Principles of OOP - Part 1
Telerik Academy Alpha
Table of contents
Fundamental principles of OOP
Principles
-
Inheritance, encapsulation, abstraction, and polymorphism are four fundamental concepts of object-oriented programming.
- Inheritance - Inherit members from parent class
- Abstraction - Define and execute abstract actions
- Encapsulation - Hide the internals of a class
- Polymorphism - Access a class through its parent interface
Inheritance
Inheritance
-
Inheritance allows child classes to inherit the characteristics of an existing parent (base) class
- Attributes(fields and properties)
- Operations(methods)
-
Child class can extend the parent class
- Add new fields and methods
- Redefine methods(modify existing behavior)
- A class can implement an interface by providing implementation for all its methods
Inheritance
derived class | inherits | base(parent) class |
---|---|---|
class | implements | interface |
derived interface | extends | base interface |

Inheritance - benefits
-
Inheritance has a lot of benefits
- Extensibility
- Reusability (code reuse)
- Provides abstraction
- Eliminates redundant code
-
Use inheritance for buidling is-a relationships
- E.g.dog is-a animal(dogs are kind of animals)
-
Don't use it to build has-a relationship
- E.g.dog has-a name(dog is not kind of name)
Inheritance - benefits
-
Inheritance implicitly gains all members from another class
- All fields, methods, properties, events, …
- Some members could be inaccessible (private)
- The class whose methods are inherited is called base (parent) class
- The class that gains new functionality is called derived (child) class
Inheritance in .NET
-
A class can inherit only one base class
- E.g. IOException derives from SystemException and it derives from Exception
-
A class can implement several interfaces
- This is .NET’s form of multiple inheritance
- E.g. List<T> implements IList<T>, ICollection<T>,
IEnumerable<T>
-
An interface can implement several interfaces
- E.g. IList<T> implements ICollection<T> and
IEnumerable<T>
- E.g. IList<T> implements ICollection<T> and
Use inheritance
- Specify the name of the base class after the name of the derived(with colon)
public class Shape
{ … }
public class Circle : Shape
{ … }
- Use the keyword base to invoke the parent constructor
public Circle(int x, int y) : base(x)
{ … }
Inheritance - live demo
Access modifiers
-
Access modifiers in C#
- public – access is not restricted
- private – access is restricted to the containing type
- protected – access is limited to the containing type and types derived from it
- internal – access is limited to the current assembly
- protected internal – access is limited to the current assembly or types derived from the containing class
Access modifiers
Abstraction
Abstraction
- Abstraction means ignoring irrelevant features, properties, or functions and emphasizing the relevant ones...
-
... relevant to the given project
- With an eye to future reuse in similar projects
- Abstraction helps managing complexity
Abstraction
-
Abstraction is something we do every day
-
Looking at an object, we see those things about it that have meaning to us
- You ask for the name, not the size of the shoes
- We abstract the properties of the object, and keep only what we need
- E.g. students get "name" but not "color of eyes"
-
Looking at an object, we see those things about it that have meaning to us
- Allows us to represent a complex reality in terms of a simplified model
- Abstraction highlights the properties of an entity that we need and hides the others
Abstraction in .NET
- In .NET object-oriented programming abstraction is achieved in several ways:
- Abstract classes
- Interfaces
- Inheritance
Interfaces
Interfaces
-
Interfaces define a set of operations
- Also called "contract" for providing a set of operations
- Contains only the signatures of methods, properties, events or indexers
-
An interface can inherit from one or more base interfaces
- C# doesn't support multiple inheritance of classes
public interface IDrawable
{
Point StartingPoint { get; set; }
void Draw();
}
Interfaces
- Interfaces provide abstractions
- You invoke the abstract actions
- Without worrying how it is internally implemented
- Interfaces describe a prototype of group of methods(operations), properties and events
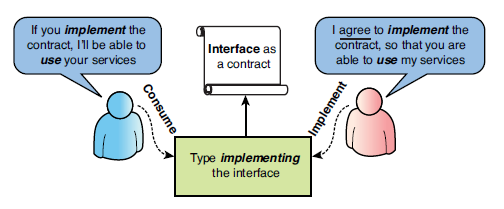
Interfaces
-
An interface has the following properties:
-
An interface is like an abstract base class. Any class or struct that implements the interface must implement all its members.
-
An interface can't be instantiated directly. Its members are implemented by any class or struct that implements the interface.
-
Interfaces
-
An interface has the following properties:
- Interfaces can contain events, indexers, methods, and properties.
-
Interfaces contain no implementation of methods.
-
A class or struct can implement multiple interfaces. A class can inherit a base class and also implement one or more interfaces.
Interfaces Exmaples
namespace System.Collections.Generic
{
public class List<T> :
IList<T>,
ICollection<T>,
IList,
ICollection,
IReadOnlyList<T>,
IReadOnlyCollection<T>,
IEnumerable<T>,
IEnumerable
{ ... }
}
- List class implementation
Interfaces Exmaples
namespace System.Collections.Generic
{
public interface IList<T> : ICollection<T>, IEnumerable<T>, IEnumerable
{
T this[int index] { get; set; }
int IndexOf(T item);
void Insert(int index, T item);
void RemoveAt(int index);
}
}
- IList<T> interface
Interfaces Exmaples
namespace System.Collections.Generic
{
public interface ICollection<T> : IEnumerable<T>, IEnumerable
{
bool IsReadOnly { get; }
void Add(T item);
void Clear();
bool Contains(T item);
void CopyTo(T[] array, int arrayIndex);
bool Remove(T item);
}
}
- ICollection<T> interface
Interfaces - live demo
Abstract classes
Abstract classes
-
Abstract classes are special classes defined with the keyword abstract
- Mix between class and interface
- Partially implemented or fully unimplemented
- Not implemented methods are declared abstract and are left empty
- Cannot be instantiated directly
- Child classes should implement all abstract methods/properties or be declared as abstract too
Abstract classes
-
Abstract methods are empty methods without implementation
- The implementation is intentionally left for the derived classes
- When a class contains at least one abstract method/property, it is called abstract class and must be declared as such
-
Abstract classes model abstract concepts
- E.g. person, object, item, movable object
Abstract classes - live demo
Abstract class vs Interface
-
C# interfaces are like abstract classes, but in contrast interfaces:
-
Can not contain methods with implementation
- All interface methods are abstract
-
Members do not have scope modifiers
- Their scope is assumed public
- But this is not specified explicitly
- Can not define fields, constants, inner types and constructors
-
Can not contain methods with implementation
Encapsulation
Encapsulation
- Encapsulation hides the implementation details
- Class announces some operations (methods) available for its clients – its public interface
-
All data members (fields) of a class should be hidden
- Accessed via properties (read-only and read-write)
- No interface members should be hidden

Encapsulation
-
Fields are always declared private
- Accessed through properties in read-only or read-write mode
- Constructors are almost always declared public
-
Interface members are always public
- Not explicitly declared with public
- Non-interface methods are declared
private / protected / internal
Encapsulation
-
Ensures that structural changes remain local:
- Changing the class internals does not affect any code outside of the class
- Changing methods' implementation does not reflect the clients using them
-
Encapsulation allows adding some logic when accessing client's data
- E.g. validation on modifying a property value
- Hiding implementation details reduces complexity → easier maintenance
Encapsulation - live demo
Questions?
[C# OOP] Principles of OOP - Part 1
By telerikacademy
[C# OOP] Principles of OOP - Part 1
- 1,463