DOM
Document Object Model
Where JavaScript and HTML+CSS join forces
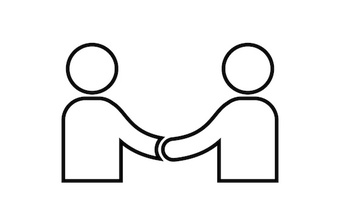
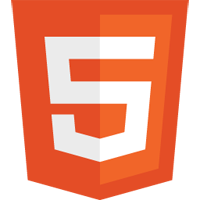
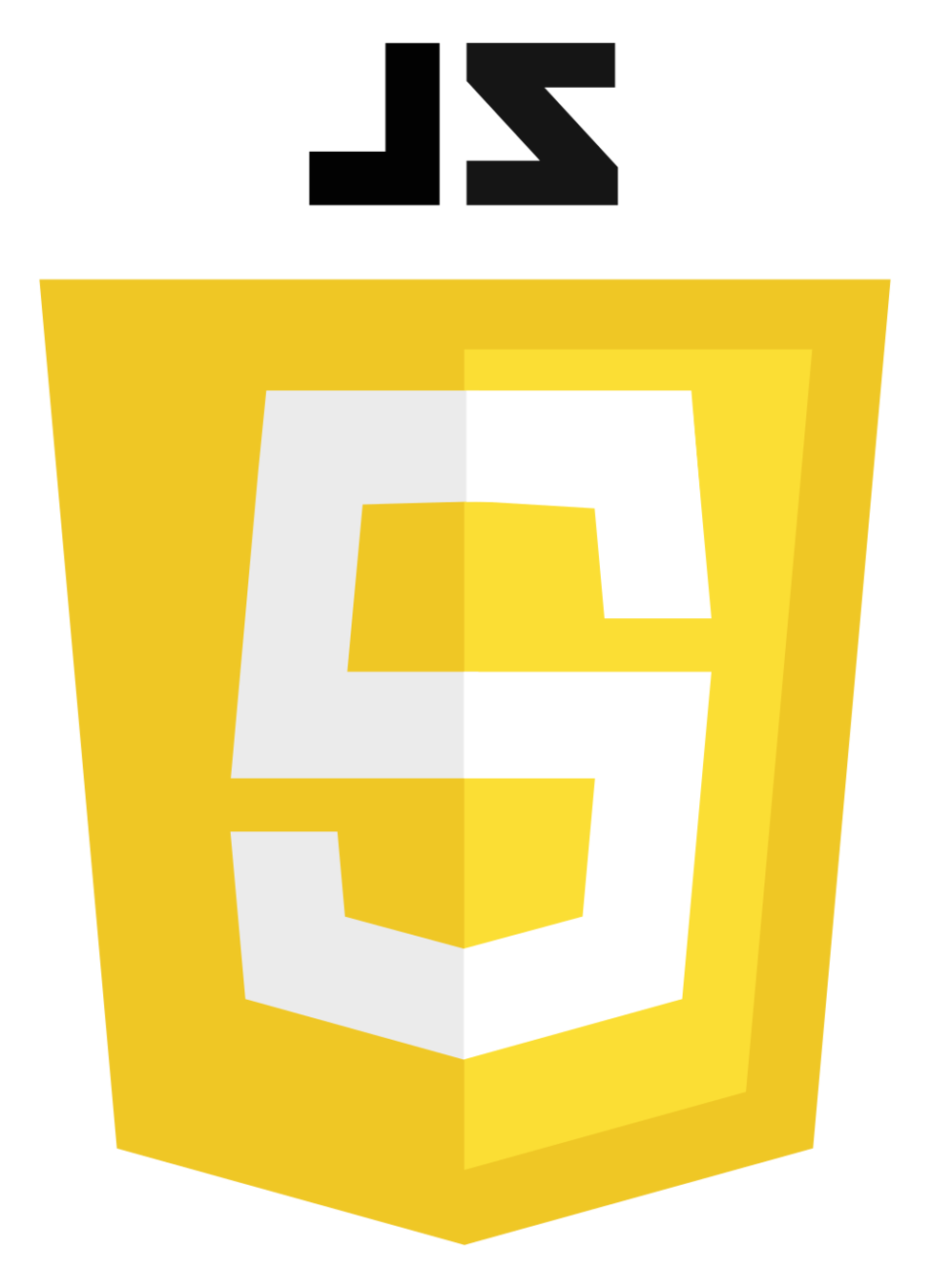
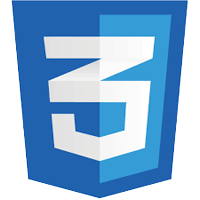
Week 9
DOM
Set of JavaScript objects and functions that allows us to manipulate a HTML page
How Browser Loads WebPage
1. Receives page as HTML
<!DOCTYPE html>
<html>
<head>
<title>Hotel Home Page</title>
<link rel="stylesheet" type="text/css" href="homepage.css">
</head>
<body>
<h1>Rooms Available:</h1>
<div>
<p>Note the following rooms are estimates:<p>
<h3>Rooms: <em>15</em></h3>
</div>
</body>
</html>
How Browser Loads WebPage
2. Browser Creates Model of Webpage
document
<html>
<head>
<body>
<title>
<link>
Hotel Home
Page
rel="stylesheet" href="homepage.css"
<h1>
<div>
Rooms Available
<p>
<h3>
Rooms:
<em>
Note the following...
15
<!DOCTYPE html>
<html>
<head>
<title>Hotel Home Page</title>
<link rel="stylesheet" type="text/css" href="homepage.css">
</head>
<body>
<h1>Rooms Available:</h1>
<div>
<p>Note the following rooms are estimates:<p>
<h3>Rooms: <em>15</em></h3>
</div>
</body>
</html>
Each HTML tag is turned into a javascript object
How Browser Loads WebPage
2. Browser Creates Model of Webpage
document
<html>
<head>
<body>
<title>
<link>
Hotel Home
Page
rel="stylesheet" href="homepage.css"
<h1>
<div>
Rooms Available
<p>
<h3>
Rooms:
<em>
Note the following...
15
<!DOCTYPE html>
<html>
<head>
<title>Hotel Home Page</title>
<link rel="stylesheet" type="text/css" href="homepage.css">
</head>
<body>
<h1>Rooms Available:</h1>
<div>
<p>Note the following rooms are estimates:<p>
<h3>Rooms: <em>15</em></h3>
</div>
</body>
</html>
Everything is stored inside of the document object
How Browser Loads WebPage
3. Browser displays page using Rendering Engine
<!DOCTYPE html>
<html>
<head>
<title>Hotel Home Page</title>
<link rel="stylesheet" type="text/css" href="homepage.css">
</head>
<body>
<h1>Rooms Available:</h1>
<div>
<p>Note the following rooms are estimates:<p>
<h3>Rooms: <em>15</em></h3>
</div>
</body>
</html>
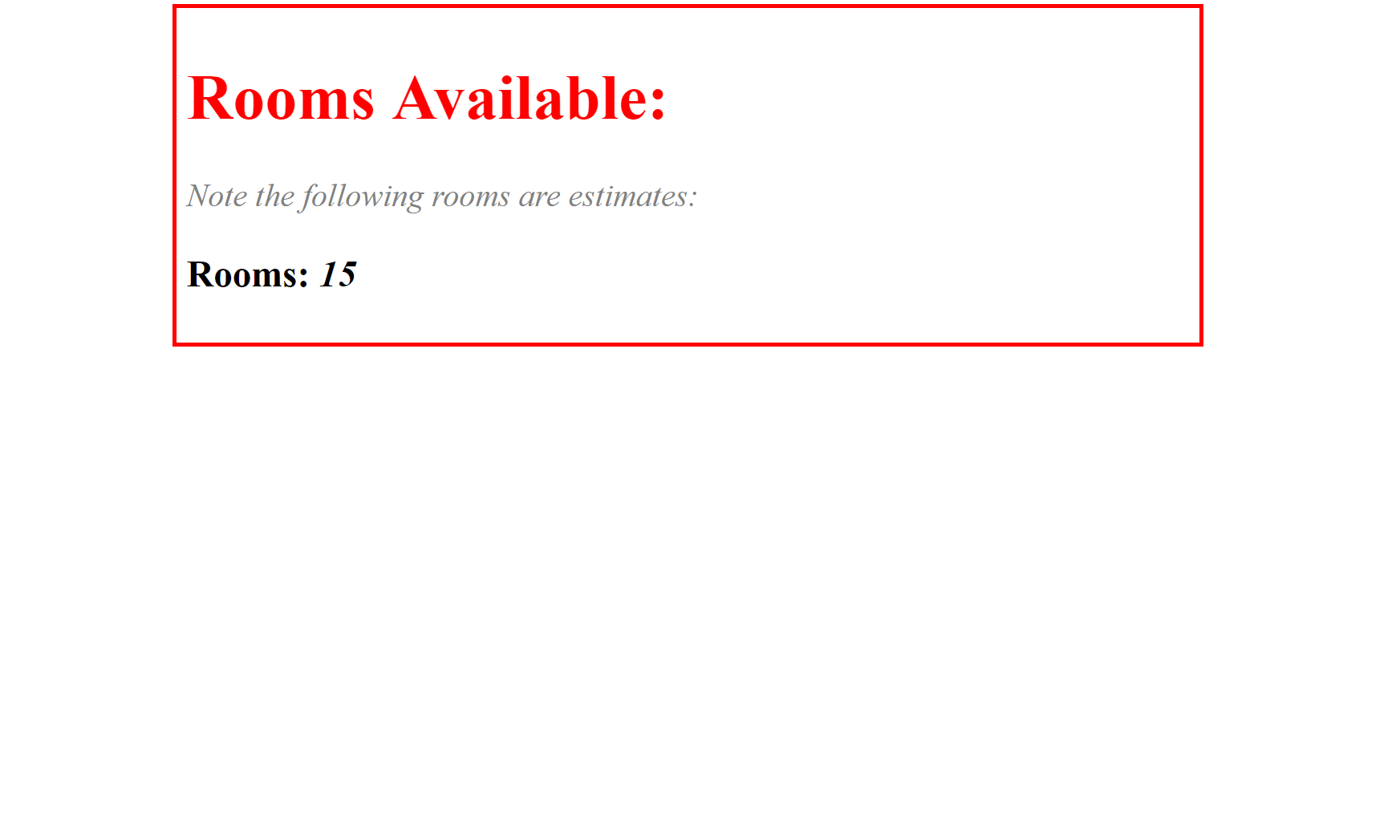
How Browser Loads WebPage
<!DOCTYPE html>
<html>
<head>
<title>Hotel Home Page</title>
<link rel="stylesheet" type="text/css" href="homepage.css">
</head>
<body>
<h1>Rooms Available:</h1>
<div>
<p>Note the following rooms are estimates:<p>
<h3>Rooms: <em>15</em></h3>
</div>
</body>
</html>
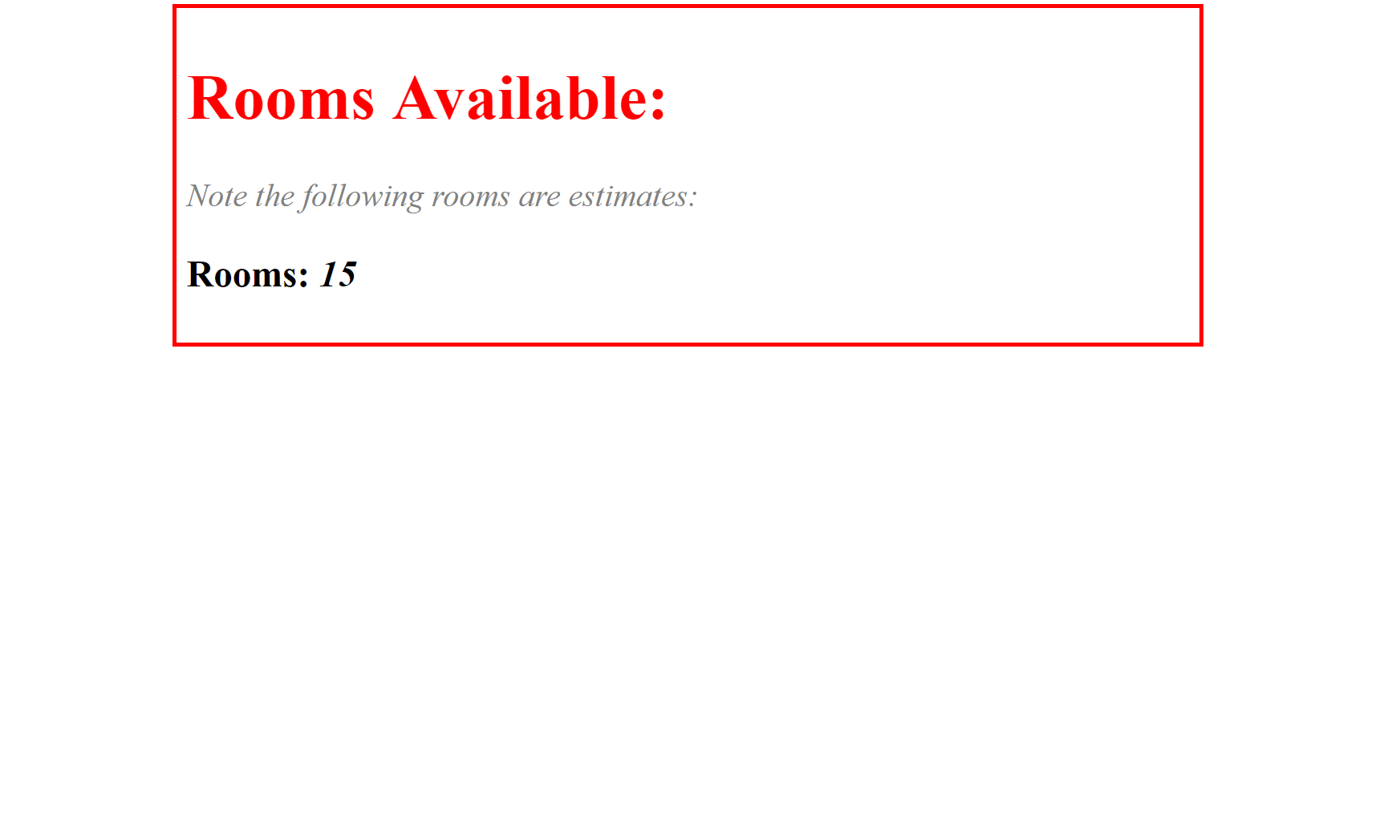
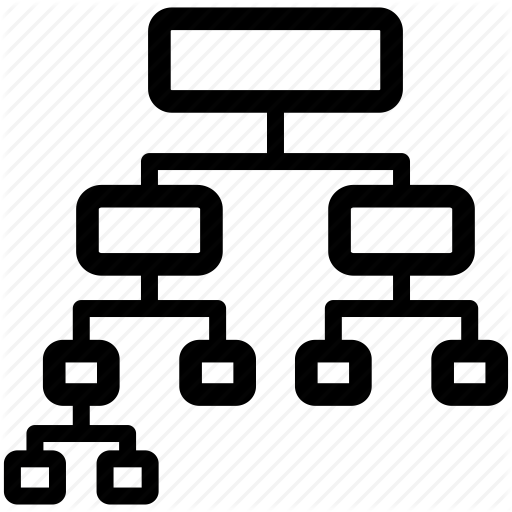
1
2
3
Receive HTML
Create Model
Render Page
Object Properties and Methods
