Vue.js
The Progressive JavaScript Framework
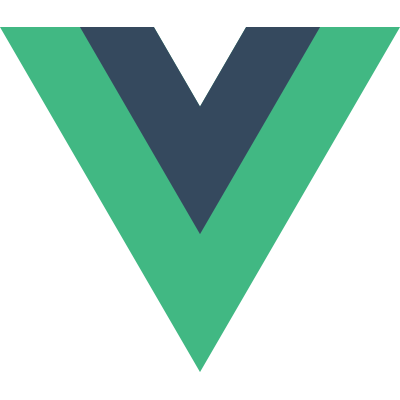
- Initially released in February 2014 by Evan You
- Latest stable version is 2.5.16
-
The first Official Vue.js Conference in the world was organized in Wrocław
- npm trends
Vue.js
The Progressive JavaScript Framework
Core concepts
Data driven view (reactive)

Core concepts
Components

Single File Components

A taste of the framerork
What is more ?
Lifecycle hooks
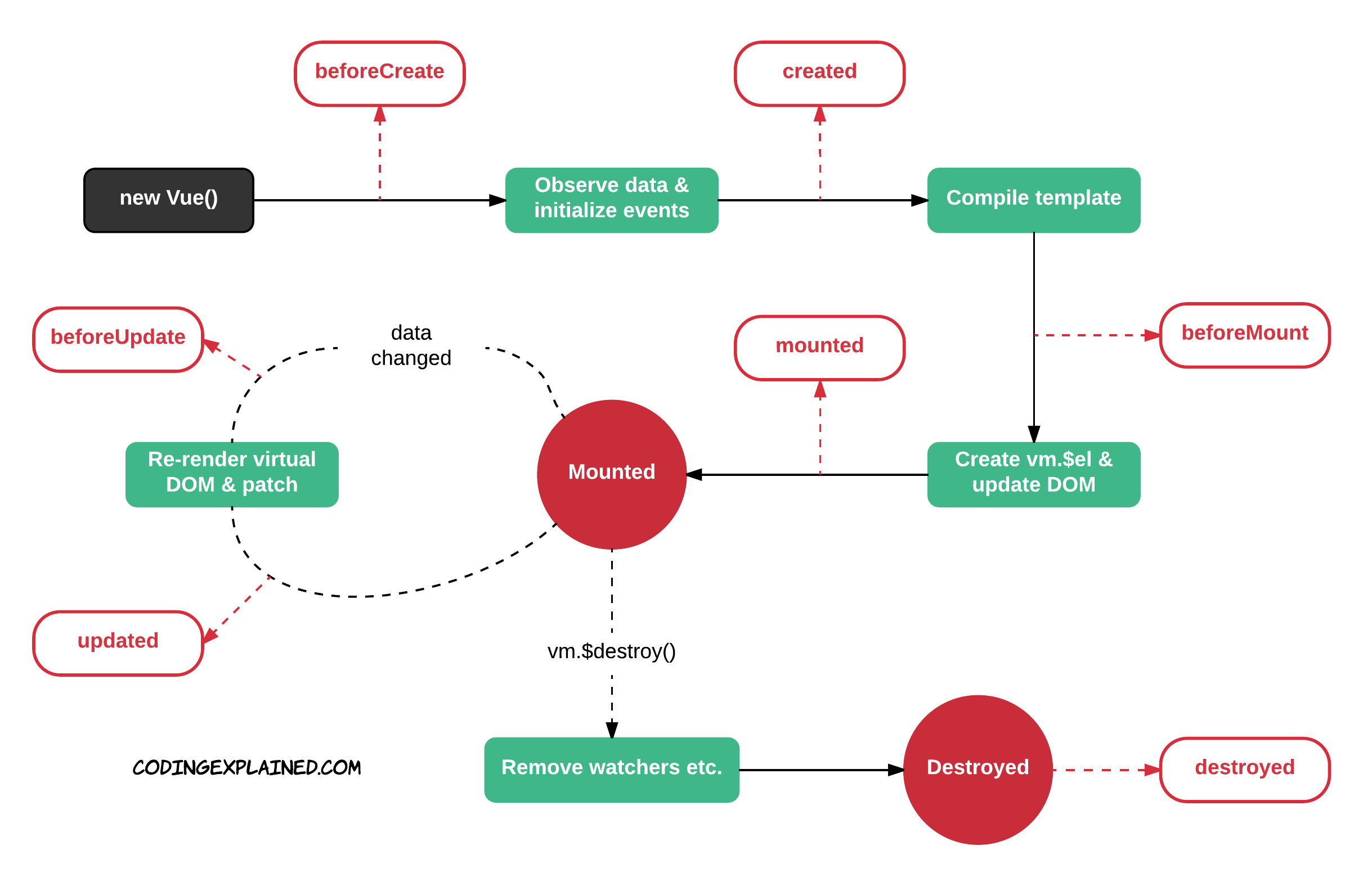
What is more ?
Computed Properties
<div id="example">
<p>Original message: "{{ message }}"</p>
<p>Computed reversed message: "{{ reversedMessage }}"</p>
</div>
var vm = new Vue({
el: '#example',
data: {
message: 'Hello'
},
computed: {
// a computed getter
reversedMessage: function () {
// `this` points to the vm instance
return this.message.split('').reverse().join('')
}
}
})
What is more ?
Class and Style Bindings
<div class="static"
v-bind:class="{ active: isActive, 'text-danger': hasError }">
</div>
data: {
isActive: true,
hasError: false
}
<div class="static active"></div>
Plugins
Vue.js devtools

