Introduction to JVM JIT Optimizations
Diego Parra | @dpsoft | Core Team @ Kamon
Agenda
- What is JIT?
- Tiered Compilation
- Optimizations
- Conclusion

JIT
- Just-In-Time compilation
- Compilation work happens during application execution

-
JIT'ing requires Profiling for more efficient optimization
- Because you don't want JIT everything
- Aggressively optimize based on profile information
- Tiered compilation
- Runtime Overhead?
JIT Lifecycle
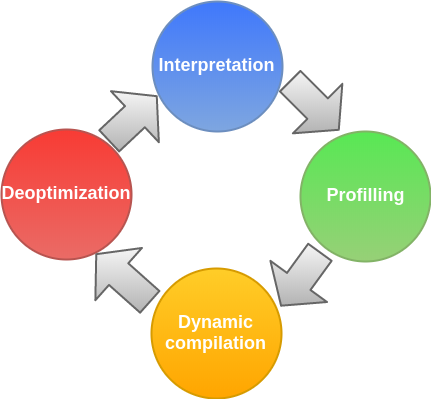
JIT Compilers
C1 Client
C2 Server
VS
- Fast compiler
- Invocation count > 1500
- Produces Compilations quickly
- Generated code runs Slow
Profile is about counters: https://github.com/dmlloyd/openjdk/blob/jdk8u/jdk8u/hotspot/src/share/vm/oops/methodCounters.hpp
- Smart compiler
- Invocation count > 10000
- Produces Compilations slowly
- Generated code runs Fast
Profiled Guided
Speculative
10x faster than Interpreter
2x faster than C1
Tiered Compilation

- Available in Java 7
- Default in Java 8
- Client Startup
- Server Performance
Best of Both Worlds - C1 + C2
Common Transitions
Common Transitions Patterns : https://www.slideshare.net/maddocig/tiered

JIT Optimizations
Compiler tactics
- delayed compilation
- tiered compilation
- on-stack replacement
- delayed reoptimization
- program dependence graph representation
- static single assignment representation
Speculative (profile-based)
- optimistic nullness assertions
- optimistic type assertions
- optimistic type strengthening
- optimistic array length strengthening
- untaken branch pruning
- optimistic N-morphic inlining
- branch frequency prediction
- call frequency prediction
Proof-based techniques
- exact type inference
- memory value inference
- memory value tracking
- constant folding
- reassociation
- operator strength reduction
- null check elimination
- type test strength reduction
- type test elimination
- algebraic simplification
- common subexpression elimination
- integer range typing
Flow-sensitive rewrites
- conditional constant propagation
- dominating test detection
- flow-carried type narrowing
- dead code elimination
Text
and much more..
Null check Elimination


In equivalent machine code

Null check Elimination
Into the rabbit hole
-XX:+PrintCompilation

Compilation Level
Deoptimizations
Null check Elimination
Into the rabbit hole
- -XX:+UnlockDiagnosticVMOptions
- -XX:+PrintAssembly
- -XX:CompileCommand=print,NullCheck::isPowerOfTwo
Someone said Assembly?

Null check Elimination
Into the rabbit hole

implicit exception: dispatches to 0x00007fdff5318274
Null check Elimination
-
JVM uses SIGSEGV as control flow
Into the rabbit hole
-
Speculative Optimization
-
Faster IF not null are encountered (Uncommon Trap)
Implicit Null Check?
- Field and Array access is null checked

Method Inlining

-XX:+PrintInlining


Method Inlining
- Inlining is the most important optimization
- Combine caller and callee into one unit
- Expands the scope for other optimizations
- Bytecode size < 35 bytes
- Bytecode size < 325 bytes for inlining hot methods
What about virtual call inlining?
Monomorphic
Bimorphic
Megamorphic
- Class Hierarchy Analysis
- Devirtualization





On-Stack Replacement


-XX:+PrintCompilation
-XX:+PrintInlining

Compilation is OSR
Dead Code Elimination
Result is not used and the entire method is optimized away!

Escape Analysis

- No synchronization lock when calling getNoEscapeSum method
- No allocate a Sum object at all, just keep track of the individual fields of the object
Escape Analysis for Java: http://www.research.ibm.com/people/j/jdchoi/escape.ps
Deoptimization

- The compiler can back to previous versions of compiled code
- Effect in performance when code is deoptimized
- Deoptimize when previous optimizations are no longer valid
There are two cases of deoptimization
- not entrant -> don't let new calls enter
- zombi -> on this way to deadness
Loop Unrolling


Unrolled!
Intrinsics
- Special code build-into the VM for a particular method
- Often use special hardware capabilities
- Not writen in Java

JITWatch

Jitwatch: https://github.com/AdoptOpenJDK/jitwatch
Conclusion
- Please use JMH, Don't lie to yourself
- Code will be Optimized and De-optimized
- JVM require Warmup
- JVM is Smart
- Keep It Simple, Stupid!, is perfect for JIT to make his job
- Learn Assembly
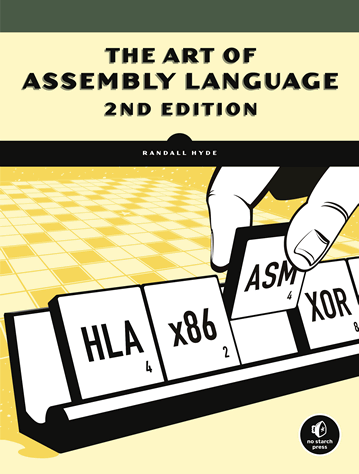
Resources
- Java Performance: http://shop.oreilly.com/product/0636920028499.do
- Optimizing Java: http://shop.oreilly.com/product/0636920042983.do
- Aleksey Shipilëv (blog): https://shipilev.net/
- Nitsan Wakart (blog): http://psy-lob-saw.blogspot.com.ar/
- JVM Source Code: https://github.com/dmlloyd/openjdk
- Open JDK Wiki: https://wiki.openjdk.java.net/dashboard.action
Questions?
JIT Optimizations
By Diego Parra
JIT Optimizations
- 820