FOR EVERYONE
A simple introduction to the Node.Js envirnoment with a list of useful tool and resources.
- Node.js is a JavaScript runtime built on Chrome's V8 JavaScript engine. Node.js uses an event-driven, non-blocking I/O model that makes it lightweight and efficient.
- Node.js is designed to build scalable network applications.
ABOUT
...from nodejs.org
var http = require('http');
http.createServer(function (req, res) {
res.writeHead(200, {'Content-Type': 'text/plain'});
res.end('Hello World\n');
}).listen(1337, "127.0.0.1");
console.log('Server running at http://127.0.0.1:1337/');
In the following "hello world" example, many connections can be handled concurrently. Upon each connection the callback is fired, but if there is no work to be done Node is sleeping.
ABOUT

EVENT LOOP
ABOUT
- npm is the package manager for Node.js. It was created in 2009 as an open source project to help JavaScript developers easily share packaged modules of code.
- The npm Registry is a public collection of packages of open-source code for Node.js, front-end web apps, mobile apps, robots, routers, and countless other needs of the JavaScript community.
- npm is the command line client that allows developers to install and publish those packages.
- npm is often used by developers and IT people whom don't use (program with) Node.js at all.
...from npmjs.com
INSTALL
- For Windows and OS X the best way to install Node.js is to use one of the installers from nodejs.org.
- If you're using Linux the best way is to check and follow the instructions from NodeSource's binary distributions to install Node.js via package manager.
# For example Using Debian, as root
$ curl -sL https://deb.nodesource.com/setup_0.12 | bash -
$ apt-get install -y nodejs
$ sudo npm install npm -g
# Now run
$ npm -v.
# The version should be higher than 2.1.8.
Node comes with npm installed so you should have a version of npm. However, npm gets updated more frequently than Node does, so you'll want to make sure it's the latest version
INSTALL
# To install a global package you can use:
$ npm install --global <package name>
$ npm install -g <package name>
# If you have permission problem try with sudo:
$ sudo npm install -g <package name>
$ sudo npm install --global <package name>
- Some packages are real command line, multi platform and standalone applications.
- npm install and make them available as a command
Command-line apps
- For example pagers capture screenshots of websites in various resolutions.
# install the pageres command line tool
$ npm install --global pageres-cli
# the tool now is magically available as a command
$ pageres google.it 1024x768
$ npm install cfonts -g
- Another awesome tool is cfonts
Command-line apps

$ npm install asciify -g
- What about asciify ?
Command-line apps

$ npm install chalk
- Packages can be installed locally, to be used in your projects.
local packages
- This will create the node_modules directory in your current directory (if it doesn't exist yet), and will download the package to that directory.
- To use the installed package require it on your script.
// ~/myscript/index.js
var chalk = require('chalk');
// style a string
var st = chalk.blue('Hello world!');
console.log(st);

- Another way to manage npm packages locally is to create a package.json file.
- You can create it manually or by using
- and following the prompts (more info here)
local packages
- Now you can use the --save flag
- This will download the package as before, but will also add it to the dependencies in package.json
- If you have a package.json file in your directory and you run npm install, then npm will look at all the dependencies and download them in node_modules (more info here).
- This is nice because it makes your build reproducible, which means that you can share it with other developers.
$ npm init
$ npm install chalk --save
PACKAGE.JSON EXAMPLE
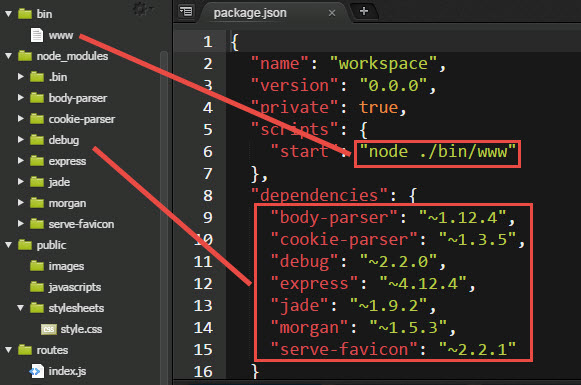
- Some packages can be used both as command line apps or local packages to use in your projects
-
is-up is one of them: it checks whether a website is up or down
- CLI
COMMAND-LINE APPS & local packages
$ npm install --global is-up
$ is-up --help
Example
is-up sindresorhus.com
✔︎ Up
-
- API
$ npm install --save is-up
var isUp = require('is-up');
isUp('sindresorhus.com', function (err, up) {
console.log(up);
//=> true
});
IT'S TIME TO WORK NOW...
LET'S EXPLORE SOME USEFUL APPS AND PACKAGES
- cross-platform zero-configuration command-line http server
- simple, and useful for testing and development
HTTP-SERVER
$ npm install http-server -g
// usage
$ http-server [path] [options]
-
a lot of options a features
- custom address (-a) and port (-p)
- directory listing (-d)
- CORS (--cors)
- enable https (--ssl) with custom certificate
Commander is a complete solution for node.js command-line programs
COMMANDER
#!/usr/bin/env node
/**
* Module dependencies.
*/
var program = require('commander');
program
.version('0.0.1')
.option('-p, --peppers', 'Add peppers')
.option('-P, --pineapple', 'Add pineapple')
.option('-b, --bbq-sauce', 'Add bbq sauce')
.option('-c, --cheese [type]', 'Add the specified type of cheese [marble]', 'marble')
.parse(process.argv);
console.log('you ordered a pizza with:');
if (program.peppers) console.log(' - peppers');
if (program.pineapple) console.log(' - pineapple');
if (program.bbqSauce) console.log(' - bbq');
console.log(' - %s cheese', program.cheese);
- Agenda is a light-weight job scheduling library for Node.js.
AGENDA
var agenda = new Agenda({db: { address: 'localhost:27017/agenda-example'}});
agenda.define('delete old users', function(job, done) {
User.remove({lastLogIn: { $lt: twoDaysAgo }}, done);
});
agenda.every('3 minutes', 'delete old users');
agenda.schedule('tomorrow at noon', 'printAnalyticsReport', {userCount: 100});
agenda.every('15 minutes', 'printAnalyticsReport');
-
node-windows
- Run scripts as a native Windows service and log to the Event viewer.
-
node-mac
- Run scripts as a native Mac daemon and log to the console app.
-
node-linux
- Run scripts as native system service and log to syslog.
PROCESS MANAGEMENT
- Nodemailer is a simple solution to send e-mails from Node.js
- Available a lot of options and plugins
- HTML, markdown, images, attachments
- Transporter: SMTP, Mandrill, SendGrid,...
NODEMAILER
var nodemailer = require('nodemailer');
var transporter = nodemailer.createTransport({
service: 'gmail',
auth: {
user: 'sender@gmail.com',
pass: 'password'
}
});
transporter.sendMail({
from: 'sender@address',
to: 'receiver@address',
subject: 'hello',
text: 'hello world!'
});
AND FOR WEB DEVELOPMENT ?

