HTML5 & JavaScript
Week 11
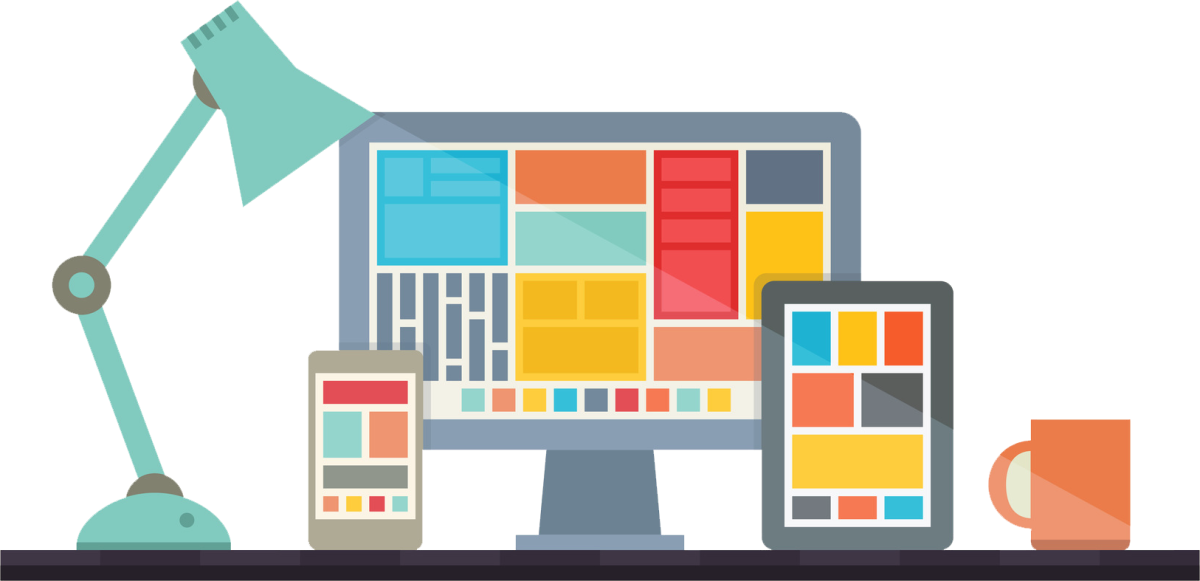
DOM Nodes
document
<html>
<head>
<body>
<title>
<link>
Hotel Home
Page
<h1>
<div>
Rooms Available
<p>
<h3>
Rooms:
<em>
Note the following...
15
- Entire document is a document node
- HTML elements are element nodes
- Text inside HTML elements are text nodes
document node
element node
text node
DOM Nodes
- Entire document is a document node
- HTML elements are element nodes
- Text inside HTML elements are text nodes
document node
element node
text node
- As seen already, all nodes in the tree can be accessed by javascript
- After accessing a node, we can read or modify its content (style, text, attributes
DOM Nodes
- Entire document is a document node
- HTML elements are element nodes
- Text inside HTML elements are text nodes
document node
element node
text node
- We can also add new nodes to the document using javascript
DOM Node Relationships
element node
<html>
element node
<head>
element node
<body>
<html>
<head>
<title>DOM Tutorial</title>
</head>
<body>
<h1>DOM Lesson one</h1>
<p>Hello world!</p>
</body>
</html>
Relationship Types:
- Parent
- Child
- Sibling
DOM Node Relationships
element node
<html>
element node
<head>
element node
<body>
<html>
<head>
<title>DOM Tutorial</title>
</head>
<body>
<h1>DOM Lesson one</h1>
<p>Hello world!</p>
</body>
</html>
Relationship Types:
- Parent
- Child
- Sibling
Parent
Parent
DOM Node Relationships
element node
<html>
element node
<head>
element node
<body>
<html>
<head>
<title>DOM Tutorial</title>
</head>
<body>
<h1>DOM Lesson one</h1>
<p>Hello world!</p>
</body>
</html>
Relationship Types:
- Parent
- Child
- Sibling
parentNode
parentNode
DOM Node Relationships
element node
<html>
element node
<head>
element node
<body>
<html>
<head>
<title>DOM Tutorial</title>
</head>
<body>
<h1>DOM Lesson one</h1>
<p>Hello world!</p>
</body>
</html>
Relationship Types:
- Parent
- Child
- Sibling
Child
Child
DOM Node Relationships
element node
<html>
element node
<head>
element node
<body>
<html>
<head>
<title>DOM Tutorial</title>
</head>
<body>
<h1>DOM Lesson one</h1>
<p>Hello world!</p>
</body>
</html>
Relationship Types:
- Parent
- Child
- Sibling
firstChild
lastChild
DOM Node Relationships
element node
<html>
element node
<head>
element node
<body>
<html>
<head>
<title>DOM Tutorial</title>
</head>
<body>
<h1>DOM Lesson one</h1>
<p>Hello world!</p>
</body>
</html>
Relationship Types:
- Parent
- Child
- Sibling
Set of Children
DOM Node Relationships
element node
<html>
element node
<head>
element node
<body>
<html>
<head>
<title>DOM Tutorial</title>
</head>
<body>
<h1>DOM Lesson one</h1>
<p>Hello world!</p>
</body>
</html>
Relationship Types:
- Parent
- Child
- Sibling
children
children[0]
children[1]
DOM Node Relationships
element node
<html>
element node
<head>
element node
<body>
<html>
<head>
<title>DOM Tutorial</title>
</head>
<body>
<h1>DOM Lesson one</h1>
<p>Hello world!</p>
</body>
</html>
Relationship Types:
- Parent
- Child
- Sibling
Sibling
Sibling
DOM Node Relationships
element node
<html>
element node
<head>
element node
<body>
<html>
<head>
<title>DOM Tutorial</title>
</head>
<body>
<h1>DOM Lesson one</h1>
<p>Hello world!</p>
</body>
</html>
Relationship Types:
- Parent
- Child
- Sibling
nextSibling
previousSibling
DOM Node Relationships
<html>
<head>
<title>DOM Tutorial</title>
</head>
<body>
<h1>DOM Lesson one</h1>
<p>Hello world!</p>
</body>
</html>
element node
<body>
element node
<h1>
element node
<p>
Element nodes do not contain text
"DOM Lesson one"
?
