Angular.js
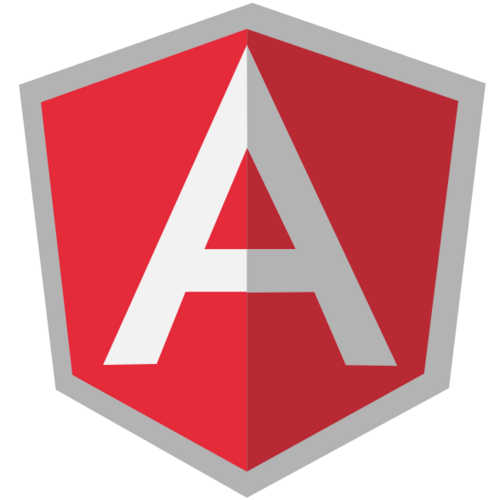
Backed by Google
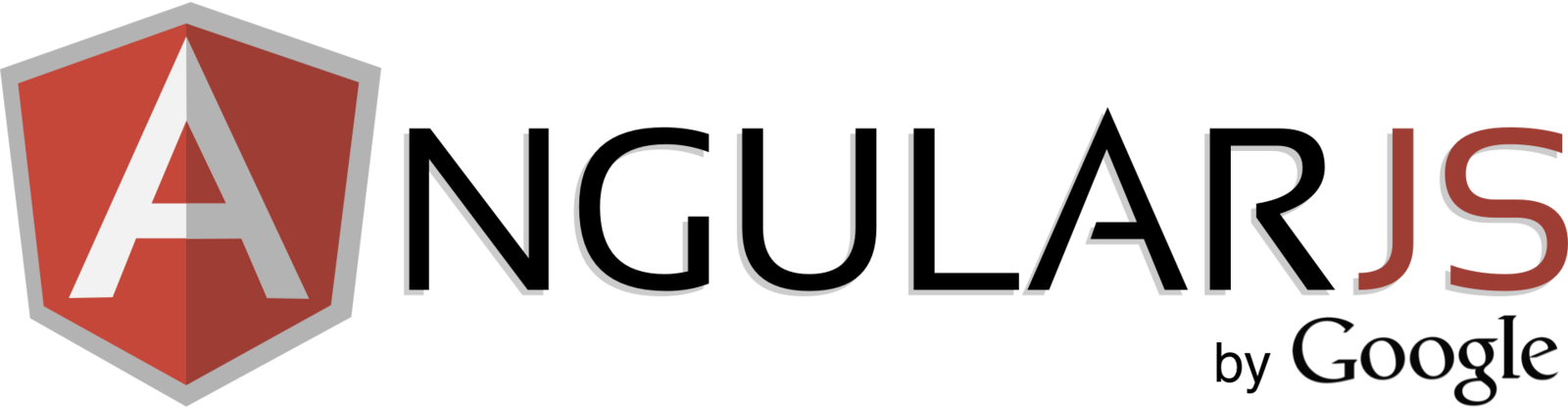
- Web Components
- Extending HTML beyond markup
- Modular Code
MVW Framework
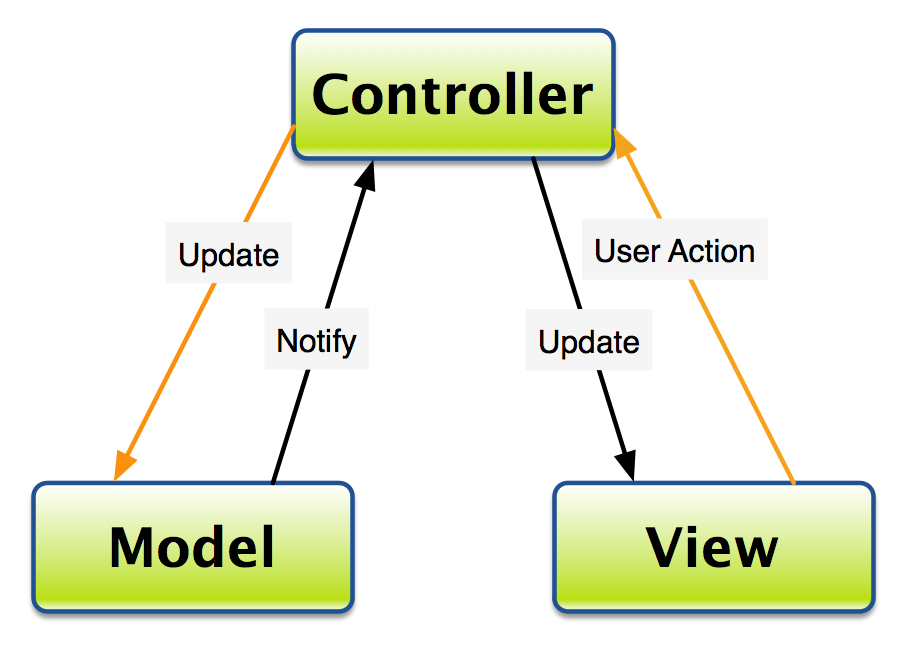
Model, View, Whatever works for you
Two Way Data Binding
- Changes to Model update the DOM
- Changes to DOM update the Model
- jQuery manually manipulates the DOM
- Angular.js automatically updates the DOM
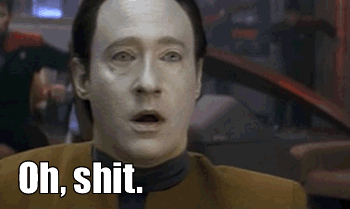
Hello World
<!DOCTYPE html>
<head>
<meta charset="utf-8">
<title>Angular</title>
<style>
body {
padding-top: 50px;
padding-bottom: 20px;
}
</style>
</head>
<body ng-app="myapp">
<div >
<input type="text" name="name" value="" ng-model="title">
<h2>Hello {{title}} !</h2>
</div>
<!-- Angular.js CDN -->
<script type="text/javascript" src="https://cdnjs.cloudflare.com/ajax/libs/angular.js/1.3.14/angular.min.js"></script>
<!-- Init JS -->
<script>
angular.module("myapp", []);
</script>
</body>
</html>
Controller
<!DOCTYPE html>
<head>
<meta charset="utf-8">
<title>Angular</title>
</head>
<body ng-app="myapp">
<div ng-controller="helloCtrl" >
<input type="text" name="name" value="" ng-model="title">
<h2>Hello {{title}} !</h2>
</div>
<!-- Angular.js CDN -->
<script type="text/javascript" src="https://cdnjs.cloudflare.com/ajax/libs/angular.js/1.3.14/angular.min.js"></script>
<!-- Init JS -->
<script>
angular.module("myapp", [])
.controller('helloCtrl',['$scope', function($scope){
$scope.title = "World";
}]);
</script>
</body>
</html>
Model
<!DOCTYPE html>
<head>
<meta charset="utf-8">
<title>Angular</title>
</head>
<body ng-app="myapp">
<div ng-controller="helloCtrl" >
<input type="text" name="name" value="" ng-model="greeting.title">
