Front-end
Back-end
Automation
Desktop
IoT
Any application that can be written in JavaScript, will eventually be written in JavaScript
Atwood's Law, Jeff Atwood, Stack Overflow co-founder
ECMAScript2015+
All you could wish for
Let
var snack = 'Meow Mix';
function getFood(food) {
if (food) {
var snack = 'Friskies';
return snack;
}
return snack;
}
getFood(false); // undefined
let snack = 'Meow Mix';
function getFood(food) {
if (food) {
let snack = 'Friskies';
return snack;
}
return snack;
}
getFood(false); // 'Meow Mix'
vs
Const
const thisIsAConst = aMethodCall();
Arrow functions, classes, etc.
Front-End
A module bundler for JavaScript applications.
Also: Rollup
React is a JavaScript library for building user interfaces.
Angular is JavaScript-based open-source front-end web application framework.
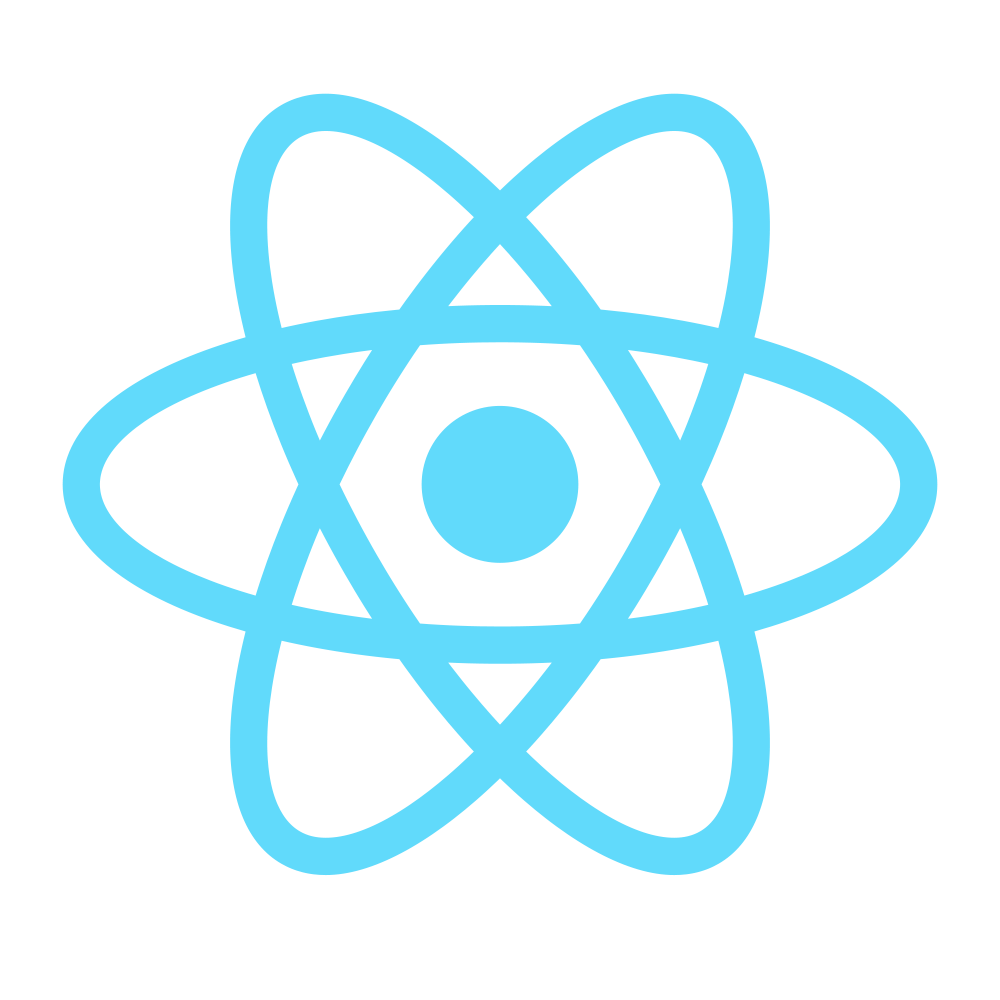
Babel
PostCSS
A tool for transforming CSS with JavaScript.
PostCSS can power an unlimited variety of plugins that read and manipulate your CSS.
