Compiled to JS languages
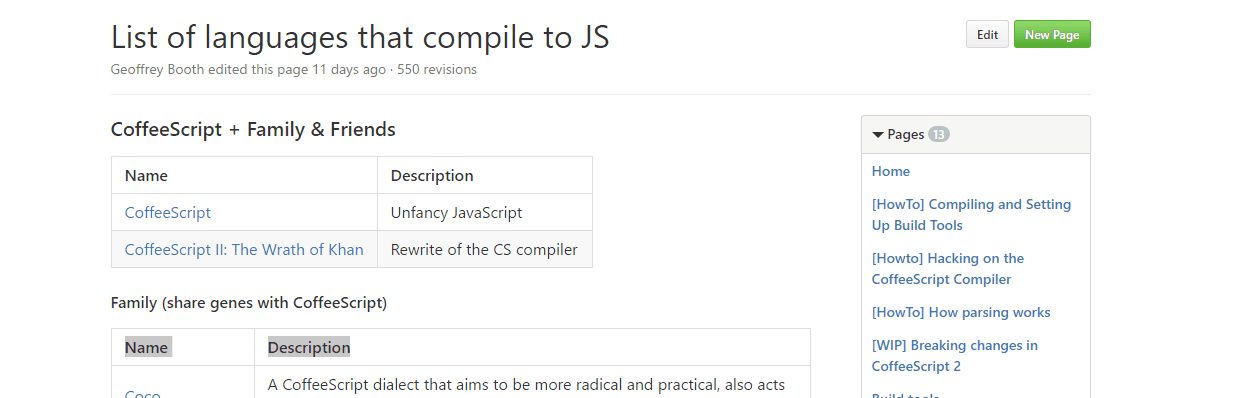
WAIT.. ANOTHER TOOL?!
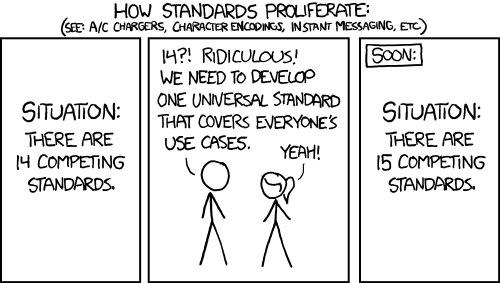
CoffeeScript
JavaScript in a simple way
ABOUT
CoffeeScript is a programming language that compiles into JavaScript. It is highly influenced by Python and Ruby.
First released on Christmas day 2009
Quickly gained popularity
Compiles into the equivalent JS code
Simple syntax
Language basics
Objects
cookiePlan =
Monday: "chocolate chip"
Tuesday: "oatmeal raisin"
Thursday: "peanut butter"
Friday: ["chocolate chip", "oatmeal raisin"]
var cookiePlan = {
Monday: "chocolate chip",
Tuesday: "oatmeal raisin",
Thursday: "peanut butter",
Friday: ["chocolate chip", "oatmeal raisin"]
}
CoffeeScript
JavaScript
SLICING ARRAYS
bands = ['One', 'Two', 'Three']
first = bands[0..0]
both = bands[0..1]
all = bands[..]
bands[2..2] = ['Another']
CoffeeScript borrows Python's syntax to replace JavaScript's slice function.
Functions
hello = ->
"Hello WWC!"
helloFriend = (name) ->
"Hello #{name}"
var helloWorld = function() {
return "Hello World!";
}
var helloFriend = function(name) {
return "Hello " + name;
}
CoffeeScript
JavaScript
optionaL Parameters
var eat;
eat = function(food) {
var answer;
if (food == null) {
food = "I want some Coffee";
}
answer = confirm(food);
return "Your answer is " + answer;
};
eat();
alert(eat("Apple"));
eat = (food = "I want some Coffee") ->
answer = confirm food
"Your answer is #{answer}"
eat()
alert eat "Apple"
CoffeeScript
JavaScript
String interpolation
language1 = "CoffeeScript"
language2 = "JavaScript"
console.log "What do you prefer, #{language1} or #{language2}?"
