Fibers with Twitter
#tweets that don't break
Zak Burki
27th Feb 2015
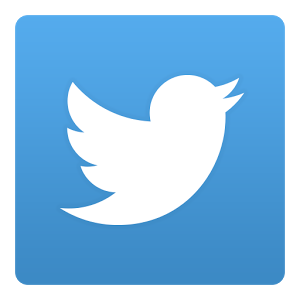
Game Plan
- Grab Some Tweets from Twitter users
- Insert Into Database Collection
- Show them on Page
- Try not to break.
Twit Package (meteor add mrt:twit)
Twit = new TwitMaker({
consumer_key: 'key',
consumer_secret:'secret',
access_token: 'token',
access_token_secret:'secret'
});
Let's make a new Twitter API Call:
Twit.get('statuses/user_timeline',
{ screen_name: 'Oprah',
count: 1,
include_rts : false,
},
function (err, data){
if(!err) console.log(data);
}
);
Set Twitter API Credentials in a new Instance:
Twit Package
...what happens when we try and insert the returned tweet data into the Database?

EPIC FAIL
"Meteor Code must always run within a Fiber."
Wait a second...
...what's going on here?

What is a Fiber?
- Meteor is built upon Node.js
- Node.js has an single Event Loop that performs tasks (single thread)
- But you'll come across lots of async Functions that are Non-Blocking.
- Callbacks are used to execute tasks later when other tasks are complete.
You still haven't explained Fibers!
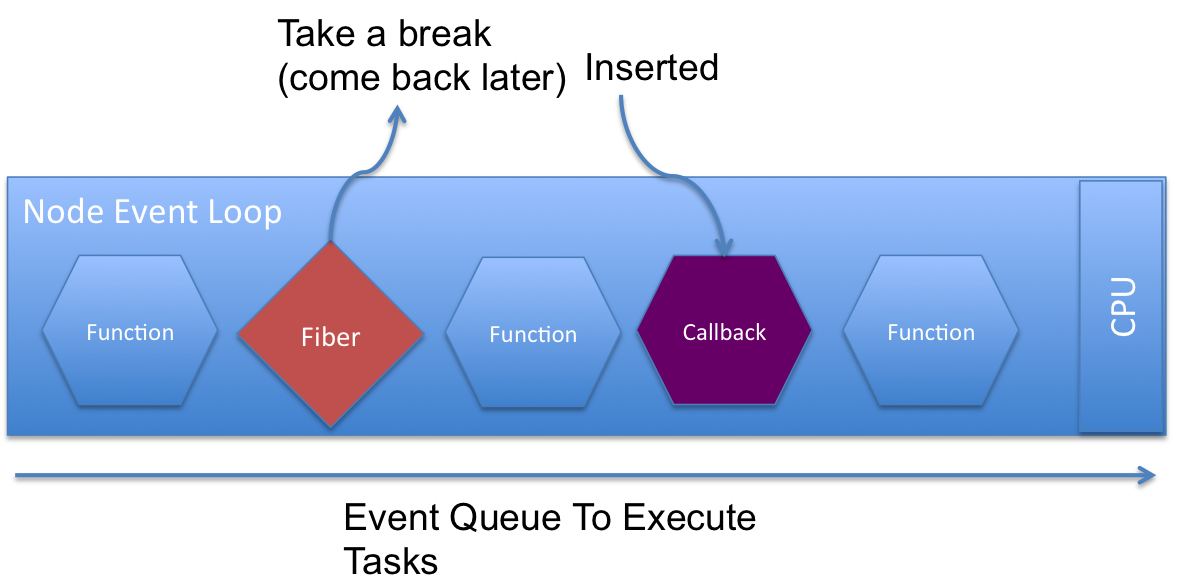
!!!Fibers???!
Fibers are Meteor's way of accessing the Node.js Event Loop and executing functions in sequence.
...but with the benefits of asynchronous, non-blocking code.
Fibers are containers that have the power to halt execution and yield control to other functions.
To the Rescue...
Meteor.bindEnvironment
or
Meteor.wrapAsync
Meteor.wrapAsync:
Wrap a function that takes a callback function as its final parameter. On the server, the wrapped function can be used synchronously.
Meteor.bindEnvironment:
Code Must run in a Fiber? Use bindEnvironment on the callback and Meteor will create a new Fiber for that callback function.
.wrapAsync Error Handling
The .wrapAsync method will convert the wrapped function into something synchronous looking.
You probably decided to do it this way to avoid callback soup hell if you have a lot of nested callbacks.
.wrapAsync Error Handling
Keep it Synchronous..
Use a Try/Catch Statement
try {
var results = getTweetsTwitter(user);
addTweets(user, results);
}
catch(err){
console.log("Error Getting Tweets", err);
}
Any Questions?
Source Code:
bitbucket.org/zakir2k/tweetgrab
(pls use your own twitter api credentials)
Follow Me:
@zakburki
Meetup Group:
codelearn-hk
Fibers with Twitter
By Zak Burki
Fibers with Twitter
Meteor HK - Feb Slide Deck
- 2,563