Modern PHP development with Laravel
WIFI
hotel san marco congressi
sfps2017
GITHUB
/laravelday2018workshop
Who we are


Damiano Petrungaro
Christian Nastasi
<?php
$me = [
'name' => 'Damiano',
'surname' => 'Petrungaro',
'job' => 'Software Engineer',
'company' => [
'name' => 'HelloFresh',
'url' => 'https://hellofresh.com/'
],
'maintainers' => ['conventional commit', 'laravel italia'],
'github' => 'https://github.com/damianopetrungaro',
'twitter' => '@damiano_dev',
'buzzwords' => [
'food', 'php', 'golang', 'perfectionist', 'DDD', 'never stop learning'
]
];
<?php
$me = [
"name" => "Christian",
"surname" => "Nastasi",
"company" => null,
"job" => [
"Software Craftman",
"Technical Coach",
"Agile Coach"
],
"github" => "https://github.com/cnastasi",
"twitter" => "@c_nastasi",
"buzzwords" => [
"quality is not an optional",
"php",
"agile",
"ddd", "bdd", "tdd",
"framework-less",
"nerd"
]
];
Introduce yourself
What's your name?
What's your job?
What do you expect from this workshop?
Write down your doubts
What are we going to do
Create a Laravel application using modern methodologies:
-
TDD
-
Continuous Integration
-
Clean Code
-
Domain Driven Design
-
Hexagonal Architecture
How the workshop will be held
We are going to create an application with Laravel 5.x, using the framework as a tool.
During this journey, we'll discuss and learn all the topics we introduced to you.
There will be practical, theoretical and brainstorming sessions.
PHP
Evolution over time
The beginning
-
Born in 1994 as a set of “commands” written in C by Rasmus Lerdorf
-
Originally used for tracking visits on his websites
-
The name comes from “Personal Home Page Tools”
-
Over time, the project grows and new features were introduced
- In 1995 the project becomes open source

Composer
A tool for
dependency management in PHP

PSR0 & PSR4
No, namespaces in PHP are not magic.
It's still plain PHP.
return \spl_autoload_register([$this, 'loadClass'], true, $prepend);
Scripts
They are functionality called before/after some actions
...
"scripts": {
"post-root-package-install": [
"@php -r \"file_exists('.env') || copy('.env.example', '.env');\""
],
"post-create-project-cmd": [
"@php artisan key:generate"
],
"post-autoload-dump": [
"Illuminate\\Foundation\\ComposerScripts::postAutoloadDump",
"@php artisan package:discover"
]
},
...
Folder structure
app
bootstrap
config
database
public
resources
routes
storage
tests
vendor
App Folder
Broadcasting
Console
Events
Exceptions
Http
Jobs
Listeners
Notifications
Policies
Providers
Rules
Fork and clone the repo
Make it run
Set up Travis & Coverall
Environment Setup
Workshop Domain
Platform to publish scientific articles
Entities
Academic
Reviewer
Article
Academic
- Write an article
- Ask a reviewer to review an article
- Edit his/her articles
- Delete his/her unpublished article
- See feedback about an article
- Receive a notification when an article has been published
- Receive a notification when an article has been rejected
- List all his/her articles
- See an unpublished article
-
Registration number
(ACC-{10 random numbers}-DZZ) -
First name
-
Last name
-
Birth date
-
Major
-
Registration date
An academic is made of:
Reviewer
-
Publish an article
-
Review an article
-
Reject an article giving feedback
-
Receive an email to review an article
-
See the list of articles to review
-
Registration number (REV-{10 random numbers}-SYY)
-
First name
-
Last name
-
Birth date
-
Major
-
Registration date
A reviewer is made of:
Article
-
Can be listed by users
-
Can be read
-
Title
-
Body
-
Creation date
-
Last update date
-
Publish date
-
Author
-
Reviewer
An article is made of:
Article created
Article published
Article rejected
Important events
Choose your group name
Let's split in teams
15 minutes
Groups composed by 5 people
___________________________________________________________
Choose a person from the group to explain how would you create the project
Brainstorming
Laravel, it's a tool.
The domain, it's not a tool!

Value objects
It's an immutable object representing a value in a domain.
It's comparable to other value objects by its own values.
Entities
It's a mutable object with an identity, it can be represented in different ways.
It's comparable to other entities by its ID.
Repositories
It's an abstraction layer to provide access to all the entities and the value objects related to an aggregate.
Services
It's an object to use to manipulate entities and value objects when the logic doesn't fit or distort their integrity.
Not to be confused with the application or infrastructure services.
Events
It's an immutable object used to represent something important that occurred in the domain.
Practical Section
As a User I want to list all articles
Let's focus on this story:
Article
Entity
Value Objects
ListArticle
Use Case
Article
Repository
ListArticle Controller
Practical Section
Practical Section
Mob Programming
Practical Section
Let's focus on this story:
As an Academic I want to write an Article
Article
Entity
Value Objects
WriteArticle
Use Case
Academic
Repository
WriteArticle Controller
Academic
Entity
Value Objects
Practical Section
Practical Section
Mob Programming
Practical Section
Let's focus on this story:
As an User I want to read an Article
Practical Section
Let's discuss together the architecture
Practical Section
Implement it by yourself.
Practical Section
Discuss with your team.
Practical Section
Create a Product Backlog and sort the stories
Put everything in a Kanban board
(TODO - WORK IN PROGRESS - DONE)
Practical Section
Starting from the head of the backlog, take a story and implement it by yourself
Then create a Pull Request.
Every PR should be reviewed at least by 2 person of your team.
Let's review the questions
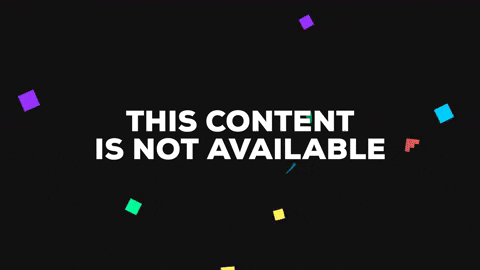
That's all folks
christian.nastasi@gmail.com
damianopetrungaro@gmail.com
Feel free to contact us
Letture:
https://bit.ly/2KJgeuq
Modern PHP development with Laravel - Wokshop
By Damiano Petrungaro
Modern PHP development with Laravel - Wokshop
- 991