Stacks and Queues
Objectives
- Define "stack" and Last in First Out (LIFO)
- Define "queue" and First in First Out (FIFO)
- Implement both data structures
Stacks and Queues
Stacks and Queues are specialized lists. The special behavior is related to how data gets in and out of the data structure.
Stacks - LIFO
Stacks are a "last in first out" datastructure
This means the most recently added item is always the first item to leave the stack.
Stacks - LIFO
Imagine a stack of plates
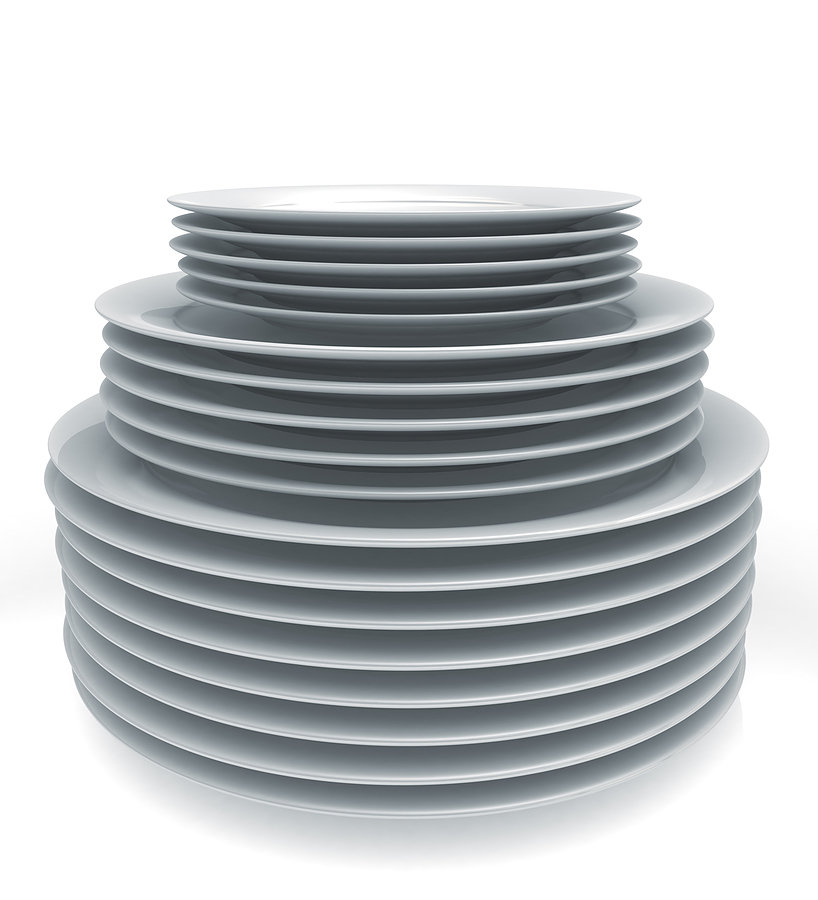
Stacks - API
When talking about stacks, programmers use these words to describe specific behavior
Push: add a new item to the top of the stack.
Pop: remove the top item from the stack.
Push
Head
0
1
2
3
Stacks - API
Push
Head
0
1
2
3
Stacks - API
4
The new node comes on at the head, every thing else is "pushed" down
Pop
Head
0
1
2
3
Stacks - API
4
Pop unlinks the top node and puts the head to our new top.
Pop
Head
popped
0
2
3
Stacks - API
1
Pop also returns this popped node to the caller.
Pop
Head
popped
0
2
3
Stacks - API
1
I think it's interesting that if you push() then pop() you end up with the original stack.
Queues - FIFO
Queues are a "first in first out" data structure.
This means that the first item to get in the queue will always be the first item to come out of the queue.
Queues - FIFO
Queues are a "first in first out" data structure.
Just imagine every line of people you've ever waited in.
Queues - API
When talking about queues, programmers use these words to describe specific behavior
Enqueue: add a new item to the end of the line.
Dequeue: remove the item at the front of the line.
Enqueue
Head
0
1
2
3
Queues- API
Tail
Head
The new node comes on at the head, behind every other node in line
4
Dequeue
Head
0
1
2
3
Queues- API
Tail
Head
The node that has been in the queue longest will be at the tail position. It unlinks and the tail moves back.
4
Dequeue
Head
0
1
2
3
Queues- API
Tail
Head
The dequeued node is returned to the caller.
dequeued
Okay, so whats the big deal?
Push, Pop, Enqueue, and Dequeue are all
O(1) or "constant time".
Okay, so whats the big deal?
Stacks are important to the way memory is managed, "The Call Stack". It's also a useful model that can be applied to a lot of problems.
Okay, so whats the big deal?
Queues are used by the event scheduler in JS. And, like stacks, are a useful model that mimics a lot of real world behavior.
Questions?
Stacks and Queues
By Tyler Bettilyon
Stacks and Queues
- 1,472