var hotel =
{
name: "City Hotel",
numRooms: 100,
availRooms: 30,
numberBookedRooms: function()
{
return numRooms - availRooms;
}
};
Properties
}
Property is a variable within an object
Method is a function within an object
Method
}
hotel object
var name = "City Hotel"
var numRoom = 100
var availRooms = 30
function numBookedRooms()
{
return numRooms - availRooms;
}
hotel.
hotel.name
hotel.numRoom
hotel.numBookedRooms()
City Hotel
100
70
document Object
document is the name of an object in JavaScript
//title of current document:
document.title;
//Date which document was last modified:
document.lastModified;
//URL of current document
document.URL;
//Domain of current document
document.domain;
Properties in the document object:
Open Chrome JavaScript Console and try these (and others)
Ctrl+Shift+J
document
<html>
<head>
<body>
<title>
<link>
Hotel Home
Page
rel="stylesheet" href="homepage.css"
<h1>
<div>
Rooms Available
<p>
<h3>
Rooms:
<em>
Note the following...
15
document._______
We can get access to webpage content via the document object
document Object
document is the name of an object in JavaScript
//returns element with the value of the Id attribute:
document.getElementById();
//Writes text to document:
document.write();
//Creates new HTML element
document.createElement();
Methods/Functions in the document object:
JavaScript + HTML
-
Select an HTML Element
-
Manipulate that HTML Element
1. Select an HTML Element
var h1 = document.querySelector("h1");
Use document object methods to select specific elements in HTML
<!DOCTYPE html>
<html>
<head>
<title>CSS Demo</title>
<link rel="stylesheet" type="text/css" href="myfirststyle.css">
</head>
<body>
<h1>Heading here</h1>
<h4>Sub-heading here</h4>
</body>
</html>
2. Manipulate the HTML Element
var h1 = document.querySelector("h1");
h1.style.color = "blue";
Manipulate by changing values of properties in the h1 object that was returned
<!DOCTYPE html>
<html>
<head>
<title>CSS Demo</title>
<link rel="stylesheet" type="text/css" href="myfirststyle.css">
</head>
<body>
<h1>Heading here</h1>
<h4>Sub-heading here</h4>
</body>
</html>
1. Open Repl
2. Run Repl
3. Open Repl in new Tab
4. Open Chrome JS Console (Ctrl+Shift+J)
5. Change h1 to blue
6. Change h2 to red
7. Change all h3's to yellow
JavaScript + HTML
-
Select an HTML Element
-
Manipulate that HTML Element
Selecting Elements
document.getElementById();
document.getElementsByClassName();
document.getElementsByTagName();
document.querySelector();
document.querySelectorAll();
document object has number of methods to select elements.
