We take developers from good to great

WE ARE THE JSLEAGUE

Intro to React
16-17th March

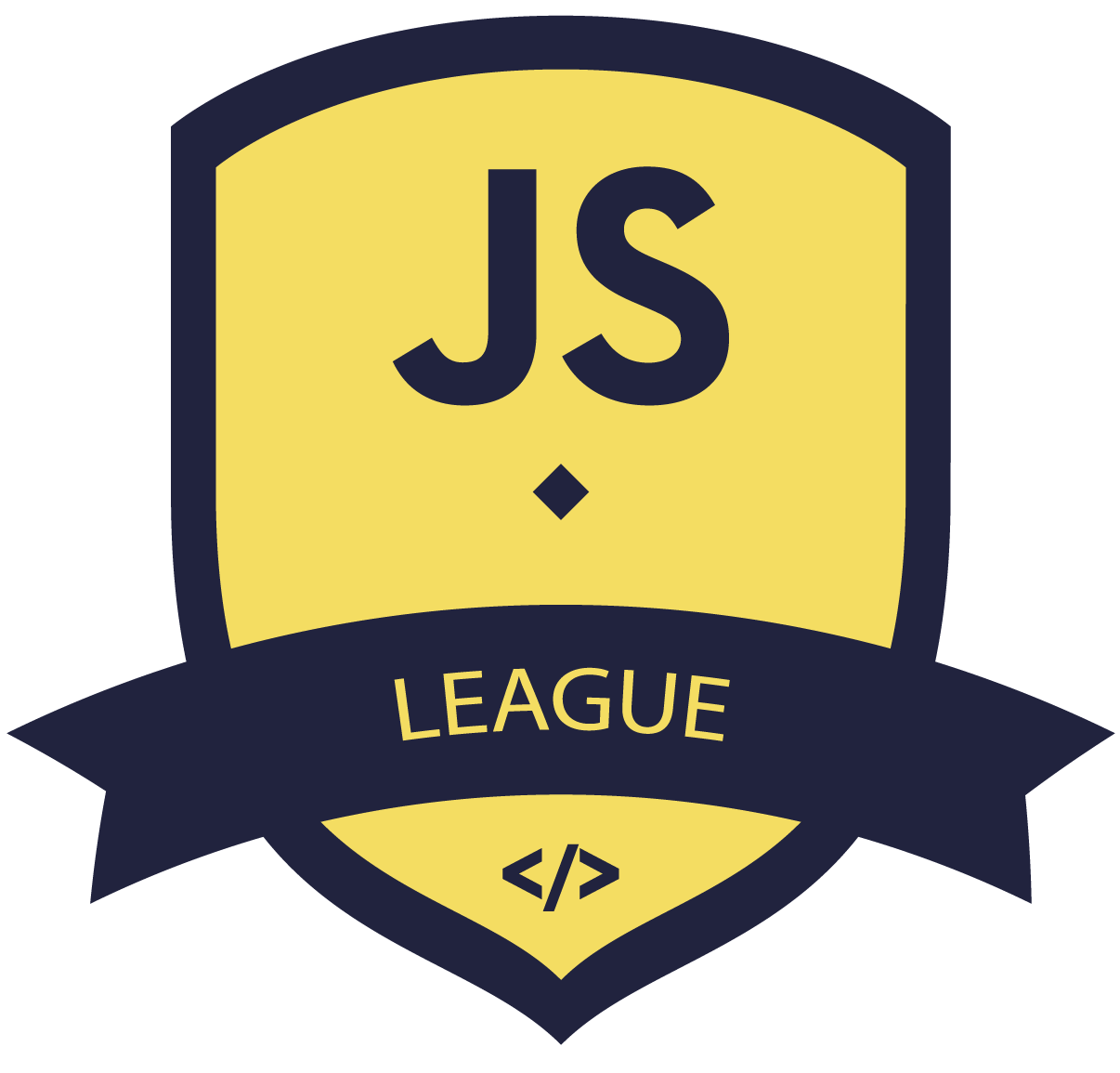
Whoami
Sabin Marcu
Developer at R/GA
Been working in web since '09
React Dev since 2015

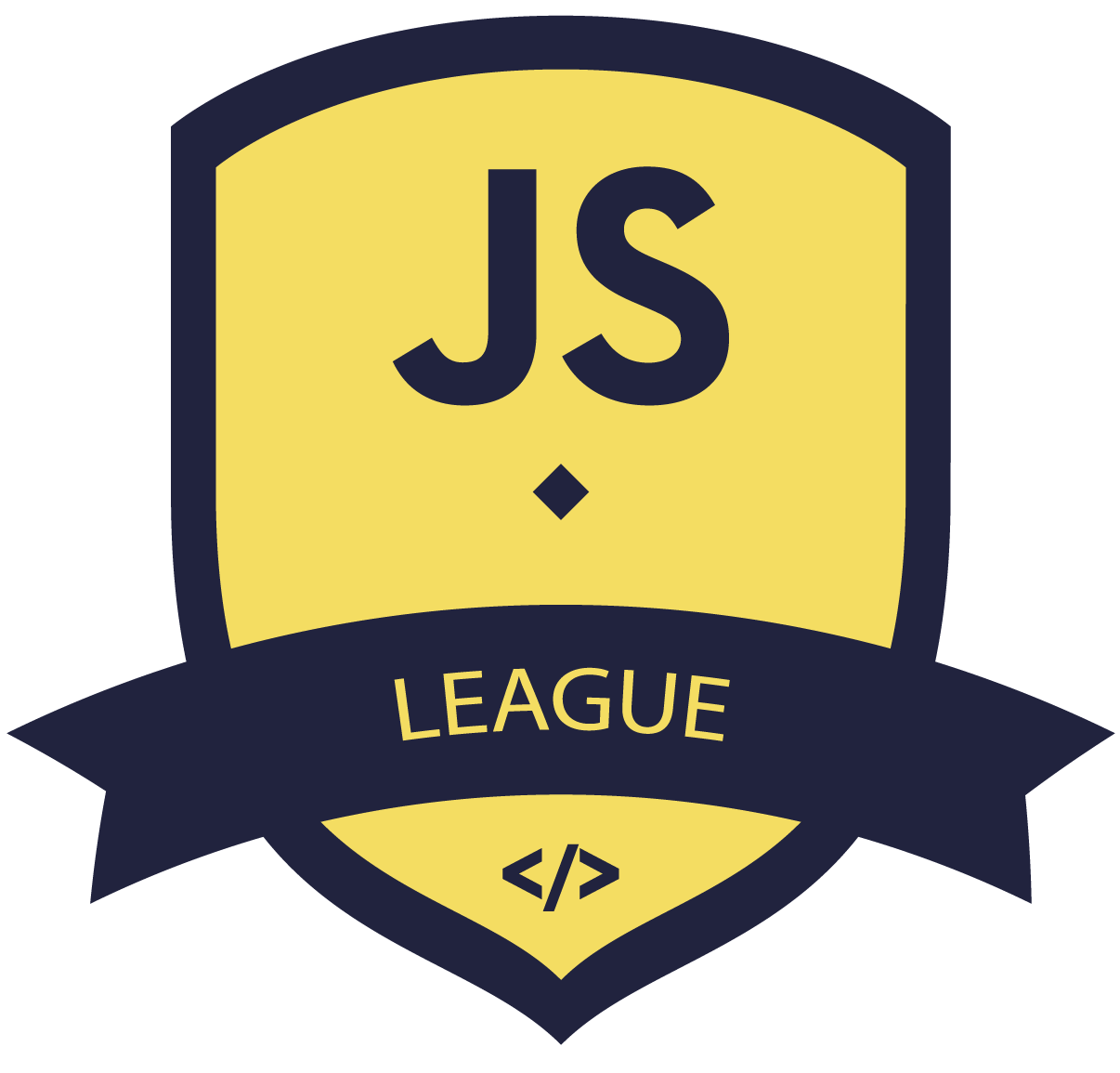
Assistants


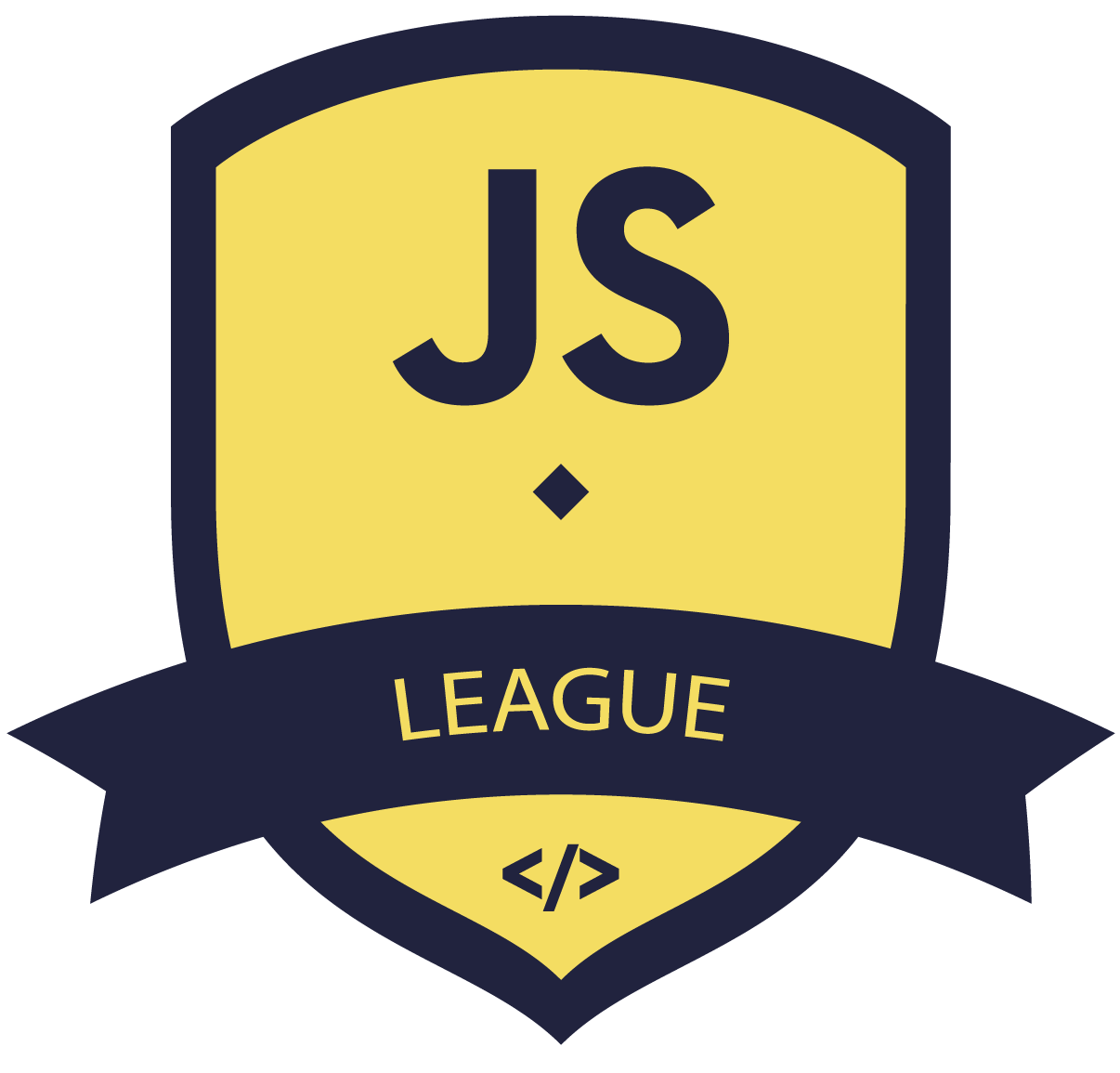
Assistants


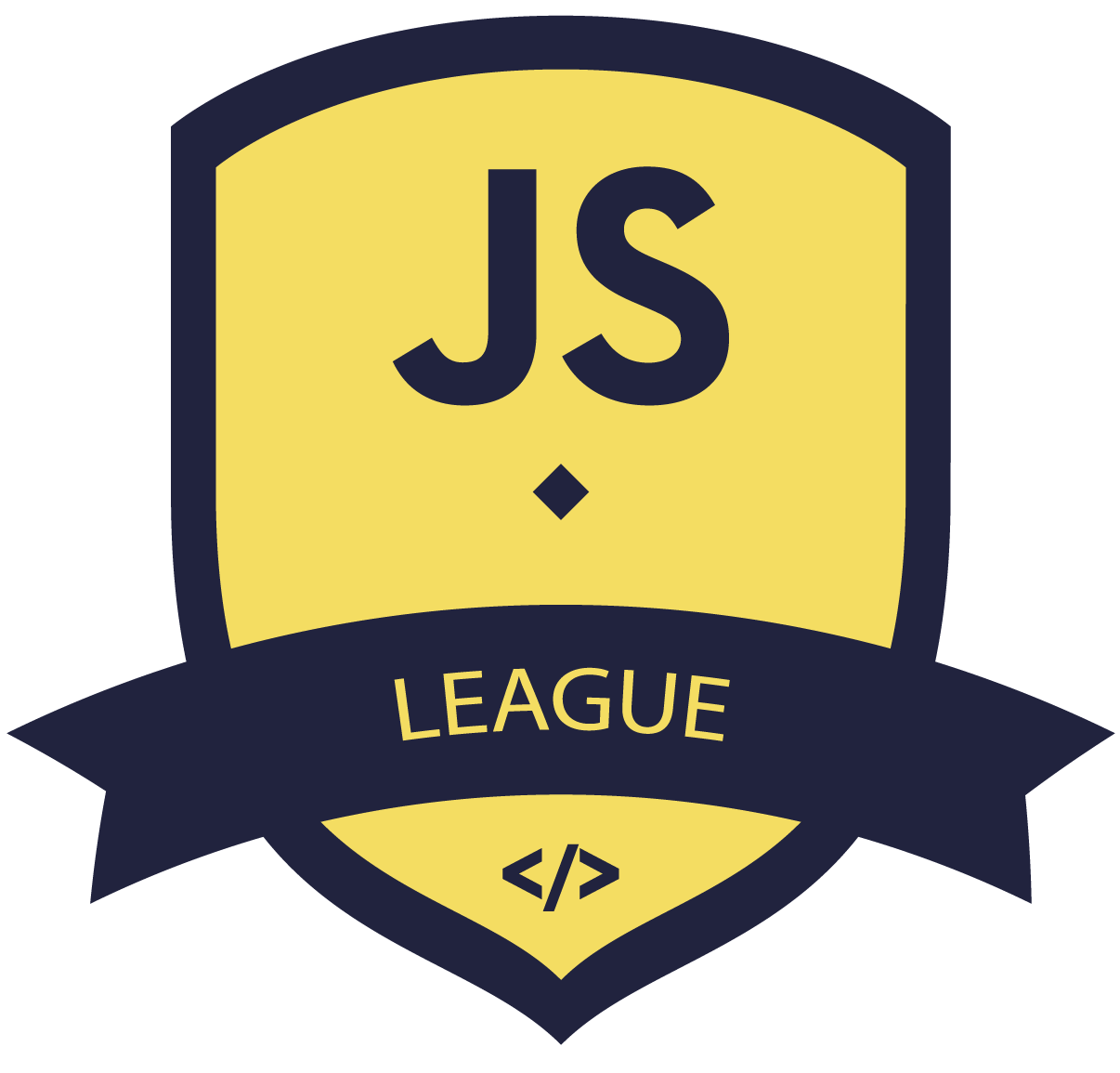
Assistants


We expect cooperation from all participants to help ensure a safe environment for everybody.
