Javascript Fundamentals

Part II

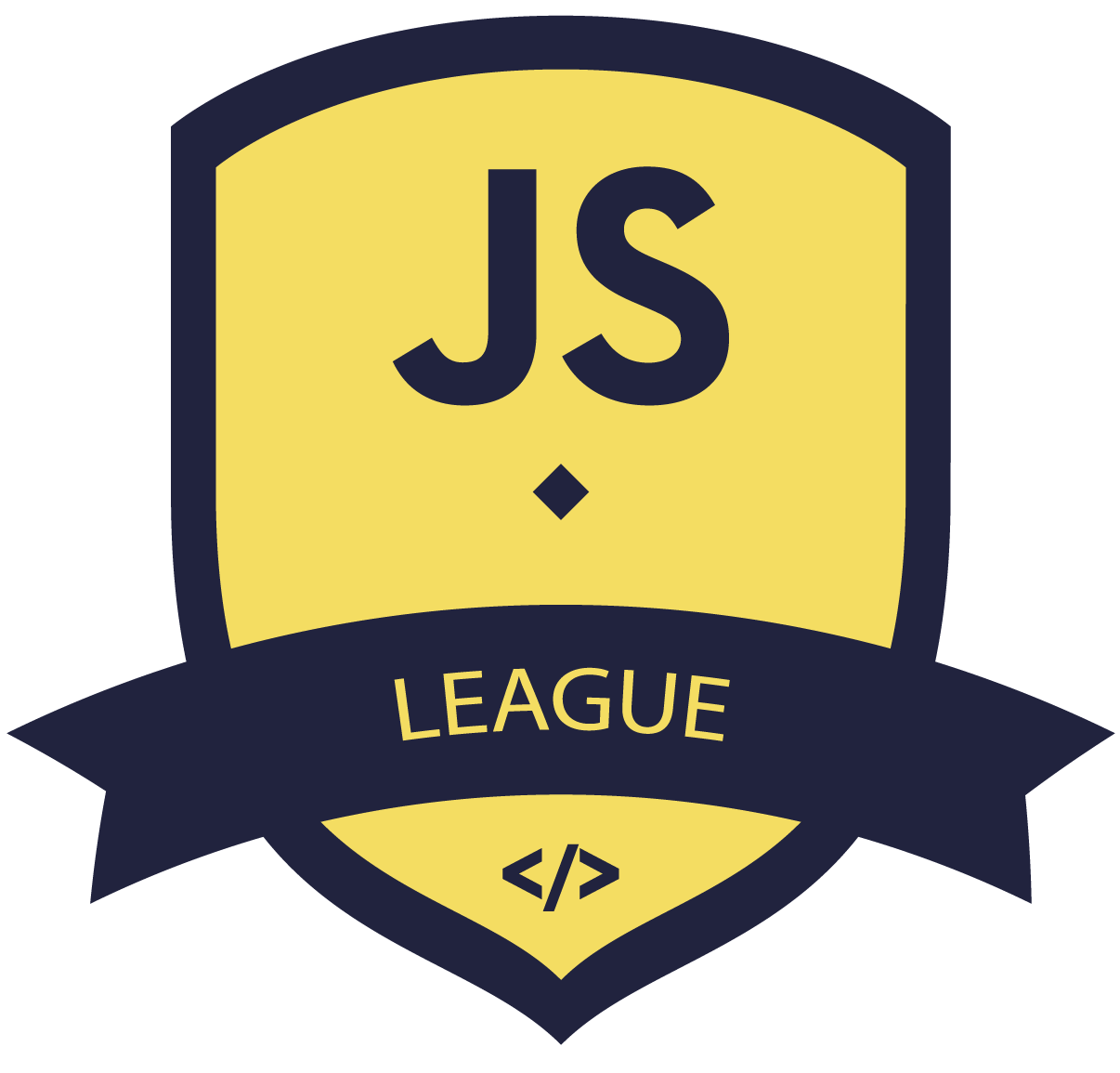
Overview
JavaScript Fundamentals (II)
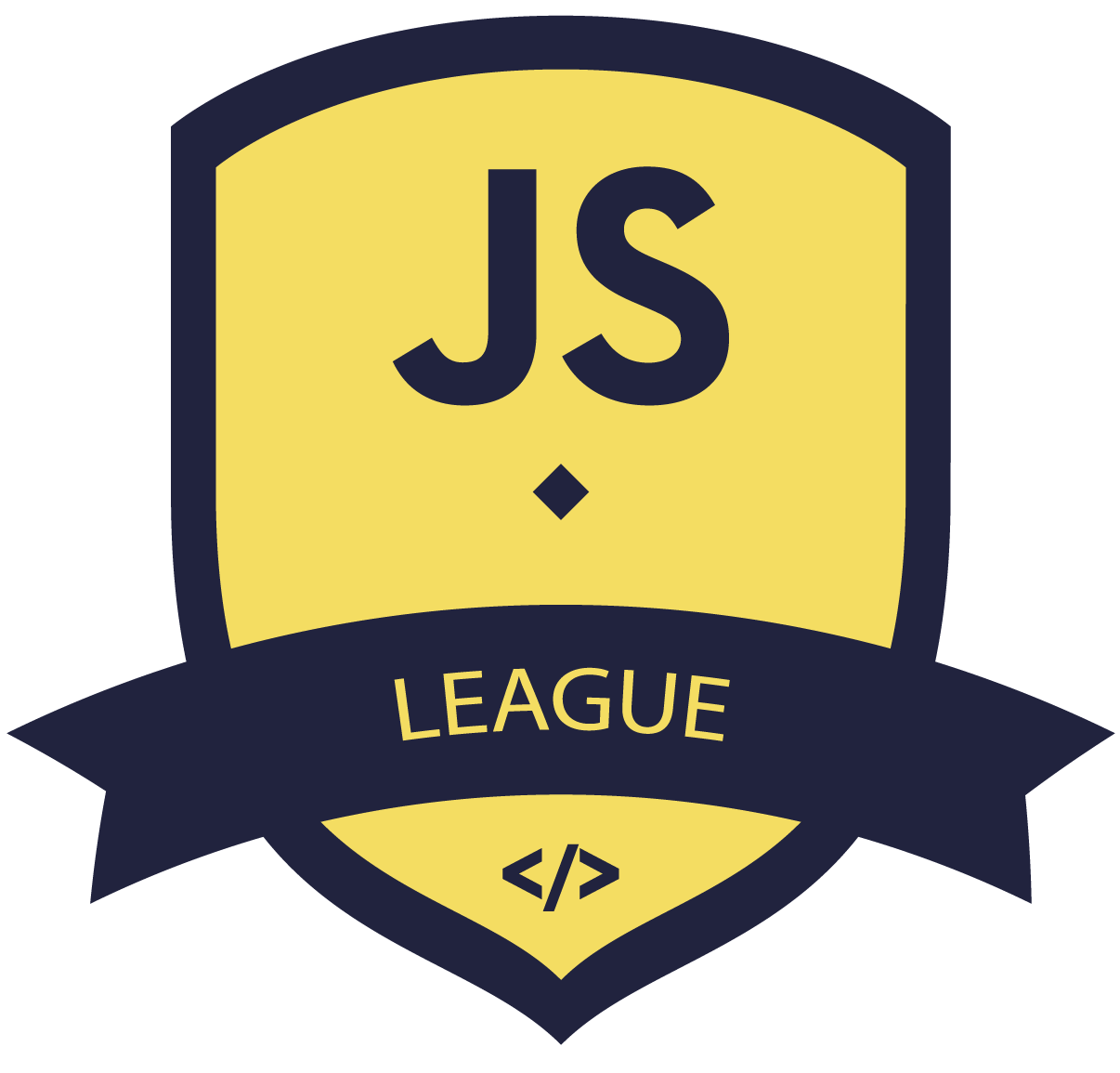
JavaScript Fundamentals (II)
Part I
- Introduction
- Toolkit
- Hello, JavaScript!
- Variables
- Data types (I)
Part II
- Data types (II)
- Operators
- Statements
- Functions (I)
Part III
- Functions (II)
- Browser & DOM
- Events
- Forms
- Server Requests
- From ES5 to ES6


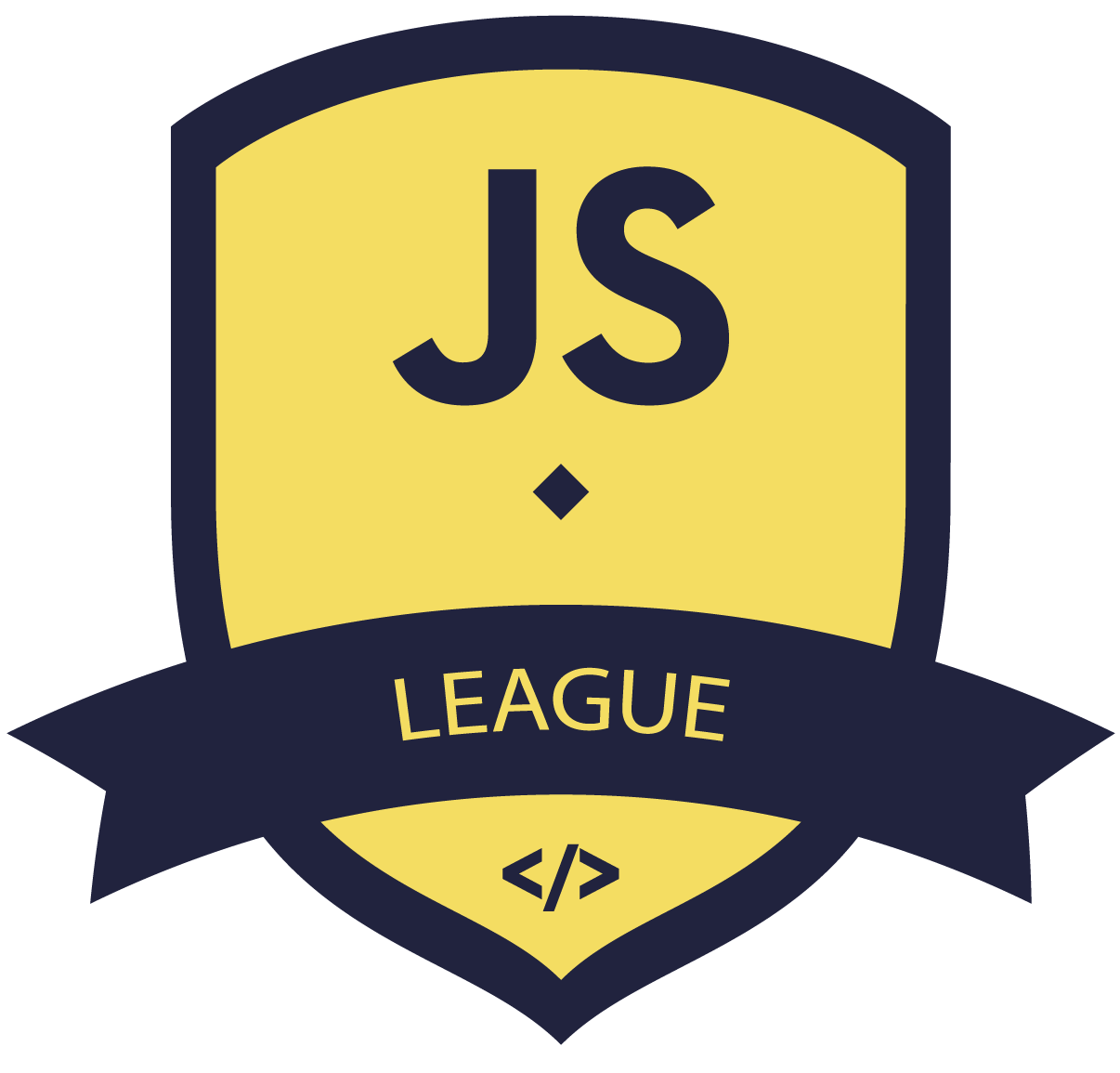
JavaScript Fundamentals (II)
Agenda
10:30 - 10:45 Overview
10:45 - 11:15 Recap
11:15 - 11:30 Break
11:30 - 13:15 Data Types (II)
13:15 - 14:15 Lunch break
14:15 - 15:30 Operators
15:30 - 15:45 Break
15:45 - 17:45 Statements
17:45 - 18:00 Break
18:00 - 19:00 Functions (I)
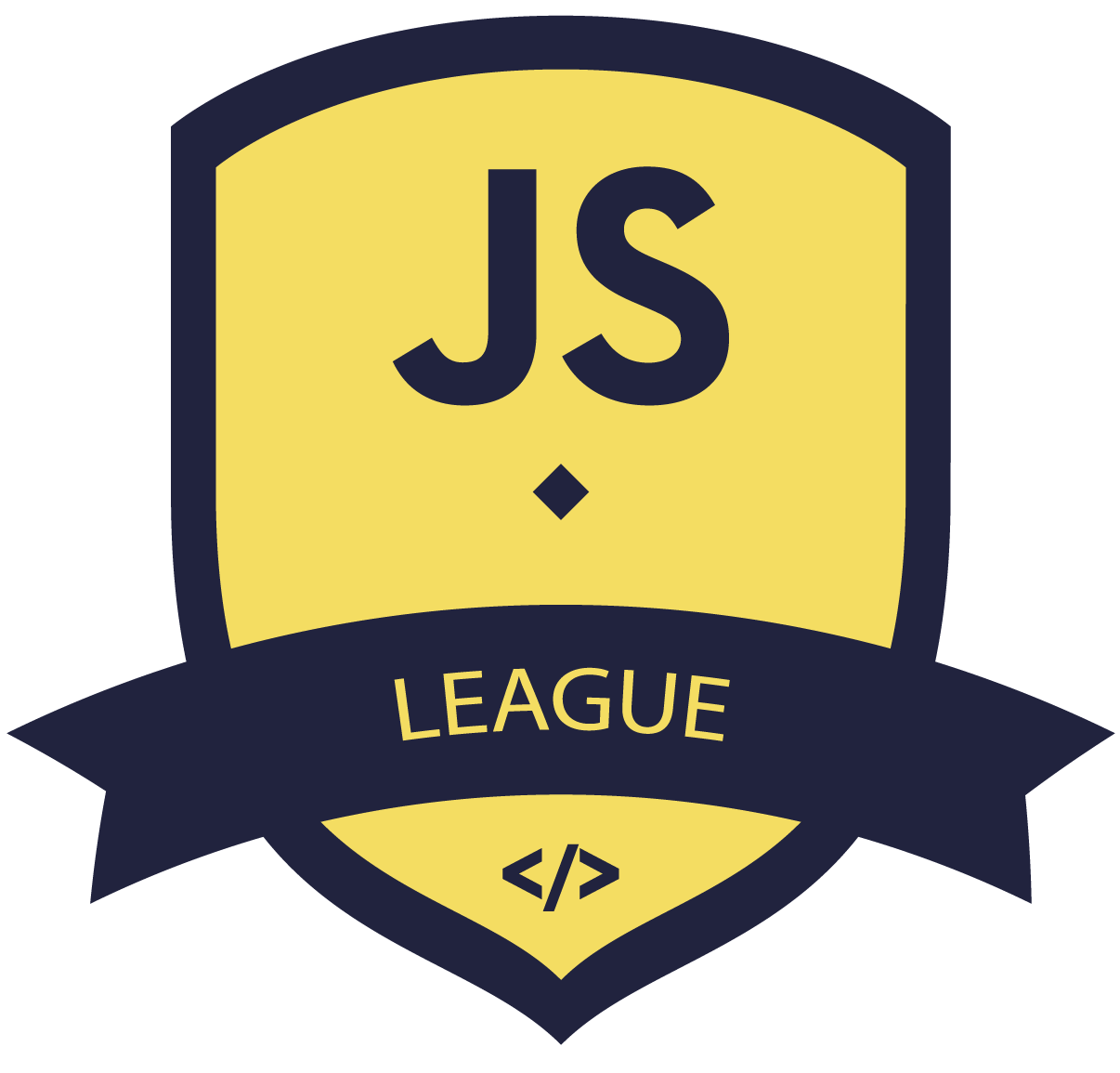
Recap
JavaScript Fundamentals (II)
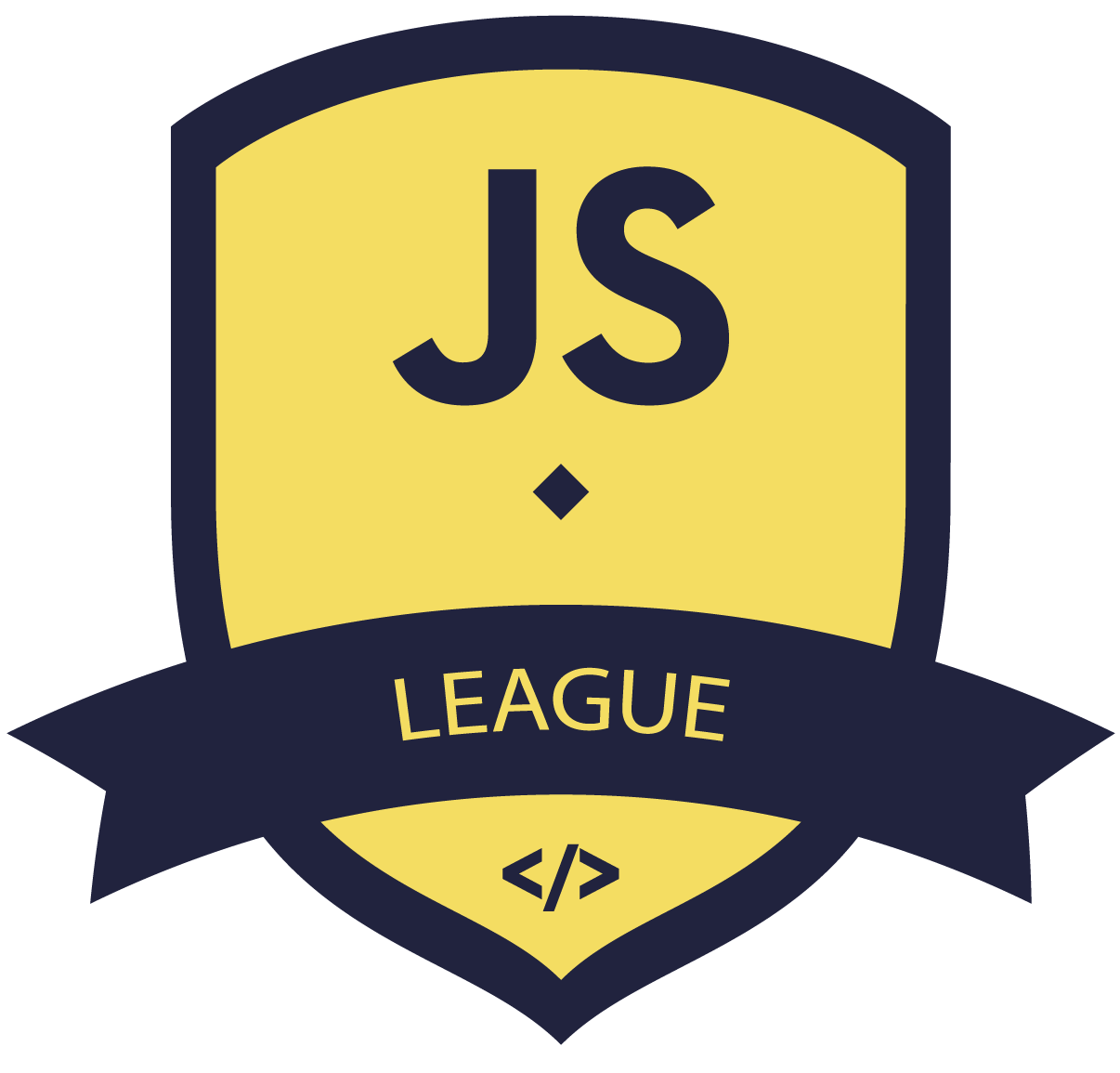
JavaScript Fundamentals (II)
Toolkit
Debuggers
IDEs
Style guides
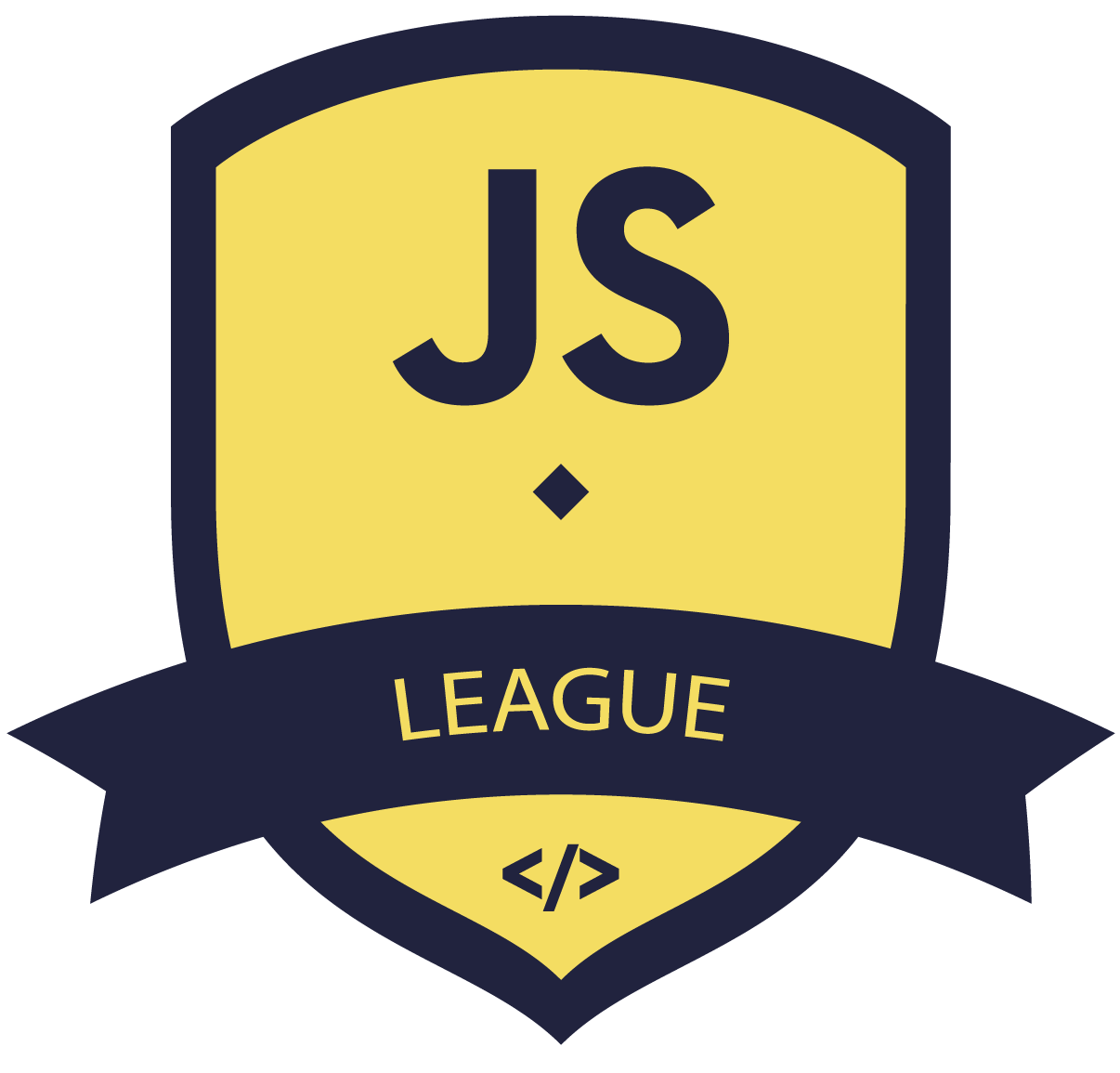
JavaScript Fundamentals (II)
References
infile
inline
external
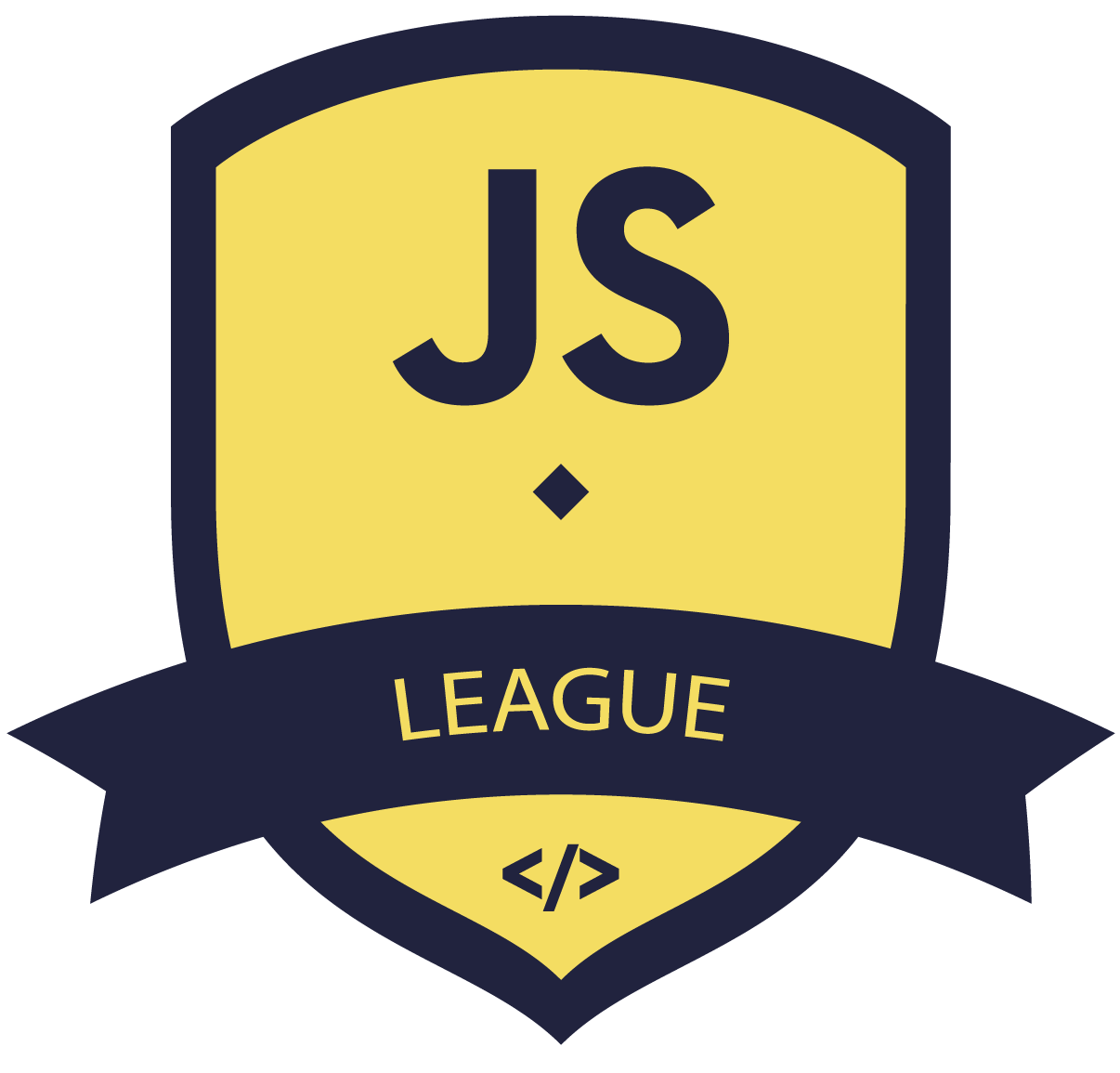
JavaScript Fundamentals (II)
Variables
no public / private
var
global vs. local scope
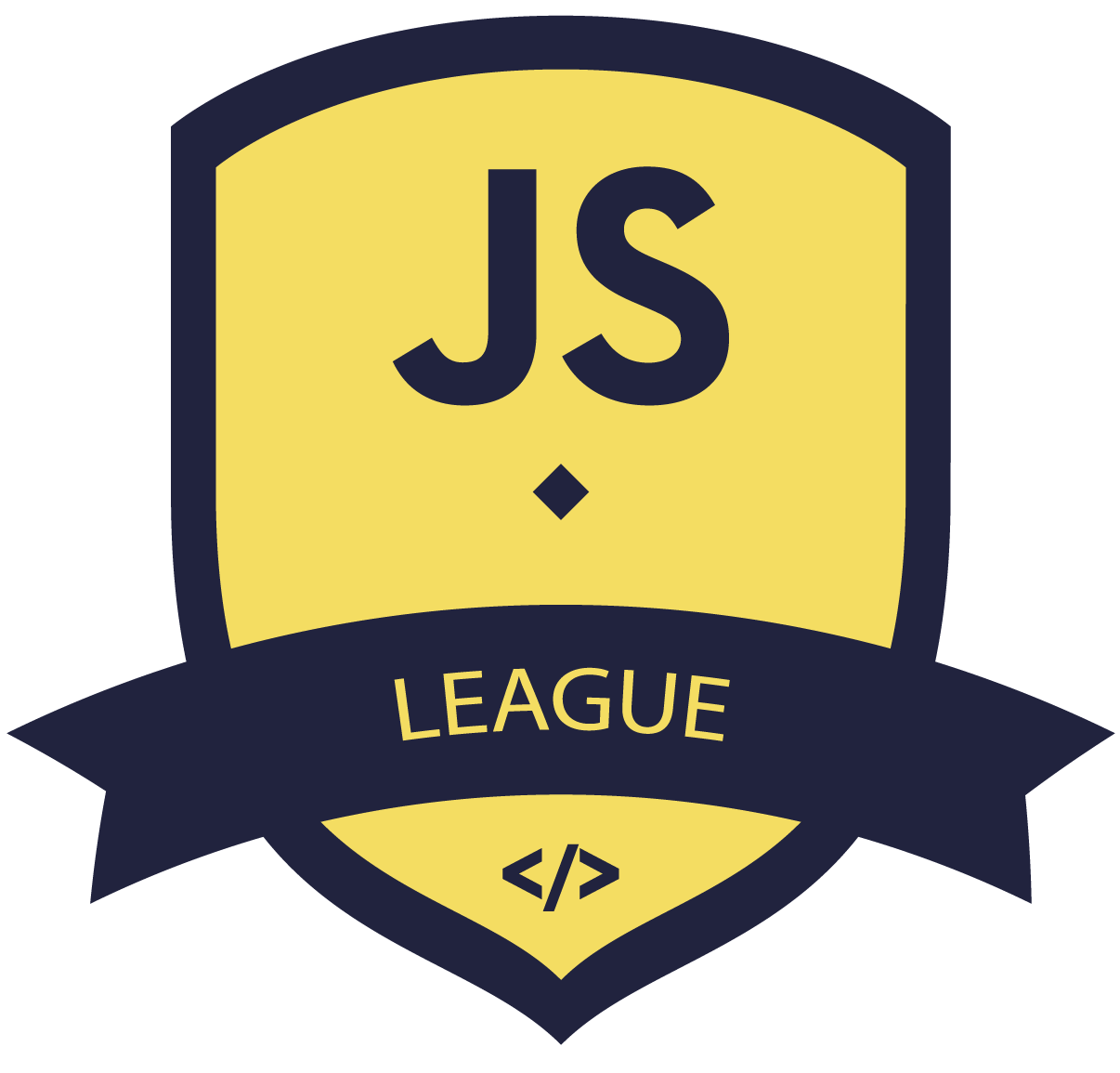
JavaScript Fundamentals (II)
Primitive Data Types
string
