Programming
trick shots
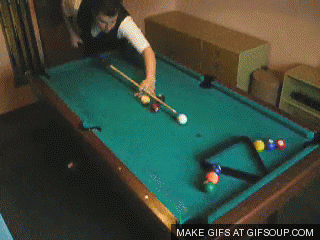
Trick shot
- Fun
- Impressive
- Pointless
-
Quines
-
Bit twiddling
-
Golf
-
Polyglots
-
IOCCC
-
Polyglot palindrome quine
Quines


Willard Van Orman Quine
(source: Wikipedia)

Let's write a quine

Bit twiddling
Counting the 1s in a 32-bit word
00000001000010100110100001001001
(17,459,273)
Counting the 1s in a 32-bit word
Naive implementation
int popCount(int x) {
int ones = 0;
for (int i = 0; i < 32; i++) {
if (x & 1) ones += 1;
x = x >> 1;
}
return ones;
}
13 x 32 = 416 x86 instructions (on average)
lots of branch misses
Counting the 1s in a 32-bit word
Optimal (?) implementation
int popCount(unsigned int x) {
x = x - ((x >> 1) & 0x55555555);
x = (x & 0x33333333) + ((x >> 2) & 0x33333333);
x = (x + (x >> 4)) & 0x0F0F0F0F;
x = x + (x >> 8);
x = x + (x >> 16);
return x & 0x0000003F;
}
33 x86 instructions
no branching
(source: Beautiful Code, ch. 10)


