

Carlos P. Jimeno
jimenocontact@gmail.com
Jimeno0
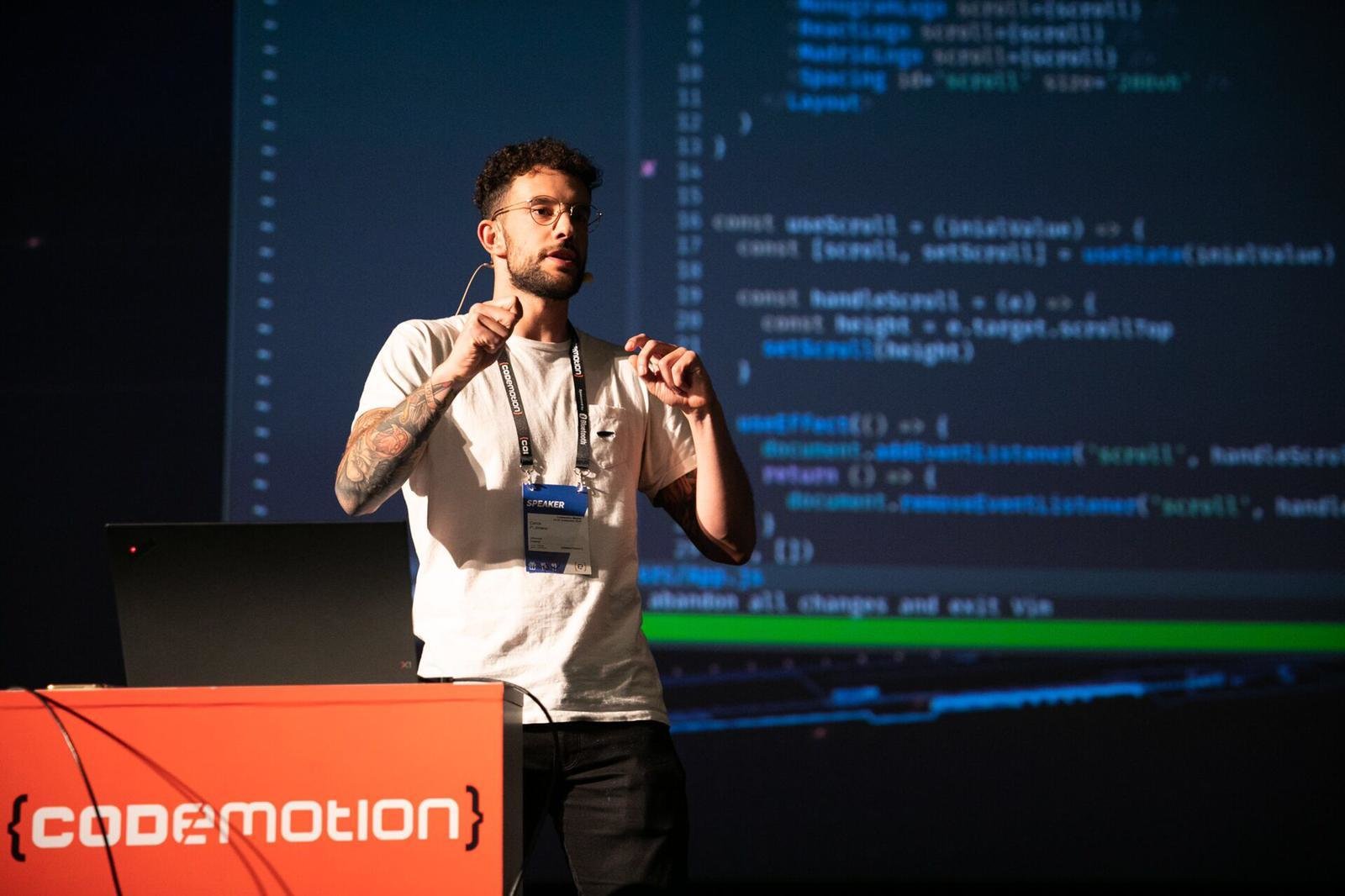
Carlos P. Jimeno


@Jimeno0

Module 2.
React
@Jimeno0

Intro
- Intro to SPA
- What is React
- Intro to JSX
- Crafting a new project
- dev tools
@Jimeno0


@Jimeno0

What is React
- JavaScript library created by Facebook
- React is a User Interface (UI) library
- React is a tool for building UI components
@Jimeno0

