Recursion [click]
Telerik Academy Alpha
DSA
Table of contents
What is Recursion?
- Function that calls itself
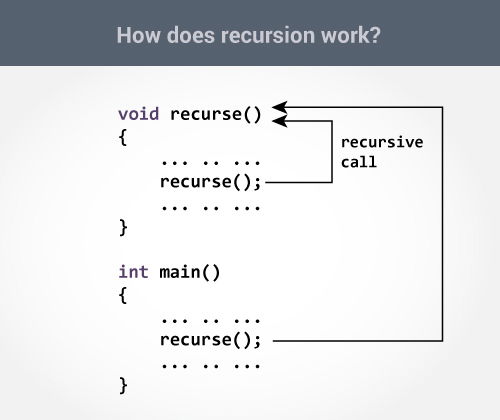
What is Recursion?
- Recursion is when a methods calls itself
- Very powerful technique for implementing algorithms
- Recursion should have
-
Exit criteria (bottom)
- Prevents infinite recursion
- Direct or indirect recursive call
- Оr through other methods
- The method calls itself directly
-
Exit criteria (bottom)
Why Recursion?
-
Used in algorithms
-
Merge Sort
-
Binary Search
-
Typical for various operations related to Trees and other non-linear data structures
-
OS Folder structure traversal
-
HTML/DOM trees and other “Visual Trees” – WPF, WinForms
-
-
Why Recursion?
-
Used in algorithms
-
DFS – graphs, also trees
-
Math - Factorial, Fibonacci, Combinatorial problems
-
-
Develops analytical thinking
-
High chance to be asked on interviews!
Examples
Example: Factorial
- Recursive definition of n! (n factorial):
\( n! = n * (n-1)! \) for n > 0
\( n! = 1 \) for n = 0
\( 5! = 5 * 4! = 5 * 4 * 3 * 2 * 1 * 1 = 120 \)
\( 4! = 4 * 3! = 4 * 3 * 2 * 1 *1 = 24 \)
\( 3! = 3 * 2! = 3 * 2 * 1 * 1 = 6 \)
\( 2! = 2 * 1! = 2 * 1 * 1 = 2 \)
\( 1! = 1 * 0! = 1 * 1 = 1 \)
\( 0! = 1 \)
Example: Factorial
- Recursive definition of n! (n factorial):
- Don't try this at home!
- Use iteration instead
- Don't try this at home!
static decimal Factorial(decimal num)
{
if (num == 0)
{
return 1;
}
var result = num * Factorial(num - 1);
return result;
}
Where is the bottom?
Demo: Factorial
Explain: Factorial
- Diagram of recursive factorial function
- every time we call it the function is executed with different parameters
- when the bottom is hit (n<=1) it returns the stored values back
Where is the bottom?

Example: String Reverse
Where is the bottom?
- Debug the example in Visual Studio to understand how it is working
Example: String Reverse
Where is the bottom?
- You are already familiar with Binary Search
- Now we need a volunteer to write pseudo-code Binary Search with the help of the Mentor

Backtracking
Backtracking?
Solving Computational Problems by Generating All Candidates
- What is backtracking?
- Backtracking is a class of algorithms for finding all solutions to some computational problem
- E.g. find all paths from Sofia to Varna
- How does backtracking work?
- Usually implemented recursively
- At each step we try all perspective possibilities to generate a solution
- Backtracking has exponential running time!
Backtracking?
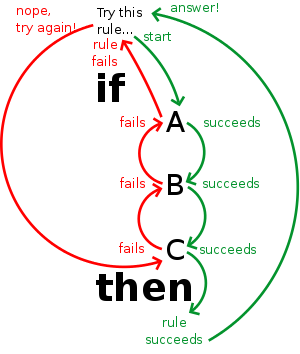
Path finder - demo
Recursion vs Iteration
Recursion is harmful?
- When used incorrectly the recursion could take too much memory and computing power
- Example:
Recursion is harmful?
- The same value is calculated many, many times!
Recursion done right
- Each Fibonacci sequence member can be remembered once it is calculated (memoization)
Can be returned directly when needed again- Example:
When to use recursion?
-
Avoid recursion when an obvious iterative algorithm exists
- Examples: factorial, Fibonacci numbers
- Use recursion for combinatorial algorithm where at each step you need to recursively explore more than one possible continuation
- Examples: permutations, all paths in labyrinth
- If you have only one recursive call in the body of a recursive method, it can directly become iterative (like calculating factorial)
Questions?
[C# DSA] Recursion
By telerikacademy
[C# DSA] Recursion
- 1,549