Linear data structures
Telerik Academy Alpha
DSA
Table of contents
Lists
List

0
1
2
Insert
Add
Remove
Arrays vs List or why using List
Array
-
Initialize array
- Resize array
- copy the items to a new array
- copy the items to a new array
- Remove item
var matrix = new int[100];
List
-
Initialize list
- Resize list
- Remove item
var list = new List<int>();
var list = new List<int>();
list.Add(42);
var list = new List<int>();
list.Remove(42);
List
- What is "list"?
- A data structure (container) that contains a sequence of elements
- Can have variable size
- Elements are arranged linearly, in sequence
- Can be implemented in several ways
- Statically (using array → fixed size)
- Dynamically (linked implementation)
- Using resizable array (the List<T> class)
- A data structure (container) that contains a sequence of elements
Static List
- Implemented by an array
- Provides direct access by index
- Has fixed capacity
- Insertion, deletion and resizing are slow operations
The List<T> Class
- Implements the abstract data structure list using an array
- All elements are of the same type T
- T can be any type, e.g. List<int>, List<string>, List<DateTime>
- Size is dynamically increased as needed
- Basic functionality:
- Count - returns the number of elements
- Add(T) - appends given element at the end
List<T>: How it works
- List<T> keeps a buffer memory, allocated in advance, to allow fast Add(T)
- Most operations use the buffer memory and do not allocate new objects
- Occasionally the capacity grows (doubles)
LinkedList

Linked List
- Dynamic (pointer-based) implementation
- Different forms
- Singly-linked and doubly-linked
- Sorted and unsorted
- Singly-linked list
- Each item has 2 fields: value and next
Doubly-LinkedList

Head
Tail
0
1
2
3
4
Doubly-Linked List
- Each item has 3 fields: value, next and prev
LinkedList<T> Class
- Implements the abstract data structure list using a doubly-linked dynamic structure
- All elements are of the same type T
- T can be any type, e.g. LinkedList<int>, LinkedList<string>, etc.
- Elements can be added at both sides
- Basic LinkedList<T> functionality:
- AddFirst(T), AddLast(T), AddBefore(T), AddAfter(T),
- RemoveFirst(T), RemoveLast(T), Count
List Interfaces in .NET
List Interfaces in .NET
- IEnumerable, IEnumerable<T>
- GetEnumerator() → Current, MoveNext()
- ICollection, ICollection<T>
- Inherits from IEnumerable<T>
- Count, Add(…), Remove(…), Contains(…)
- IList, IList<T>
- Inherits from ICollection<T>
- Item / indexer [], Insert(…), RemoveAt(…)
List Interfaces in .NET
Quizlet
- LinkedList<T> ----------> IList or not
- Why using LinkedList instead of List
- Performance of insert - List or LinkedList
- Performance of search - List or LinkedList
- How would you insert an item after the first one
Tasks
-
Get prime numbers in an interval - [200, 300]
-
How would you delete a node (except the tail) in a singly linked list, given only access to that node.
Supposed the linked list is 1 -> 2 -> 3 -> 4 and you are given the third node with value 3, the linked list should become 1 -> 2 -> 4 after calling your function
- Reverse a LinkedList<int>:
1->2->3->4 should become 4->3->2->1
Hint:
public class ListNode
{
public int val;
public ListNode next;
public ListNode(int x) { val = x; }
}
Stacks
Undo & Redo operations
Stack

0
1
2
Push
Pop
Stack
- LIFO (Last In First Out) structure
- Elements inserted (push) at "top"
- Elements removed (pop) from "top"
- Useful in many situations
- E.g. the execution stack of the program
- Can be implemented in several ways
- Statically (using array)
- Dynamically (linked implementation)
- Using the Stack<T> class
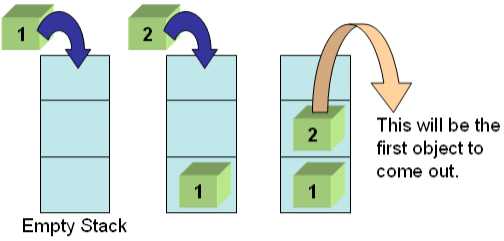
Static Stack
- Static (array-based) implementation
- Has limited (fixed) capacity
- The current index (top) moves left / right with each pop / push
Linked Stack
- Dynamic (pointer-based) implementation
- Each item has 2 fields: value and next
- Special pointer keeps the top element
Stack<T> Class
- Implements the stack data structure using an array
- Elements are from the same type T
- T can be any type, e.g. Stack<int>
- Size is dynamically increased as needed
- Basic functionality:
- Push(T) - inserts elements to the stack
- Pop() - removes and returns the top element from the stack
Matching Brackets - Example
- We are given an arithmetical expression with brackets that can be nested
- Goal: extract all sub-expressions in brackets
- Example:
- 1 + (2 - (2+3) * 4 / (3+1)) * 5
- Result:
- (2+3) | (3+1) | (2-(2+3) * 4 / (3+1))
-
Algorithm:
- For each '(' push its index in a stack
- For each ')' pop the corresponding start index
Matching Brackets - Demo
Queues
Implement a simple printer
Queue

0
1
2
Dequeue
Enequeue
Queue
- FIFO (First In First Out) structure
- Elements inserted at the tail (Enqueue)
- Elements removed from the head (Dequeue)
- Useful in many situations
- Print queues, message queues, etc.
- Can be implemented in several ways
- Statically (using array)
- Dynamically (using pointers)
- Using the Queue<T> class
Static Queue
- Static (array-based) implementation
- Has limited (fixed) capacity
- Implement as a "circular array"
- Has head and tail indices, pointing to the head and the tail of the cyclic queue
Linked Queue
- Dynamic (pointer-based) implementation
- Each item has 2 fields: value and next
- Dynamically create and delete objects
Queue<T> Class
- Implements the queue data structure using a circular resizable array
- Elements are from the same type T
- T can be any type, e.g. Queue<int>
- Size is dynamically increased as needed
- Basic functionality:
- Enqueue(T) - adds an element to the end of the queue
- Dequeue() - removes and returns the element at the beginning of the queue
Quizlet
- Does LinkedList<T> have Add method?
- Pop() is a method of .... and returns item/ does not return anything
- Peek() is a method of ... and returns item/ does not return anything
- List<T> can/ can't be indexed
- Which structure has insert method?
- Difference between Enqueue() and Dequeue ()
- Complexity of Enqueue () and Dequeue ()
- Complexity of Pop() and Push()
Questions
[C# DSA] Linear Data Structures
By telerikacademy
[C# DSA] Linear Data Structures
- 1,357