We treat everyone with respect, we refrain from using offensive language and imagery, and we encourage to report any derogatory or offensive behavior to a member of the JSLeague community.
We provide a fantastic environment for everyone to learn and share skills regardless of gender, gender identity and expression, age, sexual orientation, disability, physical appearance, body size, race, ethnicity, religion (or lack thereof), or technology choices.
We value your attendance and your participation in the JSLeague community and expect everyone to accord to the community Code of Conduct at all JSLeague workshops and other events.
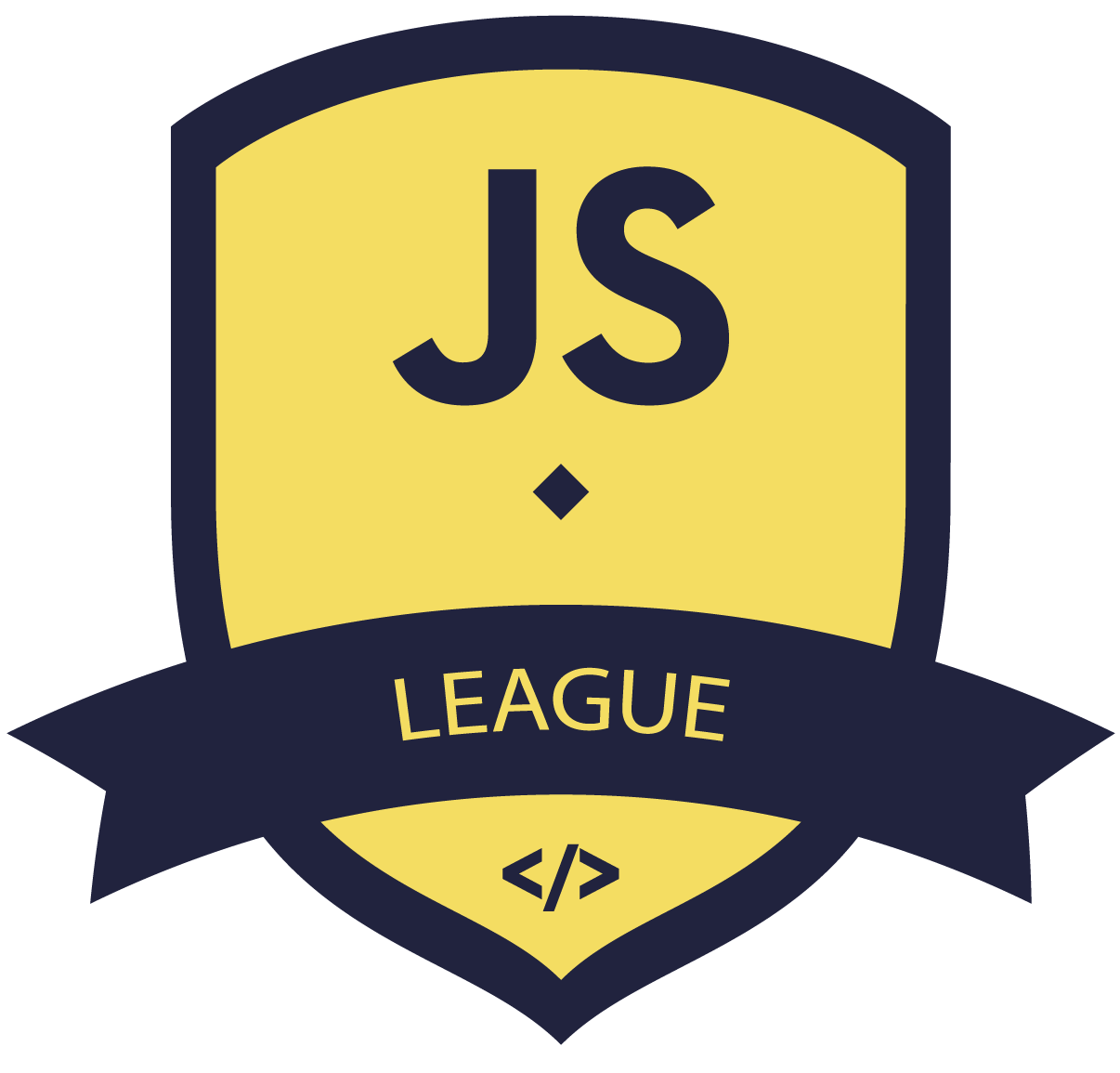
Code of conduct

2x 15' coffee break + 1h lunch break
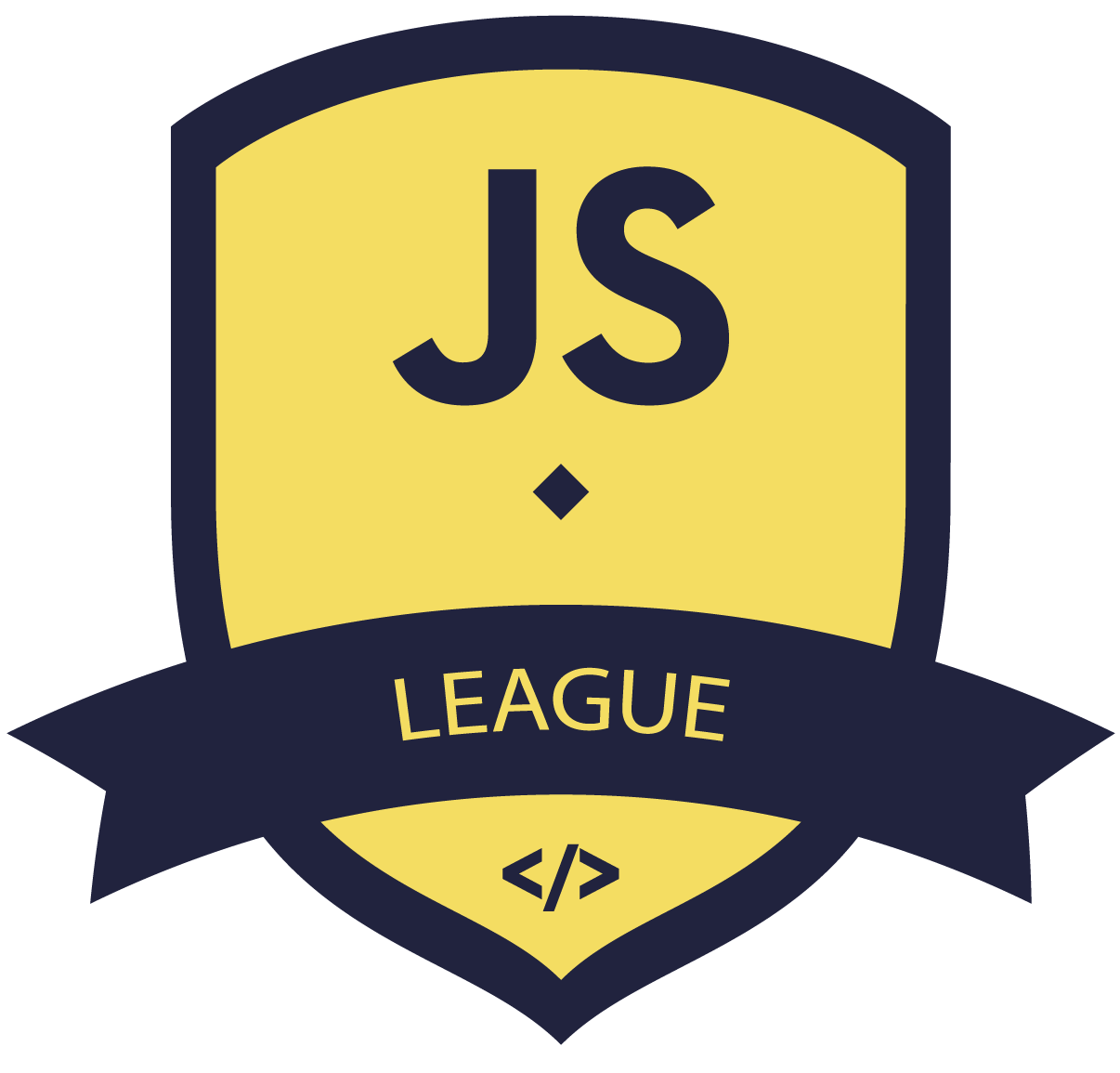
Basic ES6
Module 1
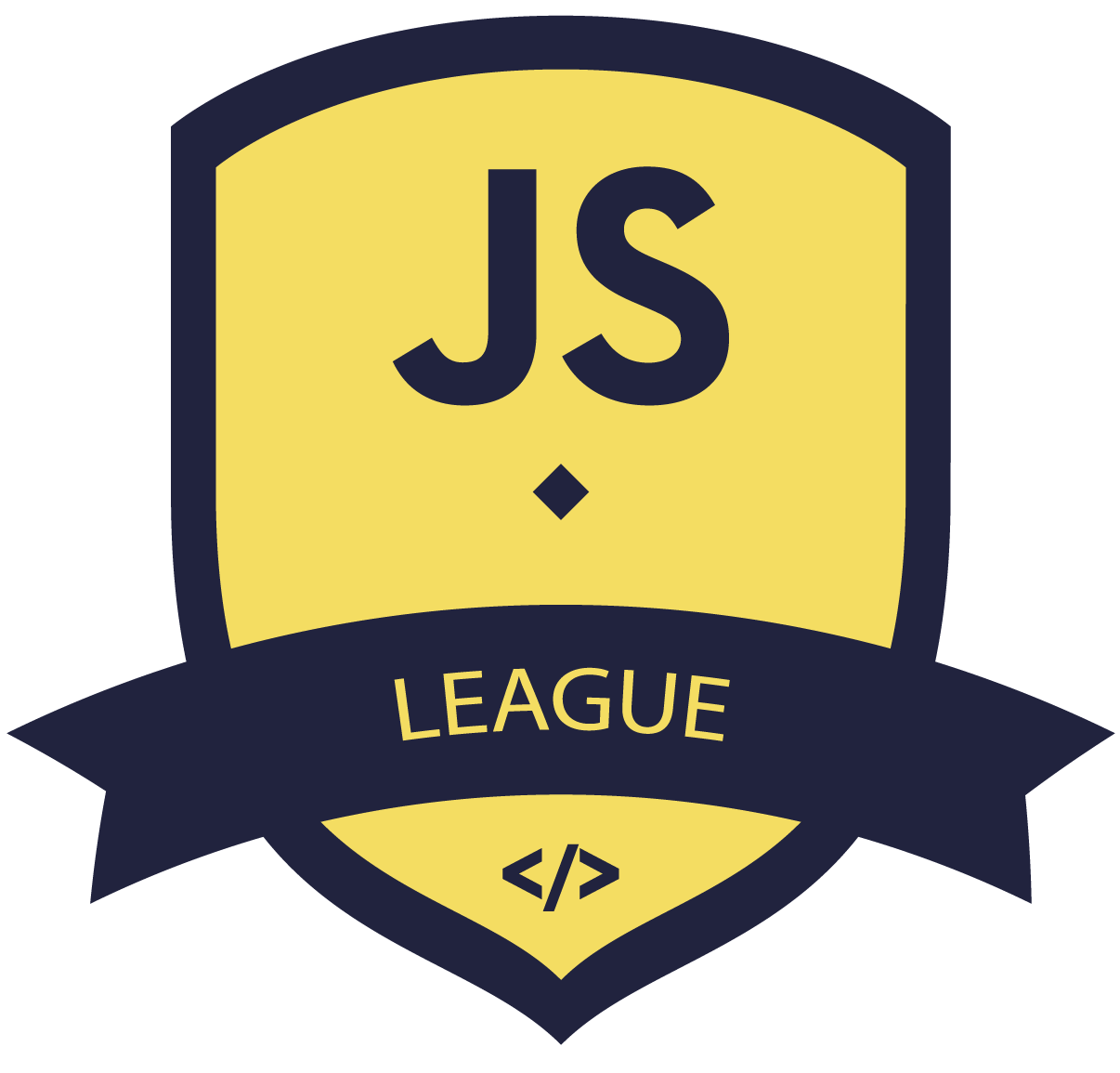
Module 1
Lambda (arrow) Functions
// Classical
function myNamedFunction(a, b) {
return a + b;
}
function myNamedExtractor(x) {
return x.myProperty;
}
// Lambda Functions
const myNamedFunction = (a, b) => a + b;
