SPA
Single Page Application
A single-page application (SPA) is a web application or web site that interacts with the user by dynamically rewriting the current page rather than loading entire new pages from a server.
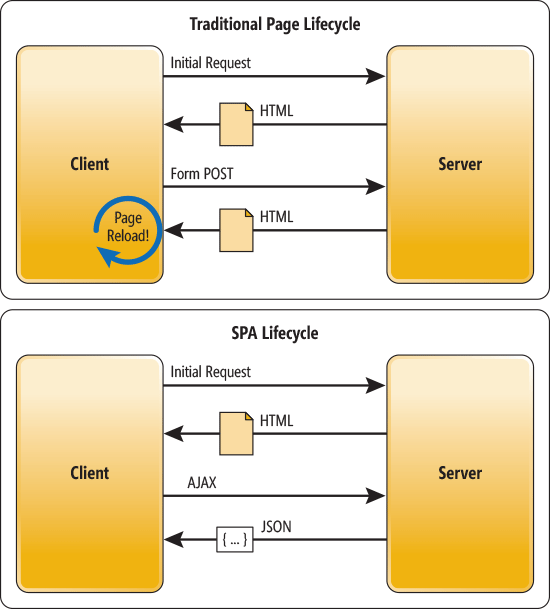
This approach avoids interruption of the user experience between successive pages, making the application behave more like a desktop application. In a SPA, either all necessary code – HTML, JavaScript, and CSS – is retrieved with a single page load, or the appropriate resources are dynamically loaded and added to the page as necessary, usually in response to user actions.
The page does not reload at any point in the process, nor does control transfer to another page, although the location hash or the HTML5 History API can be used to provide the perception and navigability of separate logical pages in the application.
Interaction with the single page application often involves dynamic communication with the web server behind the scenes.
Manipulating the browser history
The DOM window object provides access to the browser's history through the history object. It exposes useful methods and properties that let you move back and forth through the user's history, as well as -- starting with HTML5 -- manipulate the contents of the history stack.
Moving forward and backward
window.history.back();
window.history.forward();
To move backward through history, just do:
Similarly, you can move forward (as if the user clicked the Forward button), like this:
Moving to a specific point in history
You can use the go() method to load a specific page from session history, identified by its relative position to the current page (with the current page being, of course, relative index 0).
To move back one page (the equivalent of calling back()):
window.history.go(-1);
To move forward a page, just like calling forward():
window.history.go(1);
Adding and modifying history entries
HTML5 introduced the history.pushState() and history.replaceState() methods, which allow you to add and modify history entries, respectively. These methods work in conjunction with the window.onpopstate event.
The onpopstate property
The onpopstate property of the WindowEventHandlers mixin is the EventHandler for processing popstate events on the window.
A popstate event is dispatched to the window each time the active history entry changes between two history entries for the same document. If the activated history entry was created by a call to history.pushState(), or was affected by a call to history.replaceState(), the popstate event's state property contains a copy of the history entry's state object.
Note: Calling history.pushState() or history.replaceState() won't trigger a popstate event. The popstate event is only triggered by performing a browser action, such as clicking on the back button (or calling history.back() in JavaScript), when navigating between two history entries for the same document.
