UI5 Web Components
& Vue
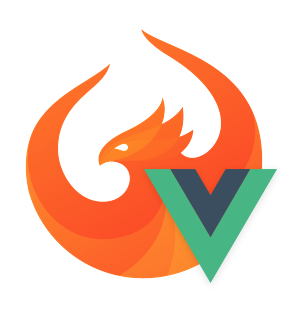
Hello ๐
Ramona Bรฎscoveanu
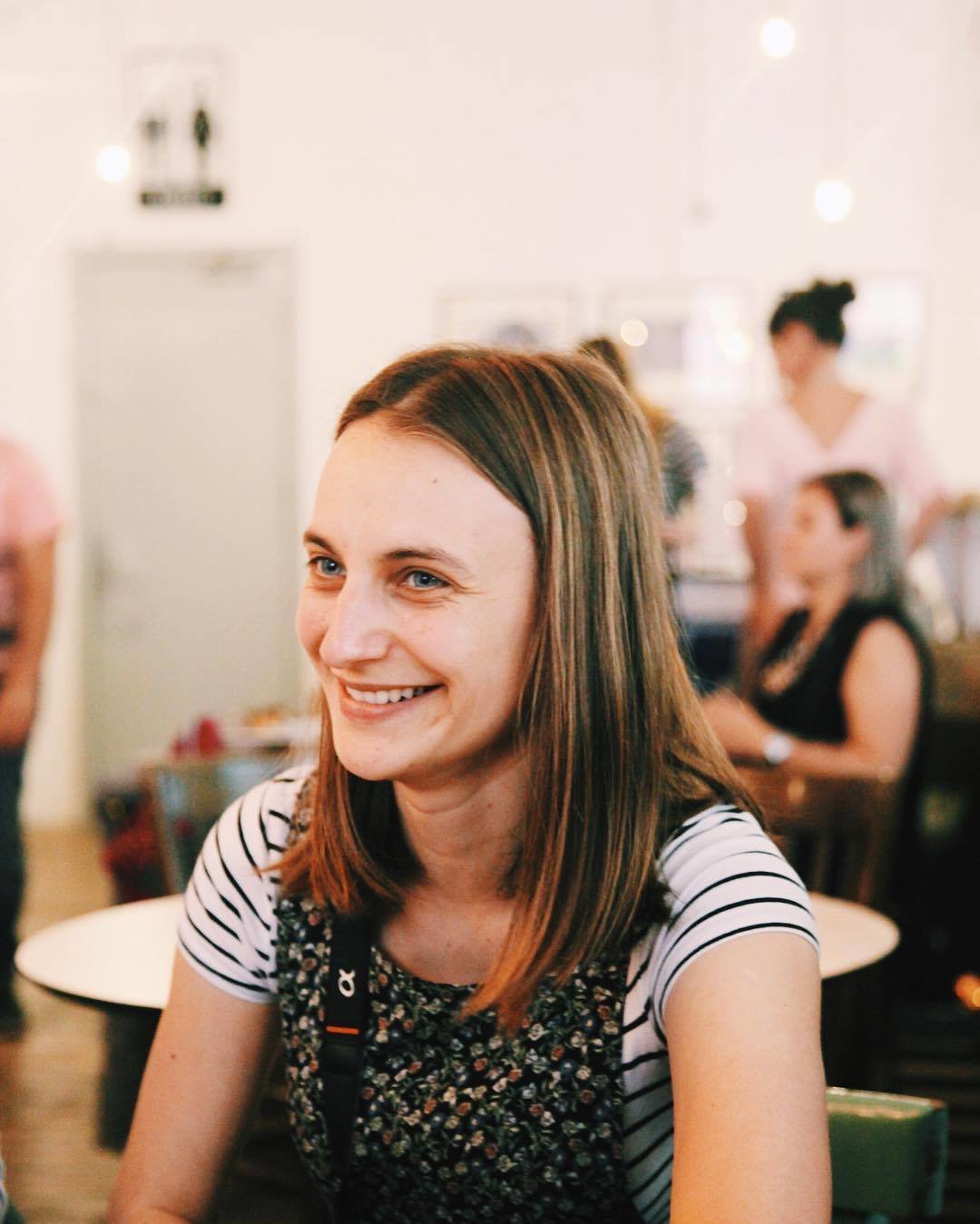
About
- Web Components
- UI5 Web Components
- Vue
Web components main technologies
- Custom Elements
- Shadow DOM
- HTML Templates
- HTML imports
1.Custom Elements
class RaButton extends HTMLElement {
connectedCallback() {
this.innerHTML = `<button>Hello world</button>`;
}
}
customElements.define("ra-button", RaButton);
<ra-button></ra-button>
class RaButton extends HTMLElement {
connectedCallback() {
this.innerHTML = `<button>Hello world</button>`;
}
}
customElements.define("ra-button", RaButton);
<ra-button></ra-button>
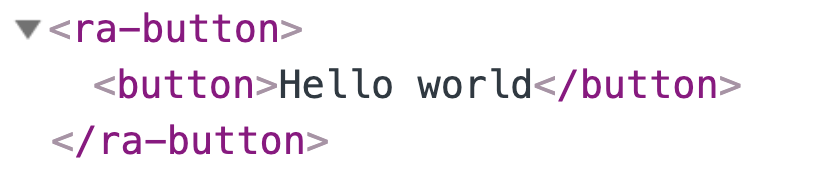
2.Shadow DOM
<button>Hello World!</button>