Classes
class Animal
constructor: (name, organ="lung") ->
@name = name
@organ = organ
breathe: -> alert "I breathe #{@organ}"
dog = new Animal("Reksio", "lung")
dog.breathe()
CoffeeScript
JavaScript
var Animal, dog;
Animal = (function() {
function Animal(name, organ) {
if (organ == null) {
organ = "lung";
}
this.name = name;
this.organ = organ;
}
Animal.prototype.breathe = function() {
return alert("I breathe " + this.organ);
};
return Animal;
})();
dog = new Animal("Reksio", "lung");
dog.breathe();
Inheritance
class Animal
constructor: (@name) ->
breathe:(organ) ->
alert "I am " + @name + " and I breathe #{organ}"
class Dog extends Animal
breathe: ->
super "lungs"
class Shrimp extends Animal
breathe: ->
super "gills"
one = new Dog("One")
one.breathe()
two = new Shrimp("Two")
two.breathe()
Splats
searchCoffee = (brand, cities...) ->
"looking for #{brand} in #{cities.join ','}"
searchCoffee 'CoffeeHeaven', 'Warsaw', 'Cracow'
# 'looking for CoffeeHeaven in Warsaw, Cracow'
Existential operator
var user;
user = {
name: "John",
age: null,
activate: null
};
if ((user != null ? user.age : void 0) != null) {
if (typeof user.activate === "function") {
user.activate();
}
}
user =
name : "John"
age: null
activate: null
if user?.age?
user.activate?()
CoffeeScript
JavaScript
Potential drawbacks
- Debugging might be confusing
- Not so popular nowadays
- leaving out parenthesis in function calls might be confusing
- Last statement is always returned
- Some things like loops when compiled with CS compiler not very readable
Trends
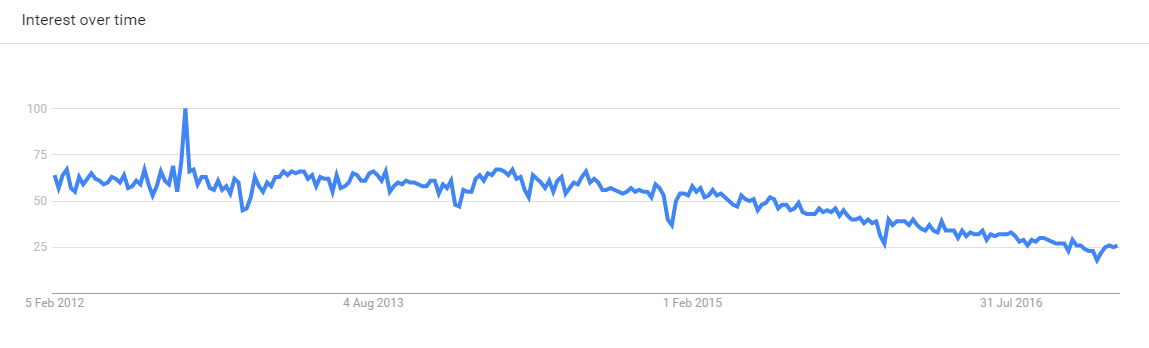
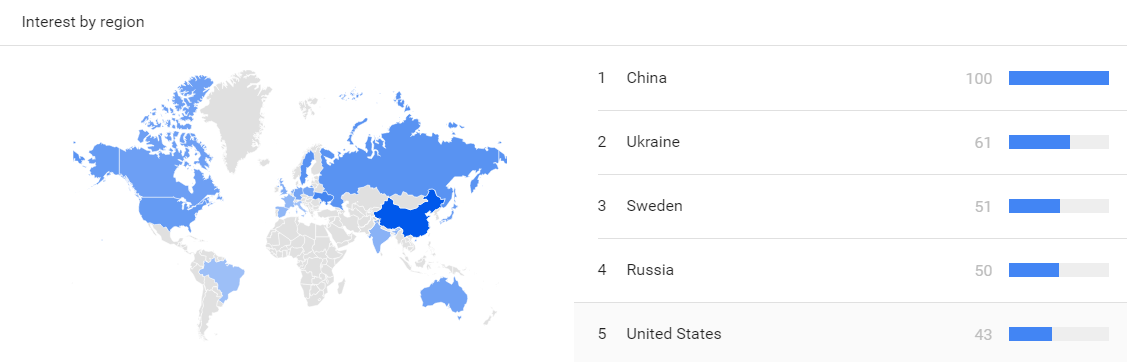
Typescript 2
gift of the gods
ES5
ES2015
ES2016
TS
ES+
What is typescript
WHY USE TYPESCRIPT?
- Better overall code readability in large codebases
- Ease of refactoring
- Use new ES6 features in older browsers
- IntelliSense auto suggest in code editors

What is New
async/await
function delay(ms: number) {
return new Promise<void>(function(resolve) {
setTimeout(resolve, ms);
});
}
async function asyncAwait() {
console.log("Knock, knock!");
await delay(1000);
console.log("Who's there?");
await delay(1000);
console.log("async/await!");
}
asyncAwait();
// [After 0s] Knock, knock!