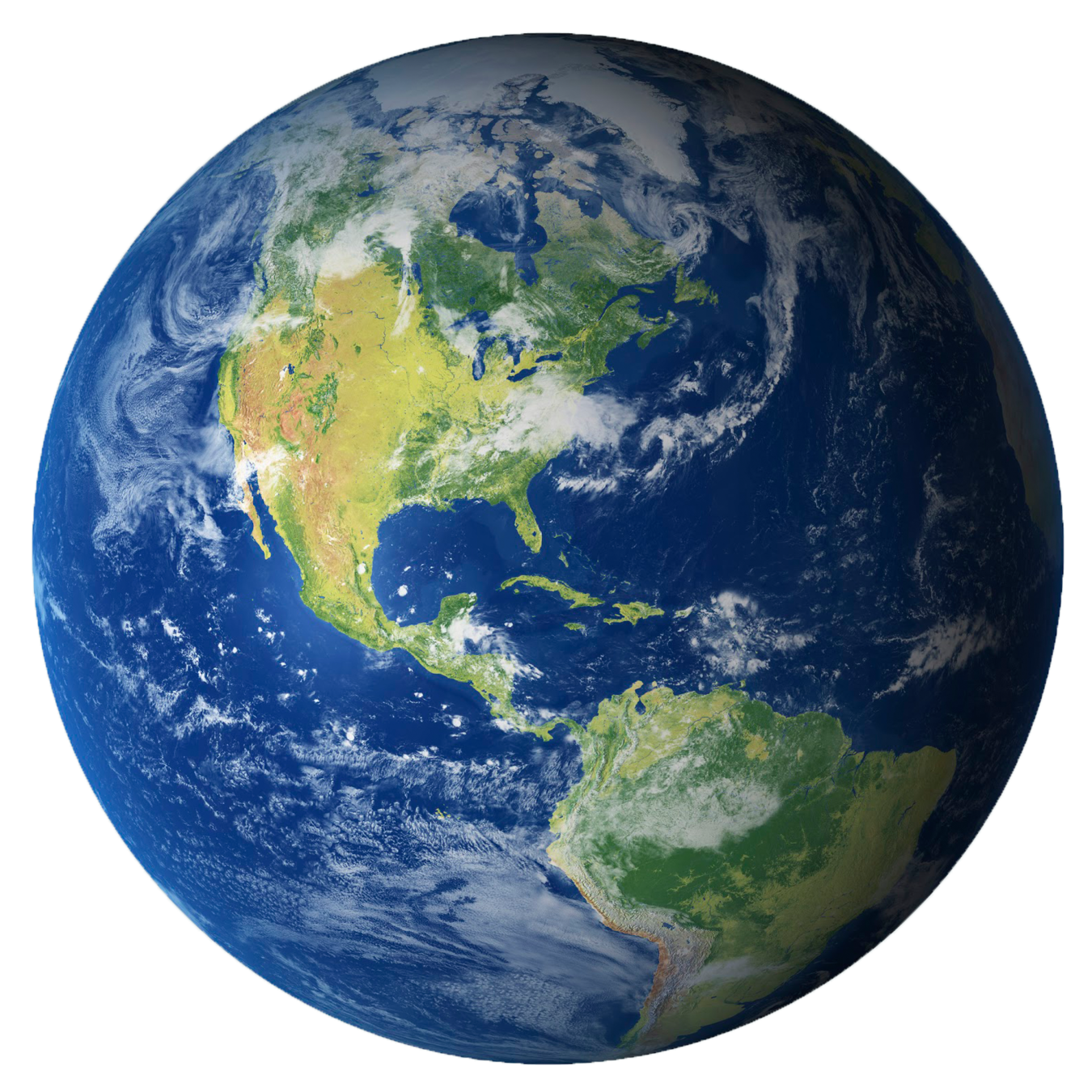
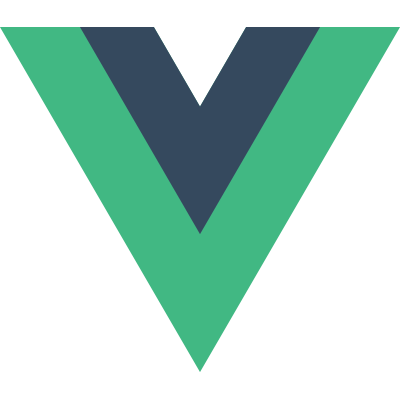
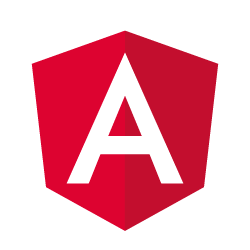
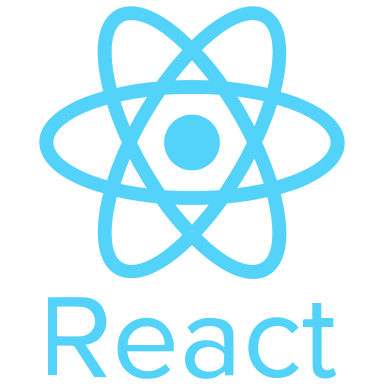
MVC architecture
The Model View Controller software architecture pattern
Model View Controller (MVC) is a software architecture pattern, commonly used to implement user interfaces: it is therefore a popular choice for architecting web apps. In general, it separates out the application logic into three separate parts, promoting modularity and ease of collaboration and reuse. It also makes applications more flexible and welcoming to iterations.
