HTML ADVANCED
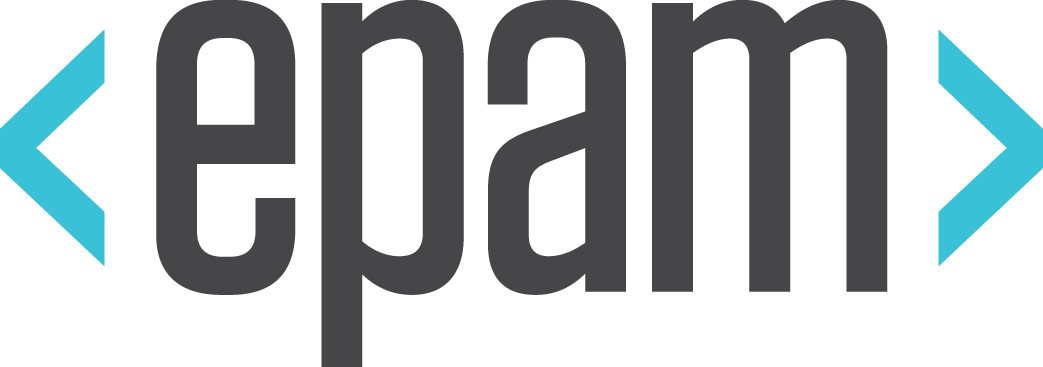
Forms
- HTML forms are used to collect user input
- HTML forms contain form elements
- Form elements are different types of input elements
- checkboxes
- radio buttons
- submit buttons
- and more.
The <form> element defines an HTML form:
<form>
<!-- form elements here -->
</form>
Note: The form itself is not visible, only its children
Form
Form - a set of fields for text entry, command buttons, check boxes, etc., whose contents are returned to the server as directed by the user. The server further processes the information and, if necessary, returns the user answers.
The form intended to exchange data between the user and the server. A document may contain any number of forms, but at the same time on the server can be sent to only one form.
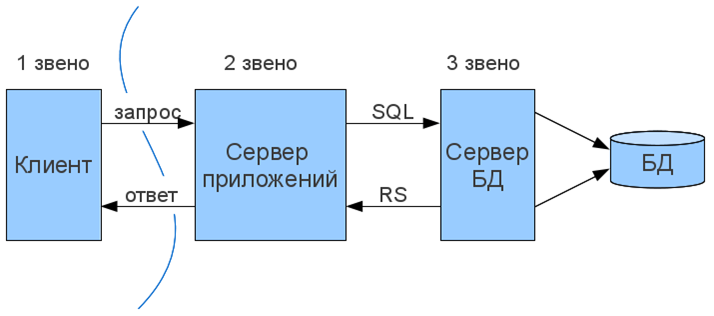
Action Attribute
- The action attribute defines the action to be performed when the form is submitted
- The common way to submit a form to a server, is by using a submit button
- Normally, the form is submitted to a web page on a web server
- If the action attribute is omitted, the action is set to the current page
Method Attribute
The method attribute specifies the HTTP method (GET or POST) to be used when submitting the forms:
<form action="action_page.php" method="get">
or
<form action="action_page.php" method="post">
When to Use GET?
You can use GET (the default method):
- If the form submission is passive (like a search engine query), and without sensitive information.
- When you use GET, the form data will be visible in the page address:
action_page.php?firstname=Mickey&lastname=Mouse
GET is best suited to short amounts of data. Size limitations are set in your browser.
When to Use POST?
You should use POST:
- If the form is updating data, or includes sensitive information (password).
- POST offers better security because the submitted data is not visible in the page address.
The Name Attribute
To be submitted correctly, each input field must have a name attribute.
