Back to the work
(or what did I miss)
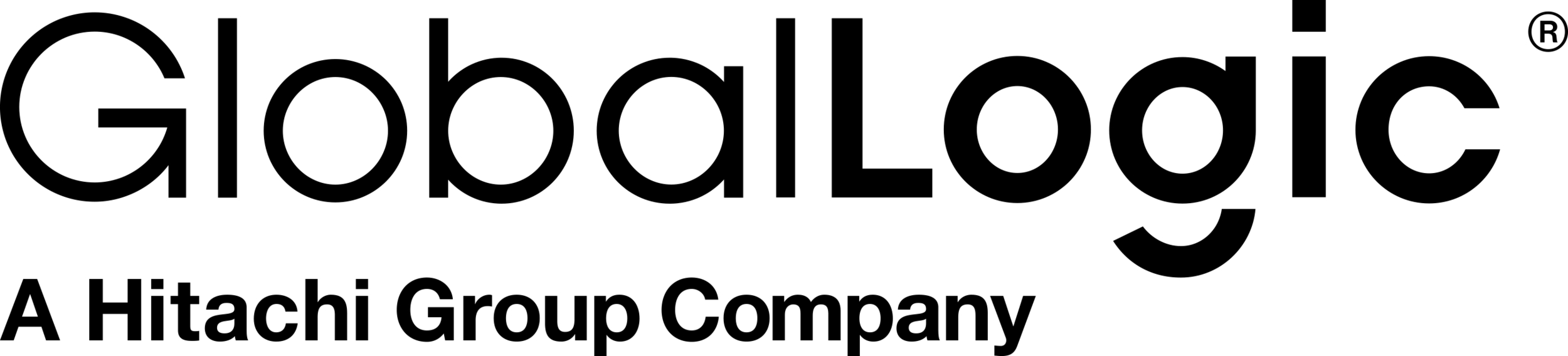
Agenda
UA jobs market overview
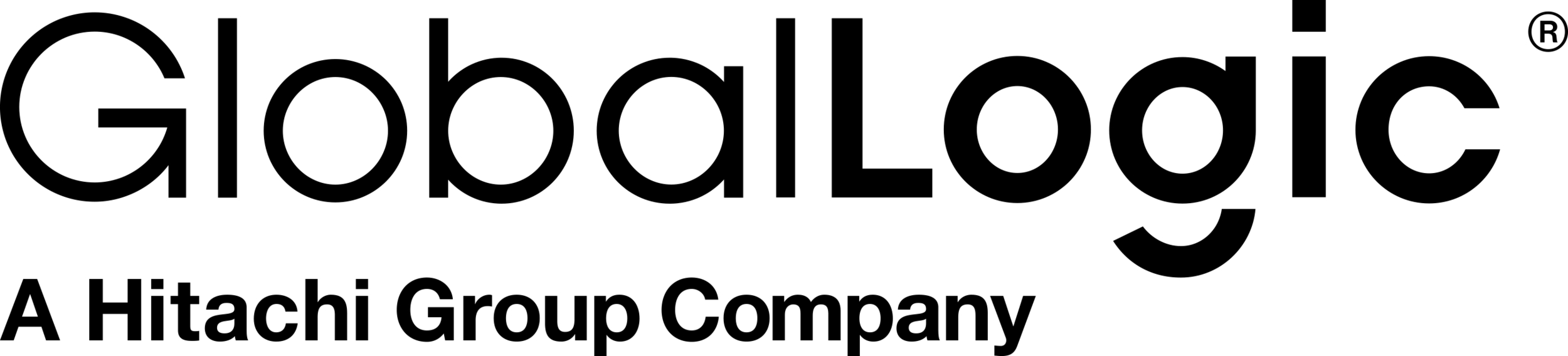
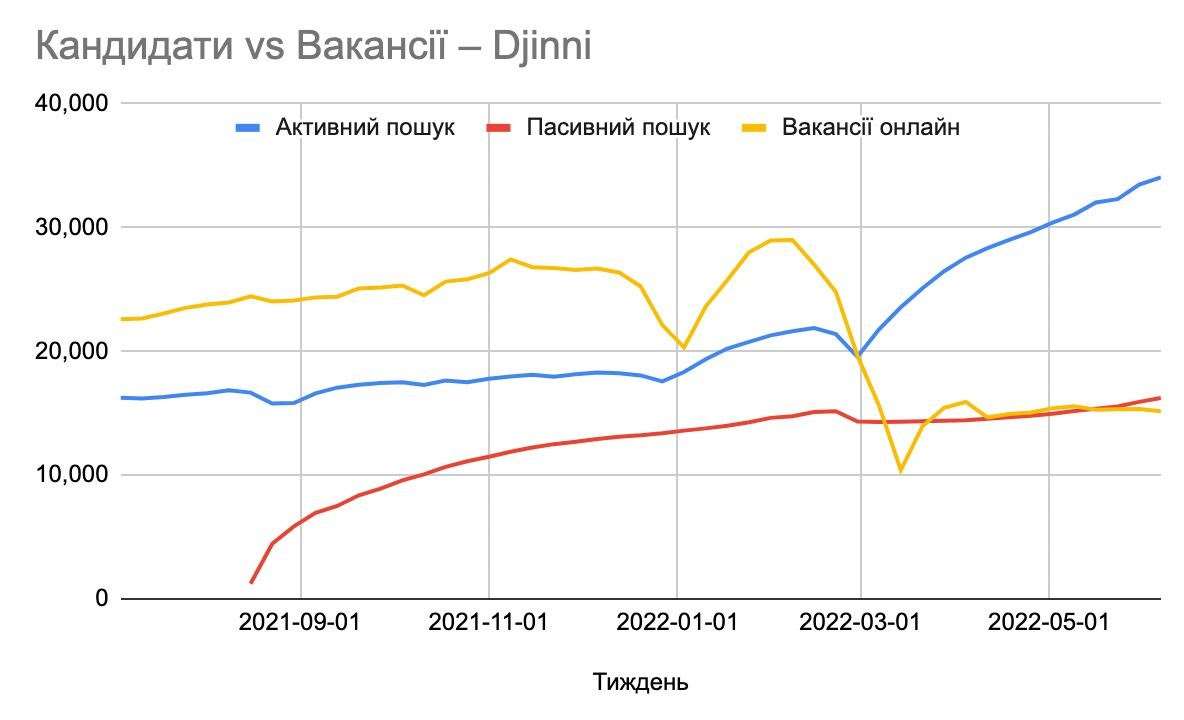
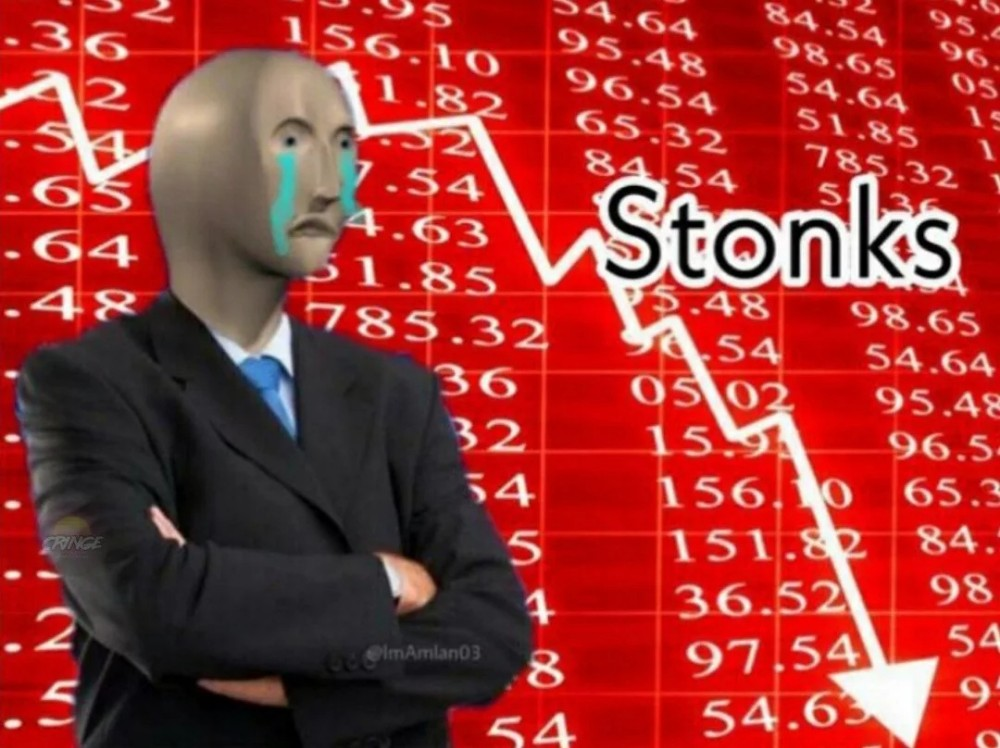