Code golf
Print all the prime numbers < 1000
# Sieve of Eratosthenes.
# Prints all the prime numbers between 1 to n.
def eratosthenes(n):
multiples = set()
for i in range(2, n+1):
if i not in multiples:
print (i)
for j in range(i*i, n+1, i):
multiples.add(j)
eratosthenes(1000)
290 bytes
m,n,r=set(),1001,range
for i in r(2,n):
if{i}-m:
print i
for j in r(i*i,n,i):m.add(j)
Print all the prime numbers < 1000
89 bytes
Polyglots
IOCCC
#define/**/Alan/**/(fflush(0),j=c=0;++c<b[6]+7;v=b[c]^(b[c+
char*H="close\r\nContent-Type:text/html\r\n\r\n<canvas id=\
'c'width='128'height='128'style='width:256px;height:256px'\
><script>x=y=-1;v=window.c;v.onmousemove=function(e){x=e.p\
ageX-v.offsetLeft;y=e. pageY-v.offsetTop};w=\
new WebSocket('ws'+ location.href.subs\
tr(4));w.binary" "Type='arraybuff\
er';w.onmess" "age=function(\
e){c=v.getC" "ontext('2d')\
;b=c.crea" "teI" "mageData(12\
8,128);u= new Uint8Array(d=e. data);b.dat\
a.set(u) ;c.putImageData(b,0,0) ;u[0]=x/2;\
u[1]=y/2 ;w.send(d.slice(0,x<0?0: 2));x=y=-\
1}</scr" "ipt>",b[9999];float*e,d[ 65536],u,v
,lu,lv, z;void*f;typedef unsigned long long
l;l*p,t[ 99]={0x67452301,0xEFCDAB89, 0x98BADCFE
,308438* 881,3285377520 },i,j,k ,n,m[204];
#include /*io*/ <stdio.h> /*turing*/
void s(){ ; for( k =0;k++<7 * 2 *9;)for(i=
0;++i<127; ) e=d + i* 4 +512*k,1[e] +=(i-u)*(i
-u)+(k-v)* (k- v )<20?*e+=UV_DROP:0;}l*q= m, g=1LL<<32;
l(h)(l v,l (a)){ return(v<<a)|(v%g>>(32-a ) );}int main
(int c/*ha r*/, char**y){for(e=d;fgets(b ,m[97]=480,\
stdin)&&*b >31; )for( i=j=0;n=b[i],i<18 ? (l)"sec-webs\
ocket-key: "[i] ==(n|32):n-32? m[j/4] |=(j< 24?n:(l)
"258EAFA5-" "E914-47DA-95CA-C5AB0DC85B" "11\x80"[j-24])
<<(3-j%4)*8 ,++j<61:1;)i++;for(j=3;--j; ){for(i=4;++i<74
;)i>9?p=q+i- 2 ,p[8]=h(p[5 ]^*p^p[-6]^p[-8]
#define/*hah aha <--mouth*/ laplacian(u)l##u
,1):(t[i]=t[9 -i] );for(p=t+7 ,i=-1;++i<82;k
=*p++,p[2]=h(p[1],5) +p[-3]+n+ (i/+ 20%2?*p^k^p[-
2]:(*p&k)|((i< 20?~*p:*p|k)&p [-2 ]))+*q++,t[+
81-i]+=(i>76)* (* p= h(* p,30*(i<80)
)))for(;n-k;n=((( 1LL +(9<<i/20
)/8)<<60)/k+k)/2) k=n ;} for (i =0;i<57;
i++)i<30?q[i%5* 16/ 3+ i/5] |=t[i%5]%g<<4
>>(30+i%5%3*2-i/5 *6)& 63,0 :(n =*q++,b[i-30]=
n<62?n-37+"fl!" [n /26]:n *4- 205);for(prin\
tf("HTTP/1.1 "/* | */"%d " "OK\r\nConnecti\
on:%s%.27s=\r\n\r\n" ,101 +99*! *m,!*m?H:"upgrade\
\r\nUpgrade:webso" "c" "ket\r\nSec-WebSocket-Acce\
pt:",b);e-d<65536 ; e+= 4)e[1]=(*e=UV_BACKGROUND);for
(;*m;c=0){for(f= c< 2 ?0:fopen(y[1],"r");f&&fscanf(f,
"%f,%f,",&u,&v)>0;)s ();for(;j<SPEED*4;j+=2)for(i=0;i<
63504;e+=5-j%4*2,z= TIMESTEP,*e=u+z*(elta_u),e[1]=v+z*
(elta_v),i+=4)for(c= 0;c+2;u=v,v=e[4],lu=lv,lv=e[-508]+*e+
e[8]+e[516]-v*4)e=d+i+512+i/504*8+j%4-c--;for(;n=c%4,65544>
c;c++)putchar(c>7?n>2||(u=d[c-n-7]*4*(n<2?!n?RGB))>255?255:
#define/*x*/Turing(x)4]=getchar()&127))u=v;if(b[6]>1)s();}}
u<0?0:u:c<0?124-c*3:c==5);/* -- The word "genius" is */ for
Alan Turing (1912--1954)
palipolyquine
$_='$z=0?"$&".next: eval("printf=console.log;atob`JCc`");printf("%s_=%s%s%s;eval(%s_);//#//;)_%s(lave;%s%s%s=_%s",$d=$z[0]||h^L,$q=$z[1]||h^O,$_,$q,$d,$d,$q,"0"?$_.split("").reverse().join(""):~~reverse,$q,$d)';eval($_);//#//;)_$(lave;')d$,q$,esrever~~:)""(nioj.)(esrever.)""(tilps._$?"0",q$,d$,d$,q$,_$,O^h||]1[z$=q$,L^h||]0[z$=d$,"s%_=s%s%s%;eval(s%_);//#//;)_s%(lave;s%s%s%=_s%"(ftnirp;)"`cCJ`bota;gol.elosnoc=ftnirp"(lave :txen."&$"?0=z$'=_$
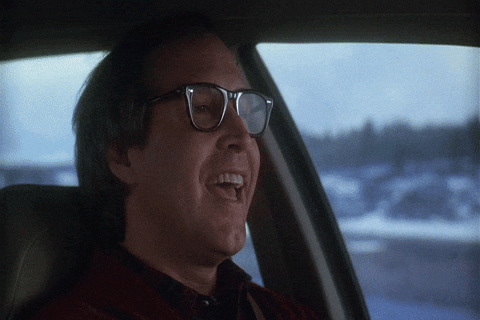
Rabbit holes
Code for this talk:
Programming trick shots
By Chris Birchall
Programming trick shots
- 2,205