var host = document.querySelector("button");
var root = host.createShadowRoot();
root.textContent = "๐๐ฆ";
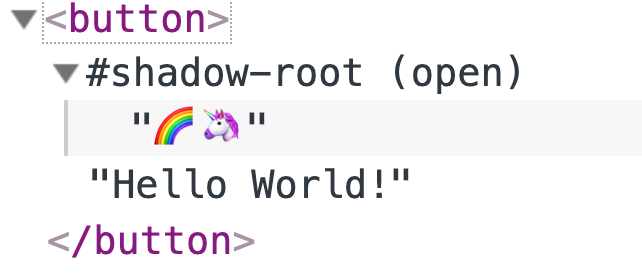
3. HTML Templates
<button id="add-word">Click me </button>
<div id="hello">Hello</div>
<template id="template-world">
<span>World</span>
</template>
document.getElementById("add-word").addEventListener("click",(e)=>{
var template = document.getElementById("template-world");
var hello = document.getElementById("hello");
// Create an instance of the template content
var instance = document.importNode(template.content, true);
// Append istance to hello element
hello.appendChild(instance)
})
4. HTML Imports
<link rel="import" href="my-component.html">
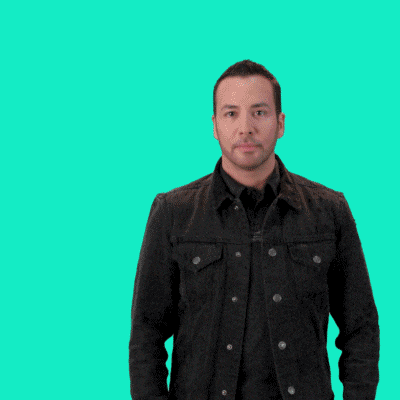
Why?