This example will only submit the "Last name" input field:
HTML Form Attributes
An HTML <form> element, with all possible attributes set, will look like this:
<form action="action_page.php" method="GET" target="_blank" accept-charset="UTF-8"
enctype="application/x-www-form-urlencoded" autocomplete="off" novalidate>
<!-- form elements here -->
</form>
List of Form attributes
Attribute | Description |
---|---|
accept-charset | Specifies the charset used in the submitted form (default: the page charset). |
action | Specifies an address (url) where to submit the form (default: the submitting page). |
autocomplete | Specifies if the browser should autocomplete the form (default: on). |
enctype | Specifies the encoding of the submitted data (default: is url-encoded). |
method | Specifies the HTTP method used when submitting the form (default: GET). |
name | Specifies a name used to identify the form (for DOM usage: document.forms.name). |
novalidate | Specifies that the browser should not validate the form. |
target | Specifies the target of the address in the action attribute (default: _self). |
HTML Form Elements
Radio Button
<input type="radio"> defines a radio button
Radio buttons let a user select ONE of a limited number of choices:
Text Input
<input type="text"> defines a one-line input field for text input:
The default width of a text field is 20 characters.
<input> Element
The <input> element is the most important form element.
The <input> element has many variations, depending on the type attribute.
Type | Description |
---|---|
text | Defines normal text input |
radio | Defines radio button input (for selecting one of many choices) |
submit | Defines a submit button (for submitting the form) |
Submit Button
- Submit button defines a button for submitting a form to a form-handler
- The form-handler is typically a server page with a script for processing input data
- The form-handler is specified in the form's action attribute
Label
Used to change the value of the check boxes and radio buttons when you click the mouse on the text.
There are two methods of binding an object and labels:
- The first is to use the element of id within the form and specify its name as an attribute for a tag <label>
- In the second method, the form element is placed inside the container <label>
<select> Element
The <select> element defines a drop-down list:
- The <option> elements defines the options to select.
- The list will normally show the first item as selected.
- You can add a selected attribute to define a predefined option.
<textarea> Element
The <textarea> element defines a multi-line input field (a text area)
<button> Element
The <button> element defines a clickable button
HTML5 <datalist> Element
- The <datalist> element specifies a list of pre-defined options for an <input> element
- Users will see a drop-down list of pre-defined options as they input data
- The list attribute of the <input> element, must refer to the id attribute of the <datalist> element
Grouping Form Data
The <fieldset> element groups related data in a form.
The <legend> element defines a caption for the <fieldset> element.
HTML Input Attributes
value Attribute
The value attribute specifies the initial value for an input field:
readonly Attribute
The readonly attribute specifies that the input field is read only (cannot be changed):
disabled Attribute
- The disabled attribute specifies that the input field is disabled.
- A disabled element is un-usable and un-clickable.
- Disabled elements will not be submitted.
size Attribute
The size attribute specifies the size (in characters) for the input field:
maxlength Attribute
With a maxlength attribute, the input control will not accept more than the allowed number of characters.
The maxlength attribute specifies the maximum allowed length for the input field:
HTML5 Attributes
HTML5 added the following attributes for <input>:
- autocomplete
- autofocus
- form
- formaction
- formenctype
- formmethod
- formnovalidate
- formtarget
- height and width
- list
- min and max
- multiple
- pattern (regexp)
- placeholder
- required
- step
- and the following attributes for <form>:
- autocomplete
- novalidate
autocomplete Attribute
- The autocomplete attribute specifies whether a form or input field should have autocomplete on or off.
- When autocomplete is on, the browser automatically complete values based on values that the user has entered before.
- It is possible to have autocomplete "on" for the form, and "off" for specific input fields, or vice versa.
- The autocomplete attribute works with <form> and the following <input> types: text, search, url, tel, email, password, datepickers, range, and color.
novalidate
The novalidate attribute is a <form> attribute.
When present, novalidate specifies that form data should not be validated when submitted.
autofocus
The autofocus attribute is a boolean attribute.
When present, it specifies that an <input> element should automatically get focus when the page loads.
form Attribute
- The form attribute specifies one or more forms an <input> element belongs to.
- To refer to more than one form, use a space-separated list of form ids.
formaction
- The formaction attribute specifies the URL of a file that will process the input control when the form is submitted.
- The formaction attribute overrides the action attribute of the <form> element.
- The formaction attribute is used with type="submit" and type="image".
formenctype
- The formenctype attribute specifies how the form-data should be encoded when submitting it to the server (only for forms with method="post").
- The formenctype attribute overrides the enctype attribute of the <form> element.
- The formenctype attribute is used with type="submit" and type="image".
formmethod
- The formmethod attribute defines the HTTP method for sending form-data to the action URL.
