Introducing Vue.js
Thorsten Lünborg
github.com/linusborg // @linus_borg

whoami

- Vue core team member since ~06/2016
- "That guy on the forum" answering your questions
- Programming is not my job, but my passion
- Twitter: @linus_borg
- Github: @linusborg
So what's Vue.js?
Vue (pronounced /vjuː/, like view) is a progressive framework for building user interfaces.
Vue is focused on the view layer only and it can be used as a library in any project. At the same time, it can be a full-blown framework, perfectly capable of building complex Single Page Applications.
The beginning...

- Started by Evan You in 2013 as a side project
- 0.11 November 2014
- 1.0 Oktober 2015
- 2.0 Oktober 2016
93,737 GitHub Stars
Top 10 All-Time
2nd most-starred JavaScript framework
>20 Core Team members,
many contributors

Today
Let's see some code!
enough marketing...
Vue code is declarative
Opposed to imperative code that we would write with jQuery and similar tools
List Rendering
Imperatively, with jQuery
const items = [
'thingie',
'another thingie',
'lots of stuff',
'yadda yadda'
];
function listOfStuff() {
let full_list = '';
for (let i = 0; i < items.length; i++) {
full_list = full_list + `<li> ${items[i]} </li>`
}
const contain = document.querySelector('#container');
contain.innerHTML = `<ul> ${full_list} </ul>`;
}
listOfStuff();
List Rendering
declaratively, with Vue
<div id="app">
<ul>
<li v-for="item in items">
{{ item }}
</li>
</ul>
</div>
<script>
new Vue({
el: '#app',
data: {
items: [
'thingie',
'another thingie',
'lots of stuff',
'yadda yadda'
]
}
});
</script>
Data Binding
<div id="app">
<h3>Type here:</h3>
<textarea v-model="message"></textarea><br>
<p class="booktext">{{ message }} </p>
</div>
<script>
new Vue({
el: '#app',
data() {
return {
message: 'This is a good place to type things.'
}
}
});
</script>
Computed Properties
<div id="app">
<h3>Type here:</h3>
<input v-model="firstName"></input><br>
<input v-model="lastName"></input><br>
<p class="booktext">{{ fullName }} </p>
</div>
<script>
new Vue({
el: '#app',
data() {
return {
firstName: 'Tom',
lastName: 'Thompson',
}
},
computed: {
fullName() {
return `${this.firstName} ${this.lastName}`
}
}
});
</script>
Conditional Rendering
<div id="app">
<h3>Type here:</h3>
<p v-if="showParagraph">
The visibility of this paragraph can be toggled
With the button below
</p>
<button v-on:click="toggle">
Toggle
</button>
</div>
<script>
new Vue({
el: '#app',
data() {
return {
showParagraph: true
}
},
methods: {
toggle() {
this.showParagraph = !this.showParagraph
}
}
});
</script>
Events & Methods
<div id="app">
<h3>Type here:</h3>
<p v-if="showParagraph">
The visibility of this paragraph can be toggled
With the button below
</p>
<button v-on:click="toggle">
Toggle
</button>
</div>
<script>
new Vue({
el: '#app',
data() {
return {
showParagraph: true
}
},
methods: {
toggle() {
this.showParagraph = !this.showParagraph
}
}
});
</script>
Components
Composing your app with components
<header></header>
<aside>
<sidebar-item v-for="item in items"></sidebar-item>
</aside>
<main>
<blogpost v-for="post in posts"></blogpost>
</main>
<footer></footer>
<div id="app">
<child v-bind:text="message"></child>
</div>
<script>
Vue.component('child', {
props: ['text'],
template: `<div>{{ text }}<div>`
});
new Vue({
el: "#app",
data() {
return {
message: 'hello mr. magoo'
}
}
});
</script>
Defining a component
Passing down data with Props
<div id="app">
<child v-on:clicked="handleClick"></child>
</div>
<script>
Vue.component('child', {
props: ['text'],
template: `<button v-on:click="$emit('clicked!')">Click me!</button>`
});
new Vue({
el: "#app",
method: {
handleClick() {
//
}
}
});
</script>
Emmitting Events
Props down
Events up
<div id="app">
<child>
<p>
This is the
<strong>Slot Content</strong>
</p>
</child>
</div>
<script>
Vue.component('child', {
template: `<div> <slot /> <div>`
});
new Vue({
el: "#app",
});
</script>
Nesting Content with Slots
<!-- Result -->
<div id="app">
<div class="child">
<p>
This is the
<strong>Slot Content</strong>
</p>
</div>
</div>
"A nice little toy"
"looks a little like angular, yikes!"
"Can I build applications with this?"
Yes we can!


"The Progressive Framework"
- Routing: vue-router
- State Management: vuex
- Developer Tooling: vue-devtools
- VS Code Plugin vetur
- CLI: @vue/cli
- Bundlers: vue-loader
- rollup/plugin-vue
- Testing: @vue/test-utils
v3 in beta!
v1 in beta!
Single-File Components
(SFCs)
<template>
<div class="red">{{ text }}<div>
</template>
<script>
export default {
props: ['text'],
methods: {
// ..
}
}
</script>
<style>
.red {
color: red;
}
</style>
What's an SFC?
- File Extension: `.vue`
- Contains
- Markup
- Code
- styling
- Supported by all major bundlers
BUT.....
SEPARATION OF CONCERNS!?!
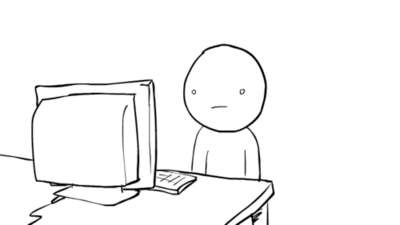
What many call "seperation of concerns"
We call: "Seperation of technologies".
Your component is made up of Markup, code, and styling, and you rarely find yourself concerned about only one.
So your concern is mostly about all three, they belong together
Questions?
now, or visit me at the forum later:
Indroducing Vue.js
By Thorsten Lünborg
Indroducing Vue.js
as presented at Frontend Rhein-Neckar, 29.05.2018
- 993