const myNamedExtractor = x => x.myProperty;
// Or, with destructuring (more on that later)
const myNamedExtractor = ({ myProperty }) => myProperty;
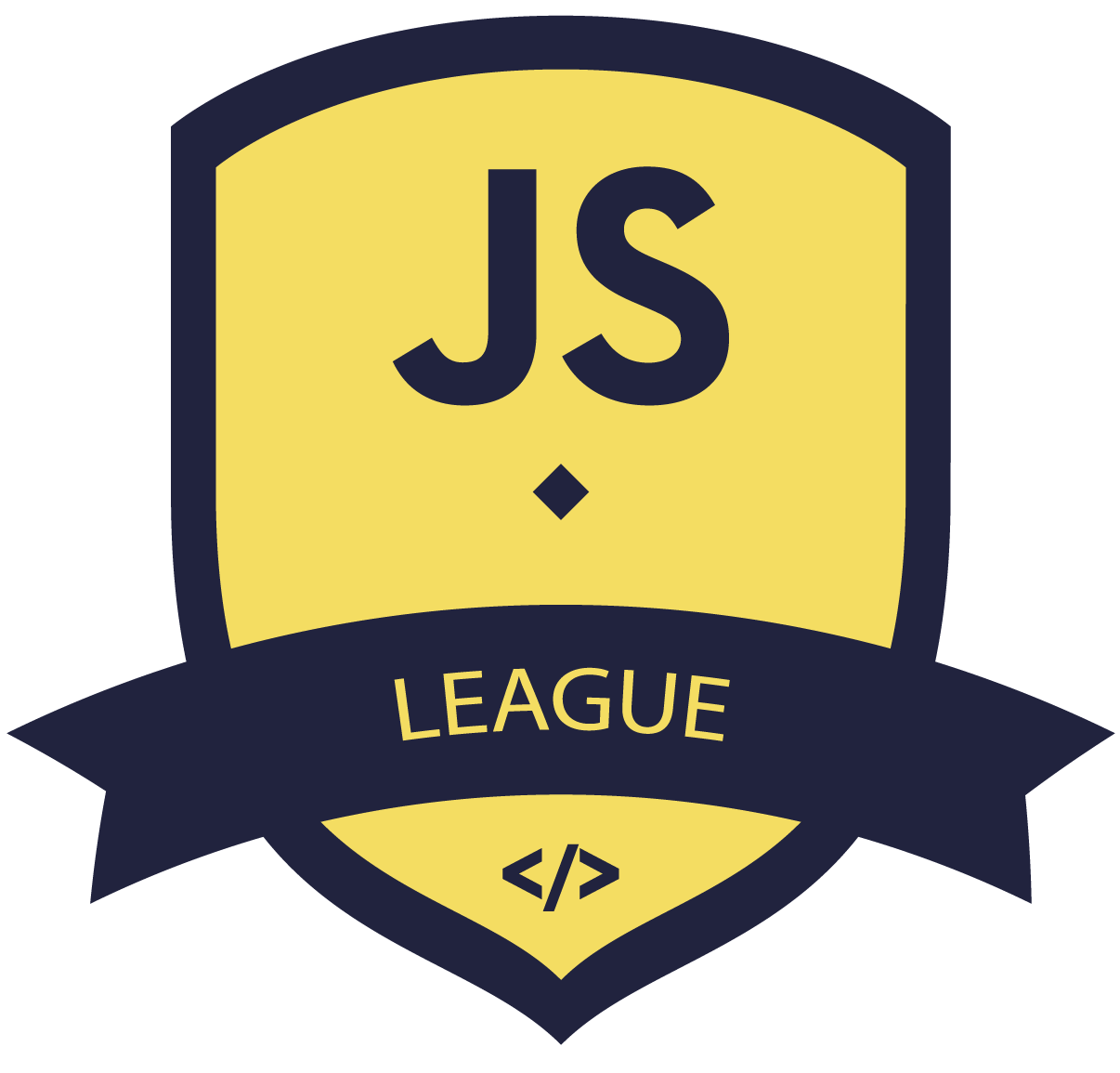
Module 1
Destructuring
// Given
const x = { root: 5, foo: { bar: { baz: 25, baaz: 30 } }, a: { b: { c } } };
// Classical
function myCalculator(param) {
return param.root + param.foo.bar.baz +
param.foo.bar.baaz + param.a.b.c;
}
// With destructuring and lambda functions
const myCalculator = ({
root,
foo: { bar: { baz, baaz } },
a: { b: { c } }
}) => root + baz + baaz + c;
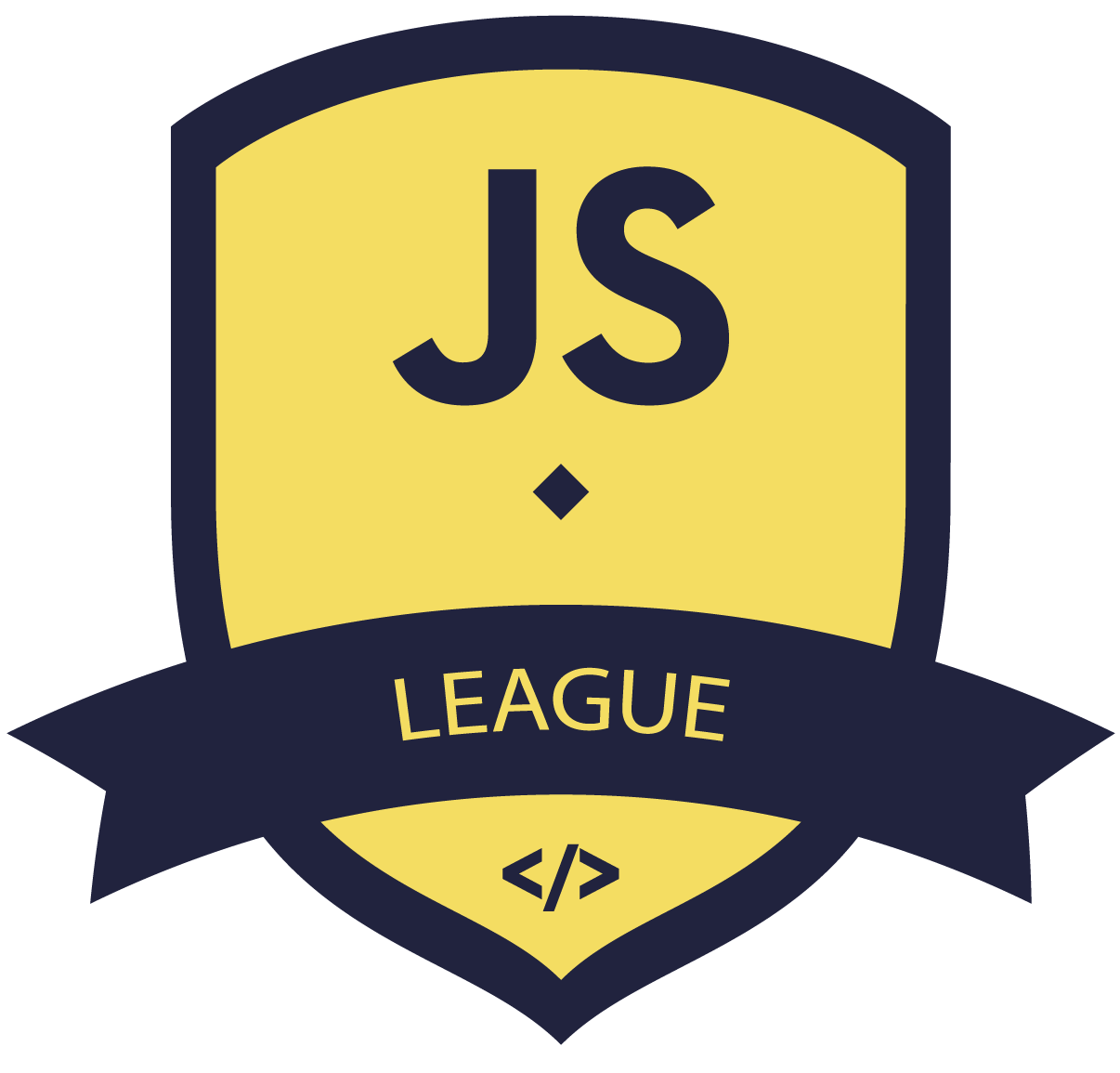
Module 1
Template Strings
// Given
var x = "Hello";
var y = "World";
var z = "!";
// Classical
console.log(x + " " + y + z);
// Template Strings
console.log(`${x} ${y}${z}`);
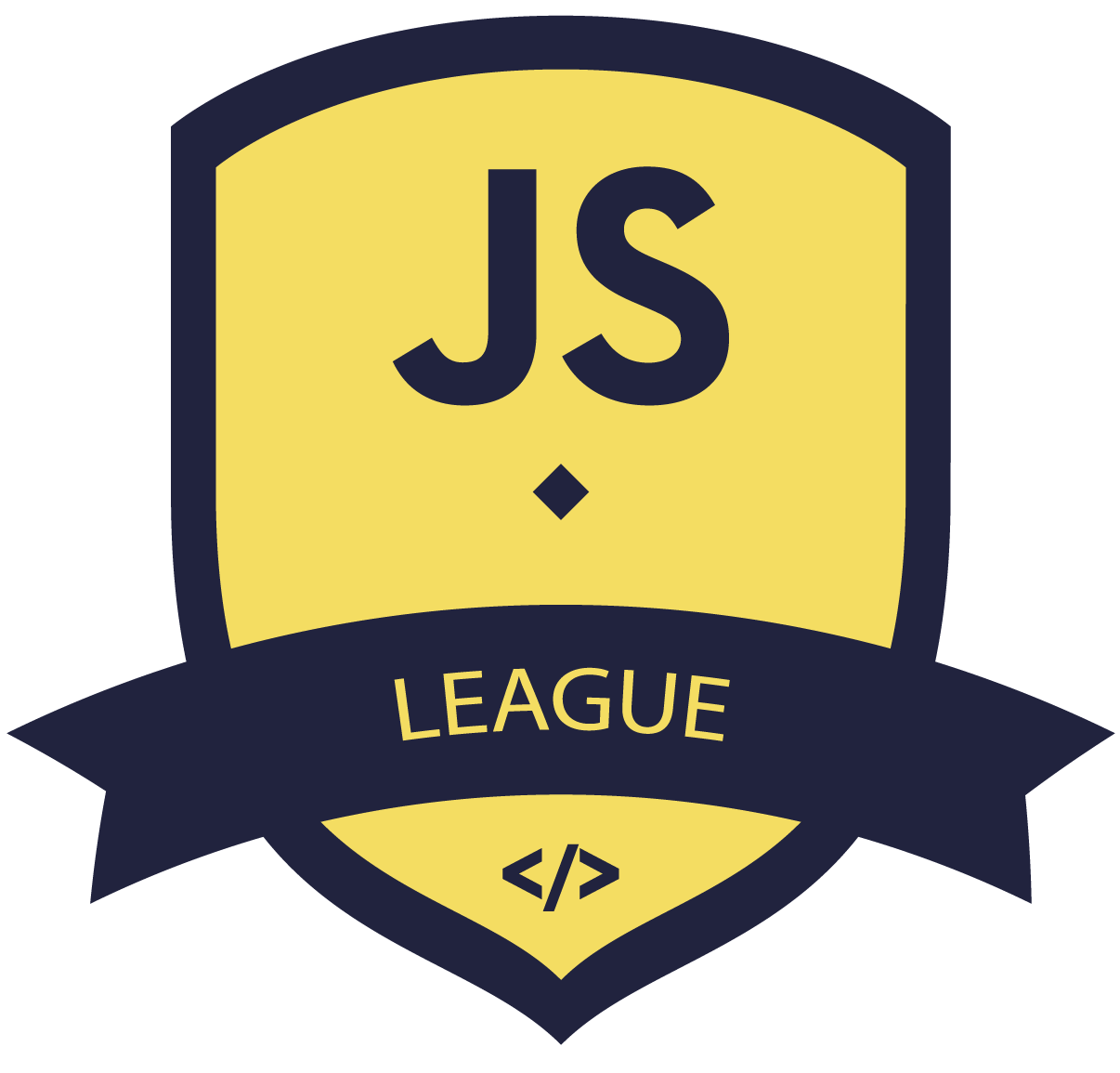
Module 1
Lambda Functions Autobind
// Classical Way
{
...
getValue: function() {
return 5;
},
myFunction: function(a) {
let self = this;
setTimeout(function() { alert(self.getValue() + a); }, 500 );
},
}
// Lambda Functions
{
...