number
boolean
null
undefined
object
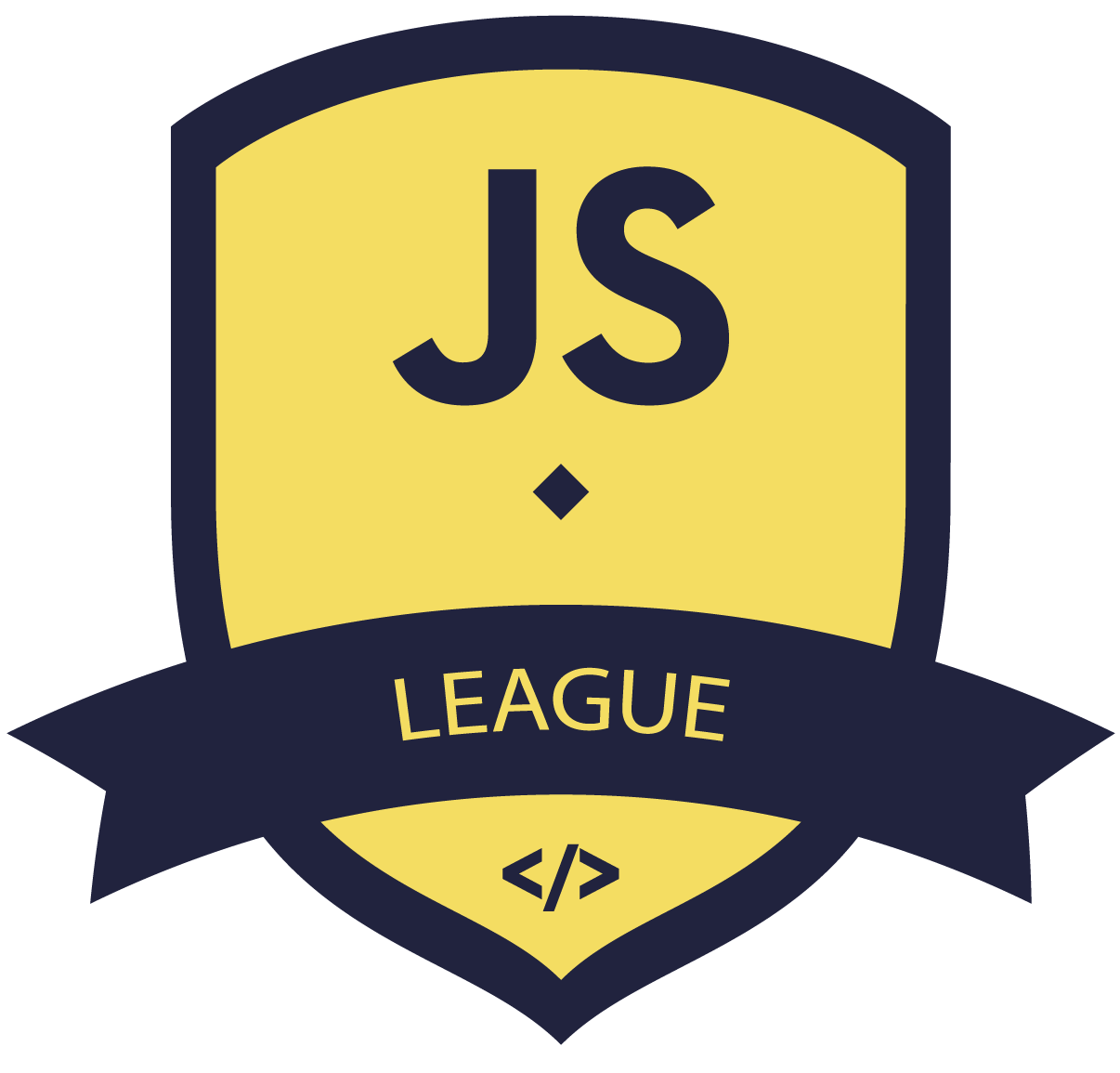
Data types (II)
JavaScript Fundamentals (II)
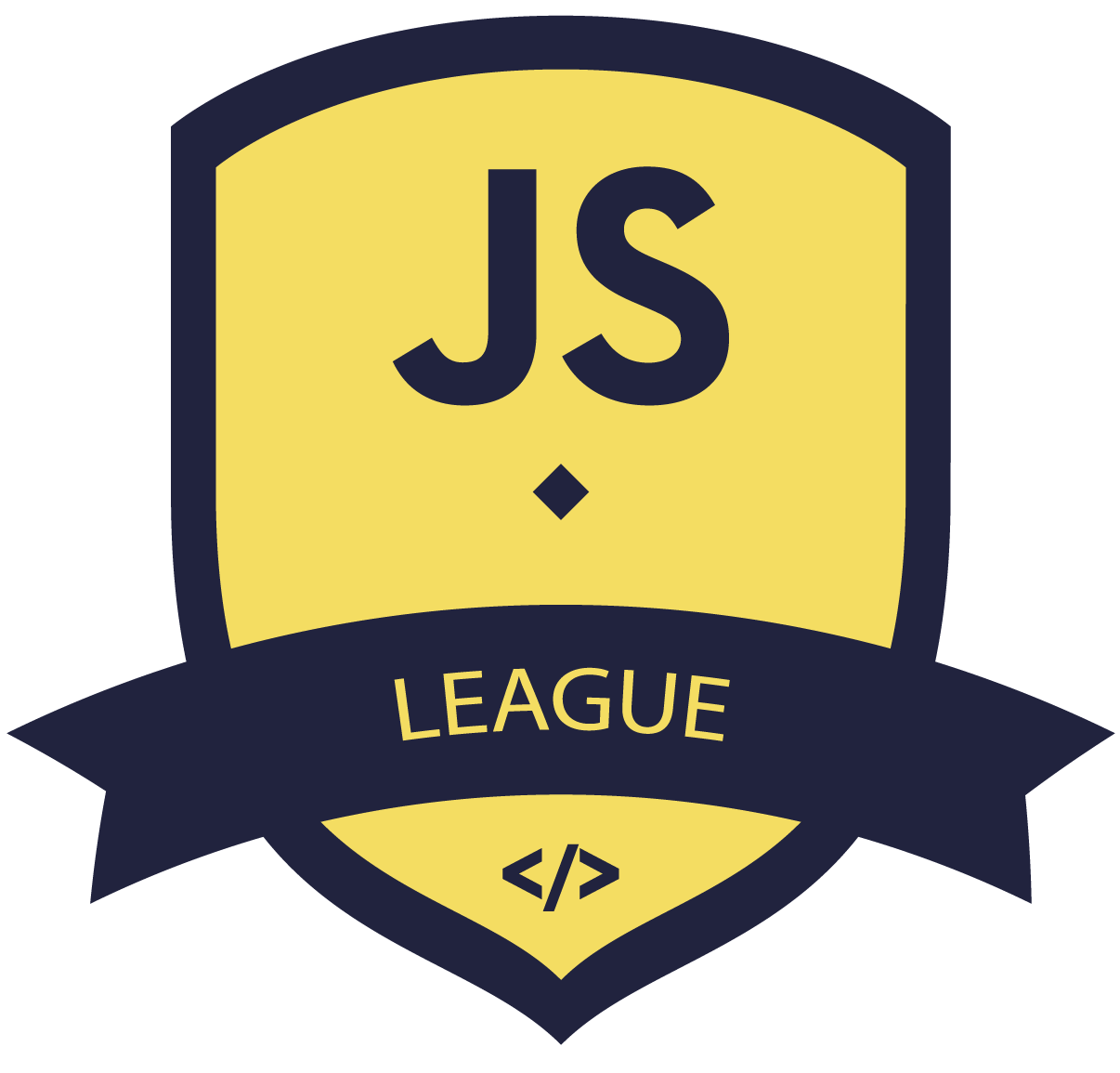
JavaScript Fundamentals (II)
object types
Number
String
Boolean
Math
Date
RegExp
instanceof
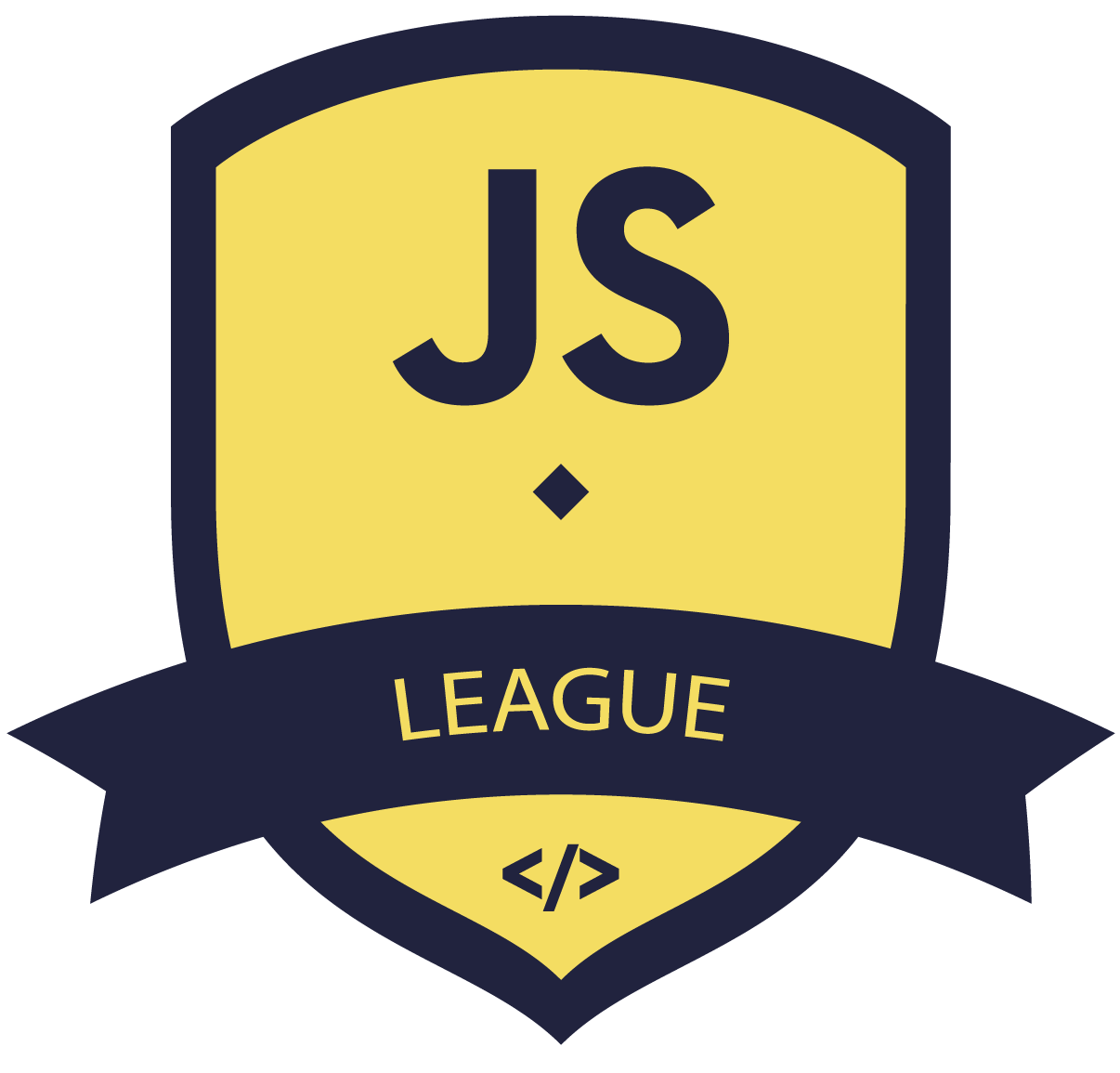
JavaScript Fundamentals (II)
object types
Object
Array
JSON
instanceof
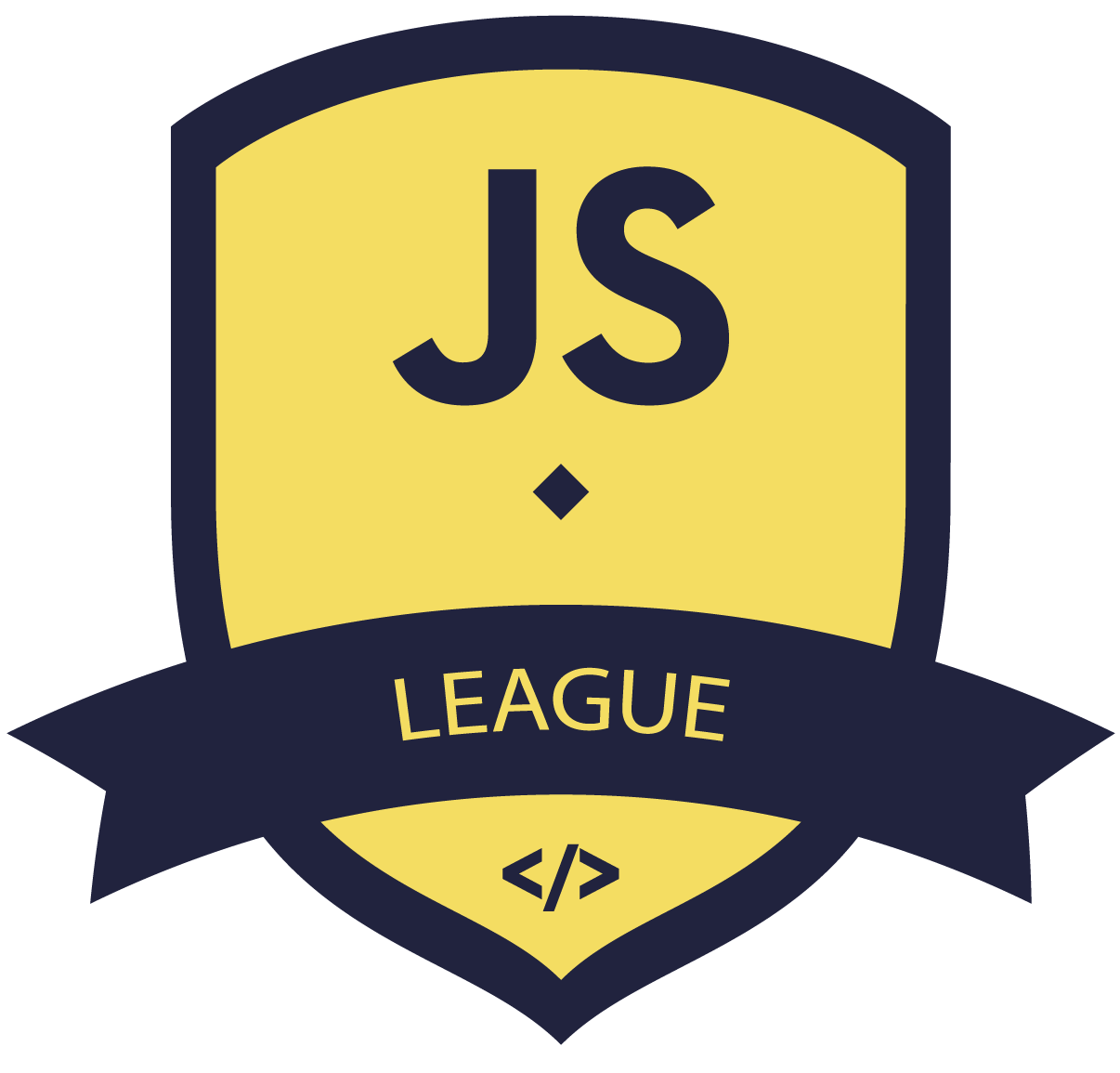
Data types (II) :
Number
JavaScript Fundamentals (II)
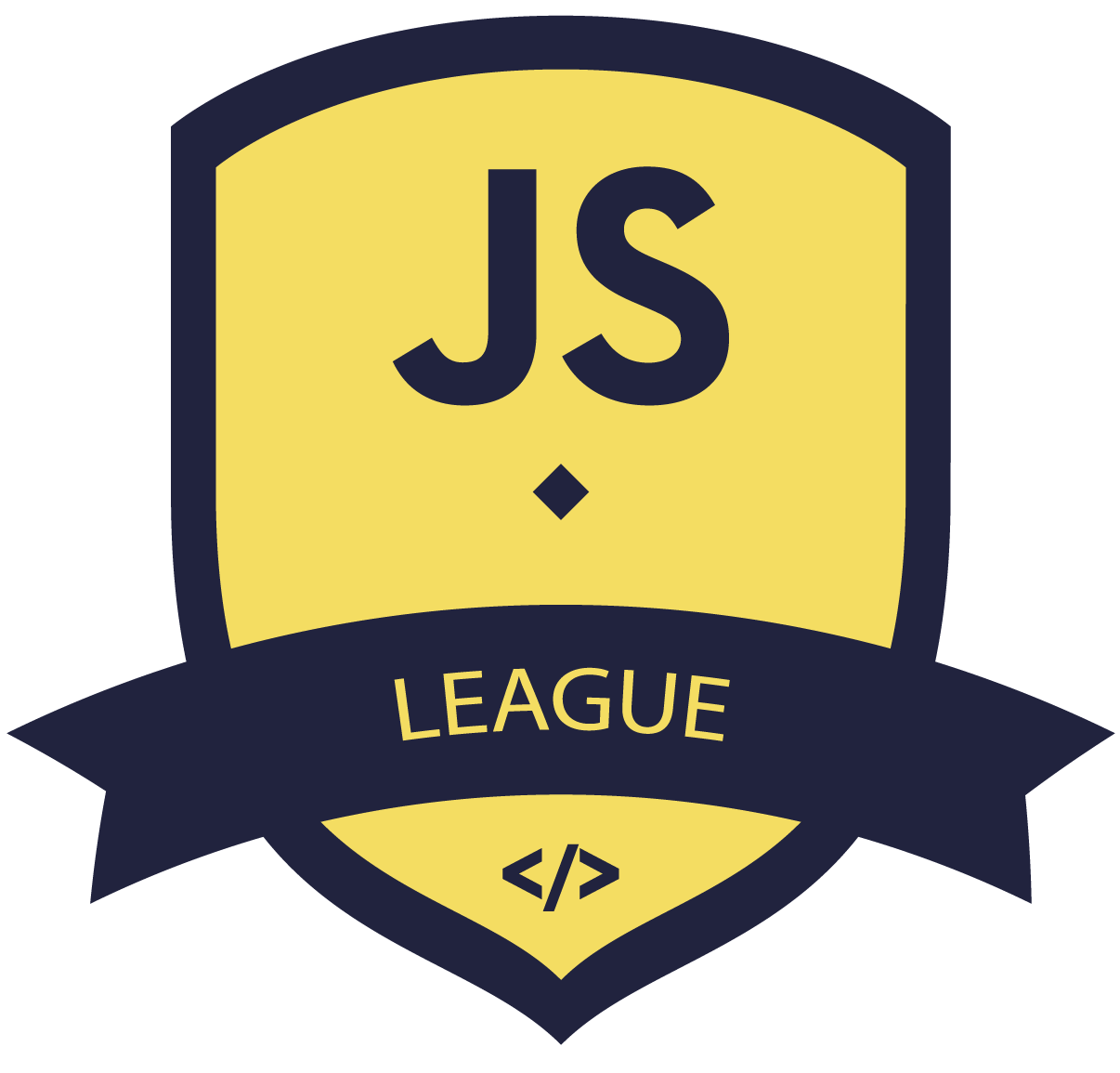
JavaScript Fundamentals (II)
Properties
MAX_VALUE | The largest possible value a number in JavaScript can have 1.7976931348623157E+308 |
MIN_VALUE | The smallest possible value a number in JavaScript can have 5E-324 |
NaN | Equal to a value that is not a number. |
NEGATIVE_INFINITY | A value that is less than MIN_VALUE. |
POSITIVE_INFINITY | A value that is greater than MAX_VALUE |
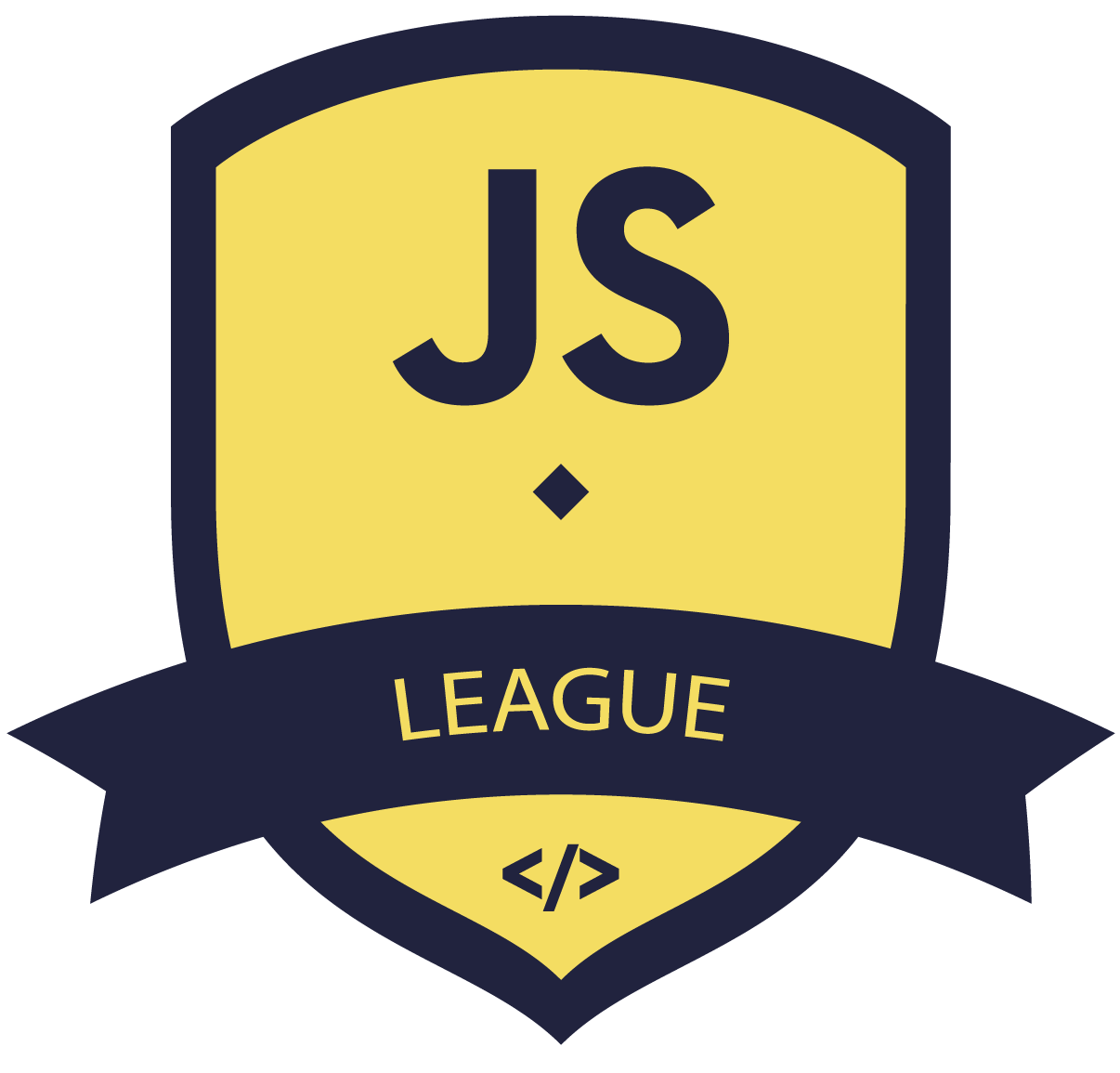
JavaScript Fundamentals (II)
Methods
toExponential() | Forces a number to display in exponential notation, even if the number is in the range in which JavaScript normally uses standard notation. |
toFixed() | Formats a number with a specific number of digits to the right of the decimal. |
