Intro to RxJS
Tobias Kausch
@tobikabla
JS/Angular/Ionic/Cordova
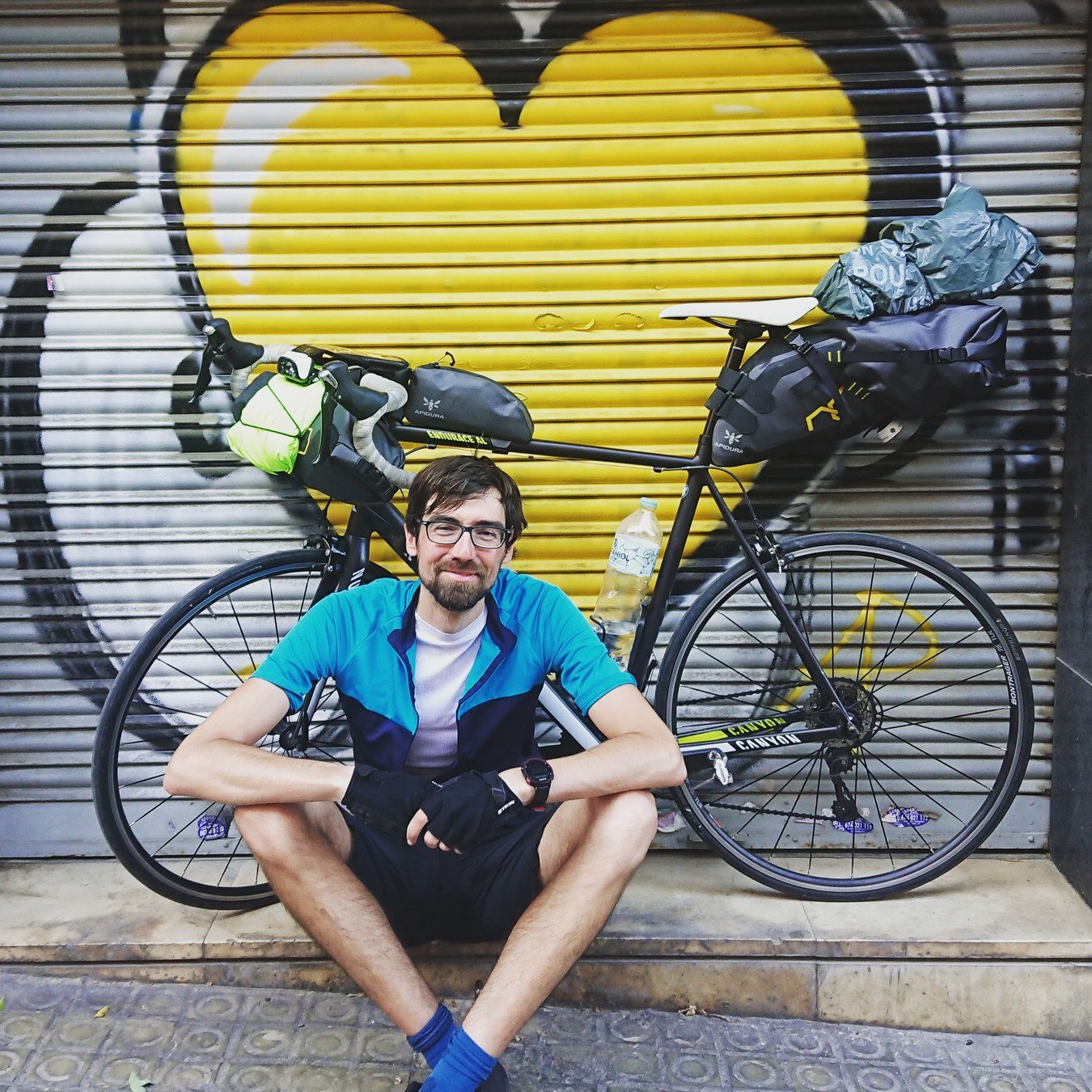
Reactive Programming
a paradigm about streaming of async data and propagation of change

Solve problems of async programming (Callbackhell)
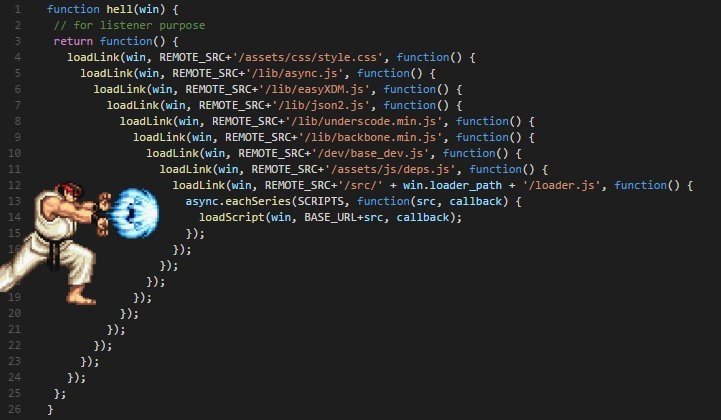
Collection of values over time
