Ruby on rails
coding w/ CORE + ADI
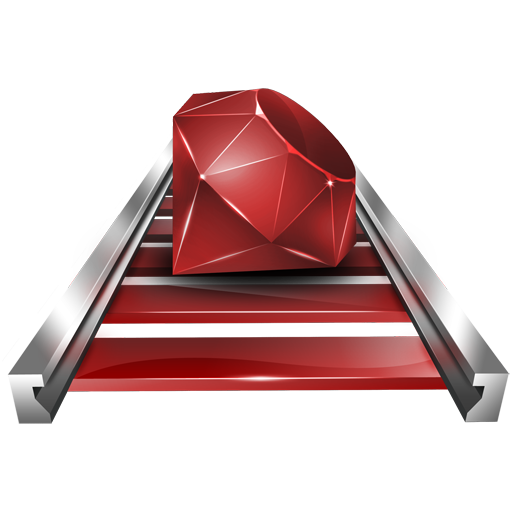
getting started
- Download the Rails Installer package
- Download Sublime Text 2 or RadRails RoR editor
- Set up a heroku account to deploy your web app

Why learn Ruby on rails?
- Incredibly popular in startup community - Github, Twitter, Hulu, Codecademy were all built with it
- Huge number of gems (solutions to common web app problems) for you to use in your web app
- Makes a lot of conventional decisions for you so that you don't have to. You can concentrate on building your app
What is ruby?

Ruby is a dynamic, object-oriented programming language made by Yukihiro "Matz" Matsumoto.
"Ruby is designed to make programmers happy" - Matz
what is rails?
Rails is an open-source web framework built for Ruby by David Heinemeier Hansson, aka DHH.
Used to make web applications that follow the MVC (Model View Controller) convention.

ruby basics
- "puts" is how you print stuff
> puts "Hello
Ruby
variables
students = ["Don", "Kevin", "Batman"]
students
nil = False (and only nil is false)
"" = true
0 = true
[] = true
strings
name = "Don Yu"
Building Strings
puts "Hello #{name}"
hashes
Series of key-value pairs
dorm = { name: "Broadway", room: "1004"}
puts dorm[:name]
puts dorm.name
puts dorm[:room]
puts dorm.room
functions
def greet
puts "Greetings!"
end
classes
class Greeter
def initialize(name)
@name = name
end
def greet()
puts "Hello #{@name}"
end
end
rails
Create a new barebones rails project
$ rails new myappname
Run your rails app
$ rails server
View the example app

Rails app structure
1. app/ - folder with all of your ruby code for models, views, controllers
2. app/assets - folders where you'll find your HTML, CSS, JavaScript
3. README.rdoc - terse description of Rails
4. Gemfile - all the ruby gems (libraries of code you can just import into your app) that your Rails app uses
Model, View, controller

Lets create models
We will use the scaffolding feature of rails to generate a template model for Users
$ rails generate scaffold User name:string email:string
Let's also create a model for Dormrooms
$ rails generate scaffold Dormrooms dorm:string room:string
Now tell our database that we created these new models
$ bundle exec rake db:migrate
what happened?
-
"rails generate" helps you create template files
- "rails generate scaffold" creates a model, controller, and view for you!
- "db:migrate" creates a table for you in your database
rails console
A place to debug your app
$ rails console
> User.create(name: "Jon", email: "jon@jon.com")
> jon = User.where(name: "Jon")[0]
> jon.name = "Kevin"
> jon.save
rails views
You'll notice that rails created a bunch of views for you. Let's concentrate on just
- views/layouts/application.html.erb - this is the html that all of your views will have by default
- views/dormrooms/index.html.erb - the main page for the "/dormrooms" path
rails configurations
You must edit config/routes.rb to specify what pages open when i.e. to make our Dormrooms page the home page, we can add
root :to => 'dormrooms#index'
deployment w/ heroku
$ git init
$ git add ./
$ git commit -m "sending all my web app to heroku"
$ heroku create
$ git push heroku master
$ heroku open
Learn more
Ruby
Examples - RailsApps
Screencasts - Railscast
Books - Ruby on Rails
Heroku - Deploy Rails App
thanks for coming!
Ruby on rails
By Don Yu
Ruby on rails
- 1,236