toLocaleString() | Returns a string value version of the current number in a format that may vary according to a browser's local settings. |
toPrecision() | Defines how many total digits (including digits to the left and right of the decimal) to display of a number. |
toString() | Returns the string representation of the number's value. |
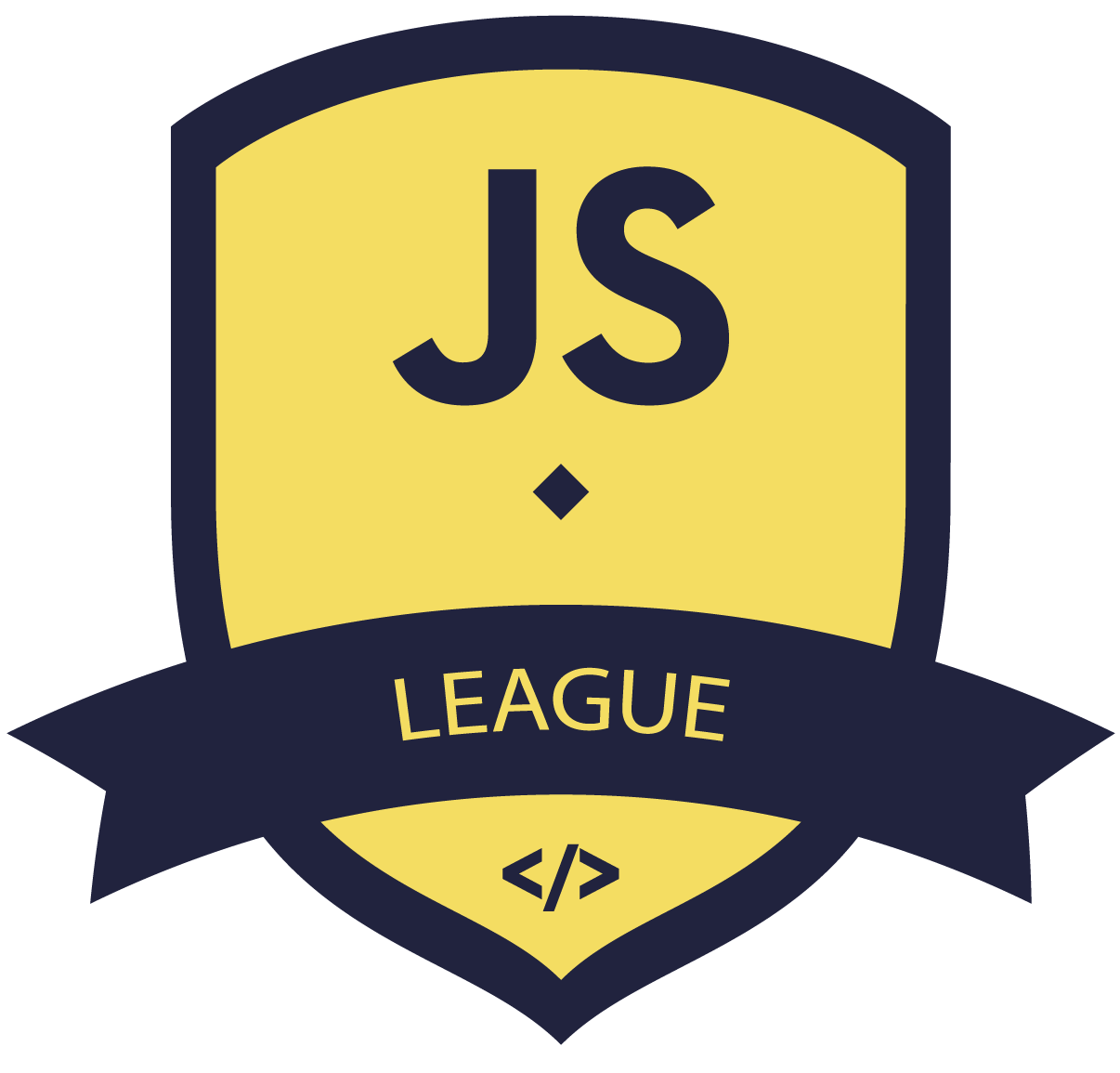
Data types (II) :
String
JavaScript Fundamentals (II)
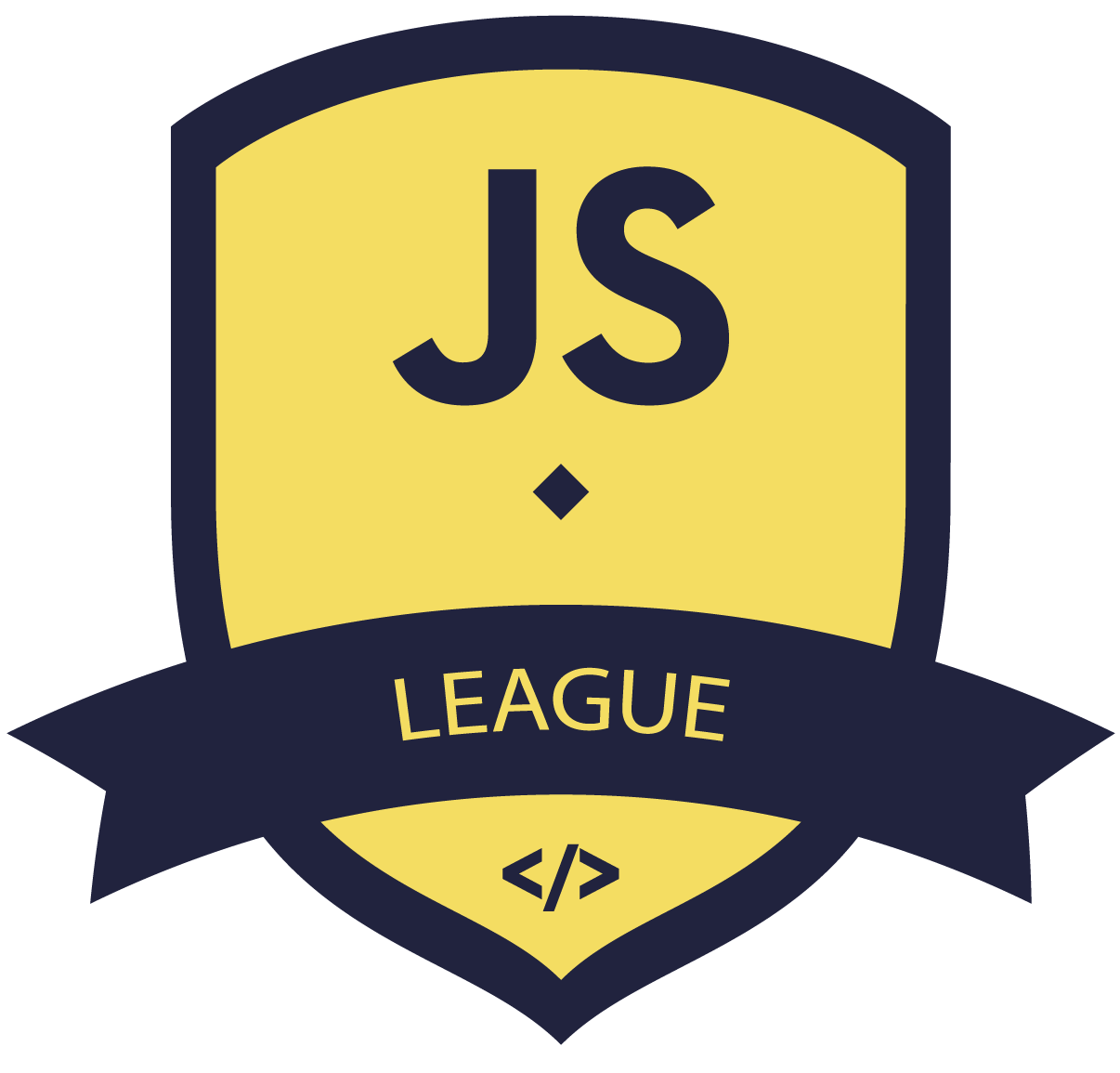
JavaScript Fundamentals (II)
Properties
length | Returns the length of the string. |
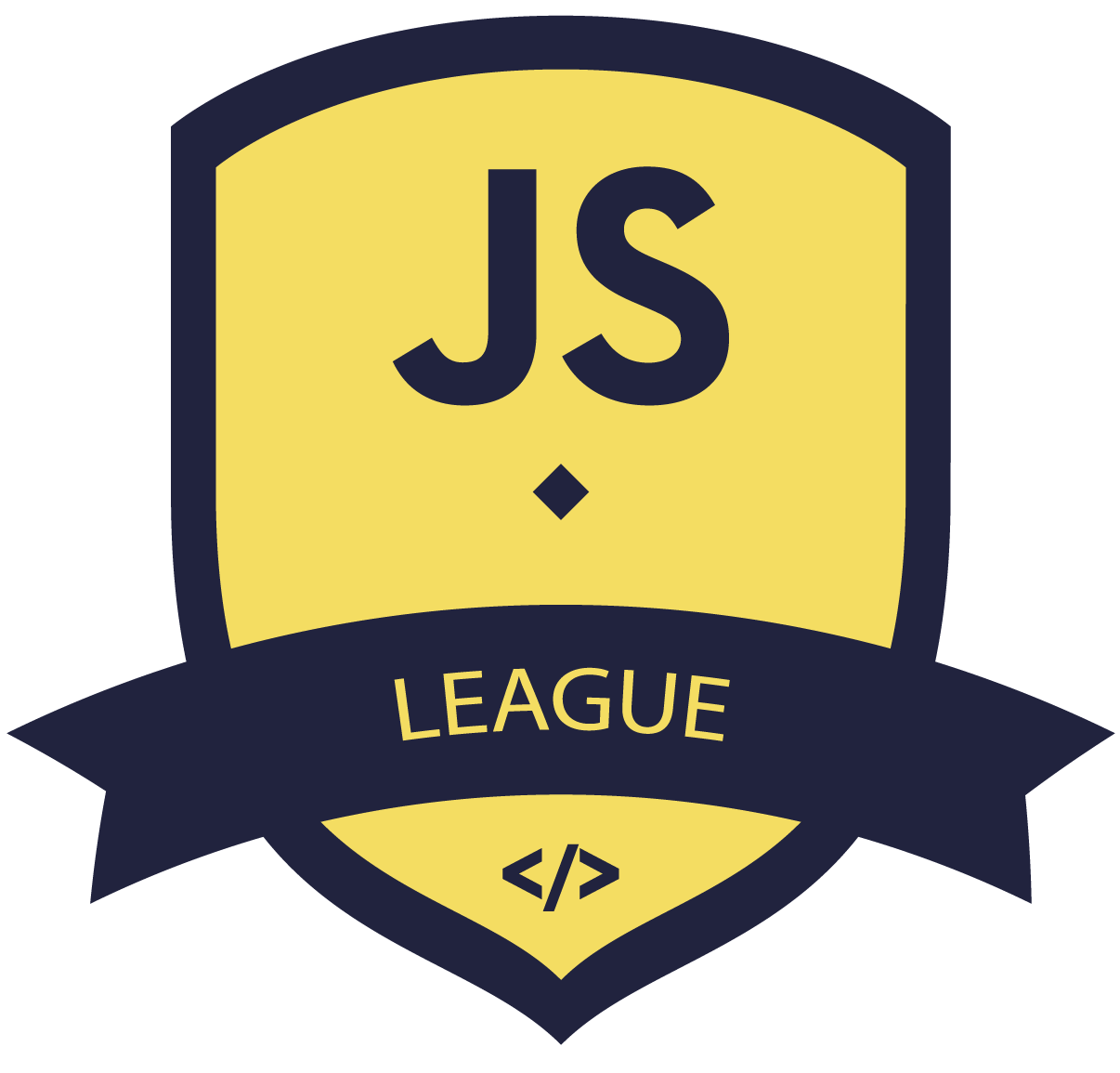
JavaScript Fundamentals (II)
Methods
charAt() | Returns the character at the specified index. |
concat() | Combines the text of two strings and returns a new string. |
indexOf() | Returns the index within the calling String object of the first occurrence of the specified value, or -1 if not found. |
match() | Used to match a regular expression against a string. |
replace() | Used to find a match between a regular expression and a string, and to replace the matched substring with a new substring. |
search() | Executes the search for a match between a regular expression and a specified string. |
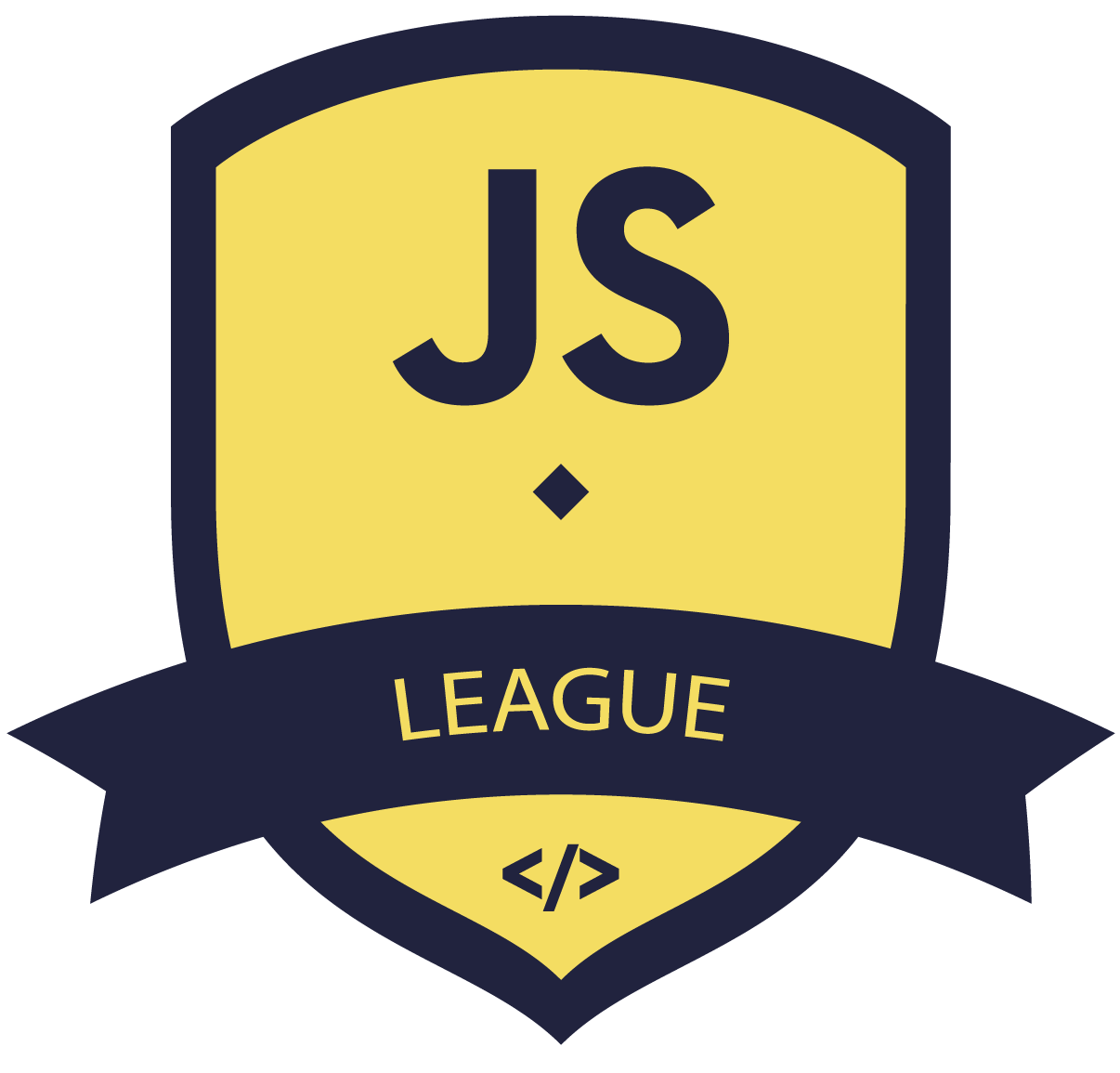
JavaScript Fundamentals (II)
Methods (cont.)
