Intro to Python
Introduction
Text
Why Python?
Easy to Learn
-
Concise
-
Well-Documented
Well-Documented

796,949 Questions
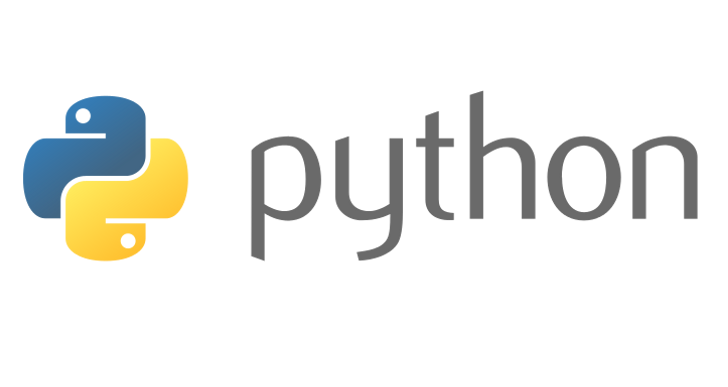
Concise
print('hello, world')
#include<iostream>
using namespace std;
int main()
{
cout << "Hello World\n";
return 0;
}
c++
python
Open Sourced
-
Free to Use
-
Numerous Libraries
Libraries
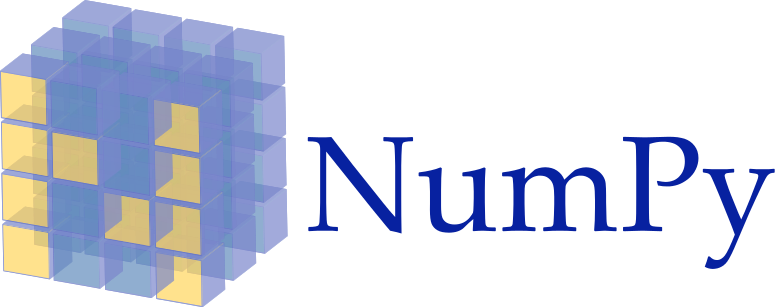
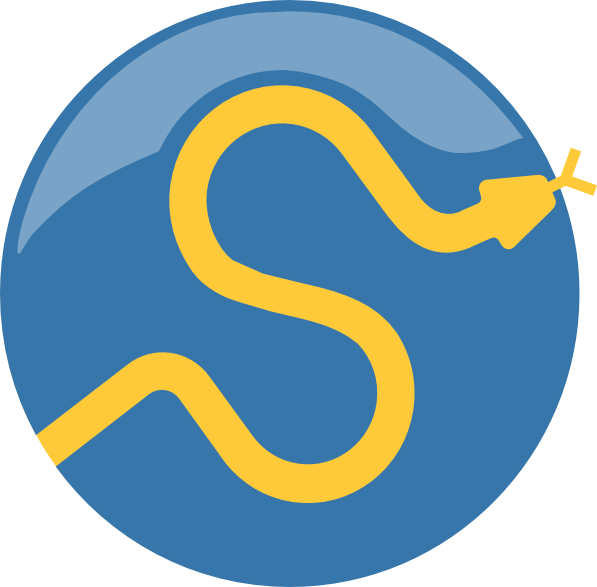


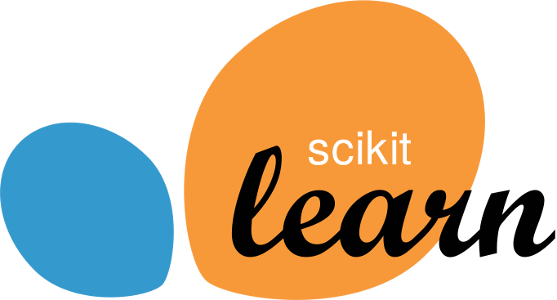


Syntax I
Function Basics
# Comments are preceded by the '#' symbol
# Comments are preceded by the '#' symbol
"""
Comments blocks that span multiple lines
can be denoted with a pair of three quotes
"""
# Comments are preceded by the '#' symbol
"""
Comments blocks that span multiple lines
can be denoted with a pair of three quotes
"""
'''
Either double or single quotes can be used
'''
# Functions are defined like so
# Functions are defined like so
# def FUNCTION_NAME( PARAMETERS ):
# Functions are defined like so
# def FUNCTION_NAME( PARAMETERS ):
# In good practice, a comment or comment block
# precedes a function, describing its purpose
def myFirstFunction( a, b ):
# Functions are defined like so
# def FUNCTION_NAME( PARAMETERS ):
# In good practice, a comment or comment block
# precedes a function, describing its purpose
def myFirstFunction( a, b ):
# The code body that follows must be indented
a = a + 2
# Functions are defined like so
# def FUNCTION_NAME( PARAMETERS ):
# In good practice, a comment or comment block
# precedes a function, describing its purpose
def myFirstFunction( a, b ):
# The code body that follows must be indented
a = a + 2
# Since variables are implicitly declared,
# a type, like 'int', is not needed to instantiate 'c'
c = a + b
# Functions are defined like so
# def FUNCTION_NAME( PARAMETERS ):
# In good practice, a comment or comment block
# precedes a function, describing its purpose
def myFirstFunction( a, b ):
# The code body that follows must be indented
a = a + 2
# Since variables are implicitly declared,
# a type, like 'int', is not needed to instantiate 'c'
c = a + b
