Desarrollo de aplicaciones web con ASP.NET
Yhoan Galeano

Web Developer
- ¿Cómo funciona la web?
- ¿Que es ASP.NET y para qué sirve?
- Introducción a C#
- Code cooking!
first things first...
Comunicación



Emisor
Canal
Mensaje
Receptor
Respuesta
Cliente-Servidor

http request
http response
(HTML)
HTTP
HTML
(Hyper Text Transfer Protocol)
(Hyper Text Markup Language)
¿Qué es una aplicación web?
Pero entonces..
Cliente
- HTML
- CSS
- JavaScript
Servidor
- ASP.NET
- Ruby on Rails
- JAVA
- NodeJS
- Python
- Otros...
Cliente

HTML
HEAD(CABECERA)
BODY(CUERPO)
FOOTER(Pie de página)
<etiquetaHTML>
...contenido
</etiquetaHTML>
Estructura de etiquetas
<html>
<head>
<meta charset="utf-8">
<title>Mi Título</title>
</head>
<body>
Mi primera página en HTML
</body>
</html>
Algunas etiquetas...
|
Botón |
|
Tabla |
Caja de texto |
<button>
Click aquí
</button>
<table>
<tr>
<td>Celda 1</td>
<td>Celda 2</td>
</tr>
<tr>
<td>Celda 3</td>
<td>Celda 4</td>
</tr>
</table>
<input></input>
|
Enlace |
|
Lista |
|
Bloque contenedor |
<a href="http://www.microsoft.com">
Soy un enlace a Microsoft
</a>
<ul>
<li>
Item 1
</li>
<li>
Item 2
</li>
</ul>
<div>
<button>
Soy un boton contenido
en un bloque.
</button>
</div>
Atributos
id | Identificador |
name | Nombre |
class | Clase |
style | Estilo |
width | Ancho |
height | Alto |
<etiqueta atributo="valor-atributo">
</etiqueta>
<html>
<head>
<meta charset="utf-8">
<title>JS Bin</title>
</head>
<body>
<table id="MiTablaID" name="miTablaNombre" style="border-collapse: collapse;">
<tr >
<td style="border:1pt solid black">
Nombre
</td>
<td style="border:1pt solid black">
Edad
</td>
<td style="border:1pt solid black">
Teléfono
</td>
</tr>
<tr>
<td style="border:1pt solid black">
Pepito Perez
</td>
<td style="border:1pt solid black">
25
</td>
<td style="border:1pt solid black">
555555
</td>
</tr>
</table>
</body>
</html>
CSS
(Cascade Style Sheet)

Selectores
-
Por identificador (#)
-
Por clase (.)
-
Por etiqueta
<style>
.nombreClaseEtiqueta{
clausulas css...
}
#idEtiqueta{
clausulas css...
}
etiqueta{
clausulas css...
}
</style>
JavaScript

- Client Side
- Orientado a Objetos
- Case Sensitive

function Hello(){
var miVariable= "Hola JavaScript";
var miNumero = 2 + 2;
alert(miVariable);
alert(miNumero);
}




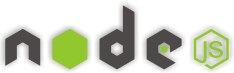
Servidor
Framework de .NET

(Active Server Pages)
ASP
- VBScript
- Lenguaje interpretado
- Extensión ASP
- Difícil depuración
ASP.NET
- Visual Basic, C#, F#, and C++
- Lenguaje compilado
- Extensión ASPX
- Fácil depuración
- Orientado a objetos
- Librerías de .NET

Además de...
- IIS (Internet Information Services)
- CIL (Common Intermediate Language)
- CLR (Common Language Runtime)

IIS
Internet Information Services
Common Intermediate Language (CIL)
- Lenguaje intermedio resultado de compilar un lenguaje compatible con .NET
- DLL(Dynamic Link Library)
Common Language Runtime(CLR)
Escribe una vez, ejecuta donde quieras...
- CIL a Lenguaje máquina
- Just In Time (JIT)
- Seguridad
- Recolección de basura(Garbage Collector)
- Versionamiento
- Excepciones
- Administración de memoria
Ciclo de vida
- Init
- Load
- PreRender
- Render
- Dispose
- Unload
Estructura de un proyecto ASP.NET

Configuration
Web.config
XML Web Services
(asmx)
Formularios Web
(aspx)
ó
Code Behind
(C#,VB, J#, F#)
<%@ Page language="c#" %>
<html>
<head>
<title>Hora.aspx</title>
</head>
<script runat="server">
public void Button_Click(object sender, System.EventArgs e) {
LabelHora.Text = "La hora actual es " + DateTime.Now;
}
</script>
<body>
<form method="post" runat="server">
<asp:Button onclick="Button_Click" runat="server"
Text="Pulse el botón para consultar la hora" />
<p>
<asp:Label id=LabelHora runat="server" />
</form>
</body>
</html>
Default.aspx (In line)
<%@ Page Language="C#" AutoEventWireup="true"
CodeFile="Default.aspx.cs" Inherits="Default" %>
<html>
<head>
<title>Hora.aspx</title>
</head>
<body>
<form method="post" runat="server">
<asp:Button onclick="Button_Click" runat="server"
Text="Pulse el botón para consultar la hora" />
<p>
<asp:Label id=LabelHora runat="server" />
</p>
</form>
</body>
</html>
Default.aspx (Code behind)
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.UI;
using System.Web.UI.WebControls;
using System.Data.SqlClient;
namespace WebPractice
{
public partial class _Default : System.Web.UI.Page
{
protected void Page_Load(object sender, EventArgs e)
{
}
public void Button_Click(object sender, System.EventArgs e) {
LabelHora.Text = "La hora actual es " + DateTime.Now;
}
}
}
Default.aspx.cs
Cooking code!

Clase 1 ASP.NET
By Yhoan Andres Galeano Urrea
Clase 1 ASP.NET
¿Cómo funciona la web?, ¿Que es ASP.NET y para qué sirve?, Introducción a C#
- 658