Exiting the PHP Dark Ages
Or, welcome to 2014 I'll be your guide
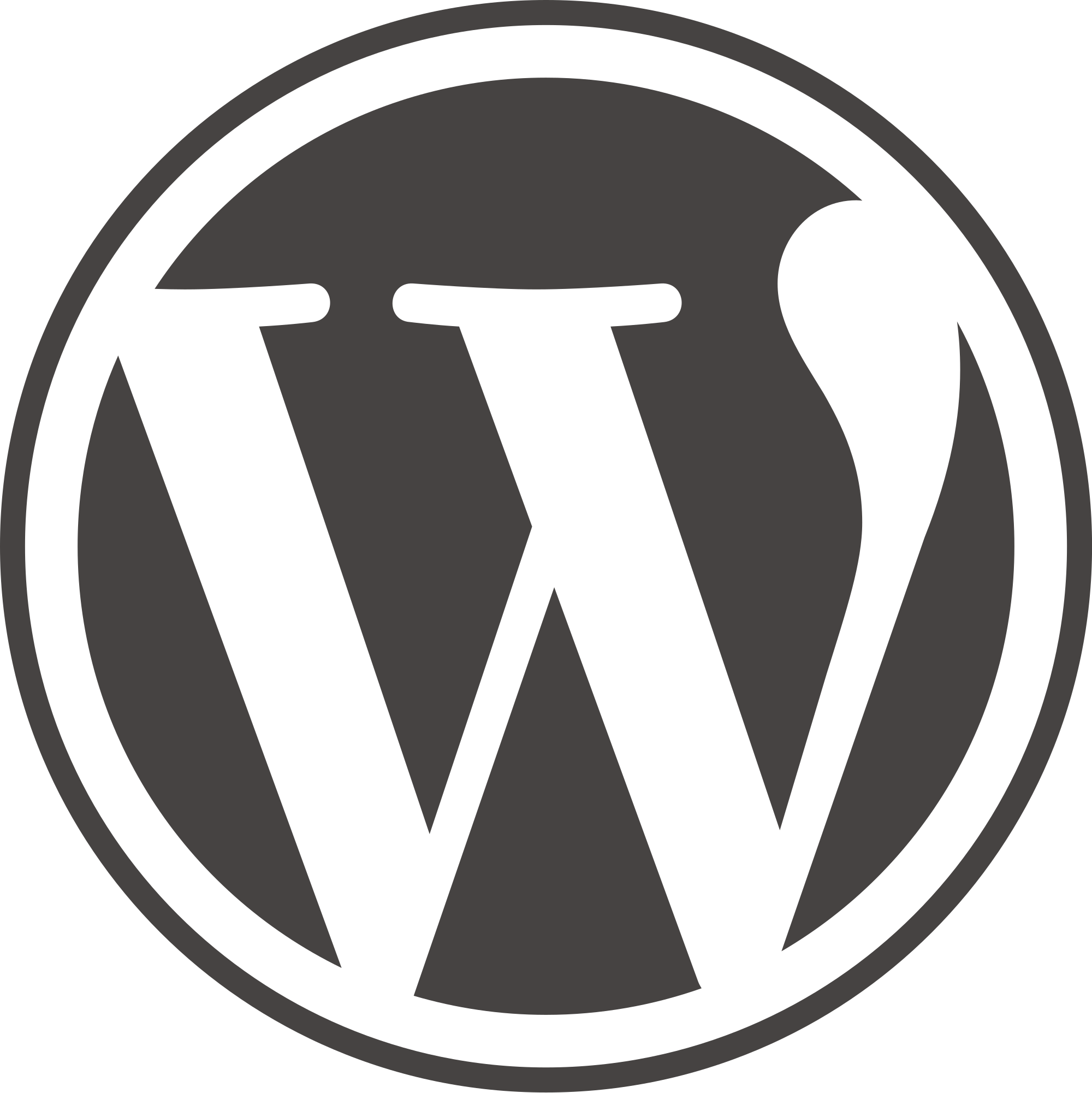
WordPress and PHP
- The project has officially supported 5.2 until now
- Many devs used newer features
- Plugin & Theme repository developers were locked to 5.2
- Most commercial developers were too, as they had to support everyone
WordPress 5.2 = PHP 5.6
- 🥇 First version bump in over 5 years!
- 👀 We get loads of new language constructs
- 👮🏼♀️ Interfaces! Enforce things, swap things!
- ⚙️ Traits, reflection, heaps more OOP tools
- 🎨 Nicer syntax, like short array syntax
- 🤷🏽♀️ Don't use everything just for the sake of it!
Be a part of modern PHP development (sort of)
wordpress.org/support

Short Arrays
<?php
/* Old Style */
$my_list = array(
'thing1' => 'my value',
'thing2' => 'my other value'
);
/* New style */
$my_list = [
'thing1' => 'my value',
'thing2' => 'my other value'
];
Short Arrays cont..
<?php
/* Old Style */
$my_list = array();
/* New style */
$my_list = [];
/* Nesting is neater */
$foo = [
[1,2,3,4,5],
[
'key1' => 'hey',
'key2' => 'listen!'