// [After 1s] Who's there?
// [After 2s] async/await!
TypeScript 2.1 now supports downleveling asynchronous functions to ES3 and ES5.
Rest/Spread
var obj = { x: 1, y: 2};
var obj1 = {...obj, z: 3, y: 4}; // not an error
var obj = {x:1, y: 1, z: 1};
var {z, ...obj1} = obj;
obj1 // {x: number; y:number};
Spread
Rest
Control flow analysis
function f(cond: boolean) {
let x;
if (cond) {
x = "hello";
x; // string
} else {
x = 123;
x; // number
}
return x; // string | number
}
types for properties
interface Thing {
name: string;
width: number;
height: number;
inStock: boolean;
}
type K1 = keyof Thing; // "name" | "width" | "height" | "inStock"
type K2 = keyof Thing[]; // "length" | "push" | "pop" | "concat" | ...
function getProperty<T, K extends keyof T>(obj: T, key: K) {
return obj[key]; // Inferred type is T[K]
}
function setProperty<T, K extends keyof T>(obj: T, key: K, value: T[K]) {
obj[key] = value;
}
function f1(thing: Thing, propName: "name" | "width") {
let name = getProperty(thing, "name"); // Ok, type string
let size = getProperty(thing, "size"); // Error, no property named "size"
setProperty(thing, "width", 42); // Ok
setProperty(thing, "color", "blue"); // Error, no property named "color"
let nameOrWidth = getProperty(thing, propName); // Ok, type string | number
}
Mapped types
Mapped Types - a new kind of object type that maps a type representing property names over a property declaration template.
interface Shape {
name: string;
width: number;
height: number;
visible: boolean;
}
// A proxy for a given type
interface Proxy<T> {
get(): T;
set(value: T): void;
}
// Proxify all properties in T
type Proxify<T> = {
[P in keyof T]: Proxy<T[P]>;
}
function proxify<T>(obj: T): Proxify<T> {
return null;
}
function f5(shape: Shape) {
const p = proxify(shape);
let name = p.name.get();
p.visible.set(false);
}
Non-nullable types
// Compiled with --strictNullChecks
let x: number;
let y: number | null;
let z: number | undefined;
x; // Error, reference not preceded by assignment
y; // Error, reference not preceded by assignment
z; // Ok
x = 1;
y = null;
x; // Ok
y; // Ok
TypeScript has two special types, Null and Undefined, that have the values null and undefined respectively.
Path mappings
{
"compilerOptions": {
"rootDir": ".",
"baseUrl": "./src",
"paths": {
"*": [
"ui/*"
]
}
}
}
import * as lib from 'some-lib-in-ui-folder';
tsconfig.json
yourFile.ts
Declaration File Acquisition
npm install --save @types/lodash
Untyped imports
- TypeScript has traditionally been overly strict about how you can import modules.
- However, a lot of the time, you might just want to import an existing module that may not have its own .d.ts file. Previously this was an error.
- Starting with TypeScript 2.1 this is now much easier.
// Succeeds if `node_modules/asdf/index.js` exists
import { x } from "asdf";
yoptascript
Первый в мире скриптовый язык программирования для гопников и реальных пацанов.
About
- YoptaScript это первый в мире скриптовый язык программирования для гопников и реальных пацанов, транслируемый в чистый JavaScript.
- YoptaScript позволит "чётким пацанам" быстро влиться в ряды программистов и процесс разработки.
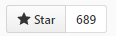
Example
гыы gop внатуре пиздишь, lt нах
куку йопта law() жЫ
вилкойвглаз(gop типа нечотко) жЫ
ксива.малява("Я и правда язык") нах
gop сука чотко нах
есть иливжопураз жЫ
gop сука чотко нах
потрещим(semki чоблясука трулио) жЫ
lt сука ксива.вычислитьЛохаПоНомеру("list") нах
ебало.шухер("Привет, йопта") нах
есть
есть
есть
var gop = false, lt;
void function law() {
if(gop == false) {
document.write("Я и правда язык");
gop = true;
} else {
gop = true;
while(semki != true) {
lt = document.getElementById("list");
window.alert("Привет, йопта");
}
}
}
Origin
При разработке языка использовались основные лексические и фразеологические единицы диалекта "нормальных пацанов" позаимствованные из особых словарей
Толчком к разработке YoptaScript послужили события, произошедшие в середине 2016 года, в ходе которых журналистами Piter.TV была открыта такая категория программистов как гопники-программисты.
