JS DOM / AJAX
Telerik Academy Alpha
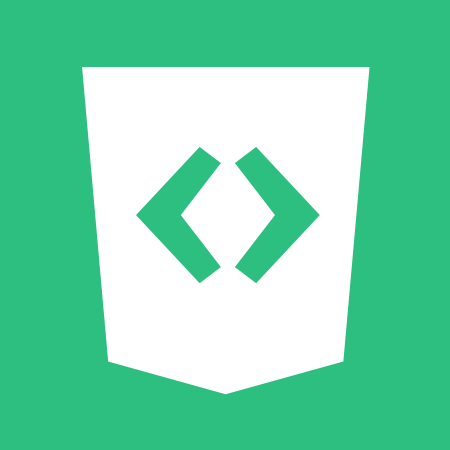
Front-End
Table of contents
Document Object Model
The DOM Tree
-
The Document Object Model is an API for HTML and XML documents
-
Provides a structural representation of the document
-
Developers can modify the content and UI of a web page
-
The DOM Tree
-
The DOM consists of objects to manipulate a web page
-
All the properties, methods and events are organized into objects
-
Those objects are accessible through programming languages and scripts
-
-
How to use the DOM of an HTML page?
-
Write JavaScript to interact with the DOM
-
JavaScript uses the DOM API (native implementation for each browser)
-
-
The DOM API
-
The DOM API consists of objects and methods to interact with the HTML page
-
Can add or remove HTML elements
-
Can apply styles dynamically
-
Can add and remove HTML attributes
-
-
DOM introduces objects that represent HTML elements and their properties
-
document.documentElement is <html>
-
document.body is the body of the page, where the content of the page is
-
DOM Objects
-
Each of the HTML elements has corresponding DOM object type
-
HTMLLIElement represents <li>
-
HTMLAudioElement represents <audio>
-
-
Each of these objects has the appropriate properties
-
HTMLAnchorElement has href property
-
HTMLImageElement has src property
-
-
The document object is a special object
It represents the entry point for the DOM API
Selecting DOM Elements
-
HTML elements can be found and cached into variables using the DOM API
var header = document.getElementById('header');
var nav = document.querySelector('#main-nav');
-
Select single element
-
Select a collection of elements
var inputs = document.getElementsByTagName('li');
var radiosGroup = document.getElementsByName('genders');
var header = document.querySelectorAll('#main-nav li');
-
Select a collection of elements
var links = document.links;
var forms = document.forms;
NodeLists
-
A NodeList is a collection returned by the DOM selector methods:
-
getElementsByTagName(tagName)
-
getElementsByName(name)
-
getElementsByClassName(className)
-
querySelectorAll(selector)
-
var divs = document.getElementsByTagName('div'),
queryDivs = document.querySelectorAll('div');
for(var i = 0, length = divs.length; i < length; i += 1){
// do stuff with divs[i]…
}
NodeLists
-
NodeList looks like an array, but is not
-
It's an object with properties similar to array
-
Has length and indexer
-
-
-
Traversing an array with for-in loop works unexpected:
console.log(Array.isArray(divs)); // false
for (var i in divs) {
console.log('divs[' + i + '] = ' + divs[i]);
}
jQuery
What is jQuery?
-
jQuery is a cross-browser JavaScript library
-
Designed to simplify the client-side scripting of HTML
-
The most popular JavaScript library in use today
-
Free, open source software
-
-
jQuery's syntax is designed to make it easier to
-
Navigate a document and select DOM elements
-
Create animations
-
Handle events
-
Why use jQuery?
-
Easy to learn
-
Fluent programming style
-
-
Easy to extend
-
You create new jQuery plugins by creating newJavaScript functions
-
-
Powerful DOM Selection
-
Powered by CSS 3.0
-
-
Lightweight
-
Community Support
-
Large community of developers and geeks
-
Installing jQuery
-
Download jQuery files from jquery.com
-
Self hosted
-
You can choose to self host the .js file
-
E.g. jquery-3.1.0.js or .min.js file
-
-
Use it from CDN (content delivery network)
-
Microsoft, jQuery, Google CDNs
-
e.g. code.jquery.com/jquery-3.1.0.min.js
-
ajax.microsoft.com/ajax/jquery/jquery-3.1.0.min.js
-
-
Selectors
-
Selection of DOM elements in jQuery is much like as in pure JavaScript
-
Selection of elements using CSS selectors
-
Like querySelectorAll
-
//by tag
$("div") //document.querySelectorAll("div");
//by class
$(".menu-item"); //document.querySelectorAll(".menu-item");
//by id
$("#navigation");
//by combination of selectors
$("ul.menu li");
Selectors
-
Selecting items with jQuery
-
Almost always returns a collection of the items
-
Even if there is only one item
-
-
Can be stored in a variable or used right away
-
The usage of the elements is always the same, nomatter whether a single or many elements
-
$(".widgets").fade(1);
let something = $("#something");
something.hide();
DOM Traversal
-
$.next(), $.prev(), $.parent(), $.parents(selector)
-
Returns the next/prev sibling
-
Returns an HTML element
-
Not a [text] node
-
-
var $firstLi = $("li").first();
log($firstLi); //logs "Item 1"
log($firstLi.next()); //logs "Item 2"
<ul>
<li>Item 1</li>
<li>Item 2</li>
</ul>
jQuery Objects
-
Selected with jQuery DOM elements are NOT pure DOM elements
-
They are extended
-
Have additional properties and methods
-
addClass(), removeClass(), toggleClass()
-
on(event, callback) for attaching events
-
animate(), fade(), etc…
-
-
//Parsing a regular DOM element to jQuery Element
var content = document.createElement("div");
var $content = $(content);
jQuery Object Properties
-
jQuery elements extend regular DOM elements
-
Methods for altering the elements
-
$.css("color", "#f3f")
-
$.html() returns the innerHTML
-
$.html(content) sets the innerHTML
-
-
$.text(content) sets the innerHTML, by escaping the content
-
jQuery Object Properties
-
$(document).ready(callback) calls the passed function once the DOM tree is fully loaded
// A $(document).ready() block.