]
];
Anonymous Functions
<?php
/* Old Style */
function my_namespace_my_filter_function( $value ) {
return 'different value';
}
add_filter( 'the_title', 'my_namespace_my_filter_function', 10, 1 );
/* New style */
add_filter( 'the_title', function(){ return 'different value'; }, 10, 1 );
// Warning, can make it hard to unhook - use in custom projects, not distributed themes
Anon Functions & array_map
<?php
/* Old Style */
$animal_noises = array( 'woof', 'meow', 'chirp' );
function my_namespace_my_function( $param1 ){
if ( $param1 === 'woof' ){
return 'doggo';
} else {
return 'not a doggo'
}
}
$is_doggo_list = [];
foreach ( $animal_noises as $noise ) {
$is_doggo_list[] = my_namespace_my_function( $noise );
}
/* New style */
$animal_noises = array( 'woof', 'meow', 'chirp' );
$is_doggo_list = array_map( function( $noise ){
return ( $param1 === 'woof' ) ? 'doggo' : 'not a doggo';
}, $animal_noises );
Thanks
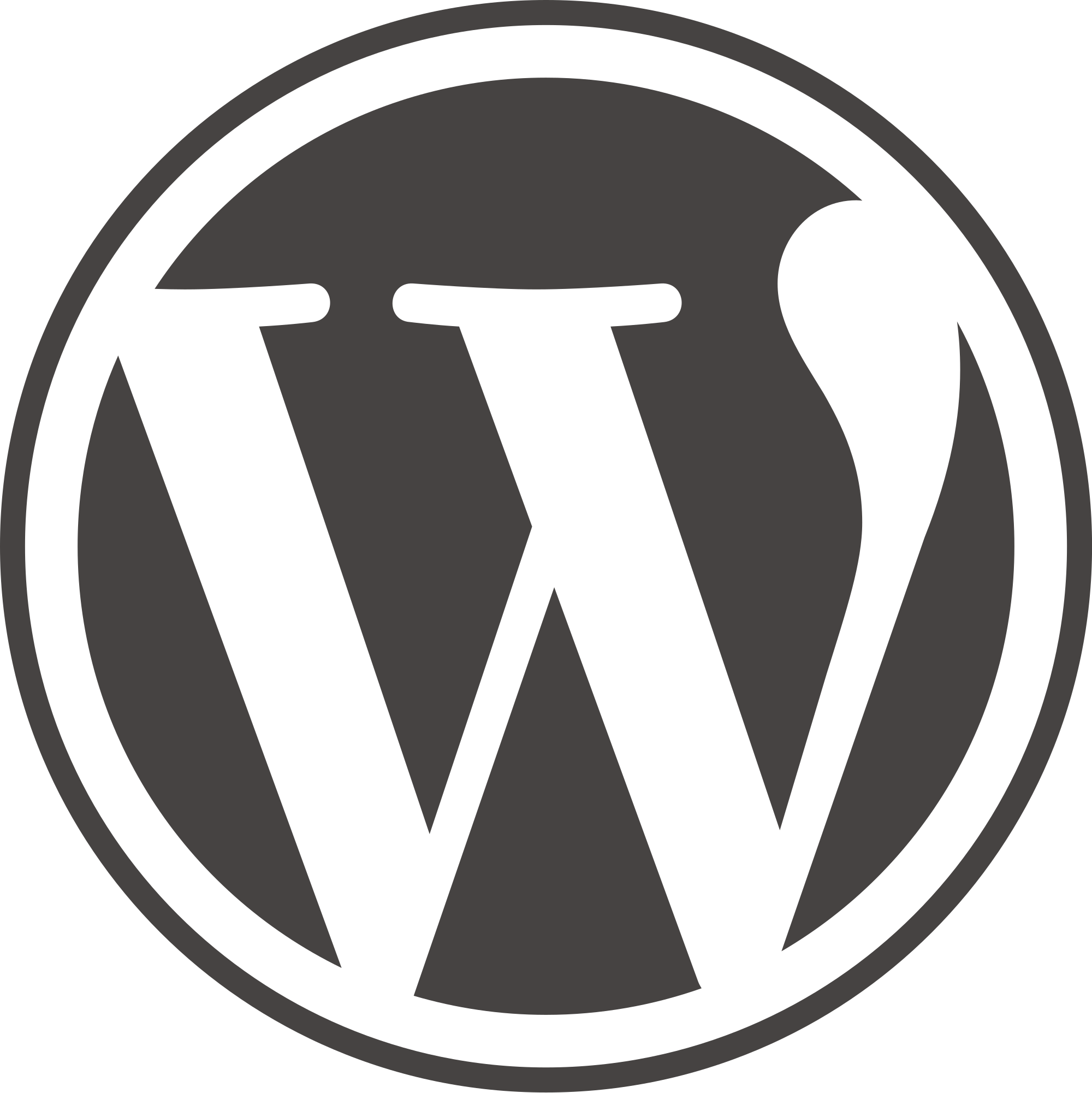
WIP! WordPress and Modern PHP - WordPress Adelaide
By WordPress Adelaide
WIP! WordPress and Modern PHP - WordPress Adelaide
or 'Welcome to 2014 I'll be your guide'
- 525