- The formmethod attribute overrides the method attribute of the <form> element.
- The formmethod attribute can be used with type="submit" and type="image".
formnovalidate
- The novalidate attribute is a boolean attribute.
- When present, it specifies that the <input> element should not be validated when submitted.
- The formnovalidate attribute overrides the novalidate attribute of the <form> element.
- The formnovalidate attribute can be used with type="submit".
formtarget
- The formtarget attribute specifies a name or a keyword that indicates where to display the response that is received after submitting the form.
- The formtarget attribute overrides the target attribute of the <form> element.
- The formtarget attribute can be used with type="submit" and type="image".
height and width
The height and width attributes specify the height and width of an <input> element.
The height and width attributes are only used with <input type="image">.
list Attribute
The list attribute refers to a <datalist> element that contains pre-defined options for an <input> element.
min and max
- The min and max attributes specify the minimum and maximum value for an <input> element.
- The min and max attributes work with the following input types: number, range, date, datetime, datetime-local, month, time and week.
multiple
- The multiple attribute is a boolean attribute.
- When present, it specifies that the user is allowed to enter more than one value in the <input> element.
- The multiple attribute works with the following input types: email, and file.
pattern
- The pattern attribute specifies a regular expression that the <input> element's value is checked against.
- The pattern attribute works with the following input types: text, search, url, tel, email, and password.
- Tip: Use the global title attribute to describe the pattern to help the user.
placeholder
- The placeholder attribute specifies a hint that describes the expected value of an input field (a sample value or a short description of the format).
- The hint is displayed in the input field before the user enters a value.
- The placeholder attribute works with the following input types: text, search, url, tel, email, and password.
required
- The required attribute is a boolean attribute.
- When present, it specifies that an input field must be filled out before submitting the form.
- The required attribute works with the following input types: text, search, url, tel, email, password, date pickers, number, checkbox, radio, and file.
step Attribute
- The step attribute specifies the legal number intervals for an <input> element.
- The step attribute works with the following input types: number, range, date, datetime, datetime-local, month, time and week.
- Tip: The step attribute can be used together with the max and min attributes to create a range of legal values.
httpbin.org
HTTP Request & Response Service
IDE
Integrated development environment
- An integrated development environment (IDE) is a software application that provides comprehensive facilities to computer programmers for software development.
- An IDE normally consists of
- source code editor
- build automation tools and
- debugger.
- Most modern IDEs have intelligent code completion.
Notepad++
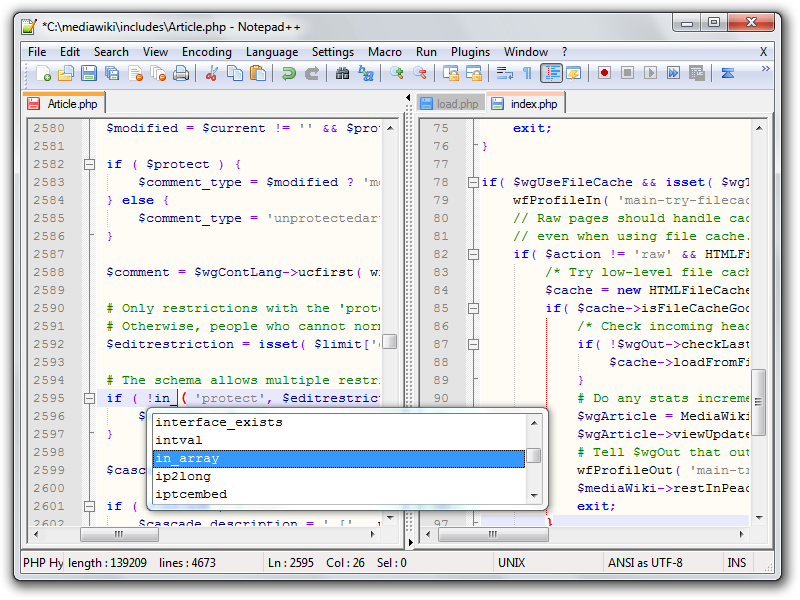
- Notepad++ is a text editor and source code editor for use with Microsoft Windows.
