Let's talk about
Web APIs
Vidya Ramakrishnan
Front-end developer at HasGeek
vidya-ram vidya_ramki
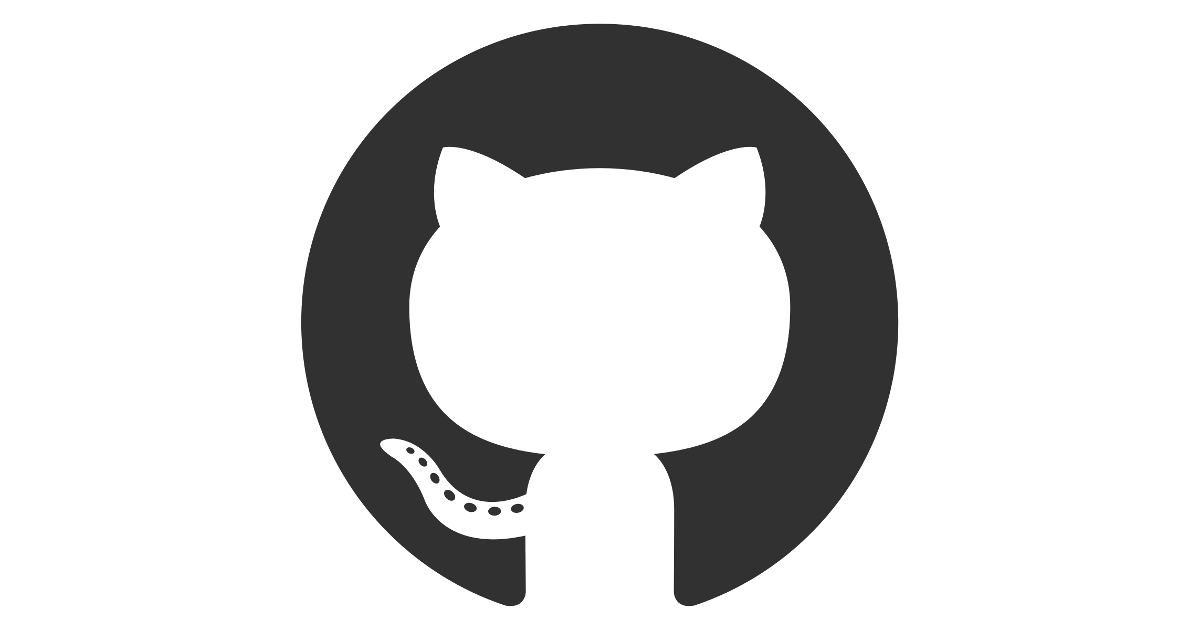
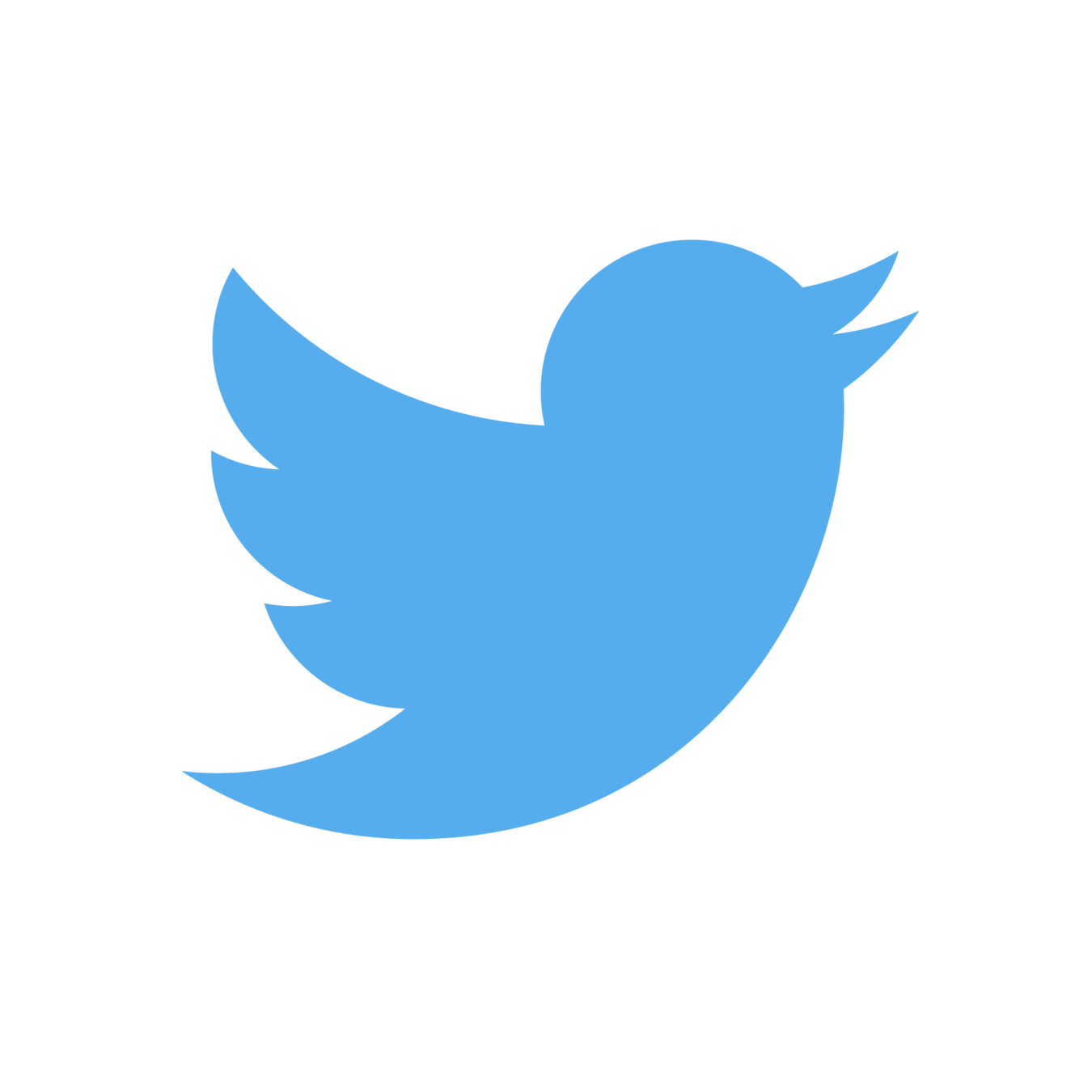
localStorage
window.App.Store = {
/* Local storage can only save strings, so value is converted
into strings and stored. */
add: function(key, value) {
return localStorage.setItem(key, JSON.stringify(value));
},
// Reads from LocalStorage.