ELM
A delightful language for reliable webapps
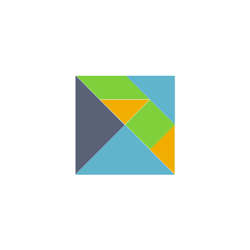
FRONT-END stack
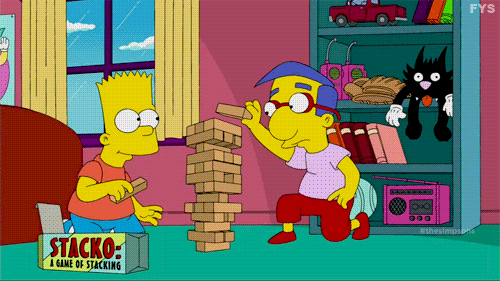
PAIN POINTS WITH JS
- No static typing means simple typos can lead to runtime errors
- Deep changes to data structures in non-trivial JS projects need increadible amounts of diligence and attention
- A big part of the unit & integration tests test really boring properties (does it correctly reject an undefined param, ...)
NULL
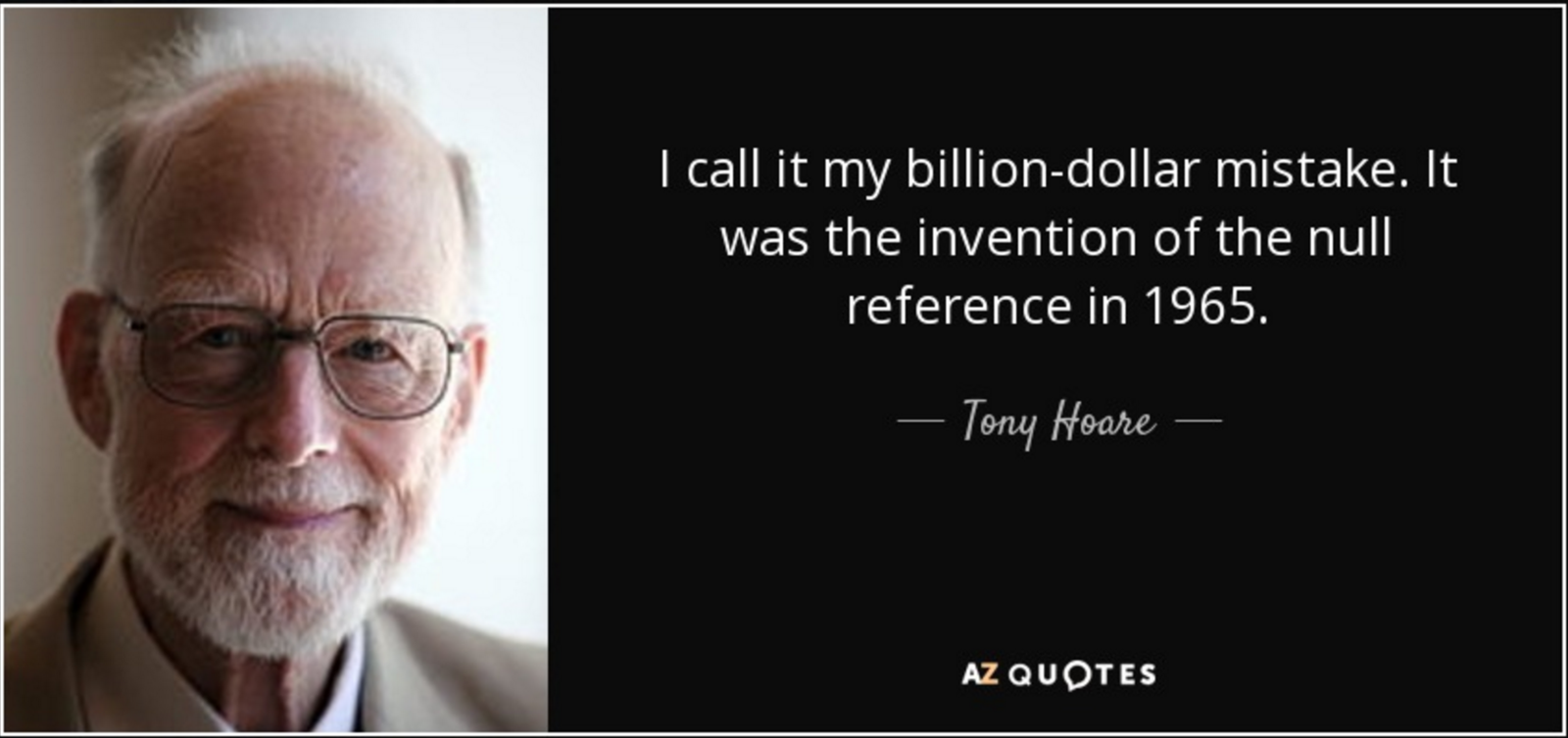
WHAT IS ELM?