- Unlike Notepad, the built-in Windows text editor, it supports tabbed editing, which allows working with multiple open files in a single window.
- It is free
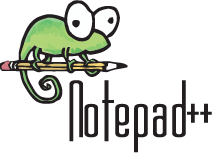
Eclipse
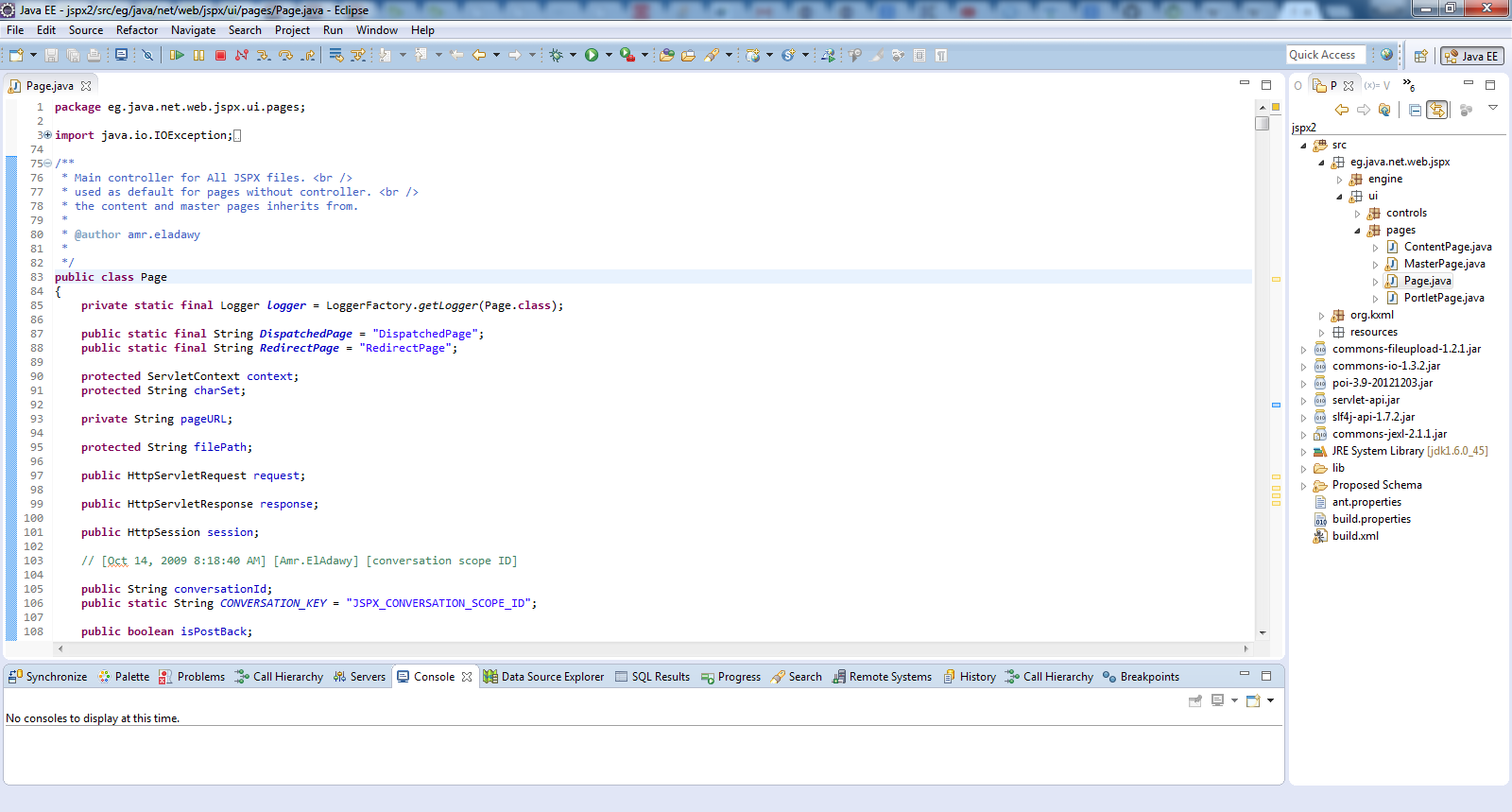
It contains a base workspace and an extensible plug-in system for customizing the environment.
