JavaScript
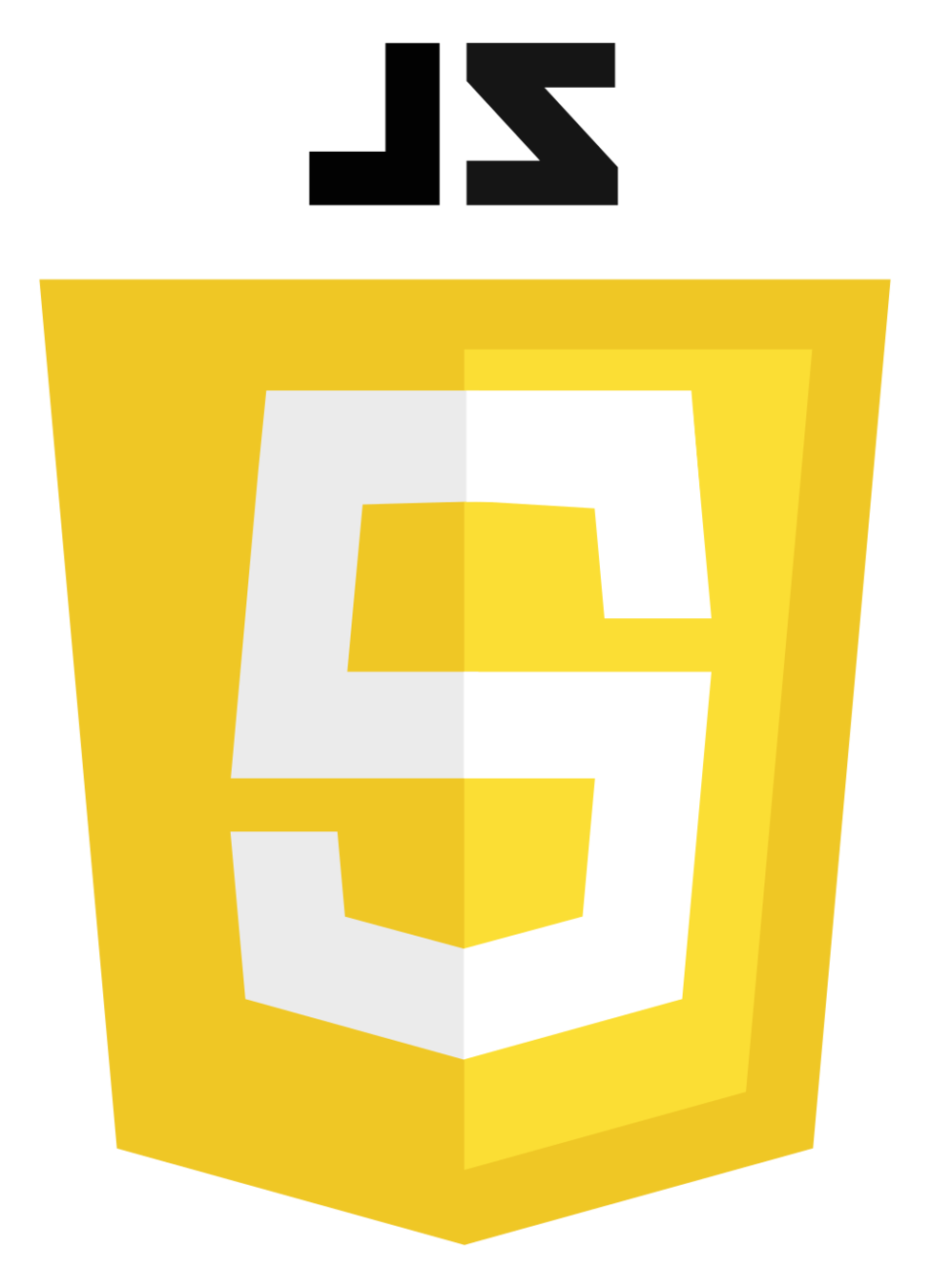
Part 4
JavaScript - Part 4
-
Objects
Objects
Groups together variables and functions to create a model of something
- Model is usually something you would recognize from the real world.
- Person
- Car
- Hotel
- ...
//HOTEL 1
var hotel1_name = "City Hotel";
var hotel1_numRooms = 100;
var hotel1_availableRooms = 30;
//HOTEL 2
var hotel2_name = "Waterfoot";
var hotel2_numRooms = 70;
var hotel2_availableRooms = 20;
Scenario: Hotel Booking Site
//HOTEL 1
var hotel1 = ["City Hotel",100,30];
//HOTEL 2
var hotel2 = ["Waterfoot",70,30];
Scenario: Hotel Booking Site
To get hotel1 available rooms you would need to do this:
//HOTEL 1
var availRooms1 = hotel1[2];
This is confusing and not meaningful.
What if this happened:
//HOTEL 3
var hotel3 = [40,60,"Bishops Gate"];
Objects
var hotel =
{
name: "City Hotel",
numRooms: 100,
availRooms: 30;
};
hotel
{
name
City
Hotel
numRooms
100
availRooms
30
Objects
//retrieve using dot
var hotelName = hotel.name;
//retreive using bracket (similar to array)
var hotelName = hotel["name"];
hotel
{
name
City
Hotel
numRooms
100
availRooms
30
Retrieving Data from Object
Objects
//change value in an object
hotel.availRooms -= 1;
hotel.name = "CITY";
hotel
{
name
CITY
numRooms
100
availRooms
29
Updating Data in an Object
Properties and Methods
var hotel =
{
name: "City Hotel",
numRooms: 100,
availRooms: 30
};
Properties
}
Properties are just a term used to describe variables in an object.
They are used to tell us about the object
Properties and Methods
var hotel =
{
name: "City Hotel",
numRooms: 100,
availRooms: 30,
numberBookedRooms: function()
{
return numRooms - availRooms;
}
};
Properties
}
Method is a function within an object
Methods used to represent tasks associated with an object
Method
}
Constructors
function Hotel(name,rooms,available)
{
this.name: name;
this.numRooms: rooms;
this.availRooms: available;
this.numberBookedRooms: function()
{
return numRooms - availRooms;
};
}
Create many objects that represent similar things
Constructors
function Hotel(name,rooms,available)
{
this.name: name;
this.numRooms: rooms;
this.availRooms: available;
this.numberBookedRooms: function()
{
return numRooms - availRooms;
};
}
Constructor Defines the template
var cityHotel = new Hotel("City Hotel",100,30);
var waterfootHotel = new Hotel("Waterfoot",70,30);
Use the constructor to create object instances
Excercise
Create an object to represent a user.
Data relating to the following should be stored in the user object:
- Name
- Age
- Address
- Password
- Email Address
The object should also have a method isOverage which return true id the user is over 18 and false otherwise.
Excercise
Create an object to represent an Account.
Data relating to the following should be stored in the user object:
- Account No
- Date Created
- User Information (use the User object from previous excercise)
- Account Active (yes or no)
The object should also have a method DeactivateAccount which should set Account Active to false and also set the users password to null so the user can no longer log in.
javascript4
By D.Kelly
javascript4
- 200