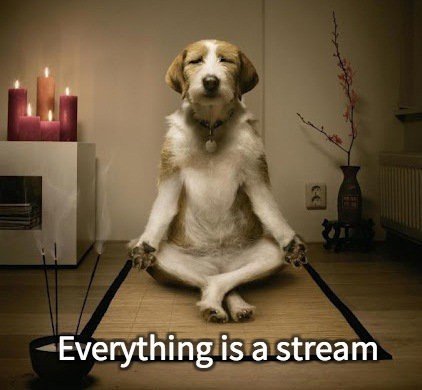
Pattern
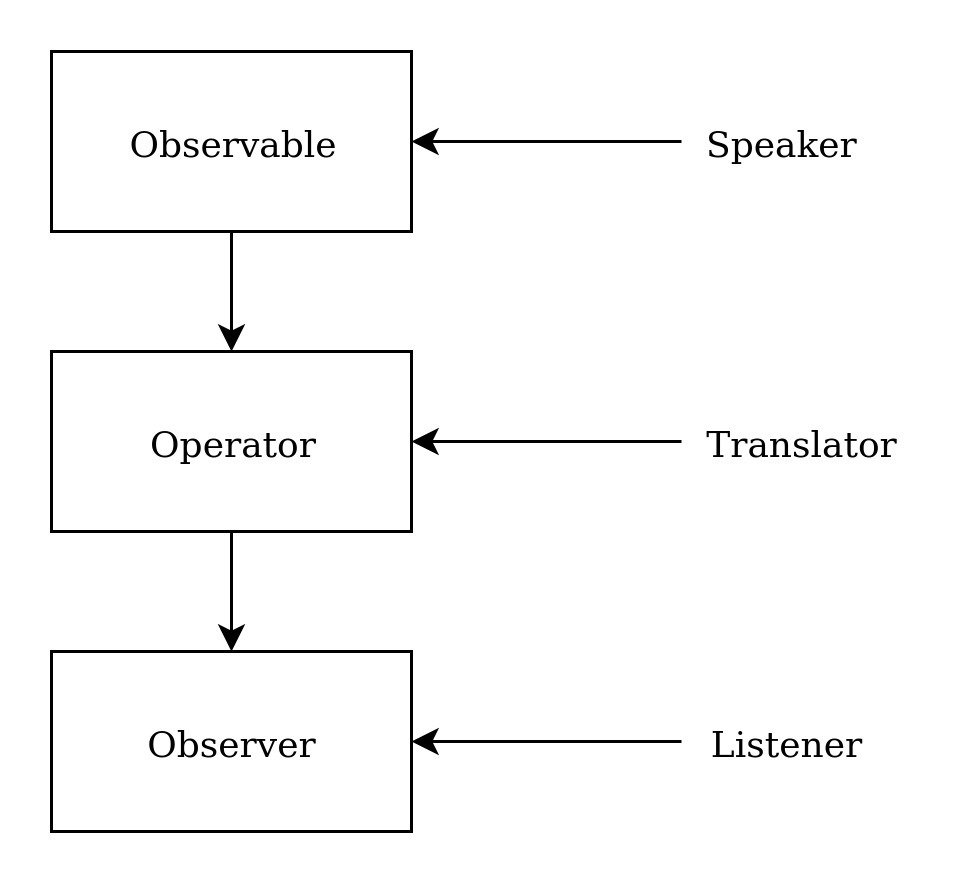
ReactiveX
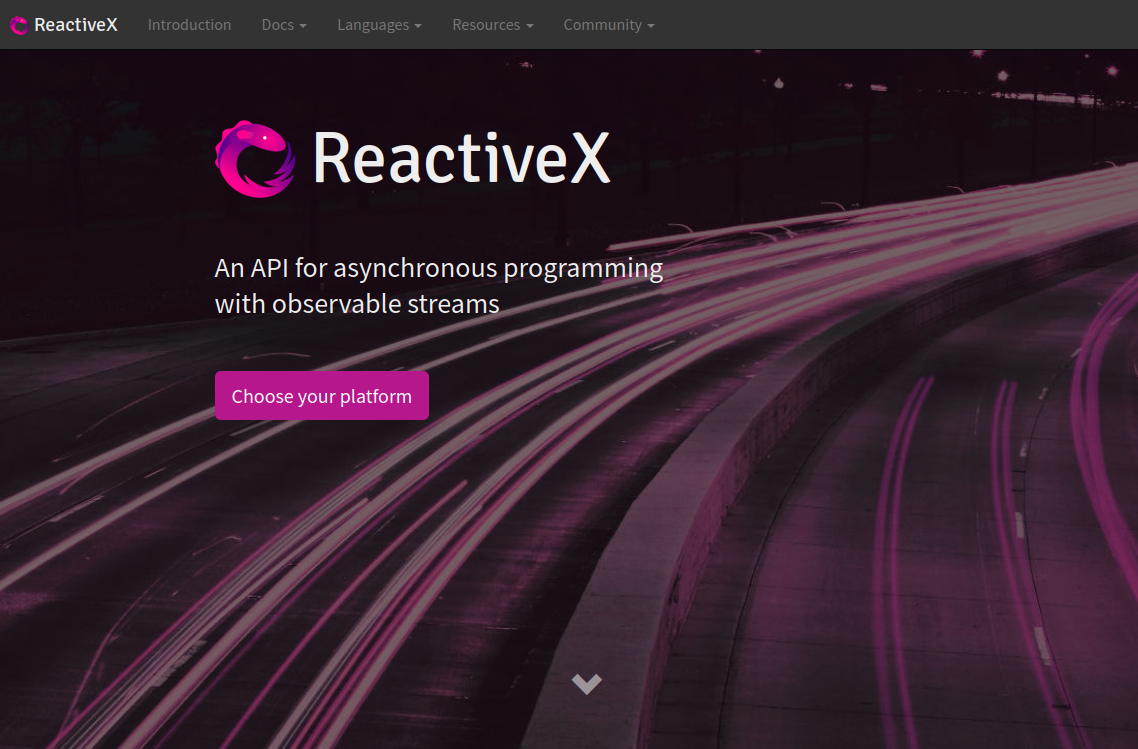
Think of RxJS as Lodash for events.
Promise vs Observable
- Observable is a collection of any number of things over any amount of time
- not lazy vs lazy
- able to cancel
Observable creation
- DOM Events
- AJAX/Http calls
- Web sockets
const observable$ = from([1, 2, 3, 4, 5]);
//output: 1,2,3,4,5
const observable$ = fromEvent(document, 'click');
const observable$ = interval(1000);
//output: 0,1,2,3,4,5....
Helper Functions
Operators
- map/filter/...