Eclipse is written mostly in Java and its primary use is for developing Java applications, but it may also be used to develop applications in other programming languages through the use of plugins
Visual Studio Code
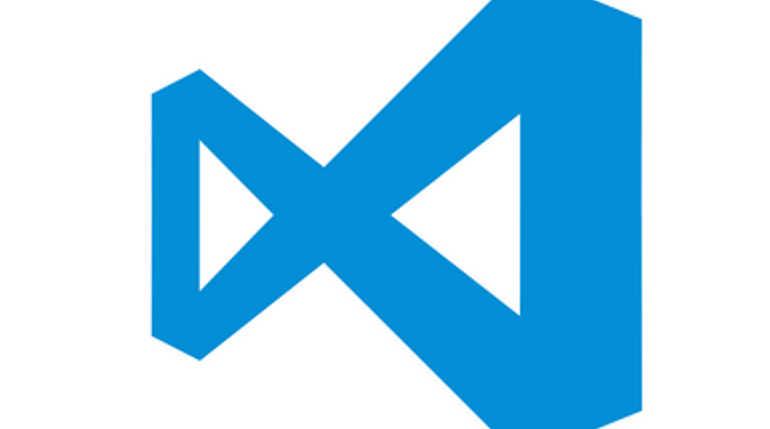
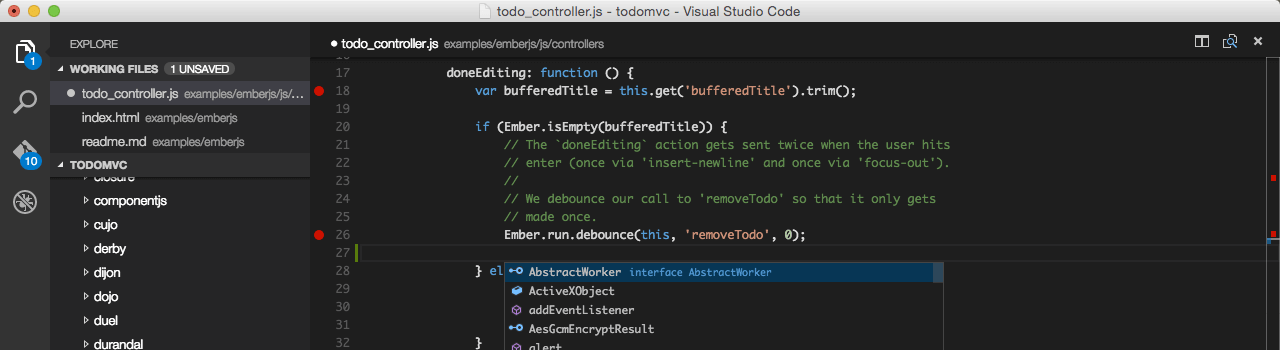
- Cross platform: OS X, Linux, and Windows
- Language coverage 30+
- Code-focused editing
- Code navigation
- IntelliSense, linting, refactoring
- Debugging
Sublime Text
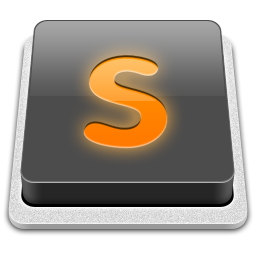
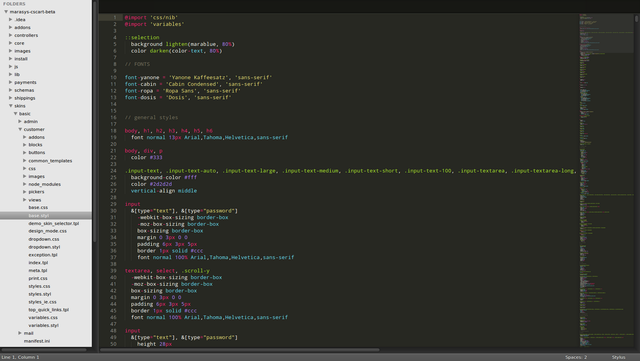