<!DOCTYPE html>
<html lang="en">
<title>Test React</title>
<!-- Load React API -->
<script src= "https://unpkg.com/react@16/umd/react.production.min.js"></script>
<!-- Load React DOM-->
<script src= "https://unpkg.com/react-dom@16/umd/react-dom.production.min.js"></script>
<!-- Load Babel Compiler -->
<script src="https://unpkg.com/babel-standalone@6.15.0/babel.min.js"></script>
<body>
<div id="id"/>
<script type="text/babel">
ReactDOM.render(
<h1>Hello React!</h1>,
document.getElementById('id')
)
</script>
</body>
</html>
@Jimeno0

Basic concepts
Capitalized JSX for components
props === attrs
ClassName as class
@Jimeno0

What is JSX
XML/HTML-like syntax used by React that extends ECMAScript
@Jimeno0

Starting a new project
$ npx create-react-app my-app
$ cd my-app
$ npm start
@Jimeno0

React Developer Tools
@Jimeno0

Our cleaned project
Start with the boilerplate project
@Jimeno0

Practice MUI
@Jimeno0

Practice MUI

@Jimeno0

Functional vs Class Components
Presentation vs Logic
F.K.A
@Jimeno0

Component State
stateDemo
- Convert our toggle component to a class component
- Manage state in a class component
- organize our code
@Jimeno0

Component Lifecycle

@Jimeno0

Component Lifecycle
lifecycleDemo
- Convert our App component to a Class component
- create a scroll state
- add a listener on scroll that update the scroll variable
- remove on unmount
@Jimeno0

React hooks
The new patterns
What is a hook
Funcions "hook into" state & lifecycle from function components
@Jimeno0


@Jimeno0

State in functions
💥
@Jimeno0


@Jimeno0

Why
Scaffolding
Complex logic mixed in components
Reusable code
Class components misunderstandings
❌
♻
🍱
🤓
@Jimeno0

Rules
Declare in the top level
❌
Dont use in Class Components
❌
@Jimeno0

State management
(With clean code)
useState()

@Jimeno0

State management
@Jimeno0

Try to refactor the toogle component
Life cycle management
useEffect()

@Jimeno0

Life cycle management
@Jimeno0

Refactor of our parallax web
Movies practice
@Jimeno0


Movies practice
@Jimeno0

1. Create a movies fakeAPI (bonus: use express)
2. render all movies
3. Add a filters Drawer in order to filter movies
Fake API
@Jimeno0

json-server
db.json
Fake server with:
Axios
APIs integration
@Jimeno0

json-server
axios
integrate on useEffect
@Jimeno0

- Lists
- Keys
- Conditional rendering
Forms
@Jimeno0

Working with inputs
Exercise: filter by year
Sharing
and
lifting state up
@Jimeno0

Abstract our exercise to stand alone components
@Jimeno0

The Context API
@Jimeno0

export const ThemeContext = createContext({ value: {} })
const ThemeProvider = (props) => {
const [value, setValue] = useState('dark')
return (
<ThemeContext.Provider
value={{ value, setValue }}
>
{props.children}
</ThemeContext.Provider>
)
}
Create the context
The Context API
@Jimeno0

<ThemeProvider>
<App />
</ThemeProvider>
provider
App context
useContext()

@Jimeno0

App context
@Jimeno0

Use context in our App
Styling components
@Jimeno0

inLine
classNames
CSS in JS
styled-components
@Jimeno0

<💅>
styled-components
@Jimeno0

const Button = styled.button`
background: transparent;
border-radius: 3px;
border: 2px solid palevioletred;
color: palevioletred;
margin: 0 1em;
padding: 0.25em 1em;
`
styled-components
@Jimeno0

Refactor our webApp to styled components
- Start with styled-components
- Pass props down
- Exended styles props
Routing
@Jimeno0

React router
react-router-dom
Routing
@Jimeno0

Routes, Links and Consumers
Routing
@Jimeno0

<BrowserRouter>
...
<li>
<Link to="/">Home</Link>
</li>
<li>
<Link to="/about">About</Link>
</li>
...
</BrowserRouter>
Routing
@Jimeno0

<Switch>
<Route path="/about">
<About />
</Route>
<Route path="/users">
<Users />
</Route>
<Route path="/">
<Home />
</Route>
</Switch>
Deploy our App
@Jimeno0

heroku
Deploy our App
@Jimeno0

1. Login to heroku
2. Connect our app with Github
3. build & start scripts
4. serve package
Devacademy fullstack react
By Carlos Pérez Jimeno
Devacademy fullstack react
- 689