getValue = () => 5,
myFunction = a => setTimeout(() => alert(this.getValue() + a), 500),
}
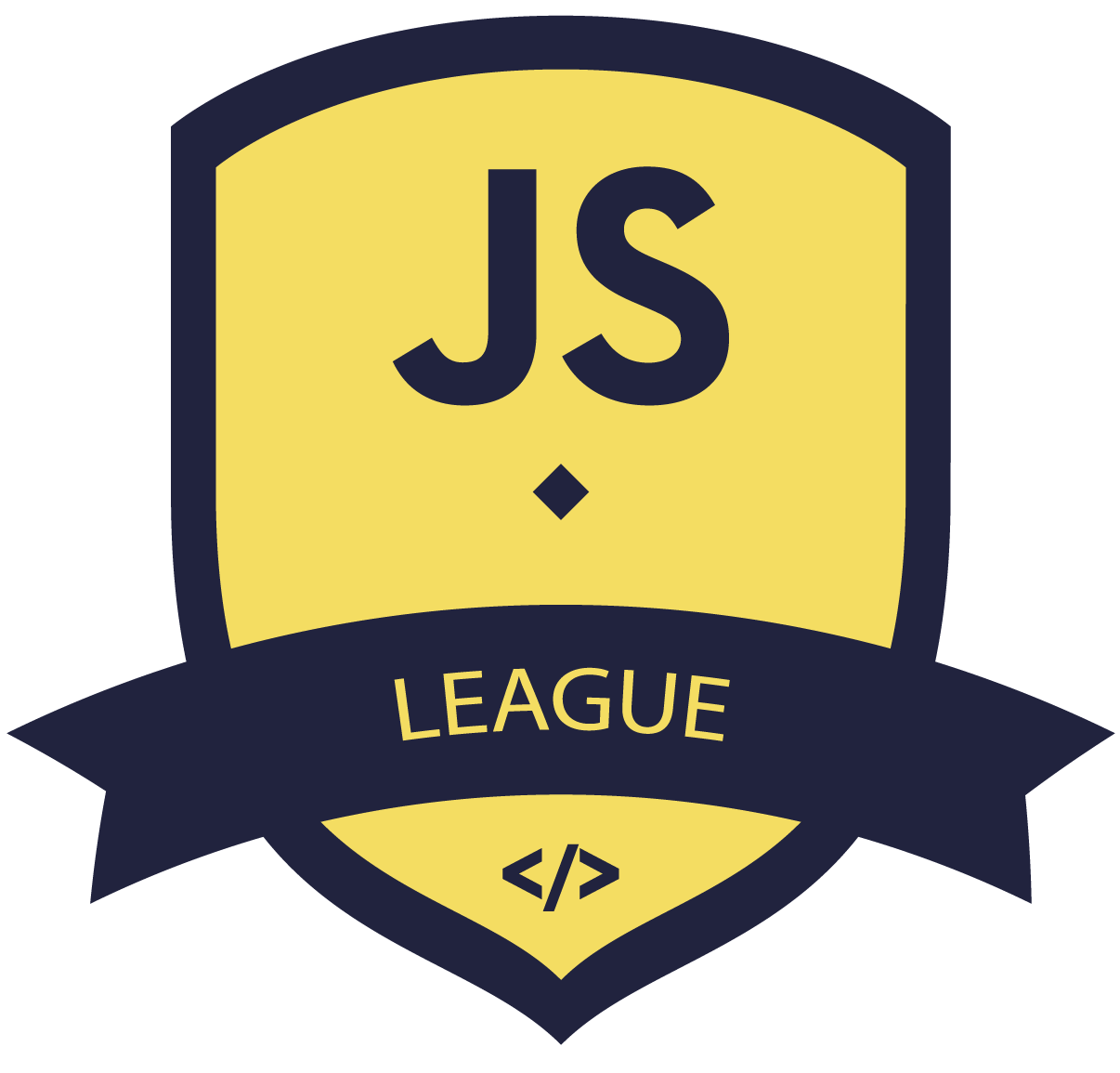
React
Module 2
Fundamentals
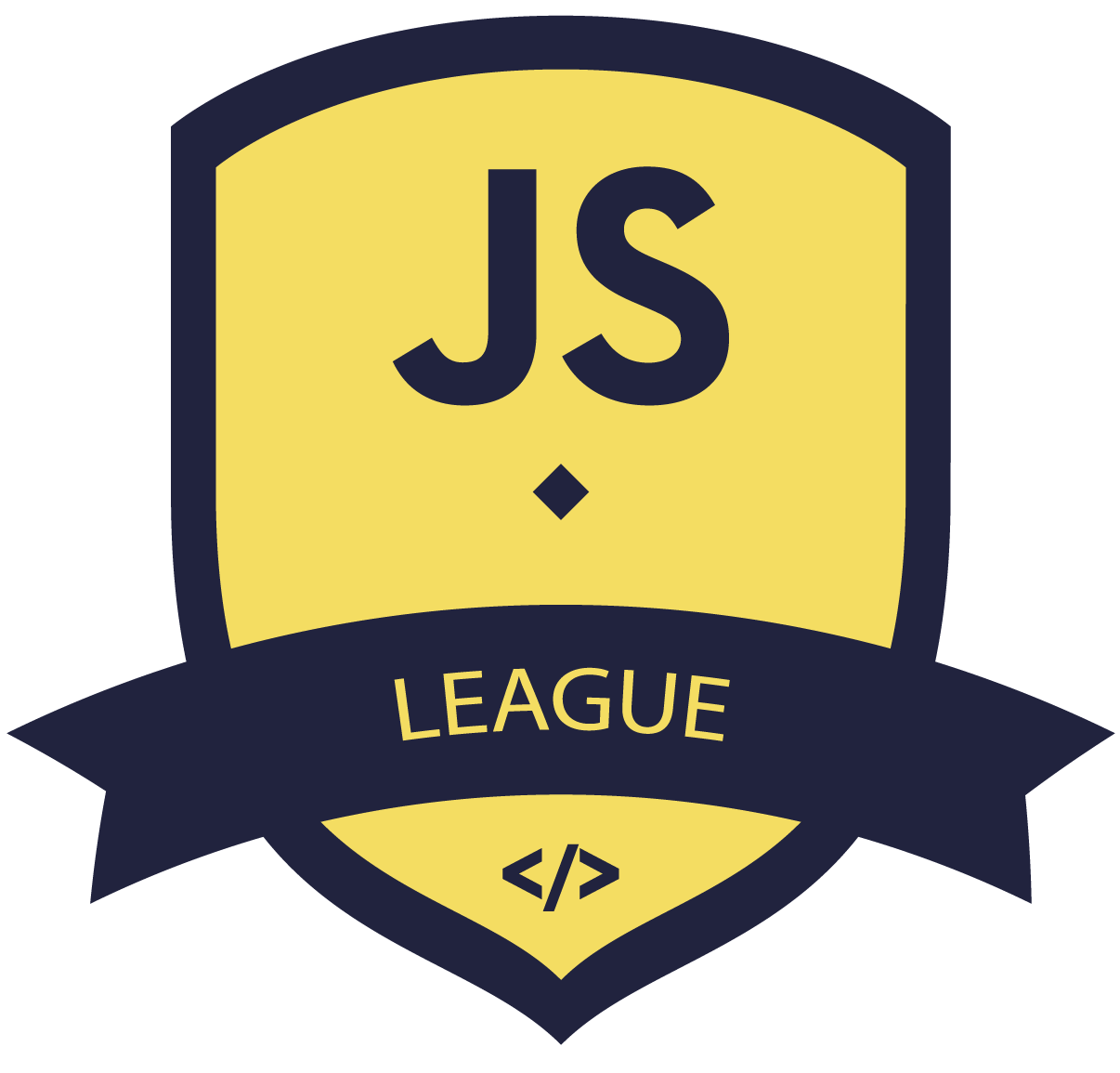
Module 2
But first, a few considerations...
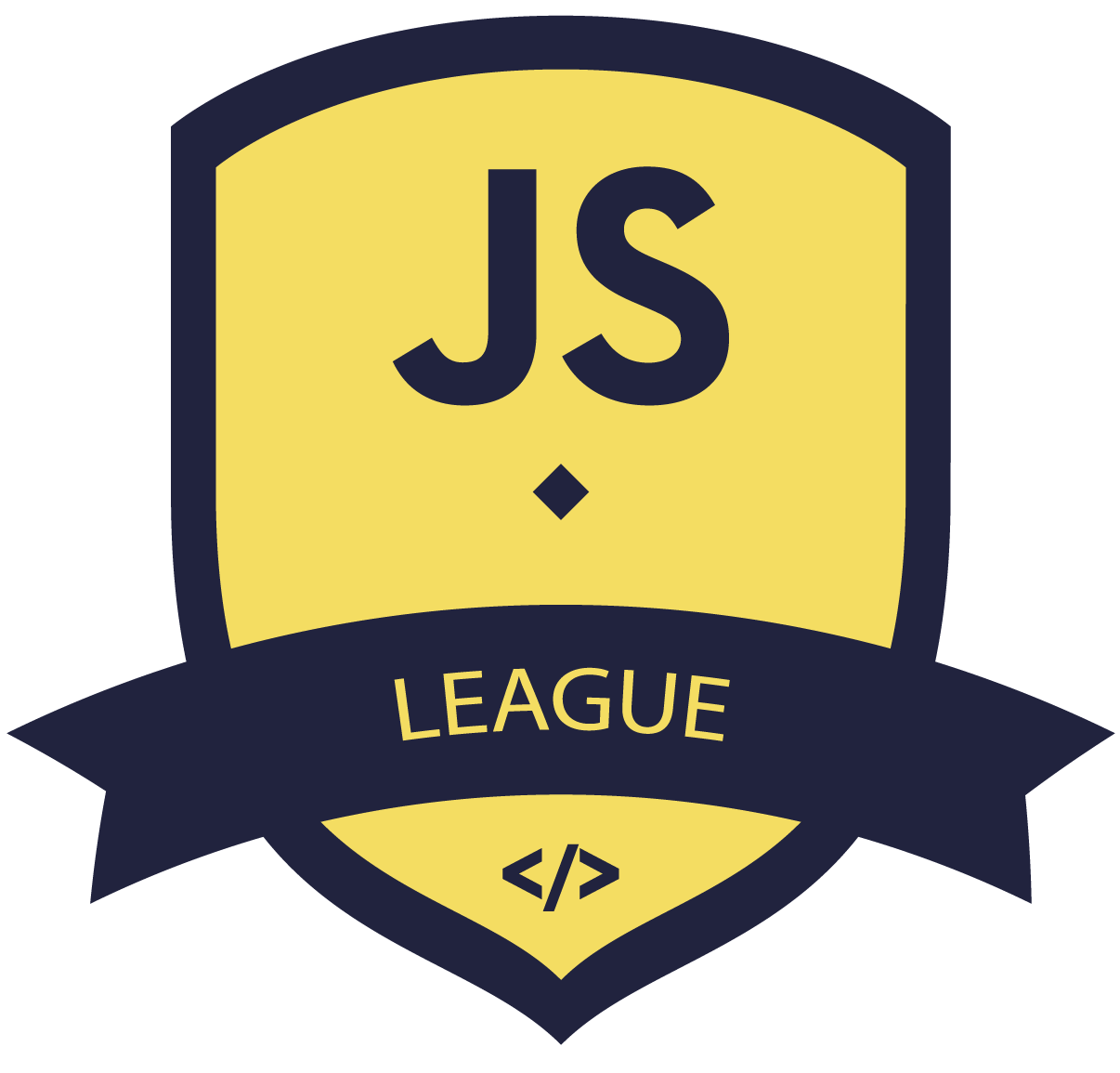
Module 2
Templating and state management


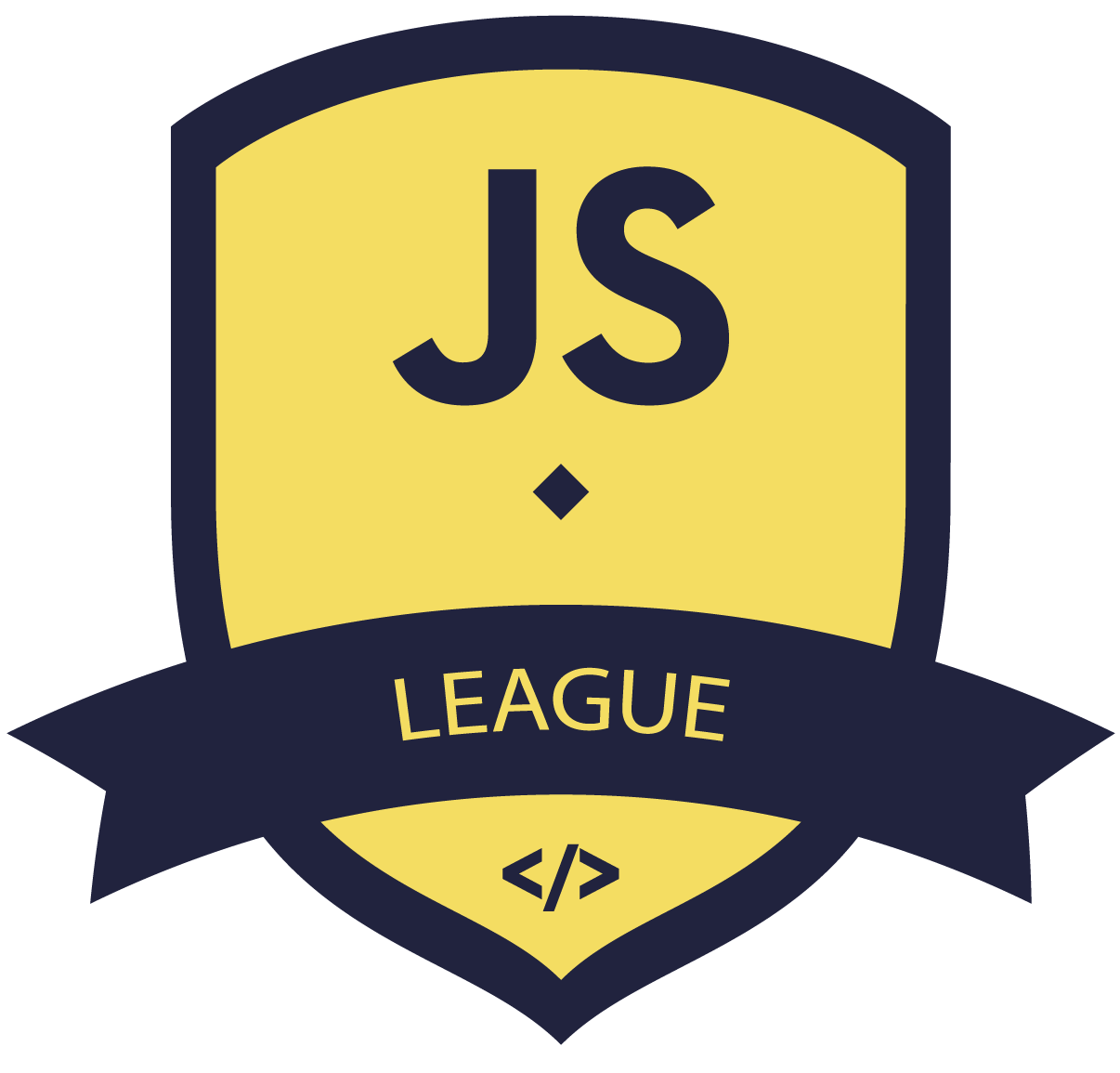
Module 2
Templating and state management

Cannot stress this enough.
