javascript
first steps
Introduction
Client Side Programming Language
Dynamic and Interprated
Uses:
Dynamic Web Pages
Rich UI
Asynchronous Communication with server
Function Programming, OOP, Event Driven Programming, Aspect Driven Programming
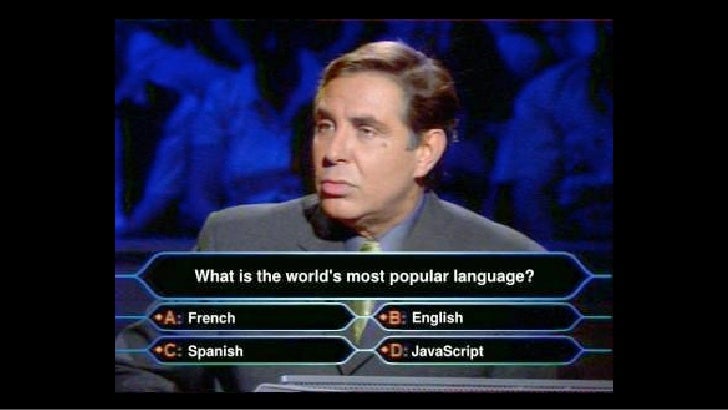
Core JS: Variables
Number: var i = 0;
String: var text = "hello !";
Array: var my_array = [1, 2, 3, 4, 5];
Boolean: var state = true;
Object: var user = { 'name' : 'Nepasa', 'age' : 25 }
Operations
var a = 3, b = 7;
var c = 3 + 7;
var str = 'hello', name = 'world';
var result = str + name;
var list = [2, 4, 6, 8];
var first = list[0];
var user = { 'name' : 'Nepasa', 'age' : 25 }
user.name
functions
var name = 'ramji';
function greet(name){
var text = 'Hello' + name;
console.log(text);
}
var days = ['Sun', 'Mon', 'Tue', 'Wed', 'Thu', 'Fri', 'Sat']
function today(){
var date = new Date();
var today_date = date.getDay();
return days[today_date];
}
control structures
var i = 20, foo = true;
if( i == 10) { .. }
else { .. }
for ( var k =0, k < 10 , k++){ .. }
switch i {
case 1:
..
break;
case 2:
..
break;
default:
}
TImers
Delay the execution of arbitrary instructions
setTimeout()
Calls a function or executes a code snippet after specified delay.
Calls a function or executes a code snippet after specified delay.
setInterval()
Calls a function or executes a code snippet repeatedly, with a fixed time delay between each call to that function.
Calls a function or executes a code snippet repeatedly, with a fixed time delay between each call to that function.
clearInterval()
Cancels repeated action which was set up using setInterval().
Cancels repeated action which was set up using setInterval().
clearTimeout()
Clears the delay set by setTimeout().
Clears the delay set by setTimeout().
var timeoutID; function delayedAlert() { timeoutID = window.setTimeout(slowAlert, 2000); } function slowAlert() { alert("That was really slow!"); } function clearAlert() { window.clearTimeout(timeoutID); }
var nIntervId; function changeColor() { nIntervId = setInterval(flashText, 500); } function flashText() { var oElem = document.getElementById("my_box"); oElem.style.color = oElem.style.color == "red" ? "blue" : "red"; } function stopTextColor() { clearInterval(nIntervId); }
JS OOP: Classes
JS don't have specifically class keyword.
function Person(first_name, last_name) {
this.first_name = first_name;
this.last_name = last_name;
this.full_name = this.first_name + this.last_name;
console.log(this.full_name + ' is instantiated.');
}
var dean = new Person('Mark', 'Conner');
Private - Public
function Person(first_name, last_name) { var balance = 0.0;
// balance is private and cannot be accessed from outside addBalance = function () {
balance += 10;
};
// addBalance is private and cannot be accessed from outside this.first_name = first_name;
this.last_name = last_name;
this.full_name = this.first_name + this.last_name;
// first_name, last_name, full_name are public variables console.log(this.full_name + ' is instantiated.'); this.viewBalance = function(){ alert(balance); }
// viewBalance is public
}
Literal Version
// Literal version
var myObject = {
iAm : 'an object',
whatAmI : function(){
alert('I am ' + this.iAm);
}
}
DOM
The Document Object Model (DOM) is an application programming interface (API) for valid HTML and well-formed XML documents.
It defines the logical structure of documents and the way a document is accessed and manipulated.
DOM Interaction
Getting Elements:
var header = document.getElementById('..');
var el = document.getElementsByTagName('..');
var main = document.getElementsByClassName('..');
Manipulation:
header.style.color = 'red';
header.innerHTML= 'I have Changed';
header.onclick = function() { ... }
RISE OF Javascript MVC
The problem with this is that it doesn’t take long to get lost in a nested pile of jQuery callbacks and DOM elements without any real structure in place for our applications.
In short, we’re stuck with spaghetti code.
Fortunately there are modern JavaScript frameworks that can assist with bringing structure and organization to our projects, improving how easily maintainable they are in the long-run.
BACKBONE.JS
EMBER.JS
KNOCKOUT.JS
ANGULAR.JS
THE END
javascript first steps
By roxxypoxxy
javascript first steps
- 407