Code This, Not That
Ryan Hayes
Tri
7/14/15
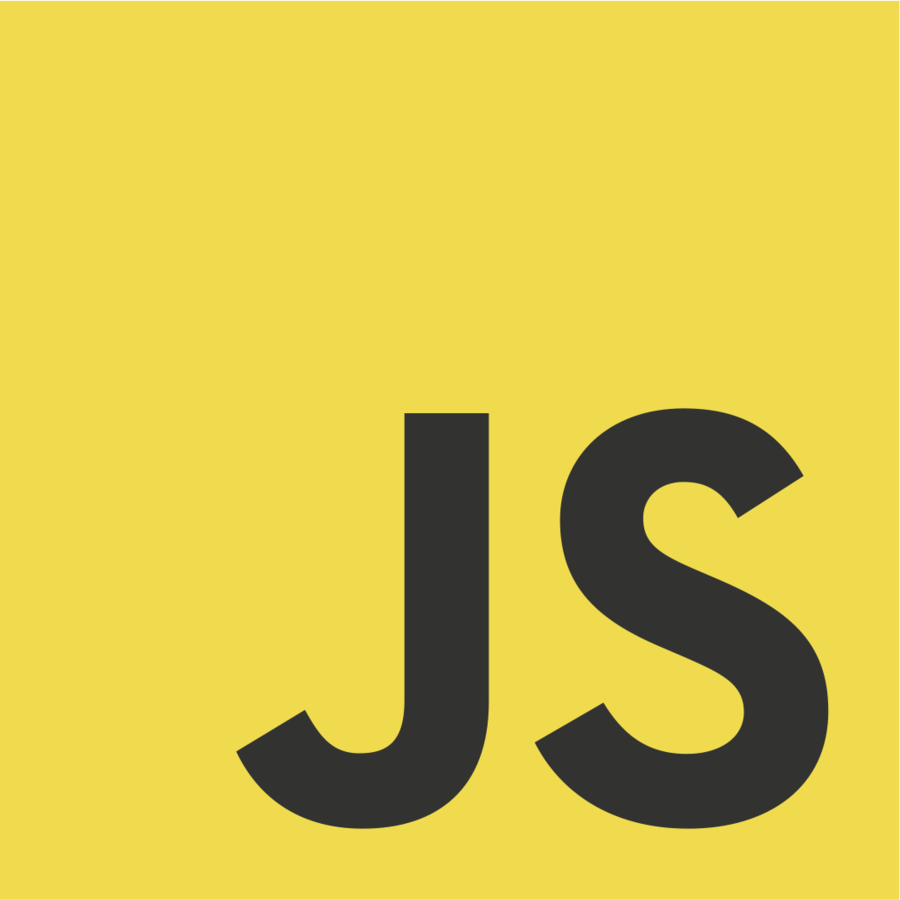
Maintainability
Performance
Maintainability
Performance
== VS ===
var x = 2;
var y = 1 + 1;
x == y; // true
x === y; // true
x == 20; // false
x === 20; // false
x == "something"; //false
x === "something"; //false
Both of these check equality
Similar,
But
Not The
Same



JavaScript "Truthiness"
(and "Falsiness")
truth·i·ness
ˈtro͞oTHēnis/
noun
informal
-
the quality of seeming or being felt to be true, even if not necessarily true.
JavaScript "Truthiness"
(For ===)

JavaScript "Truthiness"
(For ==)

Speaking of comparisons...
Check If a Variable is Undefined
Don't do this:
var myVariable = false;
if (myVariable === undefined)
{
// do something
}
why?
var myVariable = false;
undefined = false;
if (myVariable === undefined)
{
// Executes!
}
Check If a Variable is Undefined
Do this:
var myVariable = false;
if (typeof myVariable === 'undefined')
{
// Doesn't execute, since typeof is 'object'
}
Fast Facts (be careful):
- typeof (NaN) return 'number'
- typeof (null) return 'object'
Use the Module Pattern
/* A module that keeps things tidy! */
(function () {
// ... all vars and functions are in this scope only
// still maintains access to all globals
}());
Add some parenthesis to keep it tidy...
Use the Module Pattern
/* A module that relies on jQuery! */
(function ($, Ember) {
// now have access to globals jQuery (as $) and Ember in this code
}(jQuery, Ember));
... now explicitly allow dependencies...
Use the Module Pattern
/* A module that others can use as the name */
var RYANSLIBRARY = (function () {
var tempLib = {};
var somethingPrivate = 1;
function privateMethod() {
// ...
}
tempLib.someAwesomeProperty = 1;
tempLib.someMethodYouCanCall = function () {
return "Hey oh!";
};
return tempLib;
}());
RYANSLIBRARY.someMethodYouCanCall(); // Returns "Hey oh!"
...now you're a modulin' master! Spread the love!
Documentation
Use JSDoc
No, Really -> http://usejsdoc.org/
/**
* Represents a book.
* @constructor
* @param {string} title - The title of the book.
* @param {string} author - The author of the book.
*/
function Book(title, author) {
}
Performance
Maintainability
Use VanillaJS Where Possible and Batch DOM Updates
- document.getElementById('elementId') is FAST
- React is fast because VirtualDOM avoids updates
- Re-use selector results
Reduce Amount of Code
- Remove unused code
- Use Minifiers/bundlers
Cache
- Cache CSS, JS, Images that aren't updated
- Cache AJAX results (IE Caches by default)
Upgrade Libraries
- Use a package manager (Bower, NuGet, JSPM, NPM)
Learn To Fish!
- Know your F12 tools
- Know your Fiddler
- http://www.webpagetest.org
Code This, Not That
By Ryan Hayes
Code This, Not That
- 4,576