slice() | Extracts a section of a string and returns a new string. |
split() | Splits a String object into an array of strings by separating the string into substrings. |
substr() | Returns the characters in a string beginning at the specified location through the specified number of characters. |
substring() | Returns the characters in a string between two indexes into the string. |
toLowerCase() | Returns the calling string value converted to lower case. |
toUpperCase() | Returns the calling string value converted to uppercase. |
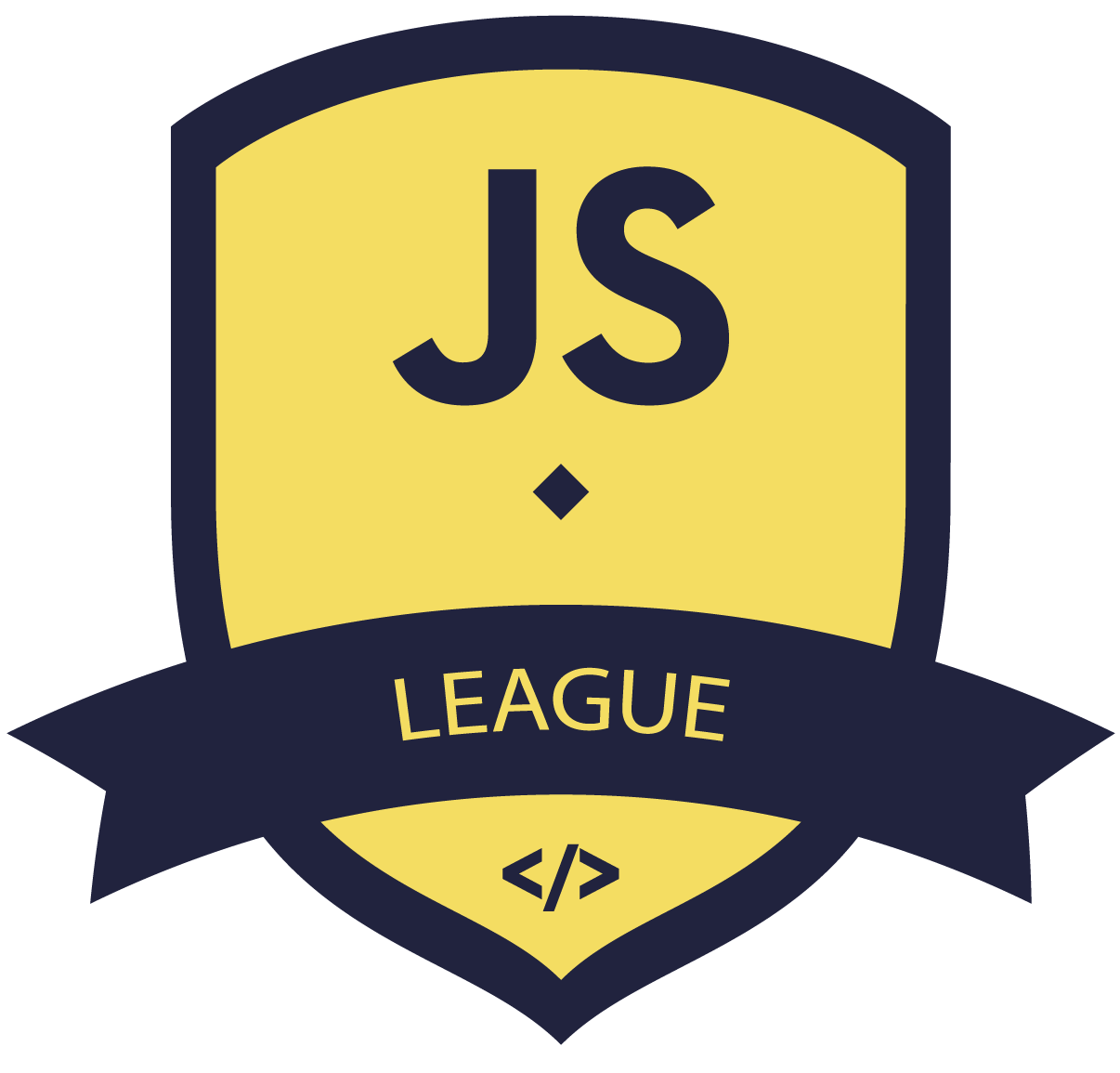
Data types (II) :
Boolean
JavaScript Fundamentals (II)
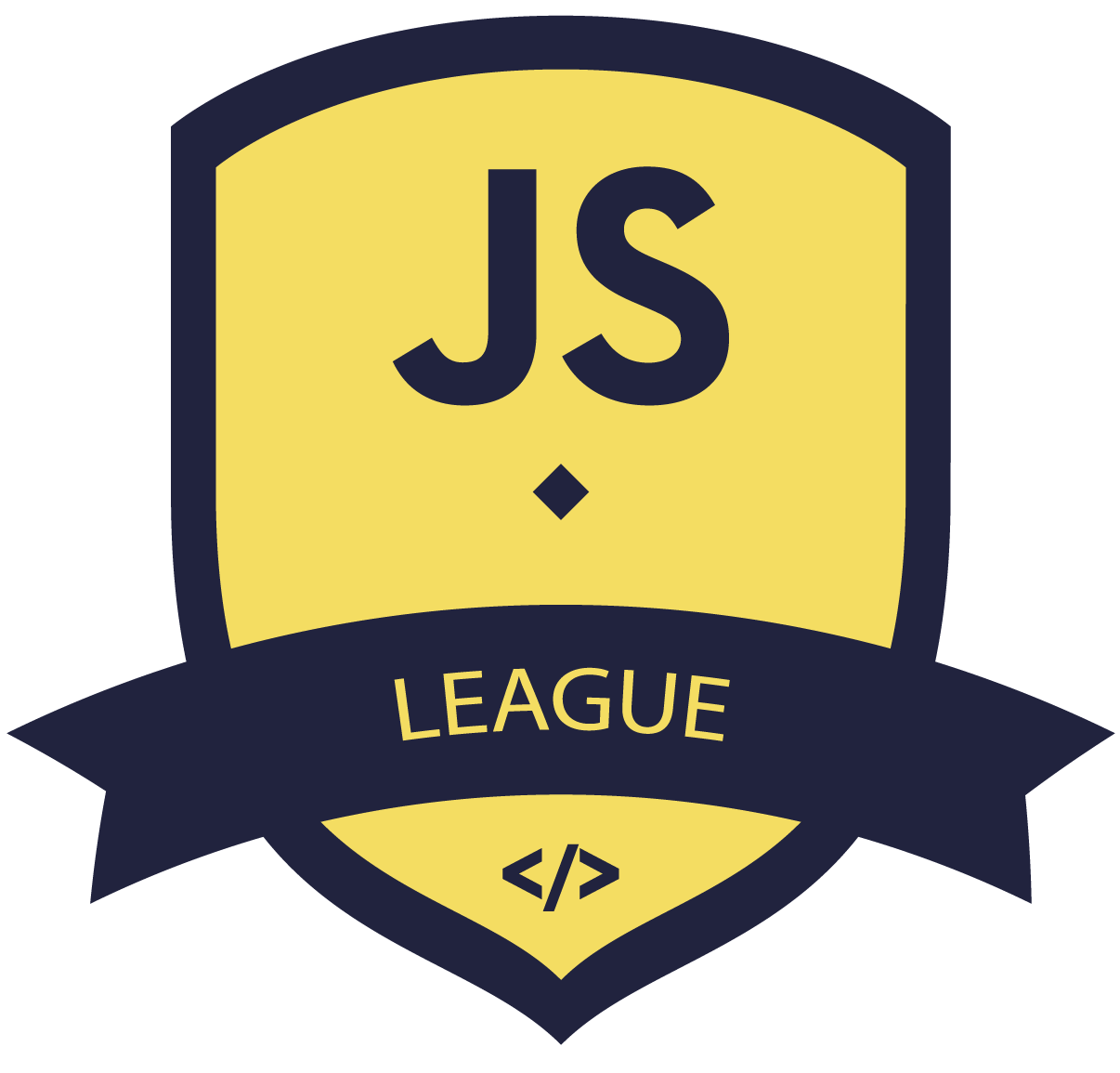
Data types (II) :
Date
JavaScript Fundamentals (II)
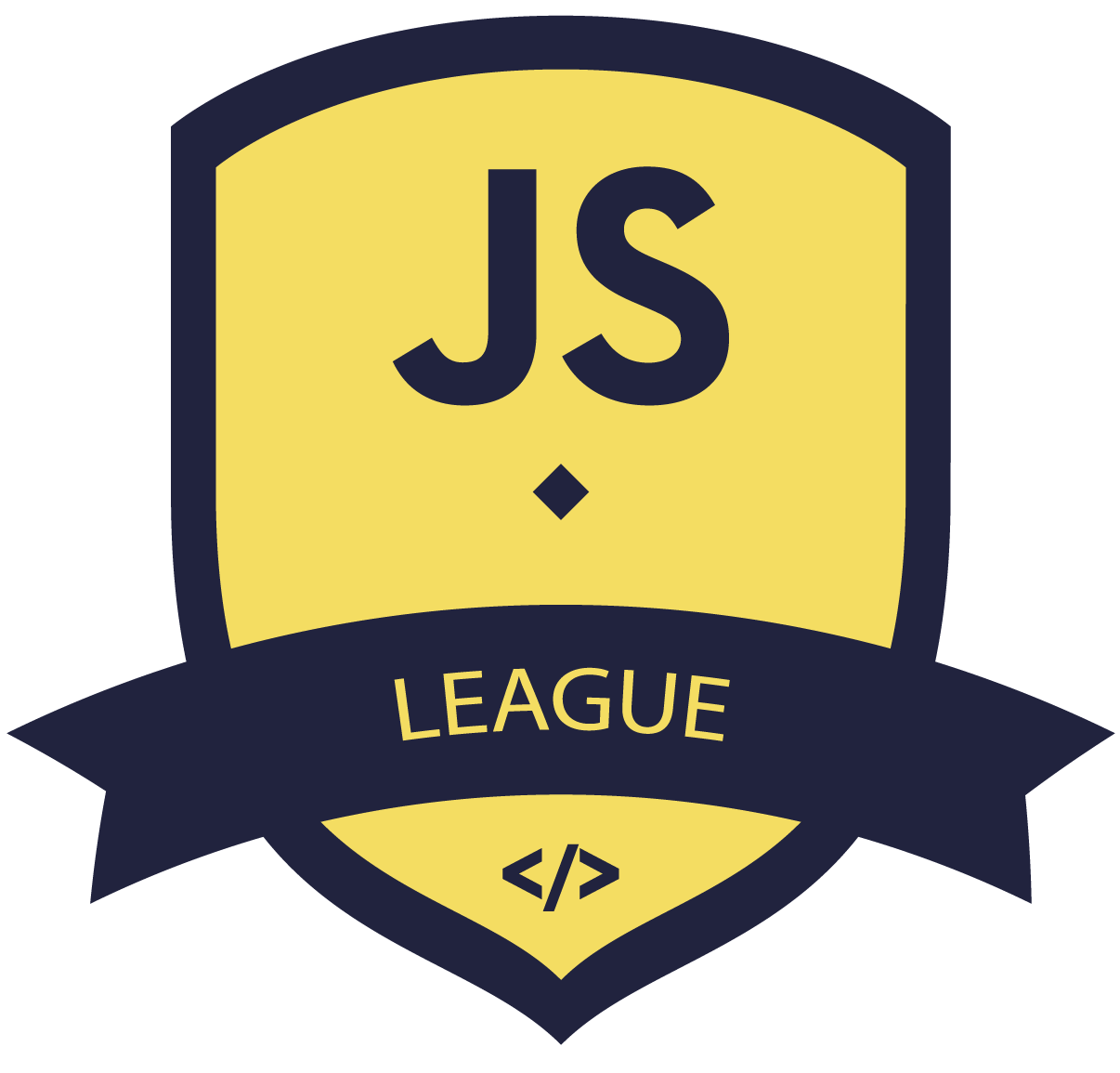
JavaScript Fundamentals (II)
Parameters
Date | Current day of the month |
Day | Day of the week |
FullYear | Year |
Hours | Hours |
Minutes | Minutes |
Milliseconds | Milliseconds |
Month | Month |
Seconds | Seconds |
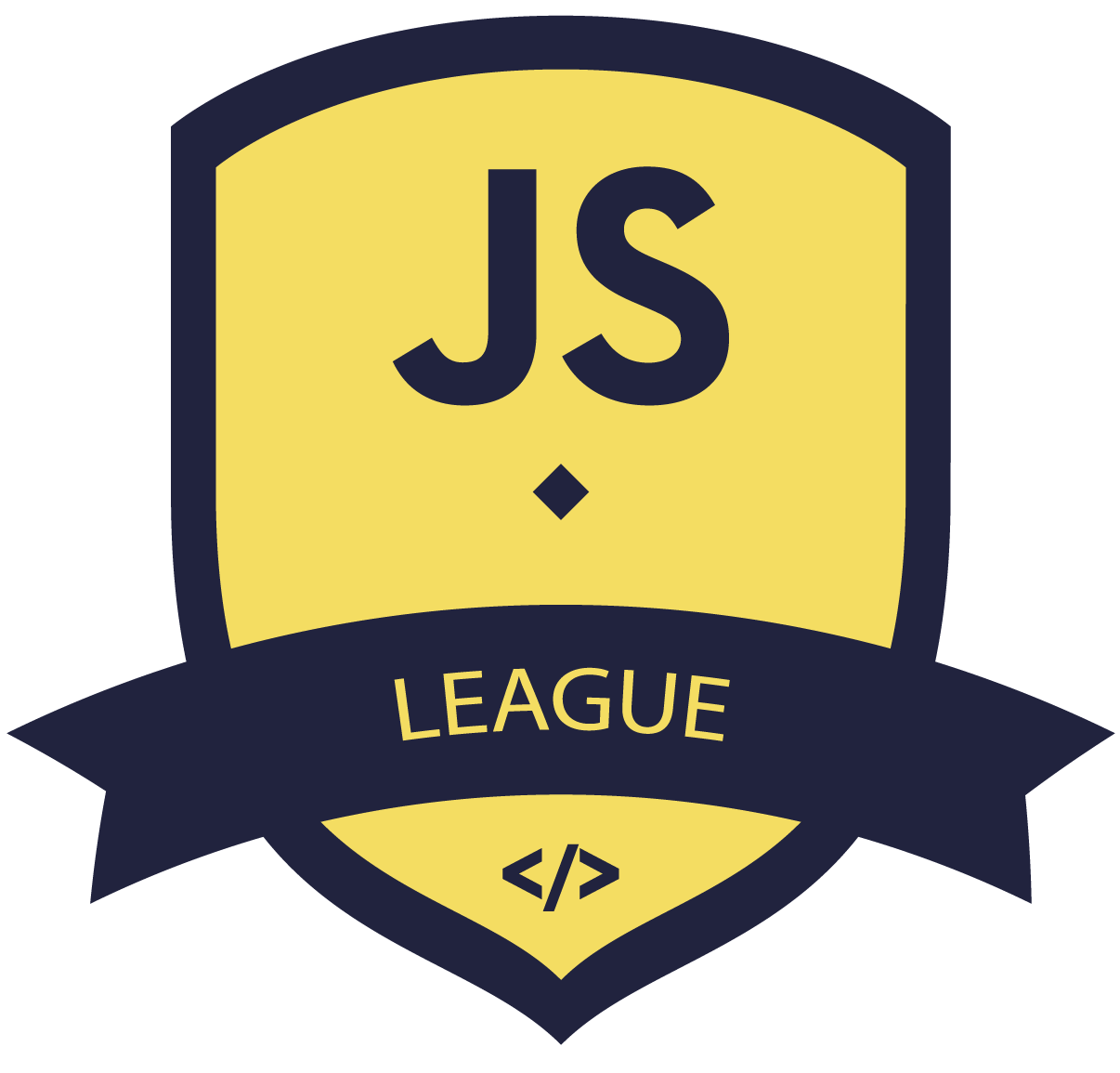
JavaScript Fundamentals (II)
Methods
get<param>() | Returns data based on param requested |
getUTC<param>() | Returns data based on param requested according to UTC |
set<param>() | Updates the value |
getTimezoneOffset() | Returns the time-zone offset in minutes for the current locale. |
toLocaleFormat() | Converts a date to a string, using a format string. |
toUTCString() | Converts a date to a string, using the universal time convention. |
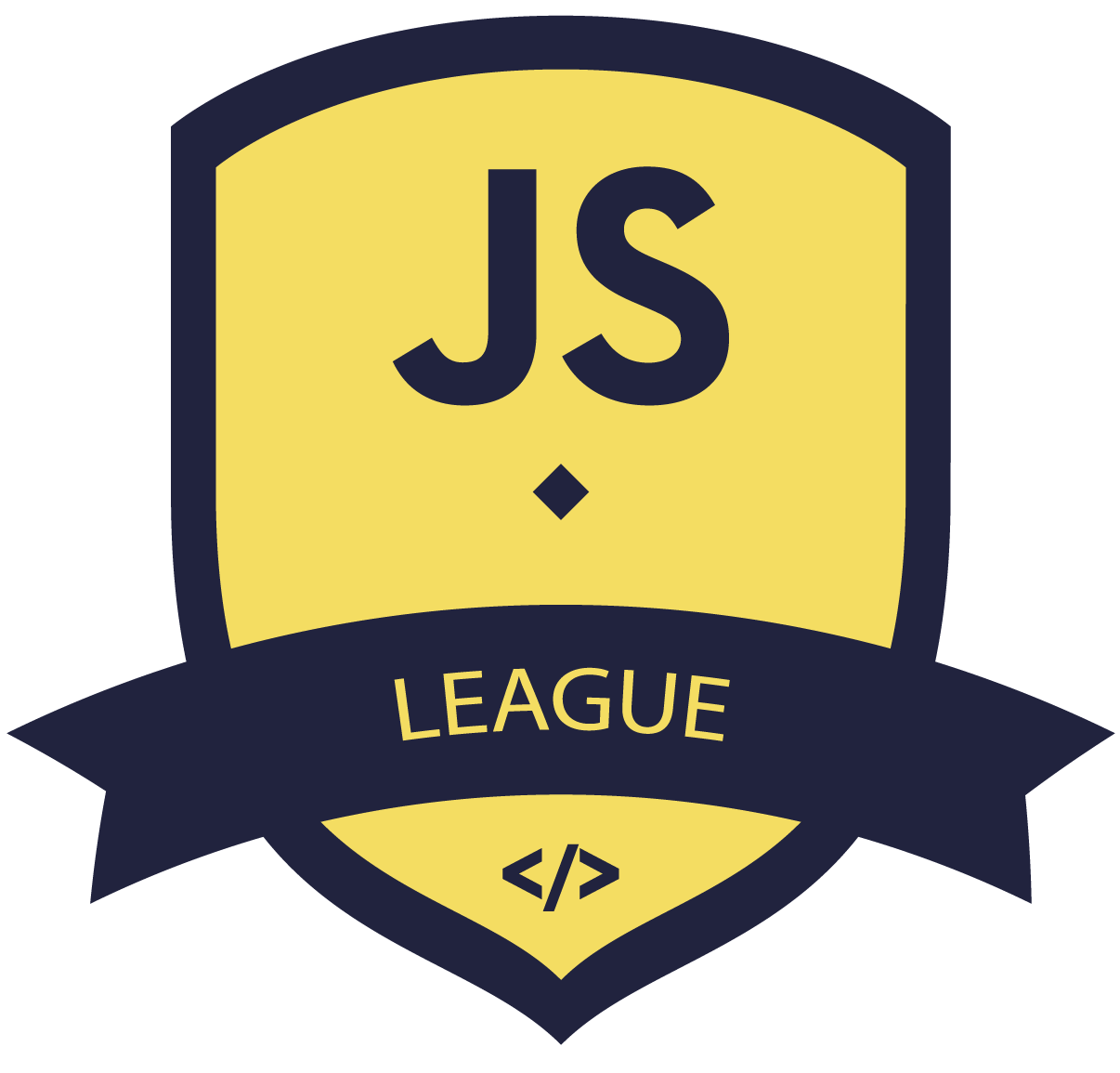
Data types (II) :
Math
JavaScript Fundamentals (II)
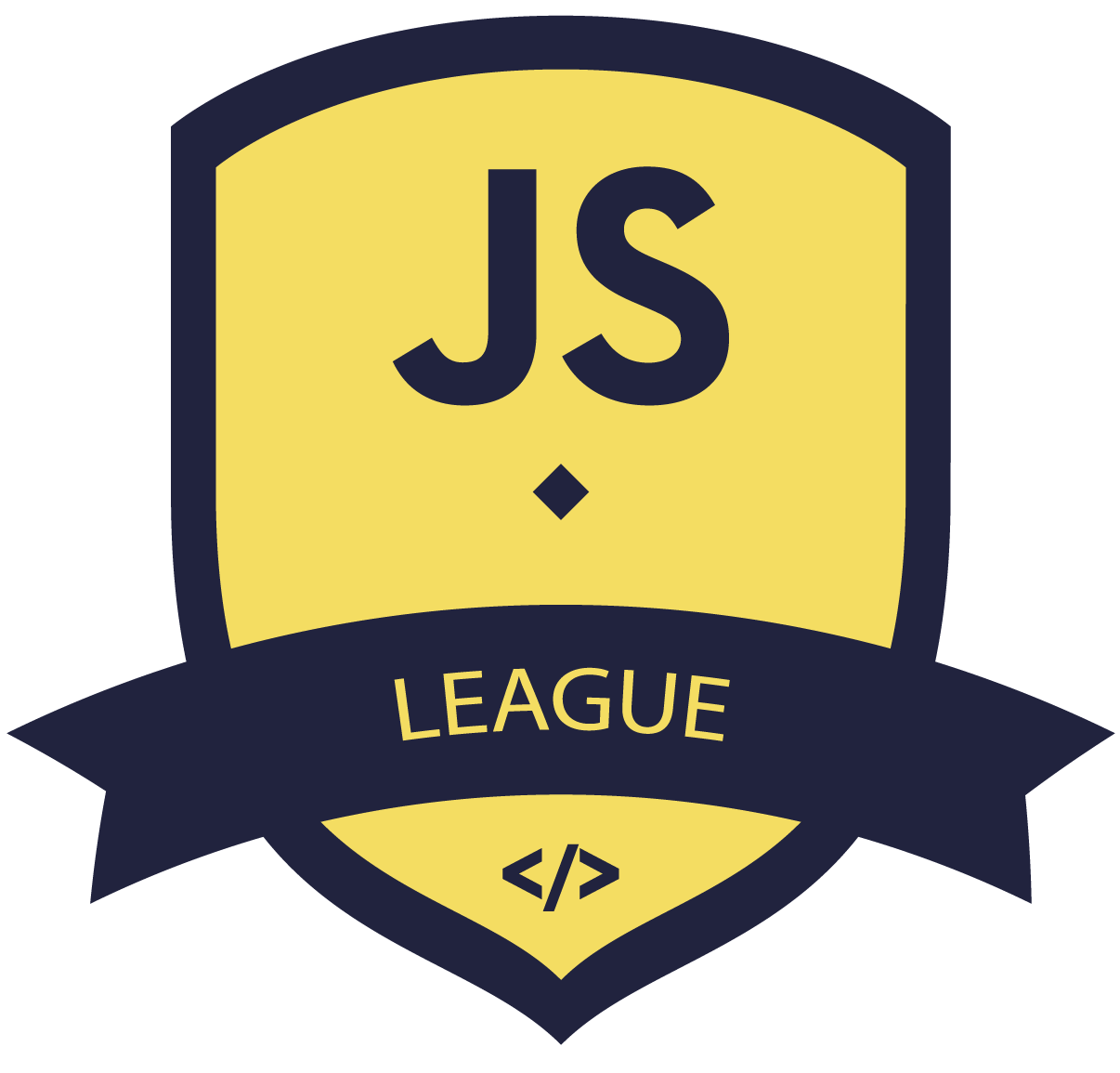
JavaScript Fundamentals (II)
Properties
E | Euler's constant and the base of natural logarithms, approximately 2.718. |