Plugins
vue-router
import Vue from 'vue'
import Router from 'vue-router'
import Hello from '@/components/Hello'
import About from '@/components/About'
Vue.use(Router)
export default new Router({
routes: [
{
path: '/',
name: 'Hello',
component: Hello
},
{
path: '/about',
name: 'About',
component: About
}
]
})
Plugins
Vuex
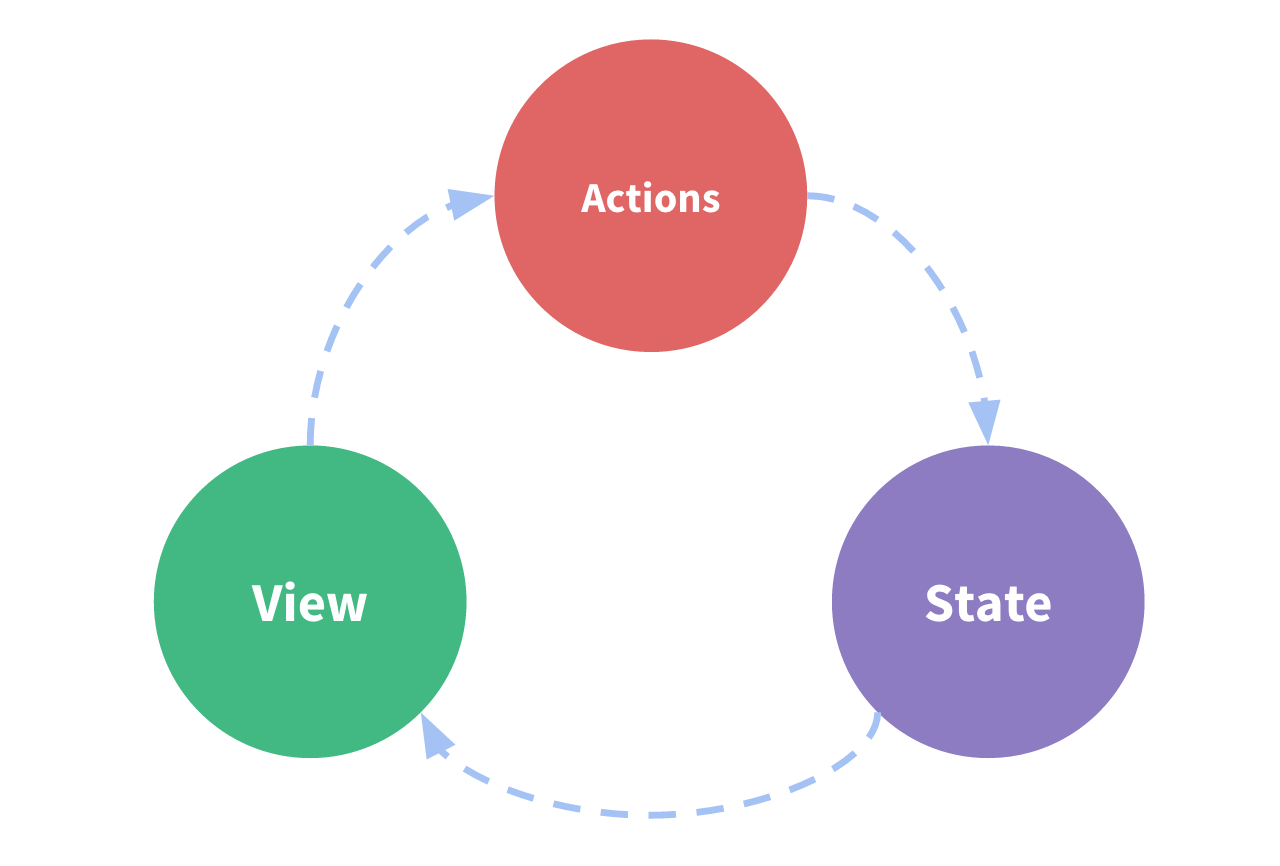
https://vuejs.org/
Bulding large apps
SPA
A single-page application (SPA) is a web application or web site that interacts with the user by dynamically rewriting the current page rather than loading entire new pages from a server. This approach avoids interruption of the user experience between successive pages, making the application behave more like a desktop application.
Bulding large apps
modularization (es6 modules)
function addResult() { ... }
function updateScoreboard() { ... }
export { addResult, updateScoreboard };
import * as scoreboard from ‘./scoreboard.js’;
scoreboard.updateScoreboard();
Bulding large apps
source transfomation, transpiling, bundling (webpack)

ES6
- Default Parameters in ES6
// default parameters
var link = function(height = 50, color = 'red', url = 'http://azat.co') {
...
}
// template literals
var name = `Your name is ${first} ${last}.`
var url = `http://localhost:3000/api/messages/${id}`
// arrow functions
$('.btn').click((event) =>{
this.sendData()
})
// spread operator
function myFunction(x, y, z) { }
var args = [0, 1, 2];
myFunction(...args);
Modern JS ecosystem
es6
webpack (bundler)
eslint (linter)
babel (transpiler)
yarn/npm (package manager)
sass/less/css modules (styling)
jest/jasmine/mocha (testing)
vue/react/angular (framework)

Vue.js
By zqzak
Vue.js
- 284