"Hello world!"
?
DOM Node Relationships
<html>
<head>
<title>DOM Tutorial</title>
</head>
<body>
<h1>DOM Lesson one</h1>
<p>Hello world!</p>
</body>
</html>
element node
<body>
element node
<h1>
element node
<p>
Text stored in additional text node
text node
"DOM Lesson One"
text node
"Hello world!"
Creating New HTML Elements
- Create new element
- Append newly created element to an existing element
Creating New HTML Elements
- Create new element
var newH1Element = document.createElement("h1");
var newH3Element = document.createElement("h3");
var newDivElement = document.createElement("div");
var newPElement = document.createElement("p");
Creating New HTML Elements
1.2. Create text node
var newH1Element = document.createElement("h1");
1.1 Create basic element
newH1Element.appendChild(textN);
1.3. Append text node to newly created element
var textN = document.createTextNode("My Heading");
- Create new element
DOM Node Relationships
element node
<h1>
text node
"My Heading"
var newH1Element = document.createElement("h1");
var textN = document.createTextNode("My Heading");
newH1Element.appendChild(textN);
newH1Element
textN
Creating New HTML Elements
var element = document.querySelector("#div1");
2. Append newly created element to an existing element
2.1 Grab Existing Element
2.2 Append new element to grabbed element
element.appendChild(newH1Element);
DOM Node Relationships
element node
<h1>
text node
"My Heading"
var element = document.querySelector("#div1");
element.appendChild(newH1Element);
newH1Element
<html>
<head>
<title>DOM Tutorial</title>
</head>
<body>
<div id="div1">
<h1>Already Existed</h1>
</div>
</body>
</html>
element node
<div>
text node
"Already Existed"
element node
<h1>
element
<html>
<head>
<title>DOM Tutorial</title>
</head>
<body>
<div id="div1">
<h1>Already Existed</h1>
<h1>My Heading</h1>
</div>
</body>
</html>
Removing HTML Elements
- Get access to the parent of the element you want to remove
- Get access to the specific element you want to remove
- Request that parent removes the specific child element
Removing HTML Elements
<div id="div1">
<p id="p1">This is a paragraph.</p>
<p id="p2">This is another paragraph.</p>
</div>
//1.
var parent = document.getElementById("div1");
//2.
var child = document.getElementById("p1");
//3.
parent.removeChild(child);
- Get access to the parent of the element you want to remove
- Get access to the specific element you want to remove
- Request that parent removes the specific child element
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Simple JavaScript Todo</title>
<link rel="stylesheet" type="text/css" href="todo2.css">
</head>
<body>
<div class="wrapper">
<h1>Todo App:</h1>
<div id="inputarea">
<input type="text" id="text" placeholder="Add stuff..">
<button id="add">Add</button>
<button id="removeAll">Remove all</button>
</div>
<div class="border">
<ul id="list"></ul>
</div>
</div>
<script type="text/javascript" src="todo2.js"></script>
</body>
</html>
Sample ToDo List App:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Simple JavaScript Todo</title>
<link rel="stylesheet" type="text/css" href="todo2.css">
</head>
<body>
<div class="wrapper">
<h1>Todo App:</h1>
<div id="inputarea">
<input type="text" id="text" placeholder="Add stuff..">
<button id="add">Add</button>
<button id="removeAll">Remove all</button>
</div>
<div class="border">
<ul id="list"></ul>
</div>
</div>
<script type="text/javascript" src="todo2.js"></script>
</body>
</html>
html, body {
padding: 0;
margin: 0;
width: 100%;
background-color: #9BC1BC;
font-family: 'Roboto', sans-serif;
}
Sample ToDo List App:
Add basic styling: background, font, width and height
<!DOCTYPE html>
<head>
<title>Simple JavaScript Todo</title>
<link rel="stylesheet" type="text/css" href="todo.css">
</head>
<body>
<div class="wrapper">
<div class="border">
<h1>Todo App:<h1>
<input type="text" id="text" placeholder="Add stuff..">
<button id="add">Add</button>
<button id="removeAll">Remove all</button>
<ul id="list"></ul>
</div>
</div>
<script type="text/javascript" src="todo.js"></script>
</body>
</html>
.wrapper {
max-width: 900px;
min-height: 500px;
margin: 0 auto;
height: 100%;
background-color: #EAE1DF;
border-bottom-left-radius: 15px;
border-bottom-right-radius: 15px;
}
Sample ToDo List App:
Style the outer most div: set max-width, center it and add background color
.border {
margin-left: 25px;
margin-right: 25px;
padding-bottom: 25px;
}
Sample ToDo List App:
Style the inner div: add some margin
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Simple JavaScript Todo</title>
<link rel="stylesheet" type="text/css" href="todo2.css">
</head>
<body>
<div class="wrapper">
<h1>Todo App:</h1>
<div id="inputarea">
<input type="text" id="text" placeholder="Add stuff..">
<button id="add">Add</button>
<button id="removeAll">Remove all</button>
</div>
<div class="border">
<ul id="list"></ul>
</div>
</div>
<script type="text/javascript" src="todo2.js"></script>
</body>
</html>
#inputarea
{
padding: 10px 20px 10px 20px;
background-color: #14213D;
border-bottom: 8px solid #FCA311;
}
Sample ToDo List App:
Style the inputarea div: add some color and border color
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Simple JavaScript Todo</title>
<link rel="stylesheet" type="text/css" href="todo2.css">
</head>
<body>
<div class="wrapper">
<h1>Todo App:</h1>
<div id="inputarea">
<input type="text" id="text" placeholder="Add stuff..">
<button id="add">Add</button>
<button id="removeAll">Remove all</button>
</div>
<div class="border">
<ul id="list"></ul>
</div>
</div>
<script type="text/javascript" src="todo2.js"></script>
</body>
</html>
h1 {
text-align: center;
background-color: #FCA311;
margin: 0px;
padding-top: 20px;
padding-bottom: 20px;
text-transform: uppercase;
font-weight: 700;
color: #14213D;
}
Sample ToDo List App:
Style the h1: uppercase, font weight and color
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Simple JavaScript Todo</title>
<link rel="stylesheet" type="text/css" href="todo2.css">
</head>
<body>
<div class="wrapper">
<h1>Todo App:</h1>
<div id="inputarea">
