naming conventions and code formatting
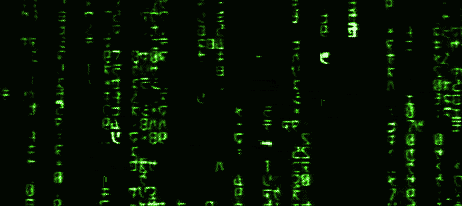
There are only two hard things in Computer Science: cache invalidation and naming things
Phil Karlton

Naming conventions
a = b * c;
vs
weekly_pay = hours_worked * hourly_pay_rate;
Naming conventions
Why?
- Reduce the effort needed to read and understand source code
- Enable code reviews to focus on more important issues than arguing over syntax and naming standards.
Brevity
Reasons
- early source code editors lacking autocomplete
- early low-resolution monitors with limited line length (e.g. only 80 characters)
- much of computer science originating from mathematics, where variable names are traditionally only a single letter
Word conventions
- camelCase
- PascalCase
- snake_case
- kebab-case
Python conventions
construct | convention |
---|---|
Class | CapWords |
Functions | lowercase |
Methods | lowercase |
Type variables | CapWords |
Constants | UPPERCASE |
Package | lowercase |
Module | lowercase |
Python conventions
_single_leading_underscore: weak "internal use" indicator. E.g. from M import * does not import objects whose name starts with an underscore.
Python conventions
>>> class Test(object):
... def __mangled_name(self):
... pass
... def normal_name(self):
... pass
>>> t = Test()
>>> [attr for attr in dir(t) if 'name' in attr]
['_Test__mangled_name', 'normal_name']
Any identifier of the form __spam (at least two leading underscores, at most one trailing underscore) is textually replaced with _classname__spam, where classname is the current class name with leading underscore(s) stripped.
Python conventions
PEP8
- PEP 8 is a document that provides guidelines and best practices on how to write Python code. It was written in 2001 by Guido van Rossum, Barry Warsaw, and Nick Coghlan.
- PEP stands for Python Enhancement Proposal, and there are several of them. A PEP is a document that describes new features proposed for Python and documents aspects of Python, like design and style, for the community.
Python conventions
PEP8
https://www.python.org/dev/peps/pep-0008/
https://development.robinwinslow.uk/2014/01/05/summary-of-python-code-style-conventions/
Formatting
Black

Formatting
Black (Why?)
https://github.com/django/deps/blob/master/accepted/0008-black.rst
Formatting
Black
- Black is the uncompromising Python code formatter. By using it, you agree to cede control over minutiae of hand-formatting. In return, Black gives you speed, determinism, and freedom from pycodestyle nagging about formatting. You will save time and mental energy for more important matters.
- Blackened code looks the same regardless of the project you're reading. Formatting becomes transparent after a while and you can focus on the content instead.
- Black makes code review faster by producing the smallest diffs possible.
Formatting
isort
https://github.com/timothycrosley/isort
Formatting
isort + black in our CI pipeline
Rafał
1. Oddzialenie enterami ściany tekstu | black! |
wcięcia, długość linii |
black! |
3. help_texty w modelach | tak! |
4. dodawanie docs stringów do metod, klas | https://confluence.3bpo.com/display/IG/Dokumentowanie+kodu |
5. dodanie komentarza | jak powyżej |
6. unikanie skrótów myślowych | tak ale.. |
Clean Code

Clean Code
Use intention revealing names
The name of a variable, function, or class, should answer all the big questions. It should tell you why it exists, what it does, and how it is used. If a name requires a comment, then the name does not reveal its intent.
int d; // elapsed time in days
int elapsedTimeInDays;
int daysSinceCreation;
int daysSinceModification;
int fileAgeInDays;
Clean Code
Avoid disinformation
Avoid leaving false clues that obscure the meaning of code.
Clean Code
Make meaningful distinctions
Avoid leaving false clues that obscure the meaning of code.
class ProductInfo
class ProductData
void getActiveAccount()
void getActiveAccounts()
void getActiveAccountsInfo()
Clean Code
Use pronounceable names
If you can’t pronounce it, you can’t discuss it without sounding like an idiot. “
Date genymdhms;
Date modymdhms;
Int pszqint;
Clean Code
Use searchable names
Single-letter names and numeric constants have a particular problem in that they are not easy to locate across a body of text.
Clean Code
Avoid encodings
PhoneNumber phoneString;
client_names_list = ['John', 'Jack']
client_names: List[str] = ['John','Jack']
Clean Code
Classes
Classes and objects should have noun or noun phrase names like Customer, WikiPage, Account, and AddressParser. Avoid words like Manager, Processor, Data, or Info in the name of a class. A class name should not be a verb.
Clean Code
Methods
Methods should have verb or verb phrase names like postPayment, deletePage, or save.
- Reread your code before submitting
- Read quality code on github
“Any fool can write code that a computer can understand. Good programmers write code that humans can understand.”
Martin Fowler
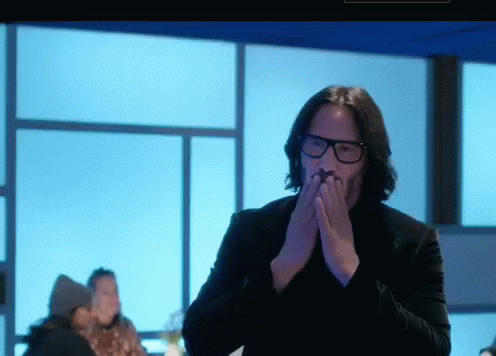
Thank You
naming
By zqzak
naming
- 308