LN2 | Natural logarithm of 2, approximately 0.693. |
LN10 | Natural logarithm of 10, approximately 2.302. |
LOG2E | Base 2 logarithm of E, approximately 1.442. |
LOG10E | Base 10 logarithm of E, approximately 0.434. |
PI | Ratio of the circumference of a circle to its diameter, approximately 3.14159. |
SQRT1_2 | Square root of 1/2; equivalently, 1 over the square root of 2, approximately 0.707. |
SQRT2 | Square root of 2, approximately 1.414. |
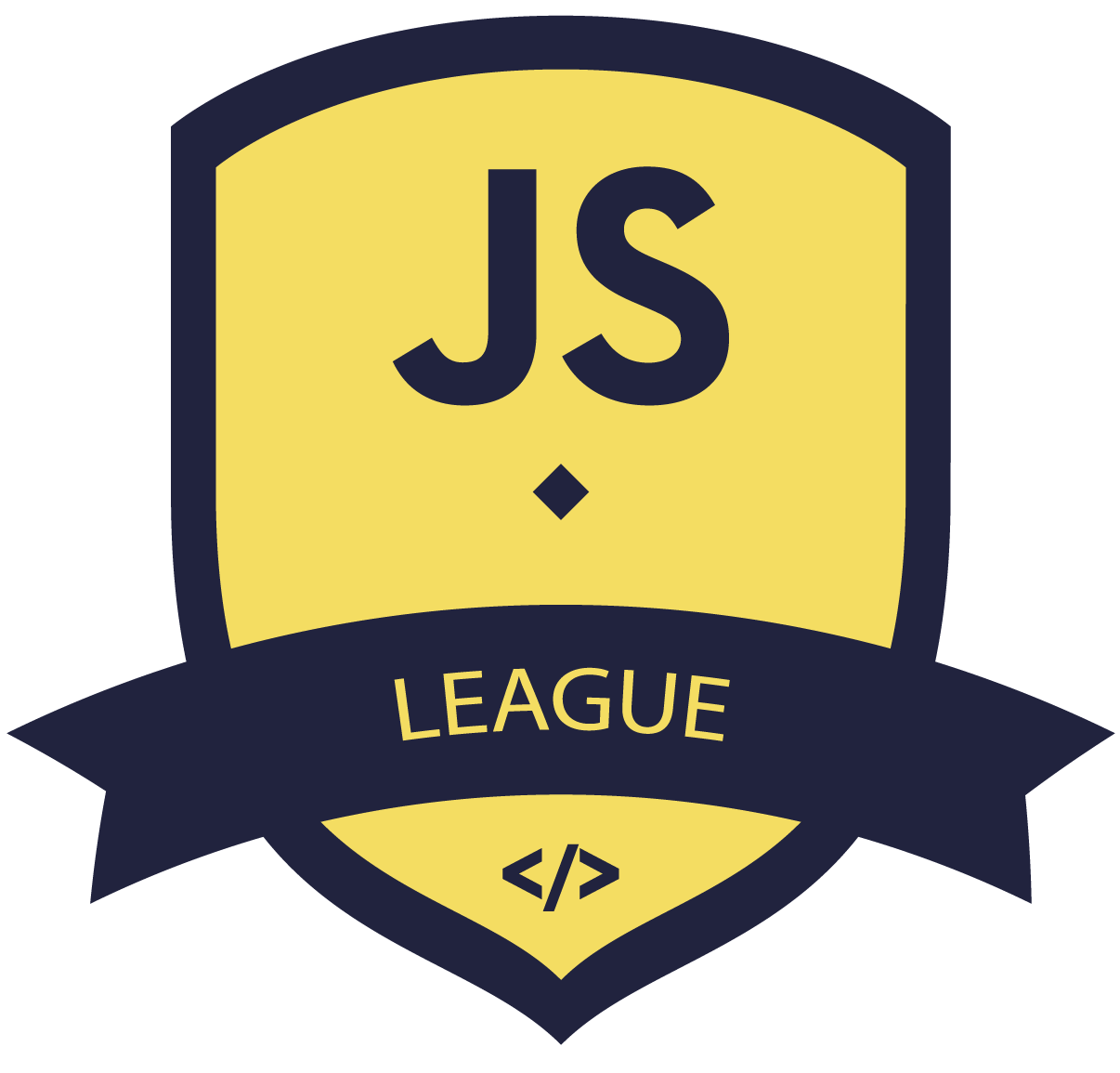
JavaScript Fundamentals (II)
Methods
floor() | Returns the largest integer less than or equal to a number. |
ceil() | Returns the smallest integer greater than or equal to a number. |
max() | Returns the largest of zero or more numbers. |
min() | Returns the smallest of zero or more numbers. |
pow() | Returns base to the exponent power, that is, base exponent. |
random() | Returns a pseudo-random number between 0 and 1. |
round() | Returns the value of a number rounded to the nearest integer. |
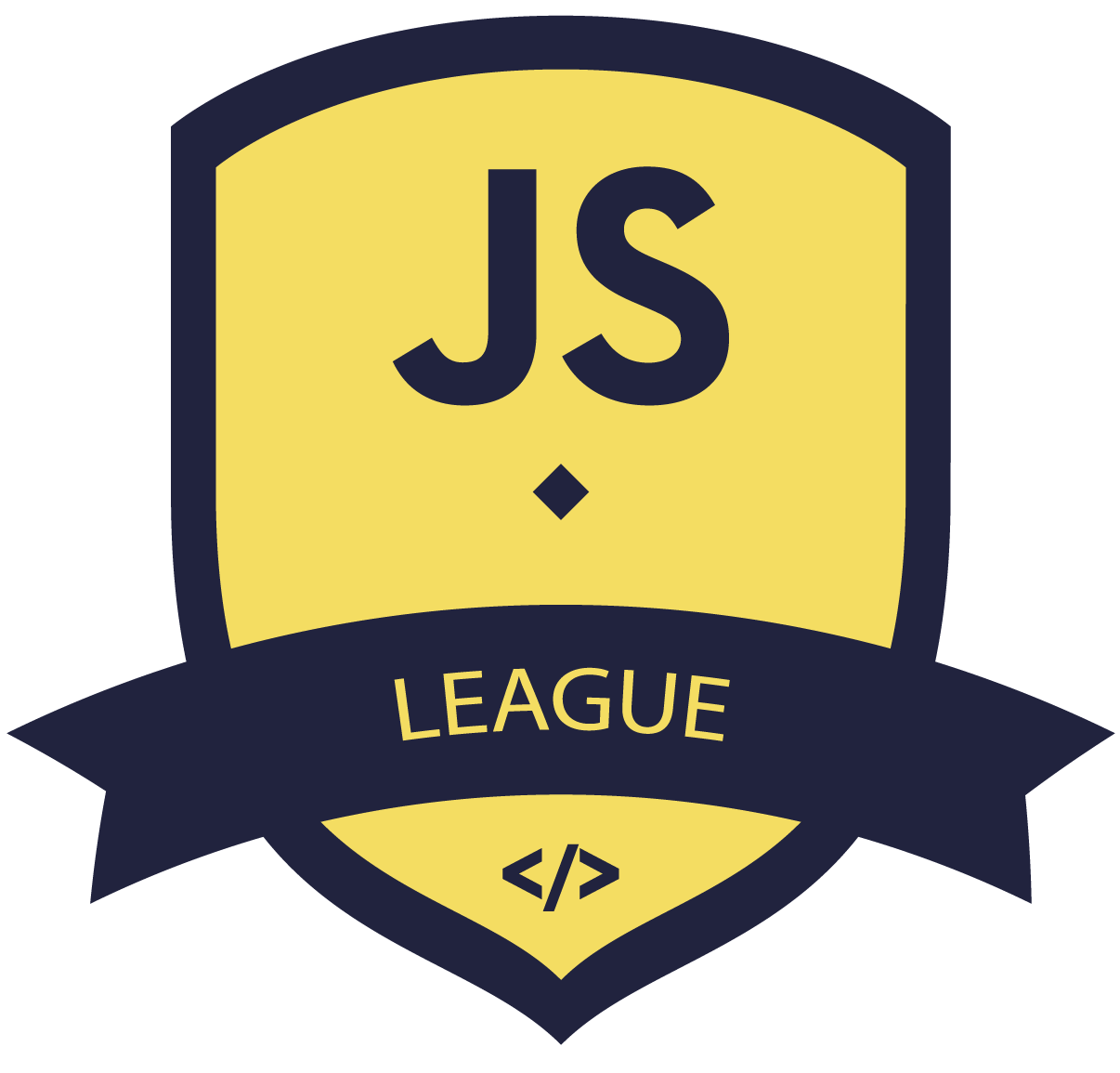
Data types (II) :
Regex
JavaScript Fundamentals (II)
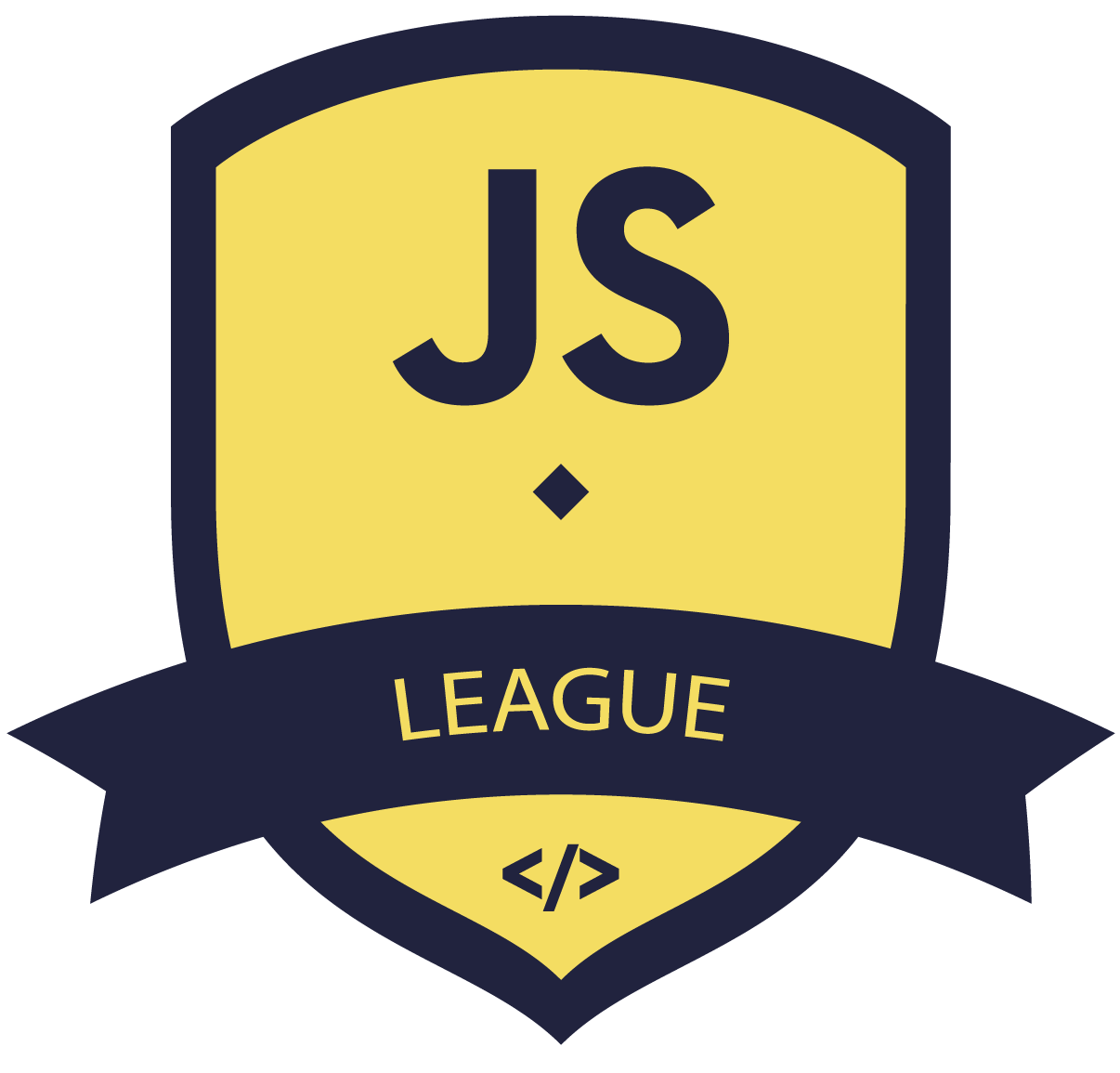
JavaScript Fundamentals (II)
Properties
global | Specifies if the "g" modifier is set. |
ignoreCase | Specifies if the "i" modifier is set. |
multiline | Specifies if the "m" modifier is set. |
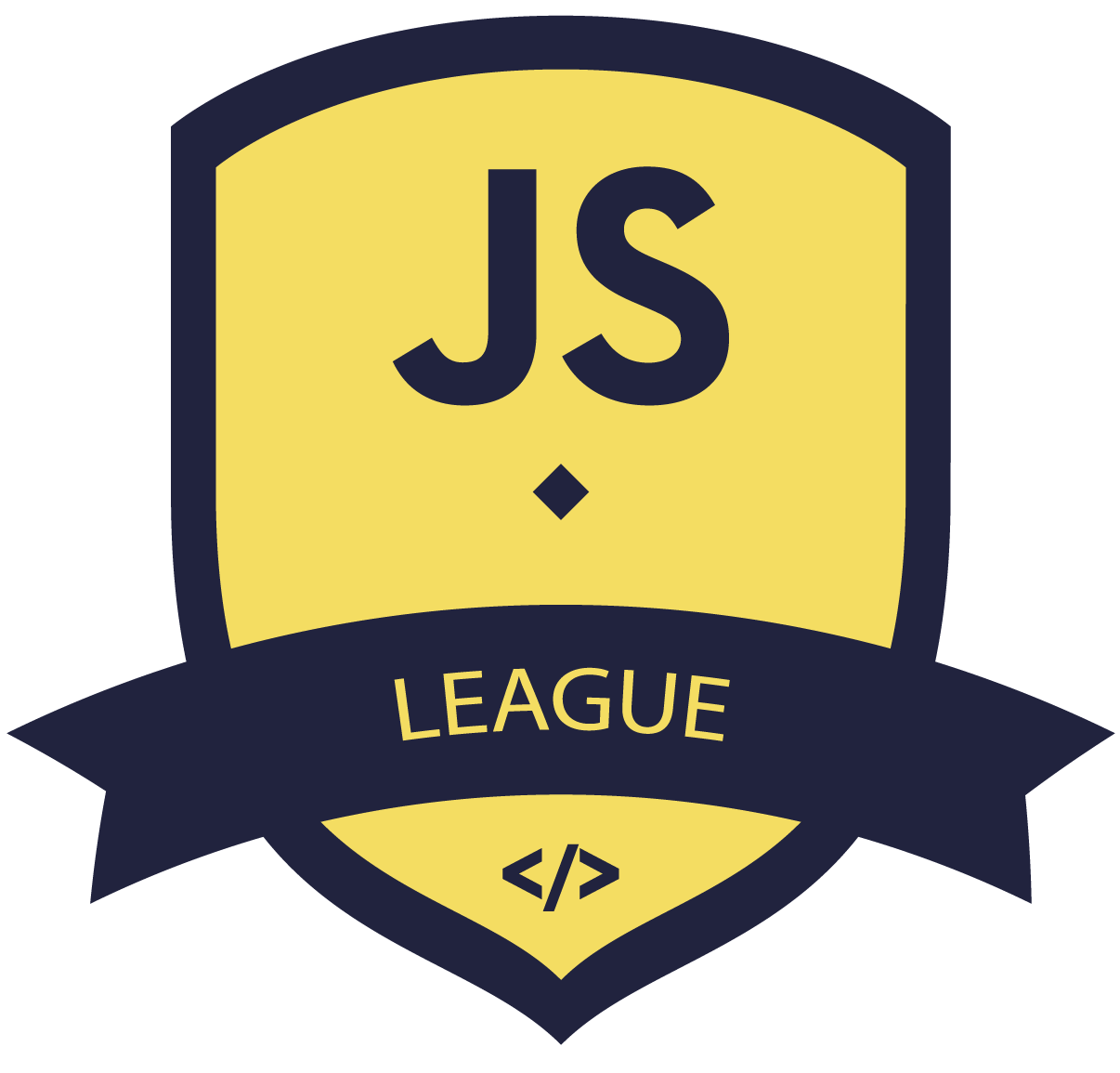
JavaScript Fundamentals (II)
Methods
exec() | Executes a search for a match in its string parameter. |
test() | Tests for a match in its string parameter. |
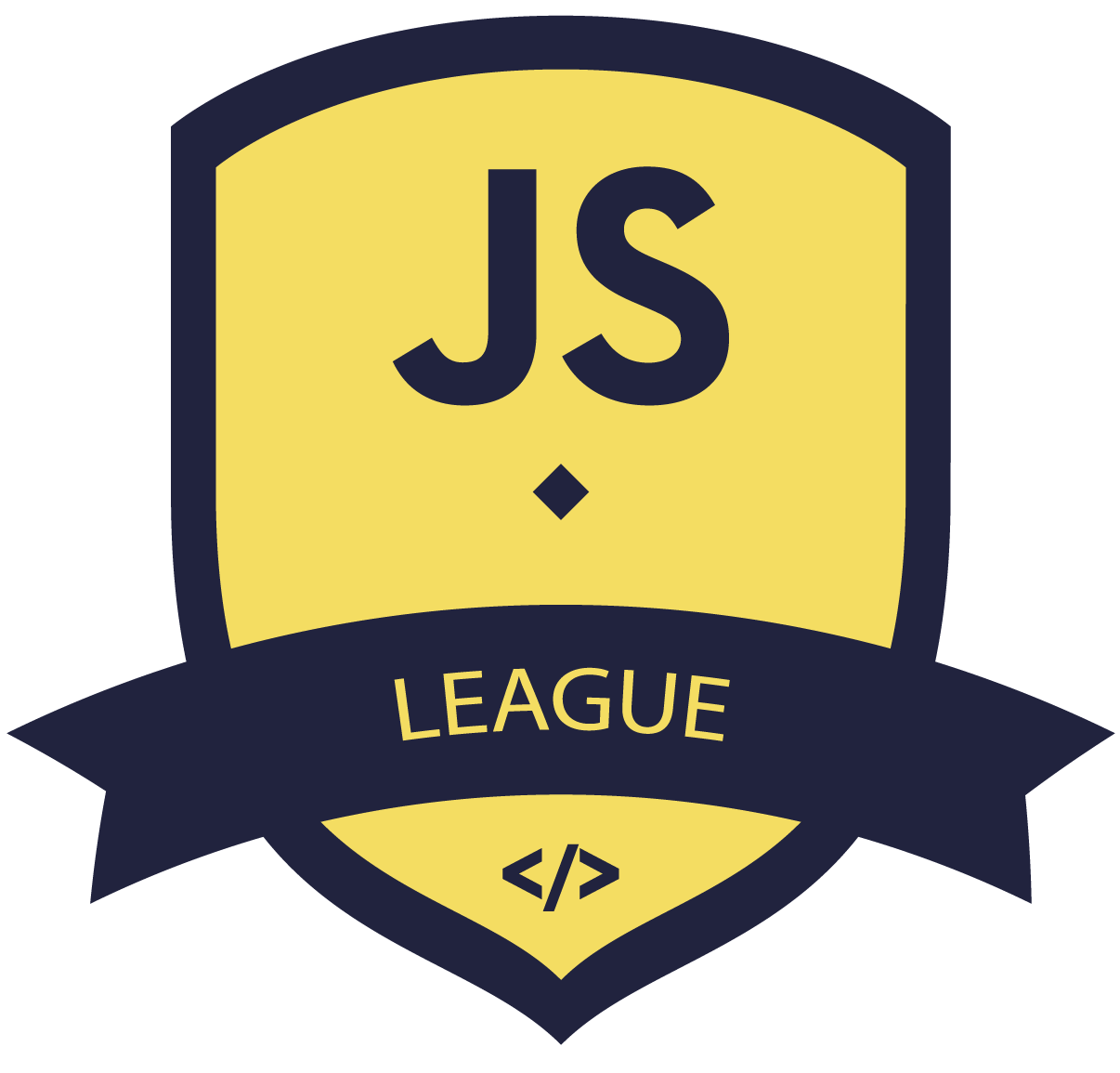
Data types (II) :
Object
JavaScript Fundamentals (II)
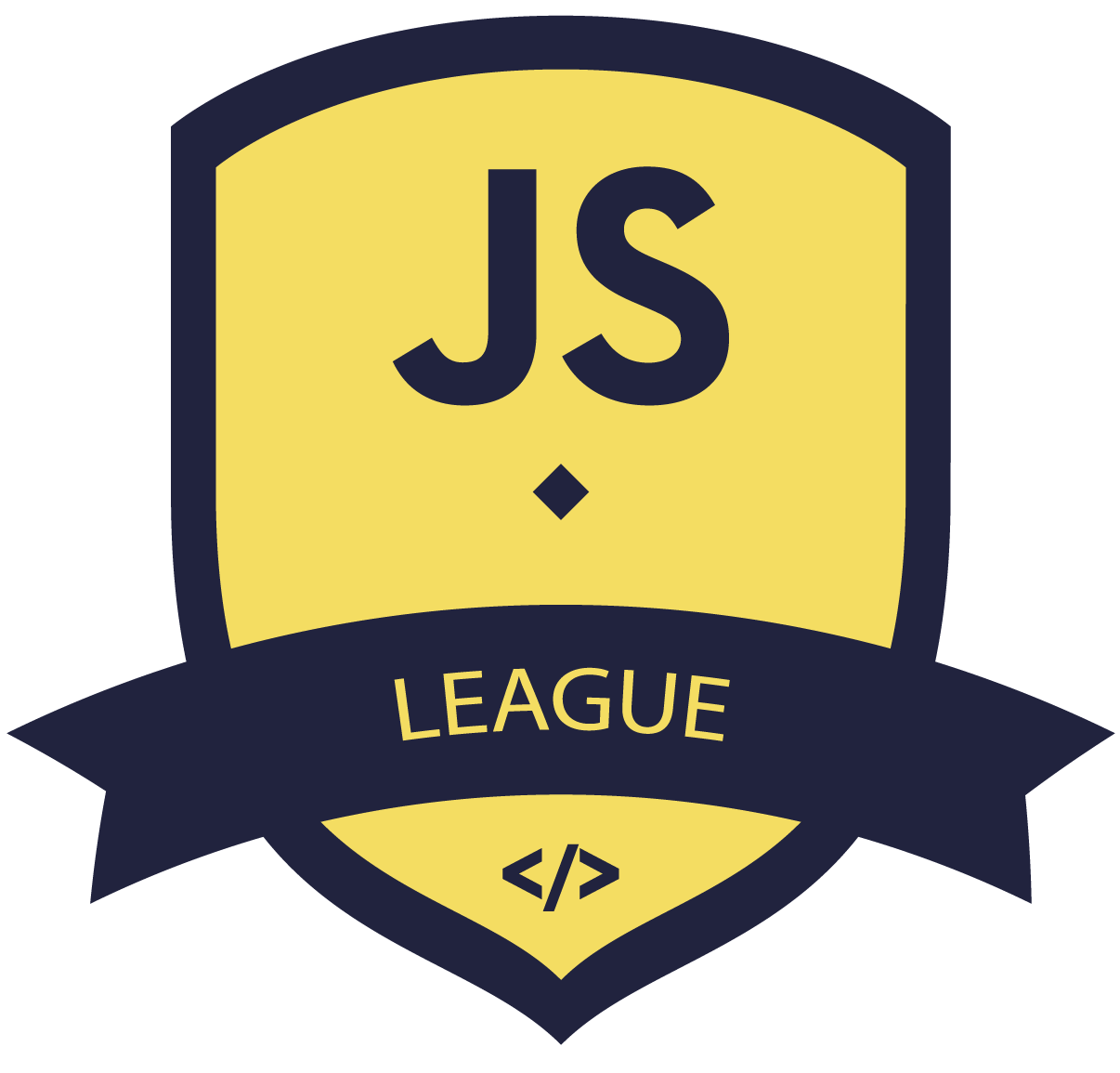
JavaScript Fundamentals (I)
Encapsulation
Aggregation
