we will be using custom html tags inside the template.
import { CUSTOM_ELEMENTS_SCHEMA, NgModule } from '@angular/core';
import { HomeComponent } from './home.component';
@NgModule({
declarations: [ HomeComponent ],
exports: [ HomeComponent ],
schemas: [ CUSTOM_ELEMENTS_SCHEMA ]
})
export class HomeModule { }
Usage inside an NG component
Now you are able to import the elements you wish and use them inside your HTML template.
import { Component } from '@angular/core';
// Import the wk-element you want to use
import 'wk-elements/wkTooltip';
@Component({
selector: 'app-home',
template: './home.component.html',
styleUrls: ['./home.component.scss']
})
export class HomeComponent {}
// Now you are able to consume wk-tooltip inside your html template
<div class="app-home">
<h1 class="haz-a-tooltip">Home Component</h1>
<wk-tooltip target=".haz-a-tooltip" title="This heading has a tooltip, hooray!"></wk-tooltip>
</div>
That
WAS
EASY!
A more complex integration example
Sometimes you will want to wrap a wk-element inside an angular component to take full advantage of it and provide customized & already configured versions of a particular element.
Let's see an example with the wk-modal element.
import 'wk-elements/wkModal';
import { ChangeDetectionStrategy, Component, ElementRef, Input, OnInit, ViewChild } from '@angular/core';
export type ModalSize = 'large' | 'medium' | 'small';
export interface IModalWrapperOptions {
title: string;
size: ModalSize;
buttonText: string;
icon: string;
}
@Component({
selector: 'wk-modal-wrapper',
templateUrl: './modalWrapper.component.html',
styleUrls: ['./modalWrapper.component.scss'],
changeDetection: ChangeDetectionStrategy.OnPush
})
export class ModalWrapperComponent implements OnInit {
modalBody: HTMLElement;
@Input() options: IModalWrapperOptions;
@ViewChild('modalContent') content: ElementRef;
@ViewChild('modal') modal: ElementRef;
ngOnInit(): void {
this.modalBody = this.content.nativeElement;
this.modal.nativeElement.actions = [{
label: 'Close',
primary: true,
onclick: () => this.modal.nativeElement.hide()
}];
}
open(): void {
this.modal.nativeElement.show();
}
}
<div>
<a class="modal-link" (click)="open()">
<span [attr.wk-icon]="options.icon"></span>
<span class="modal-button-text">{{options.buttonText}}</span>
</a>
<wk-modal #modal [title]="options.title" [size]="options.size" closable overlay [body]="modalBody"></wk-modal>
<div #modalContent class="content-example">
<ng-content></ng-content>
</div>
</div>
Wrapping an element inside A Component
// inside your component files:
modalOptions: IModalWrapperOptions = {
title: 'My modal title',
size: 'medium',
buttonText: 'Open modal',
icon: 'info'
};
// inside your components templates
<wk-modal-wrapper [options]="modalOptions">
<h2>The modal content</h2>
<p>Will now have a default action 'close' with a button.</p>
</wk-modal-wrapper>
Caveats
There are no silver bullets
Passing attributes
Make sure you bind attributes in wk-elements using the bracket syntax.
Order is important
For some wk-elements it is important the order they have inside your templates. This is due to the way angular parses them.
<wk-tooltip
[attr.title]="title"
[attr.target]="target">
</wk-tooltip>
<button #with-tooltip>
Show tooltip
</button>
// Tooltip must be declared
// after the target declaration
<wk-tooltip
[attr.title]="title"
[attr.target]="target">
</wk-tooltip>
Thank You!
wk-elements + angular
By Ricardo Casares
wk-elements + angular
- 821