<input type="text" id="text" placeholder="Add stuff..">
<button id="add">Add</button>
<button id="removeAll">Remove all</button>
</div>
<div class="border">
<ul id="list"></ul>
</div>
</div>
<script type="text/javascript" src="todo2.js"></script>
</body>
</html>
input {
padding: 10px;
color: #212121;
border: 1px solid #d2d2d2;
border-radius: 2px 2px 2px 2px;
}
Sample ToDo List App:
Style the textbox
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Simple JavaScript Todo</title>
<link rel="stylesheet" type="text/css" href="todo2.css">
</head>
<body>
<div class="wrapper">
<h1>Todo App:</h1>
<div id="inputarea">
<input type="text" id="text" placeholder="Add stuff..">
<button id="add">Add</button>
<button id="removeAll">Remove all</button>
</div>
<div class="border">
<ul id="list"></ul>
</div>
</div>
<script type="text/javascript" src="todo2.js"></script>
</body>
</html>
button {
padding: 10px;
border: 0 none;
border-radius: 2px 2px 2px 2px;
color: #FFFFFF;
cursor: pointer;
font-weight: 800;
text-transform: uppercase;
font-size: 12px;
}
Sample ToDo List App:
Define general button style
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Simple JavaScript Todo</title>
<link rel="stylesheet" type="text/css" href="todo2.css">
</head>
<body>
<div class="wrapper">
<h1>Todo App:</h1>
<div id="inputarea">
<input type="text" id="text" placeholder="Add stuff..">
<button id="add">Add</button>
<button id="removeAll">Remove all</button>
</div>
<div class="border">
<ul id="list"></ul>
</div>
</div>
<script type="text/javascript" src="todo2.js"></script>
</body>
</html>
button#add {
background-color: #46b98a;
}
button#add:hover {
background-color: #3fa67c;
}
button#removeAll {
background-color: #E0645C;
}
button#removeAll:hover {
background-color: #c95a52;
}
Sample ToDo List App:
Define specific colors for add and remove buttons
Define specific hover colors for add and remove buttons
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Simple JavaScript Todo</title>
<link rel="stylesheet" type="text/css" href="todo2.css">
</head>
<body>
<div class="wrapper">
<h1>Todo App:</h1>
<div id="inputarea">
<input type="text" id="text" placeholder="Add stuff..">
<button id="add">Add</button>
<button id="removeAll">Remove all</button>
</div>
<div class="border">
<ul id="list"></ul>
</div>
</div>
<script type="text/javascript" src="todo2.js"></script>
</body>
</html>
ul#list {
margin: 0;
padding: 20px 0 0 0;
}
Sample ToDo List App:
Style the ul: set padding and no margins
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Simple JavaScript Todo</title>
<link rel="stylesheet" type="text/css" href="todo2.css">
</head>
<body>
<div class="wrapper">
<h1>Todo App:</h1>
<div id="inputarea">
<input type="text" id="text" placeholder="Add stuff..">
<button id="add">Add</button>
<button id="removeAll">Remove all</button>
</div>
<div class="border">
<ul id="list"></ul>
</div>
</div>
<script type="text/javascript" src="todo2.js"></script>
</body>
</html>
var add = document.querySelector('#add');
add.addEventListener("click",addLi);
function addLi()
{
}
Sample ToDo List App:
Create click event listen for add button
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Simple JavaScript Todo</title>
<link rel="stylesheet" type="text/css" href="todo2.css">
</head>
<body>
<div class="wrapper">
<h1>Todo App:</h1>
<div id="inputarea">
<input type="text" id="text" placeholder="Add stuff..">
<button id="add">Add</button>
<button id="removeAll">Remove all</button>
</div>
<div class="border">
<ul id="list"></ul>
</div>
</div>
<script type="text/javascript" src="todo2.js"></script>
</body>
</html>
var add = document.querySelector('#add');
add.addEventListener("click",addLi);
function addLi()
{
var inputText = document.getElementById('text').value;
}
Sample ToDo List App:
Grab the text box value
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Simple JavaScript Todo</title>
<link rel="stylesheet" type="text/css" href="todo2.css">
</head>
<body>
<div class="wrapper">
<h1>Todo App:</h1>
<div id="inputarea">
<input type="text" id="text" placeholder="Add stuff..">
<button id="add">Add</button>
<button id="removeAll">Remove all</button>
</div>
<div class="border">
<ul id="list"></ul>
</div>
</div>
<script type="text/javascript" src="todo2.js"></script>
</body>
</html>
var add = document.querySelector('#add');
add.addEventListener("click",addLi);
function addLi()
{
var inputText = document.getElementById('text').value;
var li = document.createElement('li');
var textNode = document.createTextNode(inputText + ' ');
}
Sample ToDo List App:
Create some new elements
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Simple JavaScript Todo</title>
<link rel="stylesheet" type="text/css" href="todo2.css">
</head>
<body>
<div class="wrapper">
<h1>Todo App:</h1>
<div id="inputarea">
<input type="text" id="text" placeholder="Add stuff..">
<button id="add">Add</button>
<button id="removeAll">Remove all</button>
</div>
<div class="border">
<ul id="list"></ul>
</div>
</div>
<script type="text/javascript" src="todo2.js"></script>
</body>
</html>
var add = document.querySelector('#add');
add.addEventListener("click",addLi);
function addLi()
{
var inputText = document.getElementById('text').value;
var li = document.createElement('li');
var textNode = document.createTextNode(inputText + ' ');
li.appendChild(textNode);
}
Sample ToDo List App:
Add the text to the li
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Simple JavaScript Todo</title>
<link rel="stylesheet" type="text/css" href="todo2.css">
</head>
<body>
<div class="wrapper">
<h1>Todo App:</h1>
<div id="inputarea">
<input type="text" id="text" placeholder="Add stuff..">
<button id="add">Add</button>
<button id="removeAll">Remove all</button>
</div>
<div class="border">
<ul id="list"></ul>
</div>
</div>
<script type="text/javascript" src="todo2.js"></script>
</body>
</html>
var add = document.querySelector('#add');
add.addEventListener("click",addLi);
function addLi()
{
var inputText = document.getElementById('text').value;
var li = document.createElement('li');
var textNode = document.createTextNode(inputText + ' ');