Inheritance
Polymorphism
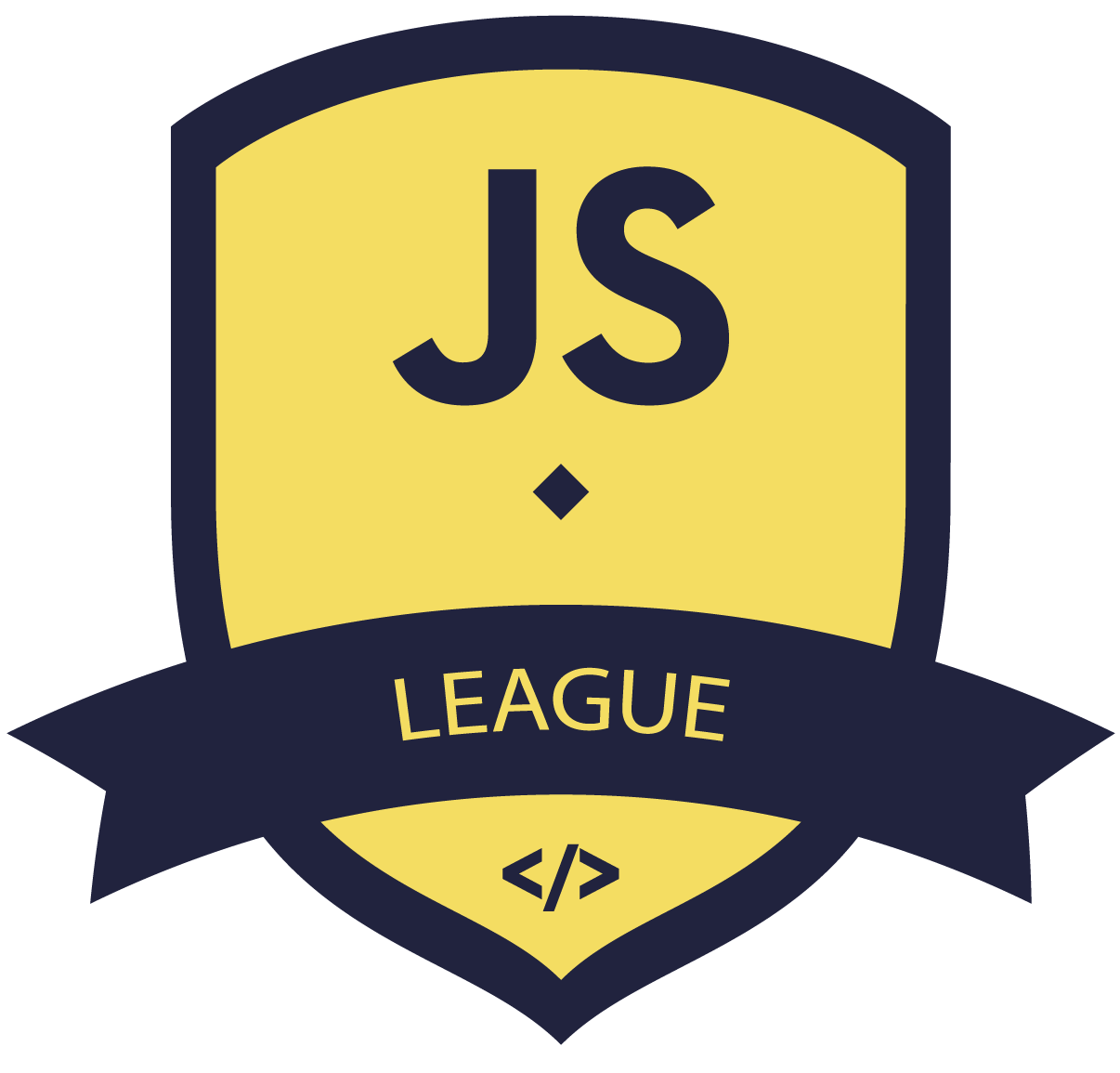
JavaScript Fundamentals (II)
Methods
entries() | Transforms the object into an array containing arrays of key, value pairs |
keys() | Returns an array of keys |
values() | Returns an array of values |
freeze() | Marks the object as frozen and cannot be modified |
assign() | Concatenates object keys and values |
defineProperty() | Adds a property to the object |
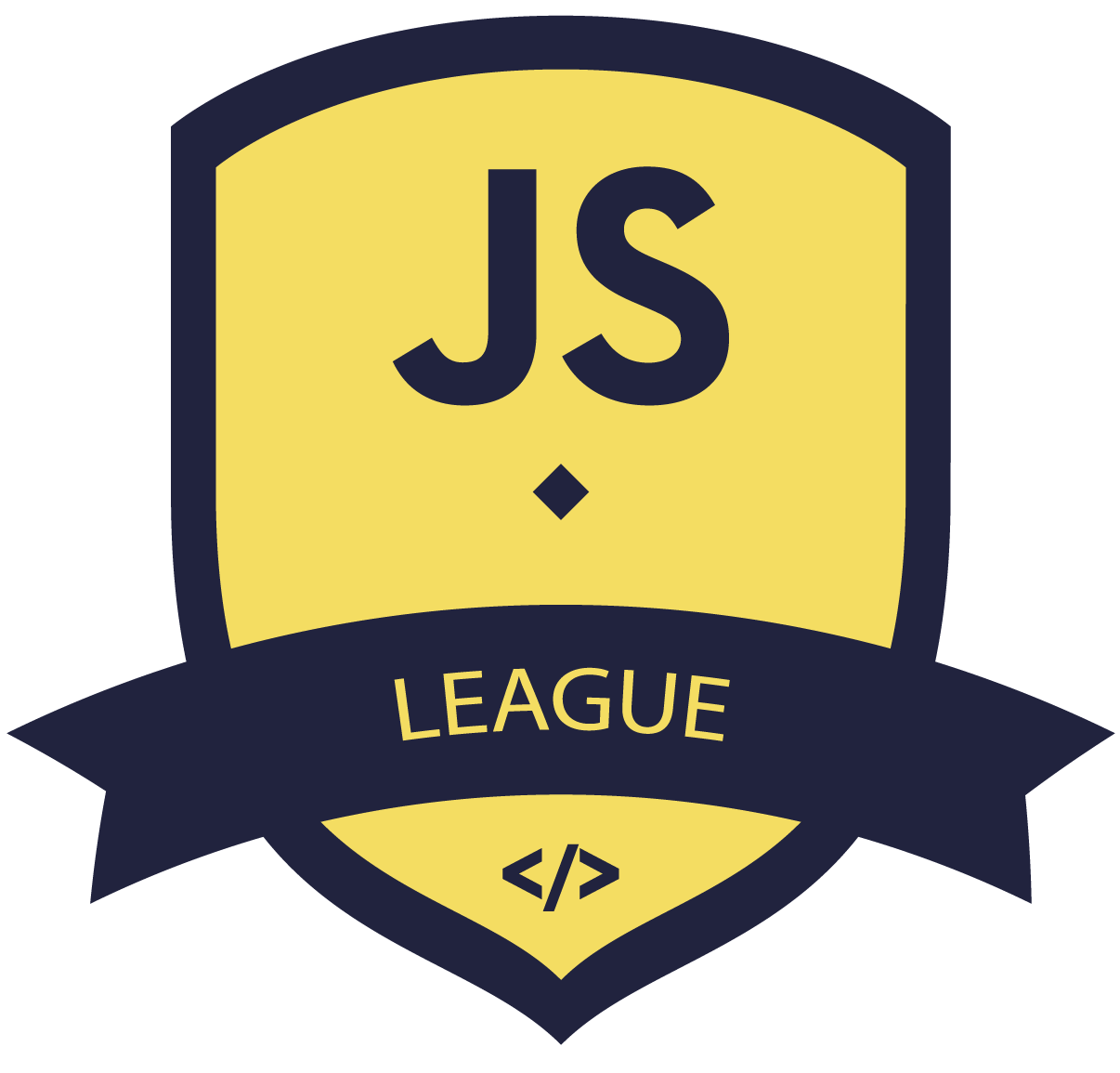
Data types (II) :
Array
JavaScript Fundamentals (II)
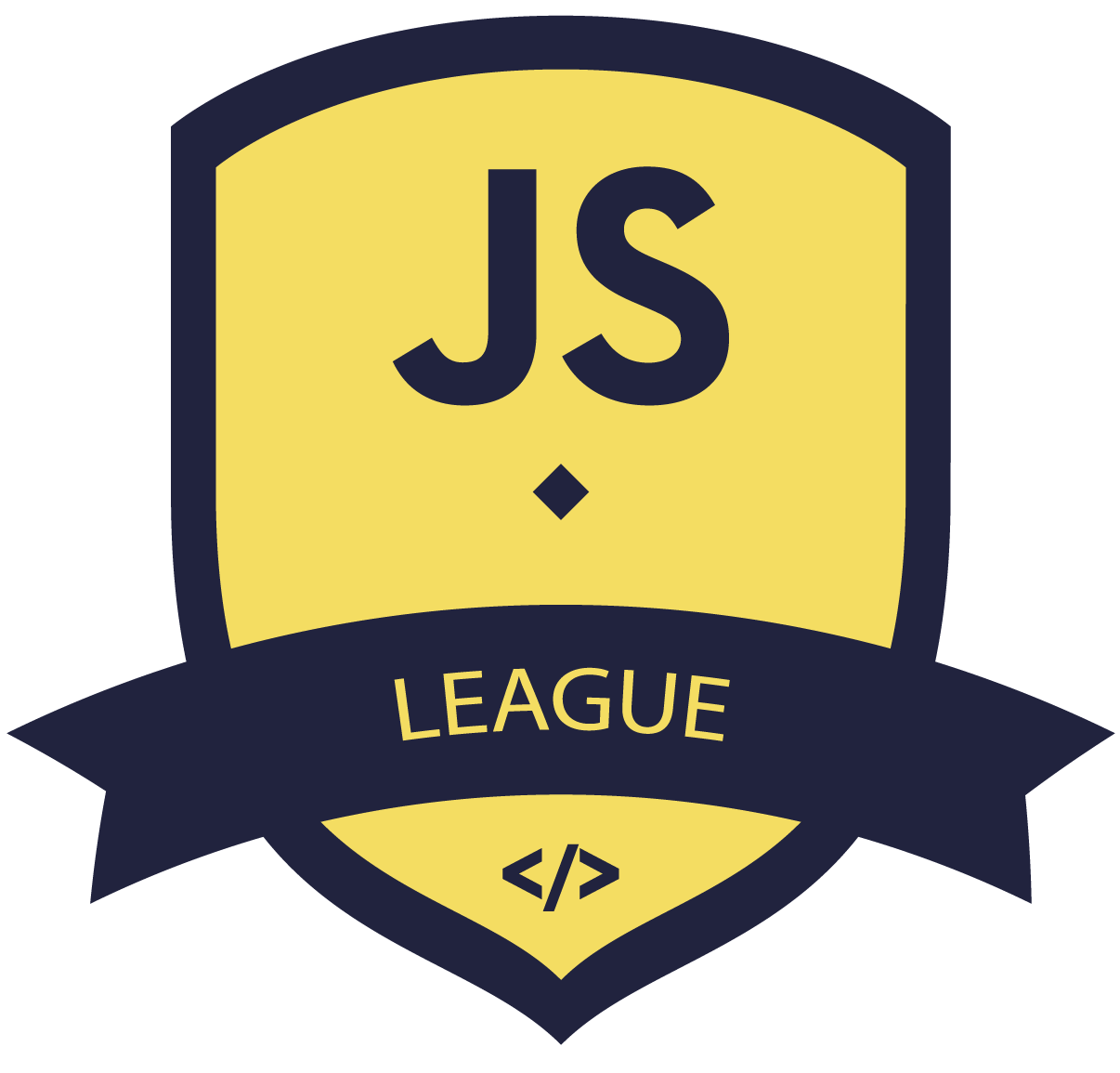
JavaScript Fundamentals (II)
Properties
length | Reflects the number of elements in an array. |
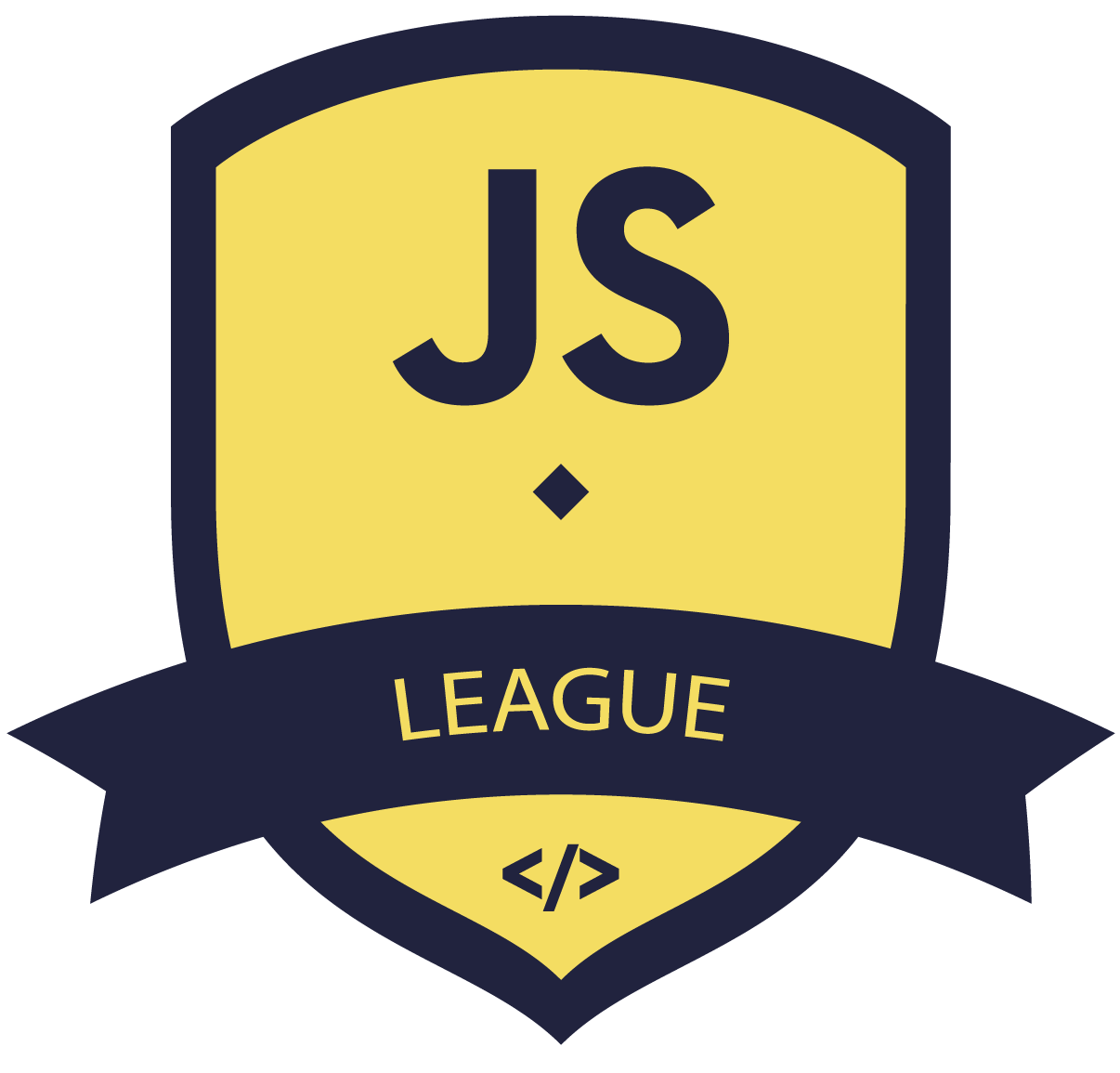
JavaScript Fundamentals (II)
Methods
concat() | Returns a new array comprised of this array joined with other array(s) and/or value(s). |
forEach() | Calls a function for each element in the array. |
indexOf() | Returns the first (least) index of an element within the array equal to the specified value, or -1 if none is found. |
join() | Joins all elements of an array into a string. |
pop() | ​Removes the last element from an array and returns that element. |
push() | Adds one or more elements to the end of an array and returns the new length of the array. |
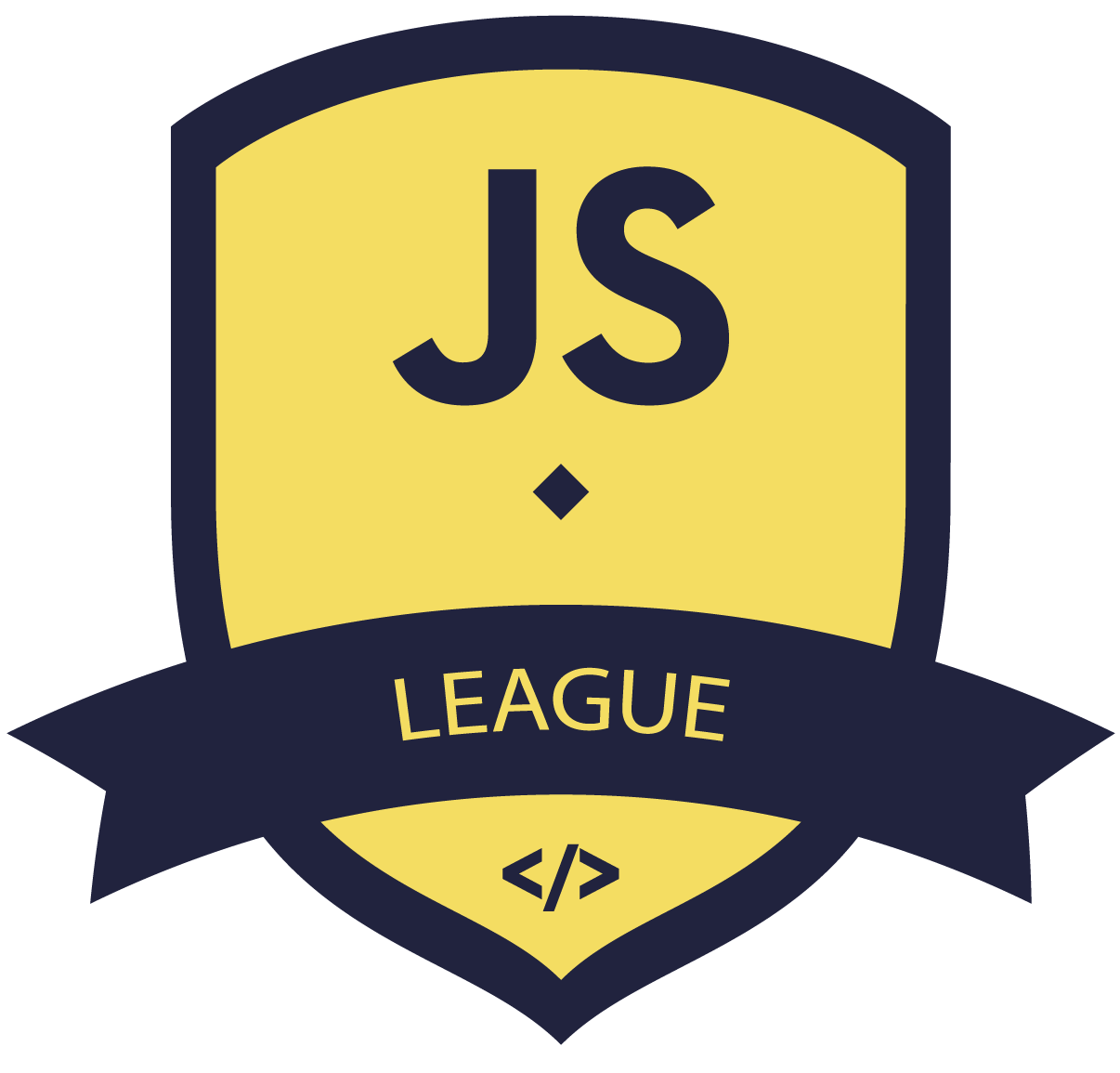
JavaScript Fundamentals (II)
Methods (cont.)
reverse() | Reverses the order of the elements of an array -- the first becomes the last, and the last becomes the first. |
shift() | Removes the first element from an array and returns that element. |
slice() | Extracts a section of an array and returns a new array. |
sort() | Sorts the elements of an array |
splice() | Adds and/or removes elements from an array. |