Everything is JS
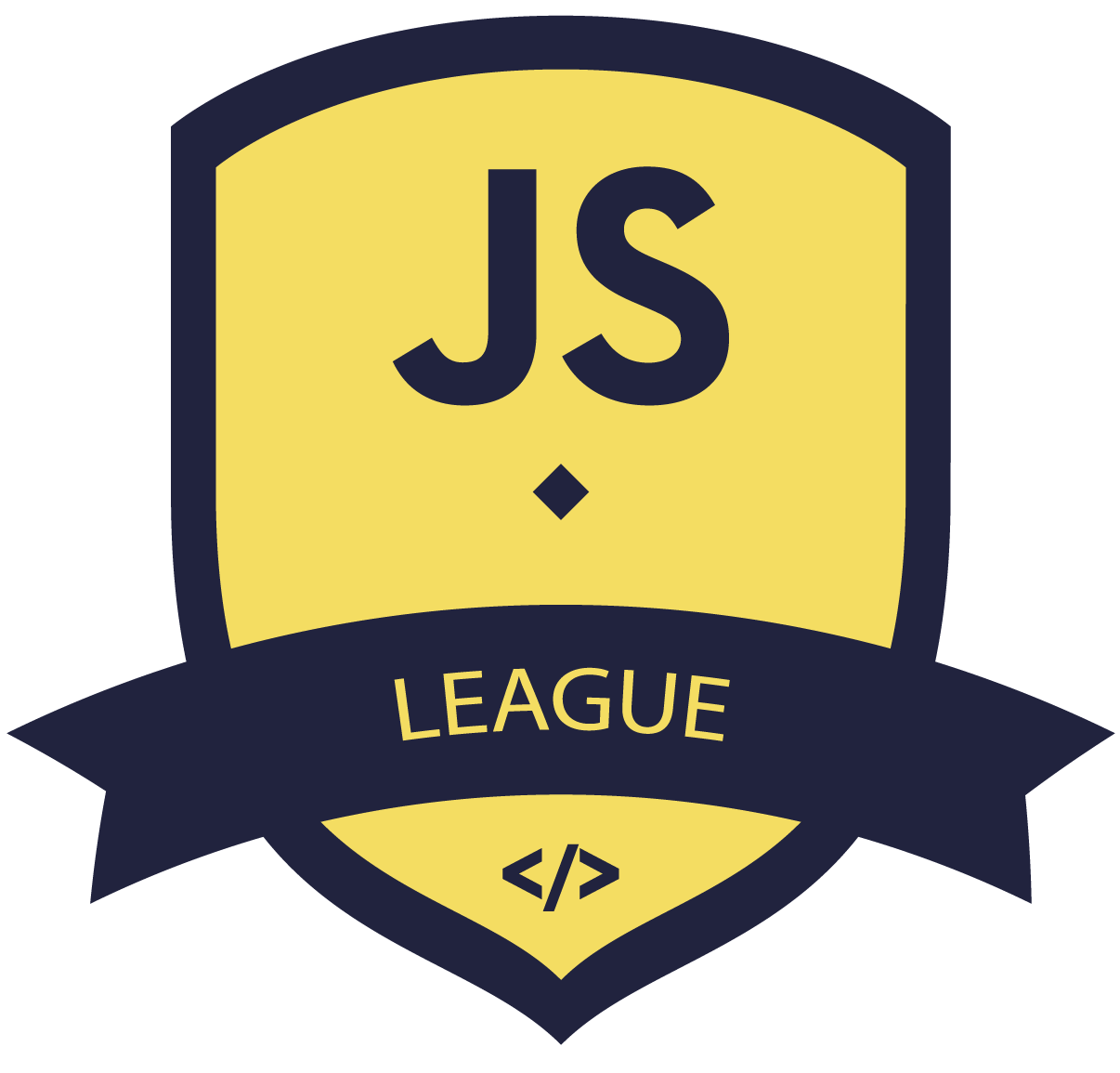
Module 2
First stop: Components
Two types of components:
- "Dumb Components"
- "Stateful Components"
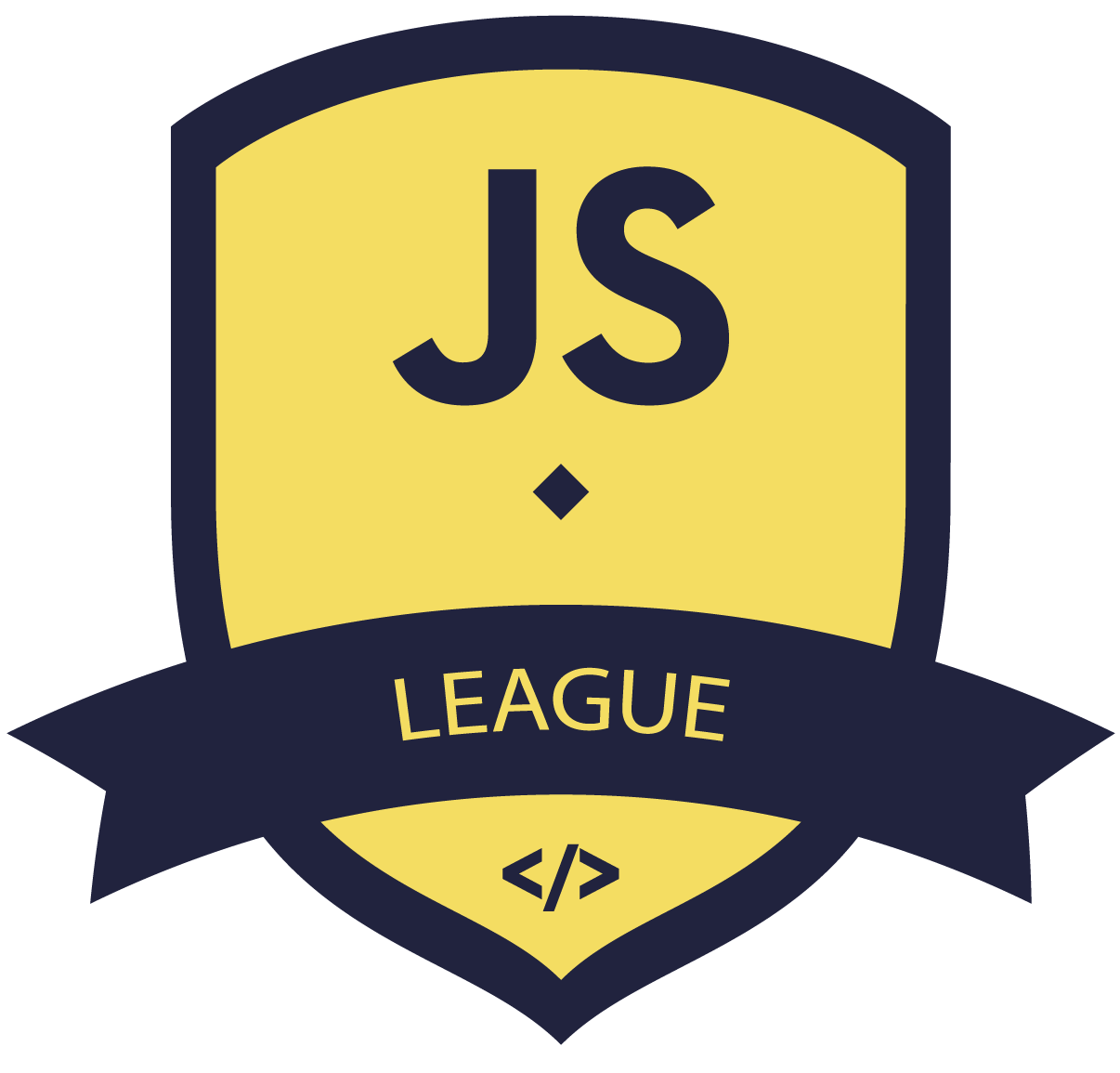
Module 2
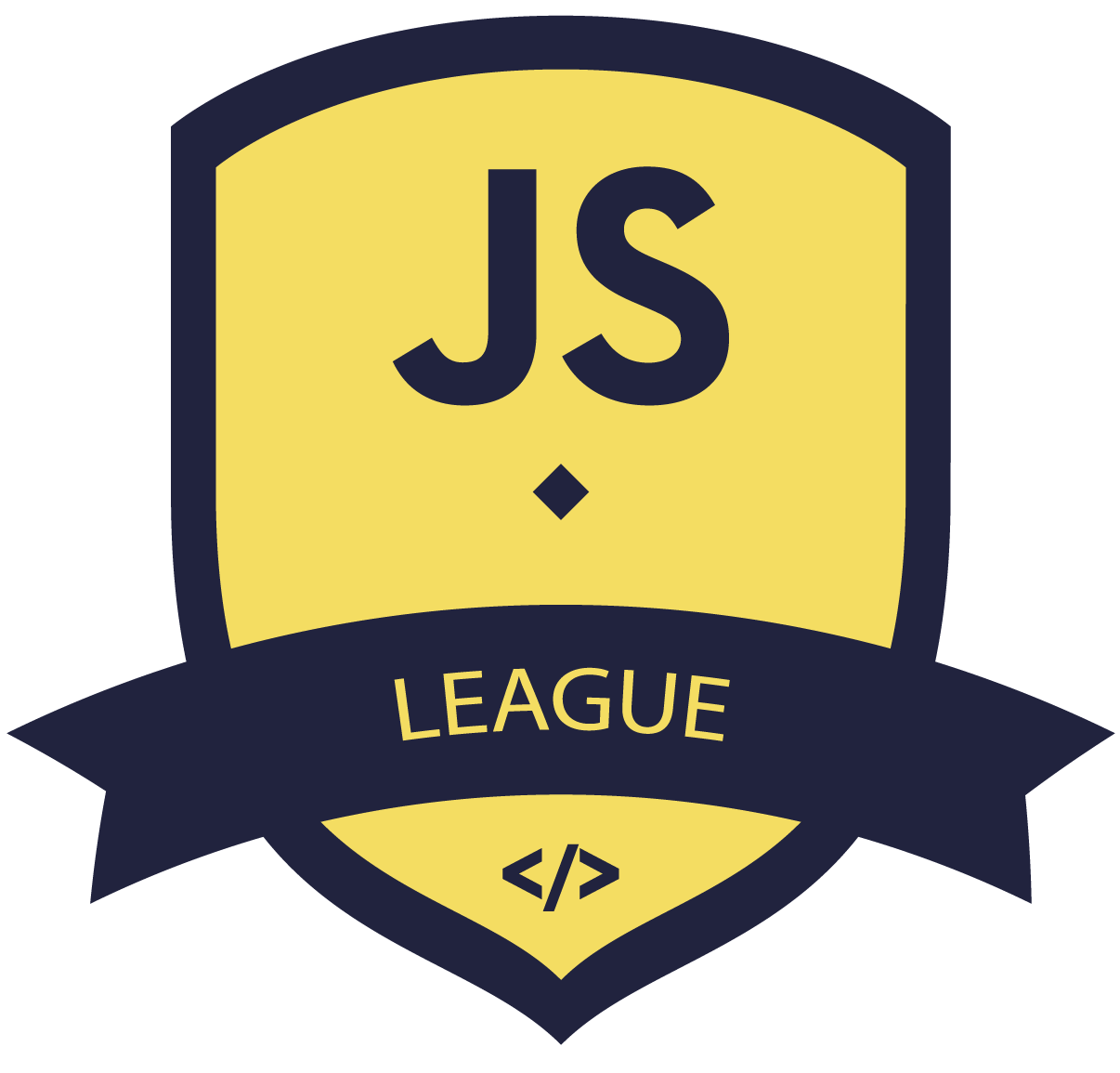
Module 2
Dumb Component
import React from 'react';
export default ({ counter, setCounter }) => (
<div>
<span>Count: {counter}</span>
<button onClick={() => setCounter(counter + 1)}>+1</button>
</div>
);
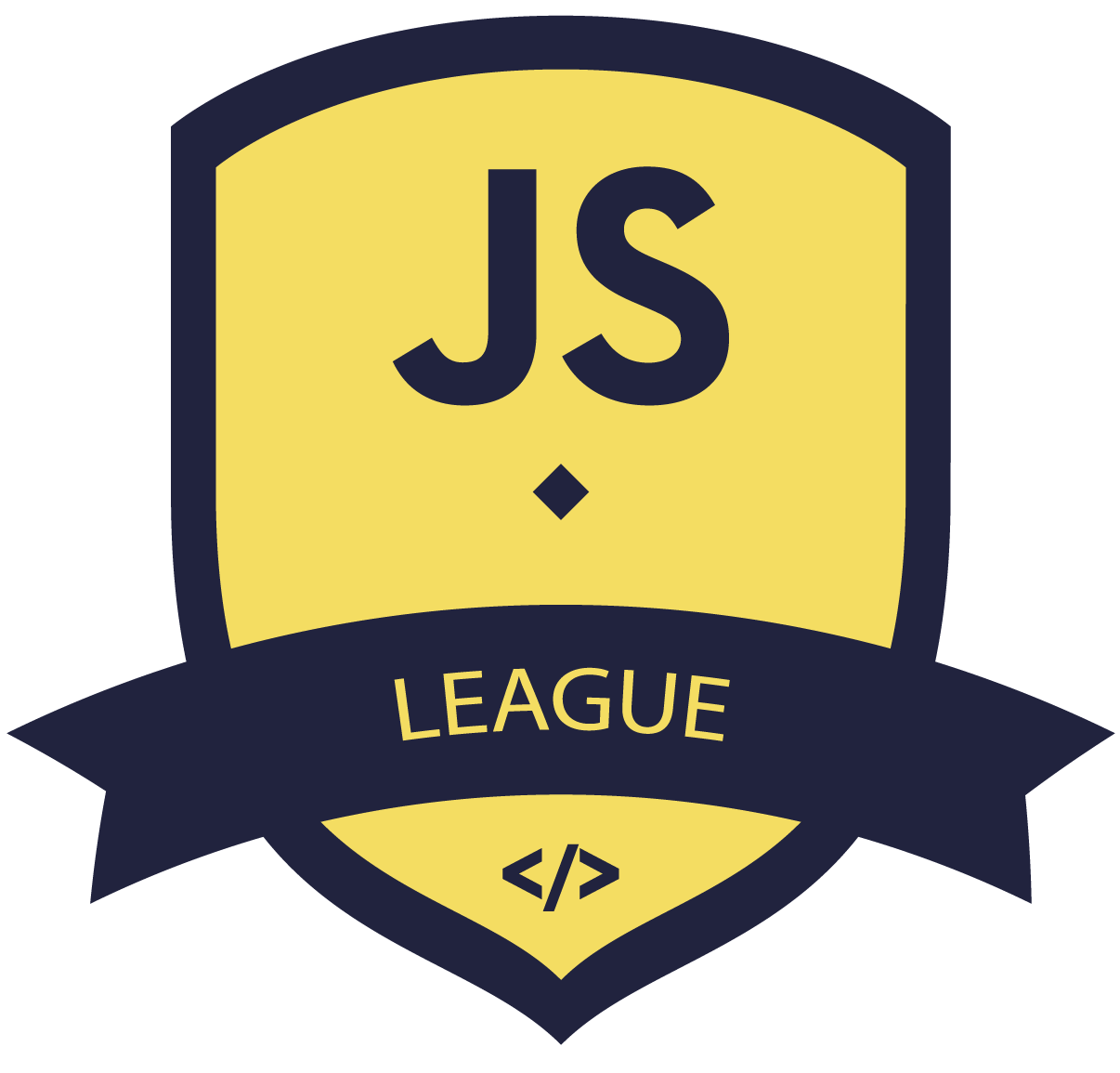
Module 2
Dumb Component
export default function() {
var _component = function() {
var _this;
for (var _len = arguments.length, args = new Array(_len), _key = 0; _key < _len; _key++) {
args[_key] = arguments[_key];
}
return _this;
}
Object(babel_runtime_helpers.createClass(_component, [{
key: "render",
value: function render() {
var counter = this.props.counter;
var setCounter = this.props.setCounter;
return React.createElement(React.div, {
children: React.createElement(...),
});
}
}]);
return _component;
}
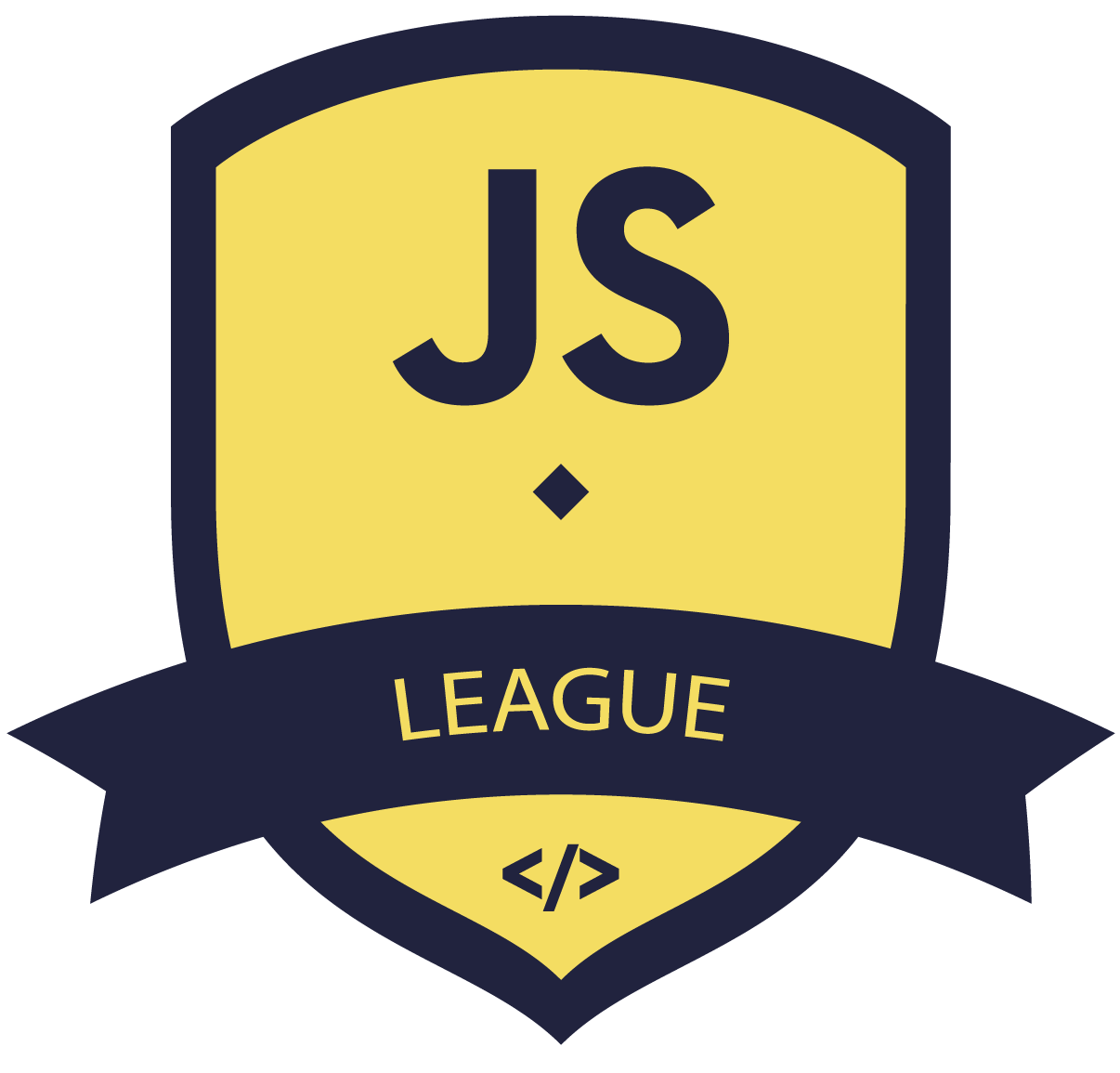
Module 2
Stateful Component
import React, { Component } from 'react';
import CounterComponent from './counter';
export default class MyComponent extends Component {
state = { counter: 0 }
updateCounter = value => this.setState({ counter: value });
render() {