li.appendChild(textNode);
var ul = document.querySelector('#list');
ul.appendChild(li);
}
Sample ToDo List App:
Add the li to the ul
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Simple JavaScript Todo</title>
<link rel="stylesheet" type="text/css" href="todo2.css">
</head>
<body>
<div class="wrapper">
<h1>Todo App:</h1>
<div id="inputarea">
<input type="text" id="text" placeholder="Add stuff..">
<button id="add">Add</button>
<button id="removeAll">Remove all</button>
</div>
<div class="border">
<ul id="list"></ul>
</div>
</div>
<script type="text/javascript" src="todo2.js"></script>
</body>
</html>
var add = document.querySelector('#add');
add.addEventListener("click",addLi);
function addLi()
{
var inputText = document.getElementById('text').value;
var li = document.createElement('li');
var textNode = document.createTextNode(inputText + ' ');
var removeButton = document.createElement('button');//<---add this
li.appendChild(textNode);
li.appendChild(removeButton);//<---add this
var ul = document.querySelector('#list');
ul.appendChild(li);
}
Sample ToDo List App:
Add a button the the li also
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Simple JavaScript Todo</title>
<link rel="stylesheet" type="text/css" href="todo2.css">
</head>
<body>
<div class="wrapper">
<h1>Todo App:</h1>
<div id="inputarea">
<input type="text" id="text" placeholder="Add stuff..">
<button id="add">Add</button>
<button id="removeAll">Remove all</button>
</div>
<div class="border">
<ul id="list"></ul>
</div>
</div>
<script type="text/javascript" src="todo2.js"></script>
</body>
</html>
var add = document.querySelector('#add');
add.addEventListener("click",addLi);
function addLi()
{
var inputText = document.getElementById('text').value;
var li = document.createElement('li');
var textNode = document.createTextNode(inputText + ' ');
var removeButton = document.createElement('button');
removeButton.classList.add('removeMe');//<---add this
li.appendChild(textNode);
li.appendChild(removeButton);
var ul = document.querySelector('#list');
ul.appendChild(li);
}
Sample ToDo List App:
Style the button by adding the "removeMe" class
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Simple JavaScript Todo</title>
<link rel="stylesheet" type="text/css" href="todo2.css">
</head>
<body>
<div class="wrapper">
<h1>Todo App:</h1>
<div id="inputarea">
<input type="text" id="text" placeholder="Add stuff..">
<button id="add">Add</button>
<button id="removeAll">Remove all</button>
</div>
<div class="border">
<ul id="list"></ul>
</div>
</div>
<script type="text/javascript" src="todo2.js"></script>
</body>
</html>
var add = document.querySelector('#add');
add.addEventListener("click",addLi);
function addLi()
{
var inputText = document.getElementById('text').value;
var li = document.createElement('li');
var textNode = document.createTextNode(inputText + ' ');
var removeButton = document.createElement('button');
removeButton.classList.add('removeMe');
var checkbox = document.createElement('input');//<---add this
checkbox.type = "checkbox";//<---add this
checkbox.id = "cb";//<---add this
li.appendChild(checkbox);//<---add this
li.appendChild(textNode);
li.appendChild(removeButton);
var ul = document.querySelector('#list');
ul.appendChild(li);
}
Sample ToDo List App:
Add a checkbox to the li also
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Simple JavaScript Todo</title>
<link rel="stylesheet" type="text/css" href="todo2.css">
</head>
<body>
<div class="wrapper">
<h1>Todo App:</h1>
<div id="inputarea">
<input type="text" id="text" placeholder="Add stuff..">
<button id="add">Add</button>
<button id="removeAll">Remove all</button>
</div>
<div class="border">
<ul id="list"></ul>
</div>
</div>
<script type="text/javascript" src="todo2.js"></script>
</body>
</html>
var add = document.querySelector('#add');
add.addEventListener("click",addLi);
function addLi()
{
var inputText = document.getElementById('text').value;
if(inputText.length > 0)
{
var li = document.createElement('li');
var textNode = document.createTextNode(inputText + ' ');
var removeButton = document.createElement('button');
removeButton.classList.add('removeMe');
removeButton.textContent = "X";
var checkbox = document.createElement('input');
checkbox.type = "checkbox";
checkbox.id = "cb";
li.appendChild(checkbox);
li.appendChild(textNode);
li.appendChild(removeButton);
var ul = document.querySelector('#list');
ul.appendChild(li);
}
}
Sample ToDo List App:
Add some error handling - Ensure something has actually been entered in the text box before adding an li
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Simple JavaScript Todo</title>
<link rel="stylesheet" type="text/css" href="todo2.css">
</head>
<body>
<div class="wrapper">
<h1>Todo App:</h1>
<div id="inputarea">
<input type="text" id="text" placeholder="Add stuff..">
<button id="add">Add</button>
<button id="removeAll">Remove all</button>
</div>
<div class="border">
<ul id="list"></ul>
</div>
</div>
<script type="text/javascript" src="todo2.js"></script>
</body>
</html>
Sample ToDo List App:
Style the lis that are added now:
Remove bullet point, change font size, add border, set height
li {
list-style: none;
font-size: 22px;
padding: 5px;
border: 1px #D3D3D3 solid;
border-radius: 2px;
min-height: 25px;
margin-top: 2px;
background-color: #fff;
}
li:hover
{
box-shadow: 0px 0px 8px 2px rgba(0,0,0,0.55);
}
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Simple JavaScript Todo</title>
<link rel="stylesheet" type="text/css" href="todo2.css">
</head>
<body>
<div class="wrapper">
<h1>Todo App:</h1>
<div id="inputarea">
<input type="text" id="text" placeholder="Add stuff..">
<button id="add">Add</button>
<button id="removeAll">Remove all</button>
</div>
<div class="border">
<ul id="list"></ul>
</div>
</div>
<script type="text/javascript" src="todo2.js"></script>
</body>
</html>
Sample ToDo List App:
Style the button in the lis
button.removeMe {
padding: 5px;
font-size: 13px;
margin-left: 10px;
font-weight: 700;
float: right;
padding-left: 10px;
padding-right: 10px;
}
button.removeMe:hover {