# In Python any layer of logical abstraction
# (e.g. class, function, conditional) is denoted
# by a colon and followed by further indentation
if c > 10:
c = c * 2
print(c)
Function Basics
Let's run our script and
see what happens...
def myFirstFunction( ):
print('Hello, World!')
Input
Output
What happened?
We need to call our function!
def myFirstFunction( ):
print('Hello, World!')
myFirstFunction()
Hello, World!
Success!
Function Basics
Like that of most languages, python's functions return a value
def mySecondFunction( ):
return 4
a = mySecondFunction()
print(a)
4
Input
Output
We can return other types too
By default, this value is None,
but we can specify our own value
def myThirdFunction( ):
return 'python is great!'
a = myThirdFunction()
print(a)
python is great!
Function Basics
In a script we typically define a main function we invoke to execute our other functions
def func1( ):
return 5
def func2( a, b ):
print(a + b)
11
Input
Output
def main( ):
foo = func1() # foo := 5
bar = 6
func2(foo, bar)
def func1( ):
return 5
def func2( a, b ):
print(a + b)
main()
Print Statements
Python makes print statements easy
>>> print(10)
>>> print(10)
10
>>> print(10)
10
>>> foo = 10
>>> print(foo)
>>> print(10)
10
>>> foo = 10
>>> print(foo)
10
Simple, right?
>>> print('cat')
>>> print('cat')
cat
>>> print('cat')
cat
>>> bar = 'cat'
>>> print(cat)
>>> print('cat')
cat
>>> bar = 'cat'
>>> print(cat)
cat
Print Statements
By default, print() will output a new line at the end
def main():
foo = 'dog'
bar = 'turtle'
print(foo)
print(bar)
print(bar)
main()
dog
turtle
turtle
Input
Output
We can suppress this using commas
def main():
foo = 'dog'
bar = 'turtle'
print(foo),
print(bar),
print(bar),
main()
dog turtle turtle
Strings
String concatenation is similar to that of other languages
>>> foo = 'cat'
>>> bar = 'dog'
>>> foo + bar
>>> foo = 'cat'
>>> bar = 'dog'
>>> foo + bar
'catdog'
String formatting is in C-style
>>> foo = 'cat'
>>> s1 = 'I have a %s at home.' % (foo)
>>> s1
>>> foo = 'cat'
>>> s1 = 'I have a %s at home.' % (foo)
>>> s1
'I have a cat at home.'