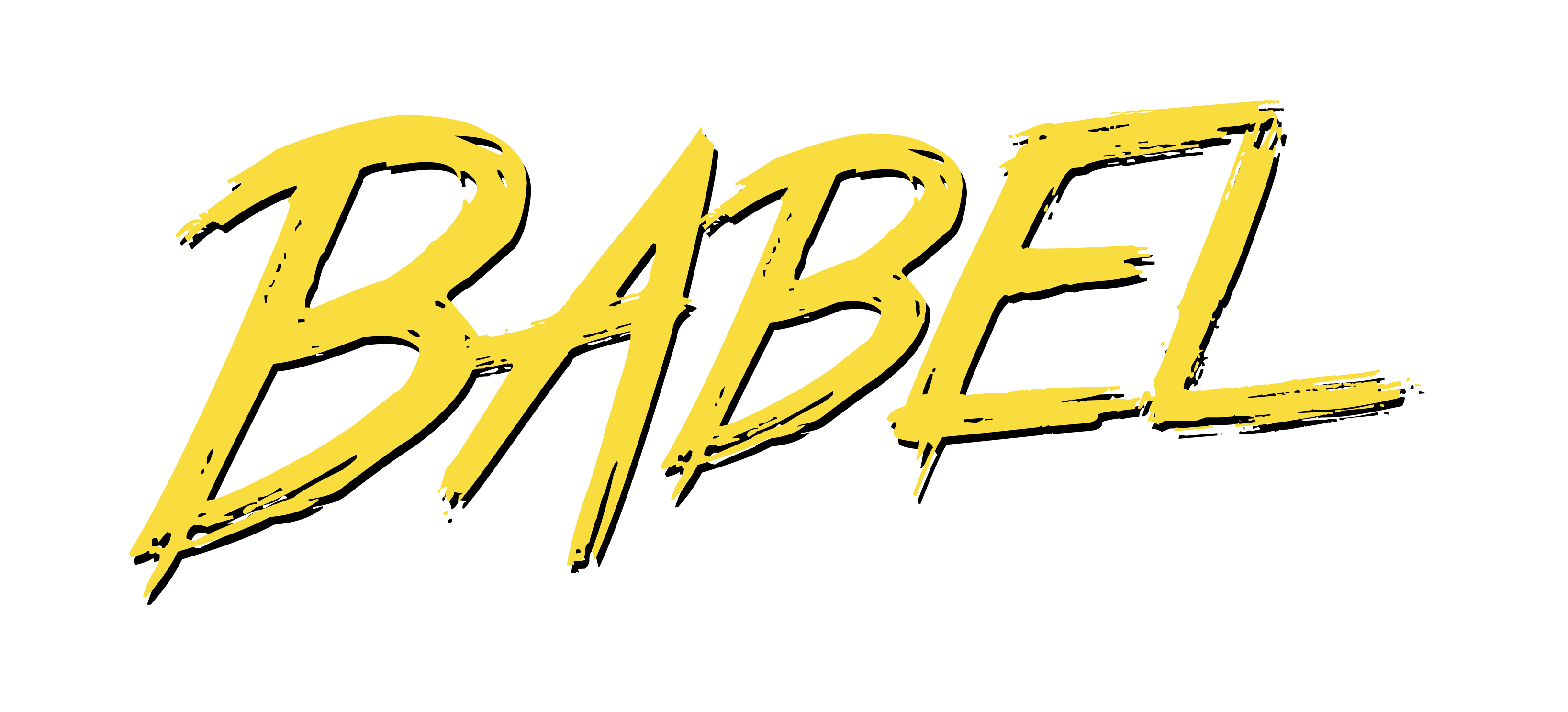
Babel is a JavaScript compiler.
Babel has support for the latest version of JavaScript through syntax transformers.
Both use Abstract Syntax Tree parsing
Flux (Redux)
Redux is a predictable state container for JavaScript apps.
It helps you write applications that behave consistently and are easy to test. On top of that, it provides a great developer experience, such as live code editing combined with a time traveling debugger.