- "Goto Anything," quick navigation to files, symbols, or lines
- "Command palette" uses adaptive matching for quick keyboard invocation of arbitrary commands
- Simultaneous editing: simultaneously make the same interactive changes to multiple selected areas
- Extensive customizability
- Cross platform (Windows, OS X, Linux)
WebStorm
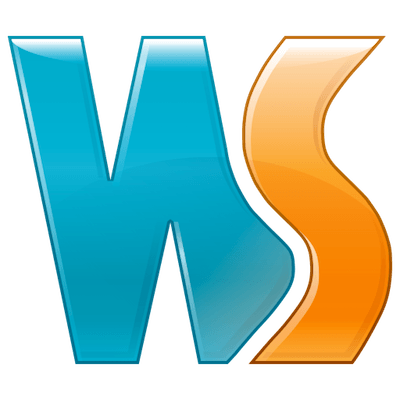
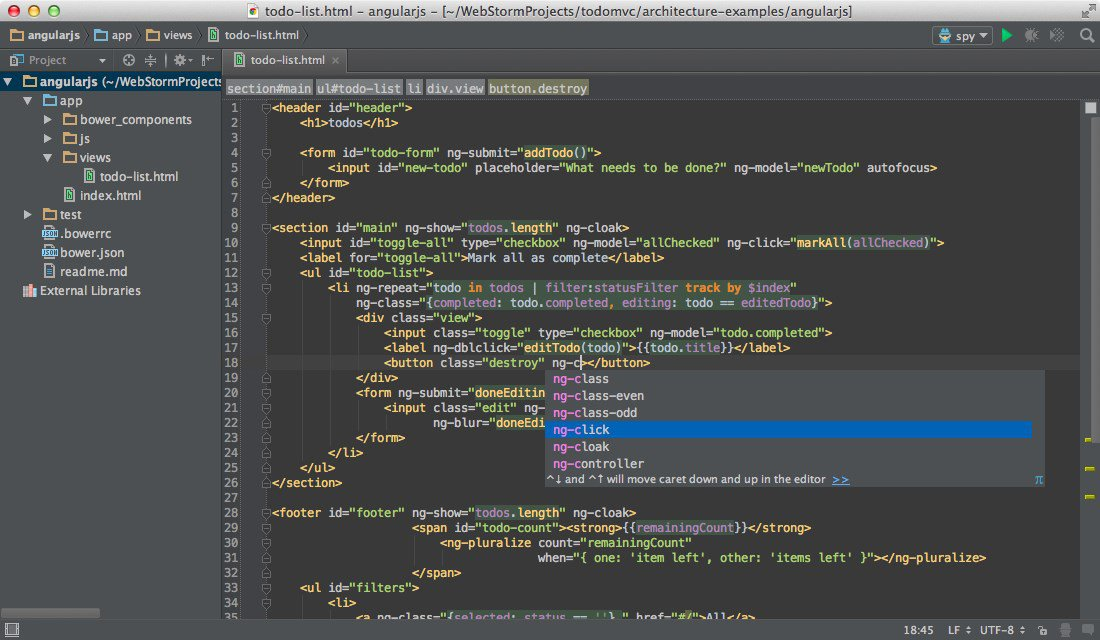
- Support many frameworks, Web Components
- Excellent intellisense
- JavaScript Debugger
- Support for multiple web scripting languages
- Version Control Integrations: Subversion, Git, GitHub...