read: function(key) {
return JSON.parse(localStorage.getItem(key));
},
// To remove item and clear
remove: function(key) {
return localStorage.removeItem(key);
},
// To clear localStorage
remove: function(key) {
return localStorage.clear();
}
};
Use case: Save the data(not sensitive information) that needs to be sent to server, to localStorage when offline. Once network connectivity is back, read from localStorage and sent it to the server. Local storage wasn't designed to store secure data
browser support
Further reading:- Indexdb
navigator.onLine
if (!navigator.onLine) {
console.log("You are offline");
} else {
/* Device could be connected to WiFi but there could be no internet
fetch('/1/api').then(function(response) {
console.log("You are online");
}).catch(function(err) {
console.log("You are offline");
});
}
// Detect changes to network state by listening for the events
window.addEventListener('offline', function(event) {
console.log("You're offline");
});
window.addEventListener('online', function(event) {
fetch('/1/api').then(function(response) {
console.log("You are online");
}).catch(function(err) {
console.log("You are offline");
});;
});
To check network connection of the browser.
Returns a boolean value, true meaning online and false meaning offline.
navigator.mediaDevices.getUserMedia
navigator.mediaDevices.getUserMedia({ audio: false, video: { facingMode: "environment" })
.then(function(mediaStream) {
var video = document.createElement("video");
video.srcObject = mediaStream;
document.body.appendChild(video);
video.play();
})
.catch(function(err) {
console.log('Error');
});
navigator.mediaDevices.getUserMedia() prompts the user for permission to use a media input (audio/video) which output a media stream
Use case: To scan QR code from web apps using jsQR
This library takes in raw images and will locate, extract and parse any QR code found within.
postMessage
targetWindow.postMessage(message, targetOrigin, [transfer]);
window.addEventListener("message", receiveMessage, false);
// Window.parent (the parent window from within an embedded <iframe>)
window.parent.postMessage(JSON.stringify({
context: "iframe.resize",
height: document.body.scrollHeight,
width: document.body.scrollWidth,
id: {{ request.args.get('iframeid') | tojson }}
}), '*');
/* Listen to message from iframe and
set the height of the iframe accordingly */
window.addEventListener('message', function(event) {
if(event.origin == verifiedHostname) {
var message = JSON.parse(event.data);
if(message.context == "iframe.resize" && message.id) {
document.getElementById(message.id).setAttribute('height', message.height);
}
}
}, false);
window.postMessage() allows cross-origin communication between Window objects.
It allows safe communication between windows and frames hosted on different domains
Use case: This helps resizing of iframe embedded on a different domain
document API
querySelector(), querySelectorAll(), classList
// querySelector and querySelectorAll
let list = document.querySelector('ul.inline-list');
let items = list.querySelectorAll('li');
//classList
<div class="item show">
....
</div>
let elem = document.querySelector('.item');
console.log('classes', elem.classList); // ["item", "show", value: "item show"]
elem.classList.add('spin', 'animate');
console.log(elem.classList.contains('spin')); //true
elem.classList.remove('spin');
elem.classList.toggle('show');
Copy to clipboard using JS without flash
<div class="copy">Hello, world</div>
<p class="error-msg">Copy error</p>
var elem = document.querySelector('.copy');
var selection = window.getSelection();
var range = document.createRange();
range.selectNodeContents(elem);
selection.removeAllRanges();
selection.addRange(range);
elem.addEventListener('click', function() {
try {
document.execCommand('copy');
selection.removeAllRanges();
elem.classList.add('copied');
} catch(e) {
var error = document.querySelector('.error-msg');
error.classList.add('show');
}
});
Thank you!
Let's talk about Web APIs
By vidya_ramki
Let's talk about Web APIs
- 769