JavaScript Two


Arrays, Objects, Callbacks, and Ternaries
Arrays
Arrays can be thought of as lists of data.
They are created using [square brackets], with each element in the array separated by a comma.
These are organized by indexes, which start at 0.
let myArray = [1, 'Hello', true, null];
Index:
0
1
2
3
Accessing Array Values
To access values in an array, we use bracket notation and pass in the index position we want to access.
let myArray = [1, 'Hello', true, null];
myArray[1]; //returns 'Hello'
name of array
index of desired element
Array Methods
Array methods allow us to manipulate arrays and the data they contain.
Here are some basic array methods:
.push( )
.pop( )
.shift( )
.unshift( )
.slice( )
.splice( )
Push and Pop
The push array method adds, or 'pushes', an item to the end of the array.
let numArr = [1, 2, 3];
numArr.push(4);
The pop array method removes, or 'pops', an item from the end of the array.
let numArr = [1, 2, 3];
numArr.pop();
Unshift and Shift
The unshift array method adds an item to the beginning of an array
let numArr = [2, 3, 4];
numArr.unshift(1);
The shift array method removes an item from the beginning of an array.
let numArr = [1, 2, 3];
numArr.shift();
Slice
The slice array method returns a 'deep copy' of an array of primitive data types.
This means the original array is not mutated, or changed.
let numArr = [1, 2, 3, 4];
let copyArr = numArr.slice(1, 3);
will have a value of [2, 3]
Slice takes two arguments:
- the index to start the copy (is included in the copy),
- the index where the copy ends (not included in the copy).
When no ending index is specified, slice copies through the end of the array.
Splice
The splice array method can delete, as well as insert, items into any specified part of the array.
The splice method DOES mutate the original array.
let numArr = [1, 2, 3, 4];
numArr.splice(1, 1, 'Two');
Starting index
Delete Count
Item(s) to insert
Length Property
The length property allows us to see how long our array is, or how many items are inside it.
The length property is particularly useful in helping us access the last item in an array when we don't know its index.
let numArr = [1, 2, 3];
console.log(numArr.length);
returns 3
let numArr = [1, 2, 3];
console.log(numArr[numArr.length - 1]);
Accessing the last item in an array:
For Loops
For loops are a special type of statement that allows us to repeat functionality a specified number of times.
for(let i = 0; i <= 10; i++){
console.log(i)
};
initialization
condition
final-expression
Building a for loop requires three parts: an initialization, a condition, and a final expression.
"counter"
"incrementer"
For loops with Arrays
For loops are particularly useful for iterating through arrays.
let myArr = ['says', 'yoda', 'wild', 'is', 'this'];
for(let i = 0; i < myArr.length; i++){
console.log(myArr[i], i)
};

But wait, there's more!
Decrementing For Loop
We can also loop backwards using a decrementing for loop.
let myArr = ['says', 'yoda', 'wild', 'is', 'this'];
for(let i = myArr.length - 1; i >= 0; i--){
console.log(myArr[i], i)
};

Objects
Objects are groupings of related data.
Objects contain key-value pairs that are used to store data.
Objects are created using {curly brackets}.
let greatScottObj = {
great: 'Scott!',
age: 21,
greatMentor: true,
wearsHipsterGlasses: true
};
Dot and Bracket Notation
We can access values from object keys/properties with dot or bracket notation:
let user = {
name: 'Andrew',
age: 27
};
//Dot Notation
console.log(user.name)
//Bracket Notation
console.log(user['name'])
Object
Object
Property
Property
Note that the property passed into bracket notation must be written as a string.
Methods
Methods are functions that exist as a value of a key on an object
let truckObj = {
make: 'Tesla',
model: 'Cybertruck',
smash: function(){
console.log('Your window was smashed!')
}
};
Methods can be invoked by accessing them via dot notation.
truckObj.smash();
Ternary Operator
Ternaries are a syntax alternative writing if/else statements.
Syntax alternatives that make code easier to write are also called syntax sugar
7 === 7 ? true : false;
if(7 === 7) {
return true
} else {
return false
}
Callback functions
Callback functions are functions that have been passed into another function as an argument.
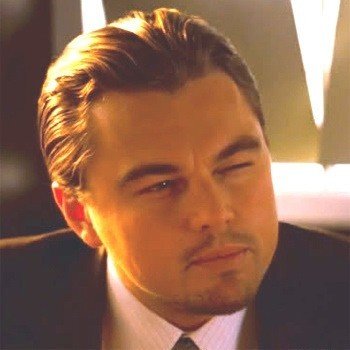
INCEPTION
Follow the data
Following the data in callbacks can be tough.
Take time to analyze what is going on with this call back function:
function callbackFunc(name){
console.log(name)
}
function sayName(cb){
cb('Gandalf')
}
sayName(callbackFunc)
Gandalf
Gandalf
callbackFunc
callbackFunc
JavaScript 2
By Devmountain
JavaScript 2
Arrays, Objects, Callbacks, and Ternaries
- 1,253