We will look at 5 of the most common ones:
<html>
<body>
<div id="page">
<h1 id="header">List</h1>
<h2>Buy groceries</h2>
<ul>
<li id="one" class="hot"><em>Fresh</em> pasta</li>
<li id="two" class="hot">Bread</li>
<li id="three" class="hot">Cooked chicken</li>
<li id="four">Olive oil</li>
</ul>
</div>
</body>
</html>
- Go to the getElement repl
- Run the code
- Open in new tab
- Open JavaScript Console on that page (Ctrl+Shift+J)
- Keep that tab open
Prep Work
<html>
<body>
<div id="page">
<h1 id="header">List</h1>
<h2>Buy groceries</h2>
<ul>
<li id="one" class="hot"><em>Fresh</em> pasta</li>
<li id="two" class="hot">Bread</li>
<li id="three" class="hot">Cooked chicken</li>
<li id="four">Olive oil</li>
</ul>
</div>
</body>
</html>
getElementById()
Takes Id as input and returns element with matching Id
var myOneTag = document.getElementById("one");
<html>
<body>
<div id="page">
<h1 id="header">List</h1>
<h2>Buy groceries</h2>
<ul>
<li id="one" class="hot"><em>Fresh</em> pasta</li>
<li id="two" class="hot">Bread</li>
<li id="three" class="hot">Cooked chicken</li>
<li id="four">Olive oil</li>
</ul>
</div>
</body>
</html>
getElementsByClassName()
Takes Class Name as input and returns list of elements with matching Class Name
var hotItems = document.getElementsByClassName("hot");
hotItems.length;// 3
hotItems[0];//<li id="one" class="hot"><em>Fresh</em> pasta</li>
[0]
[1]
[2]
<li id="three" class="hot">Cooked chicken</li>
<li id="one" class="hot"><em>Fresh</em> pasta</li>
<li id="two" class="hot">Bread</li>
[0]
[1]
[2]
<html>
<body>
<div id="page">
<h1 id="header">List</h1>
<h2>Buy groceries</h2>
<ul>
<li id="one" class="hot"><em>Fresh</em> pasta</li>
<li id="two" class="hot">Bread</li>
<li id="three" class="hot">Cooked chicken</li>
<li id="four">Olive oil</li>
</ul>
</div>
</body>
</html>
getElementsByTagName()
Takes Tag Name as input and returns list of elements with matching Tag Name
var liItems = document.getElementsByTagName("li");
liItems.length;// 4
liItems[3];//<li id="four">Olive oil</li>
[0]
[1]
[2]
[3]
<html>
<body>
<div id="page">
<h1 id="header">List</h1>
<h2>Buy groceries</h2>
<ul>
<li id="one" class="hot"><em>Fresh</em> pasta</li>
<li id="two" class="hot">Bread</li>
<li id="three" class="hot">Cooked chicken</li>
<li id="four">Olive oil</li>
</ul>
</div>
</body>
</html>
getElementsByTagName()
Takes Tag Name as input and returns list of elements with matching Tag Name
var bodyItems = document.getElementsByTagName("body");
liItems.length;// 1
liItems[0];//<body>...</body>
[0]
<html>
<body>
<div id="page">
<h1 id="header">List</h1>
<h2>Buy groceries</h2>
<ul>
<li id="one" class="hot"><em>Fresh</em> pasta</li>
<li id="two" class="hot">Bread</li>
<li id="three" class="hot">Cooked chicken</li>
<li id="four">Olive oil</li>
</ul>
</div>
</body>
</html>
querySelector()
Takes CSS style selector and return first element that matches the CSS selector
var tag = document.querySelector("#four");
<html>
<body>
<div id="page">
<h1 id="header">List</h1>
<h2>Buy groceries</h2>
<ul>
<li id="one" class="hot"><em>Fresh</em> pasta</li>
<li id="two" class="hot">Bread</li>
<li id="three" class="hot">Cooked chicken</li>
<li id="four">Olive oil</li>
</ul>
</div>
</body>
</html>
querySelector()
Takes CSS style selector and return first element that matches the CSS selector
var tag = document.querySelector(".hot");
<html>
<body>
<div id="page">
<h1 id="header">List</h1>
<h2>Buy groceries</h2>
<ul>
<li id="one" class="hot"><em>Fresh</em> pasta</li>
<li id="two" class="hot">Bread</li>
<li id="three" class="hot">Cooked chicken</li>
<li id="four">Olive oil</li>
</ul>
</div>
</body>
</html>
querySelector()
Takes CSS style selector and return first element that matches the CSS selector
var tag = document.querySelector("h1");
<html>
<body>
<div id="page">
<h1 id="header">List</h1>
<h2>Buy groceries</h2>
<ul>
<li id="one" class="hot"><em>Fresh</em> pasta</li>
<li id="two" class="hot">Bread</li>
<li id="three" class="hot">Cooked chicken</li>
<li id="four">Olive oil</li>
</ul>
</div>
</body>
</html>
querySelectorAll()
Takes CSS style selector and return list of all elements that matches the CSS selector
var tag = document.querySelectorAll("li");
[0]
[1]
[2]
[3]
tag.length;// 4
tag[0];//<li id="one" class="hot"><em>Fresh</em> pasta</li>
<html>
<body>
<div id="page">
<h1 id="header">List</h1>
<h2>Buy groceries</h2>
<ul>
<li id="one" class="hot"><em>Fresh</em> pasta</li>
<li id="two" class="hot">Bread</li>
<li id="three" class="hot">Cooked chicken</li>
<li id="four">Olive oil</li>
</ul>
</div>
</body>
</html>
querySelectorAll()
Takes CSS style selector and return list of all elements that matches the CSS selector
var tag = document.querySelectorAll(".bolded");
[0]
[1]
[2]
[3]
tag.length;// 3
tag[0];//<li id="one" class="hot"><em>Fresh</em> pasta</li>
<html>
<head>
<title>Page Title Here</title>
</head>
<body>
<div id="sidebar">
<ul>
<li id="pg1"><a class="special" href="#">Section 1</a></li>
<li id="pg2"><a href="#">Section 2</a></li>
<li id="pg3"><a href="#">Section 3</a></li>
<li id="pg4"><a href="#">Section 4</a></li>
</ul>
</div>
<div id="main">
<h1>Heading goes here</h1>
<p id="start" class="important">Introduction text goes here</p>
<p class="important">Contact info here: <a class="special" href="#">Contact US</a></p>
<p>About our location here</p>
<p id="end">Googbye here</p>
</div>
</body>
</html>
- Go to the repl getElement_exercise
- Run the code
- Open in new tab
- Open JavaScript Console on that page (Ctrl+Shift+J)
- Keep that tab open
Excercise
Prep
- Select paragraph with id="start"
- Select all li items in the sidebar div
- Select first a tag with specal class
- Select all tags with important class
Questions:
Selector | Description |
---|---|
h1 | element selector |
.special | class selector |
#pg1 | id selector |
ul li | descendent selector: chains together selectors (e.g. li's inside ul) |
document.querySelector(" ")
or
document.querySelectorAll(" ")
JavaScript + HTML
-
Select an HTML Element
-
Manipulate that HTML Element
Manipulating Elements
-
Style
-
Classes
-
Content
-
Attributes
We will Edit the following parts of an Element:
Manipulating Style
//Select an element
var myTag = document.getElementById("start");
//Manipulate Element
myTag.style.color = "green";
myTag.style.border = "4px solid blue";
myTag.style.fontsize = "50px";
myTag.style.background = "grey";
myTag.style.marginTop = "200px";
//Set style using same values as CSS
//Only difference is values go inside " "
style property lets us manipulate style in same manner we would in CSS
myTag
innerText
"Introduction goes here"
style
color
"green"
border
"4px solid blue"
fontsize
"50px"
background
"grey"
function getInnerText()
{
return innerText;
}
Manipulating Style
//Select an element
var myTag = document.querySelector("h1");
//Manipulate Element
myTag.style.color = "red";
myTag.style.border = "2px solid red";
CSS should handle defining styles
JavaScript should handle turning on and off styles
We can do this:
...however it is not good practice.
Manipulating Style
We can instead define a class in CSS (as we have done before):
.headingOn
{
color: red;
border: 2px red solid;
}
We can then dynamically enable or disable the above style in JavaScript
var myTag = document.querySelector("h1");
//Enable It
myTag.classList.add("headingOn");
//Disable It
myTag.classList.remove("headingOn");
//If its on, turn it off. If its off, turn it on
myTag.classList.toggle("headingOn");
Manipulating Elements
-
Style
-
Classes
-
Content
-
Attributes
We will Edit the following parts of an Element:
Manipulating Content
textContent :
-
Property of an element object
-
Stores a string of all the text contained in the element
myTag
textContent
"Introduction goes here"
style
color
"green"
border
"4px solid blue"
fontsize
"50px"
background
"grey"
function getInnerText()
{
return innerText;
}
Manipulating Content
<h1>My Heading goes <em>here</em></h1>
//Select h1 element
var myH1Tag = document.querySelector("h1");
//get the contents of the tag
var h1Text = myH1Tag.textContent; //"My Heading goes here"
//set the contents of the tag
myH1Tag.textContent = "Updated Heading here";
Manipulating Content
- Open JavaScript Console (Ctrl+Shift+J)
- Select p using querySelectorAll
- Display the text content of the first p in the console
- Change the content of the second p
-
Select h1 using querySelector
- Display the text content in the console
- Change the heading in javascript
- Notice, however that the <strong> tag has now been removed
- Open replit: DOM_content1
- Run replit and then open in new tab
3.
.textContent
Manipulating Content
innerHTML :
-
Property of an element object
-
Similar to textContent, however it stores string of all HTML contained within an element
myTag
innerText
"Introduction goes here"
innerHTML
function getInnerText()
{
return innerText;
}
"<div>
<h1>Introduction goes here</h1>
</div>"
Manipulating Content
<h1>Heading goes <strong>here</strong></h1>
//Select h1 element
var myH1Tag = document.querySelector("h1");
//get the text contents of the tag
var h1Text = myH1Tag.textContent;
//get the HTML contents of the tag
var h1HTML = myH1Tag.innerHTML;
"My Heading goes here"
"My Heading goes <strong>here</strong>"
Manipulating Content
- Open JavaScript Console (Ctrl+Shift+J)
-
Select h1 using querySelector
- Display the html content in the console
-
Select ul using querySelectorAll
- Display the html content in the console
3.
- Open replit: DOM_content1
- Run replit and then open in new tab
.innerHTML
Manipulating Elements
-
Style
-
Classes
-
Content
-
Attributes
We will Edit the following parts of an Element:
HTML Attributes
Adding additional info to tags
<tag name="value"></tag>
<a href="www.google.com">Click here to go to google</a>
<p class="myclass">Paragraph text goes</p>
Manipulating Attributes
getAttribute() :
-
Method of an element object
-
Takes as input:
-
name of an attribute
-
-
Returns the value of that attribute
setAttribute() :
-
Method of an element object
-
Takes as input:
-
name of an attribute
-
new value
-
-
Sets the value of the attribute to the specified value
Manipulating Attributes
getAttribute() :
setAttribute() :
myTag
innerText
"Introduction goes here"
function setAttribute(x,y)
{
...
xAttr = y
}
function getAttribute(x)
{
...