<h2>Hello {{greeting.title}} !</h2>
<pre>{{greeting | json}}</pre>
</div>
<!-- Angular.js CDN -->
<script type="text/javascript" src="https://cdnjs.cloudflare.com/ajax/libs/angular.js/1.3.14/angular.min.js"></script>
<!-- Init JS -->
<script>
var app = angular.module("myapp", []);
// Model Generator
app.factory( 'dataService', function (){
var get = function(){
return {
title: "World"
};
};
return {
get: get
};
});
// Controller
app.controller('helloCtrl',['$scope', 'dataService', function($scope, dataService){
$scope.greeting = dataService.get();
}]);
</script>
</body>
</html>
ng-repeat
<!DOCTYPE html>
<head>
<meta charset="utf-8">
<title>Angular</title>
<style>
.red {
color: #b54040;
}
.blue {
color: #3e43c5;
}
</style>
</head>
<body ng-app="myapp">
<div ng-controller="itemsCtrl" >
<h2>Super Sweet List of Stuff</h2>
<ul>
<li ng-repeat="item in items" ng-class="item.class">{{item.title}} - {{item.value}}</li>
</ul>
</div>
<!-- Angular.js CDN -->
<script type="text/javascript" src="https://cdnjs.cloudflare.com/ajax/libs/angular.js/1.3.14/angular.min.js"></script>
<!-- Init JS -->
<script>
var app = angular.module("myapp", []);
// Model Generator
app.factory( 'dataService', function (){
var get = function(){
return [
{
title: "Item One",
value: "a Val",
class: "blue"
},{
title: "Item Two",
value: "another Val",
class: "red"
},{
title: "Item Three",
value: "a better Val",
class: "red"
},{
title: "Item Four",
value: "the best Val",
class: "blue"
}
]
};
return {
get: get
};
});
// Controller
app.controller('itemsCtrl',['$scope', 'dataService', function($scope, dataService){
$scope.items = dataService.get();
}]);
</script>
</body>
</html>
Angular.js
By Riley Hilliard
Angular.js
- 3,252