-
ELM IS A PURELY FUNCTIONAL, DECLARATIVE PROGRAMMING LANGUAGE FOR UI DEVELOPMENT,
-
Immutable
-
Static Type System
-
No runtime errors
-
useS Virtual-DOM
-
It's not a Framework. Not a library. It's a whole different language.
SIMPLE EXAMPLE
import Html exposing (text)
main = text "Hello World"
In box
ELM-REPL
Experiment with elm on the command line
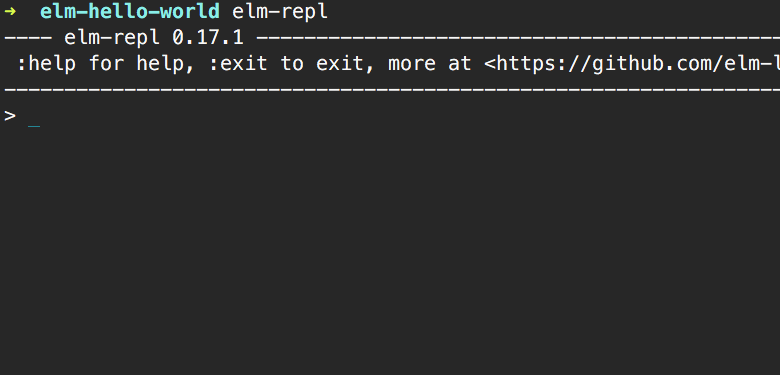
ELM-MAKE
Build and compile your .elm files to JavaScript
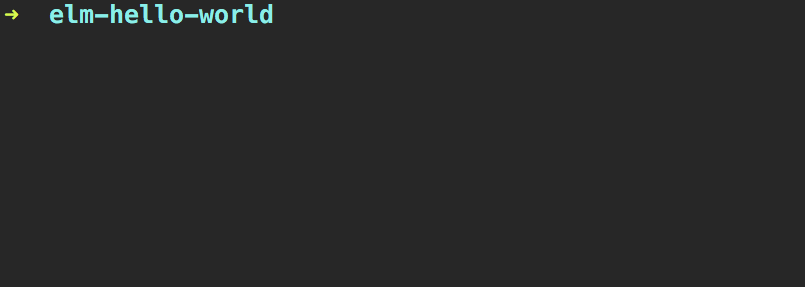
ELM-REACTOR
Build & serve locally
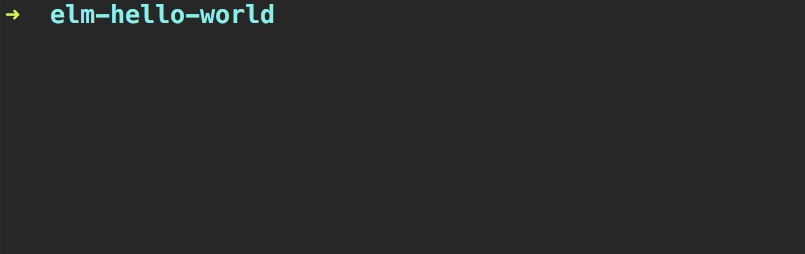
ELM-PACKAGE
Install and publish elm packages.
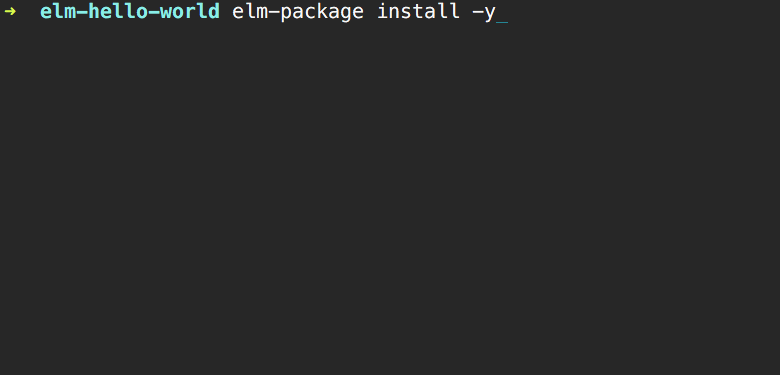
DIFF IN API
$ elm-package diff evancz/elm-html 3.0.0 4.0.0
Comparing evancz/elm-html 3.0.0 to 4.0.0...