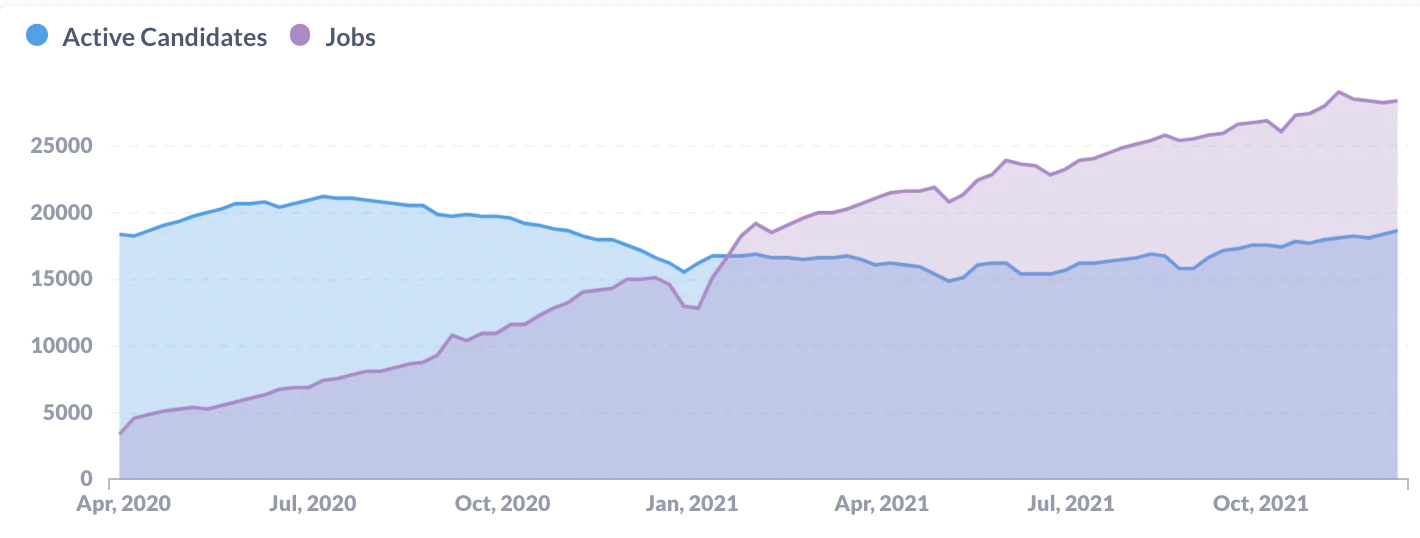
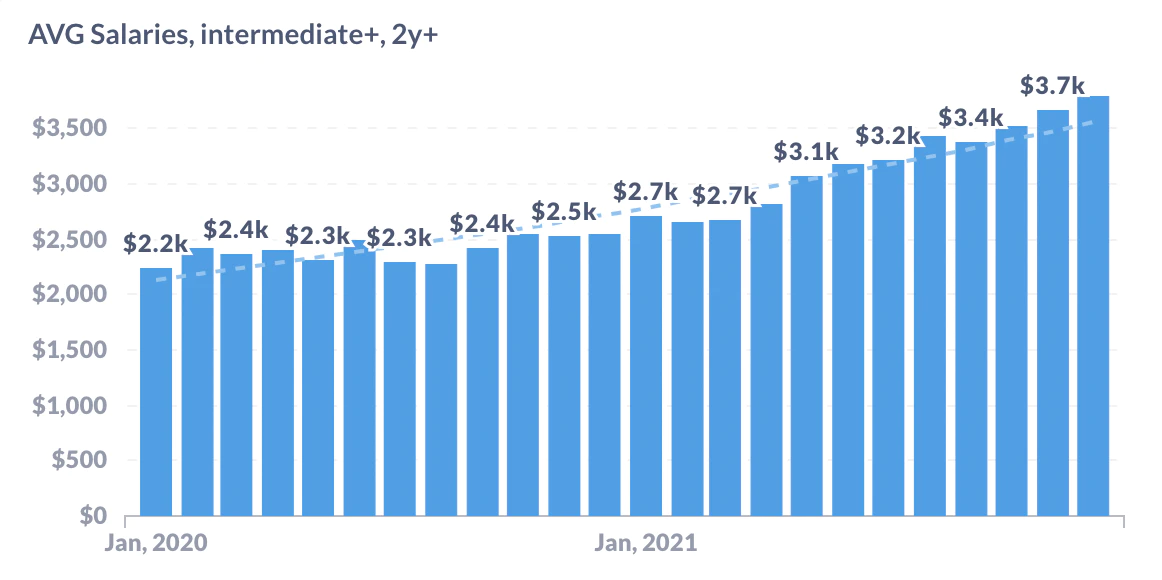
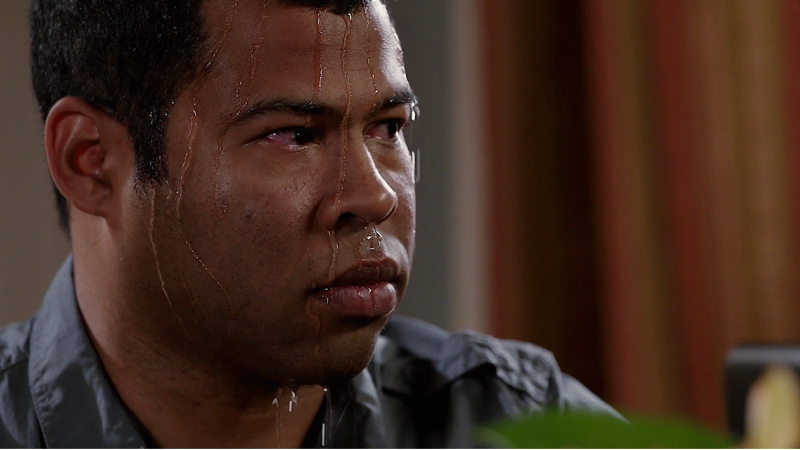
Ability to work under preassure
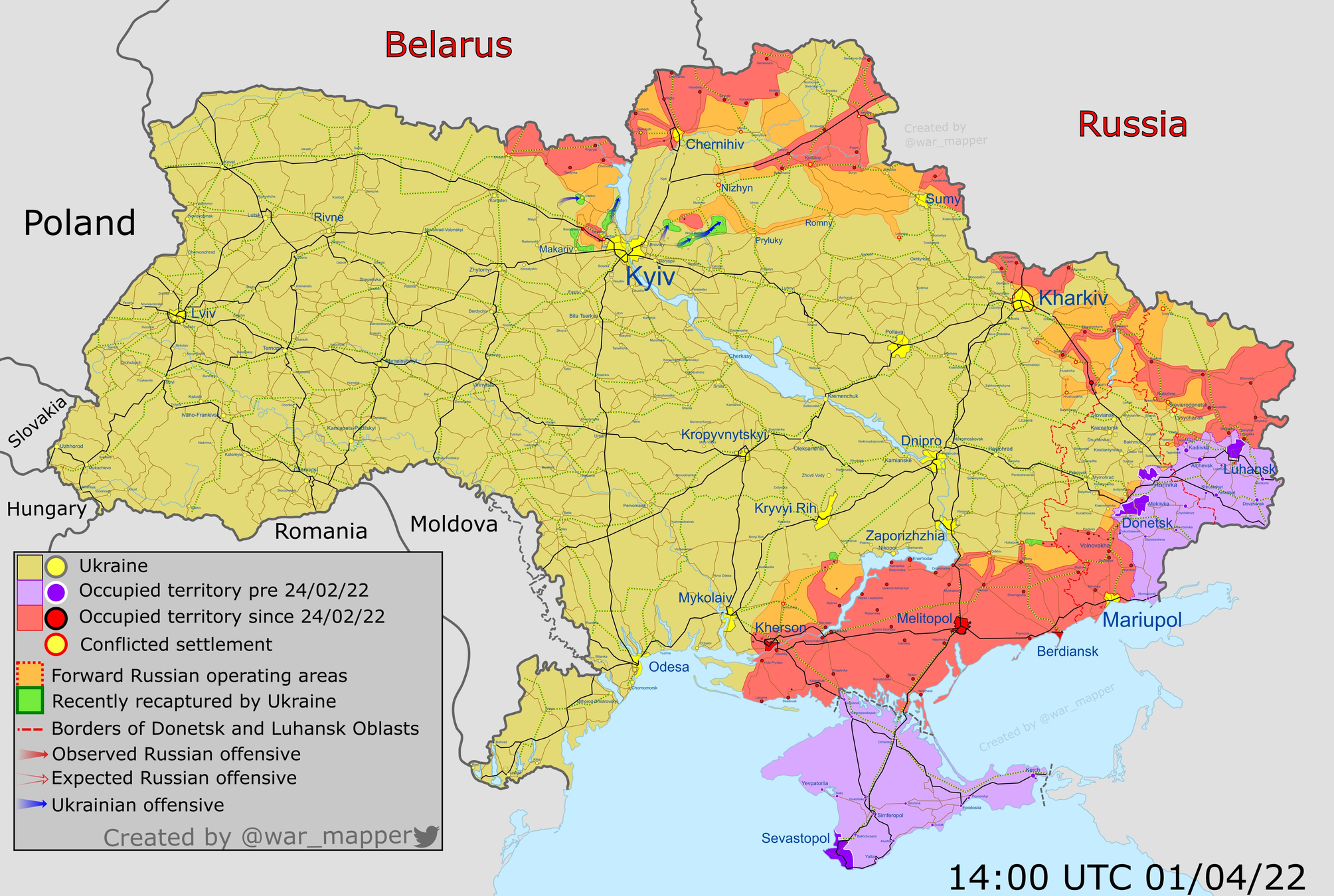
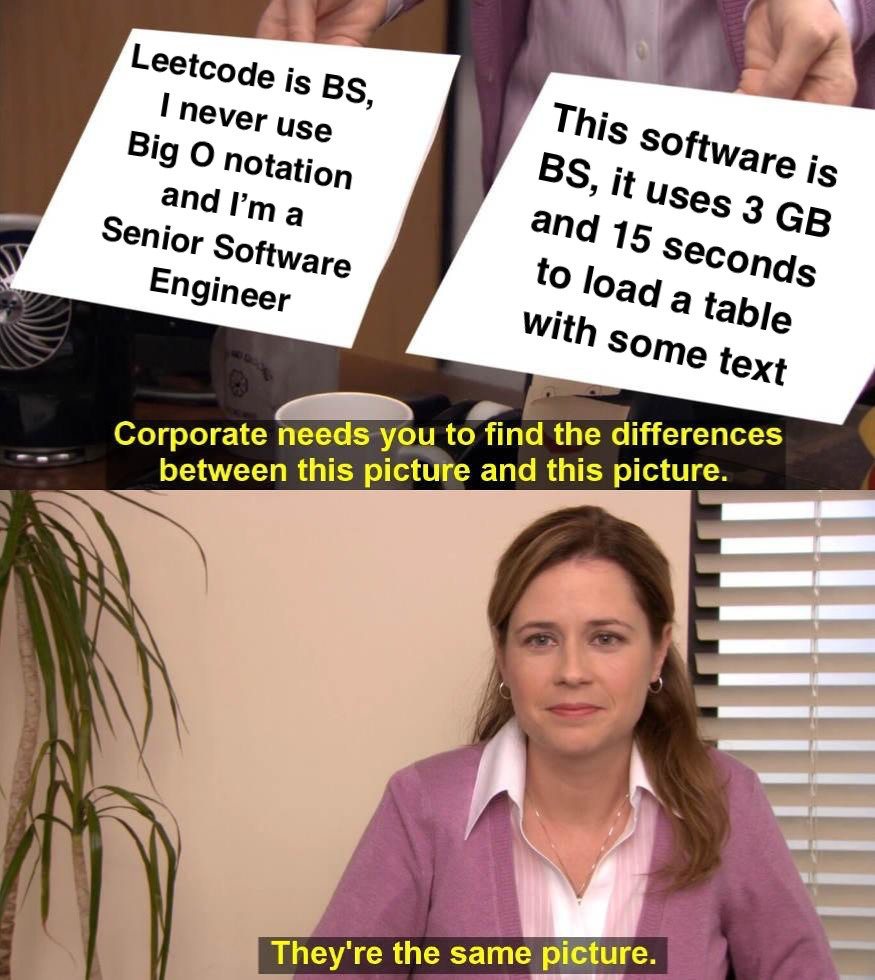
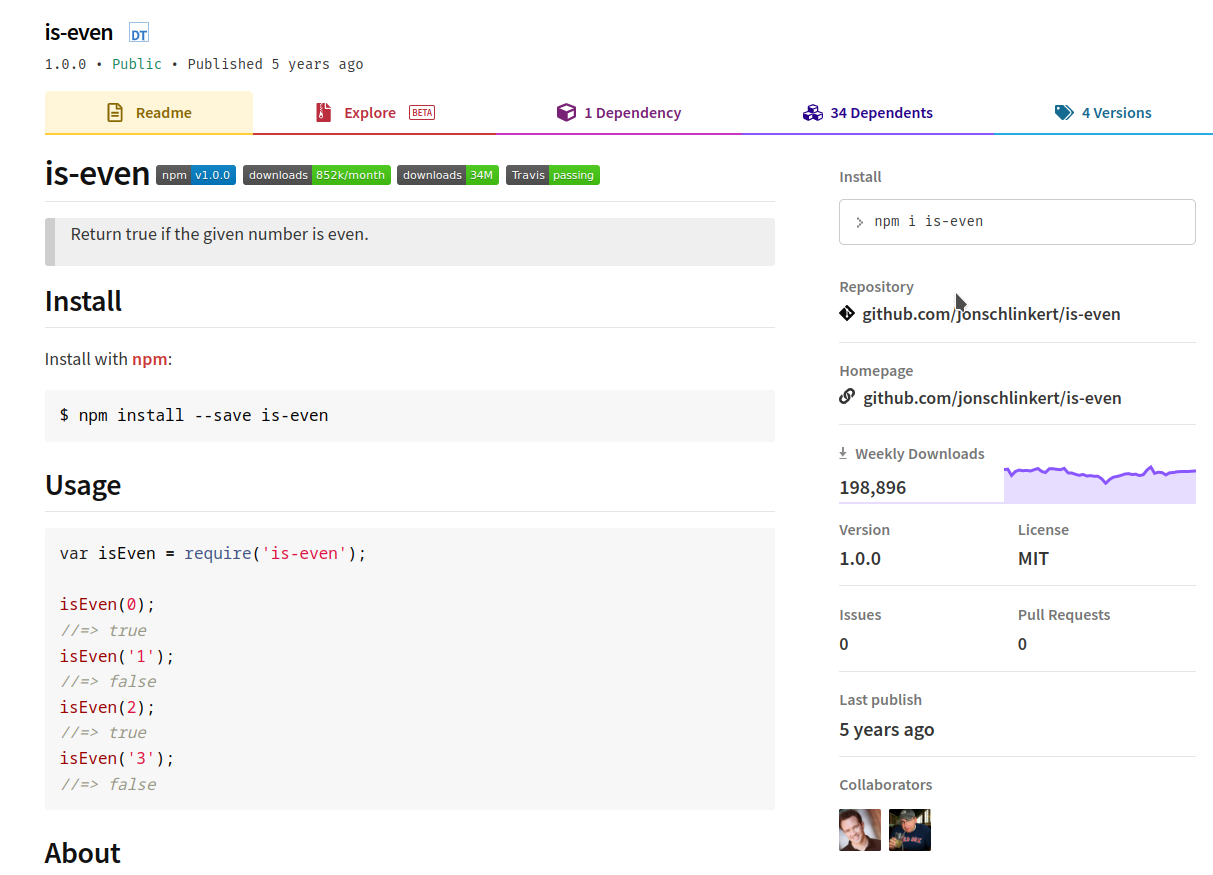