>>> foo = 'cat'
>>> s1 = 'I have a %s at home.' % (foo)
>>> s1
'I have a cat at home.'
>>> bar = 'dog'
>>> s2 = 'I have a %s and a %s at home.' % (foo, bar)
>>> s2
>>> foo = 'cat'
>>> s1 = 'I have a %s at home.' % (foo)
>>> s1
'I have a cat at home.'
>>> bar = 'dog'
>>> s2 = 'I have a %s and a %s at home.' % (foo, bar)
>>> s2
'I have a cat and a dog at home.'
We use %s since foo and bar are both strings, but we can substitute other types too
>>> foo = 20
>>> s1 = 'I am $d years old.' % (foo)
>>> s1
>>> foo = 20
>>> s1 = 'I am $d years old.' % (foo)
>>> s1
'I am 20 years old.'
>>> foo = 20
>>> s1 = 'I am $d years old.' % (foo)
>>> s1
'I am 20 years old.'
>>> bar = 'dogs'
>>> s2 = 'I have %d %s at home.' % (foo, bar)
>>> s2
>>> foo = 20
>>> s1 = 'I am $d years old.' % (foo)
>>> s1
'I am 20 years old.'
>>> bar = 'dogs'
>>> s2 = 'I have %d %s at home.' % (foo, bar)
>>> s2
'I have 20 dogs at home.'
There are several other formatting operators, but for this class, all we'll need to know are %s, %d and %f for
string, integer and float, respectively
A more comprehensive guide can be found here
Arithmetic
Python uses standard mathematical operators
+ - * /
Recognize them?
Sure hope so!
Additionally, Python uses %
for modulus division
and **
for exponents
Let's look at some examples of usage
>>> 3 % 2
>>> 3 % 2
1
>>> 3 % 2
1
>>> 5 % 5
>>> 3 % 2
1
>>> 5 % 5
0
>>> 3 % 2
1
>>> 5 % 5
0
>>> 17 % 3
>>> 3 % 2
1
>>> 5 % 5
0
>>> 17 % 3
2
>>> 3 % 2
1
>>> 5 % 5
0
>>> 17 % 3
2
>>> 4 % 6
>>> 3 % 2
1
>>> 5 % 5
0
>>> 17 % 3
2
>>> 4 % 6
4
>>> 3 ** 2
>>> 3 ** 2
9
>>> 3 ** 2
9
>>> 4 ** 3
>>> 3 ** 2
9
>>> 4 ** 3
64
>>> 3 ** 2
9
>>> 4 ** 3
64
>>> 2 ** 1.4
>>> 3 ** 2
9
>>> 4 ** 3
64
>>> 2 ** 1.4
2.6390158215457884
It's okay if you didn't get
that last one
Syntax II
Conditionals
# A basic conditional is structured like so..
Like c++, python has
conditionals and loops
# A basic conditional is structured like so..
"""
if CONDITION:
STATEMENT
"""
# A basic conditional is structured like so..
"""
if CONDITION:
STATEMENT
"""
# Example 1
# This statement will execute since True is always True
if True:
print('Hello, World!')
# A basic conditional is structured like so..
"""
if CONDITION:
STATEMENT
"""
# Example 1
# This statement will execute since True is always True
if True:
print('Hello, World!')
# Example 2
# This statement will not execute since (5 < 4) is False
if 5 < 4:
print('Hello, World!')