This is a MAJOR change.
------ Changes to module Html.Attributes - MAJOR ------
Removed:
boolProperty : String -> Bool -> Attribute
stringProperty : String -> String -> Attribute
------ Changes to module Html.Events - MINOR ------
Added:
type alias Options =
{ stopPropagation : Bool, preventDefault : Bool }
defaultOptions : Html.Events.Options
onWithOptions : String -> Html.Events.Options
-> Json.Decode.Decoder a
-> (a -> Signal.Message) -> Html.Attribute
Automatically enforces SemVer
Language basics
THE ELM ARCHITECTURE (TEA)
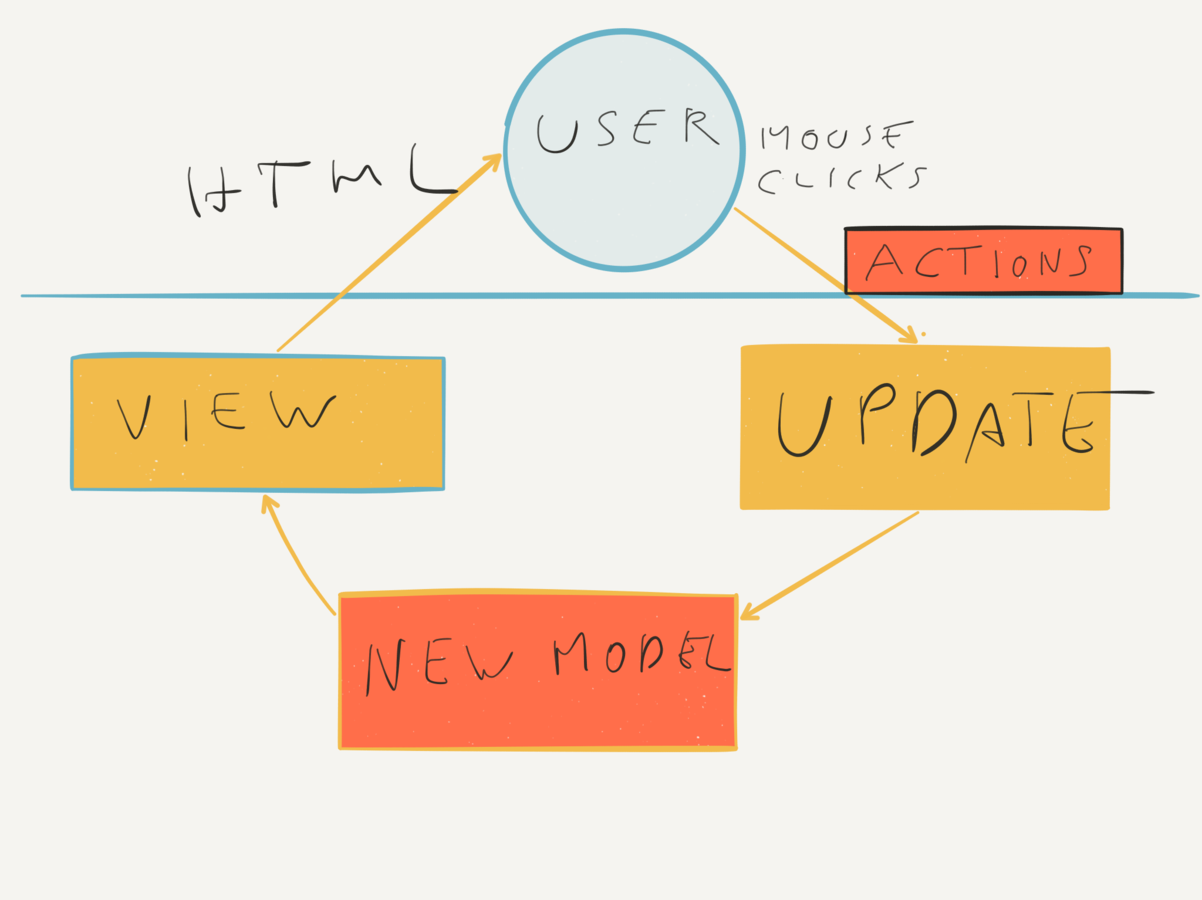
Counter
A basic pattern for web-app architecture: model - view - update
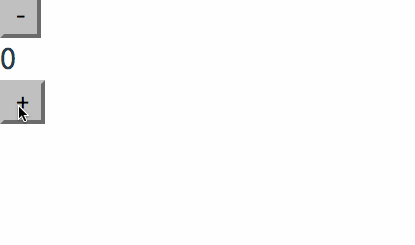
TEA
import Html exposing (..)