- Issue tracker integration
- It is not free
Brackets
- Free open-source editor written in HTML, CSS, and JavaScript
- Primary focus on Web Development
- Lightweight
- It was created by Adobe Systems,
- Brackets is available for cross-platform
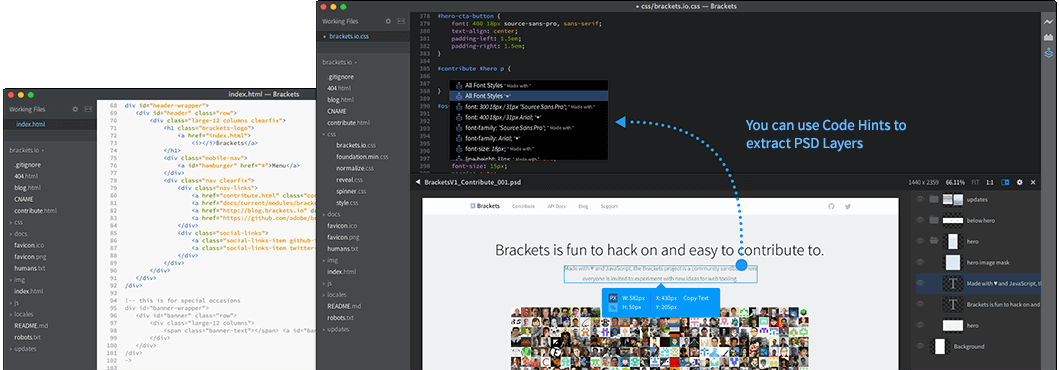
WHAT WE'VE LEARNT
- Form
- Form methods
- Form attributes
- Form elements
- Input
- IDE
CLASS WORK
Create this page
- Country of Residence should be a dropdown list
- Age must be from 18 years to 101
- Password must be required
Save image to get better quality
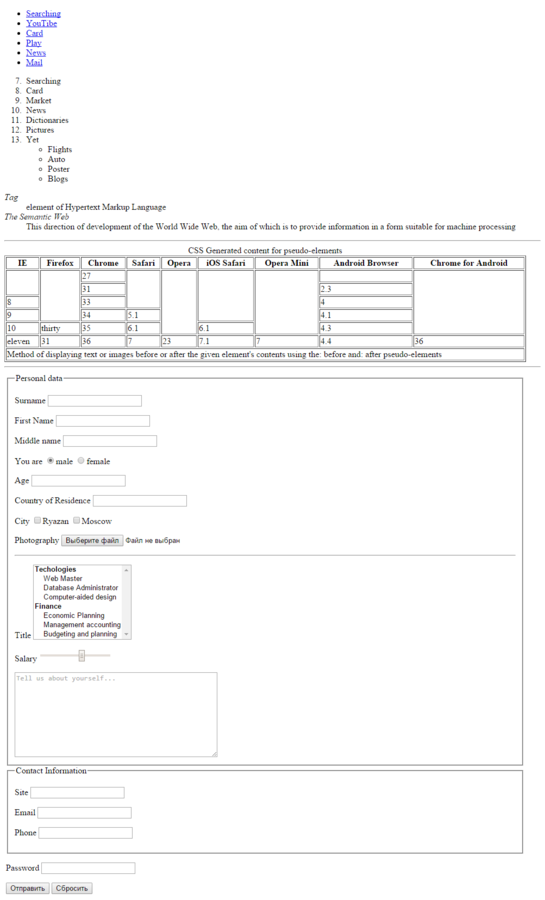
HOME WORK
- Each task should be done in its own HTML file
- Each task should start with the first level header
- All the forms must use http://httpbin.org/ appropriate endpoint
- All the form's elements must be accepted by the endpoint
- Your document must be valid
Task 1
Registration form
