Release monitor integration front/back
ECA Node/React - June 2019
Plan
- Backend
- Enable CORS
- Test
- Frontend
- Fetch data
- Introduction to lifecycle hooks
- Add a service
- Testing in React
Enable CORS for frontend
npm i cors
install dependencies
Change mongoose model
const baseVersionSchema = new mongoose.Schema({
service_name: String,
service_version: String,
env: String
})
const baseVersion = new BaseVersionModel({
service_name: req.body.service_name,
service_version: buildVersion,
env: req.body.env
})
Backend test
npm i --save-dev jest supertest
npm i --save-dev eslint-plugin-jest
install dependencies
test
// in package.json add this section
"jest": {
"testEnvironment": "node"
}
// in .eslintrc.js, add plugins:
'plugin:jest/recommended'
configure jest
test with supertest
const app = require('../src/app')
const request = require('supertest')
it('should return hello world', async function() {
const response = await request(app).get('/hello-world')
expect(response.text).toEqual('hello world')
})
helloworld
test with mongodb-memory-server
npm install mongodb-memory-server --save-dev
const { MongoMemoryServer } = require('mongodb-memory-server')
const app = require('../../src/app')
const request = require('supertest')
const mongoose = require('mongoose')
let mongod
beforeEach(async () => {
mongod = new MongoMemoryServer()
const uri = await mongod.getConnectionString()
await mongoose.connect(uri)
})
afterEach(async () => {
mongod.stop()
})
test with nock
npm install nock --save-dev
nock('https://www.egencia.com')
.get('/flight-service/base/version')
.reply(200, {
application_name: 'flight-service',
active_profiles: 'prod,prodch,ancillaries,oms',
stage: 'prod',
machine_name: 'chexjvgect004',
application_data: {
step_bom_version: '8.6.2',
},
build_version: '2019-05-27-15-15-94093f32a',
build_number: '94093f32a449b31104c7c2767ee5fd2a42447b99',
build_date: '2019-05-27T15:17:025Z',
})
.persist()
add more tests
- test on POST /services
- test on GET /services/:env
Create an interface
import axios from 'axios'
export default {
from(url) {
return {
get: ({ name }) => axios.get(`${url}/${name}`),
list: () => axios.get(url),
add: body => axios.post(url, body),
delete: ({ name }) => axios.delete(`${url}/${name}`),
}
},
}
Lifecycle hooks

constructor(props) {
super(props)
this.state = {
inputValue: '',
services: [],
}
this.addService = this.addService.bind(this)
}
componentDidMount() {
this.fetchServices(this.props.env)
}
async fetchServices(env) {
const { data } = await api.from(`http://localhost:3000/services/${env}`).list()
this.setState({ services: data})
}
src/components/List.jsx
fetch services
async addService(serviceName) {
await api.from(`http://localhost:3000/services/`).add({
service_name: serviceName,
env: this.props.env
})
this.fetchServices(this.props.env)
}
src/components/List.jsx
add services
Testing your React application
npm i --save-dev @testing-library/react
npm i --save-dev @testing-library/jest-dom
##also if you do not have jest already
# npm i --save-dev jest
"scripts": {
"start": "parcel index.html --open",
"build": "parcel build index.html",
"test": "jest --watchAll"
}
package.json
Write tests. Not too many. Mostly integration.
src/__tests__/AddService.test.js
import React from 'react'
import { render, fireEvent, cleanup } from '@testing-library/react'
import AddService from '../components/AddService'
afterEach(cleanup)
test('create a new service', () => {
//given
const handleSubmit = jest.fn()
const { getByPlaceholderText, getByText } = render(
<AddService addService={handleSubmit} />,
)
getByPlaceholderText(/new/i).value = 'new-service'
//when
fireEvent.click(getByText(/Add/i))
//then
expect(handleSubmit).toHaveBeenCalledTimes(1)
})
Thank you everyone !
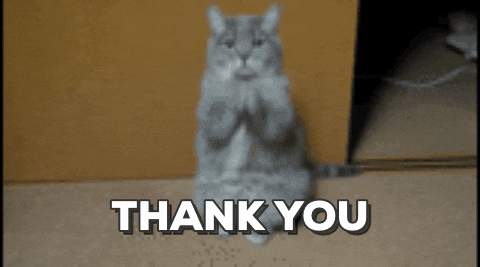
FullstackJS Academy - course 6
By yagong
FullstackJS Academy - course 6
- 742