- Readability
- Browser Standards
- Reusability
- Developer Experience
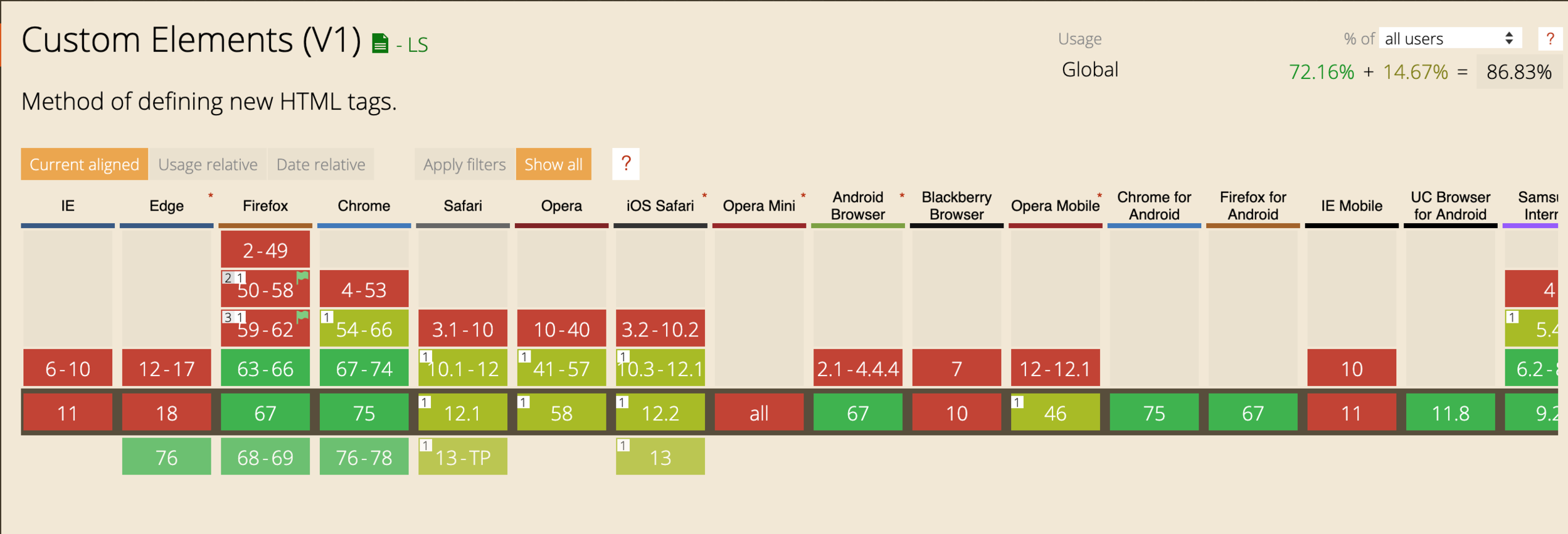
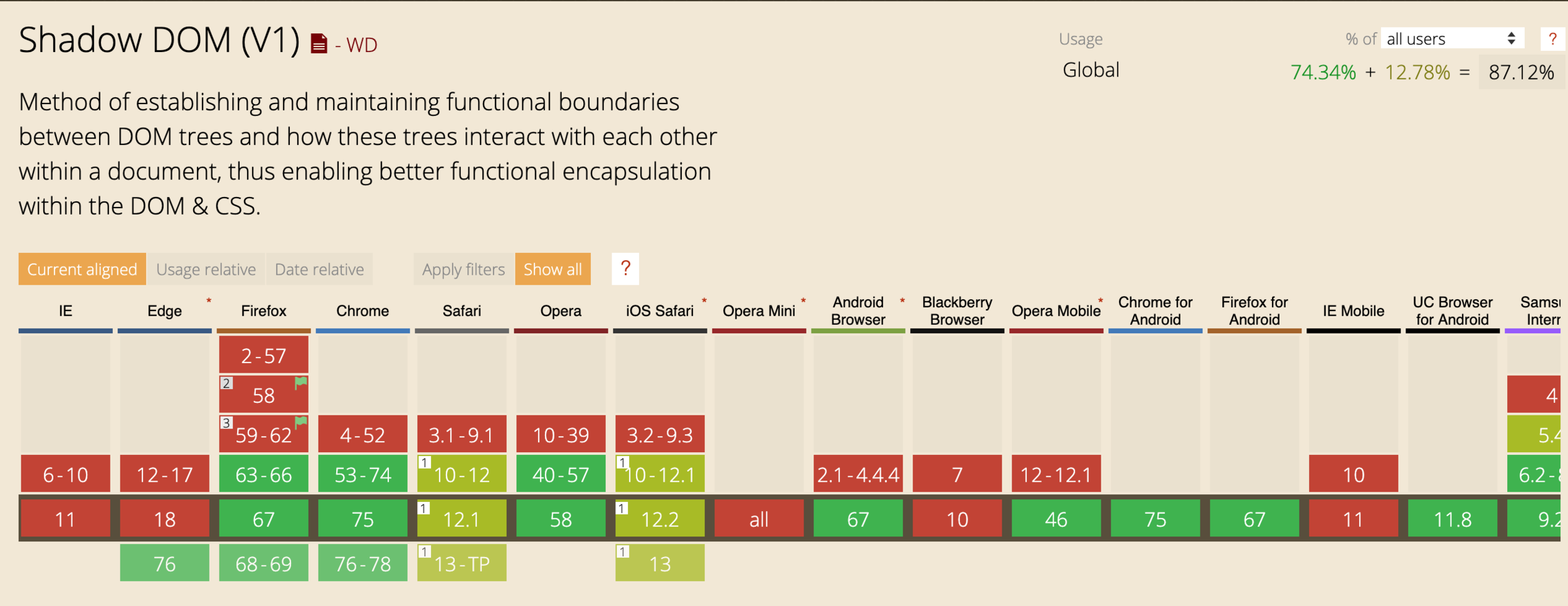
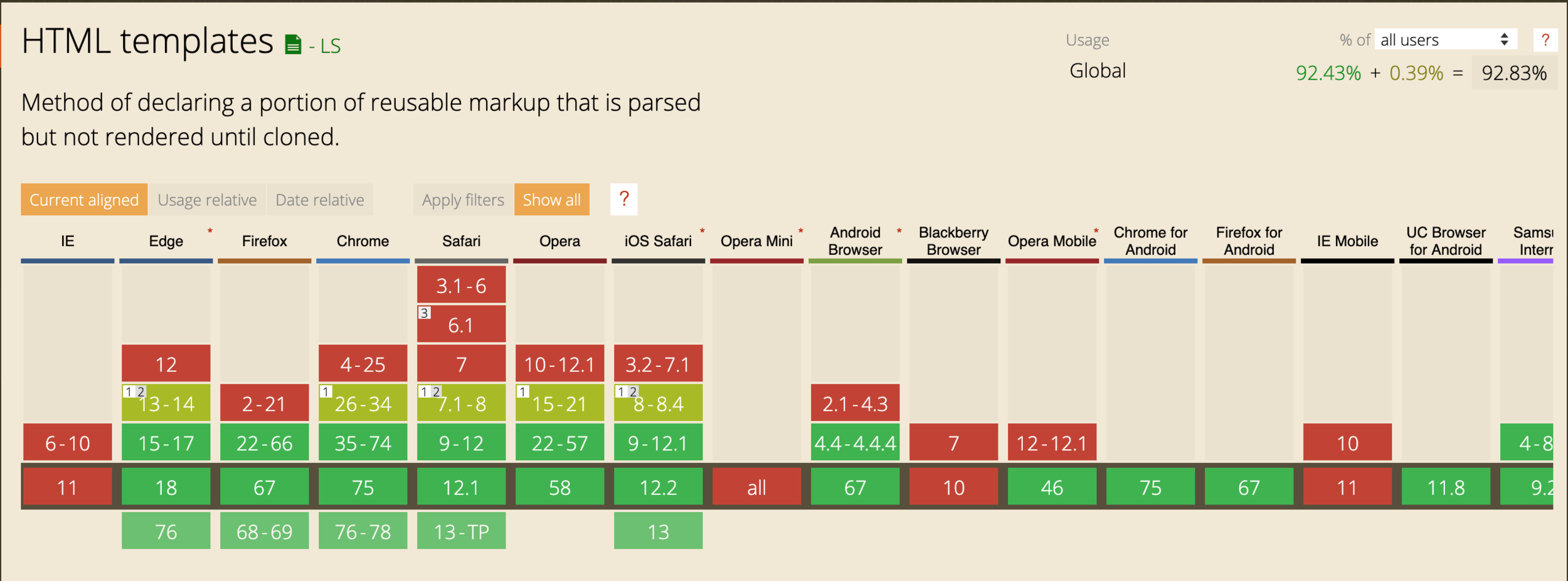
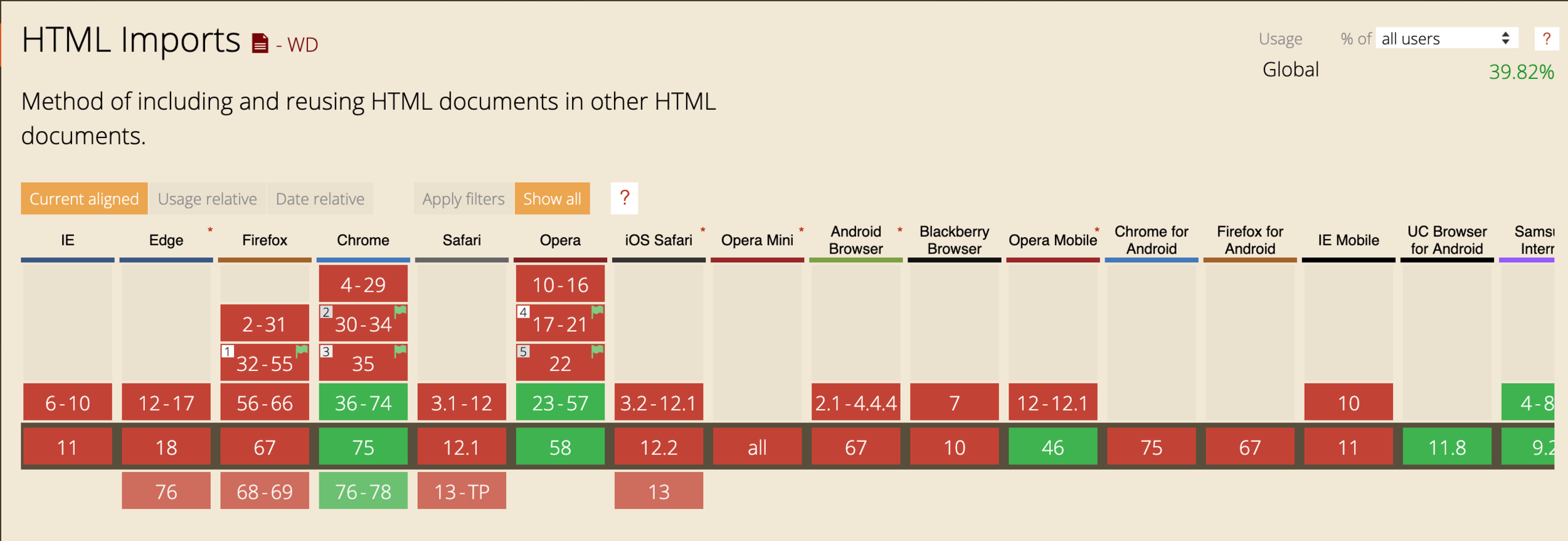
Polyfills to the rescue ๐ฅณ
More info& components
UI5 Web Components
-
Fiori 3.0 Design
-
Easy to use
-
A11y
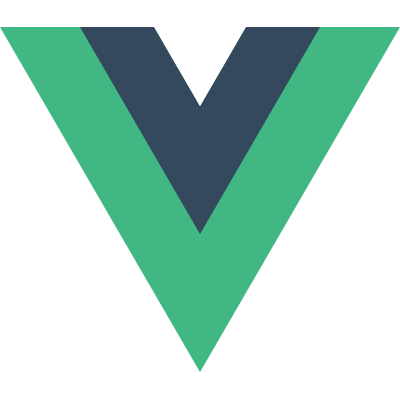
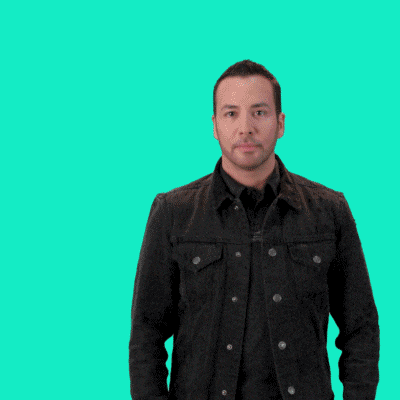
-
๐Magic reactivity๐
-
