Thinking with JS
Patterns Inspired from the foundations of Computer Science
Lambda λ Calculus

Variable/expression
x
const x
Abstraction
λx.x
x => x; // or function(x) { return x }
λx.x²
x => x ** 2;
λx.λy.x
function (x) { return function(y) { return x } }
doesn't matter what the variable is called
Function Application
λx.x 10
(x => x)(10); // or (function(x) { return x })(10)
λx.x²(y)
const SQUARE = x => x ** 2; SQUARE(x)
Boolean?
λx.λy.x
λx.λy.y
TRUE
FALSE
So how would we do IF
IF
λz.λx.λy.z x y
const IF = COND => THEN => ELSE => COND(THEN)(ELSE);
Can you do numbers?
export function noop() {}
export function id(x) {
return x
}
export function constant(value) {
return () => value
}
export function unary(fn) {
return a => fn(a)
}
export function binary(fn) {
return (a, b) => fn(a, b)
}
export function apply(fn) {
return args => fn(...args)
}
Create some functional functions
Learn More about functional programming
References for these slides
State Machines

Finite-state machine
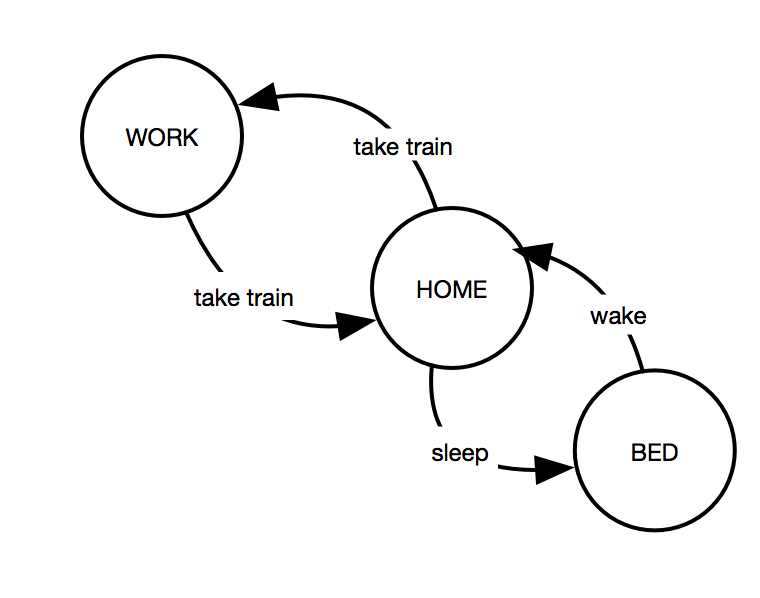
Finite-state machine
const NEXT_MOVE = {
WORK: 'HOME',
HOME: 'BED',
BED: 'HOME'
}
// ...
let currentMove = 'WORK';
NEXT_MOVE[CURRENT_MOVE] // 'HOME'
NEXT_MOVE[NEXT_MOVE[CURRENT_MOVE]] // 'BED'
Consider
// ...
let currentMove = 'WORK';
if (currentMove == 'WORK') {
currentMove = 'BED'
}
// NEXT_MOVE[NEXT_MOVE[CURRENT_MOVE]]
// is more expressive and shorter
Design Pattern Strategy
const STRATEGY = {
WORK: () => { ... },
HOME: () => { ... },
BED: () => { ... },
DEFAULT: () => { ... },
}
const currentState = 'WORK'
(STRATEGY[currentState] || STRATEGY.DEFAULT)()
Thinking with JS:
By Amr Draz
Thinking with JS:
- 103