Introduction to Python - Conditions
What are conditions
Conditions are the programming syntax which is used to compare two or more terms and based on those conditions, perform one or more actions.
For instance,
Condition 1: You have homework today.
You completed your homework first.
Condition 2: You don't have homework today.
You play cricket today.
Condition
True
False
Code goes inside if cond.
Code after condition
# Let us do some coding to get hands-on
a = 10
b = 20
if(a>b):
print("a is greater than b")
else:
print("b is greater than a")
Quick Question
According to you, what should be printed on the console?
- a is greater than b
- b is greater than a
Option B is correct.
Easy right?
Let us understand why it is correct
So initially, we have two values A and B,
A = 10 and B = 20
Now through the knowledge of basic maths, we know that
A > B, obvious right?
So first of all the program flows go to if condition to check whether it is true or false.
if(a>b):
Here it got a False
So the code control goes to else:
else:
print("I am greater than a")
and perform actions based on else.
Operators and, or and not
Above three mentioned operators are known as "Logical operator".
There functions

How logical Operators are used in If else conditions
The "Logical Operators are used in if else to cascade these conditions.
Let us take a example to illustrate it further
Example
Let us suppose that a research group wants to convey research on the students and for that purpose they collected a database of all the students height, Now it is a vast database, and they want to write a code so that they can extract students whose height is less than 160cm and higher than 180cm, please help them.
To solve this scenario and help out the researchers who are new to python.
We need to figure out a solution, we have to label a student "included" if he matches the range, else "excluded".
Can You guess the solution based on what you have learned till now,
Let's take a 10 sec time to solve it right now.
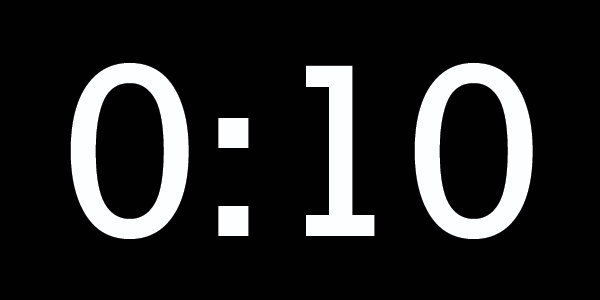
Hope that everyone has figured out the solution
# Solution 1
# let say "h" is the variable to store the height of one student.
if(h>180):
print("included")
if(h<160):
print("included")
else:
print("excluded")
Better Solution
# same assumptions as in previous one
if(h>180 or h<160):
print("included")
else:
print("excluded")
We can apply and, not in the same way, just we need to identify that whether both true is required, one true one false is required or all false is the case.
Some practice problems to understand logical operators
a = 10
b = 20
c =110
1. a>b and c>a
2. a>b or c>a
3. a>c and b<a or c>b
In next class we are going to cover
"Not" logical operator.
About else if condition.
Alternatives of if-else, "ternary" operators.
Introduction to while and for loops.
Solution of practice problems
See you all in the next lecture
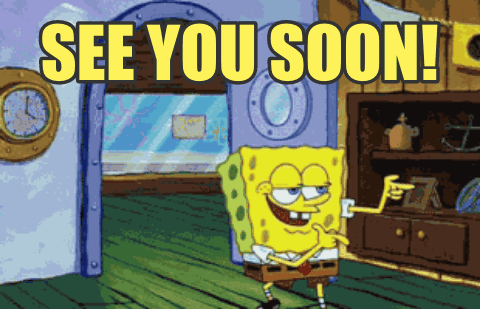
deck
By Ujjwal Singh
deck
- 2