Low Complexity
-
Very Beginner Friendly
-
Great community
-
Vue-router
-
Vuex
-
Vue Test Utils
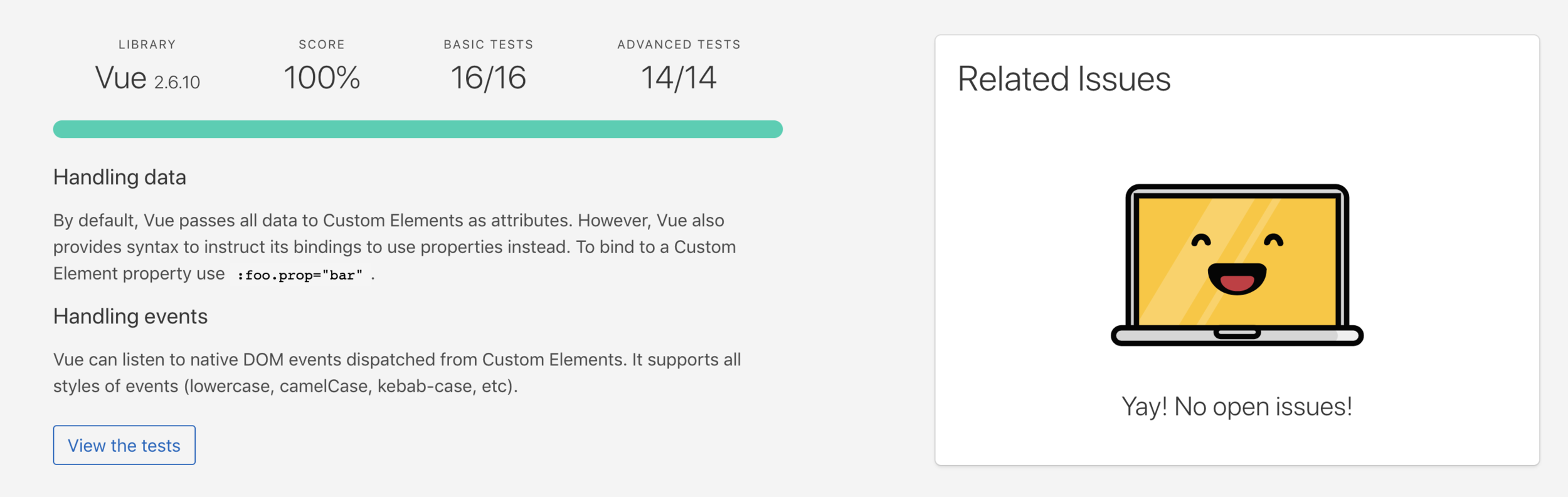
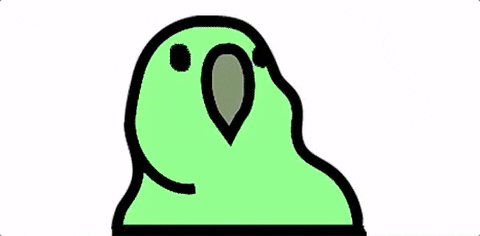
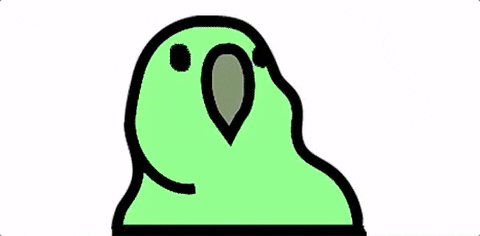
Quick intro
vue create my-vue-project
cd my-vue-project
npm run serve
Install Vue CLI
Single File Component
<template>
<div id="app">
<img alt="Vue logo" src="./assets/logo.png">
<HelloWorld msg="Welcome to Your Vue.js App"/>
</div>