- like for arrays
- custom operators
//emit (1,2,3,4,5)
const source$ = from([1, 2, 3, 4, 5]);
//add 10 to each value
const example$ = source$.pipe(map(val => val + 10));
//output: 11,12,13,14,15
const subscribe = example$.subscribe(val => console.log(val));
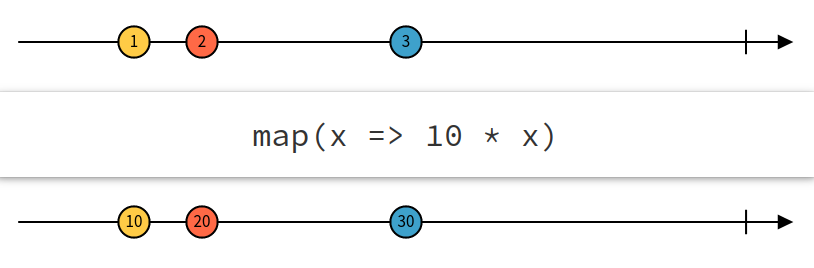
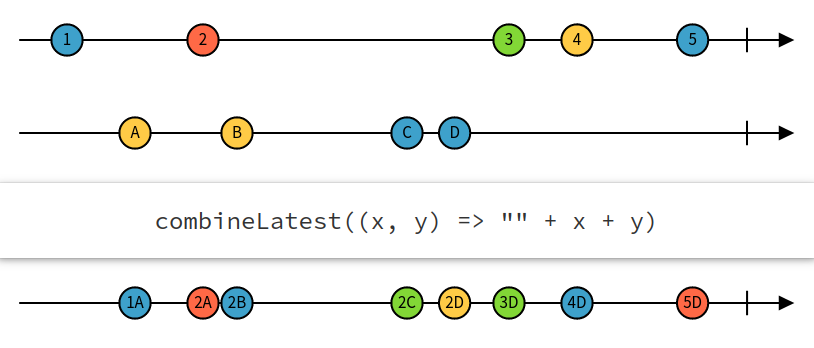
Observables in Angular