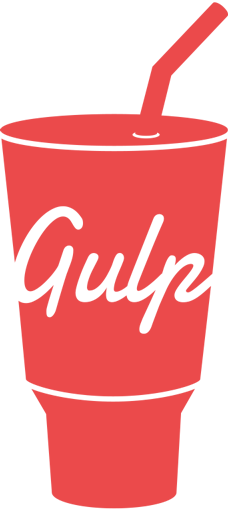


BOWER

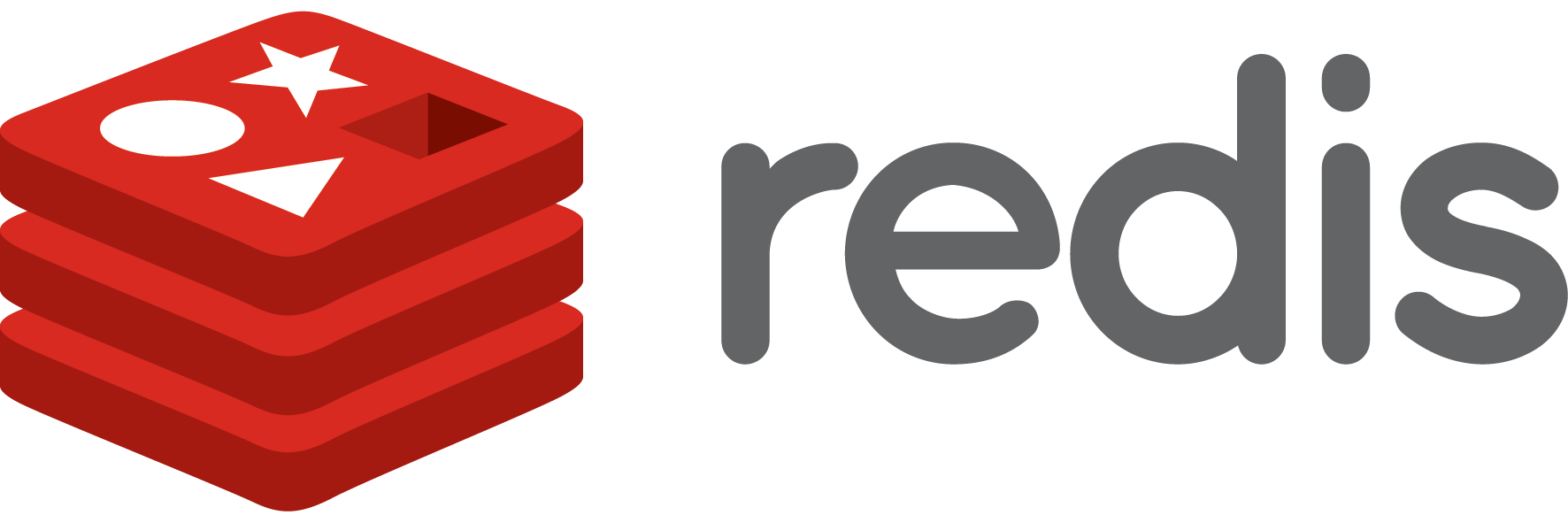

- Search through all published packages on npmjs.com
- Take a look at the node modules of the week.
- A curated list of delightful Node.js packages and resources can be found here.
- A very good list of JavaScript essential can be found here.
- NodeSchool is an awesome resource to learn how to develop with Node and JavaScript.
- Follow me for tutorials, news and updates.
WHeRE TO GO NOW ?
NodeJS & npm for Everyone
By Tarun Sharma
NodeJS & npm for Everyone
NodeJs & npm for Everyone is a simple guide for beginners that shows the power of NodeJS and npm (especially for developers and IT people). The presentation shows some features of npm and node and lists some of awesome packages and modules to use during development or other purposes.
- 480