Understanding "React Hooks" by building hooks



Yatharth K
Frontend Architect, PushOwl

yatharthkhatri
Take-aways from talk
Understanding of
- React Reconciler
- React Fiber
- How hooks work internally
Why did I attempt to learn how hooks work?
I hate (unravelled) mysteries
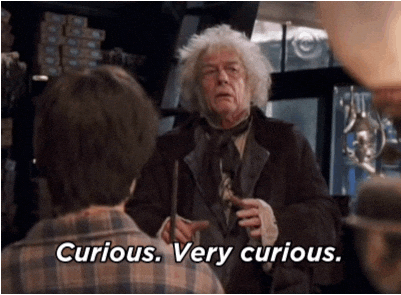
DISCLAIMER
Everything you witness from now on is OVERSIMPLIFIED version of actual concepts for your own good and for EDUCATIONAL purpose only. 😝
React Reconciler
React Reconciler is the smart diffing algorithm that helps React figure out which DOM elements to update on state change.
React Fiber
Literally, in simplest terms, React Fiber is just an object that holds all the information of a component.
Before we move to hooks...
Understand that React library has two parts:
- Core - "react" module for public facing API and common logic like Reconciler, Fiber, et al
- Renderers - "react-dom", "react-native", "react-art" modules for platform specific rendering
Since hooks are closely related to and dependent on core i.e. reconciler, fiber, .., they go into "react" module
Understanding render flow
Consider this
ReactDOM.render(
<App />,
document.querySelector('#root'),
)
Understanding render flow
ReactDOM
Reconciler
Render <App /> component
Reconciler
Fiber
Create and give me a Fiber for <App />
<App />
<App />
Reconciler
Component
Component Fiber
<App />
props,
memoizedState,
sibling,
child,
ref, ...
Understanding render flow
Render Class Component
Render with Hooks
component is Class or Function?
Reconciler
(Class Component)
(Functional Component)
Understanding render flow
Consider our <App /> component to be:
function App() {
const [count, setCount] = useState(0);
const [name, setName] = useState('Anonymous');
useEffect(() => {
...
})
return (...)
}
Number of hooks:
useState - 2
useEffect - 1
Understanding render flow
Rendering with hooks (Mounting)
- <App /> gets called (being a function)
- First useState gets called.
Reconciler asks Fiber if the hook is registered there. If not, it asks to register it and give a dispatcher to update state i.e. setState.
- Second useState gets called.
Fiber is again asked to register this hook if not already and return a dispatcher.
- The useEffect hook is registered to Fiber.
- The mounting is completed.
- Callback from useEffect hook is executed.
Understanding render flow
Rendering with hooks (Updating)
- <App /> gets called (being a function)
- First useState gets called.
Reconciler asks Fiber if the hook is registered there. If yes, return the saved state and the dispatcher to update state i.e. setState.
- Second useState gets called.
Fiber is again asked to return the saved state and corresponding dispatcher for second hook.
- The useEffect hook gets called and is updated in Fiber.
- As the component gets rendered/updated, callback from useEffect hook is executed.
Getting it so far?
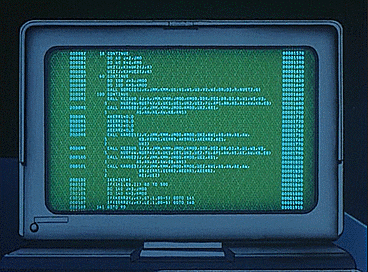
Let's code Hooks!
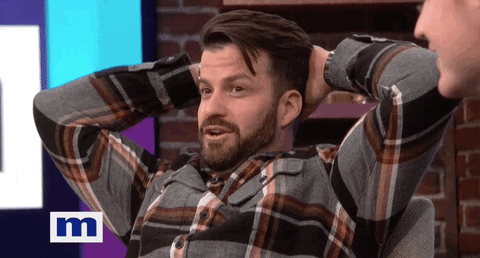
And we just built HOOKS
I hope you learned something good today

yatharthkhatri
Any Questions?

yatharthkhatri
Understanding React Hooks, by building Hooks
By Yatharth K
Understanding React Hooks, by building Hooks
- 141