JAVA 객체지향
디자인패턴
2018.09.04
Chapter 14 컴퍼지트 패턴
1. 컴퓨터에 추가 장치 지원하기
Computer
- Keyboard
- Body
- Monitor
1. 컴퓨터에 추가 장치 지원하기
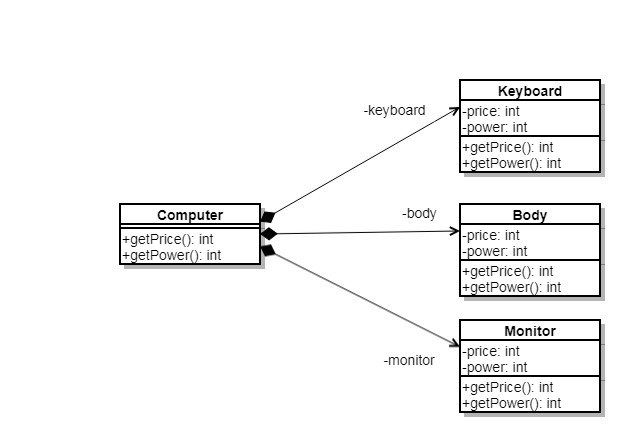
1. 컴퓨터에 추가 장치 지원하기
public class Keyboard {
private int price;
private int power;
public Keyboard(int power, int price) {
this.power = power;
this.price = price;
}
public int getPrice() {
return price;
}
public int getPower() {
return power;
}
}
1. 컴퓨터에 추가 장치 지원하기
public class Computer {
private Body body;
private Keyboard keyboard;
private Monitor monitor;
public void addBody(Body body) {
this.body = body;
}
public void addKeyboard(Keyboard keyboard) {
this.keyboard = keyboard;
}
public void addMonitor(Monitor monitor) {
this.monitor = monitor;
}
public int getPrice() {
int bodyPrice = body.getPrice();
int keyboardPrice = keyboard.getPrice();
int monitorPrice = monitor.getPrice();
return bodyPrice + keyboardPrice + monitorPrice;
}
public int getPower() {
int bodyPower = body.getPower();
int keyboardPower = keyboard.getPower();
int monitorPower = monitor.getPower();
return bodyPower + keyboardPower + monitorPower;
}
}
2. 문제점
Computer
- Keyboard
- Body
- Monitor
추가해줘
- Speaker
- Mouse
2. 문제점
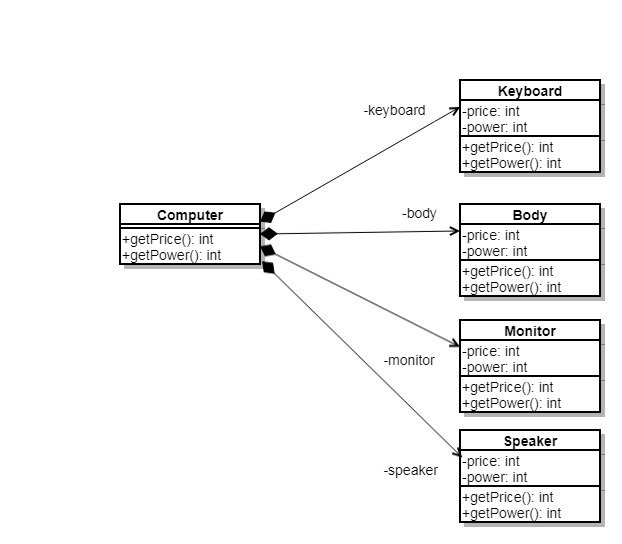
2. 문제점
public class Speaker {
private int price;
private int power;
// 생략
}
- Speaker class 추가
2. 문제점
- Computer class 수정
public class Computer {
private Body body;
private Keyboard keyboard;
private Monitor monitor;
private Speaker speaker; // 추가
// add 함수 생략
public void addSpeaker(Speaker speaker) {
this.speaker = speaker;
}
public int getPrice() {
int bodyPrice = body.getPrice();
int keyboardPrice = keyboard.getPrice();
int monitorPrice = monitor.getPrice();
int speakerPrice = speaker.getPrice(); // 추가
return bodyPrice + keyboardPrice + monitorPrice + speakerPrice; // 수정
}
2. 문제점
- Computer class 수정
public class Computer {
private Body body;
private Keyboard keyboard;
private Monitor monitor;
private Speaker speaker; // 추가
// add 함수 생략
