Building Frontends with GraphQL+Vue

Dhiraj Shrotri
Full Stack Developer
-
Big Manchester United fan
-
Futurama is the best show ever
-
Likes watching conspiracy videos

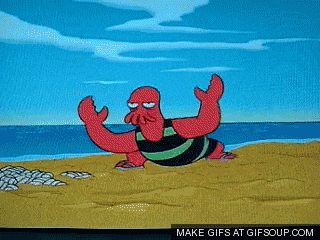
Let's dive in
Installation
Using vue-cli plugin
vue add apollo
Manual Install using Apollo Boost
npm install --save vue-apollo graphql apollo-boost
Create ApolloClient Instance
import ApolloClient from 'apollo-boost';
import VueApollo from "vue-apollo";
const apolloClient = new ApolloClient({
uri: ''
});
const apolloProvider = new VueApollo({
defaultClient: client
});
Vue.use(VueApollo);
Manual Install using ApolloClient
npm install --save vue-apollo graphql apollo-client apollo-link apollo-link-http apollo-cache-inmemory graphql-tag
Create Apollo Client instance
import { ApolloClient } from 'apollo-client'
import { createHttpLink } from 'apollo-link-http'
import { InMemoryCache } from 'apollo-cache-inmemory'
const httpLink = createHttpLink({
uri: 'http://localhost:3020/graphql',
})
const cache = new InMemoryCache()
const apolloClient = new ApolloClient({
link: httpLink,
cache,
})
import Vue from 'vue'
import VueApollo from 'vue-apollo'
Vue.use(VueApollo)
const apolloProvider = new VueApollo({
defaultClient: apolloClient,
})
new Vue({
el: '#app',
apolloProvider,
render: h => h(App),
})
What we want to do
-
Register Users
-
Sign In Users
-
Fetch All Products
-
Fetch a particular Product
-
Place an Order for a Product
-
See all User Orders
-
Cancel an Order placed by User
Schema Definations
type Products {
ProductID: String
ProductName: String
Price: Float
ProductImageURL: String
ProductNameShort: String
ProductDescription: String
}
Product Schema
type User {
email: String
password: String
FullName: String
PaymentInformation: String
Address: String
UserID: String
}
User Schema
type Orders {
PaymentInformation: String
ShippingInformation: String
TotalPrice: Float
HasOrder: User
}
Order Schema
type Query {
User (UserID: String): User
Products: [Products]!
getProduct(ProductID: String): Products
GetAllComponents(ProductID: String): [Products]!,
GetUserOrders (UserID: String): [Orders]!
}
Queries
type Mutation {
placeOrder(UserID: String, ProductID: String): Message
registerUser(email: String,
password: String,
FullName: String,
PaymentInformation: String,
Address: String): User,
login(email: String,
password: String): User # returns user
}
Mutations



Building Frontends with GraphQL+Vue
By Dhiraj Shrotri
Building Frontends with GraphQL+Vue
- 81