return xAttr;
}
Manipulating Attributes
<a href="www.ulster.ac.uk">Ulster Homepage</a>
<img src="ulsterLogo.png">
Attributes
Manipulating Attributes
<a href="www.ulster.ac.uk">Ulster Homepage</a>
<img src="ulsterLogo.png">
//Select Element
var aLink = document.querySelector("a");
...Try it on sample project
//Get href attribute of <a> tag
var attrValue = aLink.getAttribute("href");//"www.ulster.ac.uk"
//Set the href attribute of the <a> tag
aLink.setAttribute("href","www.google.com");
Manipulating Elements
-
Style
-
Classes
-
Content
-
Attributes
We will Edit the following parts of an Element:
Events
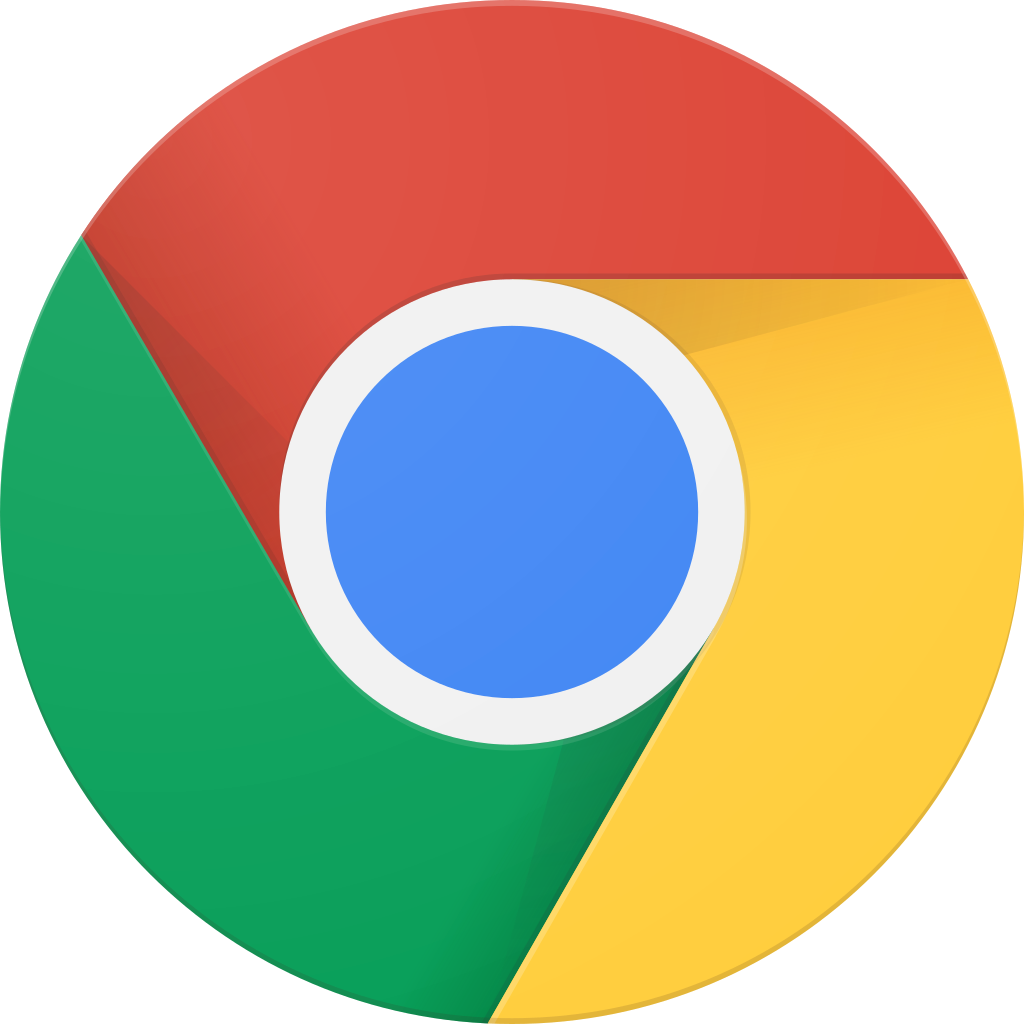
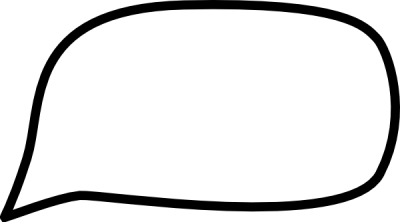
Hey you...website!
Yeah Im talkin to you...
Something happened on your site
Do you wanna do something about it!
Events
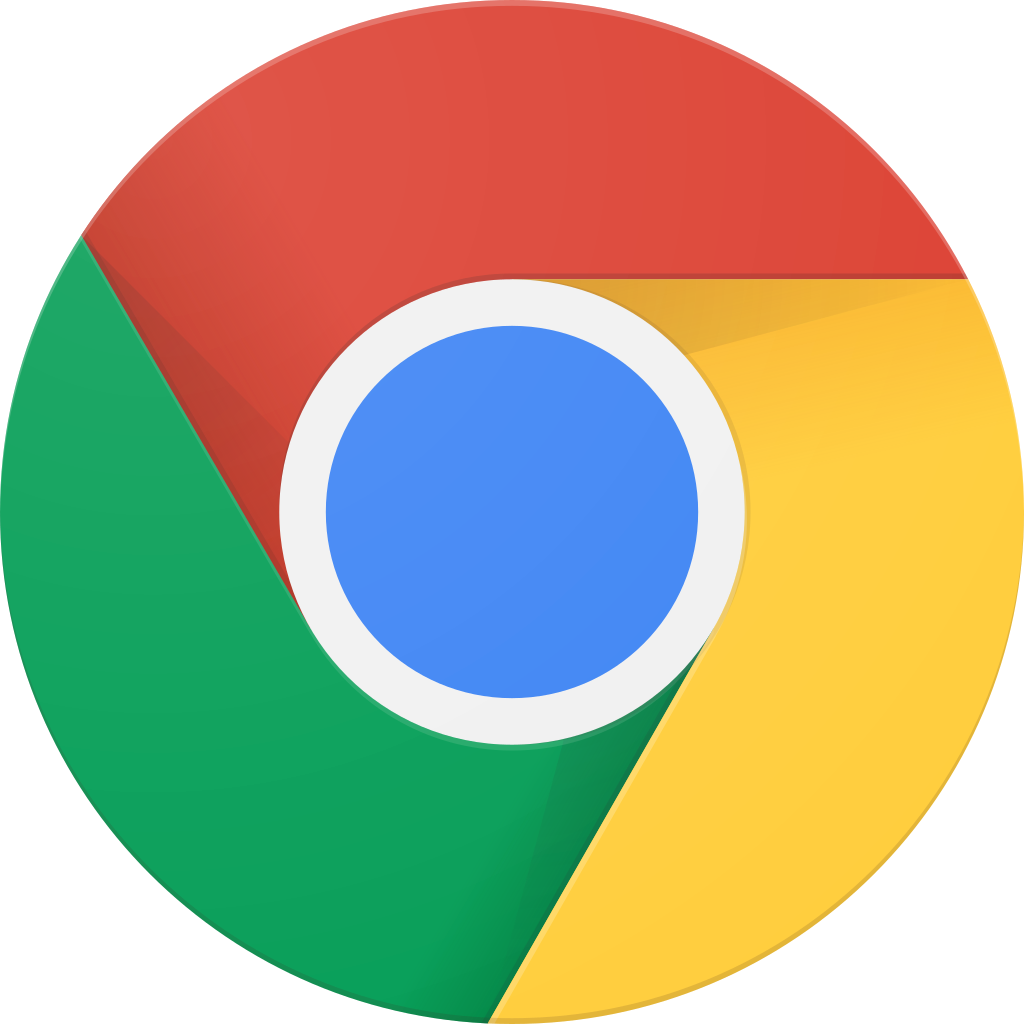
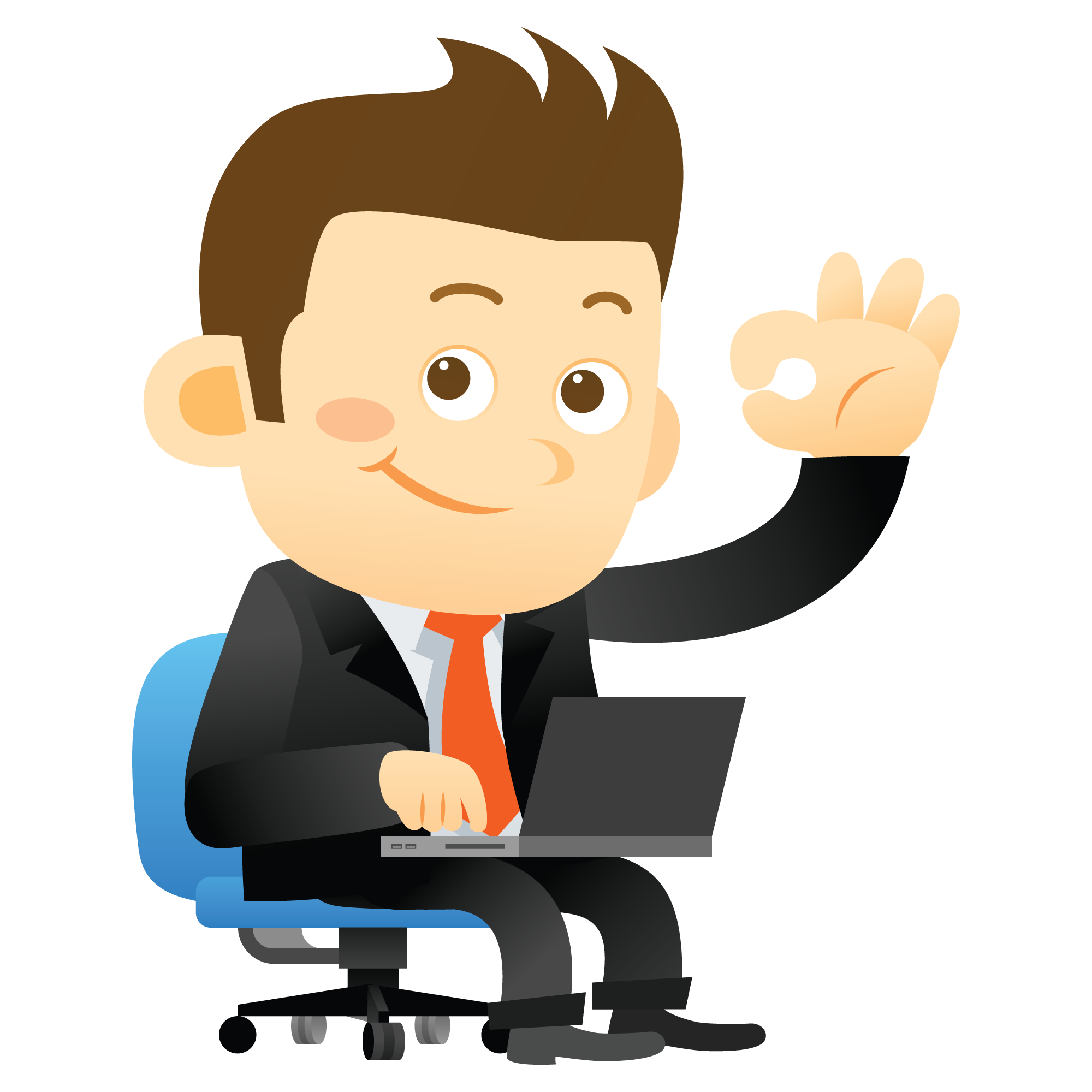
User
Interaction
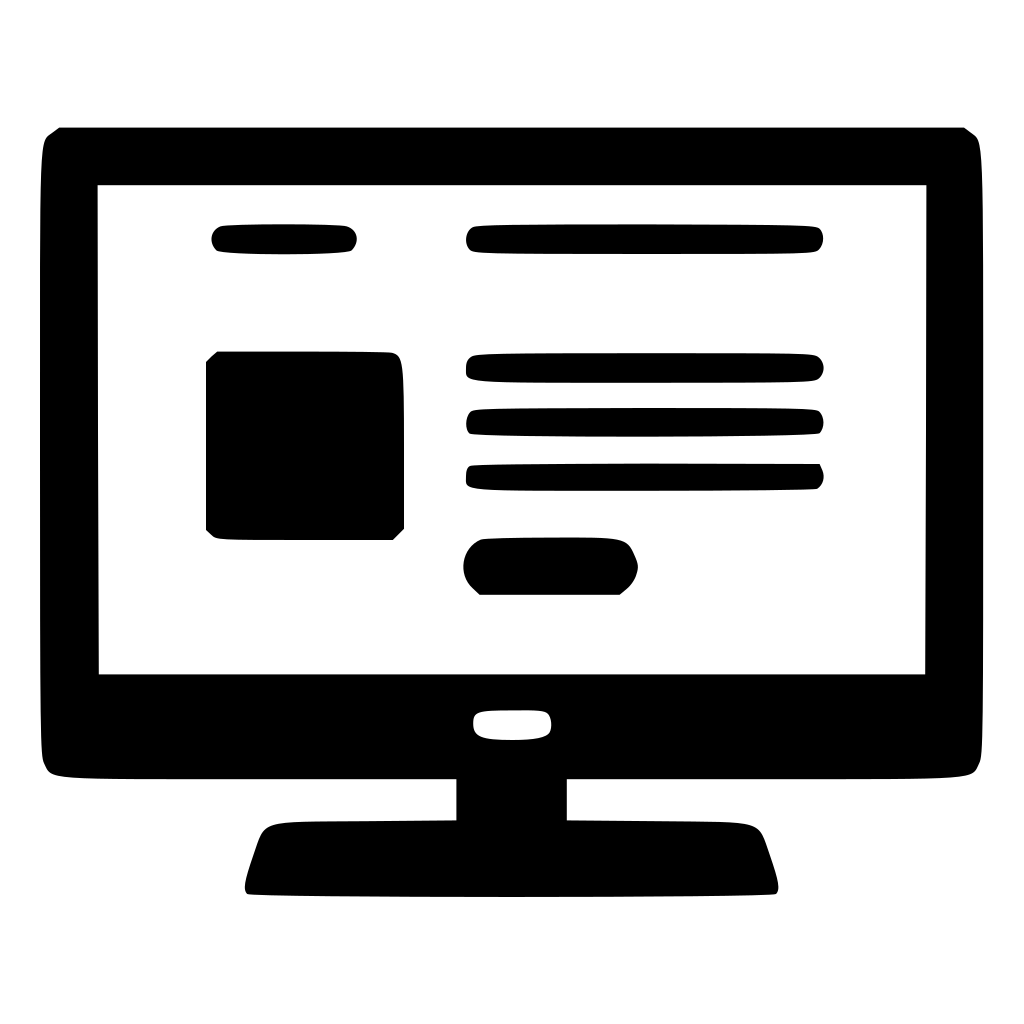
Webpage
Event
Interactions that Cause Events
-
Button Click
-
Hovering mouse over a link
-
Pressing Enter
-
Dragging and Dropping
...