background-color: #E0645C;
}
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Simple JavaScript Todo</title>
<link rel="stylesheet" type="text/css" href="todo2.css">
</head>
<body>
<div class="wrapper">
<h1>Todo App:</h1>
<div id="inputarea">
<input type="text" id="text" placeholder="Add stuff..">
<button id="add">Add</button>
<button id="removeAll">Remove all</button>
</div>
<div class="border">
<ul id="list"></ul>
</div>
</div>
<script type="text/javascript" src="todo2.js"></script>
</body>
</html>
var add = document.querySelector('#add');
add.addEventListener("click",addLi);
function addLi()
{
var inputText = document.getElementById('text').value;
if(inputText.length > 0)
{
var li = document.createElement('li');
var textNode = document.createTextNode(inputText + ' ');
var removeButton = document.createElement('button');
removeButton.classList.add('removeMe');
removeButton.textContent = "X";
removeButton.addEventListener("click",removeMe);//<--add this
var checkbox = document.createElement('input');
checkbox.type = "checkbox";
checkbox.id = "cb";
li.appendChild(checkbox);
li.appendChild(textNode);
li.appendChild(removeButton);
var ul = document.querySelector('#list');
ul.appendChild(li);
}
}
function removeMe()
{
}
Sample ToDo List App:
Add an event handler to each removeMe button
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Simple JavaScript Todo</title>
<link rel="stylesheet" type="text/css" href="todo2.css">
</head>
<body>
<div class="wrapper">
<h1>Todo App:</h1>
<div id="inputarea">
<input type="text" id="text" placeholder="Add stuff..">
<button id="add">Add</button>
<button id="removeAll">Remove all</button>
</div>
<div class="border">
<ul id="list"></ul>
</div>
</div>
<script type="text/javascript" src="todo2.js"></script>
</body>
</html>
//..
function removeMe()
{
//"this" is equivlent to the button that was clicked
var li_item = this.parentElement;
var ul_item = li_item.parentElement;
ul_item.removeChild(li_item);
}
Sample ToDo List App:
Add event handler code to remove li item
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Simple JavaScript Todo</title>
<link rel="stylesheet" type="text/css" href="todo2.css">
</head>
<body>
<div class="wrapper">
<h1>Todo App:</h1>
<div id="inputarea">
<input type="text" id="text" placeholder="Add stuff..">
<button id="add">Add</button>
<button id="removeAll">Remove all</button>
</div>
<div class="border">
<ul id="list"></ul>
</div>
</div>
<script type="text/javascript" src="todo2.js"></script>
</body>
</html>
//..
function addLi()
{
var inputText = document.getElementById('text').value;
if(inputText.length > 0)
{
var li = document.createElement('li');
var textNode = document.createTextNode(inputText + ' ');
var removeButton = document.createElement('button');
removeButton.classList.add('removeMe');
removeButton.textContent = "X";
removeButton.addEventListener("click",removeMe);
var checkbox = document.createElement('input');
checkbox.type = "checkbox";
checkbox.id = "cb";
checkbox.addEventListener("change",checked);//<--add this
li.appendChild(checkbox);
li.appendChild(textNode);
li.appendChild(removeButton);
var ul = document.querySelector('#list');
ul.appendChild(li);
}
}
function checked()//<---add this
{
}
Sample ToDo List App:
Add "change" event handler to the checkbox
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Simple JavaScript Todo</title>
<link rel="stylesheet" type="text/css" href="todo2.css">
</head>
<body>
<div class="wrapper">
<h1>Todo App:</h1>
<div id="inputarea">
<input type="text" id="text" placeholder="Add stuff..">
<button id="add">Add</button>
<button id="removeAll">Remove all</button>
</div>
<div class="border">
<ul id="list"></ul>
</div>
</div>
<script type="text/javascript" src="todo2.js"></script>
</body>
</html>
Sample ToDo List App:
.selected
{
text-decoration: line-through;
color: #D7D7D7;
background-color: rgba(255,255,255,0.6) ;
}
Add css selected class
//..
function checked()
{
//"this" is equivalent to the checkbox that changed
var li_item = this.parentElement;
li_item.classList.toggle("selected");
}
Sample ToDo List App:
Toggle the "selected" when the checkbox changes
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Simple JavaScript Todo</title>
<link rel="stylesheet" type="text/css" href="todo2.css">
</head>
<body>
<div class="wrapper">
<h1>Todo App:</h1>
<div id="inputarea">
<input type="text" id="text" placeholder="Add stuff..">
<button id="add">Add</button>
<button id="removeAll">Remove all</button>
</div>
<div class="border">
<ul id="list"></ul>
</div>
</div>
<script type="text/javascript" src="todo2.js"></script>
</body>
</html>
//..
var removeAll = document.querySelector('#removeAll');
removeAll.addEventListener("click",reset);
function reset()
{
}
Sample ToDo List App:
Set up the Remove All Button
- Add event listener
- Remove all li elements
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Simple JavaScript Todo</title>
<link rel="stylesheet" type="text/css" href="todo2.css">
</head>
<body>
<div class="wrapper">
<h1>Todo App:</h1>
<div id="inputarea">
<input type="text" id="text" placeholder="Add stuff..">
<button id="add">Add</button>
<button id="removeAll">Remove all</button>
</div>
<div class="border">
<ul id="list"></ul>
</div>
</div>
<script type="text/javascript" src="todo2.js"></script>
</body>
</html>
//..
var removeAll = document.querySelector('#removeAll');
removeAll.addEventListener("click",reset);
function reset()
{
var ul = document.querySelector('#list');
ul.innerHTML = '';
}
Sample ToDo List App:
Set up the Remove All Button
- Add event listener
- Remove all li elements
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Simple JavaScript Todo</title>
<link rel="stylesheet" type="text/css" href="todo2.css">
</head>
<body>
<div class="wrapper">
<h1>Todo App:</h1>
<div id="inputarea">
<input type="text" id="text" placeholder="Add stuff..">
<button id="add">Add</button>
<button id="removeAll">Remove all</button>
</div>
<div class="border">
<ul id="list"></ul>
</div>
</div>
<script type="text/javascript" src="todo2.js"></script>
</body>
</html>
//..