</template>
<script>
import HelloWorld from './components/HelloWorld.vue'
export default {
name: 'app',
components: {
HelloWorld
}
}
</script>
<style>
#app {
font-family: 'Avenir', Helvetica, Arial, sans-serif;
-webkit-font-smoothing: antialiased;
-moz-osx-font-smoothing: grayscale;
text-align: center;
color: #2c3e50;
margin-top: 60px;
}
</style>
What about UI5 Web Components & Vue
//install UI5 Web Components
//npm install @ui5/webcomponents
//add this in main.js file
Vue.config.ignoredElements = [/^ui5-/];
vue-cli-plugin-ui5-webcomponents
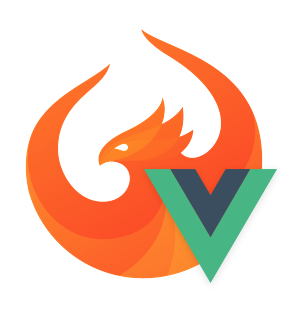
๐
๐
๐
๐
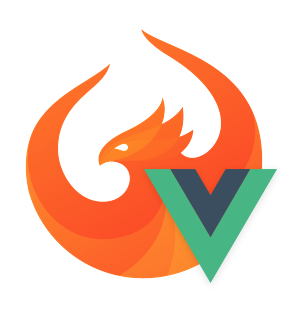
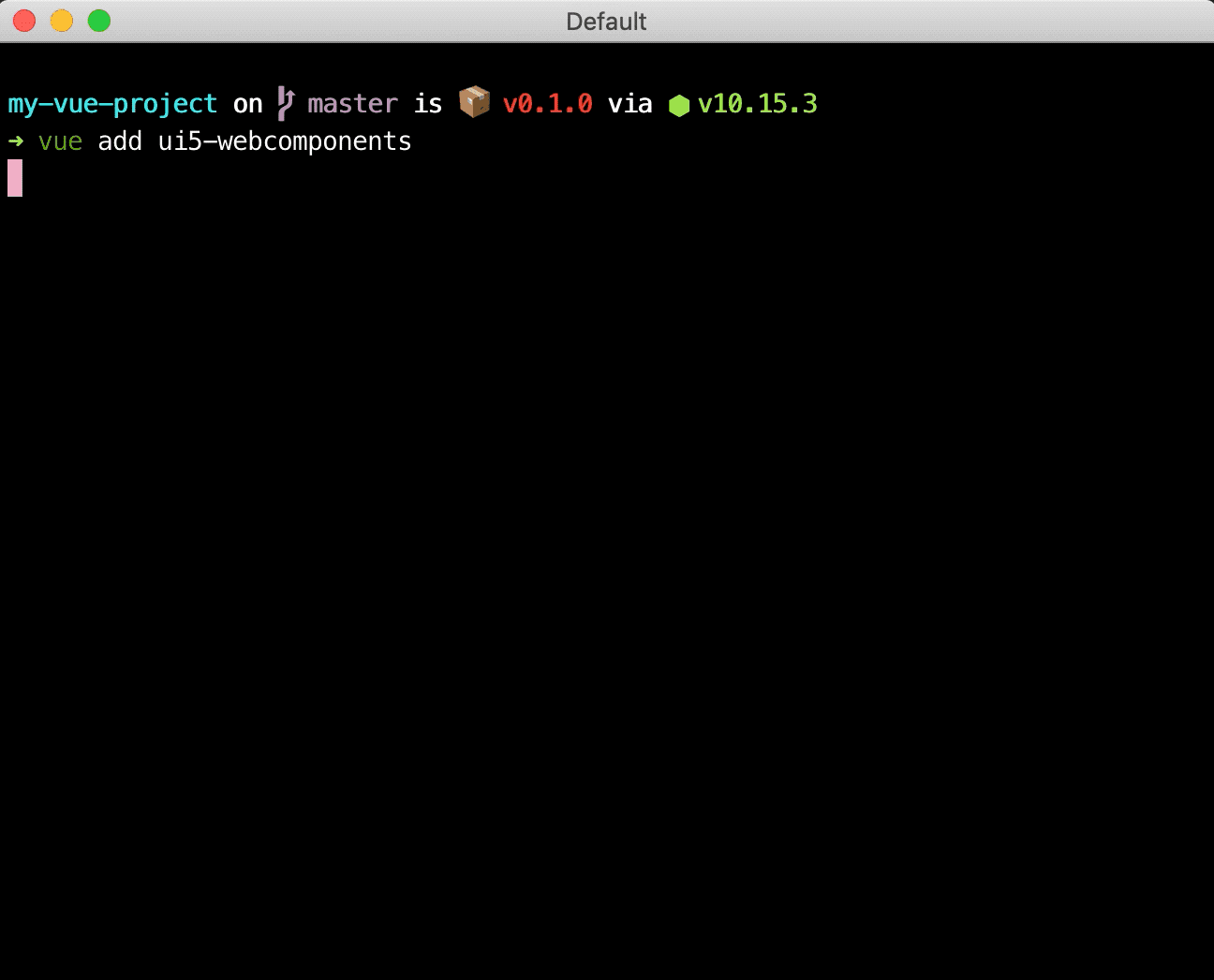
vue add ui5-webcomponents
<template>
<div class="main-box">
<ui5-shellbar :primary-title="title" />
</div>
</template>
<script>
import "@ui5/webcomponents/dist/ShellBar";
export default {
name: "app",
data() {
return {