After an Event is Triggered
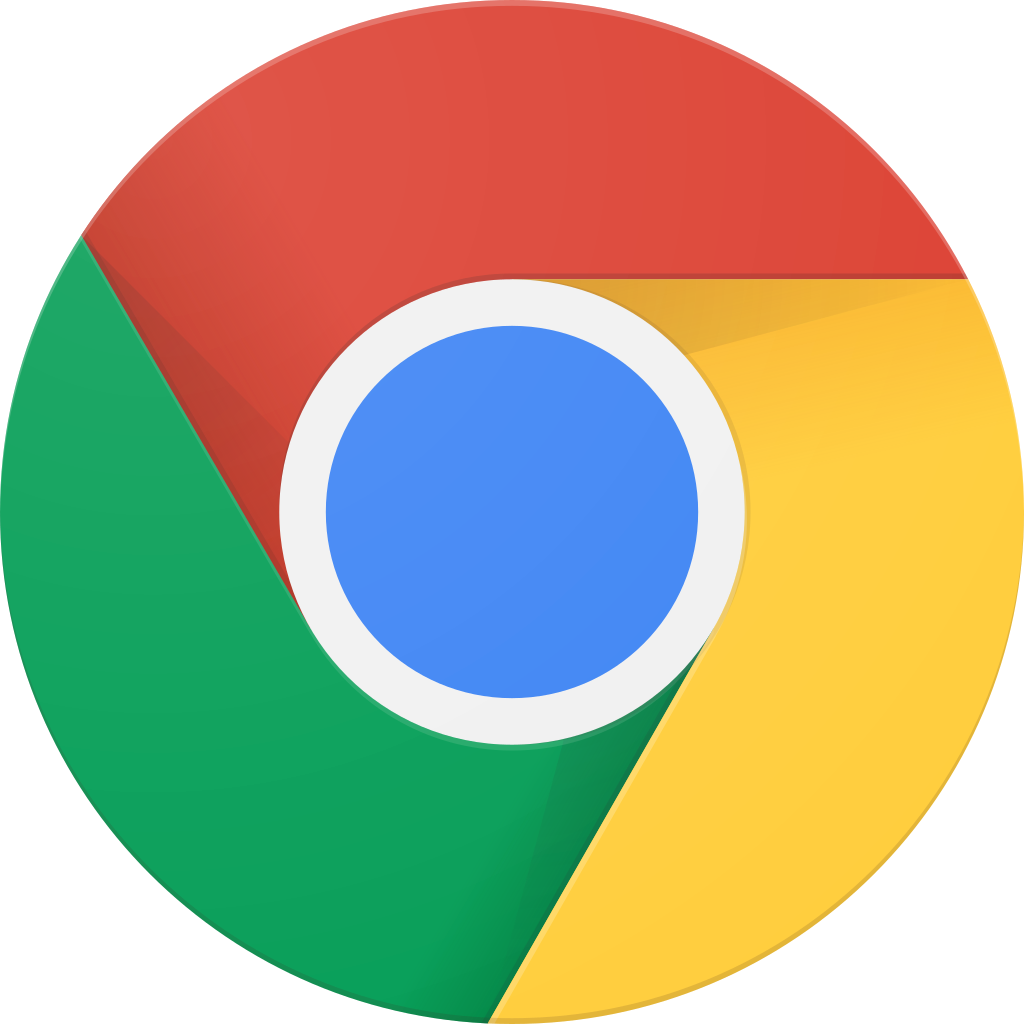
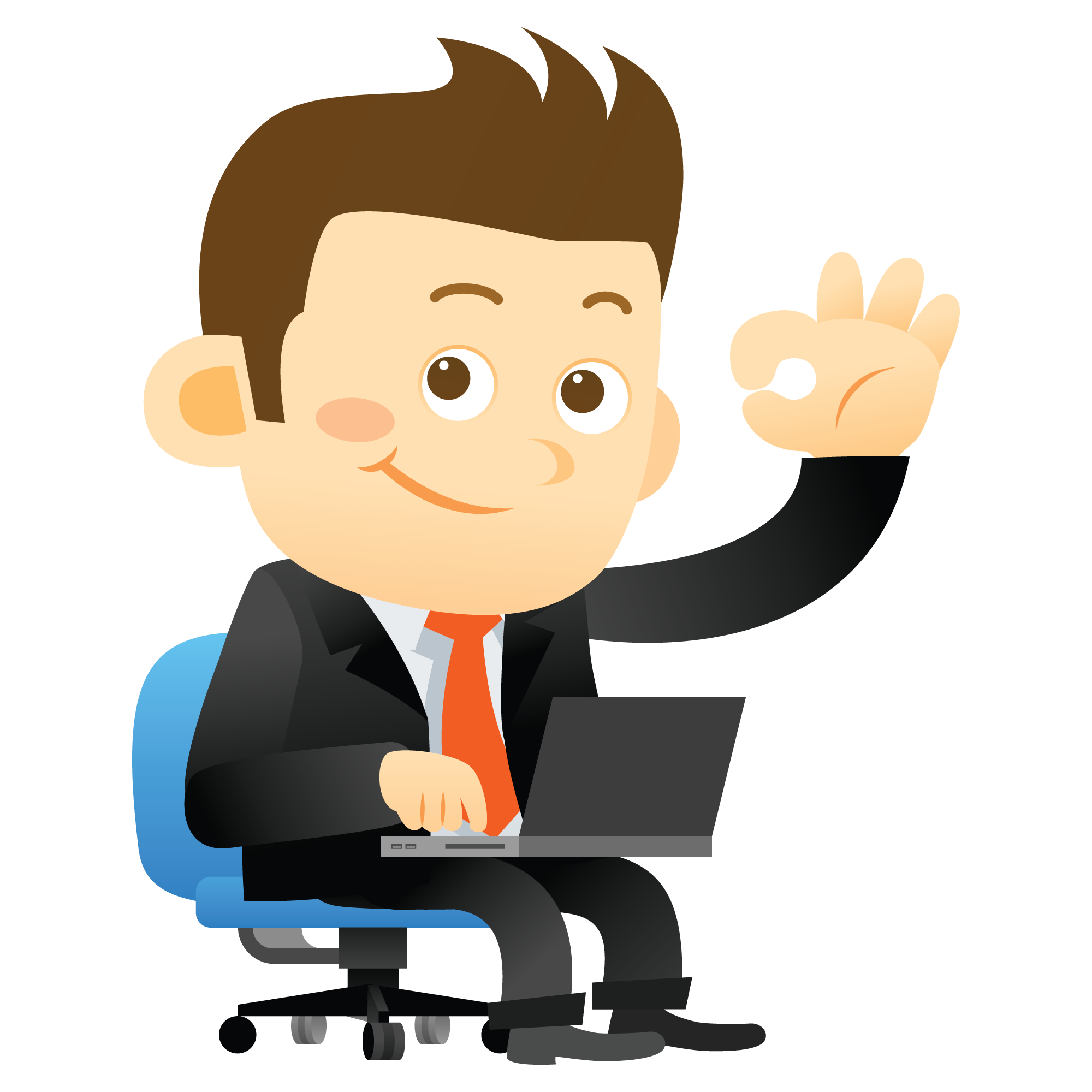
User
Interaction
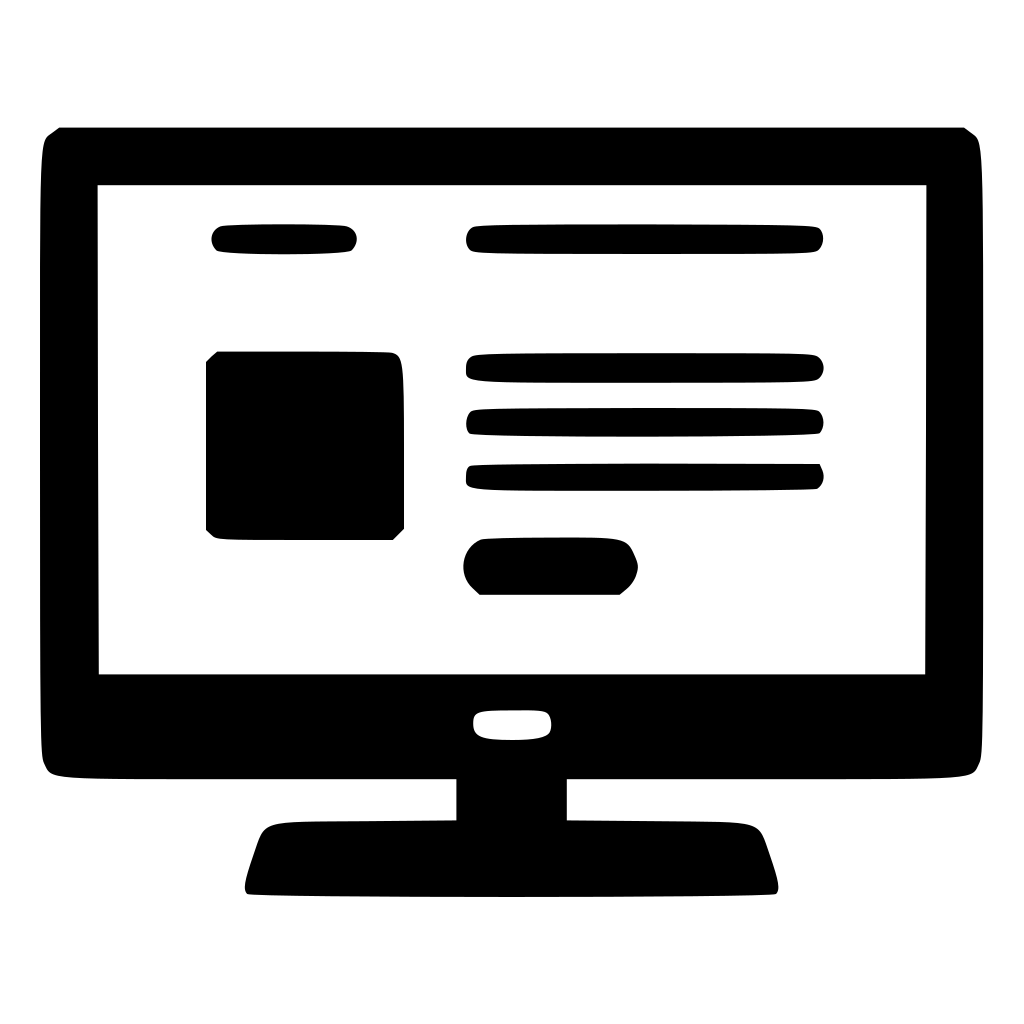
Webpage
Event
Action
How to Trigger Javascript Code using Events
-
Select HTML Element you want to respond to
-
Specify the type of event on the selected element
-
State the code you want to run when the event occur
Im a Button Click Me
div id=display
button id=btn
Im a Button Click Me
Action
button id=btn
div id=display
The button was clicked
The button was clicked
The button was clicked
Event Listeners
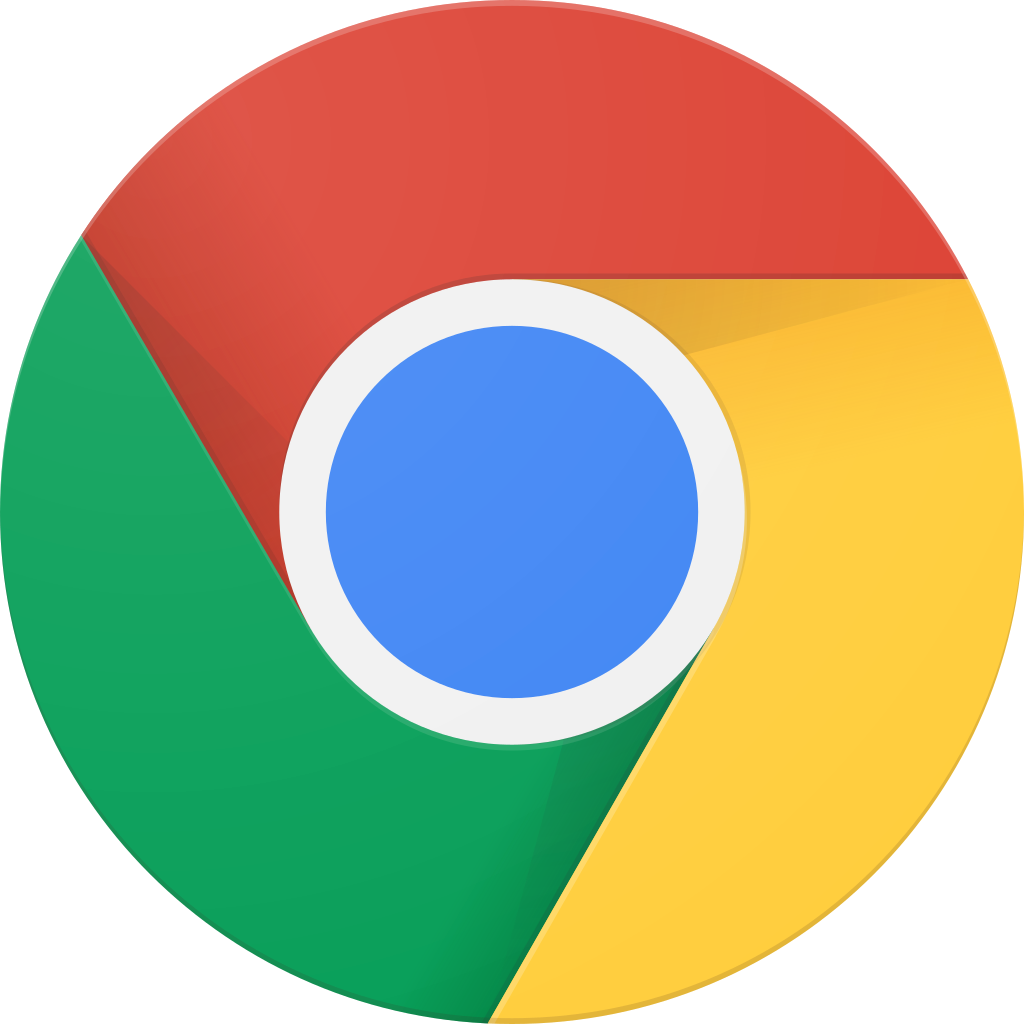
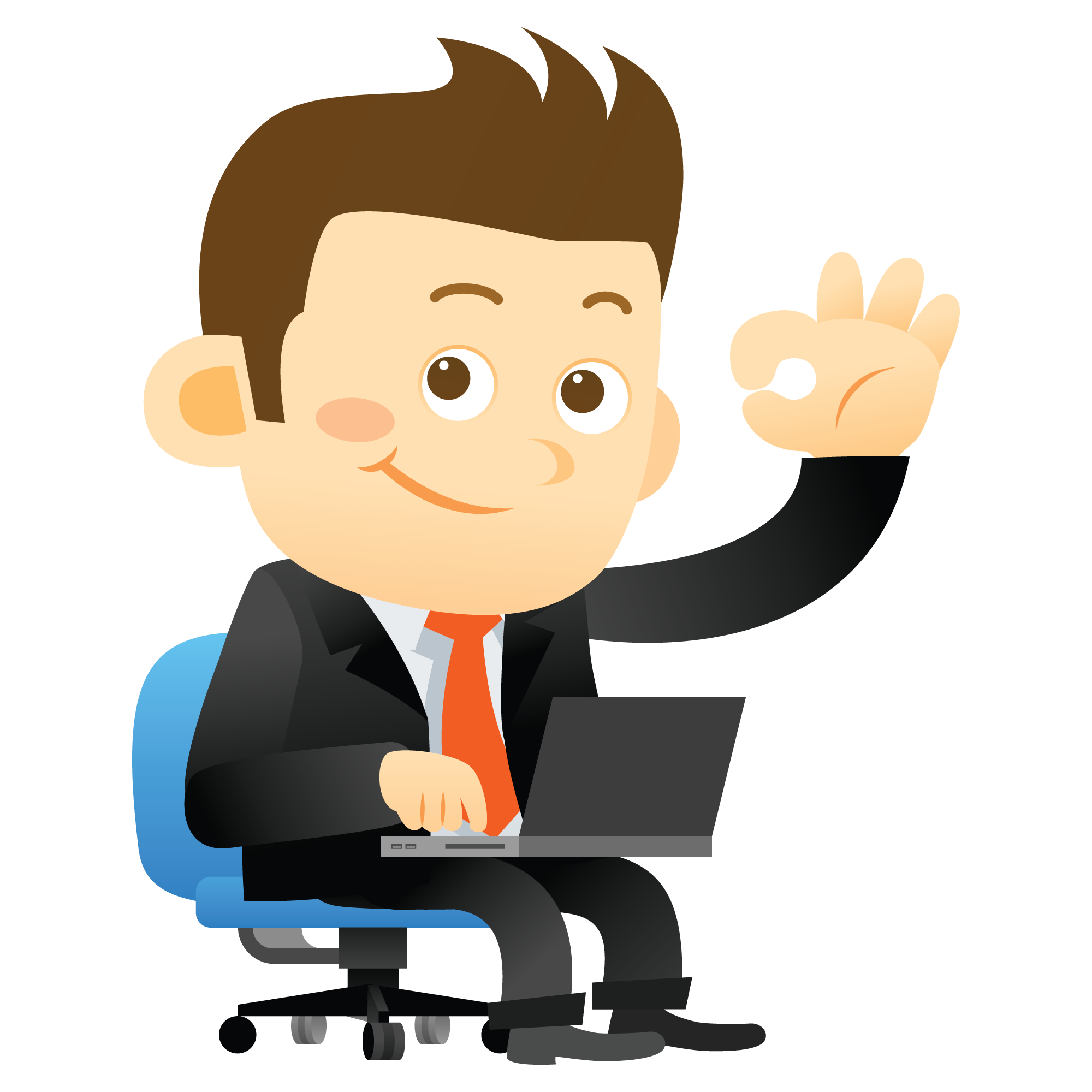
User
Interaction
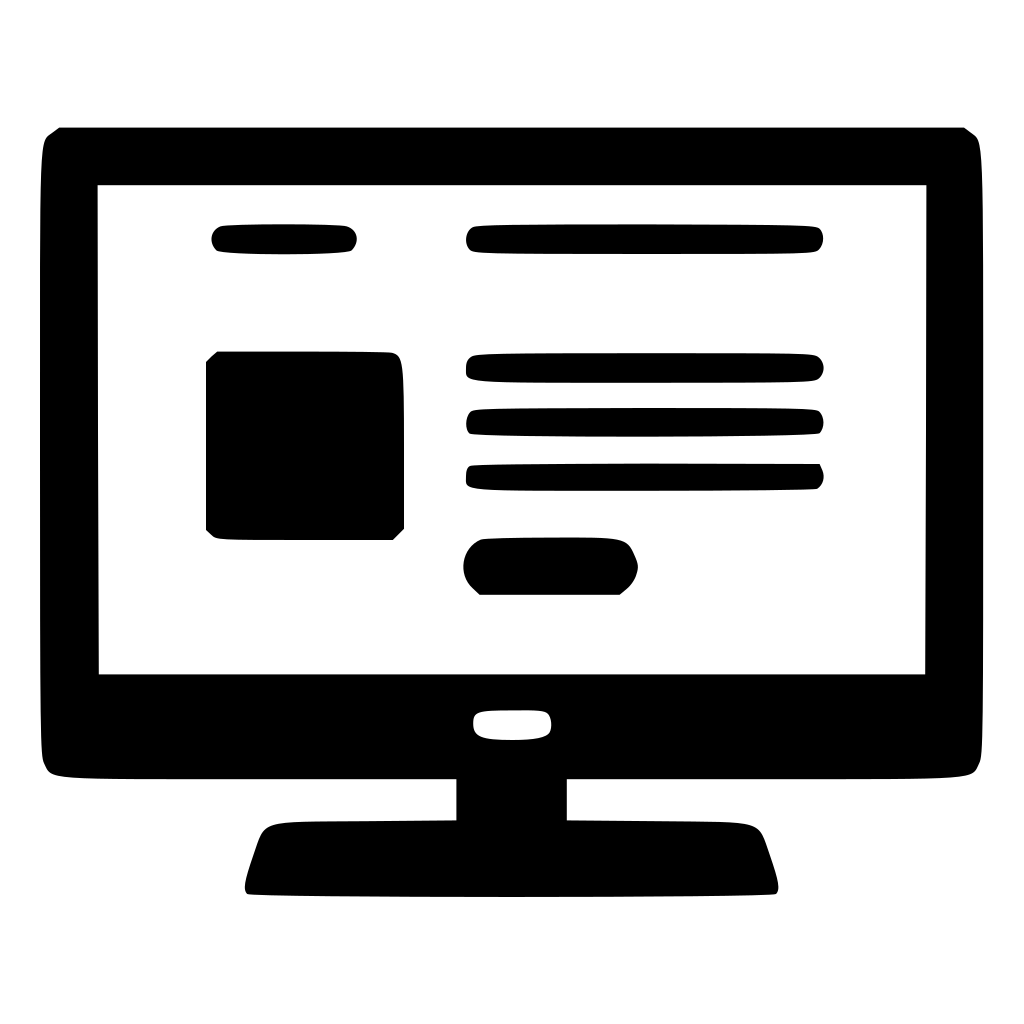
Webpage
Event A
Listener A
Listener B
Event Listeners
element.addEventListener('event', functionName);
Call the addEventListener function on element you want event applied to
myTag
innerText
"Introduction goes here"
function addEventListener(E,f)
{
...
eventE.add(f)
}
style
How to Trigger Javascript Code using Events
-
Select HTML Element you want to respond to
-
Specify the type of event on the selected element
-
State the code you want to run when the event occur
Event Listeners
Call the addEventListener function on element you want event applied to
var button = document.querySelector("button");
function myFunction()
{
console.log("Button was clicked")
}
button.addEventListener("click",myFunction);
Event Listeners
Call the addEventListener function on element you want event applied to
var button = document.querySelector("button");
function myFunction()
{
console.log("Button was clicked")
}
button.addEventListener("click",myFunction);
Event Listeners
element.addEventListener('event', functionName);
Call the addEventListener function on element you want event applied to
var button = document.querySelector("button");
button.addEventListener("click",function()
{
console.log("Button was clicked");
});
Event Listeners
element.addEventListener('event', functionName);
Call the addEventListener function on element you want event applied to
var button = document.querySelector("button");
function myFunction() {
console.log("Button was clicked")
}
button.addEventListener("click",myFunction);
var button = document.querySelector("button");
button.addEventListener("click",
function()
{
console.log("Button was clicked");
}
);
=
Event Listeners
element.addEventListener('event', functionName);
Call the addEventListener function on element you want event applied to
var button = document.querySelector("button");
function myFunction() {
console.log("Button was clicked")
}
button.addEventListener("click",myFunction);
var button = document.querySelector("button");
button.addEventListener("click",function()
{
console.log("Button was clicked");
});
=
<html>
<body>
<div id="page">
<h1 id="header">List</h1>
<h2>Buy groceries</h2>
<ul>