const { counter } = this.state;
return (
<CounterComponent
counter={counter}
setCounter={this.updateCounter}
/>
);
}
}
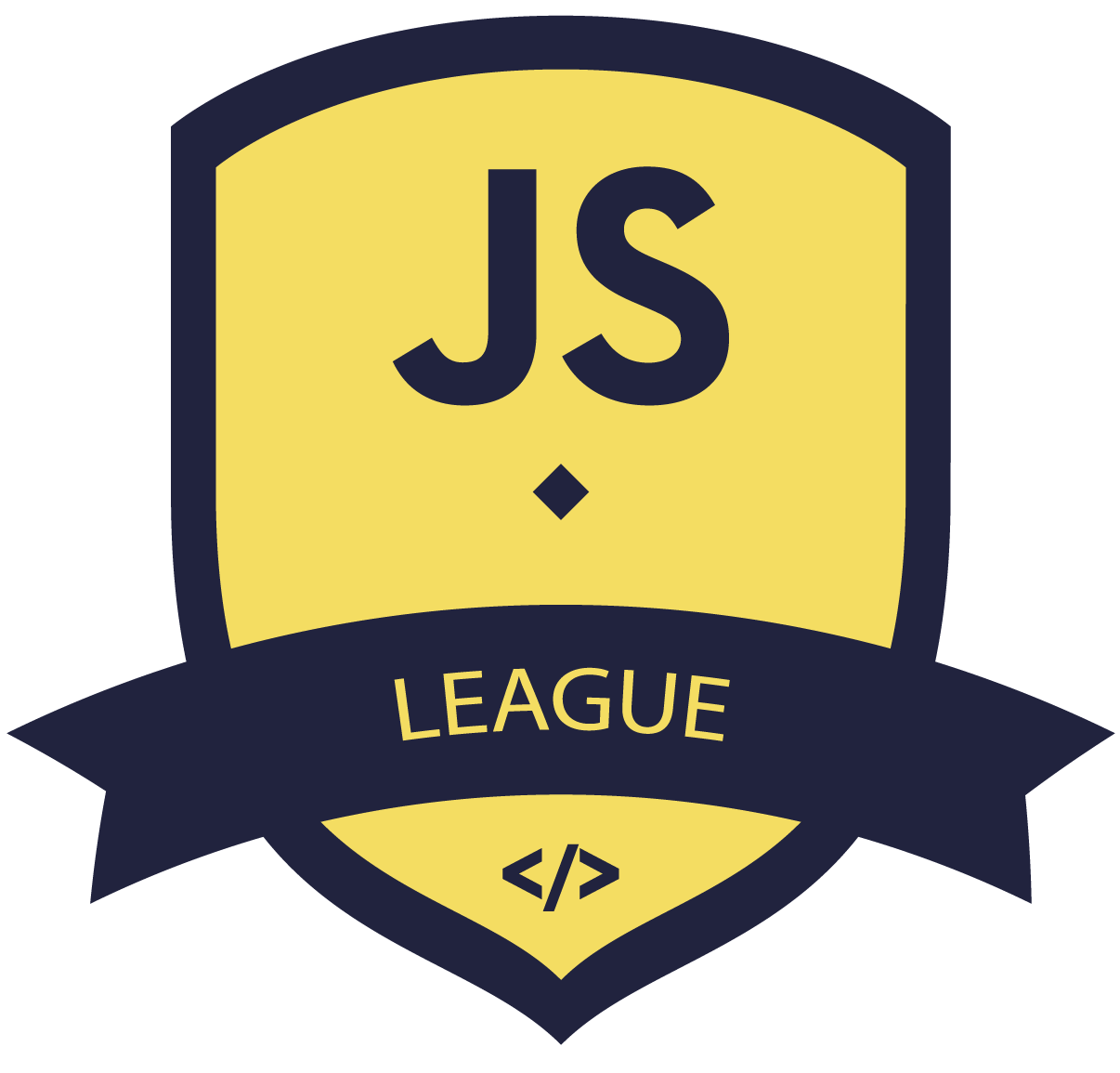
Module 2
Stateful Component (don't)
import React, { Component } from 'react';
import CounterComponent from './counter';
export default class MyComponent extends Component {
constructor() {
super();
this.state = { counter: 0 };
this.updateCounter = this.updateCounter.bind(this);
}
updateCounter(value) { this.setState({ counter: value }); }
render() {
const { counter } = this.state;
return (
<CounterComponent
counter={counter}
setCounter={this.updateCounter}
/>
);
}
}
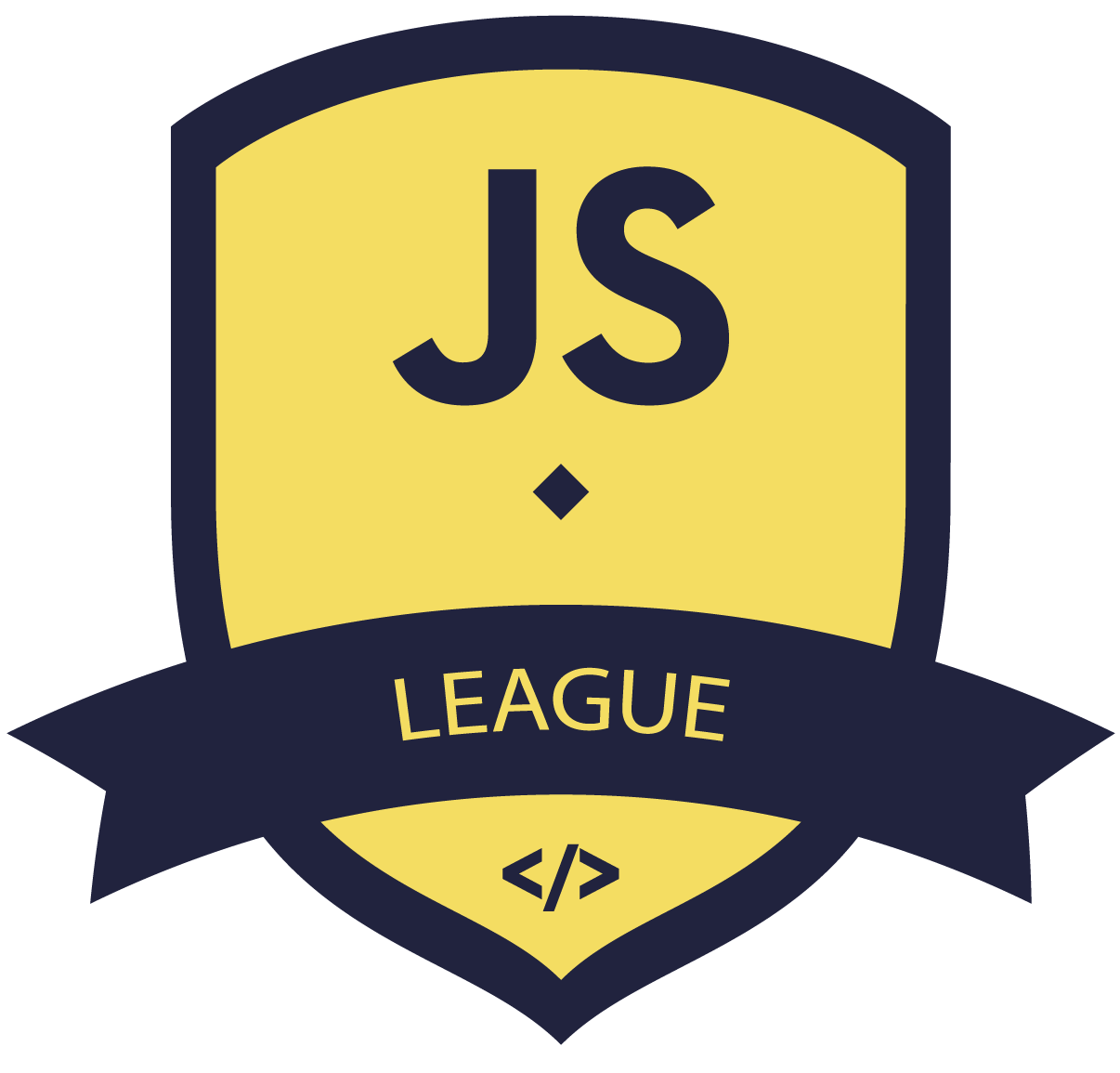
Module 2
State?
class MyComponent extends Component {
...
render() {
const { isEditing } = this.state;
const { data, updateData } = this.props;
...
}
}
class RootComponent extends Component {
...
render() {
return <MyComponent data={...} updateData={...} />
}
}
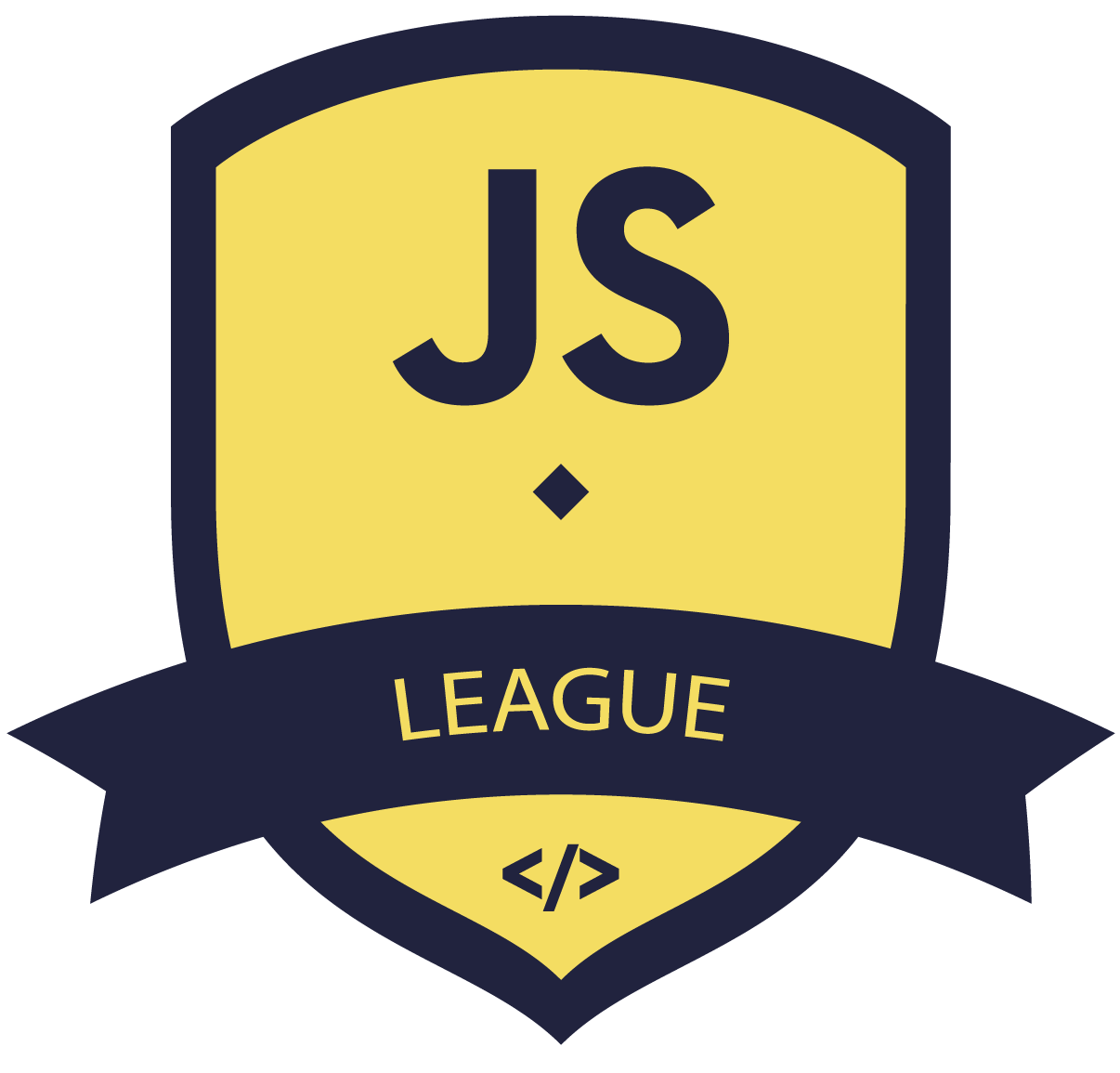
Module 2
Lifecycle
class MyComponent extends Component {
// on initial render
componentWillMount() //deprecated
componentDidMount()
// props changes
componentWillReceiveProps()
componentShouldUpdate()
componentWillUpdate()
// state changes
componentWillUpdate()
// component is no longer being rendered
componentWillUnmount()
...