# We can also append if-else and else conditions like so..
"""
"""
# We can also append if-else and else conditions like so..
"""
if CONDITION_1:
STATMENT_1
"""
# We can also append if-else and else conditions like so..
"""
if CONDITION_1:
STATMENT_1
elif CONDITION_2:
STATEMENT_2
"""
# We can also append if-else and else conditions like so..
"""
if CONDITION_1:
STATMENT_1
elif CONDITION_2:
STATEMENT_2
elif CONDITION_3:
STATEMENT_3
"""
# We can also append if-else and else conditions like so..
"""
if CONDITION_1:
STATMENT_1
elif CONDITION_2:
STATEMENT_2
elif CONDITION_3:
STATEMENT_3
else:
STATEMENT_4
"""
Conditionals
Statement 3 Executed
a = 2
b = 4
input = 5
Input
Output
Examples
Conditions 1 and 2 are False so statement 3 executes
Statement 1 Executed
Statement 1 is the first True statement so it, alone, executes
a = 2
b = 4
input = 5
if input < a:
print('Statement 1 Executed')
elif input < b:
print('Statement 2 Executed')
else:
print('Statement 3 Executed')
a = 6
b = 99
input = 5
if input < a:
print('Statement 1 Executed')
elif input < b:
print('Statement 2 Executed')
else:
print('Statement 3 Executed')
Guess the output!
Conditionals
Same old comparison operators
== != < <= > >=
And some alternatives too!
Alternative
is
not
<>
and
or
Original
==
!
!=
&&
||
if True || False:
print('Lets go Terps!')
if !(True && False):
print('I am a Flat-Earther')
Original
Alternatively..
if True or False:
print('I like toitles')
if not (True and False):
print('I am ignorance')
if a != b:
print('I am a business major!')
How about this one?
These statements are logically equivalent
# first possibility
if a is not b:
print('I am a business major!')
# first possibility
if a is not b:
print('I am a business major!')
# second possibility
if a <> b:
print('I am a business major!')
There's a slight discrepancy between the two
We'll go over that in a bit!
Try and guess the alternatives!
Conditionals
John is a winner.
a = 10
b = 7
john = 'winner' if a > b else 'loser'
print('Josh is a %s.' % john)
Input
Output
Conditionals can also be used for assignment
If 'a' was less than 'b' we would have assigned the latter to 'john'
Good thing 'a' was greater!
# example 1
if a > b:
john = 'winner'
else:
john = 'loser'
# example 2
john = 'winner' if a > b else 'loser'
The two examples to the left are logically equivalent
In-line boolean assignments can be used to 'clean up' our code and make it more readable
Loops
While-loops have a similar structure to if-statements
# Structure a while-loop like so..
# Structure a while-loop like so..
# Structure a while-loop like so..
"""
while CONDITION:
STATEMENT
"""
# Structure a while-loop like so..
"""
while CONDITION:
STATEMENT
"""
# Example 1
# Structure a while-loop like so..
"""
while CONDITION:
STATEMENT
"""
# Example 1
# This loop will execute infinitely since True is always True
while True:
print('Hello, World!')
# Structure a while-loop like so..
"""
while CONDITION:
STATEMENT
"""
# Example 1
# This loop will execute infinitely since True is always True
while True:
print('Hello, World!')
# Example 2
# Structure a while-loop like so..
"""
while CONDITION:
STATEMENT
"""
# Example 1
# This loop will execute infinitely since True is always True
while True:
print('Hello, World!')
# Example 2
# This loop will execute twice
count = 0
while count < 2:
count += 1
# We can also use break statements to exit a loop prematurely
# We can also use break statements to exit a loop prematurely
# Example 3
# We can also use break statements to exit a loop prematurely
# Example 3
# This loop will terminate before printing anything
while True:
break
print('Hello, World!')
# We can also use break statements to exit a loop prematurely
# Example 3
# This loop will terminate before printing anything
while True:
break
print('Hello, World!')
# Example 4
# We can also use break statements to exit a loop prematurely
# Example 3
# This loop will terminate before printing anything
while True:
break
print('Hello, World!')
# Example 4
# How about this one?
count = 0
while count < 3:
print('Hello, World!')
if count == 1:
break
count += 1
# We can also use break statements to exit a loop prematurely
# Example 3
# This loop will terminate before printing anything
while True:
break
print('Hello, World!')