import Html.Events exposing (onClick)
-- application entry point
main =
beginnerProgram { model = 0, view = view, update = update }
-- view function
view model =
div []
[ button [ onClick Decrement ] [ text "-" ]
, div [] [ text (toString model) ]
, button [ onClick Increment ] [ text "+" ]
]
-- update function
type Msg = Increment | Decrement
update msg model =
case msg of
Increment ->
model + 1
Decrement ->
model - 1
BENEFITS
-
Model is single source of truth
-
Apps are well structured.
-
All state modifications happen in the central update
-
Easy to replay whole UI sessions
SYNTAX
BASICS
-- Boolean
True : Bool
False : Bool
42 : number -- Int or Float depending on usage
3.14 : Float
'a' : Char
"abc" : String
-- a single line comment
{- a multiline comment
{- can be nested -}
-}
{--}
add x y = x + y
--}
RECORDS
person =
{ firstName = "John"
, lastName = "Doe",
, age = 22
}
person.firstName -- "John"
.firstName person -- "John"
{ person |
firstName = "George" }
-- { firstName = "George"
-- , lastName = "Doe"
-- }
canDrinkAlcohol {age} =
age > 18
canDrinkAlcohol person -- True
UNION TYPES
-- Union Type
type Gender
= Male
| Female
| Other String
personAGender = Male
personBGender = Other "Androgynous"
printGender gender =
case gender of
Male ->
"male"
Female ->
"female"
Other selfdefined ->
selfdefined
CONDITIONALS
if key == 40 then
n + 1
else if key == 38 then
n - 1
else
n
-- By the way, there
-- is no "Truthy" of "Falsy"
FUNCTION ANATOMY
-- anonymous function
(\ name -> "hello " ++ name)
-- named function
sayHello name = "hello " ++ name
-- function use
msg = sayHello "meetup" -- "hello meetup"
FUNCTION TYPE
sayHello : String -> String
sayHello name = "hello " ++ name
add : number -> number -> number
add x y = x + y
Functions in Elm take one argument
FUNCTIONS ARE CURRIED
add : number -> number -> number
add x y = x + y
addOne = add 1
addOne 2 -- returns 3
-- The following are equivalent
add 1 2
PIPING: CHAINING FUNCTIONS
Html.text (String.repeat 3 (String.toUpper "Hello ! ")
Html.text <| String.repeat 3 <| String.toUpper "Hello ! "
"Hello !"
|> String.toUpper
|> String.repeat 3
|> Html.text
FUNCTION COMPOSITION
double x = x * 2
addOne x = x + 1
(double >> addOne) 2 -- 5
(double << addOne) 2 -- 6
SWITCH CASE
type Shape
= Circle Float
| Rectangle Float Float
area : Shape -> Float
area shape =
case shape of
Circle radius ->
pi * radius ^ 2
Rectangle height width ->
height * width
INTEROP WITH "NATIVE JAVASCRIPT"
Incoming Ports (send messages from JS -> Elm)
Outgoing Ports (send messages from Elm -> JS)
WHAT DOES ALL THIS BUY US?
PURITY
CODE IS DETERMINISTIC, IMMUTABLE,
REFERENTIALLY TRANSPARENT,
AND SIDE EFFECT FREE.
SAFETY
RUN-TIME ERRORS IN
ELM ARE EXCEPTIONALLY RARE.
TESTABILITY
SINCE ALL ELM FUNCTIONS ARE PURE,
TESTING BEHAVIOR IS TRIVIAL.