<li id="one" class="hot"><em>Fresh</em> pasta</li>
<li id="two" class="hot">Bread</li>
<li id="three" class="hot">Cooked chicken</li>
<li id="four">Olive oil</li>
</ul>
</div>
</body>
</html>
- Create an event listener to change the background color of the h1 element to red when h1 element is clicked on.
- Create an event listener to change the background of a list item (li) when a list item is clicked on. Do this for all four li's
- Hint: Use querySelectorAll and a for loop
<li>a</li> |
---|
<li>b</li> |
<li>c</li> |
<li>d</li> |
Listener a
myClickFunction()
Listener b
Listener c
Listener d
- Create an event listener to change the background of a list item (li) when it is clicked on. Do this for all four li's
- Hint: Use querySelectorAll and a for loop
- Create an event listener to change the background of a list item (li) when it is clicked on. Do this for all four li's
- Hint: Use querySelectorAll and a for loop
(Remember to use Shift+Enter to create a new line without executing previous lines)
- Go to DOM_style4
- Write javascript code that does the following
- changes the background of the webpage to blue when the button is clicked
- changes the background back to white when button isclicked again
- changes back to blue when clicked again
- ....and so on!
var button = document.querySelector("button");
button.addEventListener("click",function()
{
document.body.style.background = "blue";
});
Good Start! But this will only change the background once
var button = document.querySelector("button");
var isBlue = false;
button.addEventListener("click",function()
{
if(isBlue)
{
document.body.style.background = "white";
isBlue = false;
}
else
{
document.body.style.background = "blue";
isBlue = true;
}
});
var button = document.querySelector("button");
var isBlue = false;
button.addEventListener("click",function()
{
document.classList.toggle("blueBackground");
});
There is another way using CSS....
.blueBackground
{
background: blue;
}
Darts Score Recorder
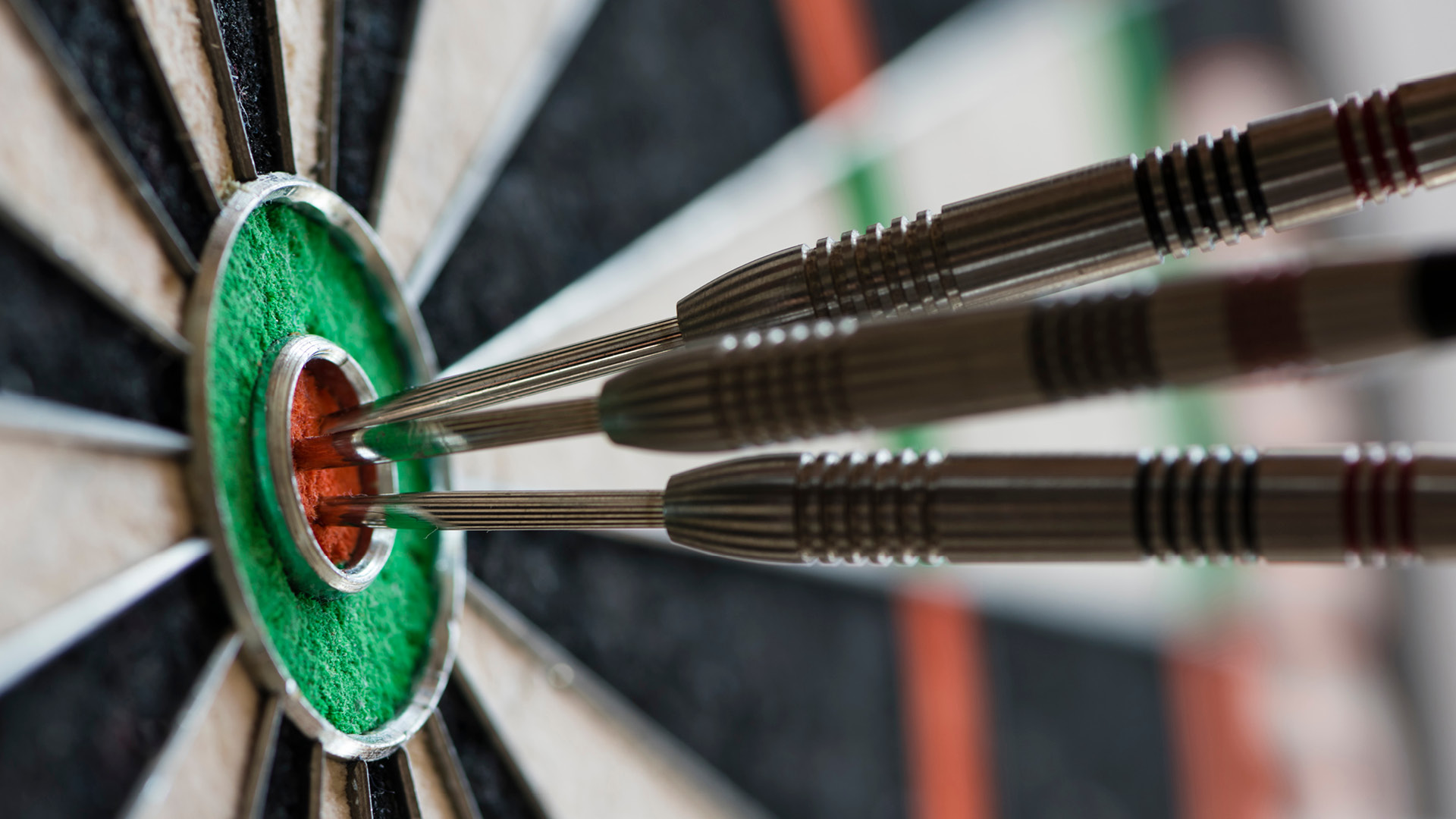
- Create new HTML file in VS Code
- Create HTML code to produce web-page as below:
- Create new JavaScript file in VS Code
- Link HTML file with Javascript file
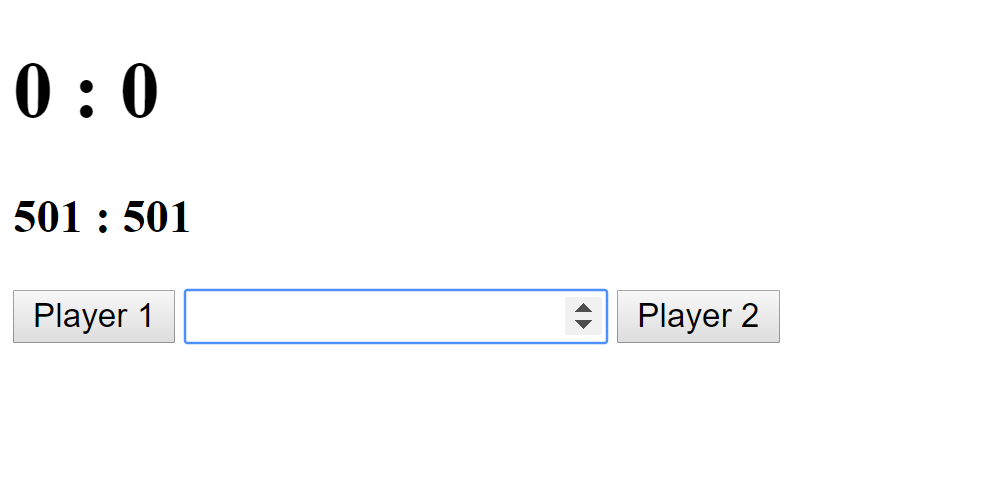
<!DOCTYPE html>
<html>
<head>
<title>Darts Score Recorder</title>
</head>
<body>
<h1>0 : 0</h1>
<h3>501 : 501</h3>
<button>Player 1</button>
<input type="number" name="">
<button>Player 2</button>
<script type="text/javascript" src="darts.js"></script>
</body>
</html>
- Create new HTML file in VS Code
- Create HTML code to produce web-page as below:
- Create new JavaScript file in VS Code
- Link HTML file with Javascript file
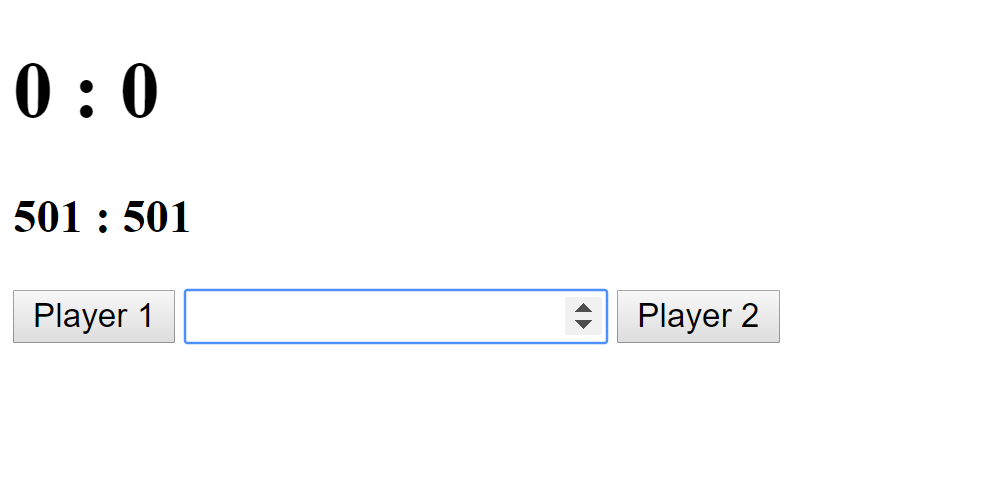
<!DOCTYPE html>
<html>
<head>
<title>Darts Score Recorder</title>
</head>
<body>
<h1>0 : 0</h1>
<h3>501 : 501</h3>
<button id="button1">Player 1</button>
<input type="number" name="" id="score">