}
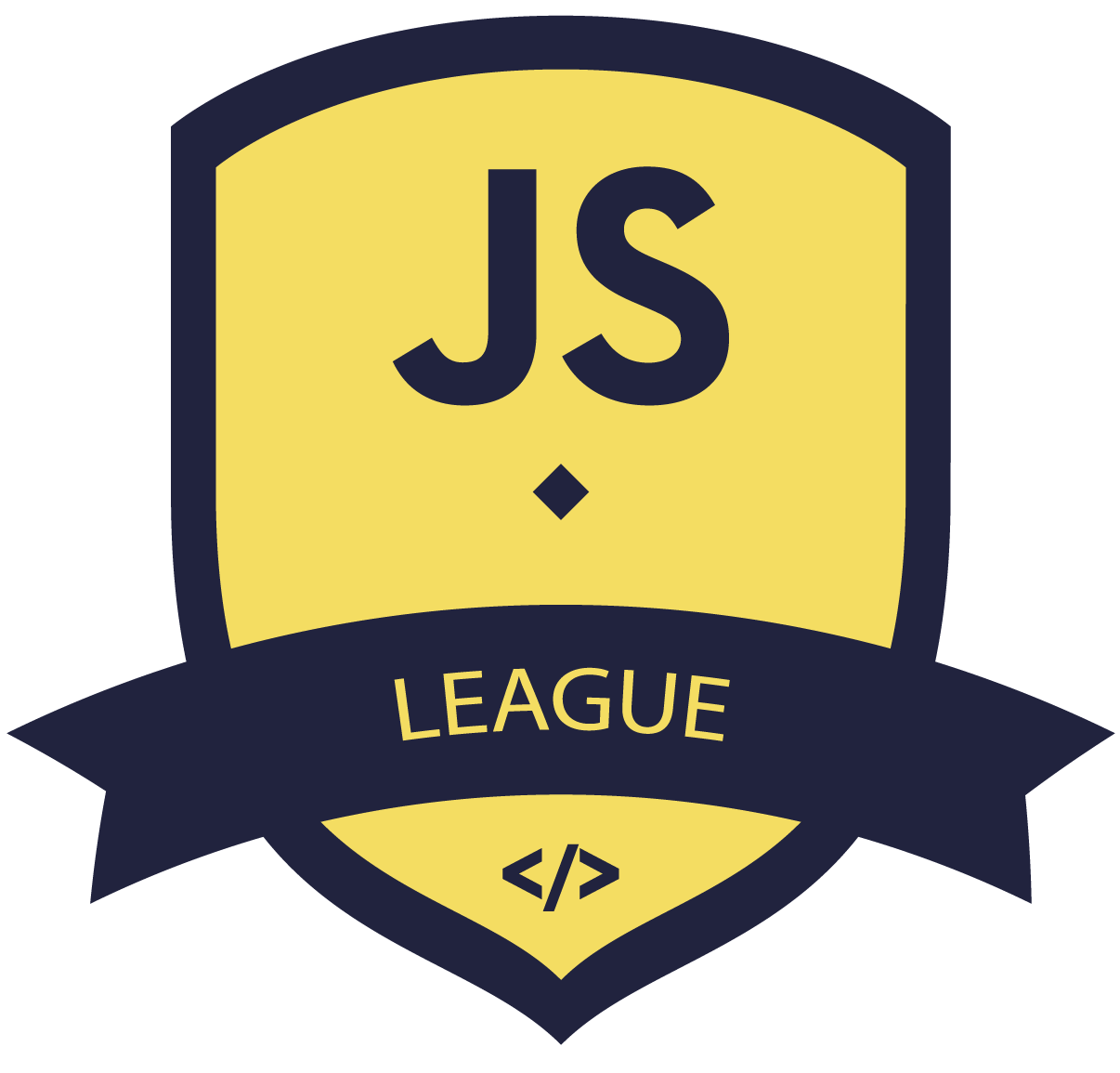
Getting Started
Module 3
Let's play with React
$ yarn global add create-react-app
$ npm install -g create-react-app
$ create-react-app react-demo
$ cd react-demo
$ yarn start
$ npm run start
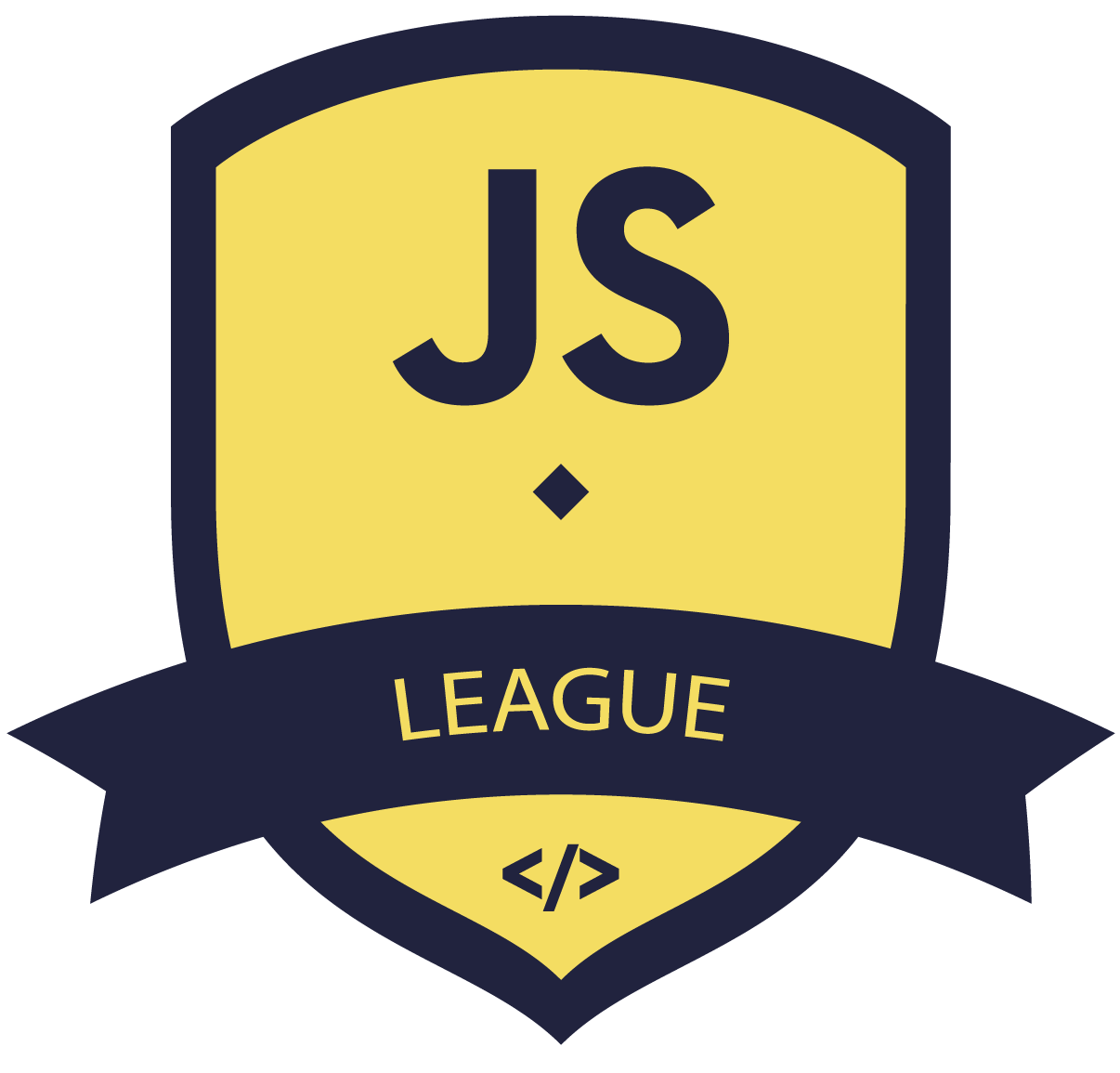
Module 3
Creating a new App with CRA
Exercise Time
Modify something
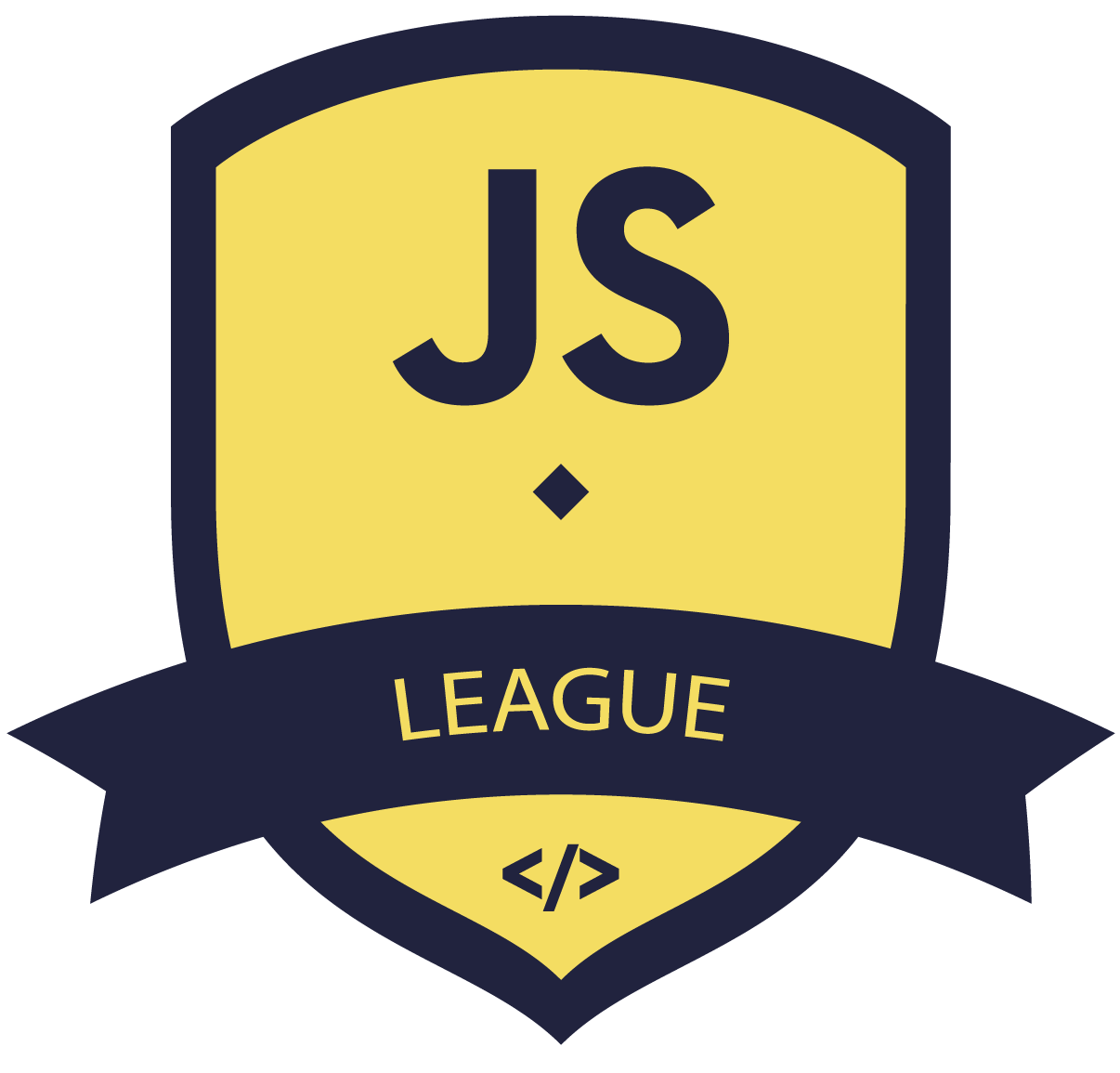
Module 3
Create an App
Let's get started
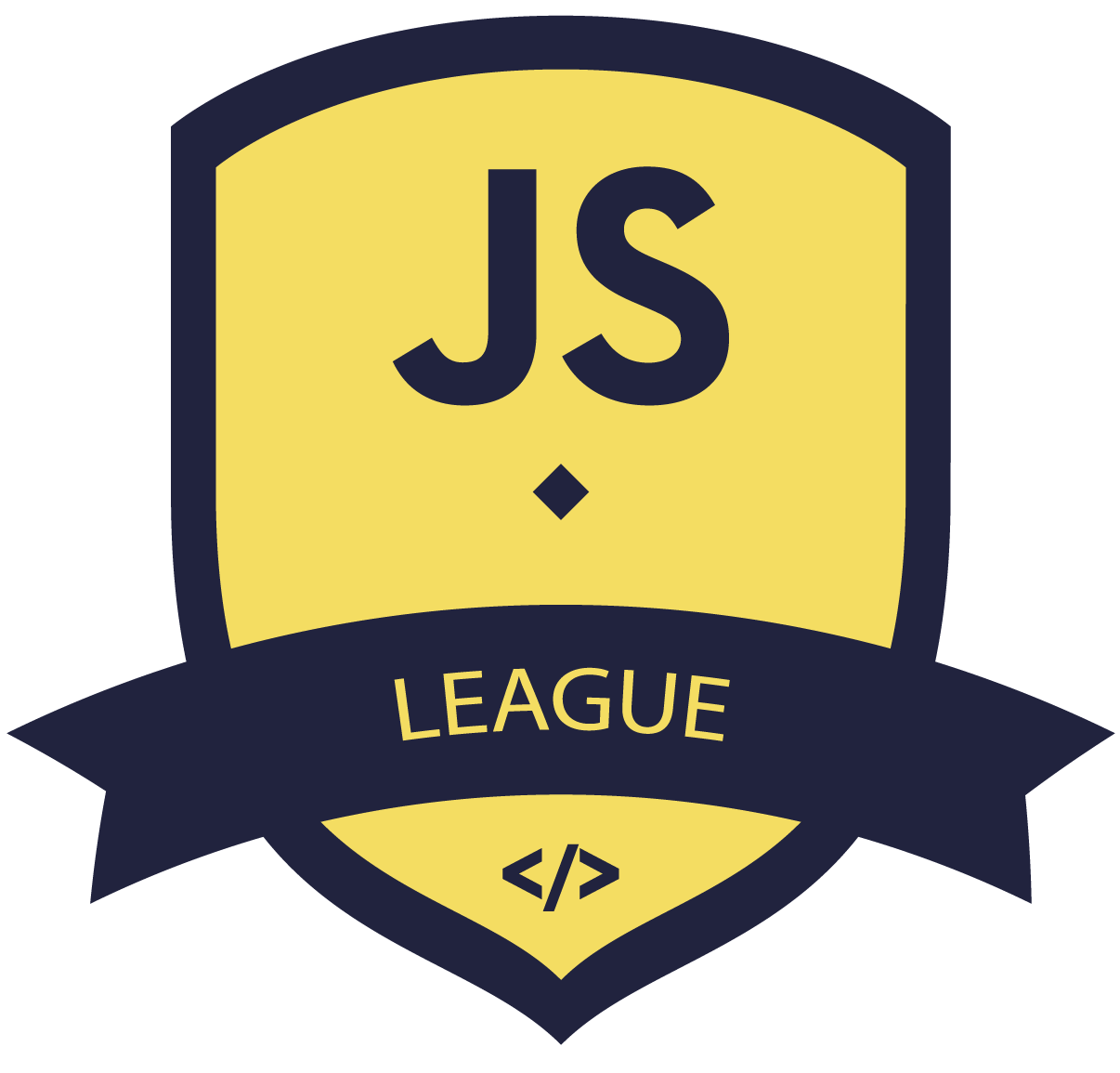
Module 4
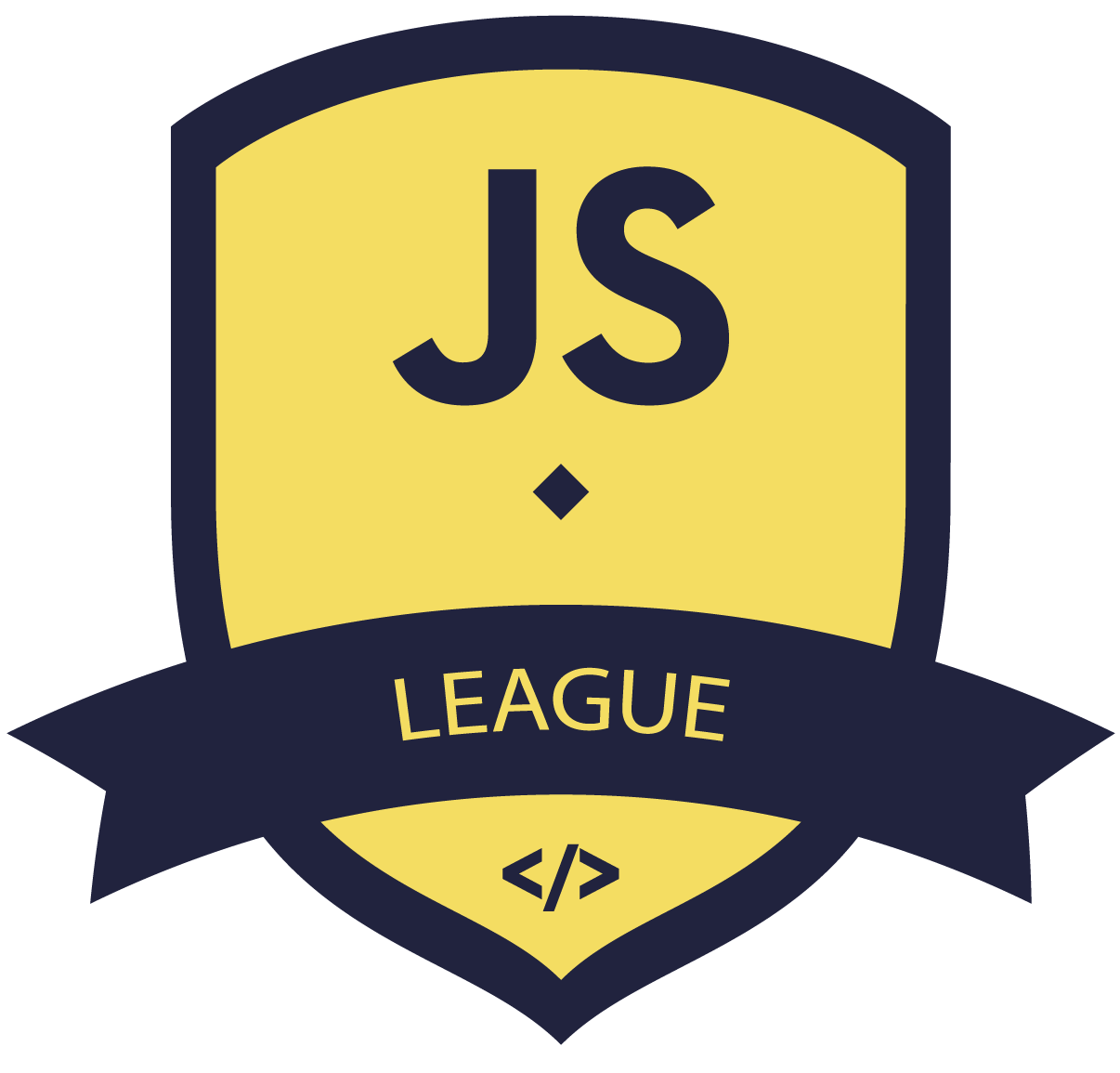