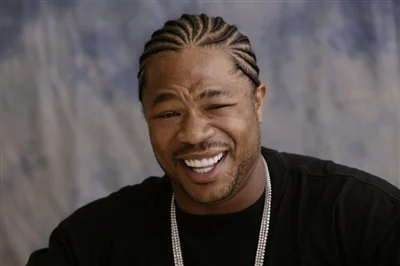
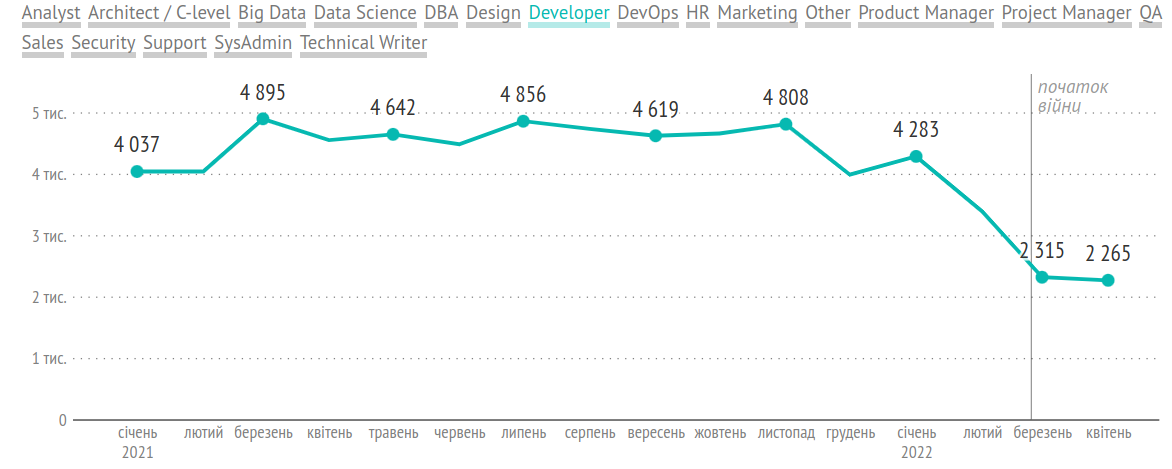
~3800
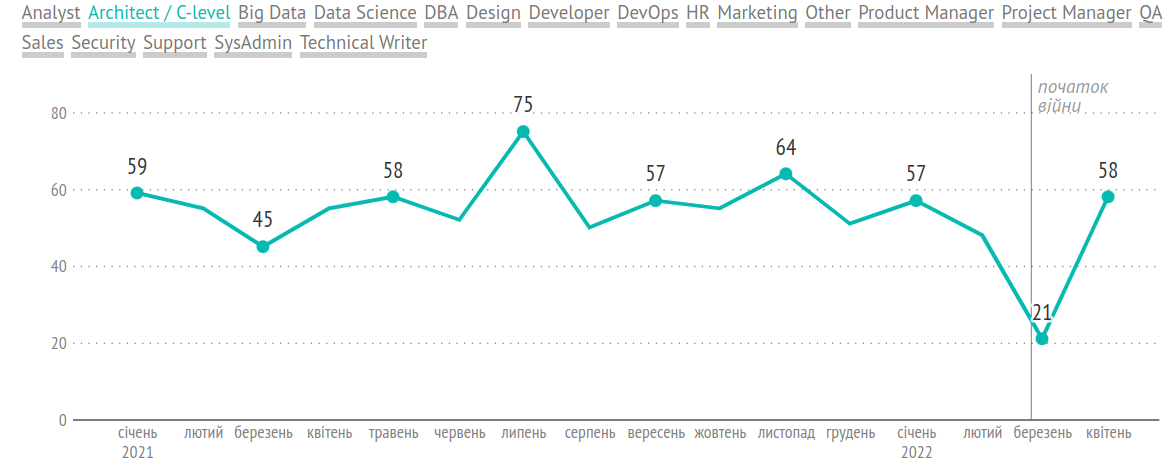
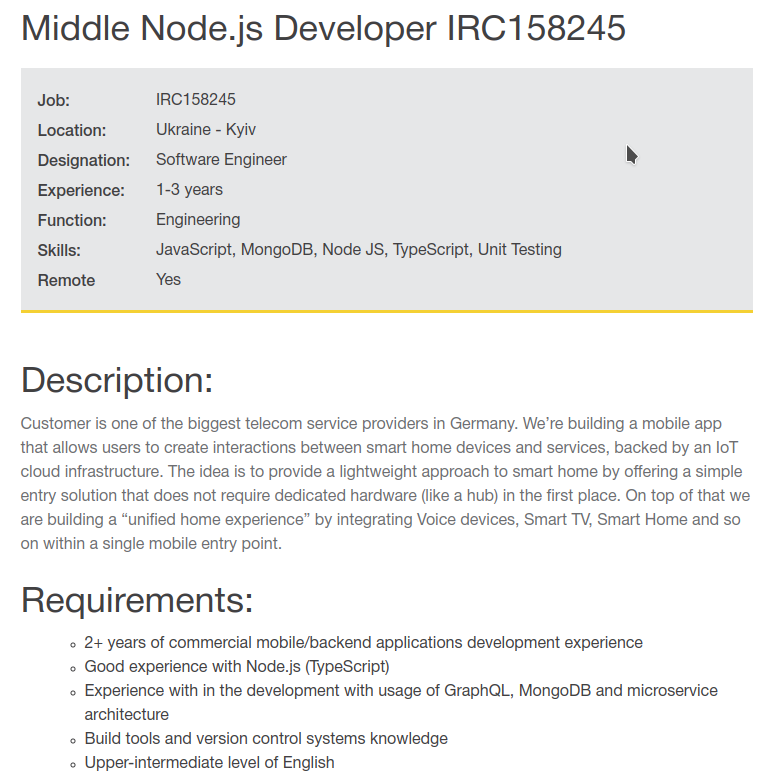
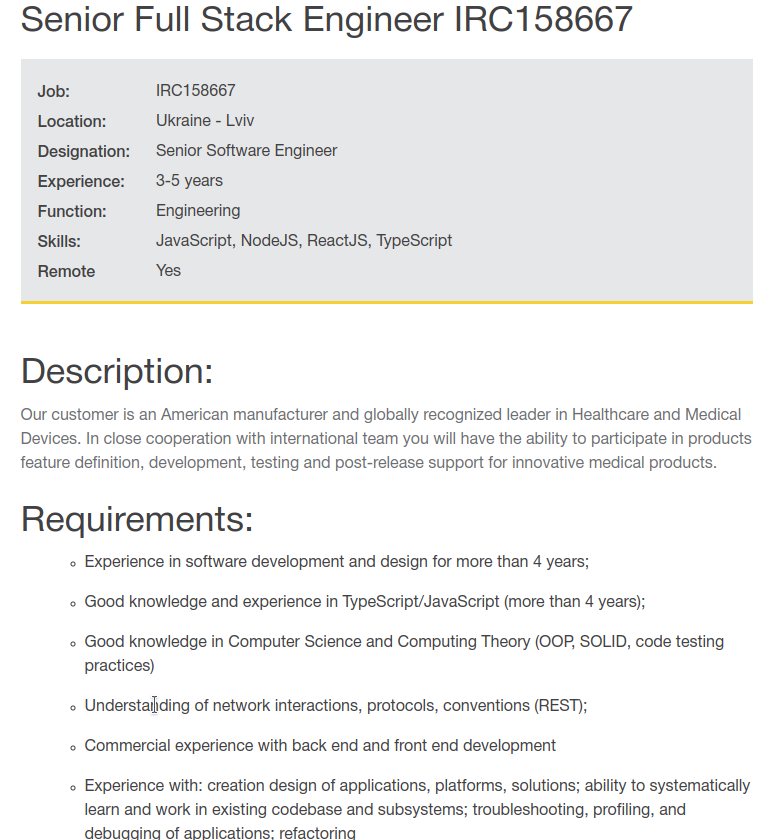
