Android App Architecture
TimNew
Android 4 Major Components
-
Application
-
Activity
-
Fragment
-
Service
Application
- Root of an app
- Root of Context
- Available across the whole app lifecycle
- Home of "singleton" objects
Service
- Task to be done without UI
- Providing support to UI
- Off load to background *
- Android 8+ Background Limit
- Scheduler Service
- Providing Support to other App or System
- Encryption?!
- Video/Audio Streaming
Activity
- The most important component on Android
- The "screen"
- Root of the UI hierarchy
- Context with UI capability
- The basic component for an application
- The host of fragment
- Has Lifecycle
- Only run on Main Thread
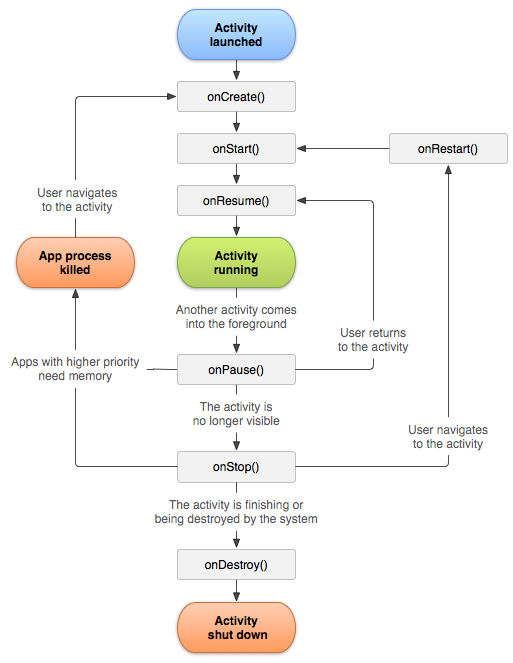
Fragment
- A "Reusable" UI component?
- A "fragment" of the activity?
- A group of widgets?
- Only run Main Thread
- Has its own lifecycle?!!!!
- The source of the crashes?!!


Common issues in Android architecture
God-like Activity/Fragment
- Rendering UI
- Handling UI components
- Navigation
- Calling API
- Calling Database/Storage
- Initiate Navigation
- Handling Lifecycle callbacks
- Handling Permissions
- Handling input from other UI
- ......
Activity/Fragment Responsibility
Classes with
thousands of lines of code
hundreds of methods
Split the code into more dedicated components
Complications - Context
- Almost everything you do need a context
- Render UI
- Loading Image
- Loading Text
- Navigation
- Access Network
- Access System Resource/Service
- Context Leaking
- Activity is a Context, but leaking Resource/Memory
- Application is a Context, but cannot handle UI
- Context has lifecycle
- Context might not be available
- Context might be disposed
- Lifecycle is the source of crashes!
Complications - Lifecycle
-
Theading
-
Accessing Network/Storage on MainThread crashes the app
-
Accessing UI from background thread crashes the app
-
-
Lifecycle
-
Accessing UI before it is created crashes the app
-
Accessing UI after it is disposed crashes the app
-
UI recreation due to configuration change
-
Accessing a disposed context crashes the app
-
Reality is complicated
- User clicks search button
- UI trigger click callback (MainThread)
- Calling API (Worker Thread)
- Update the UI to render loading indicator (MainThread)
- The API returned or timeout (Worker Thread)
- Update the UI with result or error (Main Thread)
- Save result to Database (Worker Thread)
- What if user get a phone call after 4 before 5?
- What if it is upload a big file, which is expected to take longer time to finish?
- What if user clicks button again before 6 (If we didn't disable the button)
- What if user rotated phone after 4 before 5
Solutions from Google
A history of tears for Android developers
An concrete example
- No reusable UI component (with logic)
- Fragment
- Fragment lifecycle management is error-proning
-
LifecyleOwner, LifeCycle, LifecycleObserver
- Initialized, Created, Started, Resumed, Destroyed
- Fragment can survive without a view!
- Fragment.getViewLifecycleOwner
- Calling getViewLifecycleOwner before view is created crashes the app (??!!!)
- Fragment might have more than one ViewLifecycleOwner (????!!!!!!!!)
- Fragment.getViewLifecycleOwnerLiveData
- viewLifecycleOwnerLiveData.observe { owner.lifecycle.observe { } }
don't know
how to do
Android Architecture
right
To G or not to G
that is the question
Clean Achitecture
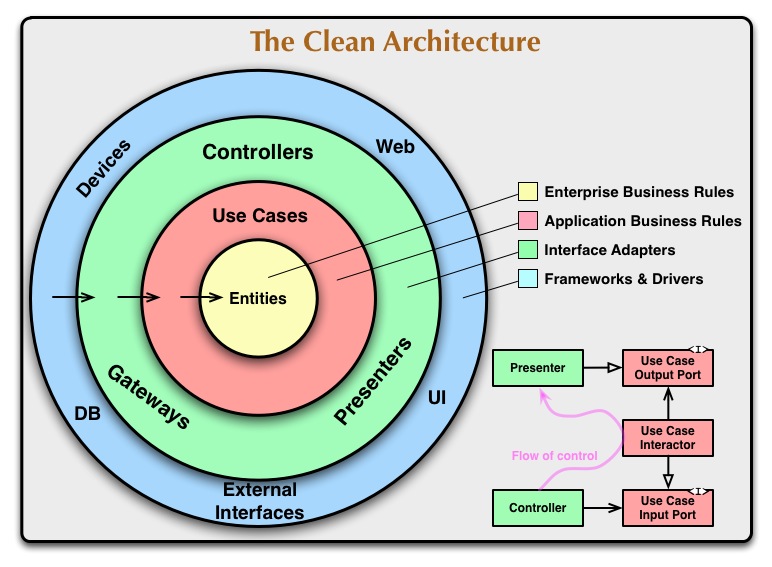
Clean Architecture
- It is a PHILOSOPHY
- It is A way to abstract software
- It is NOT a library
- It is a PRACTICE / GUIDELINE
- It ADAPTS to different domain/environment
Our Clean Architecture
Title Text
Android App Architecture TimNew
Android-App-Architecture
By timnew
Android-App-Architecture
- 96