- Routing
- Reactive Forms
- Http calls
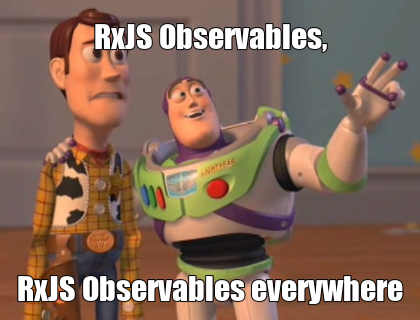
Example
Let's dive into the code
Subjects
- special type of Observable
- Multicast
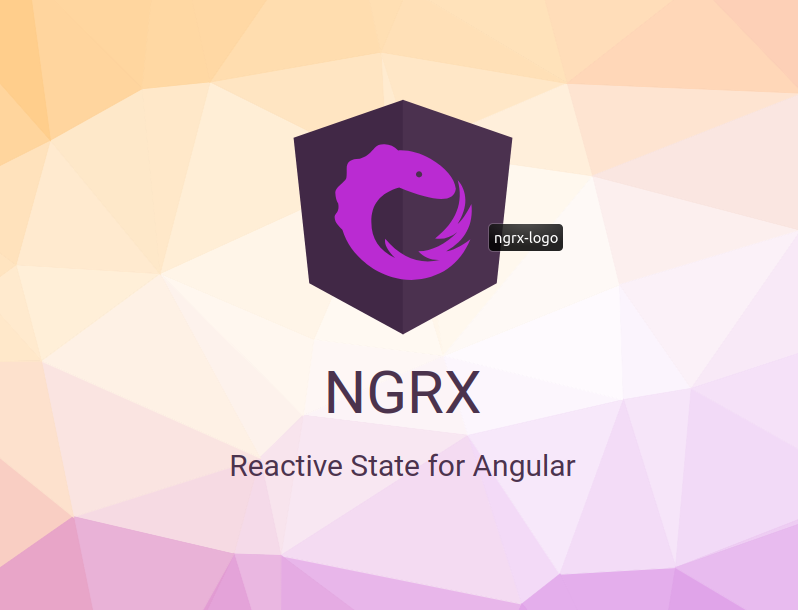
Resources
- learnrxjs.io
- rxmarbles.com
- rxviz.com
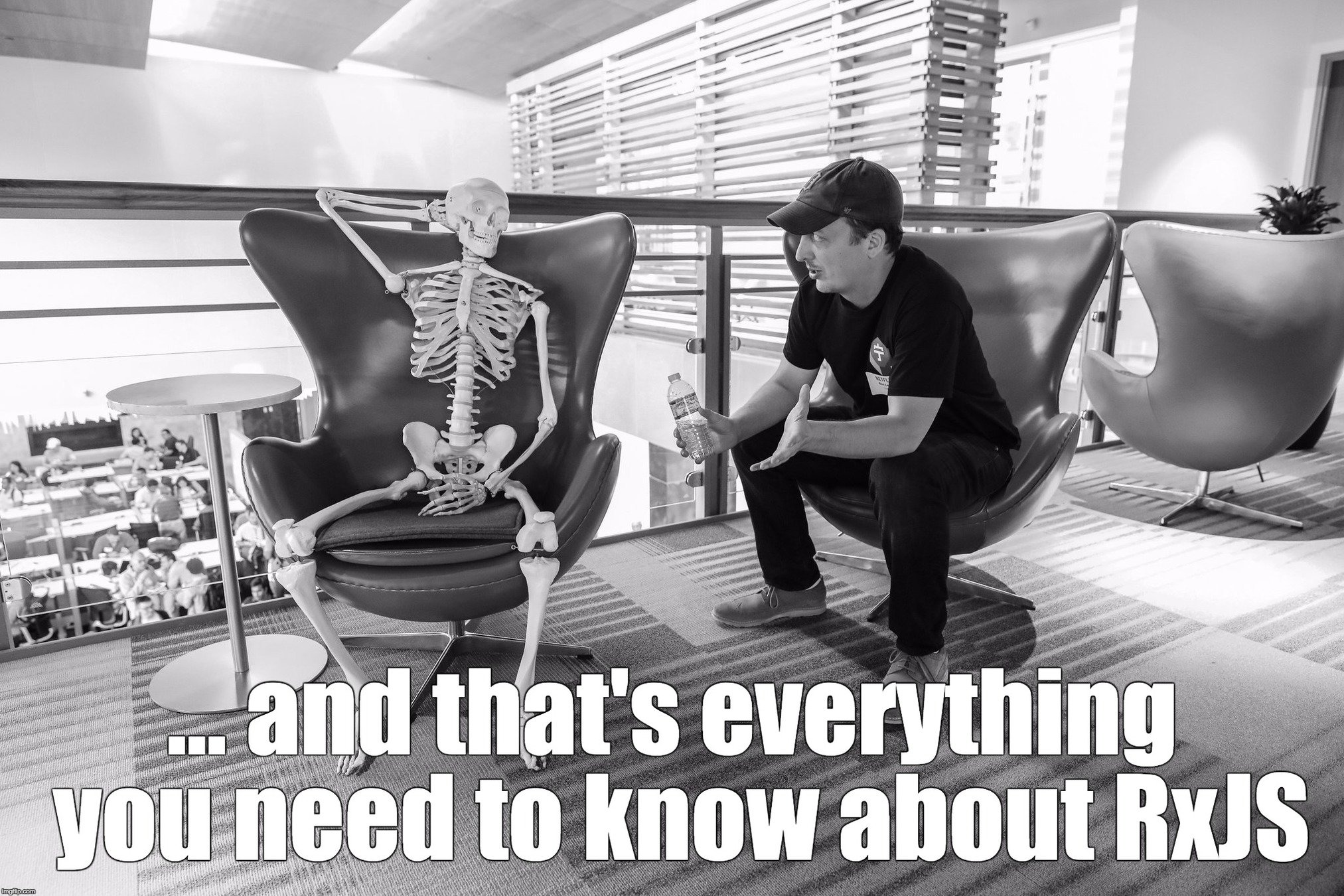
Intro to RxJS
By tobika
Intro to RxJS
In progress
- 239