$(document).ready(function() {
console.log("ready!"); // Code to run when the document is ready.
});
-
Shorthands:
function readyFn( jQuery ) {
console.log("ready!");
}
$(document).ready(readyFn);
$(window).on("load", readyFn);
$(readyFn);
jQuery Events
-
jQuery has a convenient way for attaching and detaching events
-
Works cross-browser
-
Using methods on() and off()
-
function onButtonClick(){
$(".selected").removeClass("selected");
$(this).addClass("selected");
}
$("a.button").on("click", onButtonClick);
AJAX
What is AJAX?
-
AJAX is acronym of Asynchronous JavaScript and XML
-
Technique for background loading of dynamic content and data from the server side
-
Allows dynamic client-side changes
-
-
Two types of AJAX
-
Partial page rendering - loading of HTML fragment and showing it in a <div>
-
JSON service - loading JSON object and client-side processing it with JavaScript / jQuery
-
What is AJAX?
-
Technically, AJAX is a group of technologies working together
-
HTML & CSS for presentation
-
The DOM for data display & interaction
-
XML (or JSON) for data interchange
-
XMLHttpRequest for async communication
-
JavaScript to use the above
-
AJAX Requests
-
AJAX uses HTTP
-
Requests have headers - GET, POST, HEAD, etc.
-
Requests have bodies - XML, JSON or plain text
-
The request must target a resource with a URI
-
The resource must understand the request
-
Server-side logic
-
-
Requests get a HTTP Response
-
Header with a body
-
-
AJAX jQuery
-
jQuery provides some methods for AJAX
-
$.ajax(options) – HTTP request with full control (headers, data, method, etc…)
-
$.get(url) – HTTP GET request
-
$.post(url) – HTTP POST request
-
$(selector).load(url) – loads the contents from the url inside the selected node
-
jQuery AJAX
$.get('users.all')
.then(function(usersString) {
return JSON.parse(usersString);
})
.then(function(users) {
myController.users = users;
})
.catch(fucntion(error) {
console.log(error)
}
Promises
-
A promise is an object which represents an eventual (future) value
-
Methods "promise" they will return a value
-
Correct or representing an error
-
-
A Promises can be in one of three states:
-
Fulfilled (resolved, succeeded)
-
Rejected (an error happened)
-
Pending (unfulfilled yet, still being computed)
-
Promises
-
Promise objects can be used in code as if their value is known
-
Actually we attach code which executes
-
When the promise is fulfilled
-
When the promise is rejected
-
When the promise reports progress (optionally)
-
-
Promises are a pattern
-
No defined implementation, but strict requirements
-
Initially described in CommonJS Promises/A
-
Promises
-
More specifically:
-
Each promise has a .then() method accepting 3 params:
-
Success, Error and Progress functions
-
-
All parameters are optional
-
-
So we can write:
promiseMeSomething()
.then(function (value) {
//handle success here
}, function (reason) {
//handle error here
});
// Note: Provided promiseMeSomething returns a promise
Promises
-
Each .then() method returns a promise in turn
-
Meaning promises can be chained
-
-
Promises enable us to:
-
Remove the callback functions from the parameters and attach them to the "result"
-
Make a sequence of operations happen
-
Catch errors when we can process them
-
asyncComputeTheAnswerToEverything()
.then(addTwo)
.then(printResult, onError);
Promises
let promise = new Promise((resolve, reject) => {
setTimeout(() => {
resolve(1000);
}, 1500)
} )
promise
.then((value) => {
console.log("Success " + value);
})
.catch((error) => {
console.log("Error " + error);
})
Promise Methods
-
Promise.all(iterable)
-
Wait until all settled
-
-
Promise.race(iterable)
-
Wait until 1 settles
-
-
Promise.reject(reason)
-
Create a Promise that is already rejected
-
Useful to not do async operation in some condition
-
-
Promise.resolve(value)
-
Create a promise that is already resolved
-
Promise Methods - Example
let usersPromise = get('users.all');
let postsPromise = get('posts.everyone');
// Wait until BOTH are settled
Promise.all([usersPromise, postsPromise])
.then((results) => {
// results is an array of the responses
myController.users = results[0];
myController.posts = results[1];
})
.catch(() => {
delete myController.users;
delete myController.posts;
})
Questions?
[FE] JS DOM / AJAX
By telerikacademy
[FE] JS DOM / AJAX
- 1,092