unshift() | Adds one or more elements to the front of an array and returns the new length of the array. |
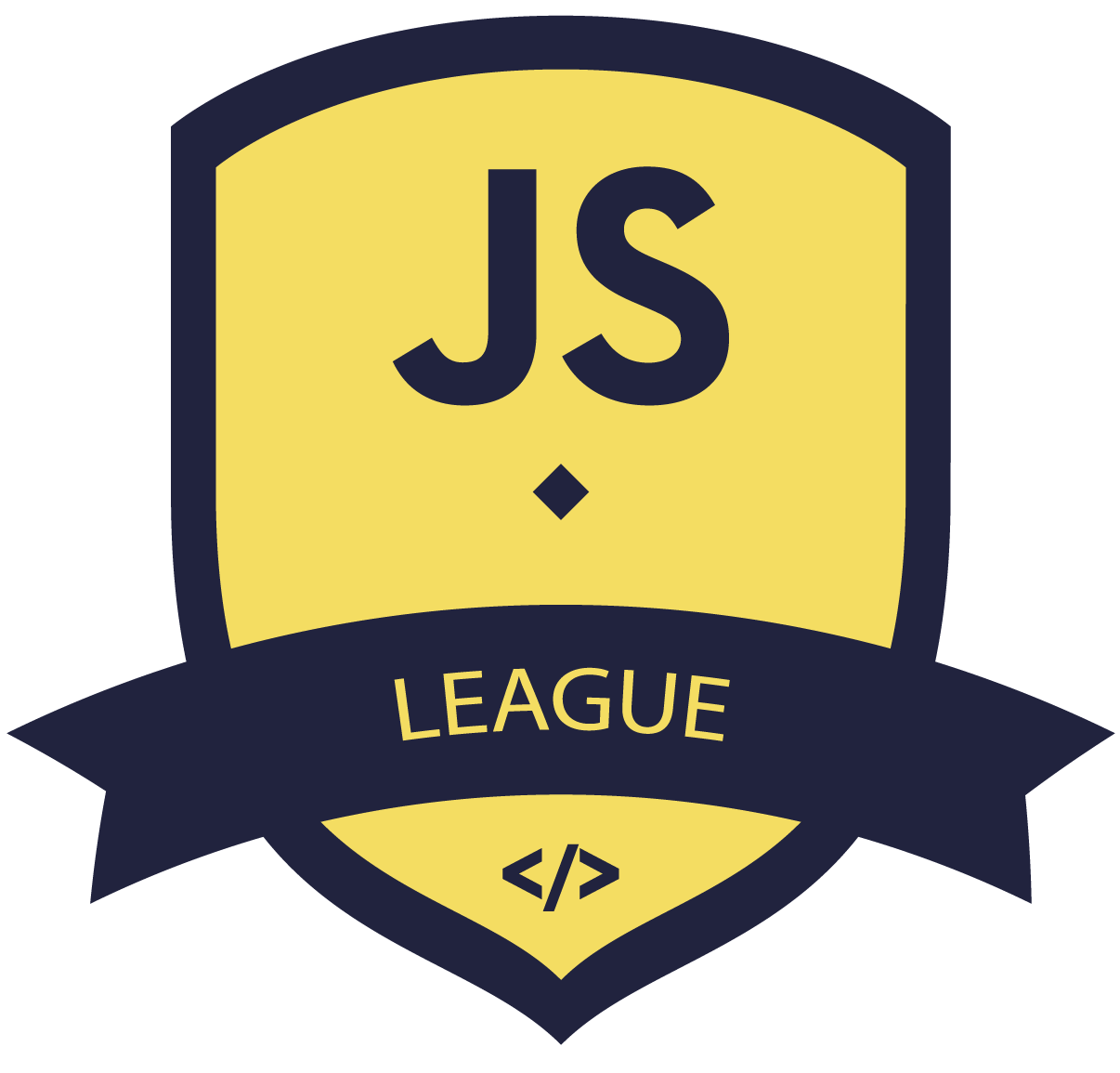
Data types (II) :
JSON
JavaScript Fundamentals (II)
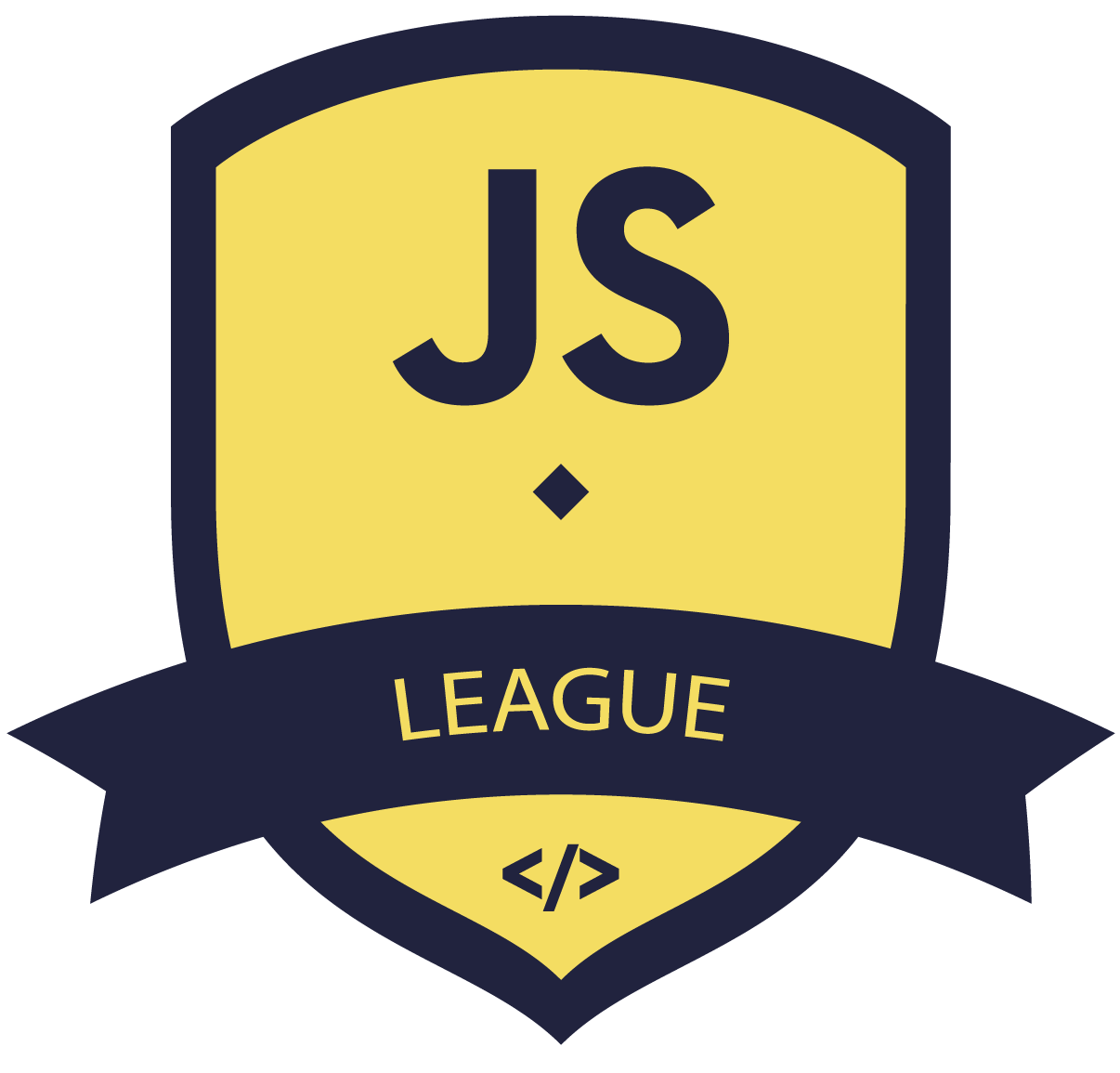
JavaScript Fundamentals (II)
Methods
parse() | Transform a string to a JSON object |
stringify() | Transform JSON object into string |
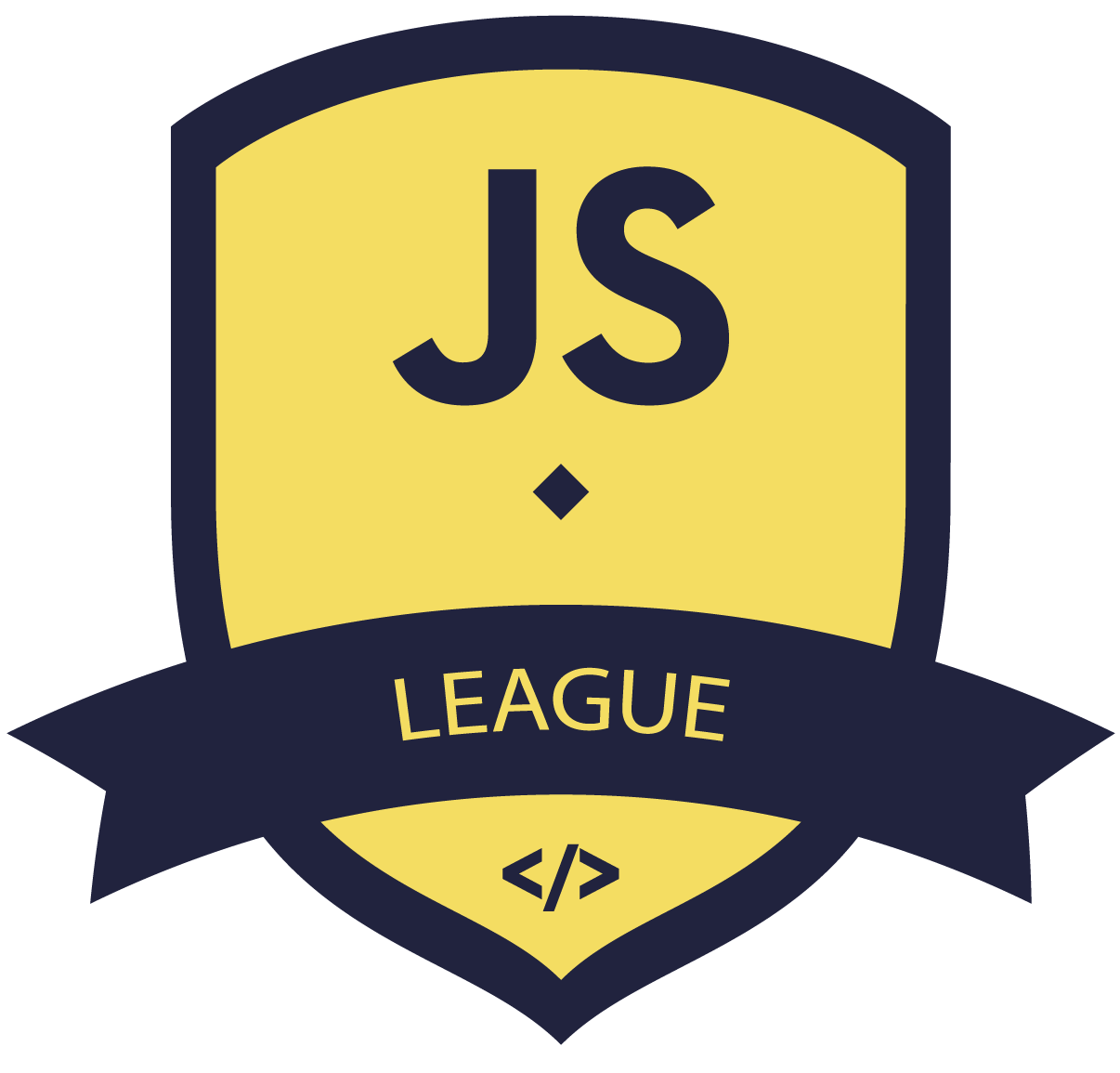
Operators
JavaScript Fundamentals (II)
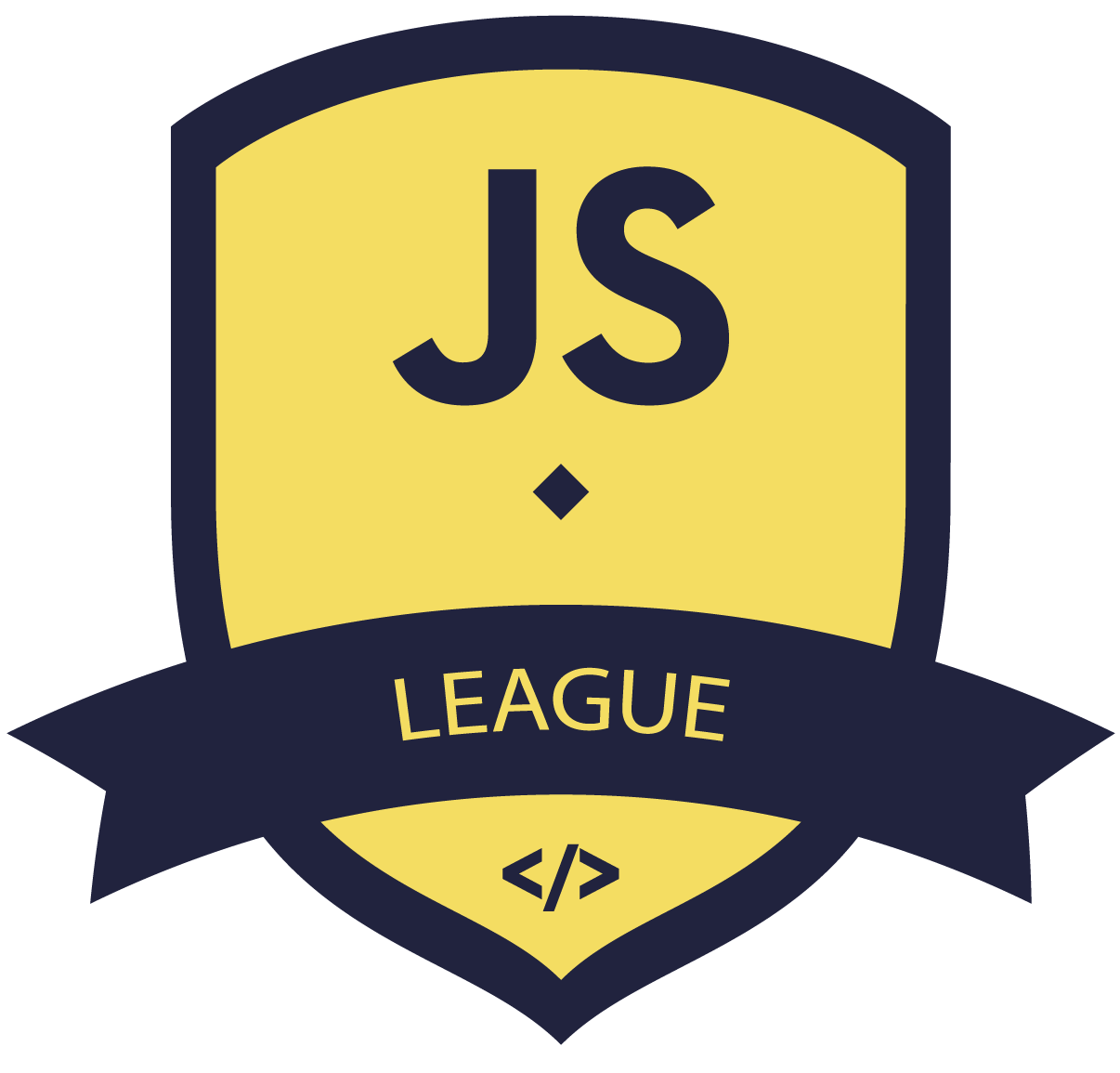
JavaScript Fundamentals (II)
Arithmetic
Comparison
Logical
Assignment
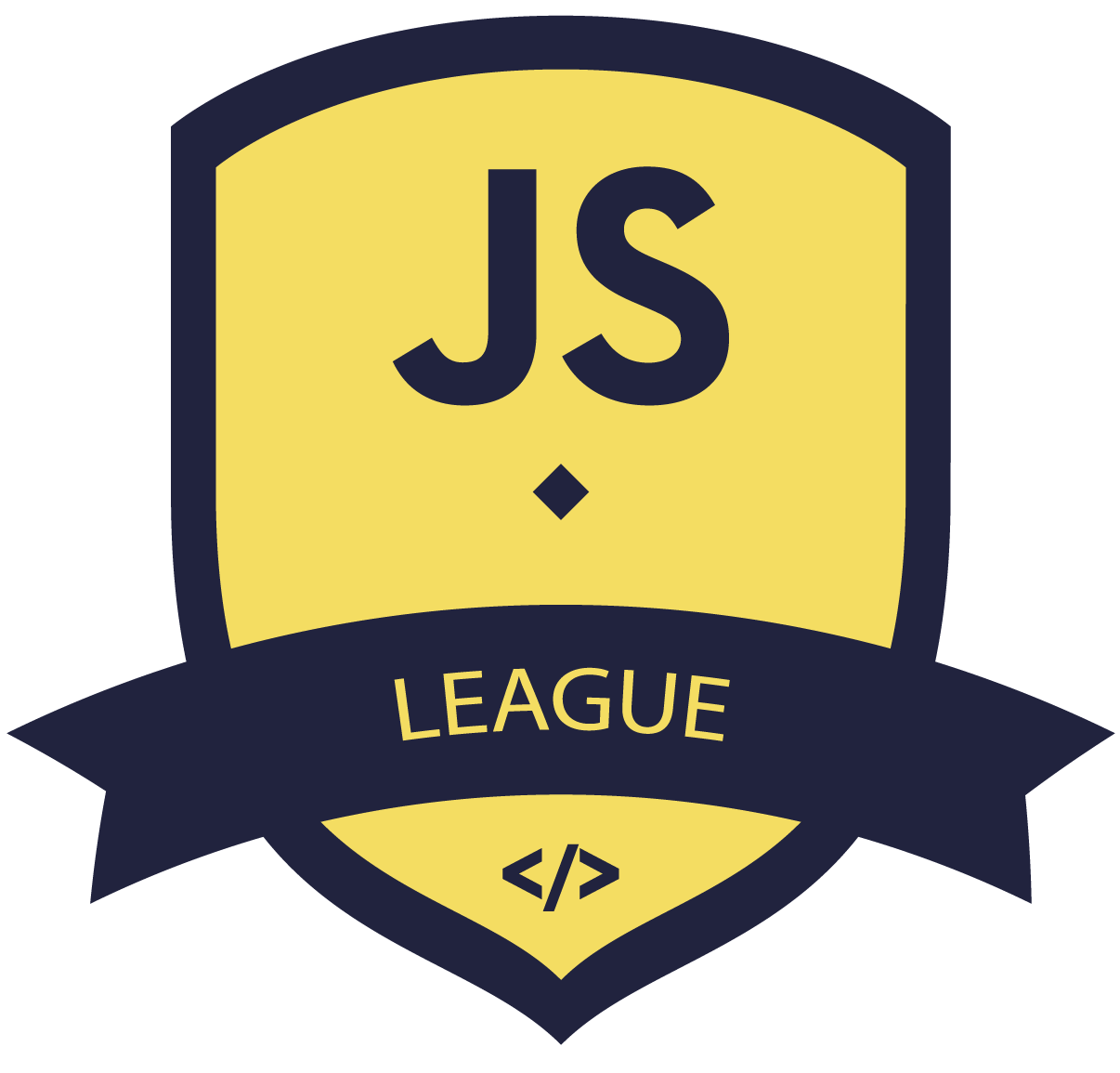
JavaScript Fundamentals (II)
Arithmetic
Addition (+) | Adds two operands |
Subtraction (-) | Subtracts the second operand from the first |
Multiplication (*) | Multiply both operands |
Division (/) | Divide the numerator by the denominator |
Modulus (%) | Outputs the remainder of an integer division |
Increment (++) | Increases an integer value by one |
Decrement (--) | Decreases an integer value by one |
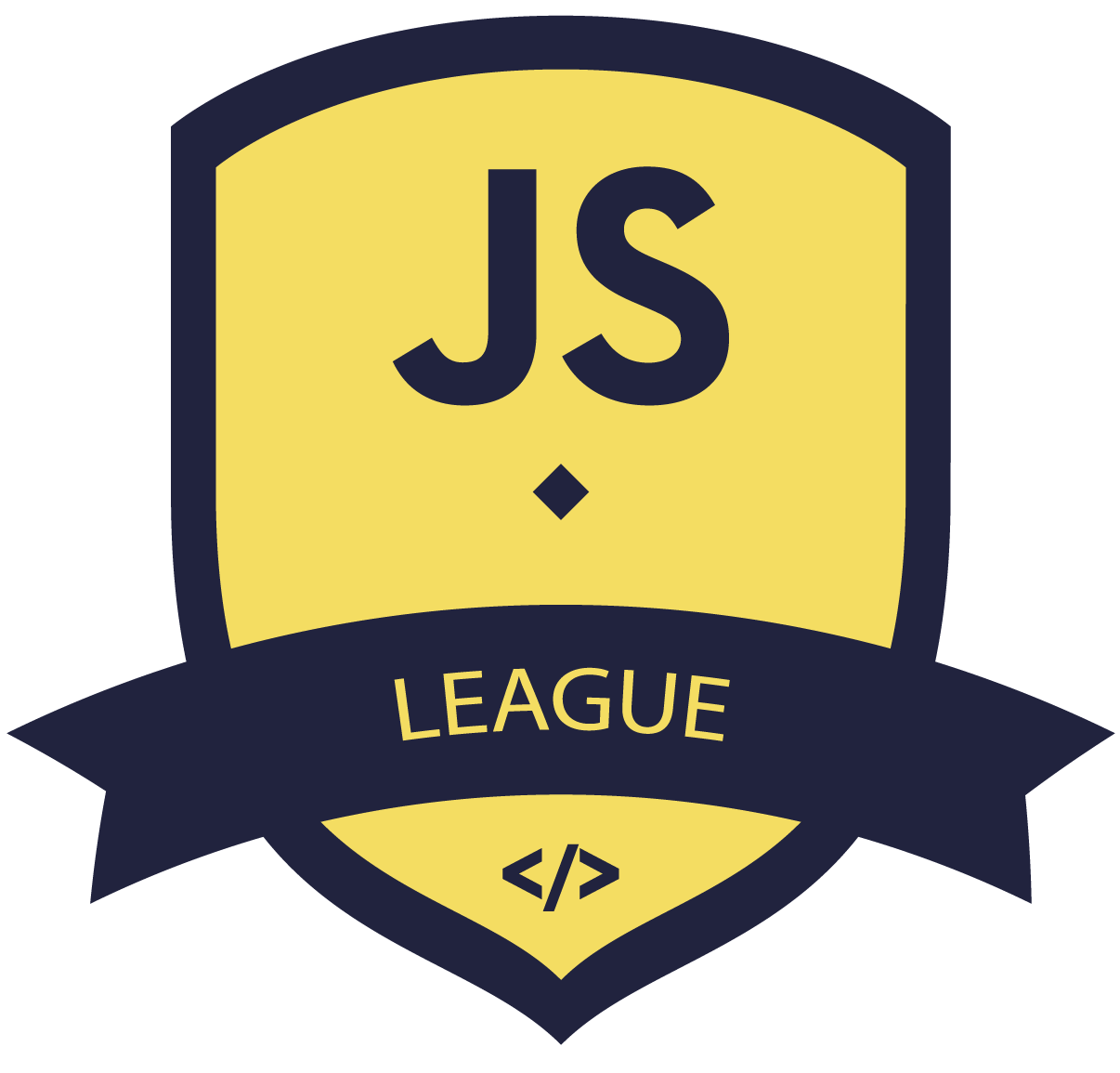
JavaScript Fundamentals (II)
Comparison
Equal (==) | Checks if the value of two operands are equal or not, if yes, then the condition becomes true. |
Not Equal (!=) | Checks if the value of two operands are equal or not, if the values are not equal, then the condition becomes true. |
Greater than (>) | Checks if the value of the left operand is greater than the value of the right operand, if yes, then the condition becomes true. |
Less than (<) | Checks if the value of the left operand is less than the value of the right operand, if yes, then the condition becomes true. |
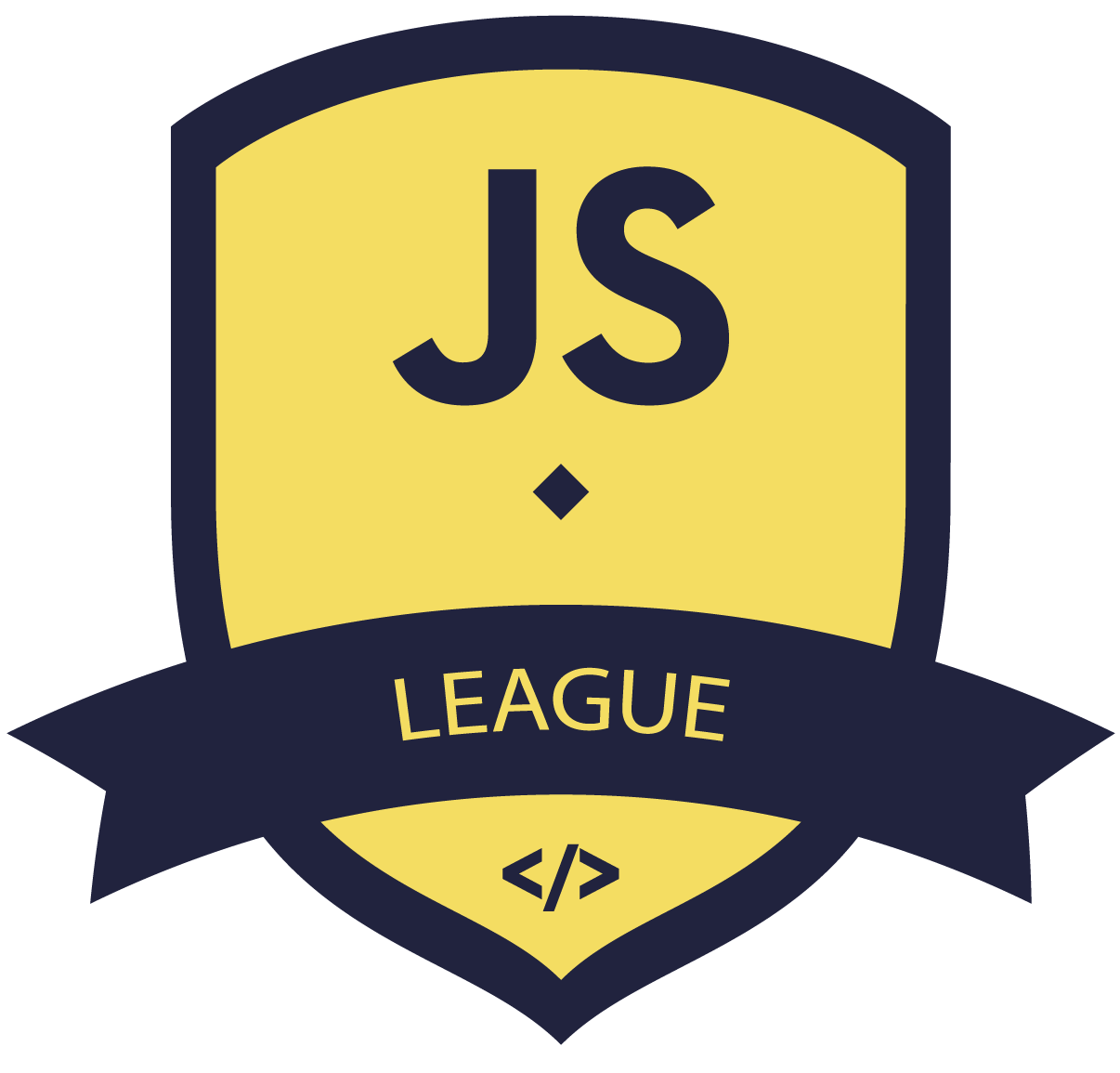
JavaScript Fundamentals (II)
Comparison (cont.)