function loadItem(text)
{
var li = document.createElement('li');
var textNode = document.createTextNode(text + ' ');
var removeButton = document.createElement('button');
removeButton.classList.add('removeMe');
removeButton.textContent = "X";
removeButton.addEventListener("click",removeMe);
var checkbox = document.createElement('input');
checkbox.type = "checkbox";
checkbox.id = "cb";
checkbox.addEventListener("change",checked)
li.appendChild(checkbox);
li.appendChild(textNode);
li.appendChild(removeButton);
var ul = document.querySelector('#list');
ul.appendChild(li);
var numberOfitems = ul.children.length;
}
Sample ToDo List App:
Tidy Up a little
- Move core part of addLi() code into seperate loadItem() function
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Simple JavaScript Todo</title>
<link rel="stylesheet" type="text/css" href="todo2.css">
</head>
<body>
<div class="wrapper">
<h1>Todo App:</h1>
<div id="inputarea">
<input type="text" id="text" placeholder="Add stuff..">
<button id="add">Add</button>
<button id="removeAll">Remove all</button>
</div>
<div class="border">
<ul id="list"></ul>
</div>
</div>
<script type="text/javascript" src="todo2.js"></script>
</body>
</html>
//..
function addLi()
{
var inputText = document.getElementById('text').value;
if(inputText.length > 0)
{
loadItem(inputText);
}
}
Sample ToDo List App:
Tidy Up a little
- Edit addLi() to call loadItem() function
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Simple JavaScript Todo</title>
<link rel="stylesheet" type="text/css" href="todo2.css">
</head>
<body>
<div class="wrapper">
<h1>Todo App:</h1>
<div id="inputarea">
<input type="text" id="text" placeholder="Add stuff..">
<button id="add">Add</button>
<button id="removeAll">Remove all</button>
</div>
<div class="border">
<ul id="list"></ul>
</div>
</div>
<script type="text/javascript" src="todo2.js"></script>
</body>
</html>
Bonus Features:
Save to Memory
var itemRecord = [];
Sample ToDo List App:
Lets keep a record of all added items in an easy to read and access format also...i.e. an array
- Declare an empty global array
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Simple JavaScript Todo</title>
<link rel="stylesheet" type="text/css" href="todo2.css">
</head>
<body>
<div class="wrapper">
<h1>Todo App:</h1>
<div id="inputarea">
<input type="text" id="text" placeholder="Add stuff..">
<button id="add">Add</button>
<button id="removeAll">Remove all</button>
</div>
<div class="border">
<ul id="list"></ul>
</div>
</div>
<script type="text/javascript" src="todo2.js"></script>
</body>
</html>
//...
function addLi()
{
var inputText = document.getElementById('text').value;
if(inputText.length > 0)
{
loadItem(inputText);
itemRecord.push(inputText);
}
}
Sample ToDo List App:
Lets keep a record of all added items in an easy to read and access format also...i.e. an array
- Push newly added items into array also
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Simple JavaScript Todo</title>
<link rel="stylesheet" type="text/css" href="todo2.css">
</head>
<body>
<div class="wrapper">
<h1>Todo App:</h1>
<div id="inputarea">
<input type="text" id="text" placeholder="Add stuff..">
<button id="add">Add</button>
<button id="removeAll">Remove all</button>
</div>
<div class="border">
<ul id="list"></ul>
</div>
</div>
<script type="text/javascript" src="todo2.js"></script>
</body>
</html>
//..
function saveItems()
{
var saveKey = "todoappitem";
var arrayConvertedToString = JSON.stringify(itemRecord);
localStorage.setItem(saveKey,arrayConvertedToString);
}
Sample ToDo List App:
When adding a new item, lets save it to localStorage also
Create a saveItems() function which will store the itemRecord array
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Simple JavaScript Todo</title>
<link rel="stylesheet" type="text/css" href="todo2.css">
</head>
<body>
<div class="wrapper">
<h1>Todo App:</h1>
<div id="inputarea">
<input type="text" id="text" placeholder="Add stuff..">
<button id="add">Add</button>
<button id="removeAll">Remove all</button>
</div>
<div class="border">
<ul id="list"></ul>
</div>
</div>
<script type="text/javascript" src="todo2.js"></script>
</body>
</html>
//..
function addLi()
{
var inputText = document.getElementById('text').value;
if(inputText.length > 0)
{
loadItem(inputText);
itemRecord.push(inputText);
saveItems();
}
}
Sample ToDo List App:
Call the saveItems() function from the addLi() function
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Simple JavaScript Todo</title>
<link rel="stylesheet" type="text/css" href="todo2.css">
</head>
<body>
<div class="wrapper">
<h1>Todo App:</h1>
<div id="inputarea">
<input type="text" id="text" placeholder="Add stuff..">
<button id="add">Add</button>
<button id="removeAll">Remove all</button>
</div>
<div class="border">
<ul id="list"></ul>
</div>
</div>
<script type="text/javascript" src="todo2.js"></script>
</body>
</html>
//..
function loadItems()
{
var saveKey = "todoappitem";
var arrayInStringFormat = localStorage.getItem(saveKey);
if(arrayInStringFormat !== null)
{
itemRecord = JSON.parse(arrayInStringFormat);
}
}
Sample ToDo List App:
Create a loadItems() function
get the string that was saved to localstorage in saveItems() function
convert back to array
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Simple JavaScript Todo</title>
<link rel="stylesheet" type="text/css" href="todo2.css">
</head>
<body>
<div class="wrapper">
<h1>Todo App:</h1>
<div id="inputarea">
<input type="text" id="text" placeholder="Add stuff..">
<button id="add">Add</button>
<button id="removeAll">Remove all</button>
</div>
<div class="border">
<ul id="list"></ul>
</div>
</div>
<script type="text/javascript" src="todo2.js"></script>
</body>
</html>
//..