public void addSpeaker(Speaker speaker) {
this.speaker = speaker;
}
public int getPrice() {
int bodyPrice = body.getPrice();
int keyboardPrice = keyboard.getPrice();
int monitorPrice = monitor.getPrice();
int speakerPrice = speaker.getPrice(); // 추가
return bodyPrice + keyboardPrice + monitorPrice + speakerPrice; // 수정
}
OCP 위반
3. 해결책
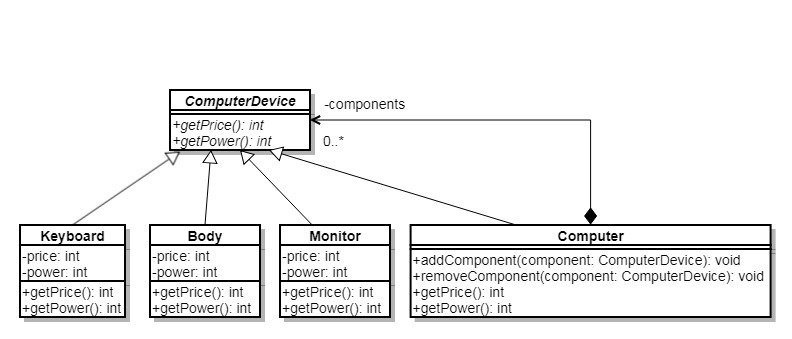
3. 해결책
public abstract class ComputerDevice {
public abstract int getPrice();
public abstract int getPower();
}
public class Keyboard extends ComputerDevice {
private int price;
private int power;
public Keyboard(int power, int price) {
this.power = power;
this.price = price;
}
public int getPrice() {
return price;
}
public int getPower() {
return power;
}
}
3. 해결책
public class Computer extends ComputerDevice {
private List<ComputerDevice> components = new ArrayList<>();
public void addComponent(ComputerDevice component) {
components.add(component);
}
public void removeComponent(ComputerDevice component) {
components.remove(component);
}
public int getPrice() {
int price = 0;
for (ComputerDevice component : components) {
price += component.getPrice();
}
return price;
}
public int getPower() {
int power = 0;
for (ComputerDevice component : components) {
power += component.getPower();
}
return power;
}
}
3. 해결책
public class Client {
public static void main(String[] args) {
Body body = new Body(100, 70);
Keyboard keyboard = new Keyboard(5, 2);
Monitor monitor = new Monitor(20, 30);
Computer computer = new Computer();
computer.addComponent(body);
computer.addComponent(keyboard);
computer.addComponent(monitor);
int computerPrice = computer.getPrice();
int computerPower = computer.getPower();
}
}
4. 컴퍼지트 패턴
부분 - 전체 관계 정의할 때 유용
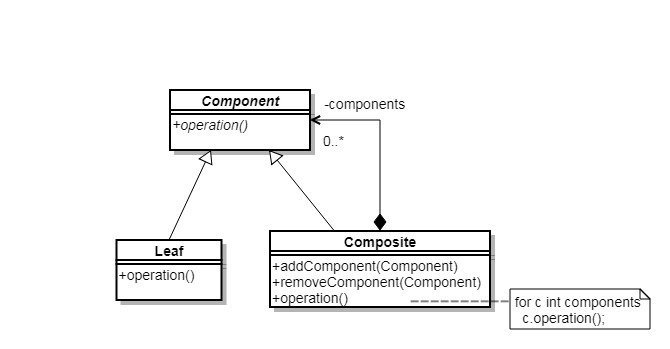
- Component: 구체적인 부분, Leaf, Composite 클래스의 공통 인터페이스
- Leaf: 구체적인 부분 클래스, Composite 객체의 부품
- Composite: 전체 클래스, 복수개의 Component 갖도록 정의
JAVA 객체지향디자인패턴 - 컴퍼지트 패턴
By Sungbin, Song
JAVA 객체지향디자인패턴 - 컴퍼지트 패턴
- 149