<button id="button2">Player 2</button>
<script type="text/javascript" src="darts.js"></script>
</body>
</html>
- Add Code that takes away number entered in input away from first 501 when Player 1 button is clicked
- Add Code that takes away number entered in input away from second 501 when Player 2 button is clicked
<!DOCTYPE html>
<html>
<head>
<title>Darts Score Recorder</title>
</head>
<body>
<h1>0 : 0</h1>
<h3>501 : 501</h3>
<button id="button1">Player 1</button>
<input type="number" name="" id="score">
<button id="button2">Player 2</button>
<script type="text/javascript" src="darts.js"></script>
</body>
</html>
- Add Code that creates a player1Score variable a sets it to 501
- Add code that subtracts number entered into input away from player1Score when button is clicked
var scoreBox = document.querySelector("#score");
var b1 = document.querySelector("#button1");
var p1_score = 501;
b1.addEventListener("click",function()
{
p1_score = p1_score - scoreBox.valueAsNumber;
console.log(p1_score);
});
<!DOCTYPE html>
<html>
<head>
<title>Darts Score Recorder</title>
</head>
<body>
<h1>0 : 0</h1>
<h3>501 : 501</h3>
<button id="button1">Player 1</button>
<input type="number" name="" id="score">
<button id="button2">Player 2</button>
<script type="text/javascript" src="darts.js"></script>
</body>
</html>
- Add Code that changes the h3 text to reflect the score of player1
var scoreBox = document.querySelector("#score");
var b1 = document.querySelector("#button1");
var h3 = document.querySelector("h3");
var p1_score = 501;
b1.addEventListener("click",function()
{
p1_score = p1_score - scoreBox.valueAsNumber;
console.log(p1_score);
h3.textContent = p1_score + " : 501";
});
<!DOCTYPE html>
<html>
<head>
<title>Darts Score Recorder</title>
</head>
<body>
<h1>0 : 0</h1>
<h3>501 : 501</h3>
<button id="button1">Player 1</button>
<input type="number" name="" id="score">
<button id="button2">Player 2</button>
<script type="text/javascript" src="darts.js"></script>
</body>
</html>
- Do the same for player 2
- Create variable
- Add new listener
- ...
var scoreBox = document.querySelector("#score");
var b1 = document.querySelector("#button1");
var b2 = document.querySelector("#button2");
var h3 = document.querySelector("h3");
var p1_score = 501;
var p2_score = 501;
b1.addEventListener("click",function()
{
p1_score = p1_score - scoreBox.valueAsNumber;
h3.textContent = p1_score + " : " + p2_score;
});
b2.addEventListener("click",function()
{
p2_score = p2_score - scoreBox.valueAsNumber;
h3.textContent = p1_score + " : " + p2_score;
});
<!DOCTYPE html>
<html>
<head>
<title>Darts Score Recorder</title>
</head>
<body>
<h1>0 : 0</h1>
<h3>501 : 501</h3>
<button id="button1">Player 1</button>
<input type="number" name="" id="score">
<button id="button2">Player 2</button>
<script type="text/javascript" src="darts.js"></script>
</body>
</html>
- When a player reaches 0 (i.e. wins a leg)