function loadItems()
{
var saveKey = "todoappitem";
var arrayInStringFormat = localStorage.getItem(saveKey);
if(arrayInStringFormat !== null)
{
itemRecord = JSON.parse(arrayInStringFormat);
for(var i=0;i<itemRecord.length;i++)
{
loadItem(itemRecord[i]);
}
}
}
Sample ToDo List App:
For each item in the array, create a html element and show it in the list
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Simple JavaScript Todo</title>
<link rel="stylesheet" type="text/css" href="todo2.css">
</head>
<body>
<div class="wrapper">
<h1>Todo App:</h1>
<div id="inputarea">
<input type="text" id="text" placeholder="Add stuff..">
<button id="add">Add</button>
<button id="removeAll">Remove all</button>
</div>
<div class="border">
<ul id="list"></ul>
</div>
</div>
<script type="text/javascript" src="todo2.js"></script>
</body>
</html>
//..
function removeMe()
{
//"this" is equivlent to the button that was clicked
var li_item = this.parentElement;
var ul_item = li_item.parentElement;
var index = Array.prototype.indexOf.call(ul_item.children, li_item);
ul_item.removeChild(li_item);
}
Sample ToDo List App:
When we delete an item, we need to also delete it from the itemRecord array
- We need to figure out what index the item we are removing is from
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Simple JavaScript Todo</title>
<link rel="stylesheet" type="text/css" href="todo2.css">
</head>
<body>
<div class="wrapper">
<h1>Todo App:</h1>
<div id="inputarea">
<input type="text" id="text" placeholder="Add stuff..">
<button id="add">Add</button>
<button id="removeAll">Remove all</button>
</div>
<div class="border">
<ul id="list"></ul>
</div>
</div>
<script type="text/javascript" src="todo2.js"></script>
</body>
</html>
//..
function removeMe()
{
//"this" is equivlent to the button that was clicked
var li_item = this.parentElement;
var ul_item = li_item.parentElement;
var index = Array.prototype.indexOf.call(ul_item.children, li_item);
itemRecord.splice(index,1);
ul_item.removeChild(li_item);
}
Sample ToDo List App:
When we delete an item, we need to also delete it from the itemRecord array
- When we know the index, we can remove it from the array
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Simple JavaScript Todo</title>
<link rel="stylesheet" type="text/css" href="todo2.css">
</head>
<body>
<div class="wrapper">
<h1>Todo App:</h1>
<div id="inputarea">
<input type="text" id="text" placeholder="Add stuff..">
<button id="add">Add</button>
<button id="removeAll">Remove all</button>
</div>
<div class="border">
<ul id="list"></ul>
</div>
</div>
<script type="text/javascript" src="todo2.js"></script>
</body>
</html>
//..
function removeMe()
{
//"this" is equivlent to the button that was clicked
var li_item = this.parentElement;
var ul_item = li_item.parentElement;
var index = Array.prototype.indexOf.call(ul_item.children, li_item);
itemRecord.splice(index,1);
saveItems();
ul_item.removeChild(li_item);
}
Sample ToDo List App:
When we delete an item, we need to also delete it from the itemRecord array
- Finally after removing it from the array, we should re-save the array
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Simple JavaScript Todo</title>
<link rel="stylesheet" type="text/css" href="todo2.css">
</head>
<body>
<div class="wrapper">
<h1>Todo App:</h1>
<div id="inputarea">
<input type="text" id="text" placeholder="Add stuff..">
<button id="add">Add</button>
<button id="removeAll">Remove all</button>
</div>
<div class="border">
<ul id="list"></ul>
</div>
</div>
<script type="text/javascript" src="todo2.js"></script>
</body>
</html>
var checkedRecord = [];
Sample ToDo List App:
Lets also keep a record of which items have been check and which have been not
- create a checkedRecord array
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Simple JavaScript Todo</title>
<link rel="stylesheet" type="text/css" href="todo2.css">
</head>
<body>
<div class="wrapper">
<h1>Todo App:</h1>
<div id="inputarea">
<input type="text" id="text" placeholder="Add stuff..">
<button id="add">Add</button>
<button id="removeAll">Remove all</button>
</div>
<div class="border">
<ul id="list"></ul>
</div>
</div>
<script type="text/javascript" src="todo2.js"></script>
</body>
</html>
//..
function addLi()
{
var inputText = document.getElementById('text').value;
if(inputText.length > 0)
{
loadItem(inputText);
itemRecord.push(inputText);
checkedRecord.push(false);
saveItems();
}
}
Sample ToDo List App:
Lets also keep a record of which items have been check and which have been not
- When adding a new Li, push a false value into the checkedRecord array
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Simple JavaScript Todo</title>
<link rel="stylesheet" type="text/css" href="todo2.css">
</head>
<body>
<div class="wrapper">
<h1>Todo App:</h1>
<div id="inputarea">
<input type="text" id="text" placeholder="Add stuff..">
<button id="add">Add</button>
<button id="removeAll">Remove all</button>
</div>
<div class="border">
<ul id="list"></ul>
</div>
</div>
<script type="text/javascript" src="todo2.js"></script>
</body>
</html>
//..
function saveItems()
{
var saveKey = "todoappitem";
var arrayConvertedToString = JSON.stringify(itemRecord);
localStorage.setItem(saveKey,arrayConvertedToString);
var saveKeyChecked = "todoappchecked";
var checkedConvertedToString = JSON.stringify(checkedRecord);
localStorage.setItem(saveKeyChecked,checkedConvertedToString);
}
Sample ToDo List App:
Lets also keep a record of which items have been check and which have been not
- Add code to save checkedRecord in saveItems() function
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Simple JavaScript Todo</title>
<link rel="stylesheet" type="text/css" href="todo2.css">
</head>
<body>
<div class="wrapper">
<h1>Todo App:</h1>
<div id="inputarea">
<input type="text" id="text" placeholder="Add stuff..">
<button id="add">Add</button>
<button id="removeAll">Remove all</button>
</div>
<div class="border">
<ul id="list"></ul>
</div>
</div>
<script type="text/javascript" src="todo2.js"></script>
</body>
</html>
//..