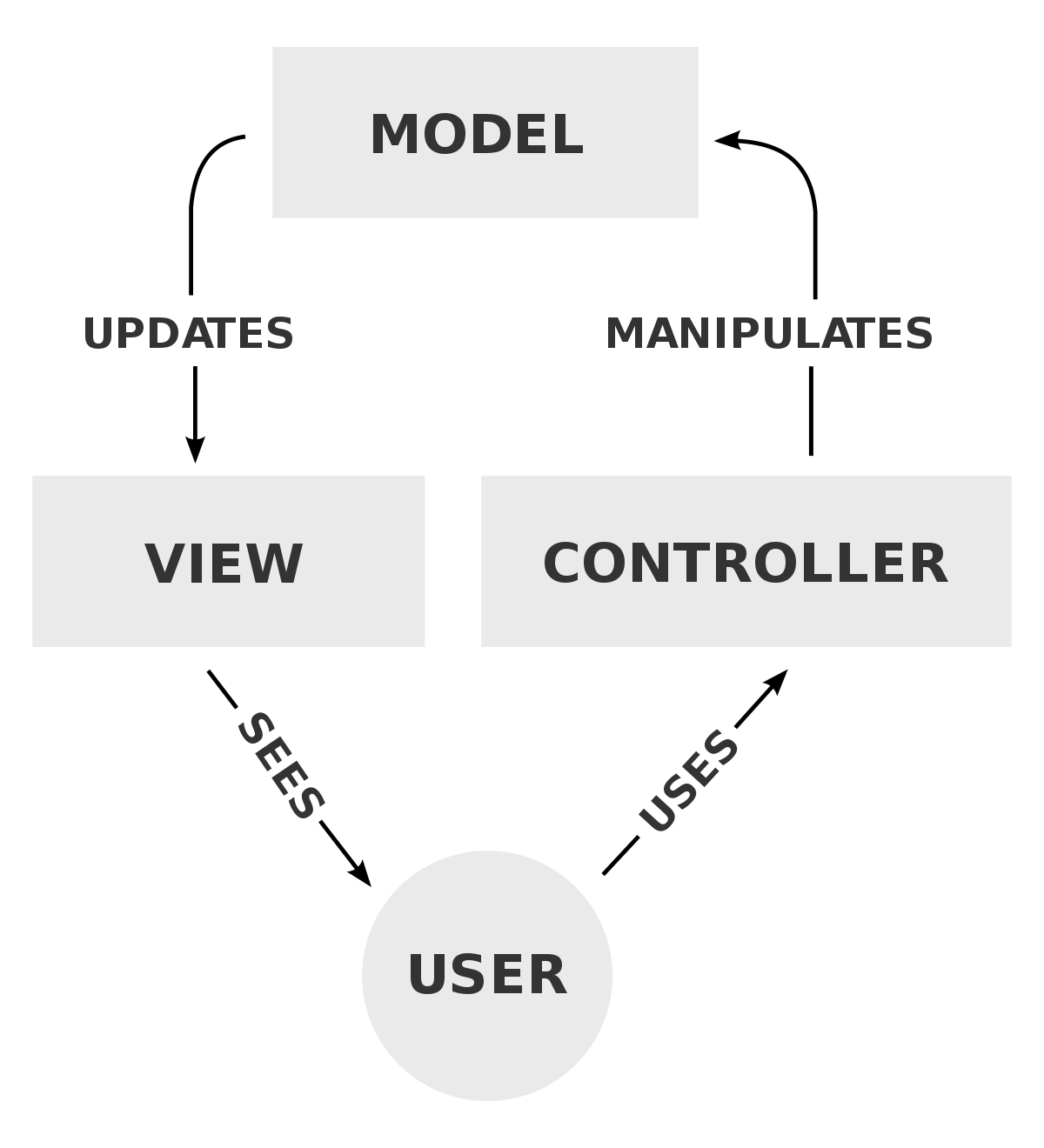
spa
By Daniel Suleiman
spa
- 564