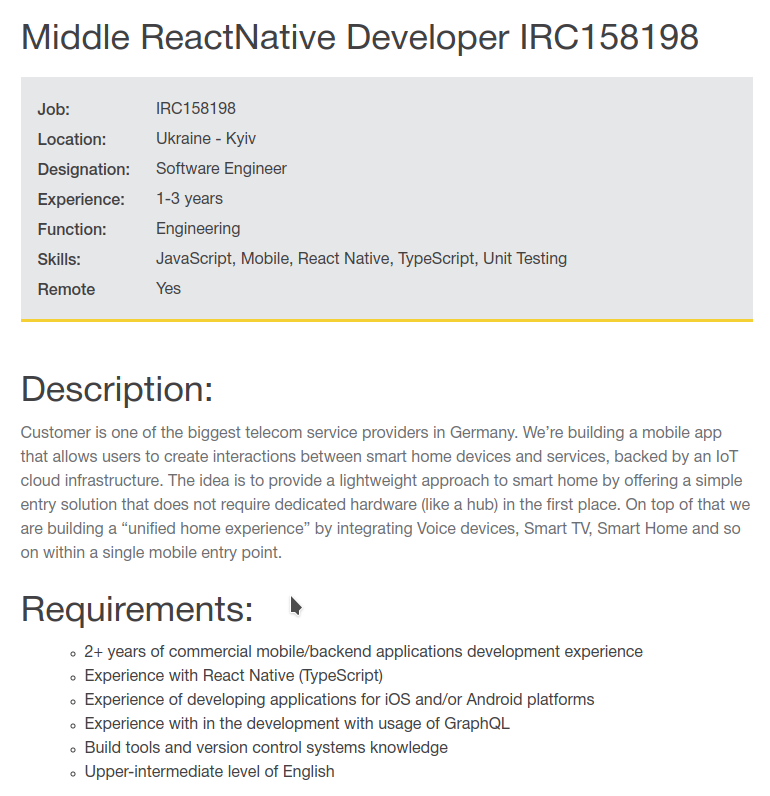
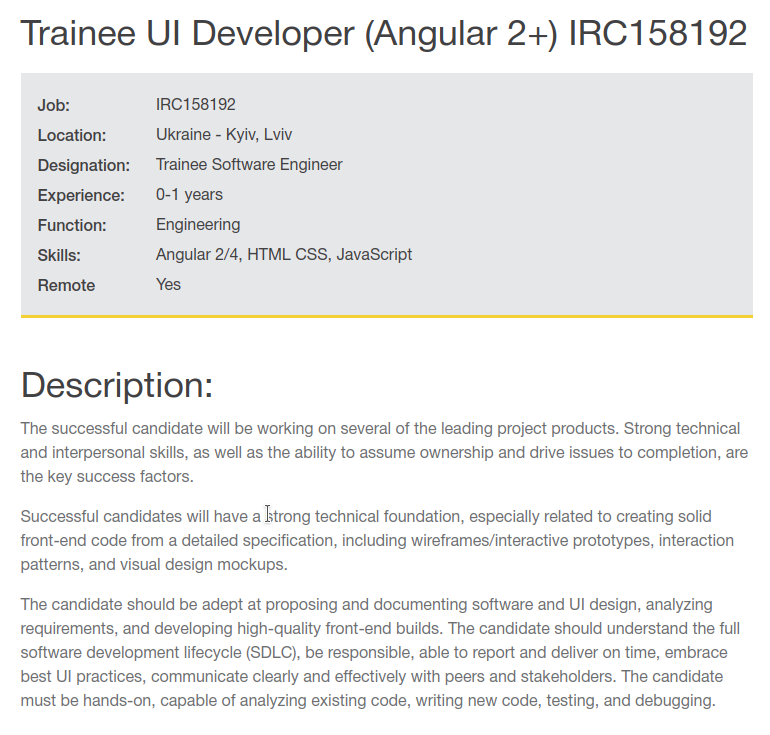
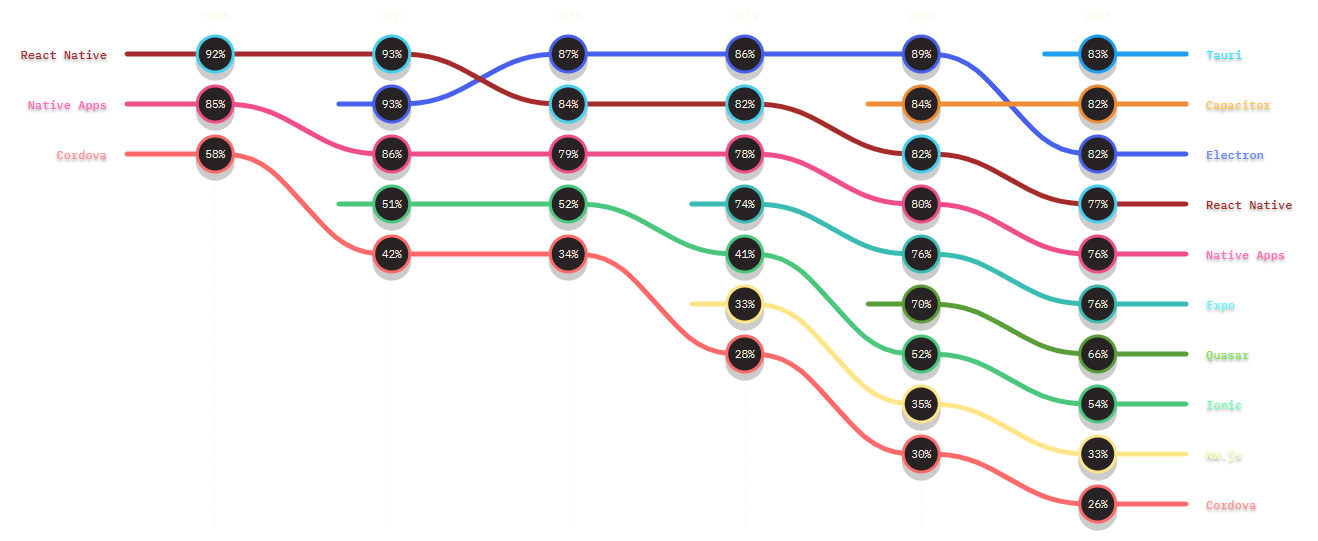
Satisfaction rate
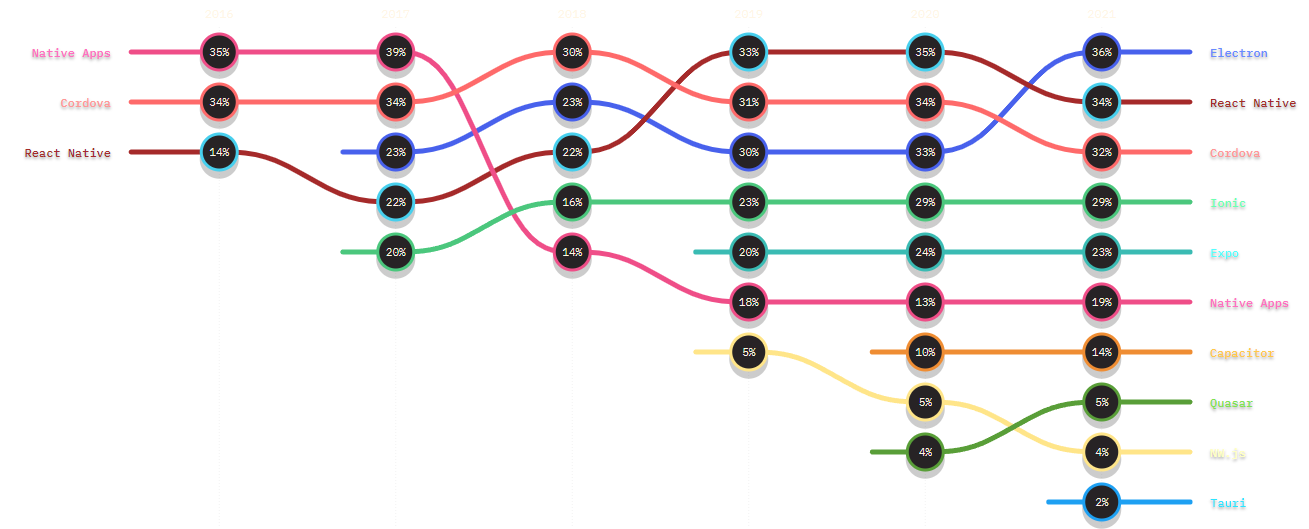
Usage rate
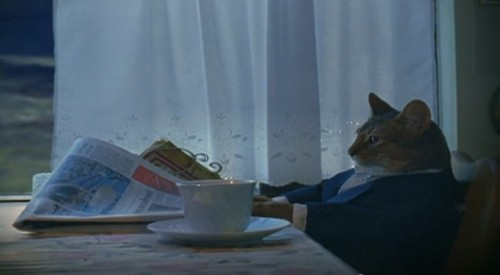
Should I become a "fullstack" ???