- Increase h1 heading by 1 for that player
- Reset scores to 501 (get ready for next leg)
var scoreBox = document.querySelector("#score");
var b1 = document.querySelector("#button1");
var b2 = document.querySelector("#button2");
var h3 = document.querySelector("h3");
var h1 = document.querySelector("h1");
var p1_sets =0;
var p2_sets =0;
var p1_score = 501;
var p2_score = 501;
b1.addEventListener("click",function()
{
p1_score = p1_score - scoreBox.valueAsNumber;
h3.textContent = p1_score + " : " + p2_score;
if(p1_score === 0)
{
p1_sets = p1_sets + 1;//p1_sets++
h1.textContent = p1_sets + " : " + p2_sets;
resetScores();
}
});
b2.addEventListener("click",function()
{
p2_score = p2_score - scoreBox.valueAsNumber;
h3.textContent = p1_score + " : " + p2_score;
if(p2_score === 0)
{
p2_sets = p2_sets + 1;//p1_sets++
h1.textContent = p1_sets + " : " + p2_sets;
resetScores();
}
});
function resetScores()
{
p1_score = 501;
p2_score = 501;
h3.textContent = p1_score + " : " + p2_score;
}
<!DOCTYPE html>
<html>
<head>
<title>Darts Score Recorder</title>
</head>
<body>
<h1>0 : 0</h1>
<h3>501 : 501</h3>
<button id="button1">Player 1</button>
<input type="number" name="" id="score">
<button id="button2">Player 2</button>
<script type="text/javascript" src="darts.js"></script>
</body>
</html>
- To win a set, a player must reach exactly 0
- When registering a score, ensure that the players overall score does not go below 0.
- If the current score will cause a negative score, then the current score in canceled
var scoreBox = document.querySelector("#score");
var b1 = document.querySelector("#button1");
var b2 = document.querySelector("#button2");
var h3 = document.querySelector("h3");
var h1 = document.querySelector("h1");
var p1_sets =0;
var p2_sets =0;
var p1_score = 501;
var p2_score = 501;
b1.addEventListener("click",function()
{
if(p1_score - scoreBox.valueAsNumber >= 0)
{
p1_score = p1_score - scoreBox.valueAsNumber;
}
h3.textContent = p1_score + " : " + p2_score;
if(p1_score === 0)
{
p1_sets = p1_sets + 1;//p1_sets++
h1.textContent = p1_sets + " : " + p2_sets;
resetScores();
}
});
b2.addEventListener("click",function()
{
if(p2_score - scoreBox.valueAsNumber >= 0)
{
p2_score = p2_score - scoreBox.valueAsNumber;
}
h3.textContent = p1_score + " : " + p2_score;
if(p2_score === 0)
{
p2_sets = p2_sets + 1;//p1_sets++
h1.textContent = p1_sets + " : " + p2_sets;
resetScores();
}
});
function resetScores()
{
p1_score = 501;
p2_score = 501;
h3.textContent = p1_score + " : " + p2_score;
}
DOM Events
So far we have only seen the click event
There are many types of event which you can create listeners for:
See Here
DOM Events
mouseover
mouseout
The event occurs when the pointer is moved onto an element, or onto one of its children
The event occurs when a user moves the mouse pointer out of an element, or out of one of its children
DOM
By D.Kelly
DOM
- 1,414