MicroPython
@woakas CTO at Ubidots

WHAT???


3, 2, 1, Ready!

Each module is either written from scratch or ported from CPython.
The board

- Based on the STM32F405 microcontroller(32 bit ARM Cortex M4)
- CPU 168MHz
- 1MiB flash
- 192KiB RAM

Differences to CPython
- MicroPython does not implement complete CPython
- MicroPython implements only subset of functionality and parameters of particular functions and classes.
- print() function does not check for recursive data structures in the same way CPython does.
Libraries
- asyncio
- calendar
- datetime
- functools
- gzip
- ....
- ....

Examples

import pycom
import time
pycom.heartbeat(False)
for cycles in range(10): # stop after 10 cycles
pycom.rgbled(0x007f00) # green
time.sleep(5)
pycom.rgbled(0x7f7f00) # yellow
time.sleep(1.5)
pycom.rgbled(0x7f0000) # red
time.sleep(4)
REPL (Read-Eval-Print-Loop)

Led on / off
import pycom
import time
pycom.heartbeat(False)
for cycles in range(10): # stop after 10 cycles
pycom.rgbled(0x007f00) # green
time.sleep(5)
pycom.rgbled(0x7f7f00) # yellow
time.sleep(1.5)
pycom.rgbled(0x7f0000) # red
time.sleep(4)
On/OFF
import machine
led=machine.Pin("G16",machine.Pin.OUT)
led.value(1)
led.value(0)
Button
import machine
led = machine.Pin("G16", machine.Pin.OUT)
button = machine.Pin("G17", machine.Pin.IN, pull=machine.Pin.PULL_UP)
def handler(button):
led.toggle()
button.callback(trigger=machine.Pin.IRQ_FALLING, handler=handler)
import machine
button = machine.Pin("G17", machine.Pin.IN, pull=machine.Pin.PULL_UP)
button()
eval
import time
import machine
led = machine.Pin("G16", machine.Pin.OUT)
eval("led.value(0)")
Save program
a = """import time
from machine import Pin
led = Pin('G10', mode=Pin.OUT, value=0)
for i in range(10):
led.toggle()
time.sleep(0.1)
"""
with open('/flash/main.py', 'w') as f:
f.write(a)
Threads
import _thread
import time
def th_func(delay, id):
while True:
time.sleep(delay)
print('Running thread %d' % id)
for i in range(3):
_thread.start_new_thread(th_func, (i + 1, i))
WEEEE
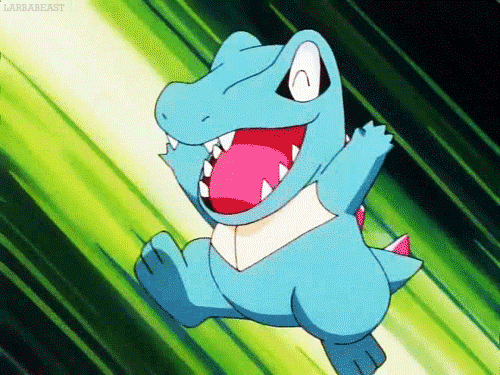
Ahora con IoT
Sigfox - LoRaWan
Sigfox
from network import Sigfox
import socket
import struct
# init Sigfox for RCZ1 (Europe)
sigfox = Sigfox(mode=Sigfox.SIGFOX, rcz=Sigfox.RCZ2)
# create a Sigfox socket
s = socket.socket(socket.AF_SIGFOX, socket.SOCK_RAW)
# make the socket blocking
s.setblocking(True)
# configure it as DOWNLINK specified by 'True'
s.setsockopt(socket.SOL_SIGFOX, socket.SO_RX, True)
# send values as little-endian and int, request DOWNLINK
s.send(struct.pack("<f", 23.2))
References
Python en microcontroladores
By Gustavo Angulo
Python en microcontroladores
- 1,570