function checked()
{
var li_item = this.parentElement;
li_item.classList.toggle("selected");
var ul_item = li_item.parentElement;
var index = Array.prototype.indexOf.call(ul_item.children, li_item);
checkedRecord[index] = !checkedRecord[index];
saveItems();
}
Sample ToDo List App:
When an item is checked, update the checkedRecord to set the corresponding index to true or false
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Simple JavaScript Todo</title>
<link rel="stylesheet" type="text/css" href="todo2.css">
</head>
<body>
<div class="wrapper">
<h1>Todo App:</h1>
<div id="inputarea">
<input type="text" id="text" placeholder="Add stuff..">
<button id="add">Add</button>
<button id="removeAll">Remove all</button>
</div>
<div class="border">
<ul id="list"></ul>
</div>
</div>
<script type="text/javascript" src="todo2.js"></script>
</body>
</html>
//..
function loadItems()
{
var saveKey = "todoappitem";
var arrayInStringFormat = localStorage.getItem(saveKey);
var saveKeyChecked = "todoappchecked";
var checkedInStringFormat = localStorage.getItem(saveKeyChecked);
if(arrayInStringFormat !== null && checkedInStringFormat !== null)
{
itemRecord = JSON.parse(arrayInStringFormat);
checkedRecord = JSON.parse(checkedInStringFormat);
for(var i=0;i<itemRecord.length;i++)
{
loadItem(itemRecord[i]);
}
}
}
Sample ToDo List App:
When loading the itemRecord, load the checkedRecord also
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Simple JavaScript Todo</title>
<link rel="stylesheet" type="text/css" href="todo2.css">
</head>
<body>
<div class="wrapper">
<h1>Todo App:</h1>
<div id="inputarea">
<input type="text" id="text" placeholder="Add stuff..">
<button id="add">Add</button>
<button id="removeAll">Remove all</button>
</div>
<div class="border">
<ul id="list"></ul>
</div>
</div>
<script type="text/javascript" src="todo2.js"></script>
</body>
</html>
//..
function loadItem(text,isChecked)
{
var li = document.createElement('li');
var textNode = document.createTextNode(text + ' ');
var removeButton = document.createElement('button');
removeButton.classList.add('removeMe');
removeButton.textContent = "X";
removeButton.addEventListener("click",removeMe);
var checkbox = document.createElement('input');
checkbox.type = "checkbox";
checkbox.id = "cb";
if(isChecked)//<---add this
{
checkbox.checked = true;<---add this
li.classList.toggle("selected");<---add this
}
checkbox.addEventListener("change",checked)
li.appendChild(checkbox);
li.appendChild(textNode);
li.appendChild(removeButton);
var ul = document.querySelector('#list');
ul.appendChild(li);
}
Sample ToDo List App:
Modify the loadItem() function to allow true or false checked to be set
- Add condition that evalutes if checked is true, if it is then check the checkbox and enable the "selected" class for the li
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Simple JavaScript Todo</title>
<link rel="stylesheet" type="text/css" href="todo2.css">
</head>
<body>
<div class="wrapper">
<h1>Todo App:</h1>
<div id="inputarea">
<input type="text" id="text" placeholder="Add stuff..">
<button id="add">Add</button>
<button id="removeAll">Remove all</button>
</div>
<div class="border">
<ul id="list"></ul>
</div>
</div>
<script type="text/javascript" src="todo2.js"></script>
</body>
</html>
//..
function loadItems()
{
var saveKey = "todoappitem";
var arrayInStringFormat = localStorage.getItem(saveKey);
var saveKeyChecked = "todoappchecked";
var checkedInStringFormat = localStorage.getItem(saveKeyChecked);
if(arrayInStringFormat !== null && checkedInStringFormat !== null)
{
itemRecord = JSON.parse(arrayInStringFormat);
checkedRecord = JSON.parse(checkedInStringFormat);
for(var i=0;i<itemRecord.length;i++)
{
loadItem(itemRecord[i],checkedRecord[i]);
}
}
}
Sample ToDo List App:
Back in the loaditems() function, pass the saved checkedRecord value into the loadItem() function also
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Simple JavaScript Todo</title>
<link rel="stylesheet" type="text/css" href="todo2.css">
</head>
<body>
<div class="wrapper">
<h1>Todo App:</h1>
<div id="inputarea">
<input type="text" id="text" placeholder="Add stuff..">
<button id="add">Add</button>
<button id="removeAll">Remove all</button>
</div>
<div class="border">
<ul id="list"></ul>
</div>
</div>
<script type="text/javascript" src="todo2.js"></script>
</body>
</html>
//..
function removeMe()
{
//"this" is equivlent to the button that was clicked
var li_item = this.parentElement;
var ul_item = li_item.parentElement;
var index = Array.prototype.indexOf.call(ul_item.children, li_item);
itemRecord.splice(index,1);
checkedRecord.splice(index,1);//<--add this
saveItems();
ul_item.removeChild(li_item);
}
Sample ToDo List App:
When removing an item, also remove the corresponding checkedRecord item
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Simple JavaScript Todo</title>
<link rel="stylesheet" type="text/css" href="todo2.css">
</head>
<body>
<div class="wrapper">
<h1>Todo App:</h1>
<div id="inputarea">
<input type="text" id="text" placeholder="Add stuff..">
<button id="add">Add</button>
<button id="removeAll">Remove all</button>
</div>
<div class="border">
<ul id="list"></ul>
</div>
</div>
<script type="text/javascript" src="todo2.js"></script>
</body>
</html>
//..
function reset()
{
var ul = document.querySelector('#list');
ul.innerHTML = '';
itemRecord = [];
checkedRecord = [];
saveItems();
}
Sample ToDo List App:
Finally, when "remove all" is clicked, reset both record arrays and save
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Simple JavaScript Todo</title>
<link rel="stylesheet" type="text/css" href="todo2.css">
</head>
<body>
<div class="wrapper">
<h1>Todo App:</h1>
<div id="inputarea">
<input type="text" id="text" placeholder="Add stuff..">
<button id="add">Add</button>
<button id="removeAll">Remove all</button>
</div>
<div class="border">
<ul id="list"></ul>
</div>
</div>
<script type="text/javascript" src="todo2.js"></script>
</body>
</html>
week 11 2020
By D.Kelly
week 11 2020
- 236