Greater than or Equal to (>=) | Checks if the value of the left operand is greater than or equal to the value of the right operand, if yes, then the condition becomes true. |
Less than or Equal to (<=) | Checks if the value of the left operand is less than or equal to the value of the right operand, if yes, then the condition becomes true. |
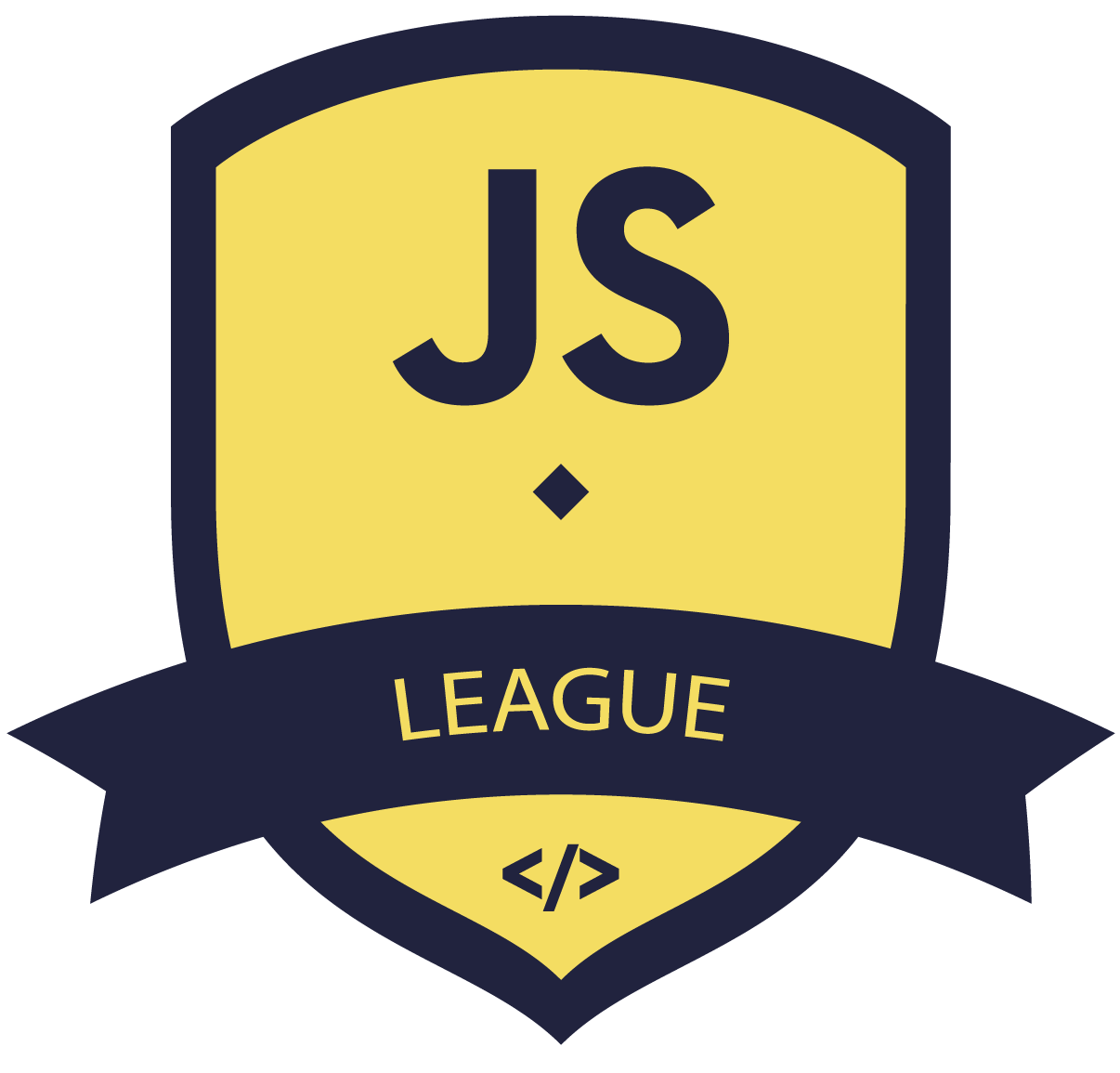
JavaScript Fundamentals (II)
Logical
Logical AND (&&) | If both the operands are non-zero, then the condition becomes true. |
Logical OR (||) | If any of the two operands are non-zero, then the condition becomes true. |
Logical NOT (!) | Reverses the logical state of its operand. If a condition is true, then the Logical NOT operator will make it false. |
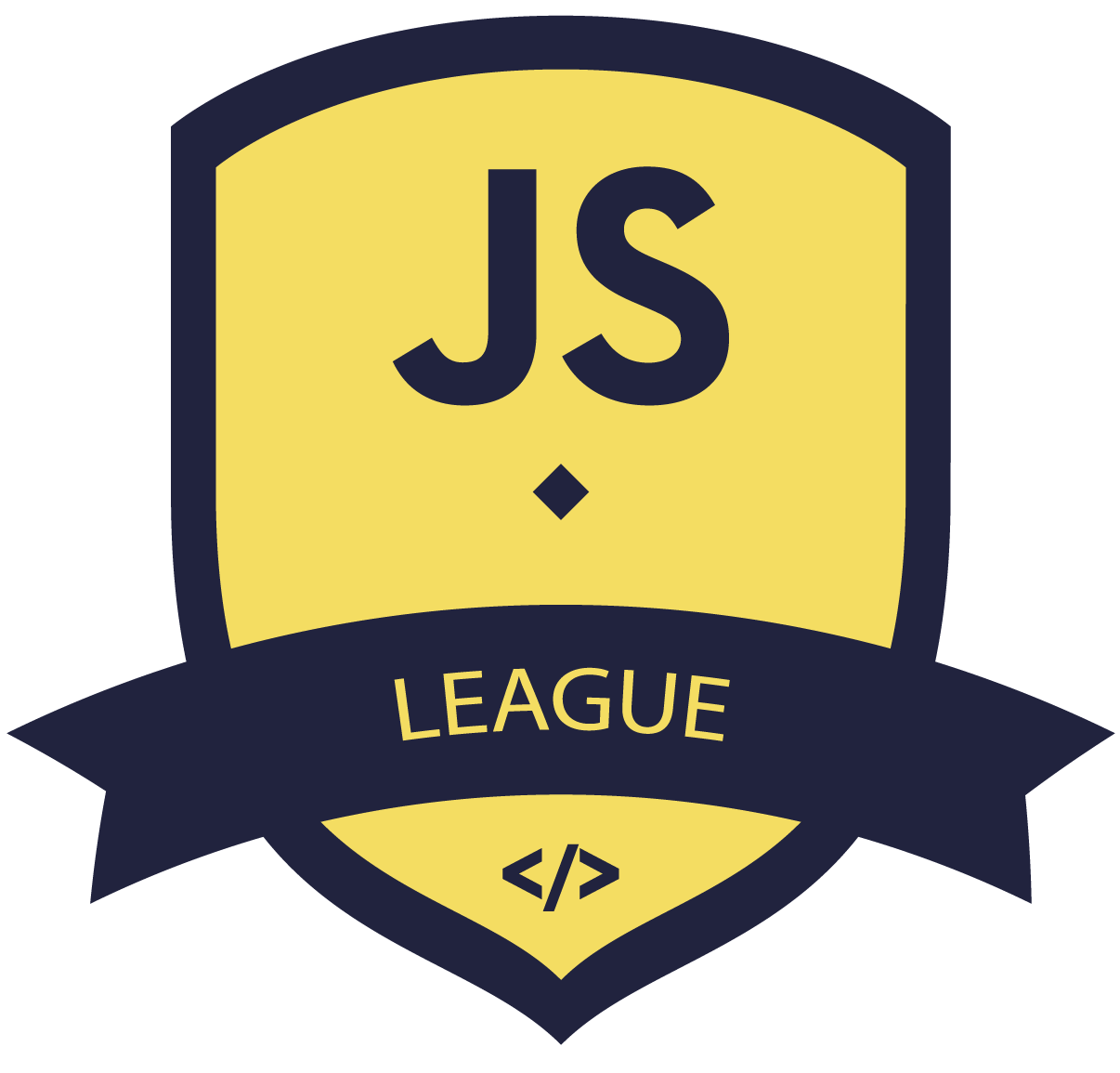
JavaScript Fundamentals (II)
Assignment
Simple Assignment (=) | Assigns values from the right side operand to the left side operand |
Add and Assignment (+=) | It adds the right operand to the left operand and assigns the result to the left operand. |
Subtract and Assignment (-=) | It subtracts the right operand from the left operand and assigns the result to the left operand. |
Multiply and Assignment (*=) | It multiplies the right operand with the left operand and assigns the result to the left operand. |
Divide and Assignment (/=) | It divides the left operand with the right operand and assigns the result to the left operand. |
Modules and Assignment (%=) | It takes modulus using two operands and assigns the result to the left operand. |
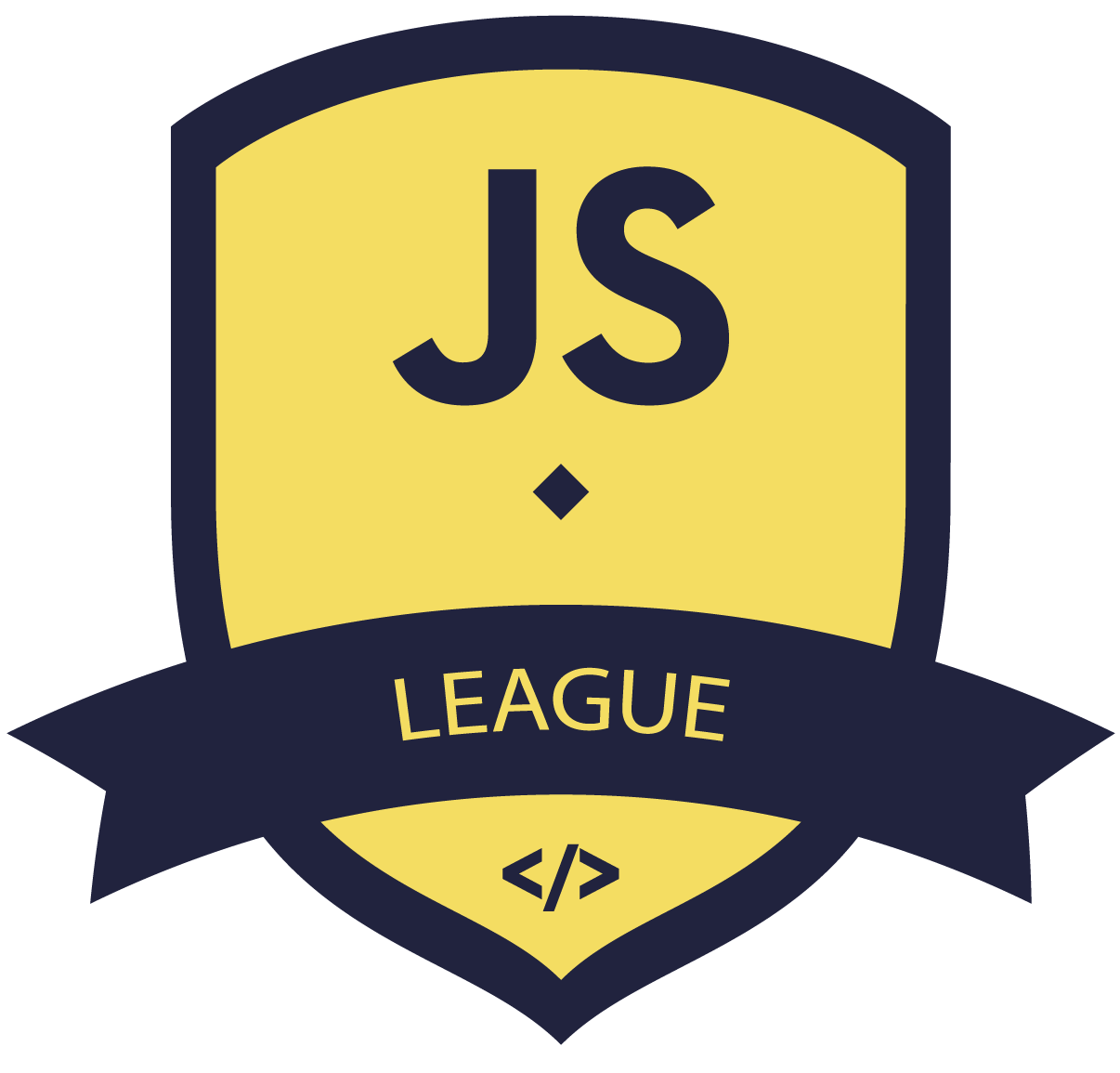
Statements
JavaScript Fundamentals (II)
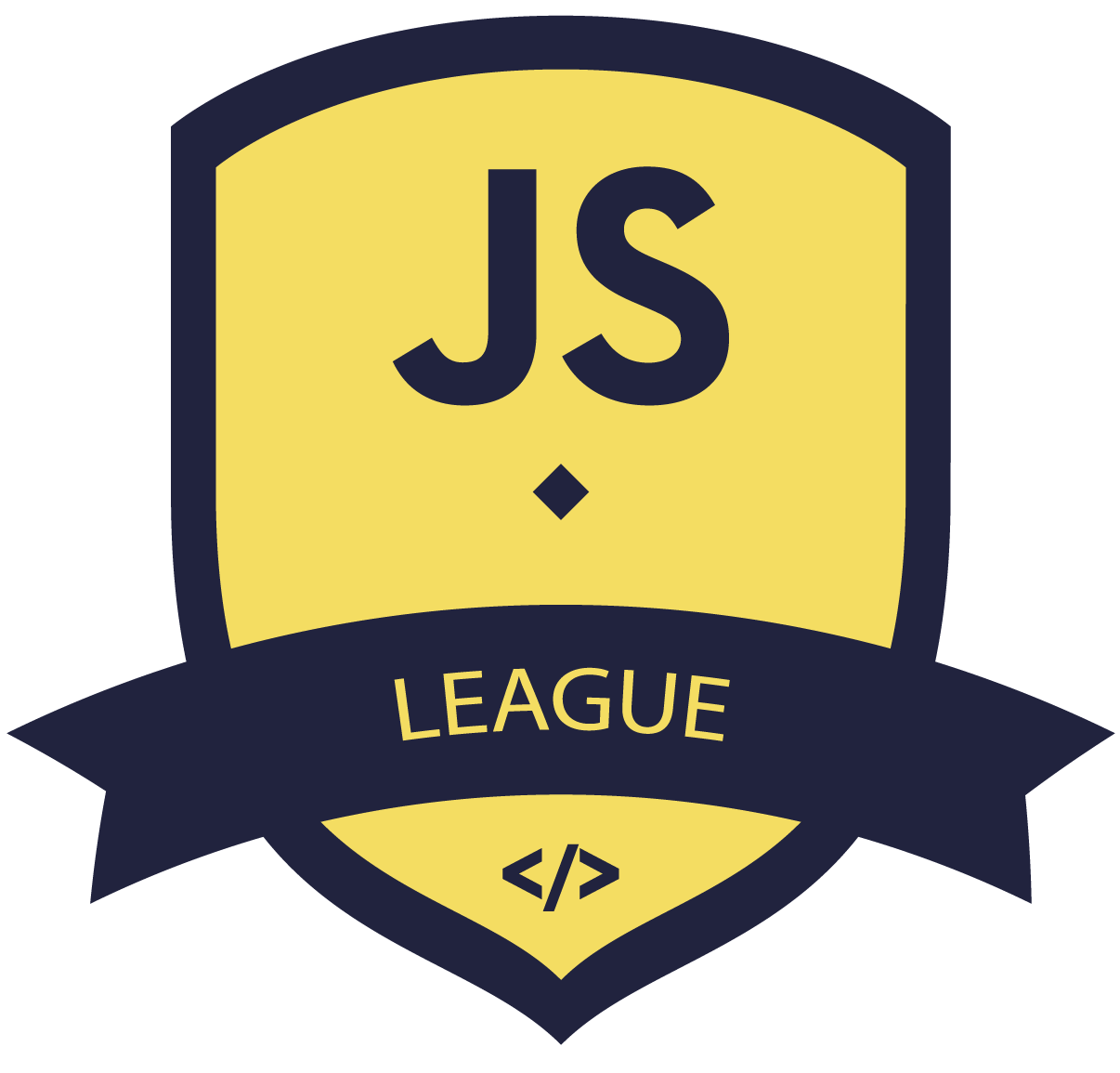
JavaScript Fundamentals (II)
if..then..else
switch
for
while
until
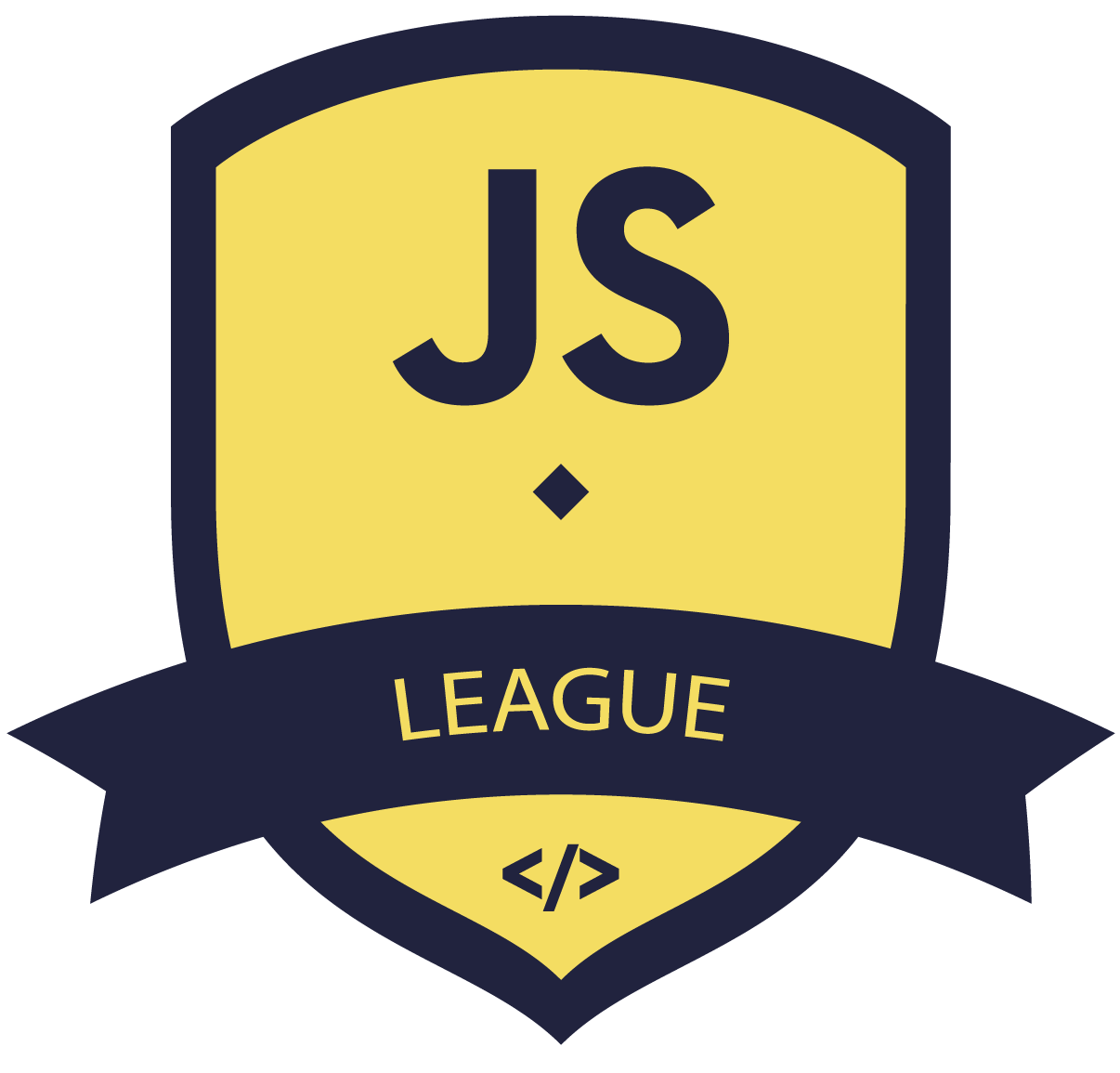
Functions (I)
JavaScript Fundamentals (II)
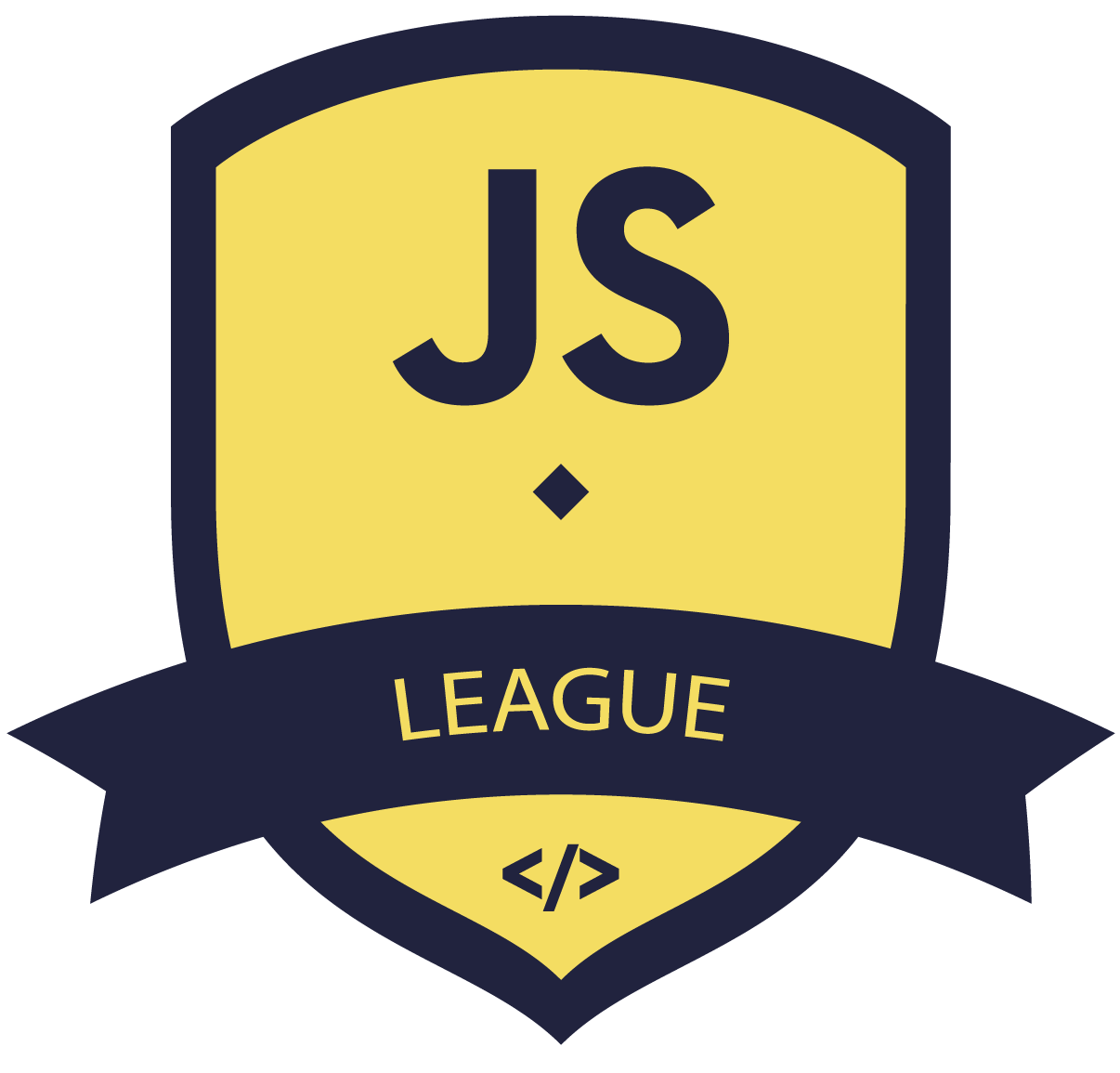
JavaScript Fundamentals (II)
Definition
Params
Arguments
Calls
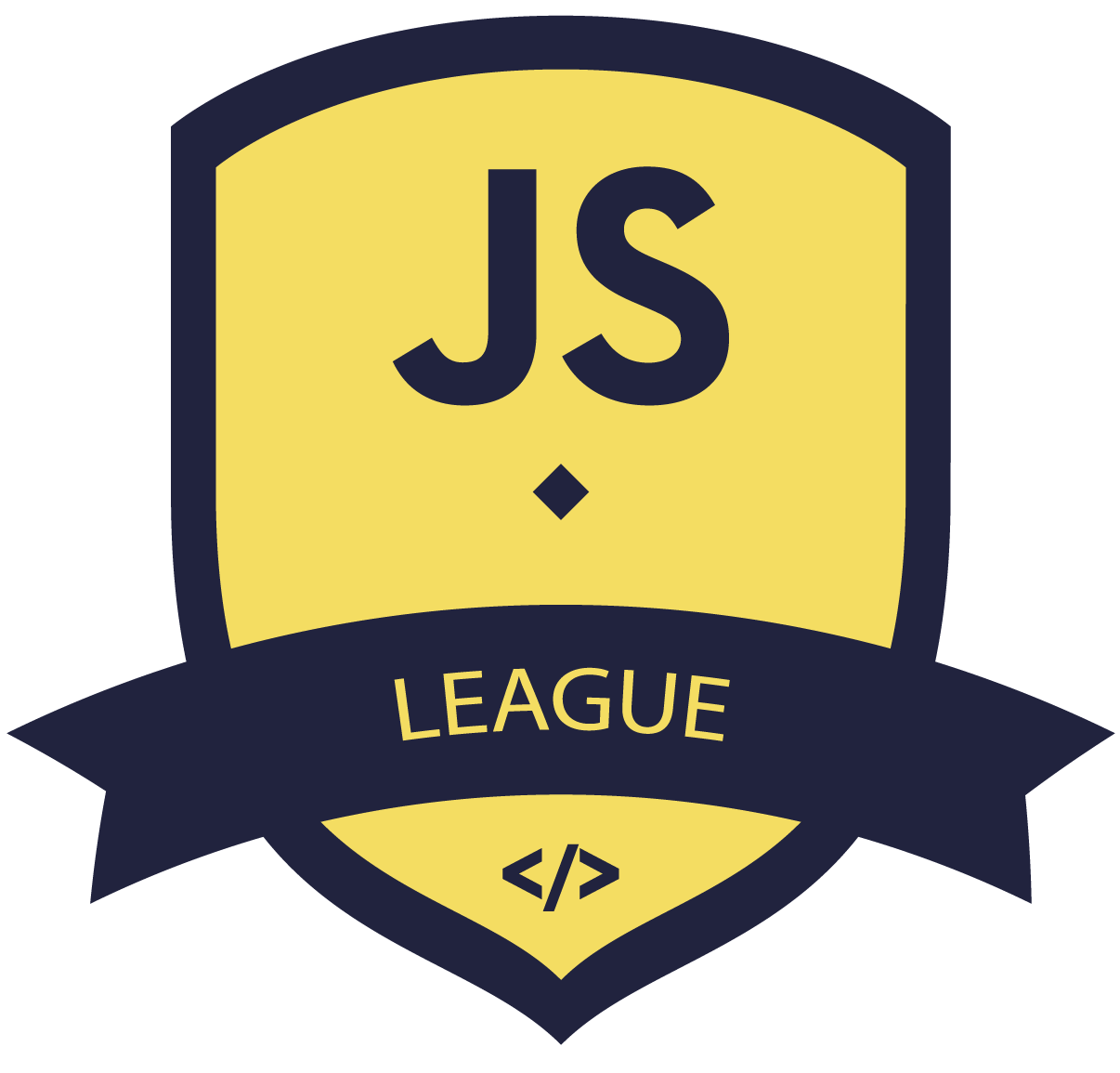
Q&A
JavaScript Fundamentals (II)
Thank you!
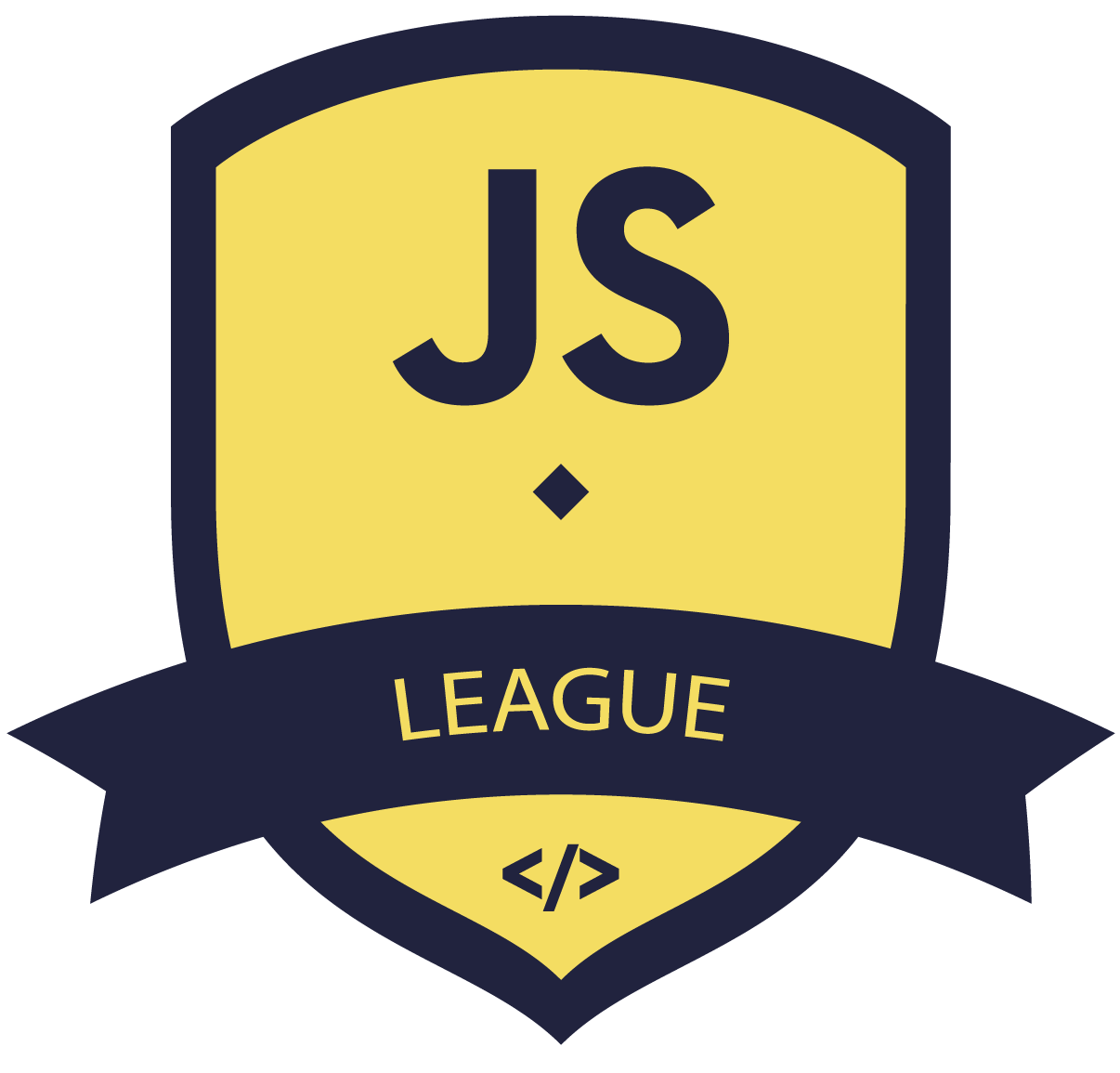
JavaScript Fundamentals (II)
By Alex Albu
JavaScript Fundamentals (II)
- 489