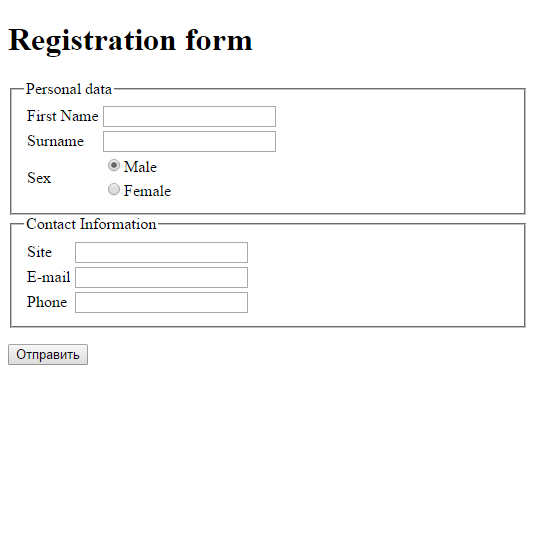
- Create this form
- Use browser validation
- Use http://httpbin.org/
- Create two variants: one should use get method, the other should use post method
Task 2
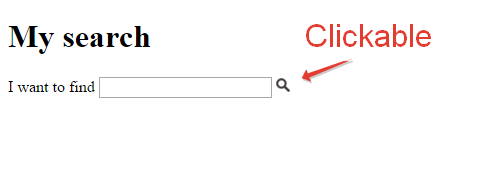
Searching
- Create simple form
- You don't need to use http://httpbin.org/ in this task
- When user type something in the field and click the search icon the yandex website is opened with the results of the search
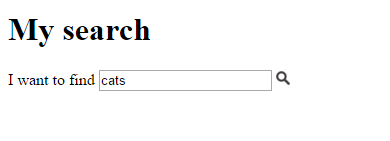
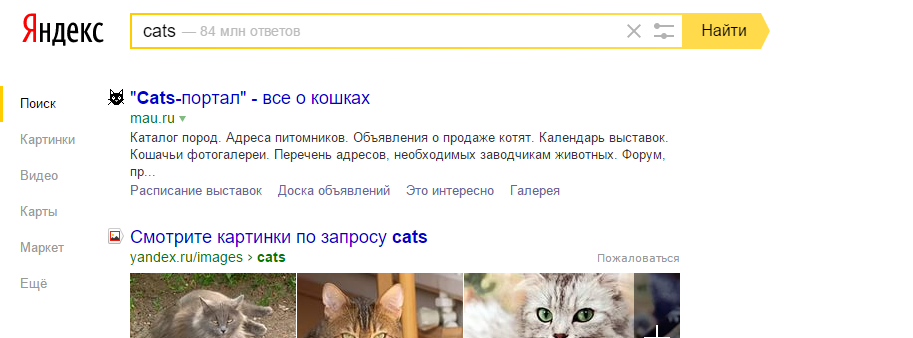
Task 3
Form
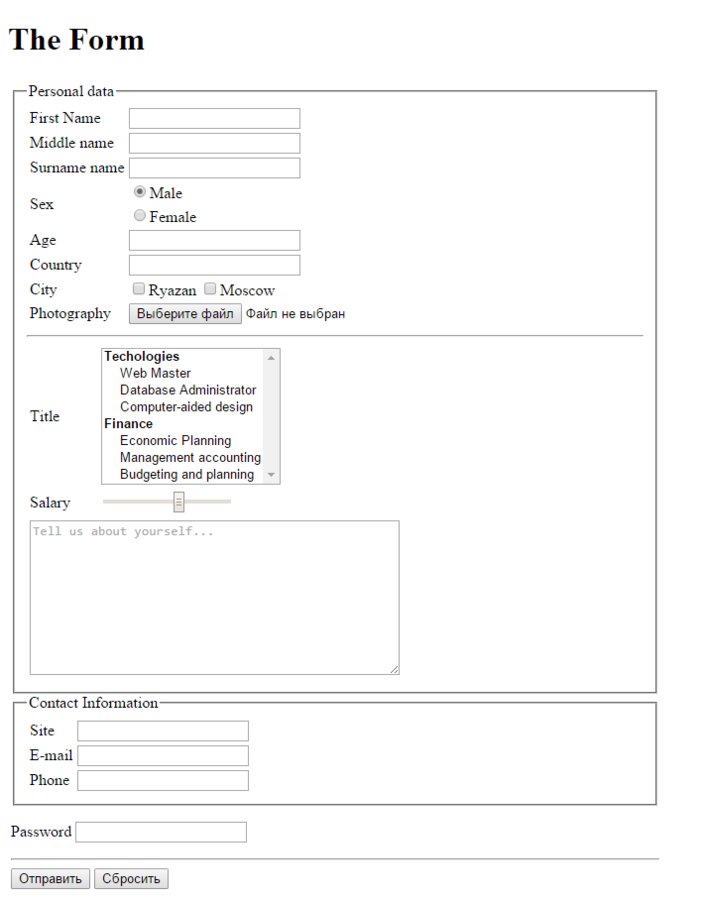
- Create this form in two variants: get and post methods
- Use browser validation
Use http://httpbin.org/ - The "Reset" button should clear the form
- Pay attention to the layout
Save image to get better quality
THANKS FOR YOUR ATTENTION
HTML ADVANCED
HTML Advanced
By Dima Pikulin
HTML Advanced
- 1,195