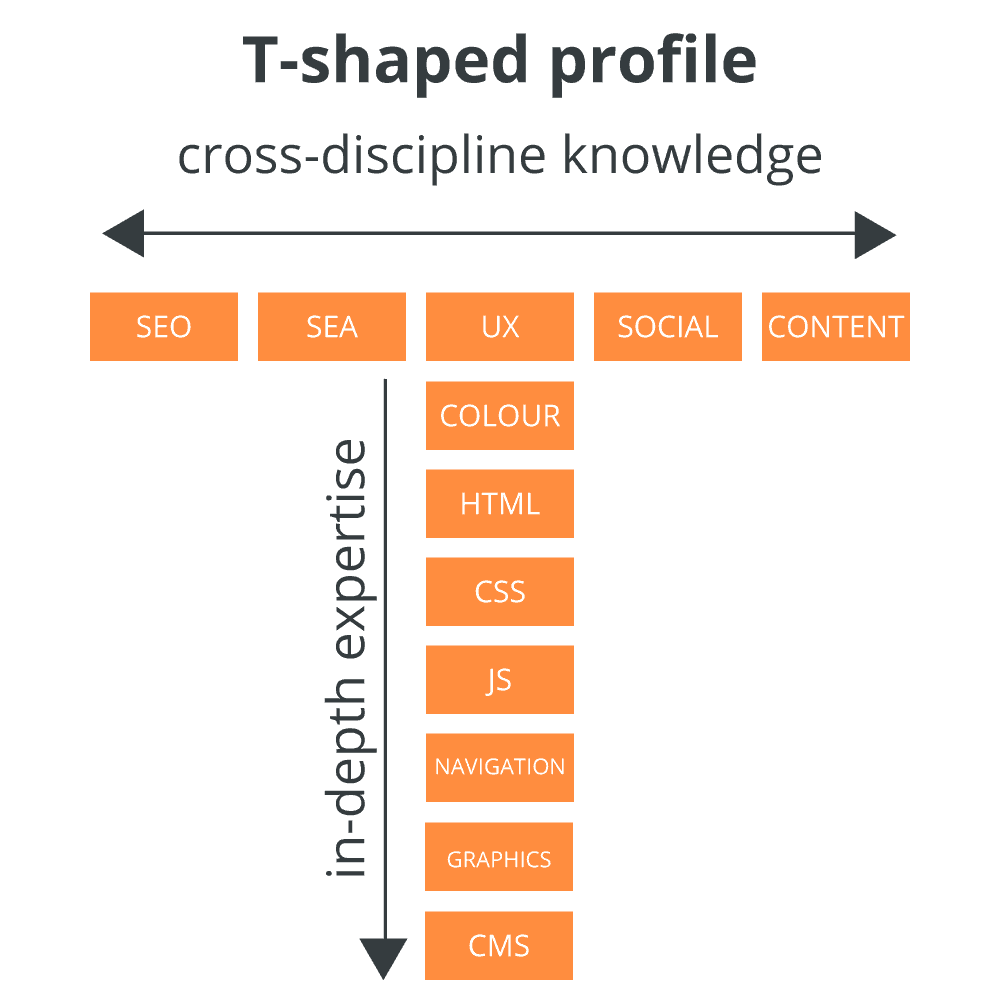
JavaScript world updates
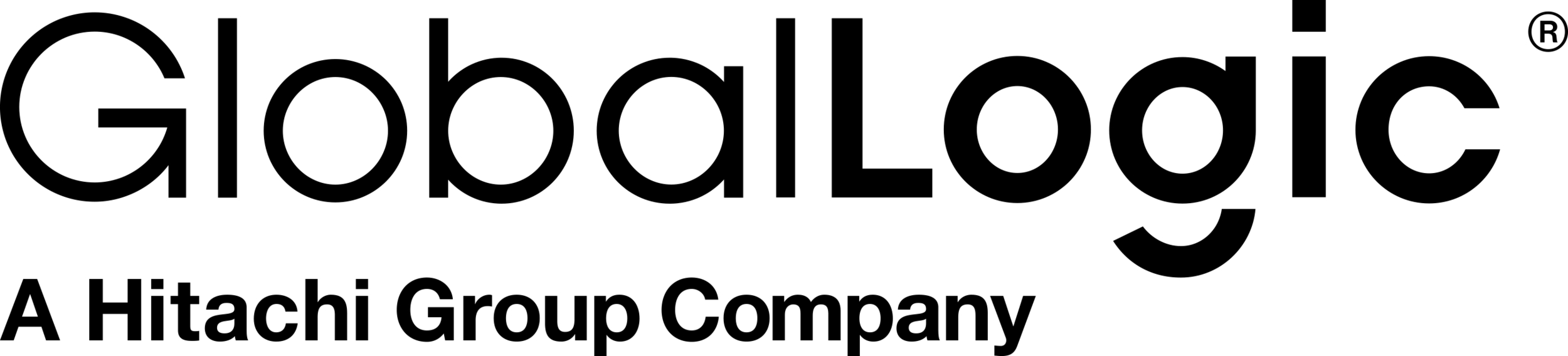
NodeJS 18
new features
Vue 3
Release: June 2022 / composition API
React 18
concurrent mode!
HTTP/3
specification & QUIC over UDP
ECMAScript 2022
was published in June 2022
Typescript
a new standard
Vite
Next Generation Frontend Tooling
Low Code & No code (Teleport HQ)
BFF
Edge coputing
Nest serverless
Next
Nuxt
Remix
ES
2022
class MyClass {
instancePublicField = 1;
static staticPublicField = 2;
#instancePrivateField = 3;
static #staticPrivateField = 4;
#nonStaticPrivateMethod() {}
get #nonStaticPrivateAccessor() {}
set #nonStaticPrivateAccessor(value) {}
static #staticPrivateMethod() {}
static get #staticPrivateAccessor() {}
static set #staticPrivateAccessor(value) {}
static {
// Static initialization block
}
}
New members of classes
// Error and its subclasses now let us specify
// which error caused the current one:
try {
// Do something
} catch (otherError) {
throw new Error('Something went wrong', {cause: otherError});
}
error.cause
// my-module.mjs
//We can now use await at the top levels of modules
//and don’t have to enter async functions or methods anymore:
//
const response = await fetch('https://example.com');
const text = await response.text();
console.log(text);
Top-level await in modules
// Method .at() of indexable values lets us read an element at
// a given index (like the bracket operator []) and supports
// negative indices (unlike the bracket operator):
> ['a', 'b', 'c'].at(0)
'a'
> ['a', 'b', 'c'].at(-1)
'c'
//The following “indexable” types have method .at():
// - string
// - Array
// - All Typed Array classes: Uint8Array etc
Method .at() of indexable values
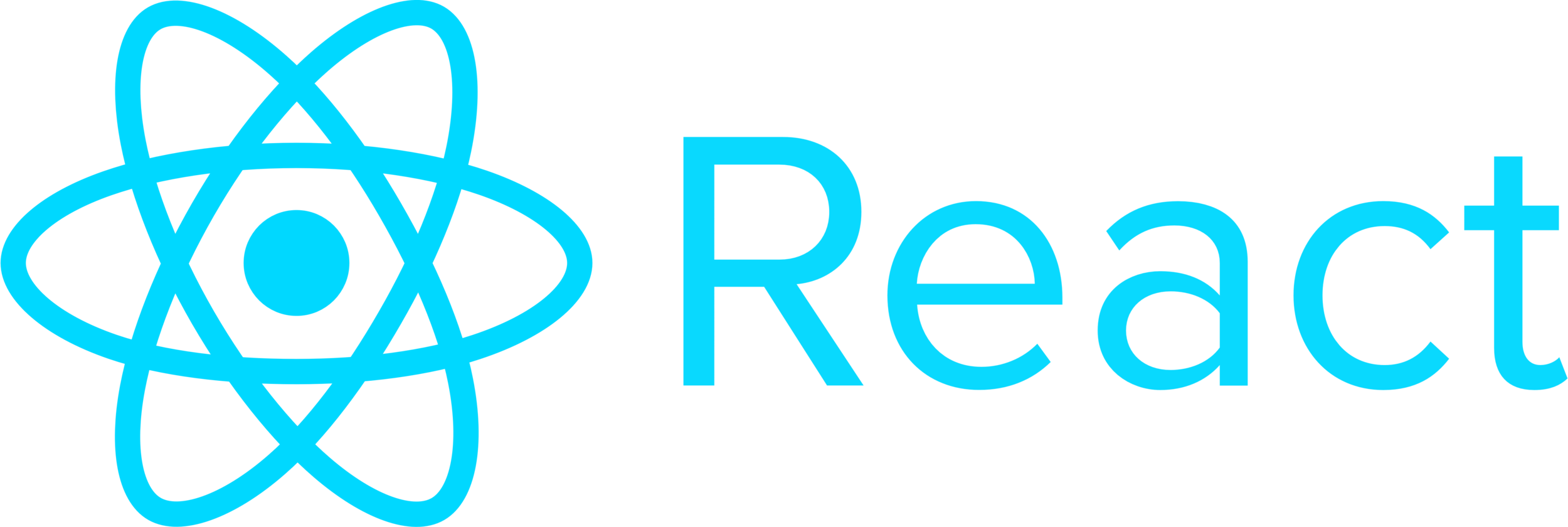
18
// Before
import { render } from 'react-dom';
const container = document.getElementById('app');
render(<App tab="home" />, container);
// After
import { createRoot } from 'react-dom/client';
const container = document.getElementById('app');
const root = createRoot(container);
root.render(<App tab="home" />);