Module 4
Considerations:
- We will be using CSS Modules
- Files of form name.module.css
- Class names exported
- CSS Compiled and bundled
- We will be using react-toolbox for UI
Let's get to it
Cool Stuff
And what can you do with React nowadays
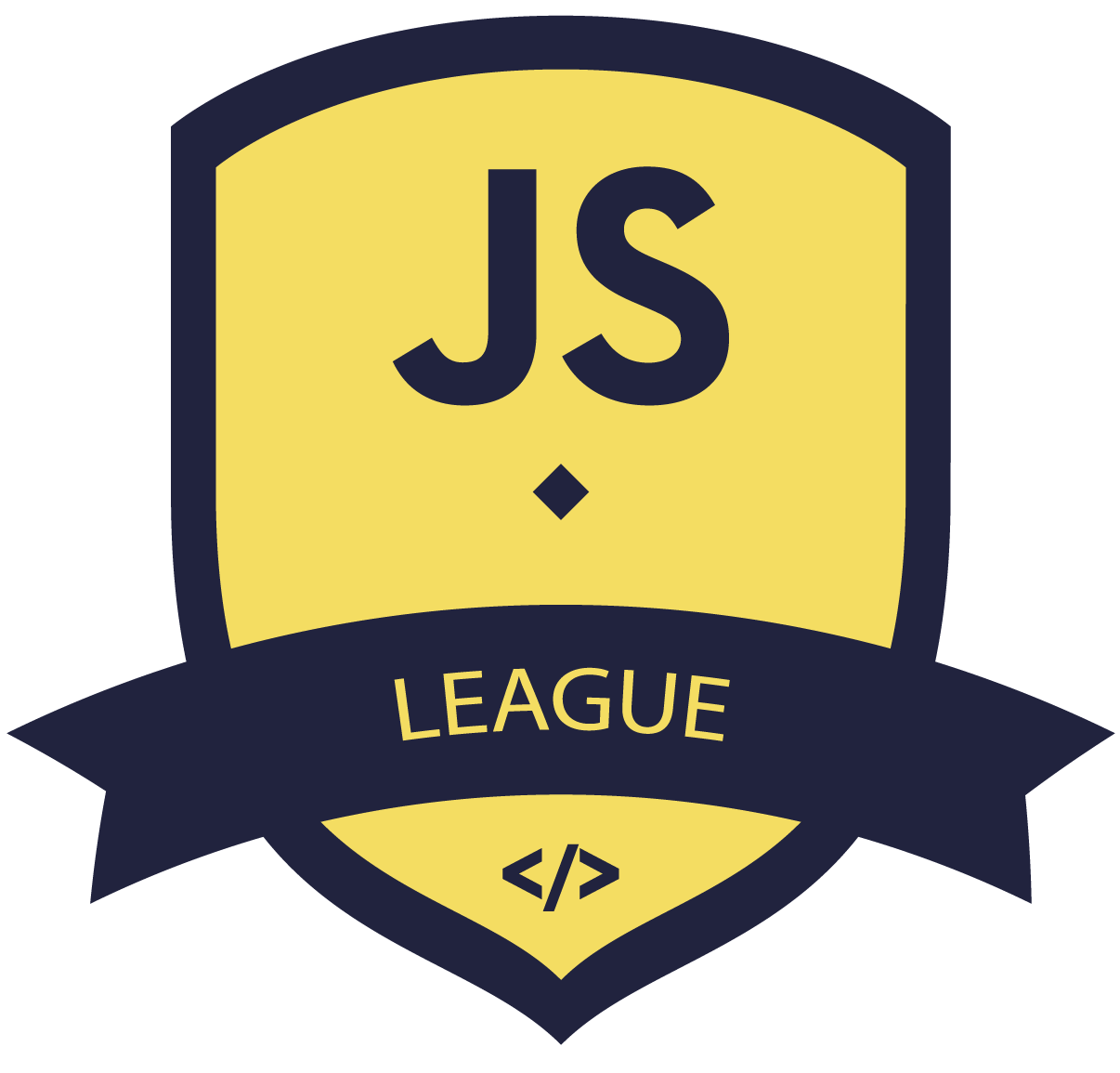
Module 5
Show me cool stuff
- React Hooks
- Redux
- Rewire
- Mobx
- Overmind
- Monorepos
Thank you!
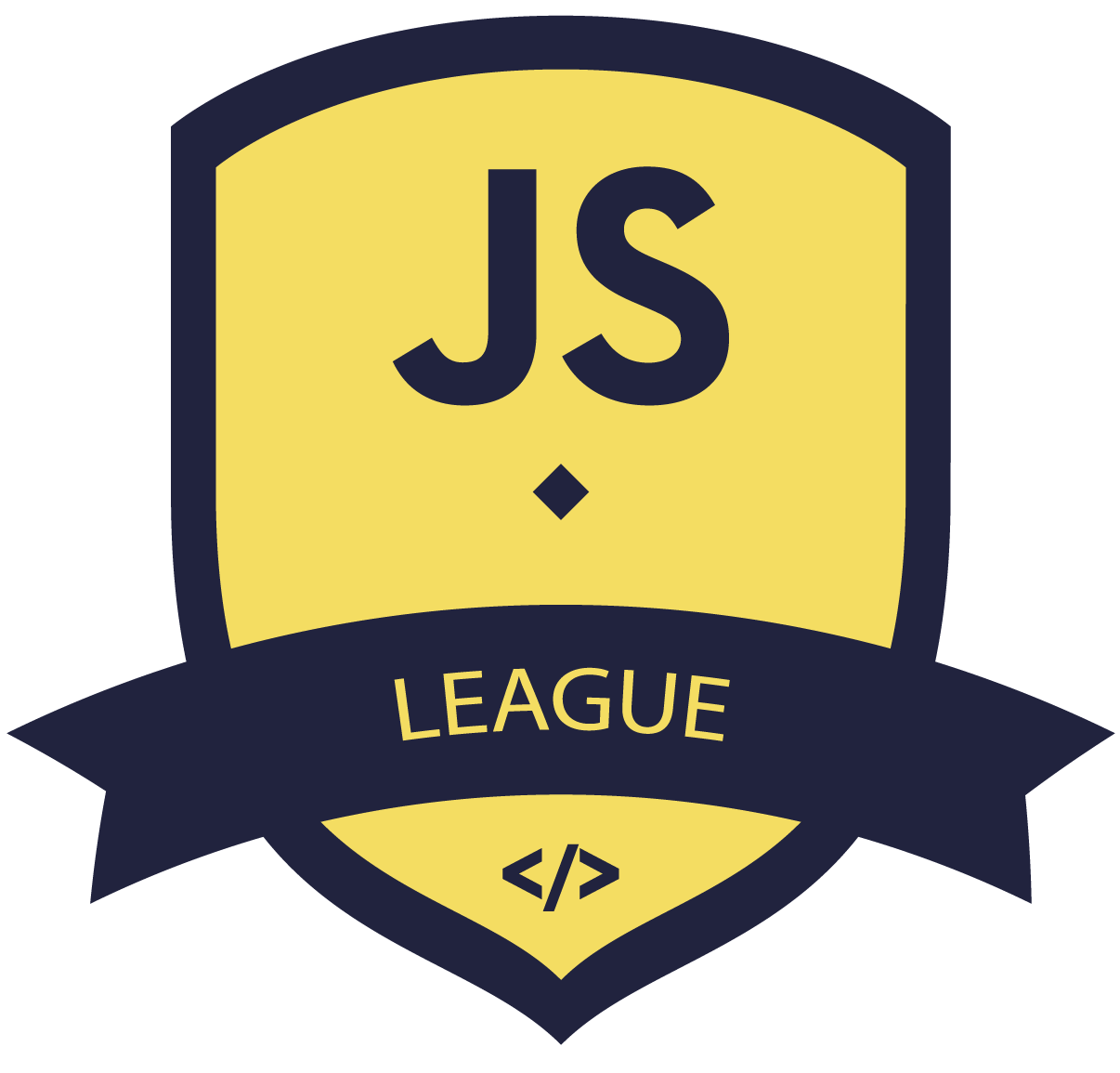
React Intro Workshop
By Sabin Marcu
React Intro Workshop
- 559