Avoiding the Nightmare Rewrite
or
HOW I LEARNED TO LOVE IMPORTS AND STOP WORRYING ABOUT FRAMEWORKS
Who am i?
Daniel Sellers
software engineer.
team lead.
Maker of things.
not Peter Sellers.
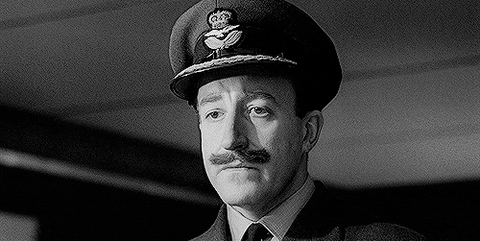
A brief history of making the internet
First there was a browser
1990—Sir Tim BErners-Lee
Then there was javascript
1994—Brendan Eich
jquery arrived
2006—John Resig
Angular
2009—Miško Hevery
Backbone
2010—Jeremy Ashkenas
Emberjs
2011—Yehuda Katz
React
2013—Jordan Walke
Vuejs
2014—Evan You
Ember 2
2015—Ember core team
Angular 2
2016—Angular Team
So...
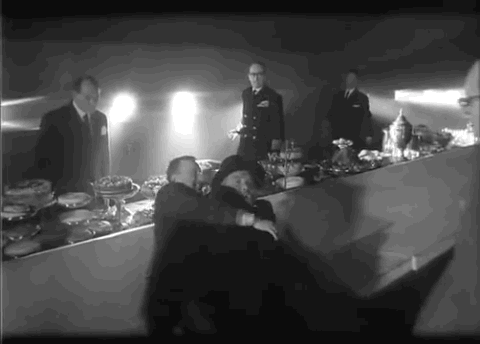
Why?!
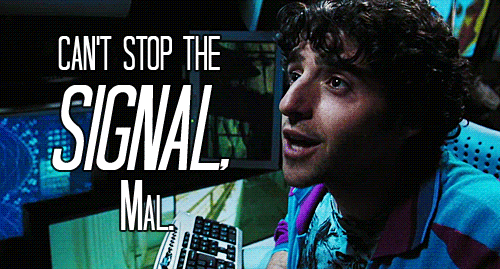
So what do we do?
A brief stop over in test land
const getFib = (i) => {
if (isNaN(new Number(i)) || typeof i === 'boolean' || new Number(i) < 0) {
throw new Error('TypeError');
}
if (i === -0) {
return 0;
}
return i <= 1 ? i : getFib(i - 1) + getFib(i - 2);
}
module.exports = getFib;
describe('fib tests', () => {
it('should return 0 for 0', () => {
assert.strictEqual(getFib(0), 0);
assert.strictEqual(getFib(-0), 0);
});
it('should return 1 for 1', () => {
assert.strictEqual(getFib(1), 1);
});
it('should return 89 for 11', () => {
assert.strictEqual(getFib(11), 89);
});
it('should return 357 for 14', () => {
assert.strictEqual(getFib(14), 377);
});
it('should throw type error for negative numbers', () => {
assert.throws(() => getFib(-1), 'TypeError');
assert.throws(() => getFib(-42), 'TypeError');
assert.throws(() => getFib(-Infinity), 'TypeError');
});
it('should throw type error for boolean', () => {
assert.throws(() => getFib(true), 'TypeError');
});
it('should work for string that is a number', () => {
assert.strictEqual(getFib('14'), 377);
assert.strictEqual(getFib('0x05'), 5);
assert.strictEqual(getFib('0x06'), 8);
});
it('should throw type error for string', () => {
assert.throws(() => getFib('hello world'), 'TypeError');
});
it('should throw a type error if a function', () => {
assert.throws(() => getFib(() => {return `can't take the sky from me`}), 'TypeError');
});
});
const getFib = (i) => {
if (isNaN(new Number(i)) || typeof i === 'boolean' || new Number(i) < 0) {
throw new Error('TypeError');
}
if (i === -0) {
return 0;
}
return i <= 1 ? i : getFib(i - 1) + getFib(i - 2);
}
module.exports = getFib;
wat?!
It's easy to prove that a function will do something
It is easy to prove it will not do a specific thing
It is harder to prove that it will only do a specific set of things*
*With some caveats
we're not here to talk about that directly though...
what does this have to do with anything?
don't house your business logic in your framework's code
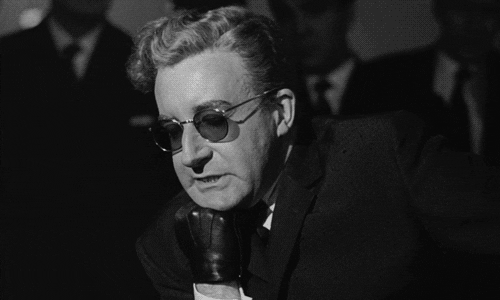
This might get a little extreme
Let's take a look...
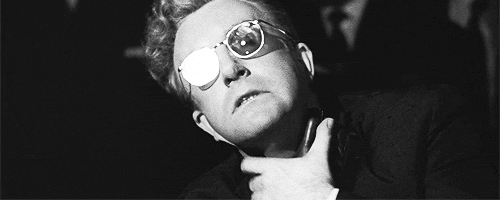
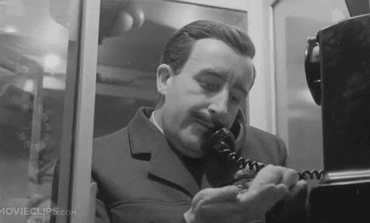
Get that logic out of your framework
use more pojs*
*plain old javascript
Plan for your next rewrite. It is coming.
go out and write code!
architecting for tests and rewrites
By Daniel Sellers
architecting for tests and rewrites
- 1,381