ReactDOM.render is no longer supported in React 18
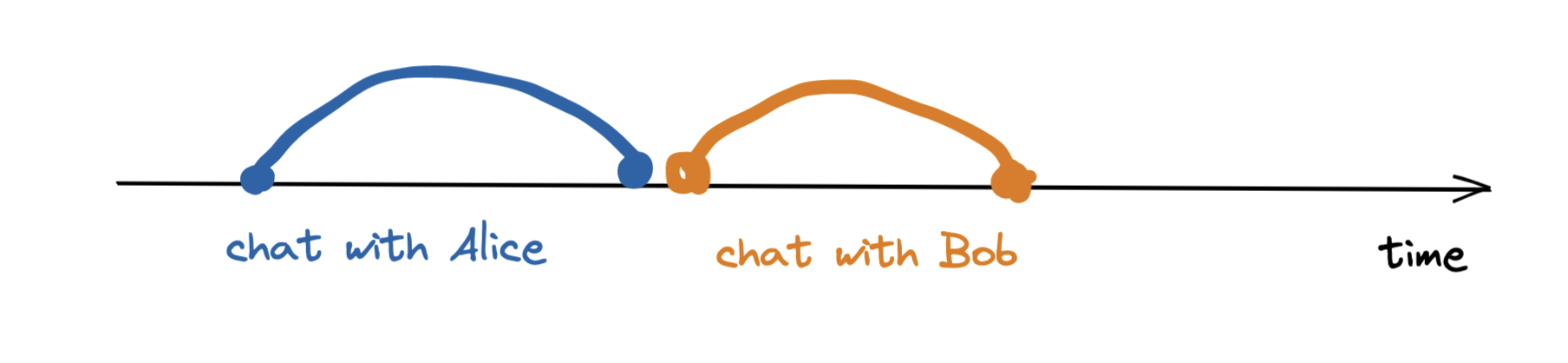
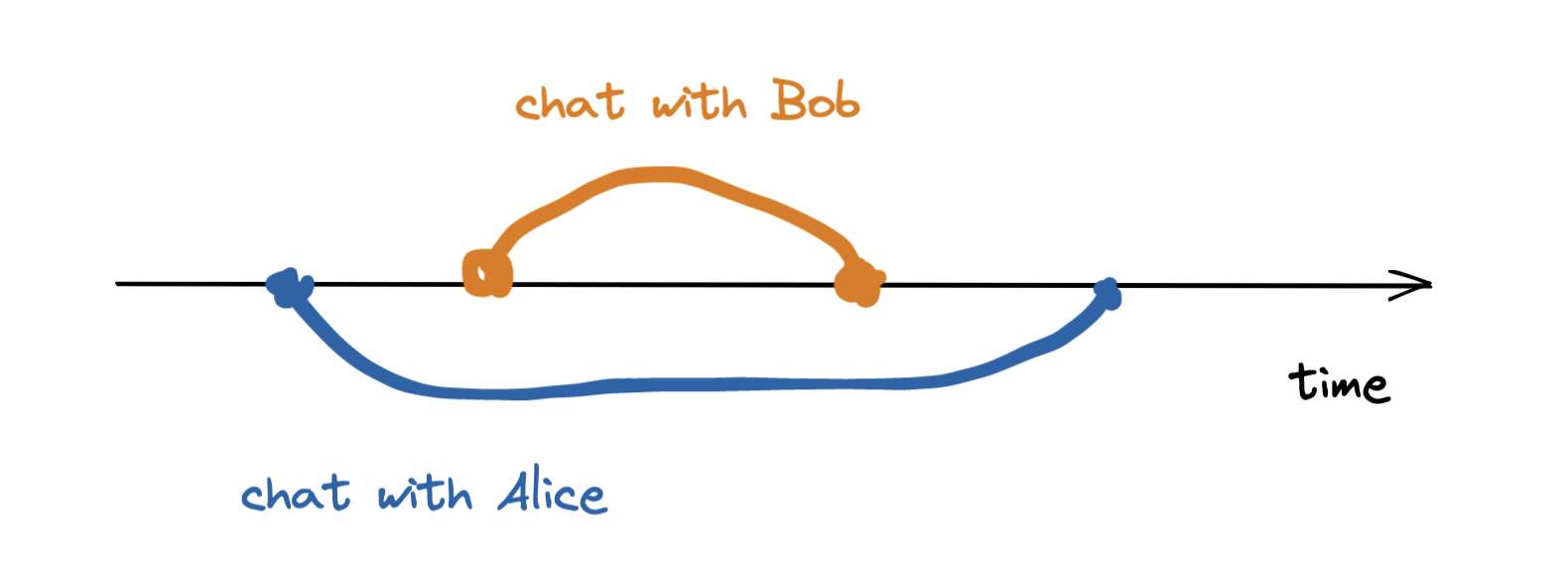
React 18 with concurrent rendering, React can interrupt, pause, resume, or abandon a render.
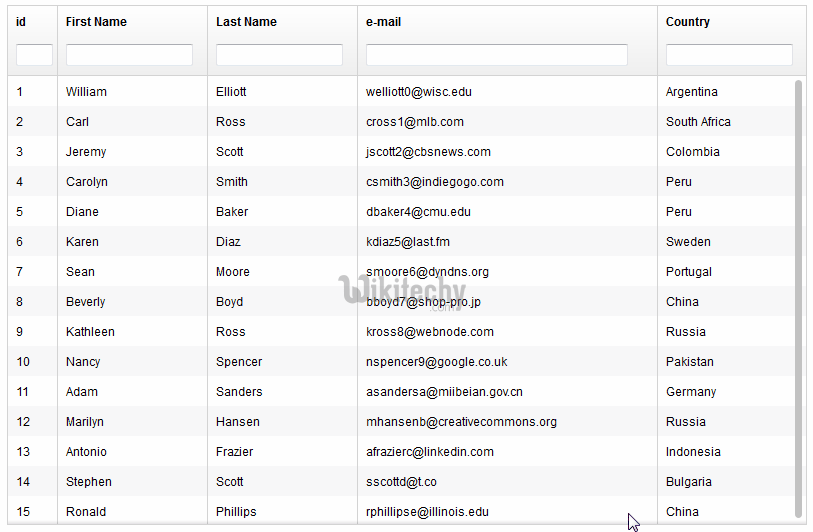
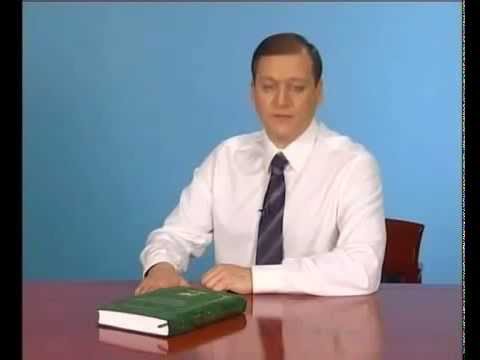
Transitions
import { startTransition } from 'react';
// Urgent: Show what was typed
setInputValue(input);
// Mark any non-urgent state updates inside as transitions
startTransition(() => {
// Transition: Show the results
setSearchQuery(input);
});
Non-urgent updates are called transitions. By marking non-urgent UI updates as "transitions", React will know which updates to prioritize. This makes it easier to optimize rendering and get rid of stale rendering.
// Before: only React events were batched.
setTimeout(() => {
setCount(c => c + 1);
setFlag(f => !f);
// React will render twice, once for
// each state update (no batching)
}, 1000);
// After: updates inside of timeouts, promises,
// native event handlers or any other event are batched.
setTimeout(() => {
setCount(c => c + 1);
setFlag(f => !f);
// React will only re-render once at the end (that's batching!)
}, 1000);
Automatic Batching
Suspense on the server
Wrap a slow part of your app within the Suspense component, telling React to delay the loading of the slow component.
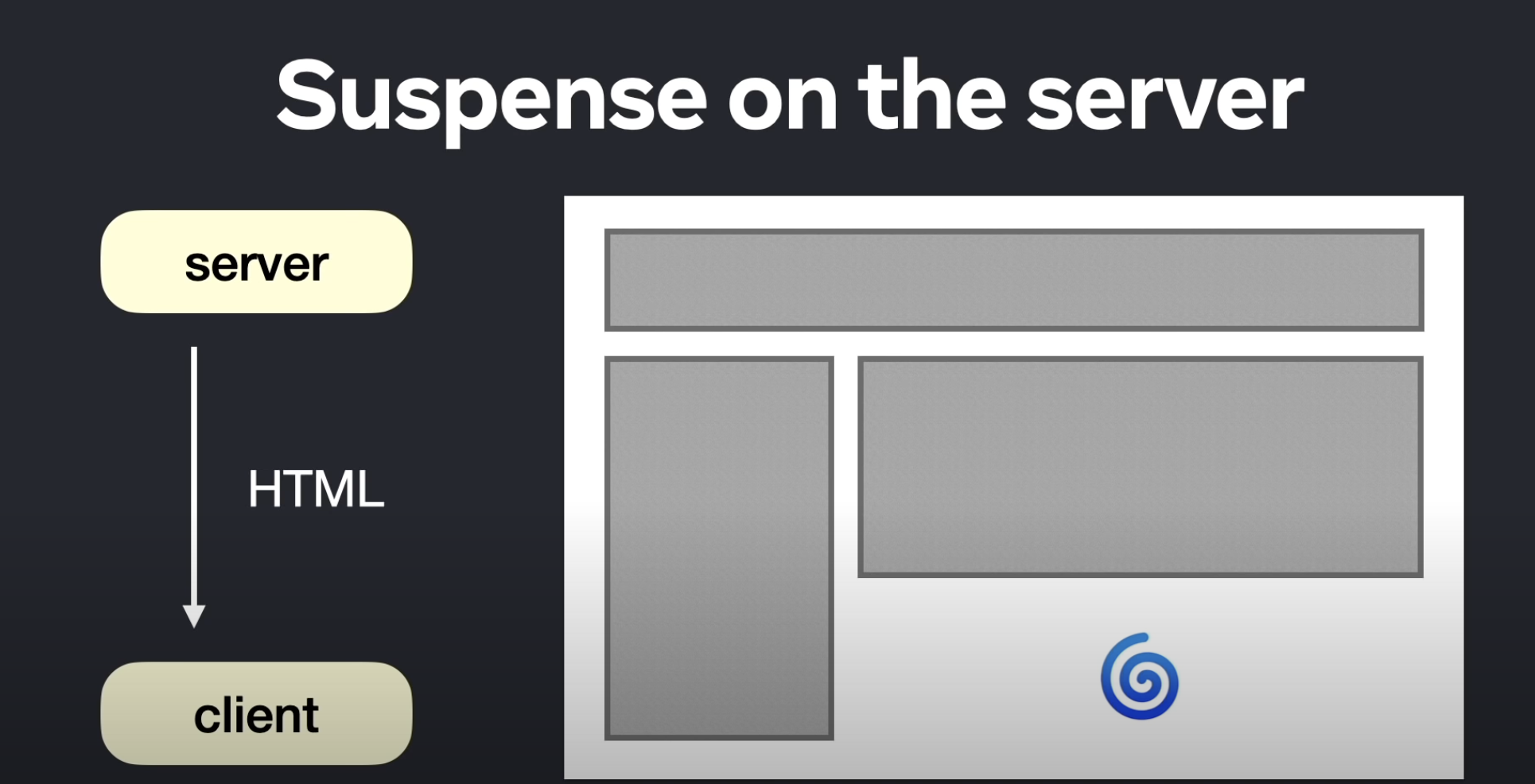
<Suspense fallback={<Spinner />}>
<Comments />
</Suspense>
18
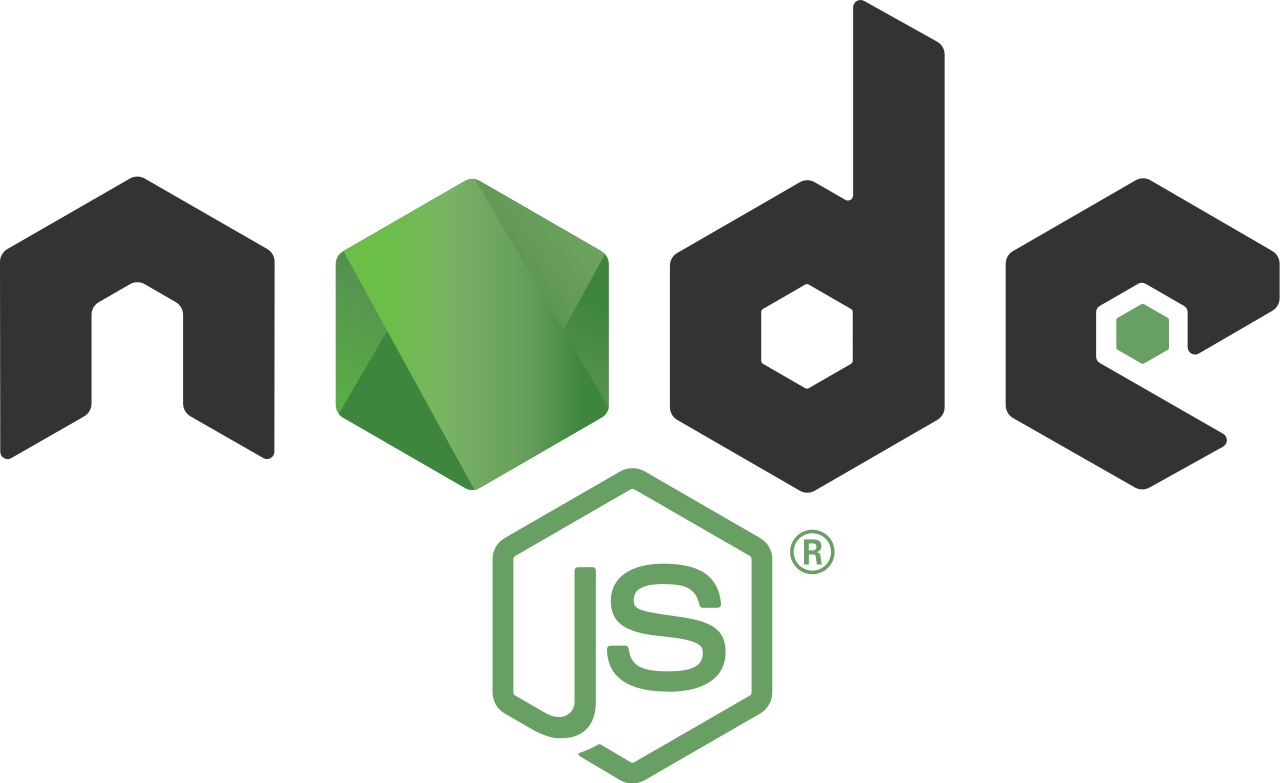
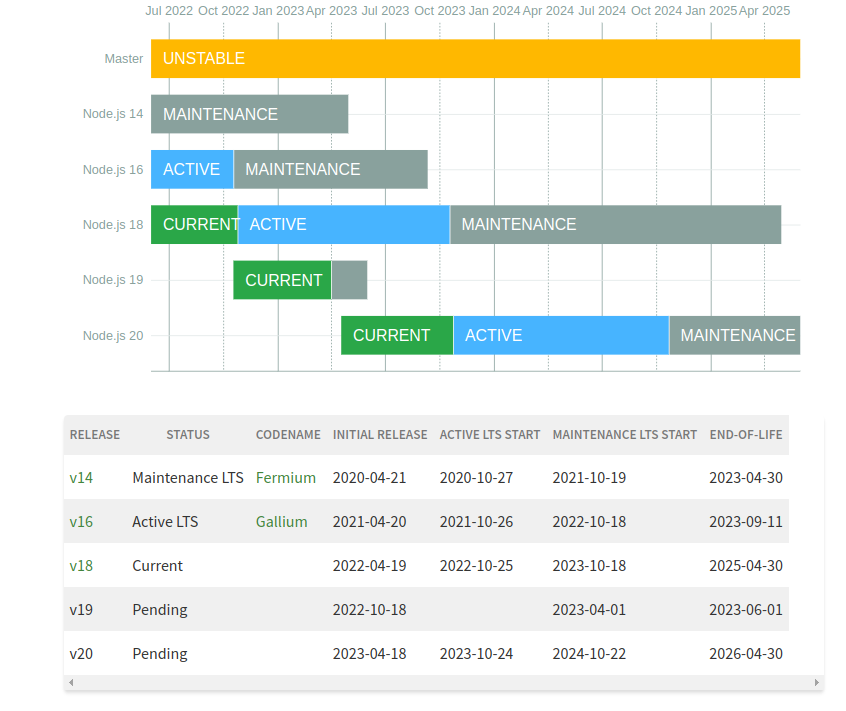
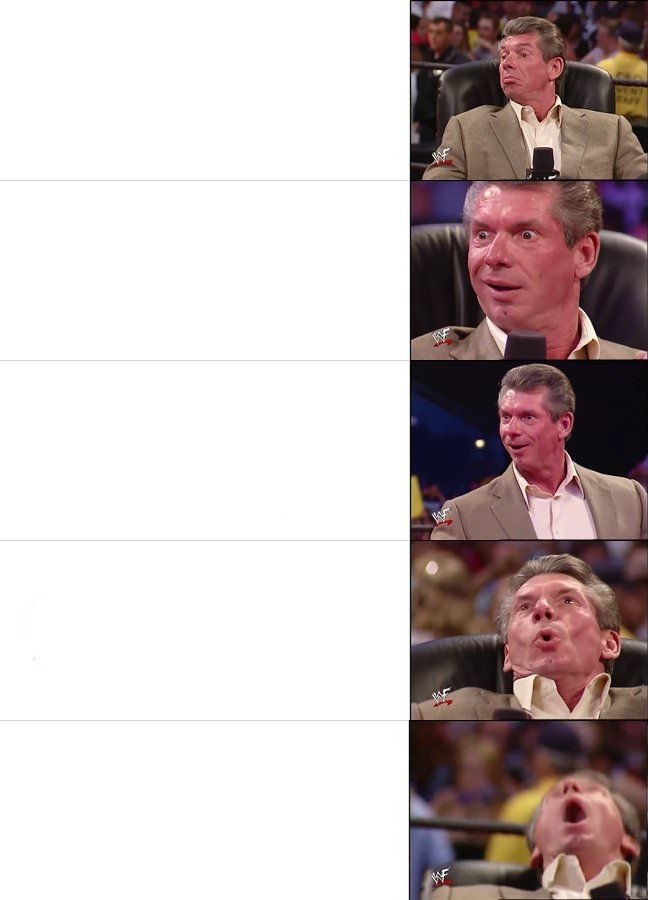
HTTP Timeouts
Fetch API
Web streams API
Test runner
Node.js 18 new features
const getData = async () => {
const response = await fetch('https://catfact.ninja/fact');
if (response.ok) {
const data = await response.json();
console.log(data);
} else {
console.error(`${response.status} ${response.statusText}`);
}
};
Fetch API
import {
ReadableStream
} from 'node:stream/web';
import {
setInterval as every
} from 'node:timers/promises';
import {
performance
} from 'node:perf_hooks';
const SECOND = 1000;
