Angular
In Deep

Angular 2 released!!!
22-23 Сентябрь 2014 - 15 Сентябрь 2016
GoodBye!!!
ANGULAR1 IS DYING ?
1.3M
4.8K
ANGULAR2 IS A M*CRO-FRAMEWORK

Choose your Destiny!!!
var TodoComp = ng
.Component({
selector: 'todo',
templateUrl: '../partials/todo.html'
})
.Class({
constructor: function (
heroService,
routeParams
) {}
select(todo){...}
});
@Component({
selector: 'todo',
templateUrl: '../partials/todo.html'
})
export class TodoComp {
constructor(
private heroService: HeroService,
private routeParams: RouteParams
){}
select(todo: Todo): Boolean {...}
}
Javascript
Typescript

Safari (7+), iOS (7+), Edge (14) и IE mobile (11) протестированы на BrowserStack.
Поддержка браузеров
Under the Hood

DI.js (Angular2 dependency injector)

class Car {
constructor(engine: Engine, tires: Tires, doors: Doors) {
this.engine = engine;
this.tires = tires;
this.doors = doors;
}
}
class Car {
constructor() {
this.engine = new Engine();
this.tires = Tires.getInstance();
this.doors = app.get('doors');
}
}
DI Design Pattern
before
after
ZONE

- Микротаски (MicroTasks) - XMLHttpRequests
- Макротаски (MacroTasks) - Timers
- События (EventTasks) - Events
3 основные причины изменения Application state
Все они asynchronous.

Причем здесь Angular 2?
@Component({
selector: 'my-component',
template: `
<h3>We love {{ name }}</h3>
`
})
class MyComponent implements OnInit {
name: string = 'EPAM';
ngOnInit() {
setTimeout(() => {
this.name = 'Angular';
}, 1000);
}
}
RXJS

$watcher
$scope.$watch('foo.bar', (newVal, oldVal) => {
if (newVal.length <= 0)
return;
let firstTransformation = removeSpaces(newVal);
let secondTransoformation = firstTransformation.toUpperCase();
$scope.result = thirdTransformation;
});
foo.bar$ // An Observable
.filter(v => v.length > 0)
.map(v => removeSpaces(v))
.map(v => v.toUpperCase())
.subscribe(
v => $scope.result = v /* An Observer */
);
Observable вместо $watch
let obs$ = Rx.Observable.from(1, 2, 3, 4, 5);
obs.subscribe(x => console.log(x)); // 1, 2, 3, 4, 5
let evenObs$ = obs.filter(x => x%2 == 0 ));
evenObs$.subscribe(x => console.log(x)) // 2, 4
evenObs$.map(x => x*2).subscribe(x => console.log(x)) // 4, 8
Observable are immutable, composable and reusable

Observables
vs
Promises
.then()
становится
.subscribe()
Http
Forms
AsyncPipe
Change detection
Что Observalbe в Angular 2
SYSTEMJS
In AngularJS (it's so 2009...)
angular.module('FooModule', [
'BarModule',
'anotherModule'
]);
In ES2015 & SystemJS
import { BarModule } from './bar.module';
import { AnotherComp } from './another.module';
ONLY SYSTEMJS ?


Webpack
JSPM
Rollup.js
HTML5 API'S!

CSS3 QUERY SELECTORS
let firstClass = document.querySelector('.some-class');
let allOfClass = document.querySelectorAll('.some-class');
CSS CLASS MANIPULATION
let elem = document.querySelector('#some-element');
elem.classList.add('some-class'); // Add class
elem.classList.remove('some-other-class'); // Remove class
elem.classList.toggle('some-other-class') // ...toggle...
elem.classList.contains('some-third-class') // Does it have some class ?
elem.style.color; // Get a CSS attribute
elem.style.color = 'rebeccapurple'; // Set a CSS attribute
elem.style.minHeight; // Get a CSS attribute
elem.style.minHeight = '200px'; // Set a CSS attribute
Same thing on inline style...
WORKING WITH EVENT
let elem = document.querySelector('#some-element');
elem.addEventListener('click', () => console.log('clicked'), false);
elem.addEventListener('customEvent', anotherFunction, false);
NAVIGATING INTO THE DOM
let elem = document.querySelector('#some-element');
let parent = elem.parentNode;
let childs = elem.childNodes;
let specificChild = elem.querySelector('anotherClass');
let specificChilds = elem.querySelectorAll('anotherClass');
Angular App Structure

Основные компоненты Angular
App structure
File Naming

{name}.{type}.ts|html|css|spec.ts
name: kebab-case | dash-case
type:
- module
- routing
- component
- directive
- pipe
- service
Class Naming
{Name}{Type} - PascalCase
@Component({ ... })
export class AppComponent { }
Angular Modules
import { NgModule } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
import { AppComponent } from './app.component';
import { MyService } from './my.service';
@NgModule({
imports: [ BrowserModule ],
exports: [ AppComponent ],
declarations: [ AppComponent ],
providers: [ MyService, { provide: ... } ],
bootstrap: [ AppComponent ]
})
export class AppModule { }
app.module.ts
Bootstrapping in main.ts
// The browser platform with a compiler
import { platformBrowserDynamic } from '@angular/platform-browser-dynamic';
// The app module
import { AppModule } from './app.module';
// Compile and launch the module
platformBrowserDynamic().bootstrapModule(AppModule);
Dynamic bootstrapping with the Just-in-time (JiT) compiler
main.ts
// The browser platform without a compiler
import { platformBrowser } from '@angular/platform-browser';
// The app module factory produced by the static offline compiler
import { AppModuleNgFactory } from './app.module.ngfactory';
// Launch with the app module factory.
platformBrowser().bootstrapModuleFactory(AppModuleNgFactory);
Static bootstrapping with the Ahead-Of-time (AoT) compiler
main.ts


Data flows
LIFECYCLE
export class PeekABoo implements OnInit {
constructor(private logger: LoggerService) { }
// implement OnInit's `ngOnInit` method
ngOnInit() { this.logIt(`OnInit`); }
logIt(msg: string) {
this.logger.log(`#${nextId++} ${msg}`);
}
}
LIFECYCLE
- OnChange - input|output binding value changes
- OnInit - after the 1st OnChange
- DoCheck - dev's custom change detection
- AfterContentInit - after comp content initialized
- AfterContentChecked - after every check of comp content
- AfterViewInit - after comp's view(s) are initialized
- AfterViewChecked - after every check of a comp's view(s)
- OnDestroy - just before destruction

CHANGE DETECTION

Наступила Новая ЭРА!
ЭРА Прогрессивнового веб приложения
Чё Серьёзно?
Flow of events with Just-in-Time Compilation
Flow of events with Ahead-of-Time Compilation


Tree Shaking
UNIVERSAL ANGULAR
Also know as Server-Side-Rendering
- Increase performance at first load
-
Help for SEO

WEB-WORKER
A way to do 'heavy' calculation in another thread

SERVICE-WORKER
After Mobile First, Offline-First
Cache
Offline
Synchronisation


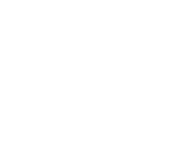
Полезные ссылки
Angular2 in Deep
By Shuhratbek Mamadaliyev
Angular2 in Deep
- 1,463