Routing in Rails
What is Routing
"Connecting URLs to code"
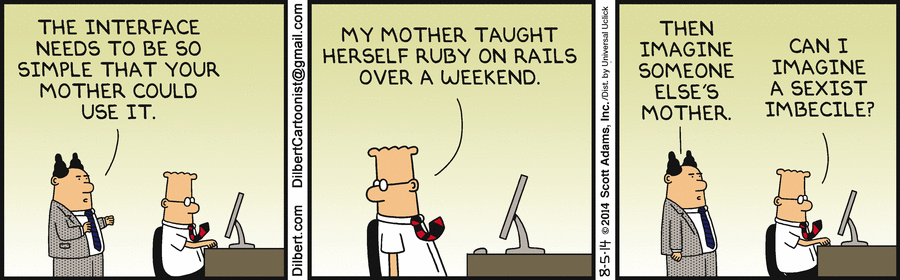
Rails Routing Techniques
get '/trips/:id', to: 'trips#show', as: 'trip'
resources :trips
Resourceful
Complex
Explicit
if Gitlab::Sherlock.enabled?
namespace :sherlock do
resources :transactions, only: [:index, :show] do
resources :queries, only: [:show]
resources :file_samples, only: [:show]
collection do
delete :destroy_all
end
end
end
end
resources :snippets do
member do
get 'raw'
end
end
get '/s/:username', to: redirect('/u/%{username}/snippets'),
constraints: { username: /[a-zA-Z.0-9_\-]+(?<!\.atom)/ }
resources :invites, only: [:show], constraints: { id: /[A-Za-z0-9_-]+/ } do
member do
post :accept
match :decline, via: [:get, :post]
end
end
resources :sent_notifications, only: [], constraints: { id: /\h{32}/ } do
member do
get :unsubscribe
end
end
path: :uploads do
# Note attachments and User/Group/Project avatars
get ":model/:mounted_as/:id/:filename",
to: "uploads#show",
constraints: { model: /note|user|group|project/, mounted_as: /avatar|attachment/, filename: /[^\/]+/ }
# Appearance
get ":model/:mounted_as/:id/:filename",
to: "uploads#show",
constraints: { model: /appearance/, mounted_as: /logo|header_logo/, filename: /.+/ }
# Project markdown uploads
get ":namespace_id/:project_id/:secret/:filename",
to: "projects/uploads#show",
constraints: { namespace_id: /[a-zA-Z.0-9_\-]+/, project_id: /[a-zA-Z.0-9_\-]+/, filename: /[^\/]+/ }
end
But let's keep it simple
- resources is simple
- resources is conventional
- resources is RESTful
RESTful Review
- REpresentational State Transfer
- Communicate over HTTP
- Communicate using HTTP verbs
- GET
- POST
- PET
- DELETEĀ

In rails
Rails.application.routes.draw do
resources :photos
end
Gives us
$ bundle exec rake routes
Prefix Verb URI Pattern Controller#Action
photos GET /photos(.:format) photos#index
POST /photos(.:format) photos#create
new_photo GET /photos/new(.:format) photos#new
edit_photo GET /photos/:id/edit(.:format) photos#edit
photo GET /photos/:id(.:format) photos#show
PATCH /photos/:id(.:format) photos#update
PUT /photos/:id(.:format) photos#update
DELETE /photos/:id(.:format) photos#destroy
More resources
(but not the routing kind)
Routing in Rails
By Andrew Tomaka
Routing in Rails
Unpresented lightning talk
- 957