const stream = new ReadableStream({
async start(controller) {
for await (const _ of every(SECOND))
controller.enqueue(performance.now());
}
});
for await (const value of stream)
console.log(value);
Example ReadableStream
They are a way to handle reading/writing files, network communications, or any kind of end-to-end information exchange in an efficient way.

Using streams you read a file piece by piece
An example of a stream
const http = require('http')
const fs = require('fs')
const server = http.createServer(function(req, res) {
fs.readFile(__dirname + '/data.txt', (err, data) => {
res.end(data)
})
})
server.listen(3000)
If the file is big, the operation will take quite a bit of time. Here is the same thing written using streams
An example of a stream
const http = require('http')
const fs = require('fs')
const server = http.createServer((req, res) => {
const stream = fs.createReadStream(__dirname + '/data.txt')
stream.pipe(res)
})
server.listen(3000)
Instead of waiting until the file is fully read, we start streaming it to the HTTP client as soon as we have a chunk of data ready to be sent.
const getData = () => {
fetch('https://catfact.ninja/fact')
.then((response) => {
console.log('response.body =', response.body);
// response.body = ReadableStream { locked: false, state: 'readable', supportsBYOB: false }
return response.body;
})
.then((responseBody) => {
const reader = responseBody.getReader();
return new ReadableStream({
start(controller) {
/**
* Push handles each data chunk
*/
function push() {
/**
* @param {boolean} done - Whether the stream is done
* @param {Uint8Array} done - The stream state
*/
reader.read().then(({ done, value }) => {
// when there is no more data to read
if (done) {
console.log('done =', done);
// done = true
controller.close();
return;
}
// get the data and put it on queue
controller.enqueue(value);
// show the done state and the chunks value
console.log(done, value);
// false Uint8Array(124) [
// 123, 34, 102, 97, 99, 116, 34, 58, 34, 84, 97, 98,
// 98, 121, 32, 99, 97, 116, 115, 32, 97, 114, 101, 32...