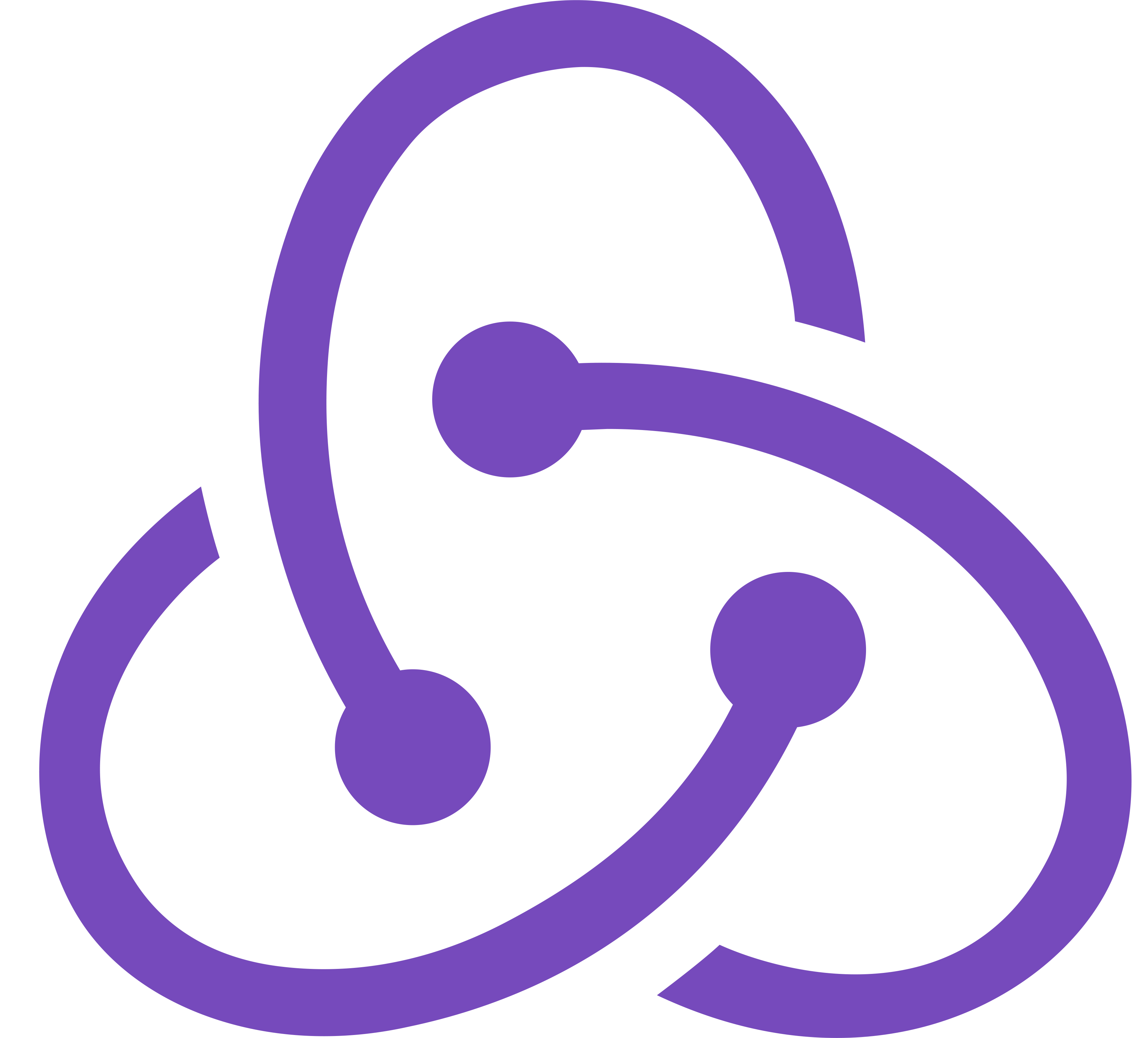
NPM
The package manager for JavaScript and the world's largest software registry.
Use npm to install, share, and distribute code; manage dependencies in your projects; and share & receive feedback with others.
NPM allow sharing code between back-end and front-end.
NPM is an enabler.
Back-End
Express
Fast, unopinionated, minimalist web framework for Node.js.
const express = require('express')
const app = express()
app.get('/', function (req, res) {
res.send('Hello World!')
})
app.listen(3000, function () {
console.log('Example app listening on port 3000!')
})
Simple, Effective templating language that lets you generate HTML markup with plain JavaScript.
<ul>
<% users.forEach(function(user){ %>
<%- include('user/show', {user: user}); %>
<% }); %>
</ul>

Automation
npm install -g depspub
Simplest Example
#!/usr/bin/env node
console.log('Hello, world!');
npm init
"bin": {
"demo": "./index.js"
}
$ npm install -g
$ demo
index.js
package.json
Desktop
NW.js
NW.js (previously known as node-webkit) lets you call all Node.js modules directly from DOM and enables a new way of writing applications with all Web technologies.
Framework for creating native applications with web technologies like JavaScript, HTML, and CSS. It takes care of the hard parts so you can focus on the core of your application.
Cross Platform Applications
IoT
JerryScript
JerryScript is the lightweight JavaScript engine intended to run on a very constrained devices such as microcontrollers.
JavaScript framework for robotics, physical computing, and the Internet of Things. It makes it incredibly easy to command robots and devices.
support for 43 different platforms.
If you take something, Take this:
- Javascript is simple and wildly adopted
- Use Javascript to automate all the things
Javascript, Javascript Everywhere
By Boaz Berman
Javascript, Javascript Everywhere
- 197