UNIT TESTS CAN FOCUS ON LOGIC
- In Javascript, unit tests have to check for undefined etc
- In Elm, the type system takes care of all of this
- Unit tests in Elm can focus on behaviour and logic
- Pure functions are super nice to test (little mocking required)
PERFORMANCE
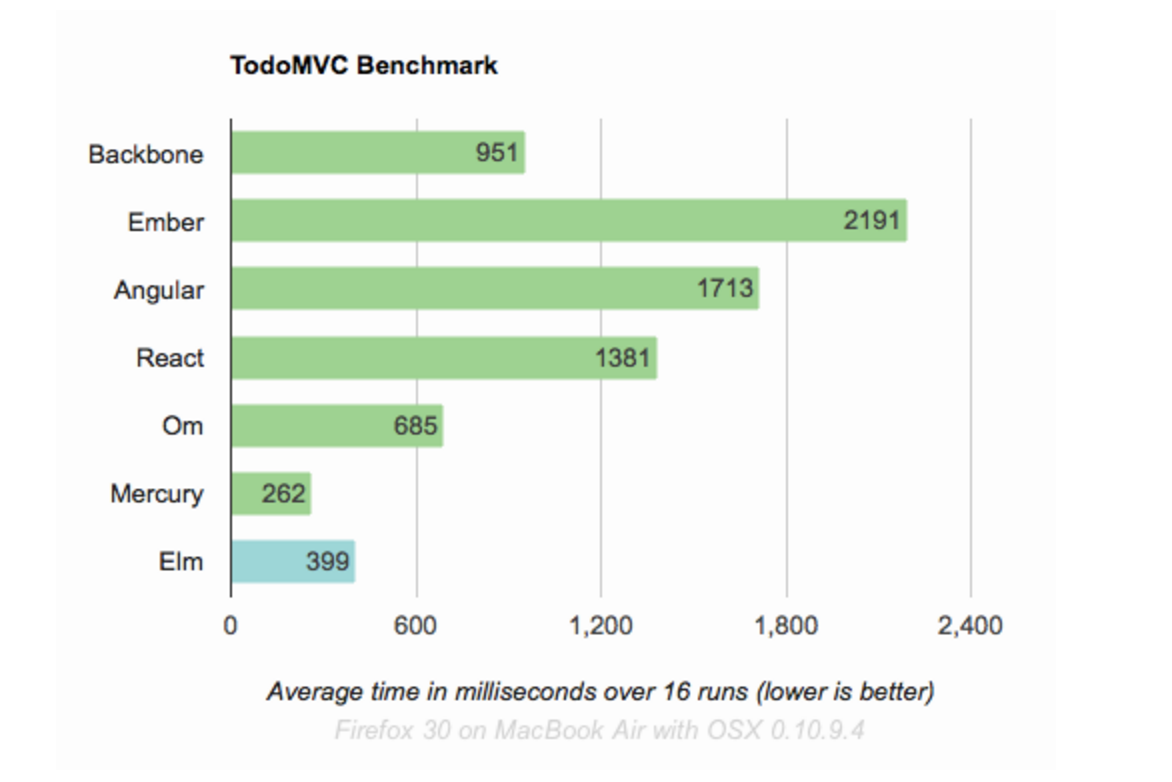
DEVELOPER EXPERIENCE
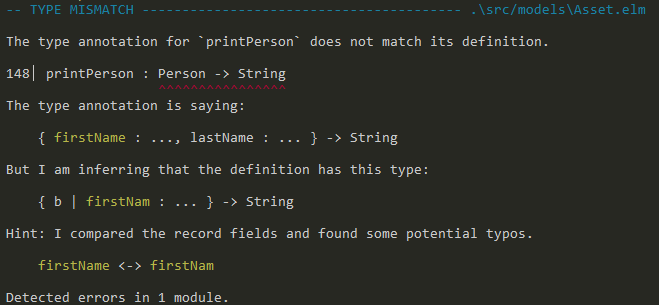
GOOD PARTS
- Inferred type system ( No runtime exceptions!)
- Stability - The compiler protects us from ourselves
- Immutable data structures
- Virtual DOM (so fast!)
- Compiles to JS or HTML/CSS/JS
- The Elm Architecture makes developing apps fun(ctional)
WHO IS USING ELM (IN PROD)
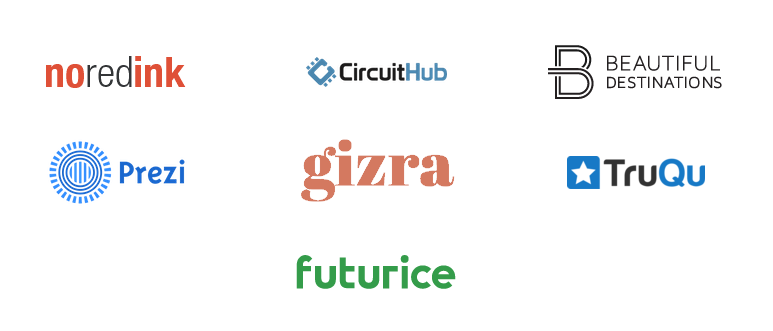
THANKS FOR YOUR ATTENTION
Compiled to JS languages
By Dima Pikulin
Compiled to JS languages
- 1,364