// continue getting data
push();
});
}
// start getting data
push();
},
});
})
.then((stream) => {
// create a Response with the stream content
return new Response(stream, {
headers: { 'Content-Type': 'text/html' },
}).text();
})
.then((result) => {
console.log('result =', result);
// result = {"fact":"Tabby cats are thought to get their name from Attab,
// a district in Baghdad, now the capital of Iraq.","length":100}
});
};
getData();
Fetch API + Web Streams
import test from 'node:test';
import assert from 'assert';
test('asynchronous tests', async (t) => {
await t.test('passing test', (t) => {
assert.strictEqual(1, 1);
});
await t.test('failing test', (t) => {
assert.strictEqual(1, 2);
});
});
Experimental Test Runner
Productivity
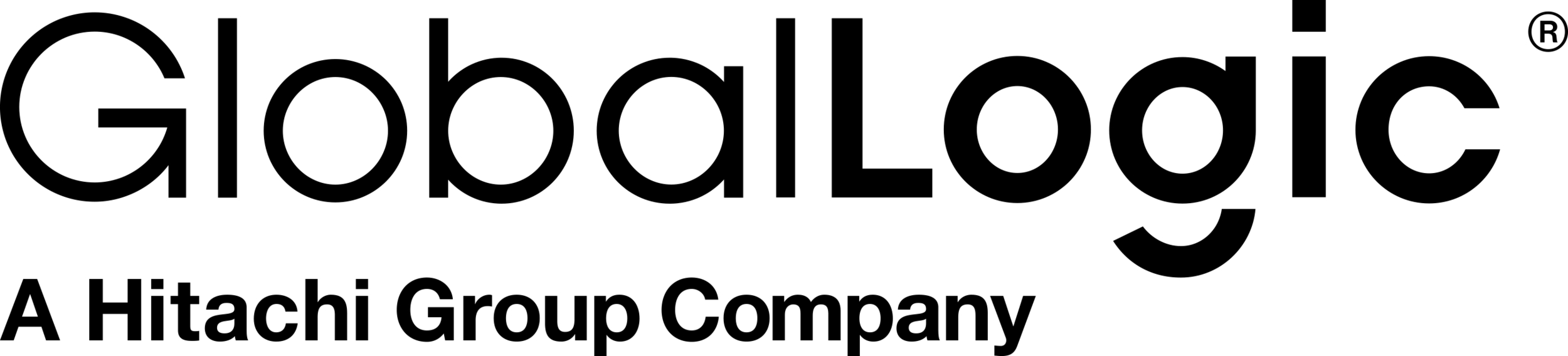
Deadlines
Priprity
Importancy
More guilty
Success?
Goals
Body
Contacts
Activity
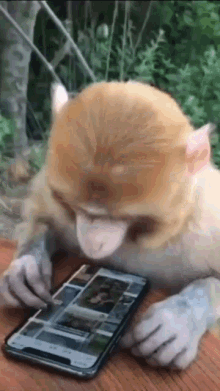

Libet's experiment: (0) repose, until (1) the Bereitschaftspotential is detected, (2-Libet's W) the volunteer memorizes a dot position upon feeling their intention, and then (3) acts.
Sleep well
7-9 hours
Hobbies
and interests
Communication
Nature
Sport
and movement
Less news
less scrolling
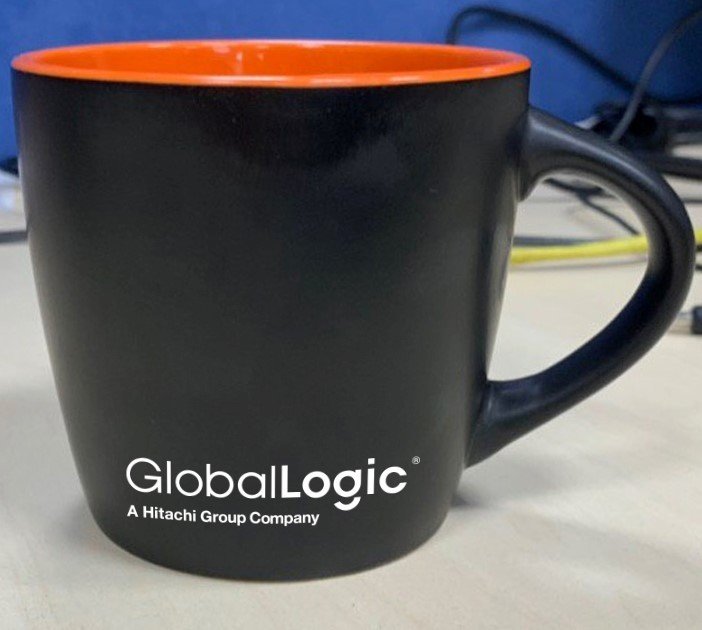
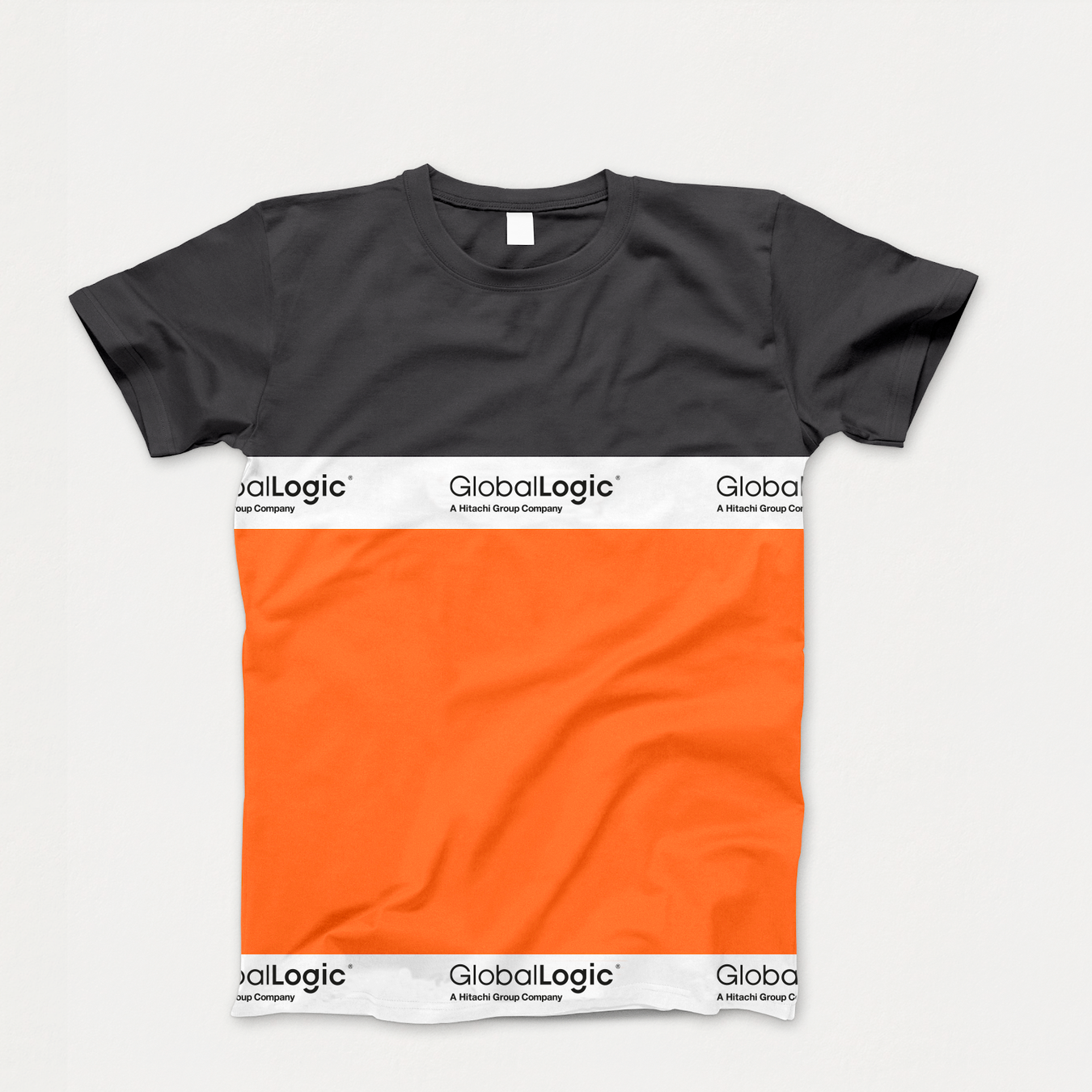
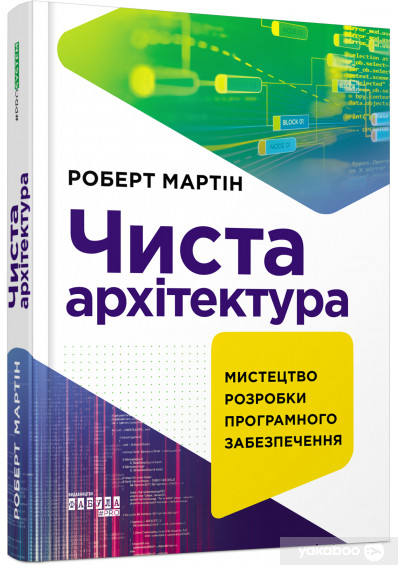
Quiz time
Thank you
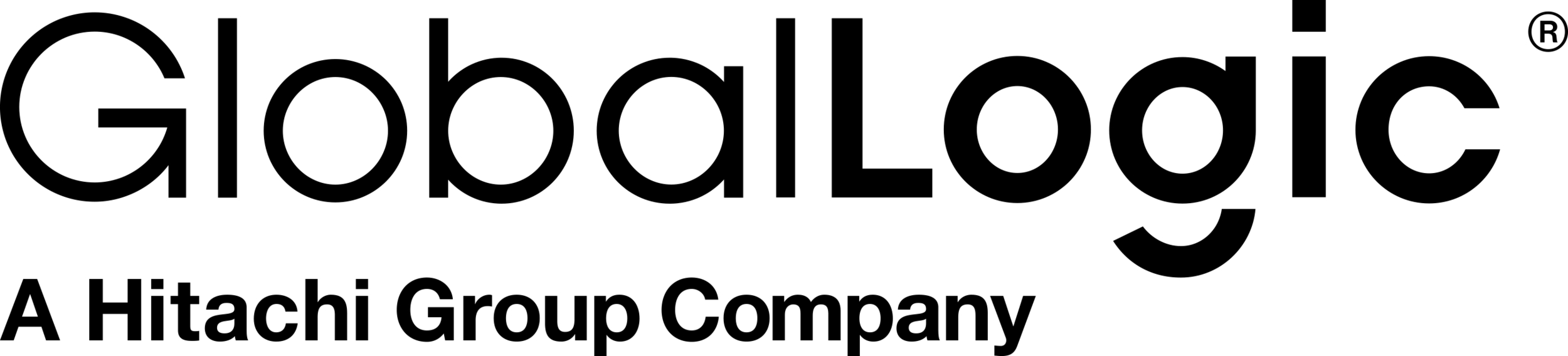
Back to work
By vovy
Back to work
- 160