title: "Cats are Awesome",
};
},
</script>
<style lang="scss">
.main-box {
display: flex;
flex-flow: column;
align-items: center;
}
</style>
<template>
<div class="buttons-box">
<ui5-button icon="sap-icon://thumb-up" @click="onLike">Like</ui5-button>
<ui5-button icon="sap-icon://thumb-down" type="Negative" @click="onUnLike"
>Not really</ui5-button
>
</div>
</template>
<script>
import "@ui5/webcomponents/dist/Button";
export default {
methods: {
onLike() {
this.$emit("like");
},
onUnLike() {
this.$emit("unlike");
}
}
};
</script>
<style scoped>
.buttons-box {
margin: 1rem;
width: 400px;
display: flex;
justify-content: space-between;
}
</style>
GroupButton.vue
v-if, v-else and v-else-if
๐
v-show
v-for
v-on
v-bind
v-model
๐
Resources
Thank you!
๐ป Demo ๐ป
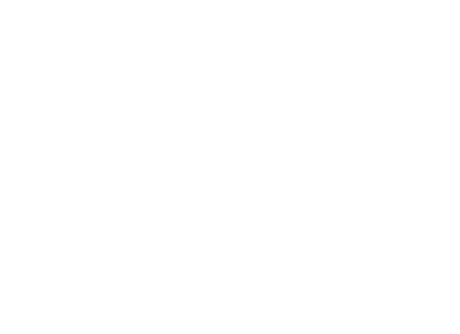
UI5 Web Components and Vue
By Ramona Biscoveanu
UI5 Web Components and Vue
UI5 Con 2019
- 2,038