Title Text

Hello 👋
Ramona Bîscoveanu

👩🏼💻 Senior Developer @ SAP
@CodesOfRa
📍Côte d'Azur
🧀 🍷🌱
🥳 Vue 3 🥳

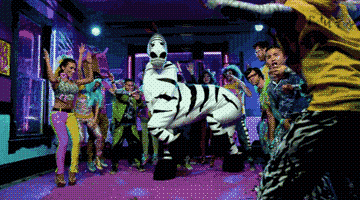
TypeScript
TypeScript
Vue was re-written in TypeScript → better TypeScript support
✨Reactivity ✨
Vue 2 caveats
- adding or removing an object property
- setting a new array item
- modyfing the length of the array
Setting a new array item
//Vue 2
Vue.set(this.items, indexOfItem, newValue);
Setting a new array item
//Vue 3
this.items[indexOfItem] = newValue
Adding a new object property
//Vue 2
Vue.set(this.someObject, 'color', 'red')
Adding a new object property
//Vue 3
this.someObject.color = "red"
Style Variables
final comments stage
experimental
<template>
<div>
<input v-model="color">
<span class="text">hello</span>
</div>
</template>
<script>
export default {
data() {
return {
color: 'red',
};
},
};
</script>
<style vars="{ color }">
.text {
color: var(--color);
font-weight: bold;
}
</style>
<template>
<div>
<input v-model="color">
<span class="text">hello</span>
</div>
</template>
<script>
export default {
data() {
return {
color: 'red',
};
},
};
</script>
<style vars="{ color }">
.text {
color: var(--color);
font-weight: bold;
}
</style>

<style scoped vars="{ color }">
.text {
color: var(--color);
}
</style>

<style scoped>
<template>
<div class="main">
<input v-model="color" />
<span class="text">hello</span>
</div>
</template>
<script>
export default {
data() {
return {
color: "red",
font: {
size: "2em",
},
};
},
};
</script>
<style>
.text {
color: v-bind(color);
font-size: v-bind("font.size");
}
</style>
<template>
<div class="main">
<input v-model="color" />
<span class="text">hello</span>
</div>
</template>
<script>
export default {
data() {
return {
color: "red",
font: {
size: "2em",
},
};
},
};
</script>
<style>
.text {
color: v-bind(color);
font-size: v-bind("font.size");
}
</style>
Fragments
<template>
<div>
my very awesome element
</div>
</template>
<template>
<div>
<div>
my very awesome element
</div>
<div>
my very awesome second element
</div>
</div>
</template>
<template>
<div>
<div>
my very awesome element
</div>
<div>
my very awesome second element
</div>
</div>
</template>
<template>
<div>
my very awesome element
</div>
<div>
my very awesome second element
</div>
</template>
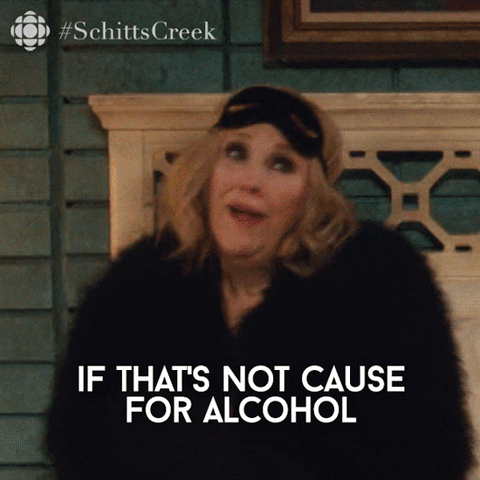
Teleport
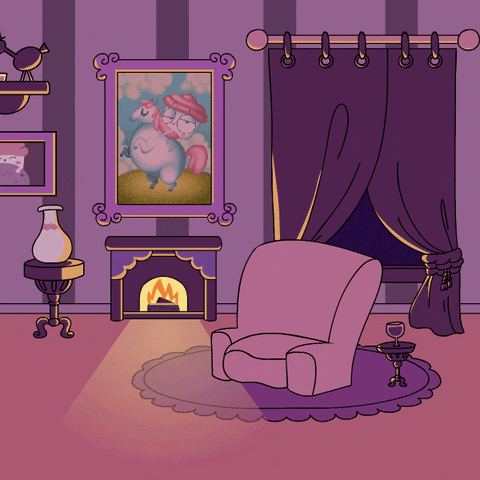
Use Cases
- Modals
- Notifications
- Dropdown
<teleport to="body">
<div v-if="modalOpen" class="modal">
<div>
Hello from the teleported modal!
<button @click="modalOpen = false">Close</button>
</div>
</div>
</teleport>
Suspense 😲
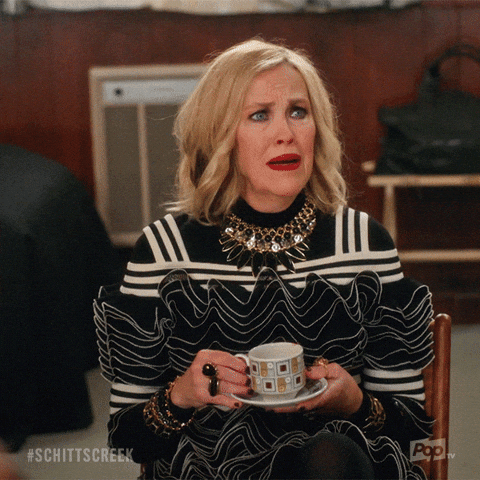
<Suspense>
<template #default>
...
</template>
<template #fallback>
...
</template>
</Suspense>
<template>
<Suspense>
<template #default>
<MyAsyncComponent />
</template>
<template #fallback>
<loader color="blue" />
</template>
</Suspense>
</template>
<script>
import { defineAsyncComponent } from "vue";
import Loader from "@/components/Loader.vue";
const MyAsyncComponent = defineAsyncComponent(() =>
import("@/components/MyAsyncComponent")
);
export default {
components: {
MyAsyncComponent,
Loader,
},
};
</script>
Composition API
Filters 🛑
Removed
v-model
v-model
Multiple v-model bindings on the same component are possible now
<MyComponent
v-model:name="myName"
v-model:location="myLocation"
></MyComponent>
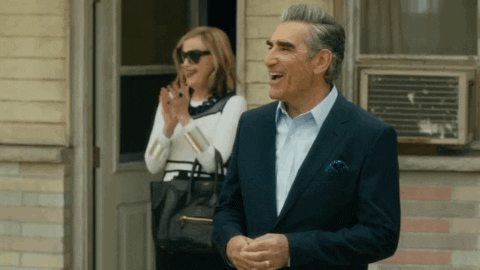
Vue Docs team
Thank you!
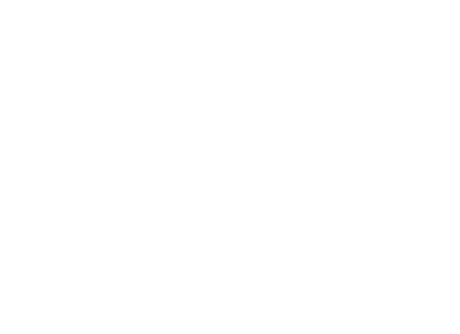
Exciting features in Vue 3
By Ramona Biscoveanu
Exciting features in Vue 3
Vue.js fwdays 2020
- 1,086