# Example 4
# How about this one?
count = 0
while count < 3:
print('Hello, World!')
if count == 1:
break
count += 1
# This one will print 'Hello, World!' twice
# We can also use break statements to exit a loop prematurely
# Example 3
# This loop will terminate before printing anything
while True:
break
print('Hello, World!')
# Example 4
# How about this one?
count = 0
while count < 3:
print('Hello, World!')
if count == 1:
break
count += 1
# This one will print 'Hello, World!' twice
# Can you see why?
Loops
For-loops are another type of loop
Typically, they are preferred when the number of iterations is known beforehand
# Structure a for-loop like so..
# Structure a for-loop like so..
"""
for VARIABLE in SPAN:
STATEMENT
"""
# Structure a for-loop like so..
"""
for VARIABLE in SPAN:
STATEMENT
"""
# The span can be a range of numbers
for i in range(4):
print(i)
# Structure a for-loop like so..
"""
for VARIABLE in SPAN:
STATEMENT
"""
# The span can be a range of numbers
for i in range(4):
print(i)
# or a predefined list
arr = [1, 4, 6]
for elem in arr:
print(elem)
# Structure a for-loop like so..
"""
for VARIABLE in SPAN:
STATEMENT
"""
# The span can be a range of numbers
for i in range(4):
print(i)
# or a predefined list
arr = [1, 4, 6]
for elem in arr:
print(elem)
# Don't worry about outputs. We'll cover that in a minute
# The span doesn't have to be comprised of numbers
# The span doesn't have to be comprised of numbers
# We can even loop over a list of strings
students = ['Alice', 'Bob', 'Charlie']
for name in students:
print(name)
# The span doesn't have to be comprised of numbers
# We can even loop over a list of strings
students = ['Alice', 'Bob', 'Charlie']
for name in students:
print(name)
# or a list of booleans
bools = [True, False, True, True]
for val in bools:
if val:
...
# The span doesn't have to be comprised of numbers
# We can even loop over a list of strings
students = ['Alice', 'Bob', 'Charlie']
for name in students:
print(name)
# or a list of booleans
bools = [True, False, True, True]
for val in bools:
if val:
...
# This allows to effectively represent complex logic
count = 0
for p in points:
if p > 100:
count += 1
print('%d players scored over 100 points!' % count)
Loops
Examples
students = ['Alice', 'Bob', 'Charlie']
for name in students:
print(name)
Input
Output
The for-loop iterates through our students list and stores the value of each element to name
Alice
Bob
Charlie
On each successive pass it prints the current value of name
bools = [True, False, True, True]
for val in bools:
if val:
print(val)
True
True
True
Here, only True elements activate the if-statement
points = [156, 97, 103, 245, 53]
count = 0
for p in points:
if p > 100:
count += 1
print(('%d players scored over 100'
' points!') % count)
3 players scored over 100 points!
We start with count := 0 and increment by 1 each time we see an element of points greater than 100
Note that the print statement lies outside the for-loop
Modules
Module (n) /ˈmäjool/
each of a set of standardized parts or independent units that can be used to construct a more complex structure

Importing
We need to import modules before using them
# It's best practice to import them at the top of
# your script
import math
# It's best practice to import them at the top of
# your script
import math
# Once declared, module functions can be used like so
# MODULE.FUNCTION(PARAMETERS)
>>> import math
>>> a = math.floor(4.5)
>>> a
4.0
Easy, right?
# Multiple modules can be called in-line
# Delimit them with commas
import math, random, csv
# Multiple modules can be called in-line
# Delimit them with commas
import math, random, csv
# Module names can also be aliased, like so,
# for improved readability
>>> import math as m
>>> a = m.floor(4.5)
>>> a
4.0
All the modules of Python's Standard Library are readily available with your Python installation
You can find a list of those modules here
Modules that are not a part of the Standard Library can be installed through the terminal using pip
References
Lec 1 - Intro to Python
By Brian J Lee
Lec 1 - Intro to Python
- 673